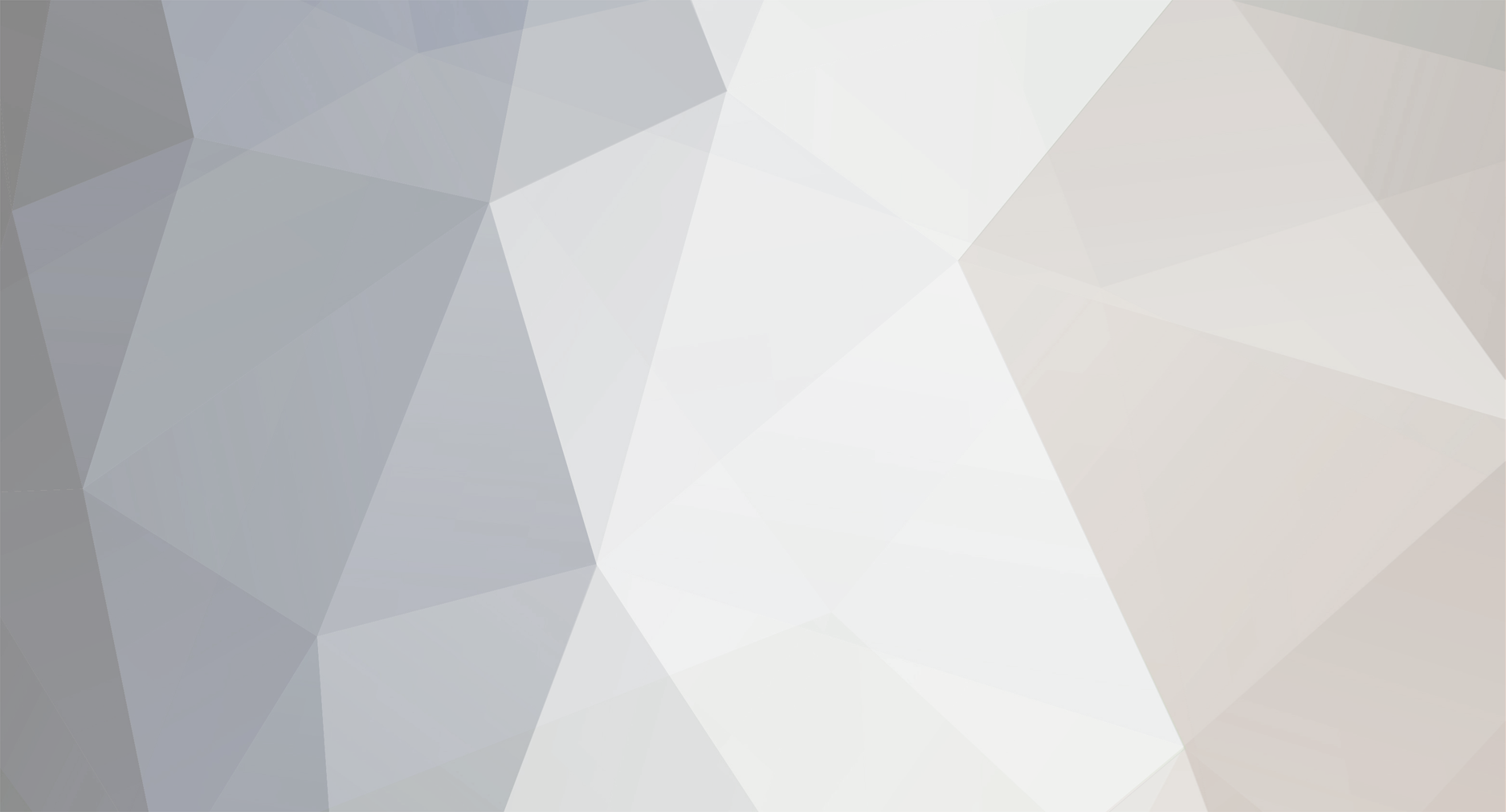
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
How to refer to DOM object in div classes (from my CSS)?
