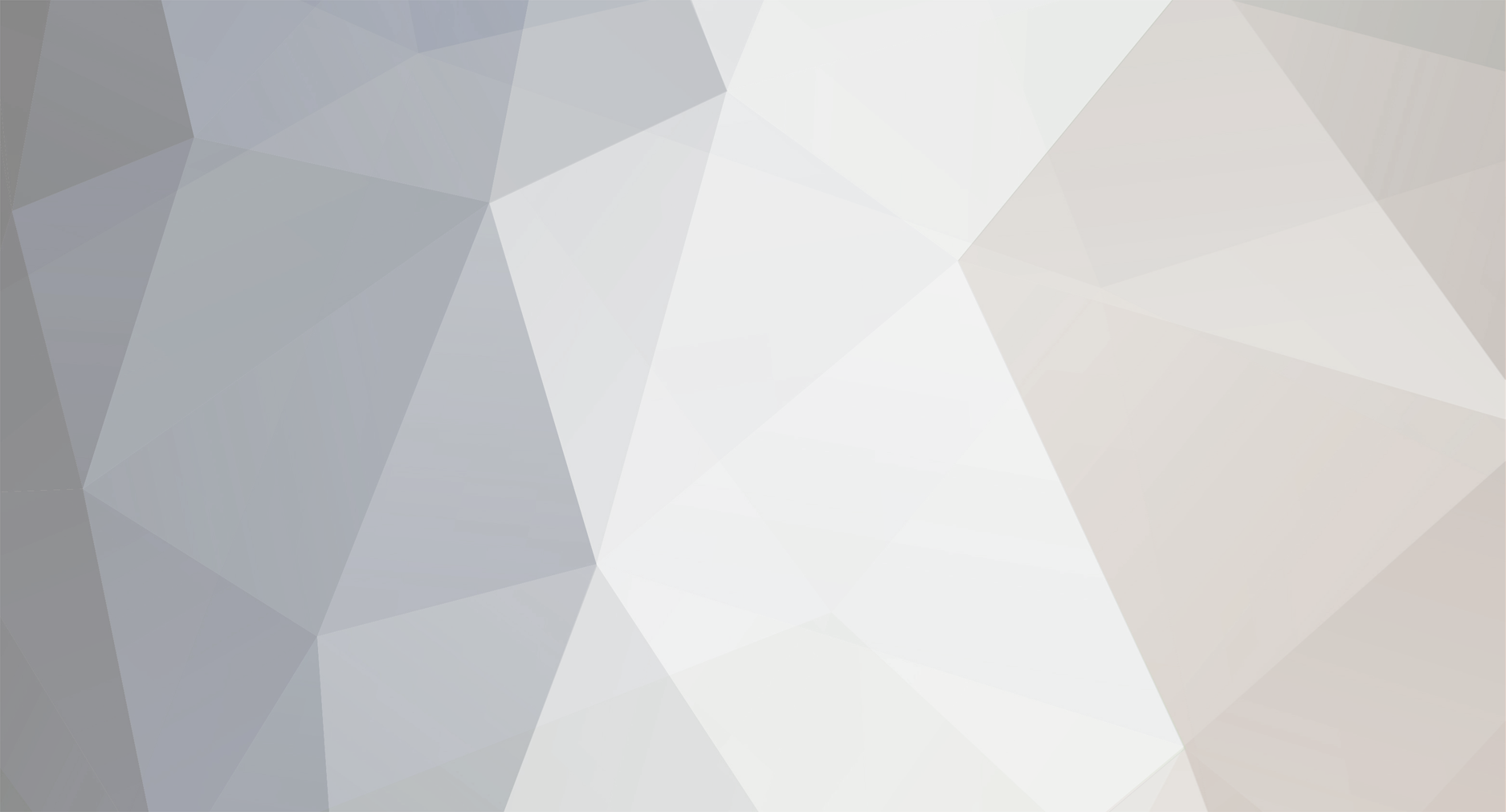
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Just as you can name a field in your output SELECT COUNT(id) AS id_count You can also name a table in the query SELECT * FROM table1 AS tb1 When only querying one table this really has no value. But, when you are querying from multiple tables you can use them to reference the different fields from those two tables. Example (a is a reference to the author table and b is a reference to the books table) SELECT a.author_name, b.book_name FROM authors AS a JOIN books ON a.author_id = b.author_id ORDER BY a.author_name This really becomes helpful when you have fields with the same name between tables and you need to reference exactly which one you want.
