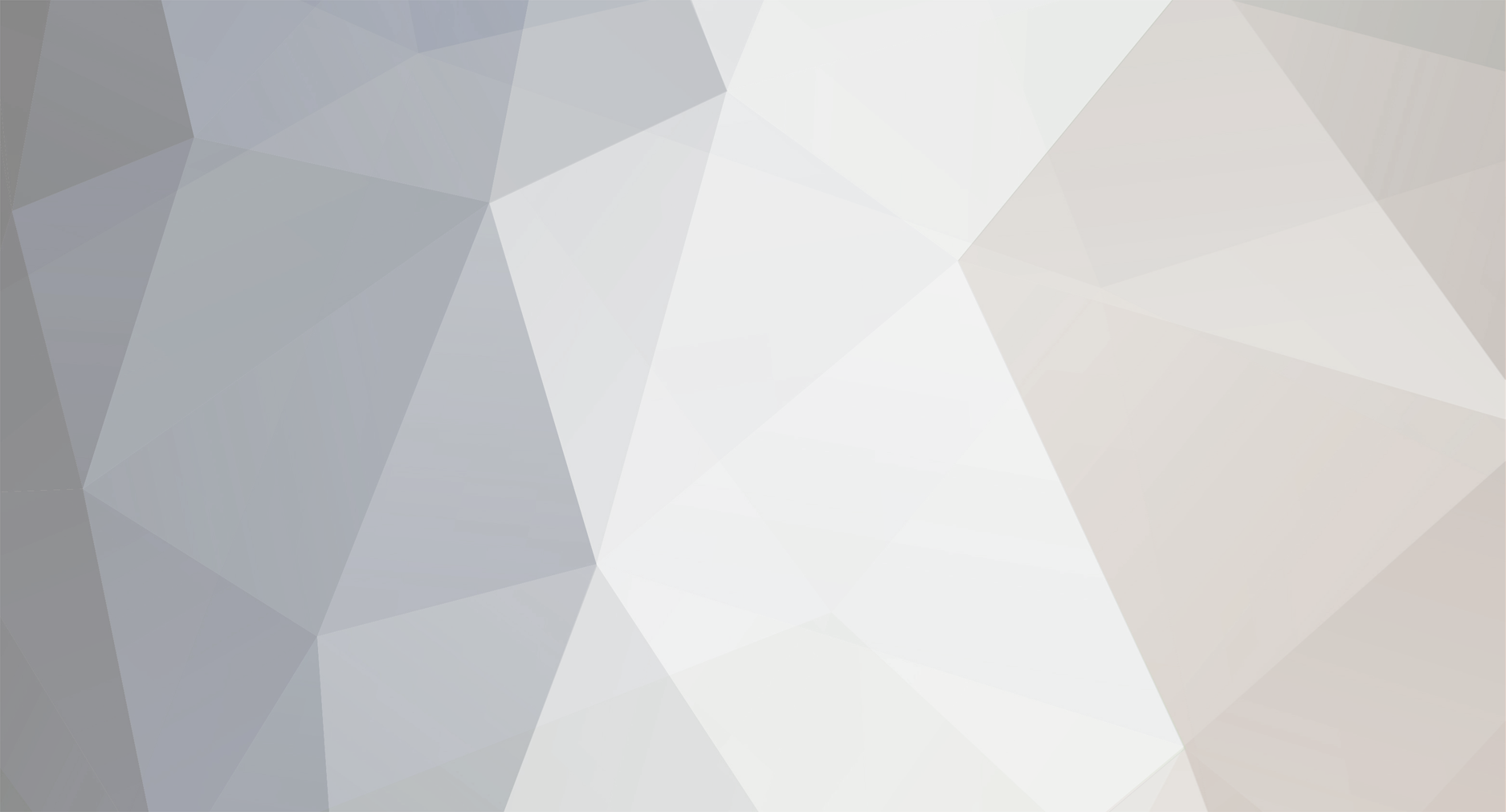
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Well, this may not be the cause of your error (based on the error message you are getting), but one problem is you trying to use header() after sending output to the page. You cannot send any output to the browser before header() statements. Currently, you are trying to display a confirmation or error message before redirecting the user to a different page. How would the user see the message anyway? Remove the redirect message for testing purposes. Once it all works then remove the messages and implement the redirect. You will have to display any messaging on the redirected page. Also, you have several if() statements lumped together - that doesn't make sense. Plus, it assumes that the user is ALWAYS uploading all five images. You need to do some validation before attempting to upload the file. EDIT: You also have problems with your query: 1. No validation to prevent SQL injection 2. You have $_POST['prefix'] duplicated in the values, etc. 3. The fields are not in the right order. For example, $_POST['account_type'] is matched up with the project field. Go back and take your time to ensure everything is kosher and add some error handling.
