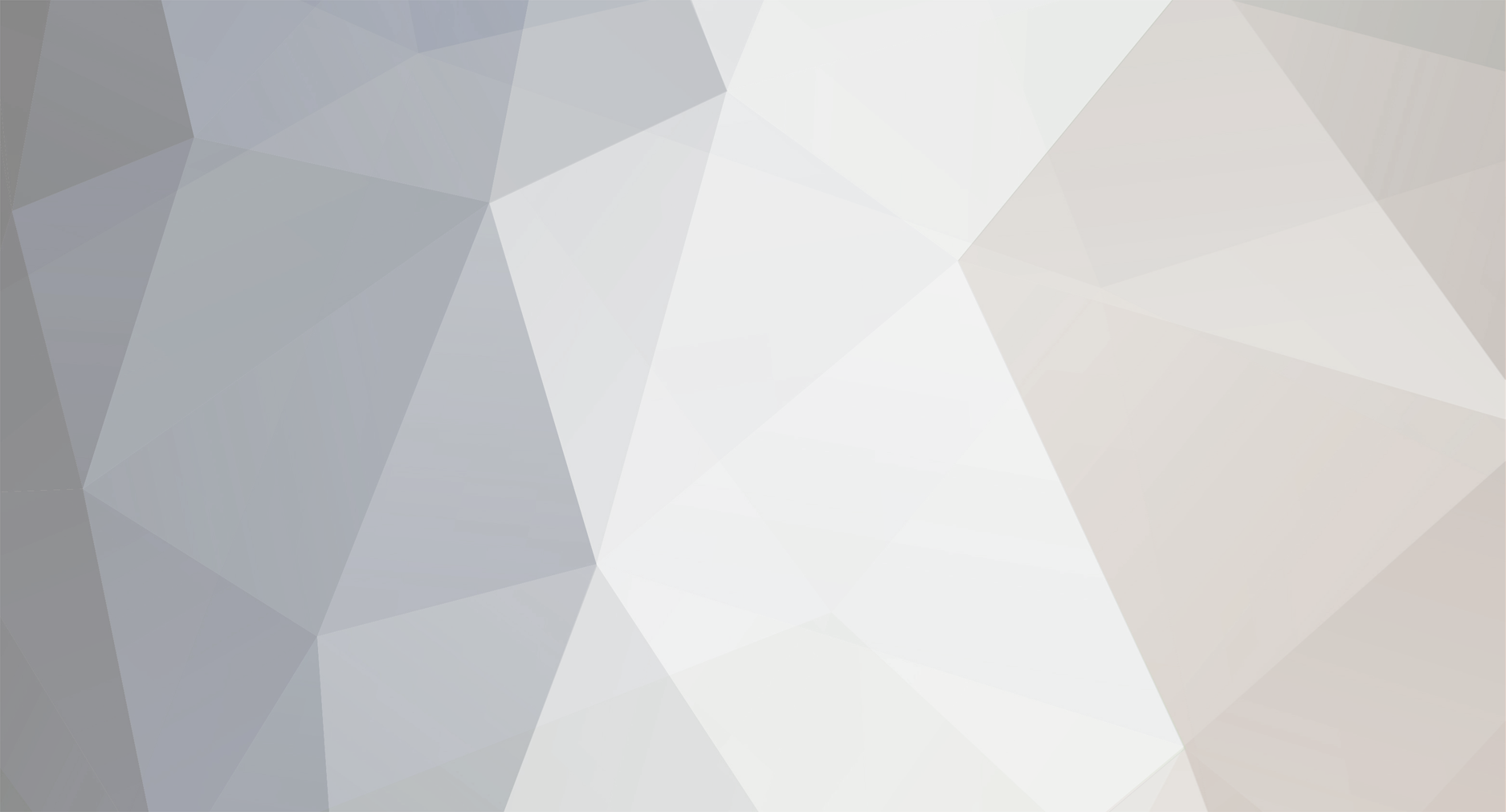
pocobueno1388
Members-
Posts
3,369 -
Joined
-
Last visited
Never
Everything posted by pocobueno1388
-
You can change the (-) to a (+), then it would GIVE them points. So yes, if someone sold something, to give them the points you would use a plus instead, but also make sure to do a query to subtract from the buyer as well. Why does this matter? Are they not allowed to buy the same thing twice? If not...instead of trying to enable the back button, make a query checking if they have that item already, if they do, then give them an error saying they already have it.