-
Don't run queries in loops. based upon your sample query you just want a count of each column that has values. Try this SELECT COUNT(Number1) AS CT1, COUNT(Number2) AS CT2, COUNT(Number3) AS CT3, COUNT(Number4) AS CT4, COUNT(Number5) AS CT5, COUNT(Number6) AS CT6 FROM Draws
-
The following is a shot in the dark since I don't know your table structures //Run ONE query to get the results for ALL stores $query = "SELECT s.store_id, s.store_name, COUNT(cp.store_id) as sales_count, SUM(cp.amount) AS total_sales FROM stores AS s LEFT JOIN checkout_page AS cp ON s.store_id = cp.store_id AND DATE(cp.checkout_date) > CURDATE() - INTERVAL 7 DAY GROUP BY s.store_id ORDER BY s.store_id, cp.checkout_date" $result = mysql_query($query); $sales_report = =''; while($row = mysql_fetch_assoc($result)) { $sales_report .= "Store: ({$row['store_id']}) {$row['store_name']}, Sales: {$row['sales_count']}, Amount: \${$row['total_sales']}<br/>"; }//end of while loop //Send email of COMPLETE sales reports mail("myemail@myemail.com","Store Sales Reports","$sales_reports");
-
First off - NEVER run queries in loops. You should do ONE query using a JOIN. Show the query you are running to get the $store_query result. Then we can show the proper way to do this. EDIT: Also, are you only wanting the TOTAL sales for each store? I see you are only outputting results if there were less than 7 results. So, I assume you did that to prevent a lot of records being in the output.
-
Well, it would update any row where the conditions sent='0' and dateofenquiry<='$date' are both true. Looking at your example data, rows with id 1 and 2 both meet that criteria and would be updated to have sent = 1. The row with ID = 3 does not meet that criteria - but the sent value for that row is already a 1. The issue is this $date = ('Y-m-d 00:00:00'); date_sub($date, date_interval_create_from_date_string('14 days')); On the second line, the function date_sub() returns a datetime object. It is not modifying the value of $date. Plus, you can't use a datetime object in your query. Try $date = date('Y-m-d 00:00:00', strtotime('-14 days'));
-
There is no easy solution here because the number of parameters is quite large. But, let's say you want to find a flight from one location to another. I think - at the very least - you need to determine the maximum amount of stops. For each potential stop you will want to create separate queries and then merge the results together using UNION. So, one query for a nonstop (if one exists). A second query for flights with one stop. A third for two stops, etc. Then for each query with one or more stops you would JOIN the table on itself using the destination of the first flight and the origination of the second flight PLUS, ensuring the flight end time of the first is before the start of the second flight. EXAMPLE 1 (nonstop flights) SELECT * FROM tbl_flight_details WHERE origin = '$origin' AND destination = '$destination' EXAMPLE 2 (one-stop flights) SELECT * FROM tbl_flight_details AS f1 JOIN tbl_flight_details AS f2 ON f1.destination = f2.origin AND f1.date = f2.date AND f1.arrivalTime < f2.departureTime WHERE f1.origin = '$origin' AND f2.destination = '$destination'
-
You are using the functions filemtime() and date() backwards. You need to use the result of fimemtime() within the date function. Don't combine functions on one line untill you know them well. Also, don't use a for() loop for an array - use a foreach loop. Try this: // print 'em echo "<table border='1' cellpadding='5' cellspacing='0' class='whitelinks'>\n"; echo "<tr>\n"; echo " <th><a style='float:left;' href='/joe' colspan='4'>Go Back Home</a></th>\n"; echo "</tr>\n"; echo "<tr>\n"; echo " <th>Filename</th><th>Filetype</th><th>Filesize</th><th>Modified</th>\n"; echo "</tr>\n"; // loop through the array of files and print them all foreach($dirArray as $filePath) { if (substr($filePath, 0, 1) == ".") { // skip hidden files continue; } $fileType = filetype($filePath); $fileSize = filesize($filePath); $fileTime = date('F d Y H:i:s', filemtime($filePath)); echo "<tr>\n"; echo " <td><a href=\"{$filePath}\">{$filePath}</a></td>\n"; echo " <td>{$fileType}</td>\n"; echo " <td>$fileSize</td>\n"; echo " <td>{$fileTime}</td>\n"; echo "</tr>\n"; } echo "</table>\n";
-
ginerjm beat me to the explanation which I was writing code: $query = "SELECT * FROM Cars ORDER BY Type_a, Type_b, Name" $result = mysql_query($query )or die(mysql_error()); $currentTypeA = false; $currentTypeB = false; while ($row = mysql_fetch_array($result)) { //Output first level - if different than last if($currentTypeA != $row['Type_a']) { //Output first level $currentTypeA = $row['Type_a']; echo "<h1>{$currentTypeA}</h1><br>\n" } //Output second level - if different than last if($currentTypeB != $row['Type_b']) { //Output second level $currentTypeA = $row['Type_b']; echo "<h2>{$currentTypeB}</h2><br>\n" } //Ouput the name echo "{$row['Name']}<br>" }
