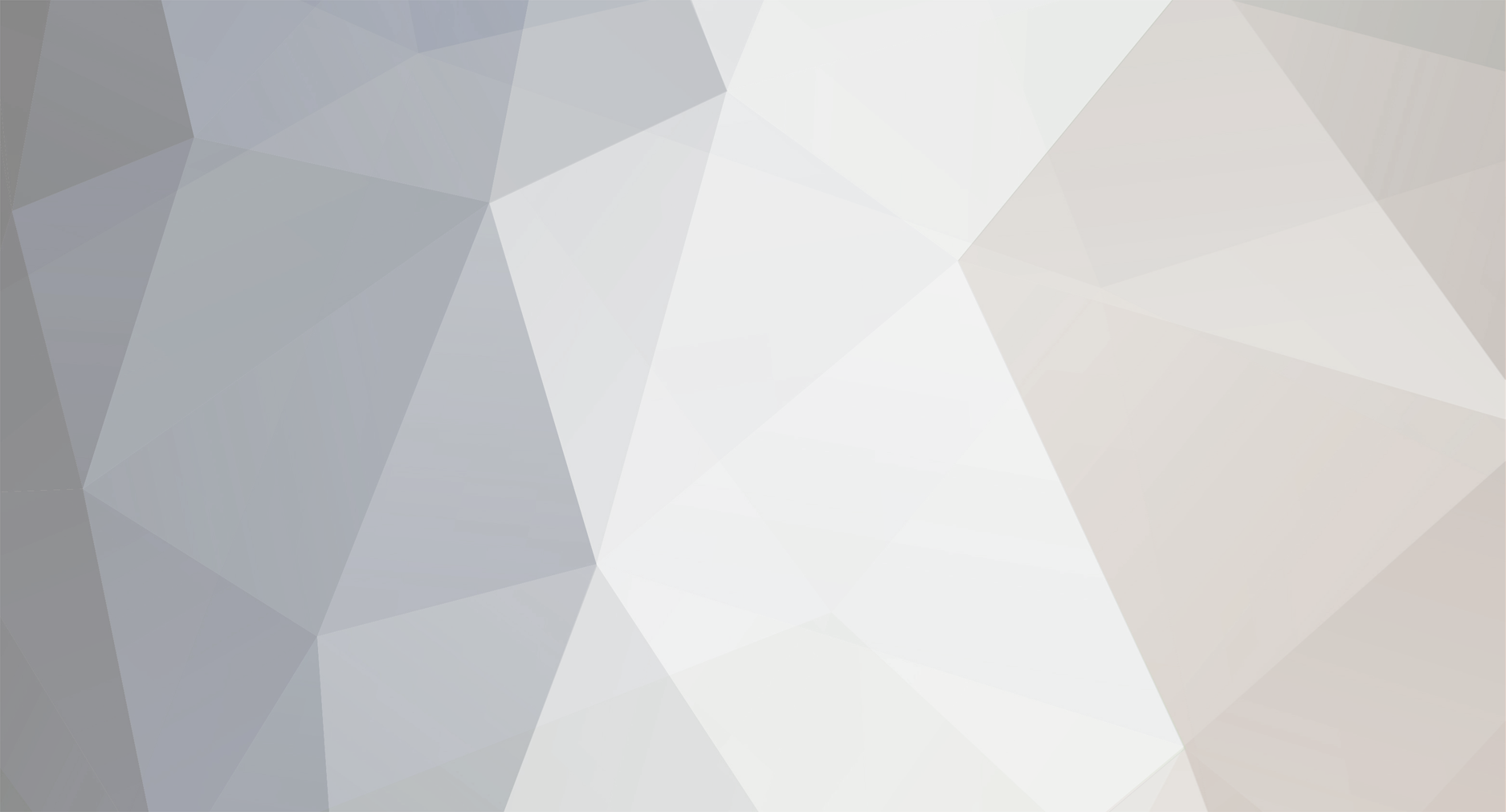
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
Well, first off I will state that you should just pre-select one of the radio options since the user can only change the selected option and cannot unselect all of the options. But, there are some cases where you do not want to pre-select an option. The problem is that a radio GROUP is an array of elements - each with it's own value (however, if there is only one element in the group then it is not an array). You need to determine which element is CHECKED and return the value of that element. Add this function to your page: function radioGroupValue(groupObj) { //Check if radio group has multiple options (i.e. is an array) if (groupObj.length) { //Radio group has multiple options //Iterrate through each option for (var i=0; i<groupObj.length; i++) { //Check if option is checked if (groupObj[i].checked) { //Return value of the checked radio button return groupObj[i].value; } } } else { //Radio group only has one option if (groupObj.checked) { return groupObj.value; } } //No ption was selected return false; } Then change your validation to this: if (radioGroupValue(formObj['gender'])!==false) { errors[errors.length] = Gender cannot be empty.'; }
-
Add an optgroup to dynamically generated dropdowns.