Psycho replied to aooga's topic in Javascript Help
Just give whatever DIV you want to modify an ID, then use document.getElementById('DIV_ID').style.backgroundColor='#ff0000'; -
How to Calculate the total value of an array of fields
Psycho replied to giba's topic in Javascript Help
Here's a very simple example. You would want to add additional validation/error handling and I suspect you would want the total fields to be read only. <html> <head> <script type="text/javascript"> function isNum(value) { return 123; } function calcTotals() { var grandTotal = 0; var row = 0; while (document.forms['cart'].elements['price[]'][row]) { priceObj = document.forms['cart'].elements['price[]'][row]; qtyObj = document.forms['cart'].elements['quantity[]'][row]; totalObj = document.forms['cart'].elements['total[]'][row]; if (isNaN(priceObj.value)) { priceObj = ''; } if (isNaN(qtyObj.value)) { qtyObj = ''; } if (priceObj.value && qtyObj.value) { totalObj.value = (parseFloat(priceObj.value) * parseFloat(qtyObj.value)); grandTotal = grandTotal + parseFloat(totalObj.value); } else { totalObj.value = ''; } row++; } document.getElementById('grand_total').value = grandTotal; return; } </script> </head> <body> <form name="cart"> <table> <tr><th>Price</th><th>Quantity</th><th>Total</th></tr> <tr> <td><input name="price[]" onchange="calcTotals()" /> </td><td><input name="quantity[]" onchange="calcTotals()" /> </td><td><input name="total[]" /></td> </tr><tr> <td><input name="price[]" onchange="calcTotals()" /> </td><td><input name="quantity[]" onchange="calcTotals()" /> </td><td><input name="total[]" /></td> </tr><tr> <td><input name="price[]" onchange="calcTotals()" /> </td><td><input name="quantity[]" onchange="calcTotals()" /> </td><td><input name="total[]" /></td> </tr><tr> <th colspan="2" style="text-align:right;">Grand Total</td> <td><input name="gTotal" id="grand_total" /></td> </tr> </table> </form> </body> </html> -
Append the variables to the URL and access them on the receiving page via $_GET Using the process I described above to get the form object in the function you would do something like this: var clientID = formObj.elements['ClientID'].value; var desc = formObj.elements['description'].value; strActionPage = CurrentPath + "/SaveToDB/SaveToDB.php?cid=" + clientID + '&desc=" + desc; Then when the page SaveToDB.php is called you can access those values in the page using $_GET['cid'] & $_GET['desc']
-
Pass the variables to "what" exactly? I don't see any AJAX code in what you posted. There are two main functions that appear to have some custom commands in them (e.g. frmScan.DynamicWebTwain1.SelectSource(). I assume those commands are defined elsewhere. I don't know anything about those custom commands or how you would implement those variables into them. Maybe they aren't even built to accept parameters. I can show you how to pass the form values to those two main functions though. It's up to you what you do with them from that point. Add 'this.form' to the onclick events like this <input type="button" value="Scan" ID = "btnScan" onclick="return btnScan_onclick(this.form)"> <input type="button" value="Upload" ID = "btnUpload" onclick="return btnUpload_onclick(this.form)"> Then change the two main functions to accept a parameter like this function btnScan_onclick(formObj) function btnUpload_onclick(formObj) Within those two functions you can now access the values on the form in this manner: formObj.elements['FIELDNAME'].value
-
update a field value for multiple records at once
Psycho replied to jeff5656's topic in PHP Coding Help
A checkbox returns the value of the checkbox when it is checked. If it is not included in the post data at all. So the checkboxes should be set up with a value equal to the ID of the record. This is a pretty simple process that consists of two steps in my opinion. 1. Create a form with a checkbox for each record. The checkboxes should have the same name in the form of an array (e.g. 