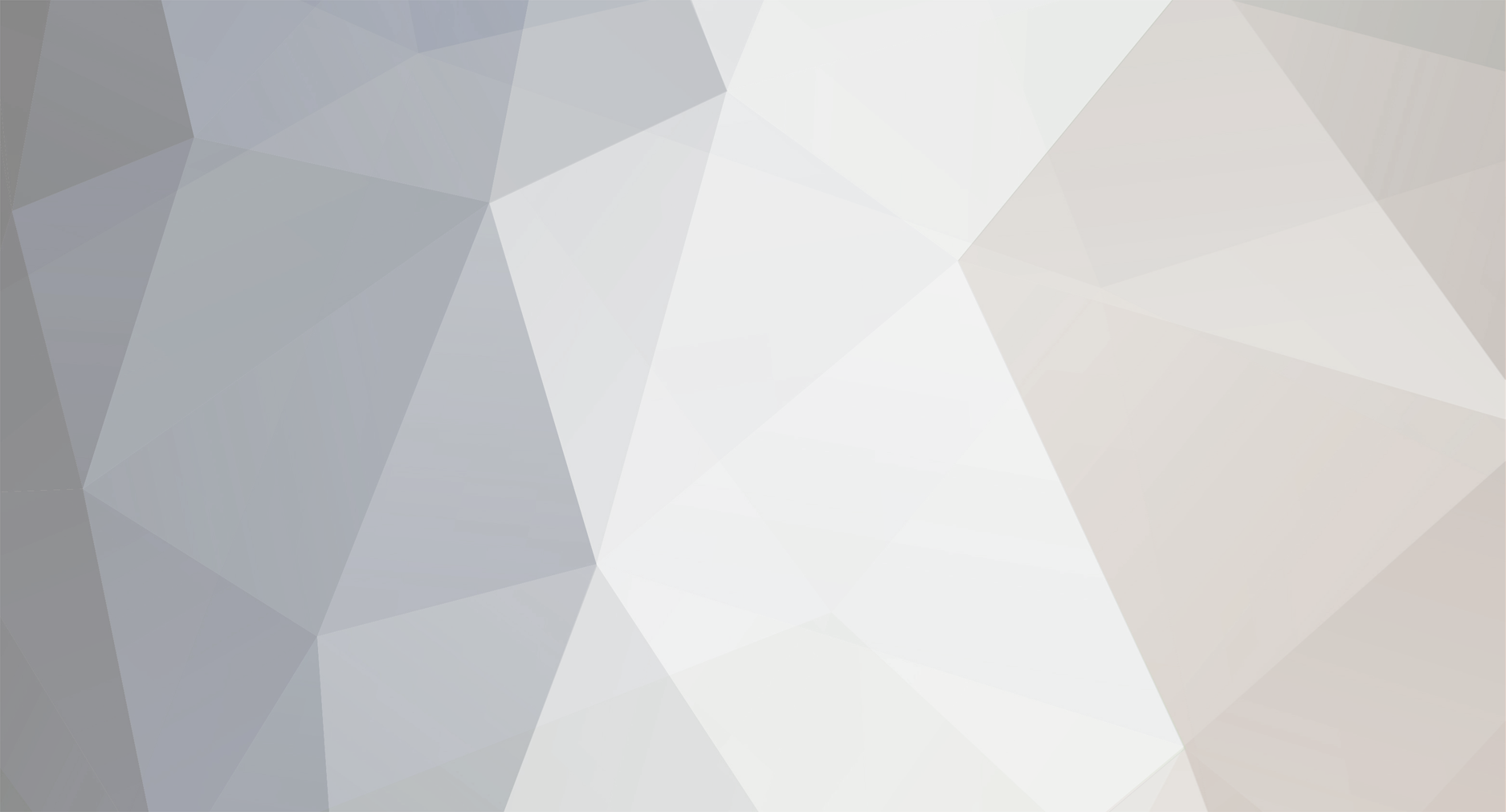
joquius
Members-
Posts
319 -
Joined
-
Last visited
Everything posted by joquius
-
mysqli_affected_rows() - using a SQL DELETE Statement
joquius replied to truegilly's topic in PHP Coding Help
Mine was a SELECT query (I added a query) -
First of all put all the PHP before the HTML, second, try using: mysql_query ("INSERT INTO `table` VALUES ('blah')") and not all that escaping, it's prone to errors. Also, you want to add, if the query is successful: if ($sql_query) { ?>yay! success!<? } else { ?>bleh form<? } or something similar
-
mysqli_affected_rows() - using a SQL DELETE Statement
joquius replied to truegilly's topic in PHP Coding Help
lets say someone inputs "delete.php?delete=10" [code] $query = mysql_query ("SELECT `id` FROM `table` WHERE `id` = '".mysql_real_escape_string ($_GET['id'])."'"); if (mysql_num_rows ($query) == 0) { $error = "Blah"; } elseif (mysql_num_rows ($query) > 1) { $error = "Bleh"; } else { delete it } [/code] -
There's no HTTP string which relates to your locale. You need to get an IP directory.
