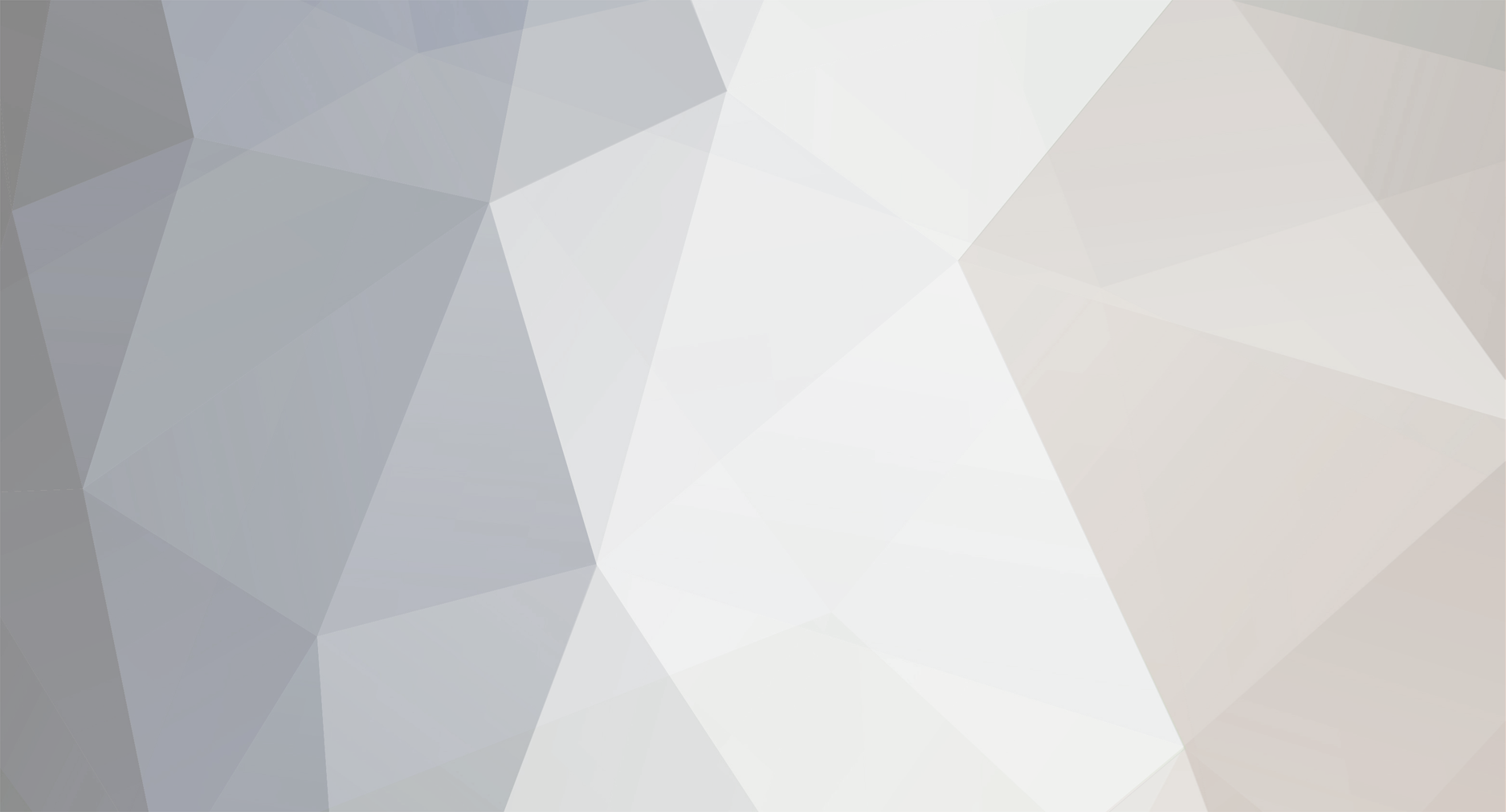
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
EDIT: Deleted, I accidentally did a quote of my previous post instead of an edit.