-
Basic OOP question: using classes in multiple files
Psycho replied to kn0wl3dg3's topic in PHP Coding Help
Why would you need to recreate the object. Do you need ALL the info for the user on every page load or wouldn't you just need the user ID. Besides, relying upon "variable" data from a previous page load on a subsequent page load can be dangerous. Take the example of a user. If you were to pull a user's permissions on a previous page load and stored that as the user navigates from page to page, then the user's permission wouldn't be updated if an administrator made changes until the user logged out and back in. FYI: This is from the manual under design patterns (http://php.net/manual/en/language.oop5.patterns.php) I'm not arguing that singleton's should or should not be used, just that the objects are typically specific to the request (i.e. page load). Again, I would go back to my previous question of why you need the user object created during login on each page load. Typically after the user logs in you would be using other objects for the user to work with different records. -
I'll add my two cents. In the case where you need to have content before and after a subset of records (i.e. the opening and closing DIV tags) I prefer to use the loop to add the records to a temp array in the loop and call a display function when a change is detected. It makes it much easier to "visualize" the output being created as well as to make edits. Just a much cleaner solution IMHO function createTagGroupDiv($portfolioArray) { if(count($portfolioArray)<1) { return false; } //Ensures nothing is created on first record echo "<div class='boxgrid'>\n"; foreach($portfolioArray as $portfolio) { echo "<a href='{$portfolio['fullPath']}' rel='prettyPhoto[{$portfolio['tag']}]'><img src='{$portfolio['thumbPath']}' title='{$portfolio['desc']}' /></a>\n"; } echo "</div>\n"; } //Create and run query $query = "SELECT id, thumbpath, fullpath, tag, desc, adddate FROM portfolio ORDER BY adddate ASC"; $result = mysql_query ($query); //you could realy use some error capture on your query stuff $current_tag = false; //Var to track changes in tag $portfolios_by_tag = array(); //Array to hold records by tag //Loop through results While($row = mysql_fetch_array($result)) // loop through the results from the query { //Check if this tag is different from the last if($current_tag != $row['tag']) { //Set current tag $current_tag = $row['tag']; //Display results from previous group createTagGroupDiv($portfolios_by_tag); //Clear last group records $portfolios_by_tag = array(); } //Add current record to group array $portfolios_by_tag[] = $row; } //Display the last group createTagGroupDiv($portfolios_by_tag);
-
From my sig
-
Basic OOP question: using classes in multiple files
Psycho replied to kn0wl3dg3's topic in PHP Coding Help
Personally, I have never implemented anything to try and "pass" an object from page load to page load. I just create the objects on each page load as needed. You stated an example of a class to log in a user. Why would you even need to reuse that object as the user moves from page to page. If they are logged in, you shouldn't need to even load that class or reuse the object from when they logged in. Simply set a session variable then check if that session var exists on page load. If yes, continue on with the code to display the requested page. If not,then initiate the login class. if(!isset($_SESSION['gogged_in'])) { include('user_login_class.php'); $login_object = new login_class(); // etc } else { //display page } -
I don't believe there was anything to be redeemed. I provided a response valid solution based upon the information (or lack thereof) given.