-
Give this a try <?php $str = array("apple is red", "banana is yellow", "pineaple is tropical fruit", "coconut is also tropical fruit", "grape is violet"); foreach ($str as $phrase){ if (strstr($phrase, 'coconut')) $coconut = $phrase; if(isset($coconut)) break; } echo $phrase; ?>
-
<?php $query = mysql_query( "SELECT id FROM topics WHERE id = '{$_GET['id']}'" ) or die(mysql_error()); $row = mysql_fetch_assoc($query); echo $row['id']; ?> If you expecting more than one result, then use a while loop <?php $query = mysql_query( "SELECT id FROM topics WHERE id = '{$_GET['id']}'" ) or die(mysql_error()); while ($row = mysql_fetch_assoc($query)){ echo $row['id'].'<br>'; } ?>
-
Ah, I see the problem now. Change ONLY the part of code I am posting <?php //check if they submitted the form if (isset($_POST['submit'])) { if ($_POST['action'] == 'buy') { echo "These are the items that were selected [they chose 'buy']<br>"; //Loop through the selected items foreach($_POST['selected'] as $itemID){ echo $itemID.'<br>'; //get the items price $get_price = mysql_query("SELECT item_price FROM phpbb_trading WHERE item_name='$itemID'") or die(mysql_error()); $price = mysql_fetch_assoc($get_price); //Check if they have enough if ($user_points['user_points'] > $price['item_price']) { echo "<p><b>Price:</b> - {$price['item_price']}<p>"; $update_q = "UPDATE phpbb_trading SET item_in_shop = '0', itemid = '$user_id' WHERE item_name = '$itemID'"; $update_q_final = mysql_query($update_q)or die(mysql_error().'With Query<p>'.$update_q); echo "Item Bought<br>"; //they have enough, now do a query to subtract their money $subtract = "UPDATE users SET user_points=user_points-{$price['item_price']} WHERE user_id='$user_id'"; $subtract_final = mysql_query($subtract)or die('ERROR: '.mysql_error().' with query<br>'.$subtract); } else { //they don't have enough money, give them an error... echo "Not Enought Boomies'"; } } } else { echo "They chose 'sell'"; } } ?>
-
I see a problem with it, but why are you suppressing the error with the @? Change it to this mysql_query( "SELECT id FROM topics WHERE id = '{$_GET['id']}'" ) or die(mysql_error()); EDIT: Also, it doesn't do you much good if you don't assign it to a variable. $query = mysql_query( "SELECT id FROM topics WHERE id = '{$_GET['id']}'" ) or die(mysql_error());
-
Whats up with this chunk of code? } else { echo "ERROR"; } You don't even have an IF statement to do an else off of...thats why your getting an error.
-
I'm thinking this query $subtract = "UPDATE users SET user_points=user_points-{$price['item_price']} WHERE itemid='$user_id'"; Should be changed to this $subtract = "UPDATE users SET user_points=user_points-{$price['item_price']} WHERE id='$user_id'"; Do you see what I'm saying? Where "id" is in the query is where you need to change it to whatever field the users ID is stored in the phpbb_users table.
-
What are you trying to do on that line? It makes no sense... $comparison = ( $version1, $version2, $operator );
-
How about the phpbb_users table? Does itemid exist in there? We are trying to update the USERS points...so I would assume you had a field called "userid" or something. You also need to tell me what variable in the script holds their ID.
-
Okay, look at this query $subtract = "UPDATE users SET user_points=user_points-{$price['item_price']} WHERE itemid='$user_id'"; It's saying the col "itemid" doesn't exist. So you need to change that to whatever the actual column name is. <?php define('IN_PHPBB', true); define('IN_CASHMOD', true); $phpbb_root_path = './'; include($phpbb_root_path . 'extension.inc'); include($phpbb_root_path . 'common.'.$phpEx); // // Start session management // $userdata = session_pagestart($user_ip, PAGE_INDEX); init_userprefs($userdata); // // End session management // // Sorry , only logged users ... if (!$userdata['session_logged_in'] ) { $redirect = "traded.$phpEx"; $redirect .= ( isset($user_id) ) ? '&user_id=' . $user_id : ''; header('Location: ' . append_sid("login.$phpEx?redirect=$redirect", true)); } if ($userdata['session_logged_in']) { // Who is looking at this page ? $user_id = $userdata['user_id']; if ((!( isset($HTTP_POST_VARS[post_USERS_URL]) || isset($HTTP_GET_VARS[post_USERS_URL]))) || ( empty($HTTP_POST_VARS[post_USERS_URL]) && empty($HTTP_GET_VARS[post_USERS_URL]))) { $view_userdata = $userdata; } else { $view_userdata = get_userdata(intval($HTTP_GET_VARS[post_USERS_URL])); } $searchid = $view_userdata['user_id']; $points = $userdata['user_points']; $posts = $userdata['user_posts']; //select the users money $query = mysql_query("SELECT user_points FROM phpbb_users WHERE user_id='$user_id'")or die(mysql_error()); $user_points = mysql_fetch_assoc($query); //check if they submitted the form if (isset($_POST['submit'])) { if ($_POST['action'] == 'buy') { echo "These are the items that were selected [they chose 'buy']<br>"; //Loop through the selected items foreach($_POST['selected'] as $itemID){ echo $itemID.'<br>'; //this is where you would do your query $update_q = "UPDATE phpbb_trading SET item_in_shop = '0', itemid = '$user_id' WHERE item_name = '$itemID'"; $update_q_final = mysql_query($update_q)or die(mysql_error().'With Query<p>'.