-
OK, here is a proof of concept. Since you already have drill positions that are assigned to stations positions I'm not sure how you want to implement this. The code below assumes you have a list of stations and positions and want to iterate over ALL the drill positions to assign them to stations then save those assignments. But, if your drillpositions are dynamic you would have to reprocess ALL of them whenever there is a change. Drill positions will be assigned to Stations according to the following: 1. If a drill position is closer to one station than the others it will be assigned to that station. 2. If a drill position is the same distance to two stations, it will be assigned to the station with the higher priority (if different) 3. If a drill position is the same distance to two stations with the same priority, it will be assigned to the station with the least amount of currently assigned drills. Now, this isn't perfect since the order in which the drills are processed will affect the results. If a drill is assigned due to the third condition (number of currently assigned drills) that assignment could be different if they were processed in a different order. I even included a record to see this.The drill position with the comment next to it will be assigned to station '0' based upon where it currently exists in the data order. but, if you move that record to the end of the array it will then get assigned to station 1. Anyway, the following code works pretty much as you requested using two arrays for the station and drill positions. I even included code at the end to display the results in a grid. Stations have a specific background color and the assigned drills are given text color to match the assigned station. Note that the display code was just thrown together. It will not display duplicate items in the same cell. Also, although I hard coded the color coding for a maximum of three positions, the logic for assigning drills to stations will work with any number of records for either. <?php //Test data $stationsAry = array( array( 'x'=>3, 'y'=>4, 'priority' => 1), array( 'x'=>9, 'y'=>8, 'priority' => 1), array( 'x'=>5, 'y'=>10, 'priority' => 2) ); $drillsAry = array( array( 'x'=>3, 'y'=>, array( 'x'=>10, 'y'=>6), array( 'x'=>5, 'y'=>7), array( 'x'=>3, 'y'=>9), array( 'x'=>6, 'y'=>6), //Can Change Assignment array( 'x'=>7, 'y'=>2), array( 'x'=>9, 'y'=>3), array( 'x'=>4, 'y'=>, array( 'x'=>1, 'y'=>10), array( 'x'=>2, 'y'=>3), array( 'x'=>5, 'y'=>5), array( 'x'=>3, 'y'=>2) ); //Function to process all drill positions and //assign to a station function get_assigned_drills($drills, $stations) { //Create array to hold results $results = array(); //Process drill locations foreach($drills as $drillID => $drill) { //Tracking vars $selected_station = false; $selected_distance = false; //Compare drill to stations foreach($stations as $stationID => $station) { //Get station positions $distance = sqrt(pow(abs($station['x']-$drill['x']),2) + pow(abs($station['y']-$drill['y']), 2)); if($selected_distance===false || $distance<$selected_distance) { //First station or distance is less than prev selected station $selected_station = $stationID; $selected_distance = $distance; } elseif($distance==$selected_distance) { //Disatnce is equal to previous station distance //First check priority if($station['priority']!=$stations[$selected_station]['priority']) { //Stations have different priorities if($station['priority']>$stations[$selected_station]['priority']) { //This station has a higher priority than the prev selected station $selected_station = $stationID; } } else { //Stations have same priority, check current assigned count if(count($results[$stationID])<count($results[$selected_station])) { //This station has less assigned drills $selected_station = $stationID; } } } } $results[$selected_station][] = array('x'=>$drill['x'], 'y'=>$drill['y'], 'distance'=>$selected_distance); } return $results; } //Get an array of all drill positions assigned to appropriate station $drillAssignments = get_assigned_drills($drillsAry, $stationsAry); ######################################################### # END PROCESSING LOGIC # BEGIN SAMPLE CODE TO OUTPUT RESULTS ######################################################### $colors = array('#FF0000', '#008000', '#0000FF'); $tableData = array(); for($row=1; $row<=10; $row++) { for($col=1; $col<=10; $col++) { $tableData[$row][$col] = ' '; } } //Insert drill positions into table data foreach($drillAssignments as $stationID => $drillPositions) { $color = $colors[$stationID]; foreach($drillPositions as $drill) { $tableData[$drill['y']][$drill['x']] = "<span style=\"color:{$color}\">$stationID</span>"; } } //Insert station positions into table data foreach($stationsAry as $stationID => $station) { $color = $colors[$stationID]; $tableData[$station['y']][$station['x']] = "<b style=\"background-color:{$color}\">$stationID</b>"; } $tableHTML = ''; for($row=count($tableData); $row>0; $row--) { $tableHTML .= "<tr>\n"; $tableHTML .= "<th>{$row}</th>\n"; foreach($tableData[$row] as $cell) { $tableHTML .= "<td>{$cell}</td>\n"; } $tableHTML .= "</tr>\n"; } $tableHTML .= "</tr>\n"; $tableHTML .= "<th></th>\n"; for($col=1; $col<=10; $col++) { $tableHTML .= "<th style=\"width:20px\">{$col}</th>\n"; } $tableHTML .= "</tr>\n"; ?> <table border="1"> <?php echo $tableHTML; ?> </table>
-
Can you please provide some of the peretinent code? Also, what is the parameters of the grid (i.e the min/max x and y values)? Lastly, you say some of the drills are already assigned - are you getting this info from a database and what is the format of the data?
-
Pulling sunset time from txt file and displaying on site daily
Psycho replied to master4g's topic in PHP Coding Help
Did you LOOK at the functions linked? They take a timestamp, so time() is all you need to supply -
Pulling sunset time from txt file and displaying on site daily
Psycho replied to master4g's topic in PHP Coding Help
@master4g: Look at Pikachu2000's response. There are built-in functions in PHP (I didn't realize this) that will return the sunrise, sunset, etc. information AUTOMATICALLY. You do not need to do anything - no data entry. PHP is all knowing! Well, not really, but it is simply a mathematical calculation for the sunrise/sunset for any given latitude/longitude. Look at the functions he linked to -
As xyph has stated the table row (i.e. <tr>) does not official support a background color. It will work in some browsers though, but it is not a good idea to use it. Instead, here is the approach you should take: Give the rows (tr tags) alternating classes. Then define properties of the TDs that are children of those classes. Example .odd_row td { background-color: #cecece; } .even_row td { background-color: #eeeeee; } Now, you will be applying a background color to the TD tags using a class identifier for the TR tag! No need to apply styles to each and every TD tag. <tr class="odd_row"> <td>Cell 1</td> <td>Cell 2</td> <td>Cell 3</td> <td>Cell 4</td> </tr> Fully working example script <?php $max_rows = 10; $tableOutput = ''; $trClass = false; for($row=0; $row<$max_rows; $row++) { $trClass = ($trClass=='odd_row') ? 'even_row' : 'odd_row'; $tableOutput .= "<tr class=\"{$trClass}\">\n"; $tableOutput .= "<td>One</td><td>Two</td><td>Three</td><td>Four</td>\n"; $tableOutput .= "</tr>\n"; } ?> <html> <head> <Style> .odd_row td { background-color: #cecece; } .even_row td { background-color: #eeeeee; } </style> </head> <body> <table> <tr> <th>Column 1</th> <th>Column 2</th> <th>Column 3</th> <th>Column 4</th> </tr> <?php echo $tableOutput; ?> </table> </body> </html>
-
Pulling sunset time from txt file and displaying on site daily
Psycho replied to master4g's topic in PHP Coding Help
A better approach would be to use a database. Do you have any experience with a database? Also, how are you getting the data? That will help to determine the best approach for importing/using the data in your script. -
How can check variable value content integer or not by is_int() ?