-
Right, the problem was in the usage of mysql_result(). Per the manual, that function (which is deprecated) takes two parameters. The first is the result resource, which you provided. The second parameter is the 'row' which you did not provide. You provided strings corresponding to the column names. But, the manual states: EDIT: Since you are only expecting 1 row, no need to use a while() loop. if(mysql_numrows($result1)) { $row = mysql_fetch_assoc($result1); $column1=$row['column1']; $column2=$row['column2']; } else { //Error condition }
-
First, change your form so all the 'answers' are in a sub-key of the $_POST array, e.g. $_POST['answers'], rather than putting them in the root of $_POST. This way if there was any other information included in the POST data (for example a user ID) it will be logically separated from the answers. Then, you can easily get the list of the questions and the answers. <?php //Get the POSTed answers $answersAry = $_POST['answers']; //Get the array of question IDs $questionIDsAry = array_keys($_POST['answers']); //Convert all ID values to integers (make safe for SQL query) $questionIDsAry = array_map('intval', $questionIDsAry); //Remove invalid values $questionIDsAry = array_filter($questionIDsAry); //Convert remaining IDs to comma separated string for use in query $questionIDsSQL = implode(', ', $questionIDsAry); //Create and run query $query = "SELECT id, ans FROM questions WHERE id IN({$questionIDsSQL})"; $result = mysql_query($query); //Iterate through the query results and compare to the POSTed answers $points = 0; while($row = mysql_fetch_assoc($result)) { if($answersAry[$row['id']] == $row['ans']) { $points++; } } echo "Your score: $points"; ?>
-
No, I did not. You are apparently not reading the code correctly. I put comments in, but I will explain it in more detail: //Check if this name is the same as the last if($currentName != $row['name']) { //Name is different, set new value to check $currentName = $row['name']; } else { //name is same as last, redefine name and contact to be empty space $row['Name'] = ' '; $row['Contact'] = ' '; } $table .= "<tr>\n"; $table .= "<td>{$row['Name']}</td>\n"; // 'Name' is always output $table .= "<td>{$row['Contact']}</td>\n"; // 'Contact' is always output $table .= "<td>{$row['Code']}</td>\n"; $table .= "<td>{$row['Type']}</td>\n"; $table .= "<td>{$row['Quantity']}</td>\n"; $table .= "</tr>\n"; So: IF: ($currentName != $row['name']), we reassign $currentName as $row['name']. The values for $row['Name'] and $row['Contact'] remain unchanged for that record. ELSE: The values for $row['Name'] and $row['Contact'] are redefined to be a space characters THEN: No matter what the result of the above If/Else statement we concatenate the table cells for 'Name' and 'Contact' onto the current $table variable: $table .= "<td>{$row['Name']}</td>\n"; $table .= "<td>{$row['Contact']}</td>\n"; So, if this is the first iteration of a new name, the 'Name' and 'Contact' values from the query (which were unchanged) will be used in the output. Otherwise, if it is the same name as the last iteration, the empty space that was assigned to 'Name' and 'Contact' would be used in the output.
-
<?php $query = "SELECT name, contact, code, type, quantity FROM table_name ORDER BY name"; try { $con = new PDO('mysql:host=localhost;dbname=your_db_name', $username, $password); $con->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); $result = $con->prepare($query); $result->execute(); } catch(PDOException $e) { echo $e->getMessage(); } $table = "<table cellpadding='10' cellspacing='0' border='1'>\n"; $table .= "<tr>\n"; $table .= " <th>Name</th><th>Contact</th><th>Code</th><th>Type</th><th>Quanitiy</th>\n"; $table .= "</tr>\n"; $currentName = false; while($row = $result->fetch(PDO::FETCH_ASSOC)) { //Check if this name is the same as the last if($currentName != $row['name']) { //Name is different, set new value to check $currentName = $row['name']; } else { //name is same as last, redefine name and contact to be empty space $row['Name'] = ' '; $row['Contact'] = ' '; } $table .= "<tr>\n"; $table .= "<td>{$row['Name']}</td>\n"; $table .= "<td>{$row['Contact']}</td>\n"; $table .= "<td>{$row['Code']}</td>\n"; $table .= "<td>{$row['Type']}</td>\n"; $table .= "<td>{$row['Quanity']}</td>\n"; $table .= "</tr>\n"; } $table .= "</table>\n"; ?>
-
If you want to use PHP to extract the ID3 info for an MP3, then I would highly suggest using getID3().