'IDS[]') 2. The page that processes the form will run a single query along the lines of "UPDATE table SET field='value' WHERE field2 in ([comma separated list from the checkbox array])" Here is some sample code based upon what you posted: Code to generate teh checkboxes (needs to be in a form that posts to the processing page) $query = "SELECT dos_id, completed FROM icu INNER JOIN dos ON icu.id_incr = dos.pt_id AND dos.completed = 'n' "; $results = mysql_query ($query) or die (mysql_error()); //Create checkboxes for each record while ($row = mysql_fetch_assoc ($results)) { echo "<input type=\"checkbox\" name=\"dos_ids[]\" value=\"{$row['dos_id']}\"> {$row['completed']}<br>\n"; } Code for the processing page if (isset($_POST['dos_ids'])) { //Set 'completed' value for ALL records to 'n' $sql = "UPDATE dos SET completed = 'n'"; $result = mysql_query($sql) or die ("Invalid query: " . mysql_error()); //Set 'completed' value to 'y' for all of the checked records $idList = "'" . implode("', '", $_POST['dos_ids']) . "'"; $sql = "UPDATE dos SET completed = 'n' WHERE dos_id IN ($idList)"; $result = mysql_query($sql) or die ("Invalid query: " . mysql_error()); }[code=php:0] Note: I did not test any of that, but it should give you the process for doing what you have asked. Also, that only works if you are always going to have a page where all of the records are being updated at the same time. If you will only have some of the records to be updated you will need to pass some information from the form to the processing page to identify what records were being updated. -
Interestingly that code checks to see if $_COOKIE["usPass"] is set, but doesn't even use it in the query to get the suer data. So, basically anyone could change their cookie to the name of an admin and they would get access to the admin link! (if it worked that is). Assuming you are not getting any errors, your query is either empty or the value in $row['user_status'] is not what you are testing. Passwords should be hashed with a salt What does this display? if(isset($_COOKIE["usNick"]) && isset($_COOKIE["usPass"])) { echo " <ul id=\"nav\">\n"; echo " <li class=\"active\"><a href=\"index.php\">Home</a></li>\n"; echo " <li><a href=\"viewads.php\">View Ads</a></li>\n"; echo " <li><a href=\"myaccount.php\">My Account</a></li>\n"; echo " <li><a href=\"logout.php\">Logout</a></li>\n"; echo " <li><a href=\"terms.php\">TOS</a></li>\n"; echo " <li><a href=\"advertise.php\">Advertise</a></li>\n"; if(ENABLE_FORUMS=="yes") { echo"<li><a href='".FORUM_LINK."'>Forum</a></li>"; } $sql = "SELECT * FROM yob_users WHERE username='$user'"; $result = mysql_query($sql) or die(mysql_erro()); $row = mysql_fetch_array($result); $is_admin = $row['user_status']; //For debugging only echo '<span style="background-color:#cecece;">$row['user_status'] = ' . $row['user_status'] . '<span>'; if($is_admin =="admin" && $_COOKIE["usPass"]==$row['usPass']) { echo "<li><a href='/admin'>Admin</a></li>"; } }
-
[SOLVED] Changing div backgrounds on radiobutton select
Psycho replied to Darkmatter5's topic in Javascript Help
If I may, this would be more flexible. With this function, you can add/remove options without having to modify the function (note there is also an ID parameter added to the radio button options). <html> <head> <style type="text/css"> #step1, #step2, #step3 { position: absolute; top: 0; left: 400px; width: 440px; height: 375px; display: none; /*padding: 5px;*/ background-color : #CCCC66; } #opt_but1, #opt_but2, #opt_but3 { /*background-color: #CCCC66;*/ } #steps { position: absolute; top: 0; left: 0; width: 400px; height: 375px; /*background-color: gray;*/ /*border-bottom: 1px dashed;*/ } </style> <script type="text/JavaScript"> function togdiv(radiodiv) { //Get the numeric portion of the radio button div id var ID = radiodiv.id.substr(; var i=1; while(document.getElementById('step'+i)) { document.getElementById('step'+i).style.display = (ID==i) ? 'block' : 'none'; document.getElementById('radioOpt'+i).style.backgroundColor = (ID==i) ? '#CCCC66' : '#FFFFFF'; i++; } return; } </script> </head> <body> <div id="steps"> </div> <div id="opt_but1"><input type="radio" name="toggledivs" id="radioOpt1" onclick="togdiv(this);">Optional step 1: (description & notes)</div> <div id="opt_but2"><input type="radio" name="toggledivs" id="radioOpt2" onclick="togdiv(this);">Optional step 2: (systems, publishers & developers)</div> <div id="opt_but3"><input type="radio" name="toggledivs" id="radioOpt3" onclick="togdiv(this);">Optional step 3: (UPCs)</div> <div id="step1">step1</div> <div id="step2">step2</div> <div id="step3">step3</div> </body> </html> YOu could remove the divs around the radio buttons if those are not needed for other purposes. -
Try using a valid email address here: $mailheaders = "From: my site\r\n";
-
Basically yes. But, checking to see if an object exists is good practice anyway. Especially when you get into functions/properties that are different from browser to browser.