Psycho replied to man12_patil3's topic in PHP Coding Help
I don't know if this is more efficient or not, but it is easier to "see" the logic in my opinion: if(is_numeric($val) && intval($val)==$val) EDIT: Just found that the above returns true for something such as '12.0'. But, you could just wrap the above in a function and convert to an int before returning the value. This really shouldn't be that hard. If there is a strtotime() function there should be a function to validate if a string represents an integer or not. EDIT #2: OK, this seems to work for any values. if((string) (int) $val === (string) $val) -
Well, you have to decide: should you copy the file first and then update the database or update the database first then move the file. I'd probably go with the former. Just add the code necessary as an else condition to the if() statement that uploads the file. As for adding a unique random number you would either have to check the available file names or check the file names stored in the database. Again, I would go with the former. I'd do a single check to see if there is an exact match of the file name. If no, move the file with the original name. If yes, they I would use glob() to find all the "like" file names. You would need to split the file name into the path/name part and the extension. Then find all the files matching the original with additional "text" something like $matches = glob($path.$name.'*'.$ext); Then just loop through till you find an available number to use.
-
How can check variable value content integer or not by is_int() ?
Psycho replied to man12_patil3's topic in PHP Coding Help
The problem with is_numeric() is that it will return true for floats. Most times when I need something to be an int I typically need it to be an int with a value of 1 or greater. Such as when it need to be the primary id for a record. In that case I typically use something such as $value = (int) $value; //Force value to be an integer if($value<1) { //Validation failed } But, it all depends on what are valid values for the variable you are using as to how you should validate it. But, as stated previously, if the field is an int type in the database - it will be an int. Unless of course you allow a null value in that field. -
This doesn't have anything to do with the problem (Freedom already addressed that). But, this if ($row == 0) {echo "";} else {echo "Small - $row['sizes']";} Should just be if ($row != 0) {echo "Small - $row['sizes']";}
-
How can check variable value content integer or not by is_int() ?
Psycho replied to man12_patil3's topic in PHP Coding Help
EDIT: Then why do you need to validate it if the database is going to enforce it as an int? Original Text: What type of field is "cad_count" in the database? Is it a varchar or float type? If the value is always supposed to be an int then you should be using that type of field. However, if the field can be other than an int, then you could validate an int using ctype_digit() to verify that it is a positive integer. If you need to allow negative integers I can give you a different solution. -
<?php if(isset($_FILES['uploaded']['name'])) { $target = "upload/"; $fileName = basename($_FILES['uploaded']['name']); $target = $target . $fileName; $errors = array(); //This is our size condition if ($uploaded_size > 350000) { $errors[] = "Your file is too large."; } //This is our limit file type condition if ($uploaded_type=="text/php") { $errors[] = "No PHP files"; } //Here we check that no validation errors have occured if (count($errors)==0) { //Try to upload it if(!move_uploaded_file($_FILES['uploaded']['tmp_name'], $target)) { $errors[] = "Sorry, there was a problem uploading your file."; } } //If no errors show confirmation message if(count($errors)==0) { echo "The file {$fileName} has been uploaded"; } else { //show error message echo "Sorry your file was not uploaded due to the following errors:<br>\n"; echo "<ul>\n"; foreach($errors as $error) { echo "<li>{$error}</li>\n"; } echo "</ul>\n"; } } else { //Show the form echo "<form enctype='multipart/form-data' action='' method='POST'>\n"; echo "Please choose a file: <input name='uploaded' type='file' /><br />\n"; echo "<input type='submit' value='Upload' />\n"; echo "</form>\n"; } ?>
-
Here is some sample code of how you can accomplish this with just storing records that have something other than an unhit, empty cell. Note: I assumed some changes as follows: 1) Database records will be stored with a row and column value instead of a value from 1 to 1088. 2) Changed the 'user' column in the database to 'hitby' since that is what it means based on the context you are using it. 3) Changed the link for the empty cells to pass two values (row and column positions) 4) A CSS style property should be added to the style sheet to give the images a 0 border instead of hard coding that for each image (not shown in the code below) NOTE: I don't know where the value for $prevamount comes from, just left it in as you had it <?php //Query all the records ordered by row/column //Note only contains cells that are hit and/or contain a ship position $query = "SELECT row, col, clicked, hitby FROM table ORDER BY row, col"; $result = mysql_query($query); //Set column and row counts $max_rows = 32; $max_cols = 34; //Get first record from result set $cell = mysql_fetch_assoc($result); //Generate the table $tableHTML = "<table width='20' border='0' cellspacing='0' cellpadding='0'>\n"; for($row=0; $row<$max_rows; $row++) { $tableHTML .