-
The problem is in the parameters being used in rand(): $digit .= ($num = $possible_letters[rand(0, strlen($possible_letters))]); $num is being defined as a letter from $possible_letters with an index defined by rand() between 0 and the length of $possible_letters. Think about 1) the index that represents the first letter (0) and 2) what you are defining as the last possible index. It might be easier to visualize with a smaller string. So, let's say you have a string of "ABCDE". That string has a length of 5. If "A" is at position 0, then "B" is at position 1, "C" at position 2, "D" at position 3, and "E" at position 4. So, setting the max index at the length of the string will make it possible to set an index that is 1 greater than the last character (i.e. 5 instead of 4).
-
Well, your code had both of those. I assume it is using the last connection defined. But, no reason to use both. Take one out.
-
Really? That's not the case based upon your code. In your code you are referencing fields from that table as follows: url, name, product, price, and ID. The only one that is the same is 'ID'. Note: "Product" is not the same as "product".
-
In the code I provided, instead of echoing the output to the page, I simply added the content to a variable names $output $output = "<div class=\"item\">\n"; $output .= "<form method=\"post\" action=\"Cart_update.php\">\n"; The reason for this is to make the code more modular and flexible. You should be able to separate the logic (i.e. PHP code) from the presentation (i.e. the HTML). That way you can create a separate file to generate the pagination results from the actual output (just put a single echo). That way you can just include the PHP file to create the pagination results. This reduces the amount of lines of code in any single file making it easier to debug your code and to reuse portions. For example, you have a lot of code for things such as the menu and footer. Those should be taken out of the main page script and put into separate files that you include() in the main script. That way you can include the same files in all your pages and only have to maintain the content in one place. So, thin about when you may want to change the footer content for your whole site. You would want to have to track down dozens of different pages to copy/paste the same content. Just have the content defined once and used many times. Then you would only have to edit one file to update the footer for your entire site. Here is a rewrite of your whole page with the code I provided above. Plus, I also modified the code to create the cart contents. All of the PHP code it at the top of the script. You could take it out and put it into separate files if you wish. Again, I did not test the code since I have not taken the time to set up a database with test data. So, there may be a few typos to fix. <?php session_start(); include_once('includes/config.php'); ###################################################### ###### Start Logic to create pagination results ###### ###################################################### $pageResults = ''; //This is the number of results displayed per page $page_rows = 4; // Connects to your Database mysql_connect("localhost", "secret", "secret") or die(mysql_error()); mysql_select_db("mydatabase") or die(mysql_error()); //Here we count the number of results $query = "SELECT COUNT(*) FROM merchant"; $result = mysql_query($query) or die(mysql_error()); $total_rows = mysql_result($result, 0); //This tells us the page number of our last page $total_pages = ceil($total_rows/$page_rows); //This checks to see if there is a page number. If not, it will set it to page 1 $pagenum = (isset($_GET['pagenum'])) ? intval($_GET['pagenum']) : 1; //this makes sure the page number isn't below one, or more than our maximum pages $pagenum = max(1, $pagenum); $pagenum = min($total_rows, $pagenum); //This sets the limit to get the records for the selected page $limit = ($pagenum - 1) * $page_rows; //This is your query again, the same one... the only difference is we add LIMIT to it $query = "SELECT * FROM merchant ORDER BY ID ASC LIMIT {$limit}, {$page_rows}"; $result = mysql_query($query) or die(mysql_error()); //current URL of the Page. cart_update.php redirects back to this URL $current_url = base64_encode($url="http://".$_SERVER['HTTP_HOST'].