-
I won't go into the details, but get the length of the text, start reading it from the last character to the first with a loop while copying each character to a new string.
-
There are many scripts on the net with IP directories. Search for them on Google.
-
As far as I can see you haven't selected a database. Never worked with mysqli functions but I'm pretty sure they're not far from mysql functions. Also you need to be using either INSERT or UPDATE, not REPLACE which i'm not sure i've ever seen.
-
Please don't repost the same problem.
-
Well you'll need to be drawing those values from something, and saving them to the same place. Lets say a database in mysql. You'll need to change the href/src to values coming out of some loop of a database result. lets say you set up a DB 'Links' with three fields: id, href, image each line would have respective values, and you would be able to add, change lines. Then you could just have a while() loop listing those links with the respective values. You'll need to know some basics before doing all this though
-
Problem with showing/hiding files with list directory.
joquius replied to ted_chou12's topic in PHP Coding Help
[code] $exclude = Array ('file1.ext', 'file2.ext'); foreach (glob ($dir."*") as $file) { if (!in_array ($file, $exclude)) echo $file; } [/code] for example -
Problem with showing/hiding files with list directory.
joquius replied to ted_chou12's topic in PHP Coding Help
Use glob (*.ext) or loop excluding files with extensions you want to hide. -
hmm...? that's quite unneccesary This is a working script with a quirk only when the file name has brackets
-
You need to use javascript. Like add a button <input type="button" onclick="link()" /> <textarea id="text"></textarea> and add some JS at the top function link () { var link = prompt ("Enter URL:", "") if (link!="") var text = prompt ("Enter text:", ""); document.getElementById('text').value=document.getElementById('text').value+"[link=\""+link+"\"]"+text+"[/link]" } but that just adds it at the end of the text..I can't remember how to add it where you're typing, and if you want to highlight text and then click the button, it's a bit more complex.
-
I have the following little quirk (upload script): [code] $file_name = basename ($_FILES['image']['name']); $ul_dir = "/something_or_other/"; $ul_name = $ul_dir.$file_name; move_uploaded_file ($_FILES['image']['tmp_name'], $ul_name); getimagesize ($image_dir.$file_name); // $image_dir is defined elsewhere [/code] What happens is I get an error on the getimagesize() when the file name contains brackets or weird chars. Now I might turn out to be quite the dunce here, but is this to do with getimagesize or the request itself? This is the error I get: "[<a href='function.getimagesize'>function.getimagesize</a>]: failed to open stream: HTTP request failed! HTTP/1.1 400 Bad Request" I don't usually get this error from getimagesize if the file doesn't exist. Is this to do with the upload or the php function?
-
In the same way you cannot echo functions, you cannot echo objects, unless it's a reference or something. Will this matter? Is there a reason it has to be inside the quotes?
-
I believe you have to ". to add in the object, as php will always parse text:: as text no matter what follows. (given you're echoing something)
-
Ok there are a few questions. One is do you want to learn PHP or simply use it. If you want to use it, download a fully functional script from one of the many available resources on the web. If you want to learn it this brings us to another question, how much do you want to learn. If you want to have a comprehensive knowledge of the fine paininthearse art of PHP, I would advise you to consult a manual, such as on http://www.php.net/. If you want to learn it just for this, give up and download a script eh? This is a help forum, not a do-it-for-me forum.
