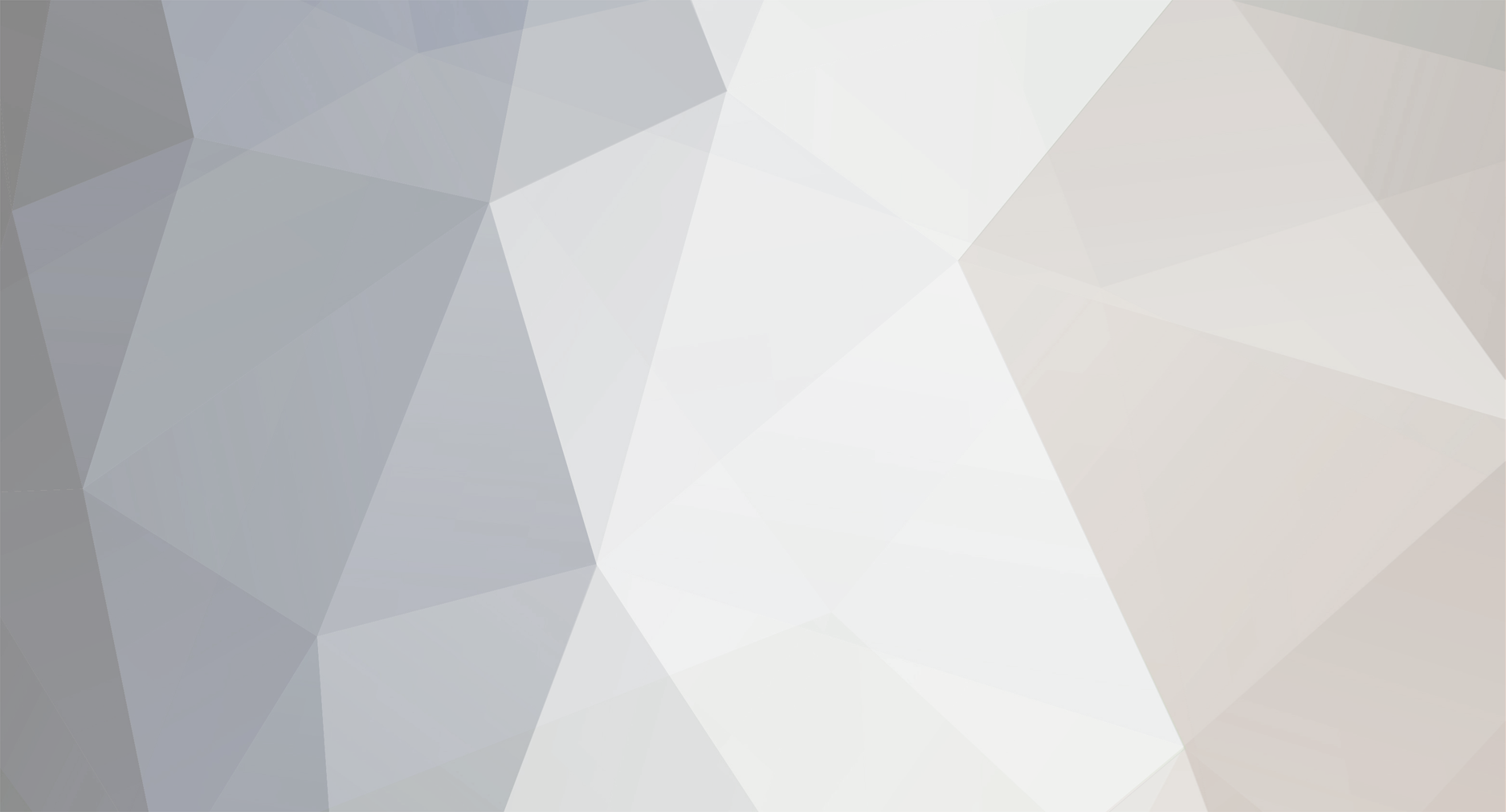
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
This is the PHP forum, thus I provided a PHP solution to do the server-side validation of the date. If you want the client-side solution for dynamically changing the date options, then post that request in the JavaScript forum.