$_SERVER['REQUEST_URI']); $results = $mysqli->query("SELECT * FROM merchant $max ORDER BY ID ASC"); //This is where you display your query results while($row = mysql_fetch_assoc($result)) { $pageResults .= "<div class=\"item\">\n"; $pageResults .= "<form method=\"post\" action=\"Cart_update.php\">\n"; $pageResults .= "<div class=\"product-thumb\"><img src=\"{$row['url']}\" width=\"20\" height=\"50\"></div>\n"; $pageResults .= "<div class=\"product-content\"><h3>{$row['name']}</h3>\n"; $pageResults .= " <div class=\"product-desc\">{$row['product']}</div>\n"; $pageResults .= " <div class=\"product-info\">Price {$row['currency']}{$row['price']} <button class=\"add_to_cart\">Add To Cart</button></div>\n"; $pageResults .= "</div>\n"; $pageResults .= "<input type=\"hidden\" name=\"product_code\" value=\"{$row['ID']}\" />\n"; $pageResults .= "<input type=\"hidden\" name=\"type\" value=\"add\" />\n"; $pageResults .= "<input type=\"hidden\" name=\"return_url\" value=\"{$row['current_url']}\" />\n"; $pageResults .= "</form>\n"; $pageResults .= "</div>\n"; } // This shows the user what page they are on, and the total number of pages $pageResults .= " --Page $pagenum of $total_pages-- <p>"; //Create var for pagination URL $pageURL = "{$_SERVER['PHP_SELF']}?pagenum="; // First we check if we are on page one. If we are then we don't need a link to the previous page or the first page so we do nothing. //If we aren't then we generate links to the first page, and to the previous page. if ($pagenum > 1) { $previous = $pagenum-1; $pageResults .= " <a href='{$pageURL}1'> <<-First</a> "; $pageResults .= " <a href='{$pageURL}{$previous}'> <-Previous</a> "; } //Add a spacer $pageResults .= " ---- "; //This does the same as above, only checking if we are on the last page, and then generating the Next and Last links if ($pagenum < $total_pages) { $next = $pagenum+1; $pageResults .= " <a href='{$pageURL}{$next}>Next -></a> "; $pageResults .= " <a href='{$pageURL}{$total_pages}'>Last ->></a> "; } ###################################################### ###### End Logic to create pagination results ###### ###################################################### ###################################################### ###### Start Logic to create cart contents ###### ###################################################### if(isset($_SESSION["products"])) { $cartContents = ''; $total = 0; foreach ($_SESSION["products"] as $cart_itm) { //echo '<li class="cart-itm">'; $cartContents .= '<span class="remove-itm"><a href="Cart_update.php?removep='.$cart_itm["code"].'&return_url='.$current_url.'">−</a></span>'; $cartContents .= '<span class="add-itm"><a href="Cart_update.php?addp='.$cart_itm["code"].'&return_url='.$current_url.'">+</a></span>'; $cartContents .= '<h3>'.$cart_itm["name"].'</h3>'; $cartContents .= '<div class="p-code">P code : '.$cart_itm["code"].'</div>'; $cartContents .= '<div class="p-qty">Qty : '.$cart_itm["qty"].'</div>'; $cartContents .= '<div class="p-price">Price :'.$currency.$cart_itm["price"].'</div>'; // echo '</li>'; $subtotal = ($cart_itm["price"]*$cart_itm["qty"]); $total = ($total + $subtotal); } $cartContents .= '<span class="check-out-txt">'; $cartContents .= '<strong>Total : '.$currency.$total.'</strong> '; $cartContents .= '<a href="View_cart.php"><img src="images/cart.gif" alt="" width="18" height="19" class="cart">Shopping Cart</a>'; $cartContents .= '</span>'; } ###################################################### ###### End Logic to create cart contents ###### ###################################################### ?> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <title>Compare and Choose</title> <meta http-equiv="Content-Type" content="text/html; charset=windows-1251"> <link rel="stylesheet" type="text/css" href="style.css" /> <style type="text/css"> body{ font-family:"Trebuchet MS", Arial, Helvetica, sans-serif;} div#pagination_controls{font-size:21px;} div#pagination_controls > a{ color:#06F; } div#pagination_controls > a:visited{ color:#06F; } </style> <script type="text/javascript"> function transparent(im) { if (!im.transparented && (/\.png/.test(im.src))) { im.transparented = 1; var picture = im.src; var w = im.width; var h = im.height; im.src = "images/spacer.gif"; im.style.filter = "progid:DXImageTransform.Microsoft.AlphaImageLoader(enabled=true, sizingMethod='scale', src='" + picture + "');"; im.width = w; im.height = h; } return "transparent"; } </script> </head> <body> <div id="content"> <div id="header"> <ul class="top_menu"> <li><a