-
Use javascript onClose event to direct you to another page which closes itself (php first, then JS) or use AJAX. Remember PHP is oblivious to what you do inside your browser after the page has loaded
-
First of all you should add this line to each loop (example for hours): [code] if ($db_hour == $i) echo "selected=\"selected=\""; [/code] Regarding the values in your database, The way you're using the dates here is a bit quirky but I don't know, just use an underscore.
-
Ok, for some reason lately (probably due to a change I made) a session script I've been using for years has stopped working properly in IE6-7. Quite simply it is not creating cookies. The strange thing is, that it does create cookies for anonymous sessions, but not for regular user sessions (they both share the same setcookie() function) Here's some code: [code] function s_create ($user_id) { global $c_time; // current time global $_config; $s_expire = db_data ("user_level", "s_expire", "level_id", db_data ("user_list", "user_level", "user_id", $user_id)); // 3600 in this case $user_ip = $_SERVER['REMOTE_ADDR']; $s_id = md5 (uniqid ($user_ip).$c_time); setcookie ($_config['s_cookie_name'], $s_id, $s_expire + $c_time, "/"); mysql_query ("DELETE FROM `user_sessions` WHERE `s_expire` + `s_active` <= '$c_time' && `user_id` = '$user_id' && `user_id` != '-1' || `user_id` = '-1' && `user_ip` = '$user_ip'"); // deletes inactive sessions mysql_query ("INSERT INTO `user_sessions` VALUES ('$s_id', '$user_id', '$user_ip', '$c_time', '$s_expire')"); // create session in database return $s_id; } [/code] It just doesn't create a cookie for '$user_id' = 1 and obviously there aren't many options because there are no problems in firefox. Is there any chance any other function here could have any difference. Any Ideas?
-
Any chance for a reply on this one? Was posted a week ago.
-
var_dump($_GET); if you want to see the stuff there but i didn't get what you're trying to do. Perhaps you want the next href="" to contain the previous _GET variables? [code] function http_referer () { foreach ($_GET as $key => $value) { $h_ref[$key] = $value; } $result = "?"; if (isset ($h_ref)) { foreach ($h_ref as $key => $value) { $result .= ($value != "") ? "&".$key."=".$value : "&".$key; } } return $result; } [/code]
-
You have to remember and if not remember realize that $_FILES['file'] is an array which contains the file name etc. I would advise you to read up on that stuff before writing the script. You've got quite a few issues here, for instance you're trying to put $s_data into the database which I don't think you wanted to do. What you might want to use for the fread or fopen is $_FILES['file']['tmp_name'] which is the uploaded file, but you should move the file first with move_uploaded_file () to an upload directory (check the function on PHP.net). for instance: [code] $file_name = basename ($_FILES['file']['name']); $directory = "/uploaddir/"; $uploaded_name = $directory.$file_name; move_uploaded_file ($_FILES['file']['tmp_name'], $uploaded_name); // now the file is in /uploaddir/file.ext and you can read it there fread(... [/code]
-
Ok, for some reason lately (probably due to a change I made) a session script I've been using for years has stopped working properly in IE6-7. Quite simply it is not creating cookies. The strange thing is, that it does create cookies for anonymous sessions, but not for regular user sessions (they both share the same setcookie() function) Here's some code: [code] function s_create ($user_id) { global $c_time; // current time global $_config; $s_expire = db_data ("user_level", "s_expire", "level_id", db_data ("user_list", "user_level", "user_id", $user_id)); // 3600 in this case $user_ip = $_SERVER['REMOTE_ADDR']; $s_id = md5 (uniqid ($user_ip).$c_time); setcookie ($_config['s_cookie_name'], $s_id, $s_expire + $c_time, "/"); mysql_query ("DELETE FROM `user_sessions` WHERE `s_expire` + `s_active` <= '$c_time' && `user_id` = '$user_id' && `user_id` != '-1' || `user_id` = '-1' && `user_ip` = '$user_ip'"); // deletes inactive sessions mysql_query ("INSERT INTO `user_sessions` VALUES ('$s_id', '$user_id', '$user_ip', '$c_time', '$s_expire')"); // create session in database return $s_id; } [/code] It just doesn't create a cookie for '$user_id' = 1 and obviously there aren't many options because there are no problems in firefox. Is there any chance any other function here could have any difference. Any Ideas?