= "<tr>\n"; for($col=0; $col<$max_cols; $col++) { //Check if current DB record is for this cell if($row==$cell['row'] && $col==$cell['col']) { //Set values from DB record and get next DB record $clicked = $cell['clicked']; $hitby = $cell['hitby']; $cell = mysql_fetch_assoc($result); //If no more cells avail from DB set row/col to false if(!$cell) { $cell['row']=fale; $cell['col']=fale; } } else { //Doesn't match current DB record set default status $clicked = 0; } //Set image based on cell status switch($clicked) { case 0; $tableHTML .= "<td><a href='index.php?r=$row&c=$col'><img src='g.gif'></a></td>\n"; break; case 1; $tableHTML .= "<td title='$col, $row'><img src='b.gif'></a></td>\n"; break; case 2; $tableHTML .= "<td title='$col, $row - Hit by $hitby.'><img src='h.gif'></a></td>\n"; break; case 3; $tableHTML .= "<td title='$col, $row - $prevamount btc won by $hitby.'><img src='chest.gif'></a></td>\n"; break; } $tableHTML .= "<td></td>\n"; } $tableHTML .= "</tr>\n"; } $tableHTML .= "</table>\n"; ?>
-
No, it does not need 1088 rows. You only need records for the cells/positions that have some status other than empty. I think there are probably four possible statuses: 1) there is no boat at that position and no attack has been made (do not store records for these cells), 2) there is no boat and an attack has been made (i.e. a miss), 3 There is a boat at that position and no attack has been made, and 4) there is a boat at that position and an attack has been made (i.e. a hit). Then at the start of a new round you would delete all the records and only create new records for the positions that have boats. Then on each attack you would create a new record (hit or miss) for the position attacked.
-
Why do you say that? In my opinion, a GIF would be a good choice since it will require much less bandwidth. A GIF is a good format for images that are not "full-color" i.e. something like a scenic image or an image of a person that requires thousands/millions of different colors. A GIF allows for up to 256 unique colors and is good for things such as logos or "artwork" which is what I would categorize these images as.
-
That would mean the same amount of entrys? Yes, but, it makes more logical sense to me to store the records by row/column. That way you can easily associate a grid position with a particular record. As, PFMaBiSmAd stated you should only store the grid positions that are not empty. That is also why storign the records by row/column makes sense. You can create a simple PHP loop to create the grid. As it does so, it checks the db results (from a single query) to see if there is something other than a blank square in each position.
-
What he ^^ said. Also, I'm curious why you decided to define the "cells" from 1 to 1088. I would have defined the database records with two fields - one for the x axis and one for the y axis.
-
I see lots of problems, but not sure which one, if any are the root of your problem: NEVER run queries in loops. Learn how to do JOINS. Why is there a while loop here: $getFromData = mysql_query("SELECT username FROM members WHERE id='$from_id'") or die (mysql_error()); while($row2 = mysql_fetch_array($getFromData)){ $wallUsername = $row2['username']; } Anyway, I tried following your code and didn't want to take the time to try and really understand it. But, I did look at the queries and think I have a solution for those. I think this is the only query you need to run. It should have ALL the data for the wall post, the username of the wall post, the post comments, and the username for the comments. Give it a try and see if the results are correct for what you need $query = "SELECT wall.id, wall.message, wall.datetime as post_date, post_member.username as post_username, wallComments.comment, wallComments.datetime as comment_date, comment_member.username as comment_username FROM wall JOIN members AS post_member ON wall.from_id = post_member.id JOIN wallComments ON wallComments.wallPostId = wall.id JOIN members AS comment_member ON wallComments.from_id = comment_member.id WHERE to_id='$id' ORDER BY wall.datetime DESC, wallComments.datetime DESC"
-
I will sometimes build sites with different "levels" of errors. Some errors are minor and you can display the page as normal with the error displayed. However, there are also critical errors that prevent you from even gathering enough data to put together a "pretty" error page. For the latter you could have a flat HTML error page when that occurs. I have noticed that this site display an all white page with a short message when DB connectivity is down. Since it cannot access the database there isn't much use in trying to show the normal page with the pretty colors and such. Even something such as the menu at the top would be dynamic based on the user's permissions (which are stored in the DB). So, make a decision on how you want to handle. If you can't pull a template do you even continue trying to display a "pretty" page, do you use a default template, or what?