-
Neil, I did state Yes, I considered that, but since I don't know "how" those divs are created or what their content is, I don't know if a loop would be easily implemented into what he had. And, I wasn't going to go out of my way to rebuild his current code when he didn't take the time to provide it. But, sure you could do something like this: $divs = array(); $divs[1] = "<div>Content of div 1</div>"; $divs[2] = "<div>Content of div 2</div>"; $divs[3] = "<div>Content of div 3</div>"; // etc . . . $divsToDisplay = (int) $_POST['fieldFromFirstPage']; if($divsToDisplay<1 || $divsToDisplay> { //Invalid value - add error handling or set default value $divsToDisplay = 1; } for($divIdx=1; $divIdx<=$divsToDisplay; $divIdx++ { echo $divs[$divIdx]; } But, again, depending on what he is actually doing that may or may not be the best approach.
-
Yes, it is possible. When you say "show only a number of those divs based on the selection of the dropdown from the previous page", do you need the other divs in the page, but just not visible? This might be the case if you have javascript to show/hide the divs dynamically. Also, it would be helpful to see your current code for displaying the divs, but here goes $divsToDisplay = (int) $_POST['fieldFromFirstPage']; if($divsToDisplay<1 || $divsToDisplay> { //Invalid value - add error handling or set default value $divsToDisplay = 1; } if($divsToDisplay >= 2) { //Show div 2 } if($divsToDisplay >= 3) { //Show div 3 } if($divsToDisplay >= 4) { //Show div 4 } if($divsToDisplay >= 5) { //Show div 5 } if($divsToDisplay >= 6) { //Show div 6 } if($divsToDisplay >= 7) { //Show div 7 } if($divsToDisplay >= { //Show div 8 }
-
Why does this happen with mysql_fetch_array ?
Psycho replied to TheStalker's topic in PHP Coding Help
A while() loop continues as long as the condition is true. In the above code you are simply attempting to assign the value of $results to $row. $results was already defined before the loop and equals the first record from the result set. So that condition is simply reassigning $results to $row on each iteration. You aren't getting any new records so $results is basically a static variable. You might was well write the condition as while($foo = 'bar') { Whereas $sql = mysql_query("SELECT * FROM cities"); while($row = mysql_fetch_array($sql)) { echo $row['City']."<br />"; } The condition in this loop is fetching the next record from the result set and assigning the value to $row. When the result set runs out of records the resulting condition is false and the loop ends. -
ManiacDan already gave you a solution. Although I would think array_unique() would be a better fit for this scenario Not tested: $sql = "SELECT project_type FROM projects ORDER BY project_type"; $result = mysql_query($sql); //Dump results into array $project_types = array(); while ($rs = mysql_fetch_array($result)) { $project_types = array_merge($project_types, explode(';', $rs['project_type'])); } //Filter out duplicates $project_types = array_unique($project_types); //Display results foreach($project_types as $type) { echo " {$type}<br />\n"; }
-
Having a problem with my first PHP script
Psycho replied to Freedom-n-Democrazy's topic in PHP Coding Help
Comments, comments, comments - Use Them! A good programmer writes just as many lines of comments as he does of code (if not more). By adding comments, it gives context to the code and many times you can "see" logical mistakes that have been done. In addition to what PFMaBiSmAd stated I think there must be another problem if you cannot register for the other two options. But, I have to ask, why are there THREE values and not TWO? You only need a "mens" value and a "womens" value - both in your form and in the database. The database table should have a field for the email address and then two fields to specify mens and womens which would be 0 (not selected) or 1 (selected). I don't know what your form looks like but, personally, I would use two checkboxes. Also, if something doesn't operate as you expect - add debugging code to echo variables and results to the page to see what went wrong where. Here is some updated code - I give no warranty as to whether it fixes your problems, but it should help identify the problem. <?php if ($_POST['action'] == 'Register') { #Only need to connect to database if there was data submitted //Connect to DB server $link = mysql_connect('localhost', 'testusr', 'testpw'); if(!