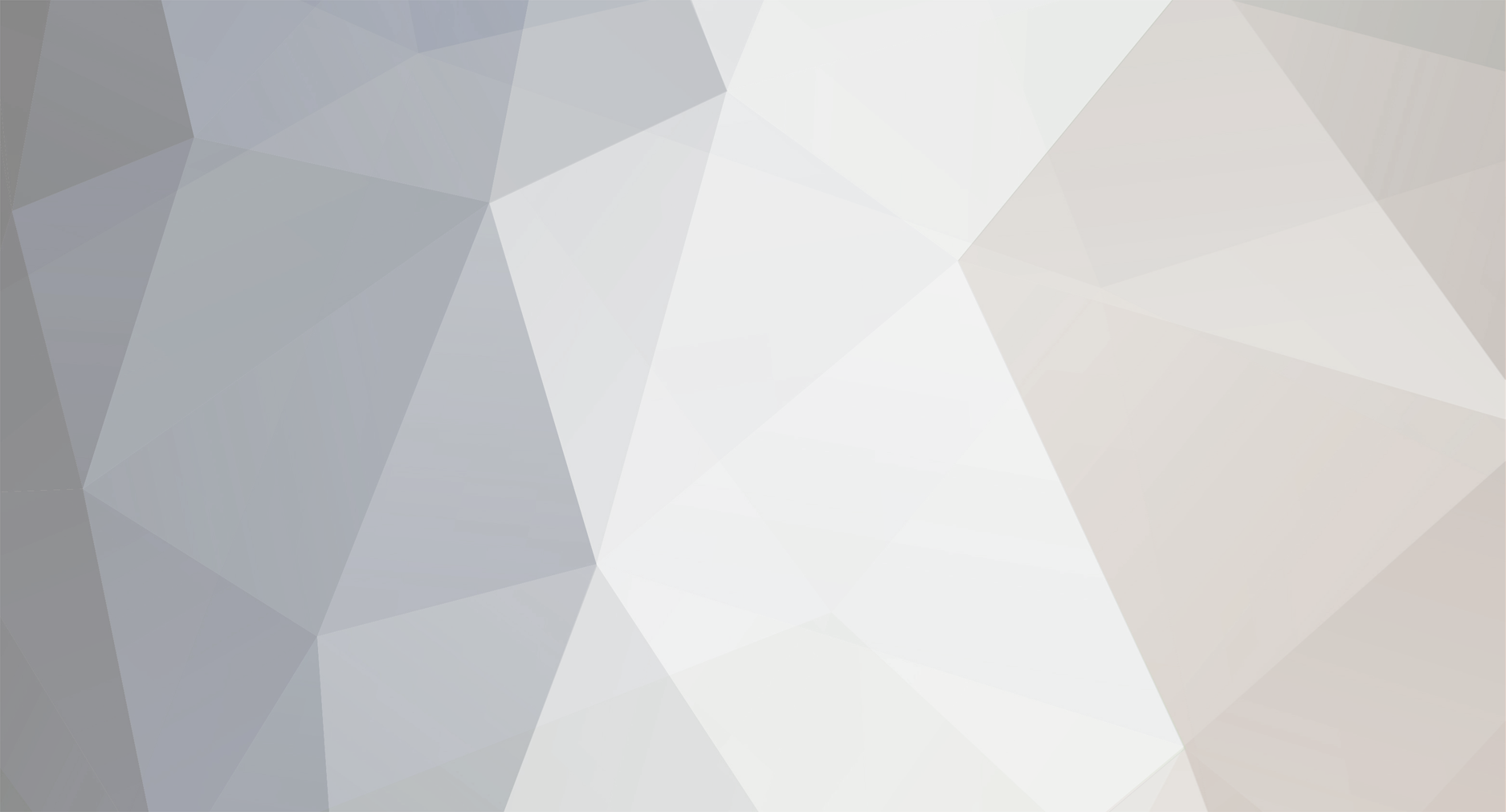
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
A couple things first - use consistent naming of your database fields. If you have a primary key called 'YearID', then use that same name when using that as a Foreign Key in a different table. That allows you to JOIN tables using the USING() function. And, byt the way, based upon what you are asking the people table is irrelevant to the problem. Since I decided to create the tables to test this, I took the liberty to rename the fields as follows: lpro_people: PersonID, PersonName lpro_years: YearID, YearName lpro_registrations: RegistrationID, PersonID, YearID You can solve this problem in several ways, here are two 1) Do a select on the years table and do a LEFT JOIN on the registration table using the YearID and only records for the specified person. This will create a result set with ALL years included, but where there is no corresponding record in the registration table, those fields would be NULL. So, just add a condition in the WHERE clause to only include those records with NULL for one of the registration fields. SELECTyr.YearName, yr.YearID FROM lpro_years AS yr LEFT JOIN lpro_registrations AS rg ON yr.YearID = rg.YearID AND PersonID = $personID WHERE PersonID IS NULL 2) Do a select query on the years table and add a WHERE condition looking for year IDs that are NOT in the list of year ids of the registration records for the person SELECT yr.YearName, yr.YearID FROM lpro_years AS yr WHERE YearID NOT IN (SELECT YearID FROM lpro_registrations WHERE PersonID = 1)
-
Yes, that will do something. That will return the field back to the "default" display style. I believe it would just override any in-line applied styles. So, if there are any style properties applied through a style sheet or the head of the document then those would get applied.