href="index.html" class="active">Home</a></li> <li><a href="#">How it works</a></li> <li><a href="#">Travel</a></li> <li><a href="#">Goods</a></li> <li><a href="#">Insurance</a></li> <li><a href="#">Services</a></li> <li><a href="#">Hire</a></li> <li><a href="#">About us</a></li> <li><a href="#">Bookmark</a></li> </ul> <ul class="top_menu"> <li><a href="#">My Account</a></li> <li><a href="#">Track Order</a></li> <li><a href="#">Order</a></li> </ul> <div id="search"> Search<br /> <form action="#"> <select> <option>Category</option> <option>Travel</option> <option>Goods</option> <option>Insurance</option> </select> <select> <option>other</option> <option>Services</option> <option>Hire</option> <option>Bookmark</option> </select> <a href="#"><img src="images/ok.jpg" alt="setalpm" width="44" height="23" /></a> </form> </div> <img src="images/tel.jpg" alt="" width="181" height="13" class="tel" /> </div> <div id="wrapper"> <div id="background"> <div id="left"> <h4 class="title1">All categories</h4> <ul id="list"> <li><a href="#" class="type1">Home</a></li> <li><a href="#" class="type2">How it works</a></li> <li><a href="#" class="type2">Travel</a></li> <li><a href="#" class="type2">Goods</a></li> <li><a href="#" class="type1">Insurance</a></li> <li><a href="#" class="type2">Services</a></li> <li><a href="#" class="type1">Hire</a></li> <li><a href="#" class="type2">About us</a></li> <li> <a href="#" class="type3">Test</a> <ul id="inside"> <li><a href="#">Test</a></li> <li><a href="#">Test</a></li> <li><a href="#">Test</a></li> <li><a href="#">Test</a></li> </ul> </li> <li><a href="#" class="type2">Test</a></li> <li><a href="#" class="type2">Test</a></li> <li><a href="#" class="type1">Test</a></li> <li><a href="#" class="type2">Test</a></li> </ul> <h4 class="title2">Test</h4> <div class="news"> <span>Xmas day</span> <p>Test item to show any promotions that an affilaite might have they can be shown around this area to the customer on specific dates. </p> <a href="#" class="more">read more</a> </div> <div class="news"> <span>17 november</span> <p>A different older promotion to be shown here. </p> <a href="#" class="more">read more</a> </div> <div class="news"> <span>05 november</span> <p>Even older promotions that an affilaite might have they can be shown around this area to the customer on specific dates. </p> <a href="#" class="more">read more</a> </div> <h4 class="title3">Currencies</h4> <div class="currencies"> <select> <option>AUD</option> <option>GBP</option> </select> </div> </div> <div id="center"> <div id="discount"> <img src="images/discount.png" alt="" width="121" height="119" border="0" usemap="#Map" class="transparent" /> <map name="Map"> <area shape="circle" coords="61,57,55" href="#"> </map> </div> <div id="photo"> <img src="images/tpart.png" alt="" width="49" height="23" class="transparent" /><br /> <div class="text"> <p><span>test</span> - test details, this is where we need to show different marketed product options for the compare. </p> <p>Another test<br /> lets make sure we double check everything here for the page layout,<br /> test test test test test<br /> out put test output<br /> plain english, </p> </div> </div> <div id="items"> <div class="item"> <?php echo $pageResults; ?> </div> </div> <div id="right"> <div class="bag"> <h4 class="title2"><img src="images/cart.gif" alt="" width="18" height="19" class="cart" />Shopping Cart</h4> <?php echo $cartContents; ?> <div class="entry"> <form action="#"> Login<br /> <input type="text" /><br /> Password<br /> <input type="text" /><br /> <a href="#" class="forgot">Forgot password</a> </form> </div> <p class="below"><a href="#">Register</a> <a href="#"><img src="images/enter.jpg" alt="" width="52" height="23" /></a></p> <div class="users"> <p>Active Users:</p> <p><font>257</font></p> </div> <h4 class="title3">Search by brand</h4> <ul class="brands"> <li class="color"><a href="#">test</a></li> <li><a href="#">test</a></li> <li class="color"><a href="#">test</a></li> <li><a href="#">test</a></li> <li class="color"><a href="#">test</a></li> <li><a href="#">test</a></li> <li class="color"><a href="#">test</a></li> <li><a href="#">test</a></li> <li class="color"><a href="#">test</a></li> <li><a href="#">test</a></li> <li class="color"><a href="#">test</a></li> <li><a href="#">test</a></li> <li class="color"><a href="#">test</a></li> <li><a href="#">test</a></li> <li class="color"><a href="#">test</a></li> <li><a