$link) { die("Unable to connect to database"); } //Select database if(!mysql_select_db('testdb', $link)) { die("Unable to select database"; } //Validate email address $email = mysql_real_escape_string(trim($_POST['e-mail'])); if(empty($email)) { echo "Error: Email address is required"; } else { //Generate query based upon newsletter selection $query = false; if ($_POST['newsletter'] == 'Mens') { $query = "INSERT INTO newsletters(mens) VALUES('$email')"; } elseif ($_POST['newsletter'] == 'Mens & Womens') { $query = "INSERT INTO newsletters(mensandwomens) VALUES('$email')"; } elseif ($_POST['newsletter'] == 'Womens') { $query = "INSERT INTO newsletters(womens) VALUES('$email')"; } //Check that a valid selection was made if(!$query) { echo "Error: No valid newsletter selection made: '{$_POST['newsletter']}'"; } else { //Run query if(!mysql_query($query)) { echo "Error: Unable to add record."; echo "<br>Query: {$query}<br>Error: " . mysql_error(); #only enable this line for debugging } else { echo "Your submission has been registered"; } } } //Close DB connection mysql_close($link); } ?> -
Having a problem with my first PHP script
Psycho replied to Freedom-n-Democrazy's topic in PHP Coding Help
A quick look at the manual for the "if" control structure should show you the problem: http://php.net/manual/en/control-structures.if.php Just look at the examples and see what is different about your code. -
The web host should let you do this - it's your web space. But, you will be restricted to only accessing your areas. You might want to consider putting the storage folder outside the web accessible directory. Aside from that, I would suggest using glob() to access the files.
-
As others have stated, youzz are the one not getting it. If you followed the advice given and a user left their browser open to your site (with no activity) for two hours then they would not be seen as "online" since it would have been more than 15 minutes (or whatever period you set) since their last activity.
-
That error means you are trying to create a function that already exists. It looks like in ver 5.3 of PHP there is an actual function called date_diff(). Doh! If you have version 5.3 you can just use that function. If not, rename the function I provided to something else. EDIT: The fact that you got that error would mean that you are using a version of PHP that has that built in function, or possibly you put the code in a loop on your page. Even if you do have a version of PHP with that built-in function you need to make sure the server(s) you will be deploying to will support it as well.
-
It does work and it is better. Rather than making a worthless comment such as "its not working" why don't you state how it is not working. It works perfectly fine for me. Maybe you need me to show you how to implement it? Here is some unit testing of that code and the output. Please state which of those results is not correct or provide valid inputs that would result in an incorrect output. Unit tests function date_diff($firstDate, $lastDate) { return (int) ((strtotime($lastDate)-strtotime($firstDate))/86400)+1; } $dates = array( array('February 5, 2011', 'April 15, 2011'), array('March 15, 2011', 'May 19, 2011'), array('January 5, 2011', 'June 12, 2011'), array('August 20, 2011', 'August 25, 2011'), array('July 14, 2011', 'December 23, 2011'), array('December 15, 2011', 'January 20, 2012') ); foreach($dates as $datePair) { $startDate = $datePair[0]; $endDate = $datePair[1]; echo "There are " . date_diff($startDate, $endDate) . " day(s) between {$startDate} and {$endDate}<br>\n"; } Output There are 69 day(s) between February 5, 2011 and April 15, 2011 There are 66 day(s) between March 15, 2011 and May 19, 2011 There are 158 day(s) between January 5, 2011 and June 12, 2011 There are 6 day(s) between August 20, 2011 and August 25, 2011 There are 163 day(s) between July 14, 2011 and December 23, 2011 There are 37 day(s) between December 15, 2011 and January 20, 2012 And, I notice you didn't address the statement I made about totally unnecessary logic to converting the timestamps into date-parts only to reconstruct them back into timestamps. That was half of the code from the solution you provided and all it would do is waste cpu cycles.