-
Simpe math. I assume you have a variable called $page (or something like it) $startLink = CEIL($page / 10); for($link=$startLink; $link <=$startLink+10; $link++) { //Insert code to create the links using $link }
-
My guess would be that you don't have any functions to hide the textarea.
-
As ,josh already stated there is no way to do that directly with any reasonable certainty. A web-page is a stateless type of technology. Even if you implemented javascript, people can turn it off, browsers crash, etc. You have to understand that you can't force the client browser to send anything and figure out how to work around that. I don't know what that piece of information you are trying to save represents. Does it change while the user is navigating the site? If not, can't you save that when the user logs on? But, assuming that value does change, I can think of at least one relatively simple workaround. When the user logs on save a tentative record to the table (e.g. create a new column called 'confirmed' where the default is 0 and another column for the session ID). Then if you need to update the value while the user is logged in use the session ID to do that. Then you can 'confirm' that record when the user logs off. If the user forgets to log off you can do a couple things: 1) The next time the user logs on, see if there are any previous unconfirmed records for the user and confirm them. However, records can remain unconfirmed until the user logs in again which could be week or days. 2) If the above will not meet your needs then create a scheduled job (i.e. CRON Job) that will run every 20 minutes (or whatever will meet your needs) and check for any unconfirmed records that do not have an active session and change them to confirmed. That's just an off the cuff suggestion, but that should give you some avenues to build something to meet your needs.
-
Applying javascript to check file size and extension
Psycho replied to nanie's topic in Javascript Help
Also, I just noticed you are calling the function using an onchange event on the upload fields. So, there is no need to have the function iterate through all of the input fields. Just pass the field object to the function since you know which field was changed. Also, the logic to check the file is off. If any ONE condition is false it triggers the error message. So, if the file has the right extension then one of the conditions will be false and the image passes - regardless of the size. It need to test the extension and the size separate as an OR condition. Lastly, the conversion to MB is wrong - there's an extra 0 at the end. This seems to work. function checkFile(fieldObj) { var FileName = fieldObj.value; var FileExt = FileName.substr(FileName.lastIndexOf('.')+1); var FileSize = fieldObj.files[0].size; var FileSizeMB = (FileSize/10485760).toFixed(2); if ( (FileExt != "pdf" && FileExt != "doc" && FileExt != "docx") || FileSize>10485760) { var error = "File type : "+ FileExt+"\n\n"; error += "Size: " + FileSizeMB + " MB \n\n"; error += "Please make sure your file is in pdf or doc format and less than 10 MB.\n\n"; alert(error); return false; } return true; } <input type="file" name="confirm" id="confirm" onchange="checkFile(this)"> <input type="file" name="approveletter" id="approveletter" onchange="checkFile(this)"> -
Applying javascript to check file size and extension
Psycho replied to nanie's topic in Javascript Help
You can name the upload fields for multiple files as an array - but you didn't do that. You named them "file", not "file[]". But there is no reason you need to do that. If one upload is for an approval letter, then give that fiel a representative name. The other people may have been confusing the fact the that uploads and the details will be in an array regardless - i.e. the $_FILES array. But, you can have multiple fields with different names and they will be handled within that global array. -
Applying javascript to check file size and extension
Psycho replied to nanie's topic in Javascript Help
Well, both fields have the same name. that may not explain why the JavaScript isn't doing what you want, but you would only ever get one file in your uploads. In your function the second if() condition belongs inside the first condition's code block. Why would you be checking the extension on the file if the first condition was false. A better alternative is to check if the type is NOT 'file' and use 'continue' to skip to the next item in the for() loop. In any event, you should do this validation on the server-side. JavaScript can only check the extension, but you can check the real file type on the server using PHP. -
Can you provide a sample of the wartermark image? FYI: Based upon the two links provided, it seems you used two different images for the watermark and you received two different results (one is partially transparent and the other is not transparent at all). Since the same code was changed, my initial reaction is that the difference is caused by the different images.