$update_q); echo "Item Bought<br>"; //get the items price $get_price = mysql_query("SELECT item_price FROM phpbb_trading WHERE item_name='$itemID'") or die(mysql_error()); $price = mysql_fetch_assoc($get_price); //Check if they have enough if ($user_points['user_points'] > $price['item_price']) { echo "<p><b>Price:</b> - {$price['item_price']}<p>"; //they have enough, now do a query to subtract their money $subtract = "UPDATE users SET user_points=user_points-{$price['item_price']} WHERE itemid='$user_id'"; $subtract_final = mysql_query($subtract)or die('ERROR: '.mysql_error().' with query<br>'.$subtract); } else { //they don't have enough money, give them an error... echo "Not Enought Boomies'"; } } } else { echo "They chose 'sell'"; } } $result = mysql_query("SELECT * FROM phpbb_trading WHERE item_in_shop = '1'and itemid = '0' ")or die(mysql_error()); $num_rows = mysql_num_rows($result); print "<br>There are $num_rows cards left in the store.<P>"; print "<form action='{$_SERVER['PHP_SELF']}' method='post'>"; print '<table width=500 height= 500 border=1>'."\n"; while ($get_info = mysql_fetch_assoc($result)) { print "<tr>"; print "\t<td><font face=arial size=1/>"; print "<input type='checkbox' name='selected[]' value='{$get_info['item_name']}' />" .$get_info['item_name'].'<br><td>'.$get_info['item_price'].'<br>'.Boomies.'<br><td>'.$get_info['item_desc']."</font></td>"; print "</tr>"; } echo '<select name="action">' .'<option value="buy">Buy</option>' .'<option value="sell">Sell</option>' .'</select><p>'; echo '<input type="submit" name="submit">'; echo '</form>'; } else { echo('You are a guest'); } ?> Okay, try buying an item and see what it does/says. This is really hard trying to fix this when I have a small amount of information to work with such as database structure and all that. It's okay though, we will just have to take it one thing at a time until we figure it all out
-
Okay, try this... <?php define('IN_PHPBB', true); define('IN_CASHMOD', true); $phpbb_root_path = './'; include($phpbb_root_path . 'extension.inc'); include($phpbb_root_path . 'common.'.$phpEx); // // Start session management // $userdata = session_pagestart($user_ip, PAGE_INDEX); init_userprefs($userdata); // // End session management // // Sorry , only logged users ... if ( !$userdata['session_logged_in'] ) { $redirect = "traded.$phpEx"; $redirect .= ( isset($user_id) ) ? '&user_id=' . $user_id : ''; header('Location: ' . append_sid("login.$phpEx?redirect=$redirect", true)); } if($userdata['session_logged_in']) { // Who is looking at this page ? $user_id = $userdata['user_id']; if ( (!( isset($HTTP_POST_VARS[post_USERS_URL]) || isset($HTTP_GET_VARS[post_USERS_URL]))) || ( empty($HTTP_POST_VARS[post_USERS_URL]) && empty($HTTP_GET_VARS[post_USERS_URL]))) { $view_userdata = $userdata; } else { $view_userdata = get_userdata(intval($HTTP_GET_VARS[post_USERS_URL])); } $searchid = $view_userdata['user_id']; $points = $userdata['user_points']; $posts = $userdata['user_posts']; //select the users money $query = mysql_query("SELECT user_points FROM phpbb_users WHERE user_id='$user_id'")or die(mysql_error()); $user_points = mysql_fetch_assoc($query); //check if they submitted the form if (isset($_POST['submit'])){ if ($_POST['action'] == 'buy'){ echo "These are the items that were selected [they chose 'buy']<br>"; //Loop through the selected items foreach ($_POST['selected'] as $itemID){ echo $itemID.'<br>'; //this is where you would do your query $update_q = "UPDATE phpbb_trading SET item_in_shop = '0', itemid = '$user_id' WHERE item_name = '$itemID'"; $update_q_final = mysql_query($update_q)or die(mysql_error().'With Query<p>'.$update_q); echo "Item Bought<br>"; //get the items price $get_price = mysql_query("SELECT item_price FROM phpbb_trading WHERE item_name='$itemID'")or die(mysql_error()); $price = mysql_fetch_assoc($get_price); //Check if they have enough if ($user_points['user_points'] > $price['item_price']){ //they have enough, now do a query to subtract their money $subtract = "UPDATE users SET user_points=user_points-{$price['item_price']} WHERE itemid='$user_id'"; $subtract_final = mysql_query($subtract)or die('ERROR: '.mysql_error().' with query<br>'.$subract); } else { //they don't have enough money, give them an error... echo "Not Enought Boomies'"; } } } else { echo "They chose 'sell'"; } } $result = mysql_query("SELECT * FROM phpbb_trading WHERE item_in_shop = '1'and itemid = '0' ")or die(mysql_error()); $num_rows = mysql_num_rows($result); print "<br>There are $num_rows cards left in the store.<P>"; print "<form action='{$_SERVER['PHP_SELF']}' method='post'>"; print '<table width=500 height= 500 border=1>'."\n"; while ($get_info = mysql_fetch_assoc($result)) { print "<tr>"; print "\t<td><font face=arial size=1/>"; print "<input type='checkbox' name='selected[]' value='{$get_info['item_name']}' />" .$get_info['item_name'].'<br><td>'.$get_info['item_price'].'<br>'.Boomies.'<br><td>'.$get_info['item_desc']."</font></td>"; print "</tr>"; } echo '<select name="action">' .'<option value="buy">Buy</option>' .'<option value="sell">Sell</option>' .'</select><p>'; echo '<input type="submit" name="submit">'; echo '</form>'; } else { echo('You are a guest'); } ?> Where the heck is "Gold Test" coming from?