-
Well, I would counter that a good editor will automatically display all the variables used in a script.
-
Without having access to your server to do benchmarking, knowing how many concurrent users there will be (for your site and the other sites on that server - if it is a shared server) there is no way to tell. Having said that, you should probably make the above script as efficient as possible. Some things you could do: 1. The top section to get the user name seems odd. You first make sure that the session var and cookie var are both set, if not you redirect to blank (should be an exit() statement right after that), but then you set $User to the cookie if it is not blank, else the session var. Why are you mandating that the cookie must be set if it can be empty? 2. You are running two queries to get the user id of the user and the friend. a) Why are you passing the user names to the page?! You should be using the user ids to begin with!!! Database transactions are expensive in terms of performance and you are running those two ever time when they shouldn't be needed. 3. I'm not understanding the logic between the sections to receive conversations upon open vs. new messages. You should just need one query to get all the relevant massages after the last time the user got the messages. Plus, you should only have the query return the fields you need - DON'T USE '*' if you don't need all the fields. This goes for any query you run. 4. The section at the end to update the status is completely unnecessary and a huge waste of resources. You should NEVER EVER run queries in loops. First of all, even if you needed to update the viewed status of those records (which you don't) you don't need to loop through each record to do it. You could just run a single UPDATE query using the same WHERE conditions as the previous select query. But, you shouldn't be using a "viewed" status anyway. As I already stated you just need the user to pass a timestamp of the last time they retrieved the messages and pull all messages from that time period forward. As it stands now there are six unique queries in that script and there is no telling how many would actually be run on each page load since two of them are in loops. However, you only need one query for for retrieving new messages and one to add a message (then an optional uery to get the names if not already stored in session). That's it. By the way, I don't see anything in there to add a new message. Using either session vars or variable passes on the query string (i.e. $_GET vars) you should have available to the script the user id, the friend id and the timestamp when the user last access the messages between him and the friend. The timestamp can be null if they are accessing the messages the first time, but that means if they then send a new message a week later they will be getting the entire history. So, you can either store a timestamp for the user-to-firend last access time to use for future conversations (which would require another DB transaction) or come up with an archiving rule (i.e. only pull messages as far back as 24 hours). So, when the messages are retrieved, you would define a new timestamp and pass it back to the javascript. The javascript would then pass that timestamp back on the next request. Anyway, here is some mock code of how it could work more efficiently. The first time the script is run for a user in a session, there is one query to get the names of the users to store in the session. Then there is a second query that is run ONLY if there is a message to add. Then there is a third query that is run to get NEW messages only. So, 99% of the time only one query will be run Again, this is just something I threw together and I have not tested any of it. There are likely some syntax and possibly even some logic errors. But, the overall concept is sound <?php //Get user ID from session and friend ID from URL $user_id = (isset($_SESSION['user_id'])) ? (int) $_SESSION['user_id'] : 0; $friend_id = (isset($_GET['friend_id'])) ? (int) $_SESSION['friend_id'] : 0; //If user or friend IDs are invalid stop if($user_id==0 || $friend_id==0) { echo "Invalid parameters"; exit(); } //Add new message if passed $new_message = (isset($_GET['new_message'])) ? mysql_real_escape_string(trim($_GET['new_message'])) : false; if($new_message) { $query = "INSERT INTO Instant_Messenger (Sendeer, Receiver, Rank, Instant_Message) VALUES ({$user_id}, {$friend_id}, {$rank}, {$new_message})" $result = mysql_query($query); //NOTES: // - not sure what rank represents or how it is defined // - The table should include