-
checkdate()
-
Need a PHP List Box that populates itself from MYSQL Server
Psycho replied to Jalsemgeest's topic in PHP Coding Help
This forum is for people to get help with code they have written. What you are asking for requires several different implementations working together. It is unrealistic to expect a complete solution to be explained in a forum post. Do one thing at a time and get it working then move to the next. If you have problems with something then post a question on exactly what you are trying to do and the problems you are experiencing. I would the following: Start by creating the first select list. This would require a simple SELECT query and processing the results into OPTION tags. Then, implement JQuery to do the client side logic. Just start with implementing an alert() when an option is selected. Then have it perform an AJAX call (passing the select list value) to a dummy PHP page that only echo's back the passed value and have the alert() display the value passed back from AJAX call. Then, build the PHP page to take the passed value to query the values for the second select list and pass those values back. Lastly, modify the PHP output to build the results into the secondary multi-select list and pass that back. The JS can then populate that secondary multi-select listbox onto the page. -
OK, a few things, so let's tackle one at a time: But, you would not have two entries for the same part number at the same warehouse, right? Then you can make the "combination" of the part number and the warehouse as a unique constraint! If you set that up in the database, then you can use the "ON DUPLICATE KEY UPDATE" so new records will be added and existing records would be updated. It would take too much time to try and explain all that here, so you should do some research, but it is definitely the right way to do this. However, you should NOT have another table to store the total stock of the products. You already have that data in this table by combining the relevant records. You should never store the same data in different tables as you will ultimately run into problems when concurrency/race conditions occur. I may have had a bug in my code, but you do not need separate counters. My code used just one counter and then applied the offset within the loop. Maintaining three separate counters is too much overhead and adds complexity where it does not need to be. And, on second thought there is an even better solution: array_chunk()! <?php ///Create array of insert values $includeValues = array(); //Split array into multidimensional array by record $records = array_chunk($print, 3); foreach($records as $record) { //Verify the record has three values if(count($record)==3) { //Process the record into an insert value $partNo = mysql_real_escape_string(trim($record[0])); $qty = intval($record[1]); $warehouse = intval($record[2]); $includeValues[] = "('{$partNo}', '{$qty}', '{$warehouse}')"; } } ?> When you have a string defined in a double quoted string you can include variables in that string and they will be parsed into their values. But, in some cases the parsing engine can get confused as to where the variable starts and ends - especially when using arrays in the string. So, you can enclose the variable within curly braces to help the parsing engine. I do this much consistently for ALL my variables within string to be consistent and it also makes it easier to "see" the variables within my code. See the manual for string parsing here: http://www.php.net/manual/en/language.types.string.php#language.types.string.parsing Scroll down after example #8 to see the explanation about complex syntax.
-
Run the string: <b>this is bold</b> through the function. If you get: <b>this is bold</b> then it works. If you get: this is bold then it doesn't work. @scootstah: But, you'd also want to throw it a multi-dimensional array with values that would need to be escaped as well. @Debbie: You know what the function is supposed to do, so you should know how to test it to see if it works. Writing some code and posting it here asking if it will work or not can be perceived as arrogant. The function is supposed to encode strings values or string within arrays (including multidimensional arrays). So, pass it both types of values and verify the results. $testString = "<b>This is a bold string value</b>"; $testArray = array(array("<b>This is a bold mutidimensional array value</b>")); echo "<br>String before encoding: " . $testString; echo "<br>String after encoding: " . entities($testString); echo "<br>Array before encoding: <pre>" . print_r($testArray, true) . "</pre>"; echo "<br>Array after encoding: <pre>" . print_r(entities($testArray), true) . "</pre>"; Expected Output (using my function):
-
Can you try explaining that again? What do you mean by setting the value to "1-7". The code you have sets the value to 1, then on the 2nd iteration sets it to 2, then 3, then 4, etc. So, when the loop completes the record will only have the value of 7. If you want a value of "1-7" then set that value. Instead of running the queries you should echo them to the page to see what is actually happening. That is why I advise to always create queries as a string variable instead of building them directly i the mysql_query() function. for($i = 1; $i <= 7; $i++) { $query = "UPDATE ".PREFIX."cup_matches SET wk='$i' WHERE type='gs' && $type_opp='0' && matchno='$ID' && clan1='{$sPT['clan1']}'"; echo $query . "<br>\n"; //safe_query($query); }
-
You should never run queries in loops. If you have multiple records to insert, then create ONE insert query. Also, you need to validate/escape the values as appropriate for their type (I guessed int eh following code) //Set Counters $idx=0; ///Create array of insert values $includeValues = array(); while ($idx < $count) { $partNo = mysql_real_escape_string(trim($print[$idx])); $qty = intval($print[$idx]); $warehouse = mysql_real_escape_string(trim($print[$idx])); $includeValues[] = "('{$partNo}', '{$qty}', '{$warehouse}')"; $idx += 3; } ///Write the Data to our Database $query = "INSERT INTO inventory (part_number, qyt_on_hand, warehouse) VALUES " . implode(', ', $includeValues); mysql_query($query) or die(mysql_error()); However, why are you truncating the table instead of updating the existing records (and inserting any new ones)? You could use an INSERT with an "ON DUPLICATE KEY UPDATE" clause. That way existing records are updated and new ones are inserted. Are any of the values unique? For example, do you have multiple entries for the same part number if different warehouses? There are better approaches to this than truncating the table, but without knowing more about the requirements and the data it's hard to provide the appropriate solution.