Psycho replied to Scooby08's topic in Javascript Help
This page has an example: http://groups.google.co.ke/group/comp.lang.javascript/msg/011abc4a091f62c5 -
That code should do that. But, here is an example page that will combine the PHP and JavaScript validation. You can use it as a guide. Either turn off JS or take out the onsubmit trigger from the form to test the PHP validations. <?php //Create an array to hold the errors $errors = array(); //Create post values as var. This is useful in case //you need to run stripslashes or some other process. $name = $_POST['name']; $phone = $_POST['phone']; $email = $_POST['email']; //Validate the form data if (isset($_POST)) { if (empty($name)) { $errors[] = 'Name cannot be empty.'; } if (empty($phone)) { $errors[] = 'Phone cannot be empty.'; } if (empty($email)) { $errors[] = 'Email cannot be empty.'; } if (!count($errors)) { //Process the form data include('processing_page.php'); //Reload page to clear POST values (in case user refreshes page) header('Location: '.$_SERVER[php_SELF]) } else { $errorMsg = "The following errors occured:<br />\n"; $errorMsg = "<ul>\n"; foreach($errors as $error) { $errorMsg .= "<li>{$error}</li>"; } $errorMsg .= "</ul>\n"; } } ?> <html> <head> <script type="text/javascript"> //Add javascript prototype to trim string String.prototype.trim = function() { return this.replace(/^\s+|\s+$/g,''); } function validate(formObj) { //Create an array to hold all the errors errors = new Array(); //Perform each validation if (formObj['name'].value.trim()=='') { errors[errors.length] = 'Name cannot be empty.'; } if (formObj['phone'].value.trim()=='') { errors[errors.length] = 'Phone cannot be empty.'; } if (formObj['email'].value.trim()=='') { errors[errors.length] = 'Email cannot be empty.'; } //If errors dispay message and return false if (errors.length != 0) { var errorMsg = 'The following errors occured:\n'; for (var i=0; i<errors.length; i++) { errorMsg += '\n - ' + errors[i]; } alert(errorMsg); return false; } //No errors, return true return true; } </script> </head> <body> Please enter your data. All fields are required. <br /> <?php echo $errorMsg; ?> <br /> <form name="test" onsubmit="return validate(this);" method="POST" action=""> Name: <input type="text" name="name" value="<?php echo $name; ?>" /><br /> Phone: <input type="text" name="phone" value="<?php echo $phone; ?>" /><br /> Email: <input type="text" name="email" value="<?php echo $email; ?>" /><br /> <br /> <button type="submit">Proceed</button> </form> </body> </html>
-
Also, you cannot do two comparisons on one value like that. In that statement it doesn't know what you are comparing to be less than or equal to 25. You would need this: case ($age >= 17 && $age <= 25)
-
Although JavaScript is a great userfriendly way to do some pre-validation, you should ALWAYS include validation server-side as well. Not everyone has JS enabled and someone could easily bypass any JavaScript validation to do some malicious things to your site. There's no problem in adding javascript valiadtion as long as it is on top of the server-side validation.