a field for created_date that is automatically populated on record creation } //Query the names ONLY if not already saved in session //Used to display the names in messages if(!isset($_SESSION['names'][$userId]) || !isset($_SESSION['names'][$friend_id])) { $query = "SELECT User_Id, Username FROM User_Info WHERE User_Id IN ('{$userId}', {$friendId})"; $result = mysql_query($query); while($row = mysql_fetch_assoc($result)) { $_SESSION['names'][$row['User_Id']] = $row['Username']; } } //Create query to retrieve new messages since last access (if passed) $last_access = (isset($_GET['last_access'])) ? (int) $_GET['last_access'] : false; $last_access_sql = ($last_access) ? ' AND created_date > ' . date('Y-m-d', $last_access) : ''; //Create timestamp for this access $this_access = time(); $this_access_sql = ' AND created_date > ' . date('Y-m-d', $this_access); $query = "SELECT Sender, Receiver, Rank, Instant_Message FROM Instant_Messenger WHERE Sender IN ('{$userId}', {$friendId}) AND Reciever IN ('{$userId}', {$friendId}) {$last_access_sql} {$this_access_sql} ORDER BY created_date"; $result = mysql_query($query); //Display the new messages while($row = mysql_fetch_array($result)) { $sender_name = $_SESSION['names'][$row['Sender']]; echo "{$row['Rank']} {$sender_name}: {$row['Instant_Message']}<br>\n"; } //Add a parameter on the return value for the timestamp of this access //The javascript should parse it off of the result and store it to send on the next request echo "-LAST_ACCESS-{$this_access}"; ?>
-
Basic OOP question: using classes in multiple files
Psycho replied to kn0wl3dg3's topic in PHP Coding Help
I am in agreement with your previous comment about not storing data that can become stale. My point was really that you have to take a critical look at what data you need and making an informed decision. My comment above about storing the user's name (not the username) as a session variable would be valid IF I needed to use that value on many/most pages of the site AND I did not also have to pull other information from the user table on those pages. As an example, there is a web application we use in our organization to help manage agile development processes. At the top of every page it says "Welcome Firstname Lastname". Now, that information could be changed by me or probably even an admin. But 99% of the pages I use most likely don't need to pull any information from the user table. They probably use my userid to in conjunction with group/permissions tables when I am working with other data. But, there is rarely a need to access specific user information. So, they could lookup my "name" when I log in and store that as a session variable. Then use that value to display at the top of every page instead of having to perform a database query on every single page just to display that value. Now, if I change my name in user settings the application could be smart enough to determine that I did it and also update the session value. However, if someone else edited my name I may not see that change until the next time I log in. But, is that really a problem? For a user's name that is just text on a page I say no. EDIT: Out of curiosity, I decided to see what happened to the name displayed at the top of the page when I changed my name in the settings. I found it was not updated in the "welcome" message - even after I logged out and restarted the browser. So, I'm guessing it is stored in a cookie. Too lazy to find and crack open the cookie at this time. I would go with a session variable, but a cookie is no more of a risk. It is non-essential information, so it would not be prudent to read it from the database on each page. At least that is my opinion. -
Basic OOP question: using classes in multiple files
Psycho replied to kn0wl3dg3's topic in PHP Coding Help
I'd just add one more thing. As I stated previously (and xyph also stated) the id of the user is what you would pass from one page to another. And you can then access any values/properties of the user as you need them on other pages. You stated above: The second one is problematic if stale data was displayed, but the first would only be a minor inconvenience to the user. If you had a need to display the user's name on every page, then I *would* store that value in a session variable. Again, not an object as it would store ALL of the object data. Basically, what I am saying is if you have some users data that you are going to use on most/all of the pages that is benign then there is no harm in storing it in session data. Something like a name would be benign, whereas permission would not.