-
I would still use array_map(), but in such a way that it will work for multidimensional arrays. For the encoding you can just define it inside the function. function entities($input) { if (is_array($input)) { return array_map('entities', $input); } return htmlentities($input, ENT_QUOTES, 'UTF-8'); } EDIT: Fixed a typo in code
-
1. You first check to see if username or password are empty. Then you do a second check to see if they are not equal to "" - ??? 2. Your select query is selecting ALL the records where it is apparent you are looking for a matching record. So, the following lines doing a check of username are only checking the first record. 3. You have a string that is sitting in the code doing nothing (i.e. there is no echo and no assignment) "<script>alert('O nome do utilizador ja existe.')</script>"; 4. Don't use JS alerts for error messaging, it is sloppy. Here is a quick rewrite <?php include 'db_connect.php'; $result_msg = ''; $username = isset($_POST['user']) ? trim($_POST['user']) : ''; $password = isset($_POST['password']) ? $_POST['password'] : ''; if($_SERVER['REQUEST_METHOD']=='POST') { if (empty($username) OR empty($password)) { $result_msg = "<span style='color:red'>Todos os campos são obrigatórios.</span>"; } else { $usernameSQL = mysql_real_escape_string($username); $passwordSQL = sha1($password); $query = "SELECT username FROM utilizadores WHERE username = '$usernameSQL'"; $result = mysql_query($query); if(mysql_num_rows($result)) { $result_msg = "<span style='color:red'>O nome do utilizador ja existe.</span>"; } else { $query = "INSERT INTO utilizadores (username, password) VALUES ('{$usernameSQL}','{$passwordSQL}')"; $result= mysql_query($query); if(!$result) { $result_msg = "<span style='color:red'>Erro a adicionar utilizador.')</span>"; } else { $result_msg = "<span>Utilizador adicionado com sucesso.</span>"; $result_msg .= "<br><a href='admin.php'>Admin Page</a>"; } } } } ?> <html> <head> <title>Adminstração</title> </head> <body style="color=#FFFFFF" bgcolor="#666666"> <?php echo $result_msg; ?></span> <table width="100%" height="100%" border="0" align="center" cellpadding="0" cellspacing="1"> <tr> <form method="post" action=""> <td align="center" valign="middle"> <table width="300px" cellpadding="0" cellspacing="3" bgcolor="#FFFFFF" style=" border: 1px solid #000000;"> <tr> <td style=" border-style:none;"> <br> </td> </tr> <tr> <td align="right" style="font-size: 13;font-family: Arial, Helvetica, sans-serif;font-weight: bold;border-style:none;"> Utlizador: </td> <td style=" border-style:none;"> <input name="user" type="text" value="<?php echo $username; ?>"> </td> </tr> <tr> <td align="right" style="font-size: 13;font-family: Arial, Helvetica, sans-serif;font-weight: bold;border-style:none;"> Password: </td> <td style=" border-style:none;"> <input name="password" type="password"> </td> </tr> <tr> <td colspan="2" align="center" style=" border-style:none;"> <button type="submit">Registar</button> </td> </tr> <tr> <td style=" border-style:none;"> <br> </td> </tr> </table> <span class="style1" style="font-size: 13;font-family: Arial, Helvetica, sans-serif;font-weight: bold;color: red;"> </span> </td> </form> </tr> </table> </body> </html>
-
Depending on the system you run on, the \w parameter may work. Not sure why you want to use such an overcomplicated line of code. This seems to work for me based on your requirements function validString($string) { return !preg_match("#[^\w\d'& ]#", $string); }
-
This topic has been moved to Application Design. http://www.phpfreaks.com/forums/index.php?topic=360147.0
-
"doesn't work" is not a helpful explanation. What DOES it do and what is it supposed to be doing differently? If you've tried some debugging, what have you tried and what were the results?