-
You can't "check" their status. You can update the last time they accessed your site. Just add a function that will run on every page load to update a "last_access" timestamp for users whenever they load a page. Then, determine who is "online" based upon that timestamp, by choosing an appropriate time period: e.g. any users who's "last_access" time is within the last 10 minutes (or whatever period you want to use). A flat file, seriously? How does a flat file save space over a database? Plus, since this will be updated every time a users access a page and when you need to get the data there is a huge possibility for file corruption.
-
Excuse me, but that code is completely unnecessary and illogical. It makes absolutely no sense to use strtotime() to convert the string date into a timestamp THEN to use that timestamp to get the parts for the dates only to convert those parts BACK into a timestamp! Plus, that does not count the days inclusive of the selected dates (need to add 1). All you need is a single line function: function date_diff($firstDate, $lastDate) { return (int) ((strtotime($lastDate)-strtotime($firstDate))/86400)+1; } $startDate = 'September 08, 2011'; $endDate = 'October 13, 2011'; echo "There are " . date_diff($startDate, $endDate) . " day(s) between {$startDate} and {$endDate}"; //Output: There are 36 day(s) between September 08, 2011 and October 13, 2011
-
how to let only certain ips use scripts on my site
Psycho replied to devWhiz's topic in PHP Coding Help
Note, that there is no fool-proof way to do this since IP addresses can be spoofed. Plus, it may not be a good idea if your users have dynamic IP addresses from their host. Their IP address can change without warning, leaving them without access. $allowed_ips = array('123.123.123.123', '125.125.125.125', '1.2.3.4', '11.22.33.44'); $user_ip = $_SERVER['REMOTE_ADDR']; if(!in_array($user_ip, $allowed_ips)) { //User's IP not in allowed list echo "Go away"; exit(); } else { //Show page } -
Since your form already requires javascript, why require the user to select the button to see the text. just add the text dynamically. <?php $carsAry = array( 'Ford' => array( 'Escort' => '£1,200', 'Focus' => '£1,300', 'Fiesta' => '£1,400' ), 'Vauxhall' => array( 'Astra' => '£2,200', 'Vectra' => '£2,300', 'Corsa' => '£2,400' ), 'Honda' => array( 'Civic' => '£3,200', 'Accord' => '£3,300', 'Odyssey' => '£3,400' ) ); $selectedModel = (isset($_POST['manufacturer'])) ? $_POST['manufacturer'] : false; //Iterate through models to generate javascript and select lists $js_model_lists = ''; $js_model_price = ''; $model_options = ''; foreach($carsAry as $make => $modelsAry) { //Select options $selected = ($selectedModel==$make) ? ' selected="selected"' : ''; $model_options .= "<option value=\"{$make}\"{$selected}>{$make}</option>\n"; //JS arrays $make_models = array(); $js_model_price .= "prices['{$make}'] = new Array();\n"; foreach($modelsAry as $model => $price) { $make_models[] = "'$model'"; $js_model_price .= "prices['{$make}']['{$model}'] = '{$price}';\n"; } $js_model_lists .= "models['{$make}'] = new Array(" . implode(', ', $make_models) . ");\n"; } ?> <html> <head> <script type="text/javascript"> // model lists var models = new Array(); <?php echo $js_model_lists; ?> // model prices var prices = new Array(); <?php echo $js_model_price; ?> function setmodel() { var manufacturerSel = document.getElementById('manufacturer'); var modelList = (models[manufacturerSel.value] != undefined) ? models[manufacturerSel.value] : false; changeSelect('model', modelList); } //**************************************************************************// // FUNCTION changeSelect(selectID, valuesAry, [optTextAry], [selectedVal]) // //**************************************************************************// function changeSelect(selectID, valuesAry, optTextAry, selectedValue) { var selectObj = document.getElementById(selectID); //Clear the select list selectObj.options.length = 0; if(valuesAry!