-
Brute-forcing with a recursive function a chess knight puzzle
Psycho replied to AnotherLife's topic in PHP Coding Help
OK, here is a corrected version. I also added a better output of the success value to make it easier to validate the results. It shows each position and the change from the last position: either 1, 2 or 2, 1. Note, the function is still only looking for 62 valid positions - but there are 64 positions on an 8 x 8 board. It took about 20 seconds to run for 62 positions. Increasing to 63 to calculate a success caused a timeout at 60 seconds. So, going to 64 will probably take good while to run. I'll let you verify the results <?php function getvalidmoves($occupiedPositions) { //Check if max moves have already been reached (should be 64) if (count($occupiedPositions) == 62) { echo "SUCCESS !!!!!!!<br>\n"; $lastPos = false; foreach($occupiedPositions as $idx => $pos) { echo "{$idx}: Position: $pos[0], $pos[1]"; if($lastPos) { echo ", Movement: " . abs($pos[0]-$lastPos[0]) . ", " . abs($pos[1]-$lastPos[1]); } echo "<br>\n"; $lastPos = $pos; } //echo "<pre>" . print_r ($occupiedPositions, 1) . "</pre>"; exit; } //Determine the current position $curPosition = end($occupiedPositions); //Determine 'possible' next positions $nextPositions = array( array($curPosition[0]+1, $curPosition[1]-2), array($curPosition[0]+2, $curPosition[1]-1), array($curPosition[0]+2, $curPosition[1]+1), array($curPosition[0]+1, $curPosition[1]+2), array($curPosition[0]-1, $curPosition[1]+2), array($curPosition[0]-2, $curPosition[1]+1), array($curPosition[0]-1, $curPosition[1]-2), array($curPosition[0]-2, $curPosition[1]-1) ); //Remove invalid next positions foreach ($nextPositions as $key => $pos) { if (($pos[0] < 0) || ($pos[0] > 7) || ($pos[1] < 0) || ($pos[1] > 7) || in_array($pos, $occupiedPositions)) { unset($nextPositions[$key]); } } //If no valid positions exist, abandon this thread if (!count($nextPositions)) { echo "FAILURE after " . count($occupiedPositions)," moves<br>\n"; return; } //Call function again for each valid next position //starting from the current occupied positions foreach ($nextPositions as $key => $nextPos) { $newPositions = $occupiedPositions; $newPositions[] = $nextPos; getvalidmoves($newPositions); } return; } echo "\n - Knight puzzle brute forcer by VGA -<br><br>\n"; $startPos = array(0,0); $occupiedPositions[] = $startPos; $validMoves = getvalidmoves($occupiedPositions); echo "<pre>" . print_r ($validMoves, 1) . "</pre>"; ?> Note, I moved some logic around to be more efficient. For example, I check if max moves has been reached before trying to determine any next moves. -
Brute-forcing with a recursive function a chess knight puzzle
Psycho replied to AnotherLife's topic in PHP Coding Help
Aha! Here's the problem foreach ($validpositions as $key => $pos) { array_push($occupiedpositions,$currpos); $currpos = $pos; getvalidmoves($currpos, $occupiedpositions); } In that loop you are appening $currpos to the end of $occupiedpositions. On the first iteration of that loop $currpos is the value that was passed to the function. Then on the subsequent iterations it is appending the previous "valid moves". There are a couple problems with that. One is that the valid moves are only valid if the current last position. Instead, you are adding valid postion2 after valid position1 - which may not be a valid move from position1. Plus, you are never adding the last valid position! So, let's say your occupied positions are A, B, & C. And you pass current position as D to the function. It then calculates that the valid positions from D are E, F, & G. You should end up with three new arrays as: A, B, C, D, E A, B, C, D, F A, B, C, D, G Instead you are getting A, B, C, D, E A, B, C, D, E, F A, B, C, D, E, F, G Instead of adding the values to the same array, you need to create a new array containing $occupiedpositions (as passed to the function) and just the next valid position. To do this, it would be easiest to have the current position already included. -