-
Well, it would have been nice if you provided the code to actually create the fields. The above only shows where you are settng properties for the element. But, in the code where you create the element you are setting the name as heightInput.name="itemHeight_"+i; But in the code to reference the field you are using var setName='itemName_'+a; 'itemHeight_' != 'itemName_' If that was just a typo for posting and they really are the same in the code, I would suggest trying to reference the fields via their ID. a=0; while(a<numberItems) { //the form has already been got by element id and stored as theForm. var setID='itemName_'+a; alert(setID); if (document.getElementById(setID) && !document.getElementById(setID)) { alert('name not set'); hasError=true; sError += " Please xxx.\n"; } a++; } // while
-
[SOLVED] radio buttons coming out of my ears
Psycho replied to cleary1981's topic in Javascript Help
Well, the HTML code is way out of wack - all of the BODY code is before your opening HTML tag! You will want to change the radio group names. The elements of the form are treated as an array and the JavaScript gets confused between an element that is at the index position '1' (the second element in the array) and an element with the name of '1'. In my example below I changed all of the radio groups to 'group_1', 'group_2', etc. Another problem is that a radio group with multiple elements is treated as an array, whereas a radio group with only 1 element is not. I accounted for that in the code below. Here's some basic code to get the value of the selected radio option: function getSelectedRadio(radioGroupObj) { if (radioGroupObj.length) { for(var i=0; i<radioGroupObj.length; i++) { if (radioGroupObj[i].checked) { radioValue = radioGroupObj[i].value; break; } radioValue = false; } } else { radioValue = radioGroupObj.value; } return radioValue; } function proceed(formObj) { alert(getSelectedRadio(formObj['group_1'])); alert(getSelectedRadio(formObj['group_2'])); alert(getSelectedRadio(formObj['group_3'])); alert(getSelectedRadio(formObj['group_4'])); alert(getSelectedRadio(formObj['group_5'])); alert(getSelectedRadio(formObj['group_6'])); alert(getSelectedRadio(formObj['group_7'])); } In addition to modifying the radio group namee, the button trigger would be changed as follows onClick="proceed(this.form);" -
How to automatically fill a value once another has been selected
Psycho replied to shadiadiph's topic in Javascript Help
Well the "value" of those select elements is the id. The text between the OPTION tags is just the text/label associated with the value. But,since you know the ID you can simply query the database for the email address. In fact, if this page will be externally accessible I would suggest removing the actual email address from the page entirely - otherwise spambots will pick them up and innundate those email boxes. -
No disrespect Mchl, but using Mod Rewrite to have a particular js file (or all js files for that matter) is a bit overkill. Just making those external files as PHP files (or whatever extension you want to use that is already processed by the server) makes more sense to me. If you were ever to migrate the pages to a new server, that is one thing that would probably get misseed. I have been on too many late night calls during server migrations because of issues like that. My opinion is to keep it simple. In fact, I'd probably name the file something like file.js.php just so it is evident that it is an external js file that is processed by php.
-
I would make one last suggestion. The javascript code that is getting produced will have the variables set twice (assuming the values are sent on the query string). Just create the javascript variables once. Note: you can just use empty() for the test. If the variable is not set, empty() will return true same as if it was set and had no value. <?php echo "var aff = '" . ((empty($_GET['aff'])) ? 'fr' : $_GET['aff']) . "';\n"; echo "var lang = '" . ((empty($_GET['lang'])) ? '1' : $_GET['lang']) . "';\n"; echo "var cpop = '" . ((empty($_GET['cpop'])) ? '10' : $_GET['cpop']) . "';\n"; echo "var size = '" . ((empty($_GET['size'])) ? 'C0C0C0' : $_GET['size']) . "';\n"; echo "var cclock = '" . ((empty($_GET['cclock'])) ? 'CC0000' : $_GET['cclock']) . "';\n"; ?>
-
It all depends on how fancy you want to get. I've done an auto-complete in the past for a text field - so it only showed the first match in the list for what you had currently typed. If youwant to show all the matches then it gets more complex as you need to have a CSS menu to show those options. Probably the best approach would be to use a free resource such as this from the Yahoo! UI Library: http://developer.yahoo.com/yui/autocomplete/ Of course it will take some time to read the documentation in order to implement. But, it seems to be a slick implementation. Here's an example page: http://developer.yahoo.com/yui/examples/autocomplete/ac_basic_array.html
-
How to automatically fill a value once another has been selected
Psycho replied to shadiadiph's topic in Javascript Help
As my sig states: As long as we got it worked out, that's all that matters! -
PHP is seeing them as strings. Convert them to floats using float() during the extraction process. http://us2.php.net/floatval
-
Mchl, I don't think that will work in the way the OP wants. It will return the vars for the parent page, not the included JS file. Waffle72, The Google sample youposted is probably doing something similar to what I just proposed. It is calling a page with server-side code that generates the appropriate javascript. Are you hosting the external javascript file? If so, just do as I showed above. It doesn't matter if the calling page is on a server that supports PHP or not.