-
It has nothing to do with your email address. Yahoo! does not allow you to use them as an SMTP service (at least not with their free accounts). GMail does, but you are required to pass a username and password. I don't know how that is configured within PHP to work. Let me try to explain this more plainly. When you want to access a webpage your browser must contact a web server and request the page. The webserver then finds the page and returns it to your browser. In the case of server-side pages, such as PHP, the web server will process the page and return the output to the browser. Your XAMPP environment has all of that and was the reason you were getting garbage by trying to make your browser open the php files directly. You were not going through the web server to process the files. In the same way you need an email server to send emails. When you use a service like yahoo, the web page is communicating with the web server to send and receive the email displayed on the web page. Your browser does not communicate with the web server, the web pages do. Non web based email is typically done with an email client like Outlook. It will be configured to send and recieve email directly with the email server. You "should" be able to find out the SMTP server for whatever ISP you are currently using and use that since most ISPs do not require authentication for people already logged into their network. I run XAMPP as well. Within my XAMPP install there is an email service called "Mercury". I have not used it, so I don't know what configurations may, or may not, be needed (it wouldn't start on my machine and I don't have the time to research). You could try enabling it through the XAMPP control panel and using localhost. however, as stated before, this would only work in your XAMPP environment. Your production environment would probably be different.
-
My script doesn't have an error in it! That was done on purpose to make the script work onload. Could you explain your script, mjdamato? I don't understand how your while loop breaks, because the condition never changes... My appologies, I recognized the reason for the first test before completing my response, but I forgot to remove that statement. As for the conditin never changing . . . it does: document.getElementById('step'+i).style.display = (fieldID==i++) ? 'block' : 'none'; When you use var++, the variable is first returned and then incremented by one. So, on the first pass it checks if 'fieldID' is equal to the current value of 'i' and then increments 'i' by one. FYI: ++var is slightly different in that it increments the value and then returns it Examples var foo = 1; alert(foo); // alerts 1 //foo++, return current value then increment it alert(foo++); // alerts 1 (foo now equals 2) alert(foo); // alerts 2 //foo++, increment current value then return it alert(++foo) // alerts 3 (foo equals 3) alert(foo); // alerts 3
-
That doesn't seem right. You hav a loop to run queries, but don't do anything with the results -they just get overwritten by the next query. Also, running multiple queries will be inefficient. This will be more compact and will also hadle a couple other problems. 1) If the string has consecutive spaces in the middle this replaces them with just one. 2) Also "cleans" the string for query purposes: //Clean up the string $find = strip_tags(strtoupper(trim($find))); //Remove multiple spaces from within string $find = preg_replace('/[ ]+/', ' ', $find); //Create MySQL code $find = explode(' ', $find); foreach ($find as $term) { $term = mysql_real_escape_string($term); $terms[] = "\n (casetype LIKE '%$term%') OR (caseinfo LIKE '%$term%')"; } //Create the query $query = "SELECT * FROM cases WHERE" . implode(' OR ', $terms); $result = mysql_query($query) or die(mysql_error()); If the search value was " one <b>two</b> three " the resulting query would look like this: SELECT * FROM cases WHERE (casetype LIKE '%ONE%') OR (caseinfo LIKE '%ONE%') OR (casetype LIKE '%TWO%') OR (caseinfo LIKE '%TWO%') OR (casetype LIKE '%THREE%') OR (caseinfo LIKE '%THREE%')
-
Yes, that is the problem. You need to point the php.ini configuration for the mail server to an active mail server. If you are not running one on your machine then you need to point it to a valid mail server. Find the mail server settings you use for your email and point it to that one.