Brute-forcing with a recursive function a chess knight puzzle
Psycho replied to AnotherLife's topic in PHP Coding Help
I tried adding some debugging and wasn't able to see why that problem was occurring either. It's not so much that it is a recursive function as much as it is exponential. Each execution triggers up 8 separate new executions. So, it increases exponentially. It's hard to say if that is due to a bug or not because, as I stated, each execution increases exponentially. Although, I do think the function could be improved. For instance, why do you need to pass $currpos to the function. Start with an array with the first position and pass it is as the $occupiedpositions array. You can then determine $currpos by getting last value in the array. I don't know where the problem is, but reducing the number of variables and complexity could only help find the problem. -
Yes, but it won't be secure. Emails are not encrypted. So someone could potentially grab that data as it travels through the servers on it's way from server sending the mail to the receiving mail server. So, your options, as I see it, are to: 1) Use a third party email encryption function or build your own. But, that means your client will need to have something to decrypt the emails when they are received. 2) Keep the messages on the server and only send a notification email. Then provide a way for the user to retrieve the messages off the server. The notification email can have a link directly to the message and provide a login screen (if the user isn't already logged in). I think this would be the least intrusive process. But, you will need to ensure the site is being run through HTTPS, otherwise the data being viewed is being sent over the internet unsecured when the user views the messages.
-
What trq is getting at is that whatever you think you are preventing - you aren't. It is very, very simple to override any JavaScript tricks you may put in. I assume you are trying to prevent users from copying the images. You do realize that as soon as a user sees the image in your page that the image is already downloaded to their PC, right? Also, users can easily disable JavaScript. And there are utilities that will automatically copy images from web pages for you. And, if all else fails, the user can take a screenshot of their screen and crop the picture out. Using JavaScript is a huge waste of time and, as trq stated, it only annoys users. If the user happened to be over an image when they need that context menu (e.g. print) they would be frustrated. The one simple trick I've seen that I would advise, is to put a transparent gif over the image. Then, when the user tries to copy the image, they will just get the gif. Of course, once the user realizes this, they can easily get the real image using any of the previously stated methods above. If you want to put your images on the net - then you have to assume people will copy them. The only thing you can really do is put a watermark on the images to prevent people from passing off the images as their own.
- 4 replies
-
- disable right click
- right click
-
(and 2 more)
Tagged with:
-
Yeah, I just saw the problem with the container for the code block and corrected that without seeing any of the other problems.
-
There should not be a semi-colon at the end of the foreach() condition. foreach ($candy as $type => $specific); The code that the foreach should run against should either be encloised in curly braces or use the alternative syntax of starting with a colon and ending with an end statement foreach ($candy as $type => $specific) { if $type == 'chocolate'{ echo $specific; } elseif { echo $type . ' ' . $specific; } else { echo 'Unknown candy'; } } foreach ($candy as $type => $specific): if $type == 'chocolate'{ echo $specific; } elseif { echo $type . ' ' . $specific; } else { echo 'Unknown candy'; } endforeach;
-
PHP Novice, trying to do file uploads on a form.