-
Then start at the beginning. Have you even verified that the page you *think* is running is the one that is actually running? At teh very start of the page do something like this echo "Start debugging<br>"; Then at the very first control you could do something like this if ($_POST['submitbtn']){ echo "POST[submitbtn] = TRUE<br>"; Also, add a debug statement for the false condition. By the way, you should rethink how you set up your IF/THEN logic. When you do something such as this if(NOT ERROR CONDITION 1) { if(NOT ERROR CONDITION 2) { //perform success condition } else { //perform error condition 2 } } else { //perform error condition 1 } It makes it very difficult to manage the conditions with their errors. It is much better to follow a pattern such as this if(ERROR CONDITION 1) { //perform error condition 1 } else if(ERROR CONDITION 2) { //perform error condition 2 } else { //perform success scenario }
-
Of course it is still failing! That code will not fix the error, but it will show you what the error IS. We can't help you because we don't know what the problem is. If you cannot implement simple debugging steps and ascertain the results for us to review then there isn't much we can do to help you.
-
Your queries are failing. You need to check that the queries succeed - if not, check what the error is. On all your queries you should define the query as a string variable and then run that in mysql_query. That way, if the query fails, you can eche the que5ry and the error to the page. $query = "SELECT * FROM user WHERE username='$username'"; $result = mysql_query($query) or die("Query: $query<br>Error: " . mysql_error()); Do that on all the queries you run.
-
And what error is displayed when you add the error handling that smoseley suggested?
-
if(empty($val)) { $val = time(); } Or use date() with whatever format you fancy
-
That comment is diametrically opposed. That solution is probably "sufficient", but is not fool-proof. Whether it is secure or not is a different thing entirely. If someone replies to a message you would rather they did not is not a security issue. But, if you want to prevent someone from replying to a message they should not then you *should* prevent it on the receiving page. Simply check if the message that is being replied to meets the requisite conditions before accepting it. Preventing users from doing something by hiding controls is typically a poor implementation. If you are not checking if the message the person is replying to is not a deleted or sent message then what else are you not checking? Are you checking if the message is even one that the user is involved in? If so, then also checking to see that it is not deleted or a sent message should only require a slight modification in the query used to perform that check.
-
Let's verify what $row['ServicesPricePerMinute'] really is. Try doing this var_dump($row['ServicesPricePerMinute'])
-
http://www.php.net/manual/en/intro.mssql.php
-
session variable is not retained when switching pages
Psycho replied to Juarez's topic in PHP Coding Help
I would guess that error is because $_POST['name'] is not set. That results in $_SESSION['name'] being overwritten with a NULL value -
On line 25 you are echo'ing content to the output page. You can send NO output to the page before any header commands.
-
What is the error message you are getting?
-
I think you should make a change in our structure. The animal_breed table should be a list of possible breeds along with unique ID. Then you would have a table (e.g. animals) that contains one record for each animal. That table would include a foreign key to the animal_breed table. But, using your current structure, this should work SELECT animal_type.name AS name, COUNT(animal_type.type_id) AS count FROM animal_type JOIN animal_map ON animal_map.type_id = animal_type.type_id JOIN animal_breed ON animal_breed.breeds = animal_map.breeds GROUP BY animal_type.type_id
-
Sorry, but I have to advise against using Drummin's solution. You should never use values from user submitted data directly in an include statement. You need to validate it first. $includeValue = isset($_POST['dropdowns']) ? $_POST['dropdowns'] : false; switch($includeValue) { case 'selection1.php': case 'selection2.php': case 'selection3.php': include($includeValue); break; default: include('selection1.php'); }