-
A radio group must all have the same name. You should also check the first option by default if section 1 will be displayed by default. <input type="radio" name="toggledivs" onclick="togdiv('step1');" checked="checked">STEP 1: (required data & description)<br> <input type="radio" name="toggledivs" onclick="togdiv('step2');">STEP 2: (systems, publishers & developers)<br> <input type="radio" name="toggledivs" onclick="togdiv('step3');">STEP 3
-
The first test in that function has an error (it is checking the field object instead of the ID). But there is a simpler way to do all of that with much less code. Tis will alllow you to add or remove div sections withouth having to change the function <html> <head> <title>A Title!</title> <script type="text/JavaScript"> <!-- function Hide(fieldID) { var i = 1; while(document.getElementById('step'+i)) { document.getElementById('step'+i).style.display = (fieldID==i++) ? 'block' : 'none'; } return; } //--> </script> </head> <body onload="Hide(1)"> <div id="step1"> Div 1 Content<br> <input type="button" id="2" value="Goto step 2 ->" onclick="Hide(this.id)" /> </div> <div id="step2"> Div 2 Content<br> <input type="button" id="1" value="<- Goto step 1" onclick="Hide(this.id)" /> <input type="button" id="3" value="Goto step 3 ->" onclick="Hide(this.id)" /> </div> <div id="step3"> Div 3 Content<br> <input type="button" id="2" value="<- Goto step 2" onclick="Hide(this.id)" /> <input type="button" id="4" value="Finish" onclick="Hide(this.id)" /> </div> </body> </html>
-
A "time" picker? A date picker I would understand, but what would be the need for a JS time picker. Simply create two select lists side-by-side. One for the hours and one for the minutes (or a single select list with all the times for a 24 hour period in increaments of 15 or 30 minutes). No need to make the page less accessible by using JS when not needed. Or, if your requirement is more complex than simply selecting/entering the time, please elaborate.
-
The web server cannot send email - it needs to make a request via an email server (web server = web pages, email server = email). Since you showed some details for XAMPP, I am assuming you are currently testing this on your local machine. So, even once you get this configured on your local machine, you may also need to make changes in the production environment. This page explains the php.ini settings you need to set in a Windows environment: http://www.php.net/manual/en/mail.setup.php
-
<?php //Make a loop to get the values $i = 1; while (isset($_POST['ch'.$i])) { $QUESTIONS[] = trim($_POST['ch'.$i]); $i++; } print_r($QUESTIONS); # For the first 13 array elements, add 1 value to $PositiveFirstCriteria $PositiveFirstCriteria = 0; for ($count=0; $count<13; $count++) { if ( $QUESTIONS['ch'.$count'] == "Question" ) { $PositiveFirstCriteria++; echo "<p>Count: $count, $PositiveFirstCriteria</p>"; } } # If $PositiveFirstCriteria > 6 (@ScreenPositive) # If yes to $PositiveSecondCriteria and moderate or serious to $PositiveThirdCriteria ?>
-
Are you opening those files in your web browser with the URL of "file:///C:/xampp/htdocs/xampp/test101.html"? If so, that was what kenrbnsn was stating. You need to call the files through a web server. In this case it would be "http://localhost/test101.html" for the first file.
-
There soon will be! As well as any other TLD that anyone wishes to create. In June of last year ICANN approved a proposal to allow custom Top Level Domains.
-
Your web server needs to be configured to run PHP. There are plenty of tutorials out there on how to install and configure it. It's not somethign that I would try and do through a forum.