href="#">test</a></li> <li class="color"><a href="#">test</a></li> <li><a href="#">test</a></li> <li class="color"><a href="#">test</a></li> <li><a href="#">test</a></li> <li class="color"><a href="#">test</a></li> <li><a href="#">test</a></li> <li class="color"><a href="#">test</a></li> <li><a href="#">test</a></li> <li class="color"><a href="#">test</a></li> <li><a href="#">test</a></li> <li class="color"><a href="#">test</a></li> <li><a href="#">test</a></li> <li class="color"><a href="#">test</a></li> <li><a href="#">test</a></li> </ul> </div> </div> </div> </div> </div> <div id="footer"> <div> <ul> <li><a href="index.html">Home</a>|</li> <li><a href="#">How it works</a>|</li> <li><a href="#">Travel</a>|</li> <li><a href="#">Goods</a>|</li> <li><a href="#">Insurance</a>|</li> <li><a href="#">Services</a>|</li> <li><a href="#">Hire</a>|</li> <li><a href="#">About us</a>|</li> <li><a href="#">Bookmark</a>|</li> <li><a href="#">My Account</a>|</li> <li><a href="#">Track Order</a>|</li> <li> </li> </ul> <p id="bft">Copyright ©<a href="http://www.compareandchoose.com.au">C&C</a>. All rights reserved.</p> </div> </div> </body> </html>
-
Why are you running htmlspecialchars() on the content before you extract the content you want? According to your regex youa re looking for content that comes after a tag with a parameter ending in 'image-carousel">'. The htmlspecialchars() will convert that closing '>' into '<' and your regex will not match it.
-
Well, two things. 1. I made a mistake in not converting those last two echo statements to have the content concatenated to the $output variable. and 2. You failed to echo the $output variable on your page. Please read my "note" again. Lastly, I do not provide the code with any guarantee it will work out-of-the-box. I don't have your database to test against, so I don't waste my time debugging the code. If you want that kind of help then post in the freelance forum.
-
Try this: (Note: I define all the output in a variable called $output. This way you can put the 'logic' at the top of your page and just echo the output into the appropriate place on the page. Makes the pages easier to manage and maintain) <?php //This is the number of results displayed per page $page_rows = 4; // Connects to your Database mysql_connect("localhost", "secret", "secret") or die(mysql_error()); mysql_select_db("mydatabase") or die(mysql_error()); //Here we count the number of results $query = "SELECT COUNT(*) FROM merchant"; $result = mysql_query($query) or die(mysql_error()); $total_rows = mysql_result($result, 0); //This tells us the page number of our last page $total_pages = ceil($total_rows/$page_rows); //This checks to see if there is a page number. If not, it will set it to page 1 $pagenum = (isset($_GET['pagenum'])) ? intval($_GET['pagenum']) : 1; //this makes sure the page number isn't below one, or more than our maximum pages $pagenum = max(1, $pagenum); $pagenum = min($total_rows, $pagenum); //This sets the limit to get the records for the selected page $limit = ($pagenum - 1) * $page_rows; //This is your query again, the same one... the only difference is we add LIMIT to it $query = "SELECT * FROM merchant ORDER BY ID ASC LIMIT {$limit}, {$page_rows}"; $result = mysql_query($query) or die(mysql_error()); //current URL of the Page. cart_update.php redirects back to this URL $current_url = base64_encode($url="http://".$_SERVER['HTTP_HOST'].$_SERVER['REQUEST_URI']); $results = $mysqli->query("SELECT * FROM merchant $max ORDER BY ID ASC"); //This is where you display your query results while($row = mysql_fetch_assoc($result)) { $output = "<div class=\"item\">\n"; $output .= "<form method=\"post\" action=\"Cart_update.php\">\n"; $output .= "<div class=\"product-thumb\"><img src=\"{$row['url']}\" width=\"20\" height=\"50\"></div>\n"; $output .= "<div class=\"product-content\"><h3>{$row['name']}</h3>\n"; $output .= " <div class=\"product-desc\">{$row['product']}</div>\n"; $output .= " <div class=\"product-info\">Price {$row['currency']}{$row['price']} <button class=\"add_to_cart\">Add To Cart</button></div>\n"; $output .= "</div>\n"; $output .= "<input type=\"hidden\" name=\"product_code\" value=\"{$row['ID']}\" />\n"; $output .= "<input type=\"hidden\" name=\"type\" value=\"add\" />\n"; $output .= "<input type=\"hidden\" name=\"return_url\" value=\"{$row['current_url']}\" />\n"; $output .= "</form>\n"; $output .