-
if ( !document.myform.Agent_TID.value.match(/^\d{6}$/) ) { alert ( "Please fill in the 'Agent TID' box." ); valid = false; } However, I would also suggest you detemine all the errors and then display the list to the user - it's much less obtrusive. Example function validate_form ( ) { errors = new Array(); if ( !document.myform.Agent_TID.value.match(/^\d{6}$/) ) { errors[errors.length] = " - Please fill in the 'Agent TID' box."; } if ( ( !document.myform.radio[0].checked ) && ( !document.myform.radio[1].checked ) ) { errors[errors.length] = " - Please choose a Province: AB or BC"; } if ( document.myform.notes.value == "" ) { errors[errors.length] = "Please add Notes:\n STN and Details required if a tracking option is selected!"; } if ( document.myform.resolutioncomments.value == "" ) { errors[errors.length] = " - Resolution Comments can not be blank."; } if ( document.myform.country.selectedIndex == 0 ) { errors[errors.length] = " - You must select a country."; } if ( document.myform.state.selectedIndex == 0 ) { errors[errors.length] = " - You must select a state."; } if ( document.myform.city.selectedIndex == 0 ) { errors[errors.length] = " - You must select a city."; } if (!errors.length) { return true; } message = 'The following errors occured:\n\n'; for (i=0; i<errors.length; i++) { message += String(i+1) + errors[i] + '\n'; } alert(message); return false; }
-
Well, if you are also using a server-side implementatin (such as PHP) there is a very simple solution. Create your external js files as PHP files (or whatever server-side language you are using). So, let's say this was your (test2.js) file where you were trying to alert() the values on the URL string used to call the file alert(aff); alert(lang); alert(cpop); alert(size); I left out the code I would have used to grab the variables from the URL because, as you stated, js can't access those values - only the ones from the parent page. So, change that external file to a PHP page such as this: <?php echo "var aff = '{$_GET['aff']}'\n"; echo "var lang = '{$_GET['lang']}'\n"; echo "var cpop = '{$_GET['cpop']}'\n"; echo "var size = '{$_GET['size']}'\n"; ?> alert(aff); alert(lang); alert(cpop); alert(size); Then, of course, change the src attribute inthe javascript call to have the php extension.
-
Whats the best way to encrypt entire javascript scripts?