-
HOW TO SHOW OTHER DATA WHEN A COUNTRY NAME IS SELECTED FROM A LIST BOX
Psycho replied to rumon007's topic in Javascript Help
<?php $display_block = "<table width=\"50%\" hight=\"90%\" cellpadding=\"10\" cellspacing=\"0\" border=\"1\" align=\"center\">"; $display_block .= "<tr>\n"; $display_block .= "<td>\n"; $display_block .= "<input type=\"hidden\" name=\"op\" value=\"edit\">\n"; $display_block .= "<select name=\"select_country\">\n"; while($country= mysql_fetch_array($get_country_result)) { $js_array .= "rates[{$country['id']}] = '{$country['rate']}';\n"; $display_block.="<option value=\"{$country['id']}\">{$country['country_name']}</option>\n"; } $display_block .= "</select></td>\n"; $display_block .= "<td><input type=\"text\" name=\"edit_rate\" size=\"10\"></td>\n"; $display_block .= "<td><input type=\"submit\" name=\"submit\" value=\"Update\"></td>\n"; $display_block .= "</tr>\n"; $display_block .= "</table>\n"; ?> <html> <head> <script type="text/javascript"> rates = new Array(); <?php echo $js_array; ?> function displayRate(selObj) { selID = selObj.options[selObj.selectedIndex].value; document.getElementById('edit_rate').value = rates[selID]; } </script> </head> <body> <?php echo $display_block; ?> <body> </html> -
1. It looks like PHP is not enabled in the environment you are running. 2. In the code for building the variables for the email you are redefining several of the variables instead of concatenating $msg = "Name: ".$_POST["name"]."\n"; $msg = "E-mail: ".$_POST["email"]."\n"; $msg = "Message: ".$_POST["message"]."\n"; $msg would only be set to that last line. Need to use something like this: $msg = "Name: ".$_POST["name"]."\n"; $msg .= "E-mail: ".$_POST["email"]."\n"; $msg .= "Message: ".$_POST["message"]."\n"; and for the $mailheaders you would need to separate the values instead of just concatenating them together as-is: $mailheaders = "From: my site" . . "\r\n"; $mailheaders .= "Reply - To: .$_POST["email"]; Here is some "corrected" code <html> <head> <title> Sending Email Form in listing </title> </head> <body> <?php $name = trim($_POST['name']); $email = trim($_POST['email']); $msg = trim($_POST['message']); echo "<p>Thank You, <b>$name</b>, for your message!</p>\n"; echo "<p>Your email address is: <b>$email</b></p>"; echo "<p>Your message was:<br />".nl2br($msg)."</p>"; //start building the mail string $msg = "Name: $name\n"; $msg .= "E-mail: $email\n"; $msg .= "Message:\n$msg\n"; //set up the mail $recipient = "jessicarondon1@yahoo.com"; $subject = "Form Submition Results"; $mailheaders = "From: my site"; $mailheaders .= "Reply - To: " .$_POST["email"]; //send the mail mail if (mail($recipient, $subject, $msg, $mailheaders)) { echo "<br><br>Message sent." } else { echo "<br><br>Problem sending email." } ?> </body> </html>
-
I think you are going to need to change this line if($ad >count($drivers)){ $ad = 0; } The drivers array has elements with indexes from 0 to 3, but the count() of the drivers array will be 4. So, when the value is equual to 4 there is no corresponding array value. But, it is also not greater than the count of 4. I think that should be this: if($ad >= count($drivers)){ $ad = 0; }
-
Using the database: <?php $drivers = array( 0 => 'PR0AMA you will receive 10', 1 => 'PR14MA you will receive 20', 2 => 'PR1EMA you will receive 30', 3 => 'PR28MA you will receive 40', 4 => 'PR32MA you will receive 50' ); $query = "SELECT LastAd from `table`"; $result = mysql_query($query) or die(mysql_error()."<br /><br />".$q); $row = mysql_fetch_assoc($result); $NewAd = ($row['LastAd']+1) % count($drivers); $query = "UPDATE `table` Set LastAd = '$NewAd'"; $result = mysql_query($query) or die(mysql_error()."<br /><br />".$q); echo "Curent Ad: {$drivers[$NewAd]}"; ?> Or using a flat file: (drivers.txt) PR0AMA you will receive 10 PR14MA you will receive 20 PR1EMA you will receive 30 PR28MA you will receive 40 PR32MA you will receive 50 PHP Code <?php $drivers_file = 'drivers.txt'; $drivers_list = file($drivers_file); $current_discount = array_shift($drivers_list); $drivers_list[] = $current_discount; $drivers_text = trim(implode("", $drivers_list)); $fileObj = fopen($drivers_file, 'w') or die("can't open file"); foreach($drivers_list as $driver) { fwrite($fileObj, $driver); } echo "Current discount: $current_discount";