= "</div>\n"; } // This shows the user what page they are on, and the total number of pages $output .= " --Page $pagenum of $total_pages-- <p>"; //Create var for pagination URL $pageURL = "{$_SERVER['PHP_SELF']}?pagenum="; // First we check if we are on page one. If we are then we don't need a link to the previous page or the first page so we do nothing. //If we aren't then we generate links to the first page, and to the previous page. if ($pagenum > 1) { $previous = $pagenum-1; $output .= " <a href='{$pageURL}1'> <<-First</a> "; $output .= " <a href='{$pageURL}{$previous}'> <-Previous</a> "; } //just a spacer $output .= " ---- "; //This does the same as above, only checking if we are on the last page, and then generating the Next and Last links if ($pagenum < $total_pages) { $next = $pagenum+1; echo " <a href='{$pageURL}{$next}>Next -></a> "; echo " <a href='{$pageURL}{$total_pages}'>Last ->></a> "; }
-
if (!(isset($pagenum))) { $pagenum = 1; } $pagenum is never defined before this - so it will always be 1. I think you meant to use something like: if(!(isset($_GET['pagenum'])) { $pagenum = 1; } else { $pagenum = intval($_GET['pagenum']); } That's just the first thing that jumped out at me. The code is a bit unorganized
-
Well, I haven't really dug into the query yet. But, I see one glaring problem based upon the schema. You don't have indexes on the necessary fields. Any field that is typically used as a lookup value (e.g. SELECT * FROM table WHERE id ='xx') or used to JOIN tables should be indexed. When looking at the table structure in MySQL you should see an icon that looks like a lightning bolt on the far right. Click it for any fields used as primary or foreign keys. Second, are the LEFT joins really necessary? The reason to use a LEFT join is where there may not be any records from the second table that match up with some of the records on the left table - but you still want those records. So, in this example: FROM 4_servicesuppliers SS LEFT JOIN 2_servicescatalogue SC ON (SS.bigint_ServiceID = SC.bigint_ServiceID) . . . is it a logic scenario that there are some records in 4_servicesuppliers which do not have any linked records in 2_servicescatalogue? If not, just use a notrmal JOIN. LEFT JOINS are much slower. That's the easy things. I would have to dig through the query structure in depth to see if there is anything else that jumps out at me. But, the indexing and removal of LEFT JOINS should have a big impact.
-
Where did you define $dbh? But, really, why are you even using prepare() at all in this instance? Prepared statements are meant for using placeholders in the query that will be 'replaced' when the query is executed. Since you aren't using placeholders the prepare statement is a waste of code.
-
What are you trying to replace it with? Do you just want to replace the km/h or do you need to first get the number to convert it to a different unit? If you need to do the latter, you will need to do a preg_match() to get the number and then a preg_replace to replace the number and the unit measurement. $subject = 'ds WSW 16km/h. Humidity will be 97% with . . .'; //Check if string contains ##km/h if(preg_match("#(\d*)km/h#is", $subject, $match)) { //Extract kilos $kmUnits = $match[1]; //Convert to miles $mileUnits = round($kmUnits * .6214); //Replace kilo reference with miles $subject = preg_replace("#\d*km/h#is", "{$mileUnits}mph", $subject); } echo $subject; Output: ds WSW 10mph. Humidity will be 97% with . . . Note: This only works if the subject has one such reference. If there can be multiple such references in the same text, then you would need to modify the above.
-
Mathematical formula to calculate spikes in line graph data?
Psycho replied to D.Rattansingh's topic in PHP Coding Help
I just picked those numbers at random. Surprising that all three of the high numbers were just outside the max for the standard deviation and the low numbers were just inside the minimum for standard deviation. But, the question I asked was still valid - but would require slightly different numbers. So, let's assume one of the high numbers was 80 and one of the low numbers was 10. The OP would need to determine if the 80 should be considered a spike and, by the same respect, if the 10 should be considered a negative spike. But, if it were me, I would stick to standard deviation calculations until and unless there is a scenario that does not produce the 'expected' results.