Psycho replied to thecard's topic in Javascript Help
For what purpose? To keep someone else from stealing your code? No matter what you do, if the code is readable to the browser then it can be reversed by anyone willing to take a little time to do so. So, what's the point? Besides, haven't you ever benefitted by reusing other's code whether it is using a free script or examing the code on a page to figure out how they did something? But, since you ask,,, There are several ways to obfuscate your code. How you do it may depend on the purpose and the use. As long as you keep the original source code in a readable state you can use a free resource such as this: http://www.javascriptobfuscator.com/Default.aspx Or you can get an application that maintains the original source and generates the obfuscated code when you need it, such as this: http://www.javascript-source.com/javascript-obfuscator.html But, I would not guarantee that any of the obfuscation methods would not make the code unreadable by some browsers. Again, I think it is waste of time, but it's your time. -
How to automatically fill a value once another has been selected
Psycho replied to shadiadiph's topic in Javascript Help
OK, there was a simple typo in your code. I'm not sure of eveything you've been changing, but if you go back to the "full page code" you posted in Reply #8, you just need to make one small change. On line 21 change this <script type="text/javascript">> To this <script type="text/javascript"> Note there was an extra closing bracket '>'. That was the problem. -
How to automatically fill a value once another has been selected
Psycho replied to shadiadiph's topic in Javascript Help
Um, ok. A little punctuation would really help. 1) I also asked "... AND post the HTML output of that code ..." The error you posted is only helpful if I can see the HTML code. Line 109 means nothing if I can't see where 109 is inthe HTML output. 2) You asked about $aid (which would be a PHP variable, and that is not in the code I posted. There is the Select field with the name of "aid", but that was in the code you posted, so I kept it that way. I don't see any obvious error inthe PHP code. So post the HTML output (or provide a link to the page) and I can help furhter. -
Then you definitely want AJAX.
-
Simple, don't use header(). Instead, use an include().
-
Or as an alternative to AJAX you could use cookies. But, it all depends on what you mean by "save".
-
[SOLVED] radio buttons coming out of my ears
Psycho replied to cleary1981's topic in Javascript Help
Post the processed (i.e. HTML) code. JavaScript is run client-side. We don't have your database to run aganst in order to create the client side code. But, a quick review of your code seems to indicate some problems in the construction. There is content being printed to the page in the PHP code at the top, but then after all of that is the opening HTML tag. I'm not sure what you are trying to accomplish after reading your comments and looking at the code. Posting the HTML output - or even a mock-up of a page - would help greatly. -
There's no form. The onchange event is onchange="reload(this.form)" but there are no form tags, thus there is no form. Although, having a properly formatted page (i.e. adding the form tags) is important, there is no reason to call the function with the form object only to then reference the field that called the function. Just pass the field object. Plus, even though that script will work (in some browsers), it is not correct. var param=form.client_id.options[form.client_id.options.selectedIndex].value; Options do not have selected indexes, but the select object does. The correct format would be var param=form.client_id.options[form.client_id.selectedIndex].value; However, as I stated above, I would not use the form object, only the field object.: <html> <head> <script type="text/javascript"> function reload(clientObj) { var param = clientObj.options[clientObj.selectedIndex].value; self.location = 'newaddjob.php?param=' + param ; } </script> </head> <body> <form> <select name="client_id" onchange="reload(this)" tabindex="2"> <option value='a'>A</option><option value='b'>B</option><option value='c'>C</option><option value='d'>D</option> <option value='e'>E</option><option value='f'>F</option><option value='g'>G</option><option value='h'>H</option> <option value='i'>I</option><option value='j'>J</option><option value='k'>K</option><option value='l'>L</option> <option value='m'>M</option><option value='n'>N</option><option value='o'>O</option><option value='p'>P</option> <option value='q'>Q</option><option value='r'>R</option><option value='s'>S</option><option value='t'>T</option> <option value='u'>U</option><option value='v'>V</option><option value='w'>W</option><option value='x'>X</option> <option value='y'>Y</option><option value='z'>Z</option><option value='num'>0-9</option><option value='all'>Show all</option> </select> <a title="Client record associated with this job."><?php $byrndb->client_list(3,'',''); ?></a> </form> </body> <html>
-
How to automatically fill a value once another has been selected
Psycho replied to shadiadiph's topic in Javascript Help
I don't have your database and data to test against. The code orked for me in a mock page I put together. If there is an error on a particular line it would be helpful for you to provide the code arount that particular line (and indicating the exact line). Where does $aid appear in the code? As for the nameID variables, I'm not sure what the confusion is. nameID is the javascript variable that is set according to the value of the select field (which is generated from the PHP variable $row['intNameID']. As for $nameid, that variable appeared in your original code. But, the code provided didn't show how/where it was set. I assumed it was set via a POST or GET variable in order to set one of the Select field options as th4e default selected value. For further help, please provide the current PHP code AND post the HTML output of that code and the errors you are getting.