Psycho replied to mstabosz's topic in PHP Coding Help
Not without creating some elaborate JavaScript/CSS to hide the actual input field and create a separate display element that interacts with the hidden field. There are several JQuery implementations that create such objects, but not one for a file input field. So you would have to build your own, which is not a good idea. For one, you would have to test the functionality in all the different browsers and in the different versions of those browsers (e.g. IE 10, 9, 8, etc.). Something that works in some browsers could break in others. Without testing ALL the browsers and versions, you run the risk of making the form completely unusable to some users. Second, a user on FireFox may like and be accustomed to the look of the file input field in that browser. Basically, there is very little upside to trying to override the default layout. Applying some style properties is fine, but those will not change the differences you mentioned. Best to let the browser do what it was programmed to do. -
Brute-forcing with a recursive function a chess knight puzzle
Psycho replied to AnotherLife's topic in PHP Coding Help
Hmm . . . yes, that's odd. I don't see anything that jumps out at me. -
Brute-forcing with a recursive function a chess knight puzzle
Psycho replied to AnotherLife's topic in PHP Coding Help
Not sure what you are saying exactly. How do you know the moves are wrong? I can verify that the success result does not duplicate any positions. But, I noticed that you are stopping the process too early! On an 8 x 8 board there are 64 total positions and you are exiting the process after only 62 positions are reached. But, just increasing the number to 63 caused the script to timeout after 60 seconds and over 379,000 different attempts. Increasing it to 64 would take exponentially longer. -
Brute-forcing with a recursive function a chess knight puzzle
Psycho replied to AnotherLife's topic in PHP Coding Help
What do you mean by " . . . after some moves it sometimes chooses the wrong square to move to . . . "? Are you saying it chooses a square not on the board or that it chooses a square that has already been used? I ran the code and the results were that there were a large number of "Failures" after x number of moves and then a "Success" at the end. Don't know how you are seeing that some are choosing the wrong positions. -
Well, what do you mean by "secure" Emailing? I would think you would start with using https to make sure communications between the client and server are secure. But, as far as sending a secure email - I don't know what your expectations are. The only way to send an email securely (prevent others from being able to obtain the content of the message) is to encrypt it. Which means the recipient will need some way to decrypt it. For many businesses that require very tight control of such messages I see them using portals. The recipient is sent an email with a link to the portal. From there the user must log in to see the message. So, basically the message is never truly sent via email - only a notification that there is a message.
-
PHP Novice, trying to do file uploads on a form.
Psycho replied to mstabosz's topic in PHP Coding Help
1. Your new form does not have the proper encoding type set for a file upload. E.g. enctype="multipart/form-data" 2. Your if() statement is checking to see if an index within the $_FILES array is set. You should verify 1) what is contained in the $_FILES array and that 2) the dynamic index value ($myUploader->getUploaderName()) contains what you think it should. 3. The class doesn't make sense. You are initiating the class during the process of verifying the data sent in the form submission. But, when you instantiate the class it automatically calls the method makeUploaderBlock(). That makes no sense since you are using the class in this instance to verify the form submission. You should never have functions/methods that send output to the browser. They should return the output for the calling code to use. EDIT: 4. Don't create the field names such as 'uploader1', 'uploader2', etc. Instead create sub-arrays. <input type="text" name="uploader[1]" /> <input type="text" name="uploader[2]" /> <input type="text" name="uploader[3]" /> -
Impossible to say without seeing the code that creates the checkboxes. Is this only an issue when more than 250 are checked or when there are more than 250 period?
-
Well, it really depends on the employer. Some employers may only be concerned if the candidate has experience with the specific language they are looking for. But many/most are really concerned about their "technical" abilities. While there are many programming languages the variances in actual syntax aren't that great. C# & PHP aren't that different in syntax. It's just a matter of knowing what functions the languages have and what they can do. Most employers are interested in the candidates ability to convert logic into code. Learning a new language should not be a significant obstacle for a good programmer. However, there are some which have significant syntax differences. For example, I used to program in PostScript. That language uses a stack based method which is a very different way of programming. But, most of the languages are mostly similar to C. In fact, thinking on it now, I would suggest you start with JavaScript (not Java). That is also C based and is a necessity for anyone developing web based applications.
-
@Realistic Just in case you didn't follow Barand's response. The array asort function will sort the original variable - no need to assign the result to a variable. A couple other things. No need to define $sizeArray as an empty array and then define it again as an array with values. You only need to define an empty array first if you need to use the variable as an array in some way. For example, if you add values to the array using $sizeArray[] = 'foo'; $product_length = 2; $product_width = 5; $product_height = 3; // work out volume. $sizeArray = array($product_length, $product_width, $product_height); echo "Presort:<br><pre>" . print_r($sizeArray, 1) . "</pre>"; echo "<br><br><br>\n"; asort($sizeArray, SORT_NUMERIC); echo "Sorted:<br><pre>" . print_r($sizeArray, 1) . "</pre>";