==false) { //Set the option text to the values if not passed optTextAry = (optTextAry) ? optTextAry : valuesAry; //Itterate through the list and create the options for (i in valuesAry) { selectFlag = (selectedValue && selectedValue==valuesAry[i])?true:false; selectObj.options[selectObj.length] = new Option(optTextAry[i], valuesAry[i], false, selectFlag); } selectObj.disabled = false; } else { selectObj.options[selectObj.length] = new Option(' - Select a Make - ', '', false); selectObj.disabled = true; } showPrice(); } function showPrice(selectedCar) { var makeObj = document.getElementById('manufacturer'); var modelObj = document.getElementById('model'); var selectedMake = makeObj.options[makeObj.selectedIndex].value; var selectedModel = modelObj.options[modelObj.selectedIndex].value; var price = (selectedMake && selectedModel) ? prices[selectedMake][selectedModel] : ''; document.getElementById('price').innerHTML = price; } </script> </head> <body onLoad="setmodel();"> <h1>Personal manufacturer Calculator</h1> <form> <fieldset> <legend><p>Approximate insurance value</p></legend> <p>Please select the vehicle manufacturer from the drop down list then choose the desired vehicle model.</p> <p> <label for="manufacturer">manufacturer:</label><br/> <select name="manufacturer" id="manufacturer" onChange="setmodel();"> <option value=""> - Select a Make - </option> <?php echo $model_options; ?> </select> </p> <p> <label for="model">Model of manufacturer:</label><br/> <select name="model" id="model" onChange="showPrice();" disabled="disabled"> <option value=""> - Select a Make - </option> </select> </p> Price: <span id="price"></span> </fieldset> </form> </body> </html>
-
For creating the query to update the records I would use the exact same array of field names that you used to create the checkboxes. That is why I suggested using the field names from the database as the names for the checkbox fields. The code would look something like this if(isset($_POST['id'])) { $project_id = (int) $_POST['id']; //Loop through all checkboxes to generate update code $updateValues = array(); foreach($checkboxFields as $field) { $updateValues[] = "{$field} = " . ((isset($_POST[$field])) ? '1' : '0'); } //Create the query $query = "UPDATE checklist SET " . implode(', ', $updateValues) . " WHERE project = {$project_id}"; } [/code]
-
I have submitted code for exactly what you are asking many times in these forums. Here is one: http://www.phpfreaks.com/forums/index.php?topic=156380.msg679722#msg679722
-
First of all, you need to state exactly what you are doing now. There was some back and forth previously over whether you would allow editing of multiple records or only one. I don't know what your decision is. If you are allowing of only one record then it is not necessary (or even a good idea) to put the record id as the value of the checkbox since, as you know, only checked field are passed in the post data. Therefore, if no checkboxes were selected then you would have no reference for the record ID. Second, it looks like you were trying to run an update query for each field individually. That is a terrible idea. You should never run queries in a loop - they are a resource/performance hog. Jsut get the updated values for ALL fields and run one update query for the record.
-
Sorry, I didn't pay attention to the variables used in the player output. This should work $currentStatus = false; //Flag to detect change in status while($player = mysql_fetch_assoc($results)) { if($currentStatus != $player['status']) { //Status has changed, display status header $currentStatus = $player['status']; echo "<div>{$currentStatus}</div>\n"; } //Display player info echo "{$player['playerFirst']} {$player['playerLast']}, {$player['year']}<br>\n"; }
-
I see a lot of problems with what you are trying to do. And, to be honest, I just don't have the time to debug it today. But, here are some comments if (isset($_POST[$fieldName])) { $id = $_POST[$fieldName]; } You are setting $id to the VALUE of the field. You need to be setting it to the INDEX of the field (if a mult-record form) or to some hidden field with the ID if a single record form. Based upon the code you have it looks to be a single record form. echo yur query to the page