-
Okay, try this and post anything you get. Look through the queries and make sure I got all the row names right and everything <?php define('IN_PHPBB', true); define('IN_CASHMOD', true); $phpbb_root_path = './'; include($phpbb_root_path . 'extension.inc'); include($phpbb_root_path . 'common.'.$phpEx); // // Start session management // $userdata = session_pagestart($user_ip, PAGE_INDEX); init_userprefs($userdata); // // End session management // // Sorry , only logged users ... if ( !$userdata['session_logged_in'] ) { $redirect = "traded.$phpEx"; $redirect .= ( isset($user_id) ) ? '&user_id=' . $user_id : ''; header('Location: ' . append_sid("login.$phpEx?redirect=$redirect", true)); } if($userdata['session_logged_in']) { // Who is looking at this page ? $user_id = $userdata['user_id']; if ( (!( isset($HTTP_POST_VARS[post_USERS_URL]) || isset($HTTP_GET_VARS[post_USERS_URL]))) || ( empty($HTTP_POST_VARS[post_USERS_URL]) && empty($HTTP_GET_VARS[post_USERS_URL]))) { $view_userdata = $userdata; } else { $view_userdata = get_userdata(intval($HTTP_GET_VARS[post_USERS_URL])); } $searchid = $view_userdata['user_id']; $points = $userdata['user_points']; $posts = $userdata['user_posts']; //select the users money $query = mysql_query("SELECT user_points FROM phpbb_users WHERE user_id='$user_id'")or die(mysql_error()); $user_points = mysql_fetch_assoc($query); //check if they submitted the form if (isset($_POST['submit'])){ if ($_POST['action'] == 'buy'){ echo "These are the items that were selected [they chose 'buy']<br>"; //Loop through the selected items foreach ($_POST['selected'] as $itemID){ echo $itemID.'<br>'; //this is where you would do your query mysql_query("UPDATE phpbb_trading SET item_in_shop = '0', itemid = '$user_id' WHERE item_name = '$itemID'"); mysql_query($query)or die(mysql_error()); echo "Item Bought<br>"; //get the items price $get_price = mysql_query("SELECT item_price FROM phpbb_trading WHERE item_name='$itemID'")or die(mysql_error()); $price = mysql_fetch_assoc($get_price); //Check if they have enough if ($user_points['user_points'] > $price['item_price']){ //they have enough, now do a query to subtract their money $subtract = "UPDATE users SET user_points=user_points-{$price['item_price']} WHERE itemid='$user_id'"; $subtract_final = mysql_query($subtract)or die('ERROR: '.mysql_error().' with query<br>'.$subract); } else { //they don't have enough money, give them an error... echo "Not Enought Boomies'"; } } } else { echo "They chose 'sell'"; } } $result = mysql_query("SELECT * FROM phpbb_trading WHERE item_in_shop = '1'and itemid = '0' ")or die(mysql_error()); $num_rows = mysql_num_rows($result); print "<br>There are $num_rows cards left in the store.<P>"; print "<form action='{$_SERVER['PHP_SELF']}' method='post'>"; print '<table width=500 height= 500 border=1>'."\n"; while ($get_info = mysql_fetch_assoc($result)) { print "<tr>"; print "\t<td><font face=arial size=1/>"; print "<input type='checkbox' name='selected[]' value='{$get_info['item_name']}' />" .$get_info['item_name'].'<br><td>'.$get_info['item_price'].'<br>'.Boomies.'<br><td>'.$get_info['item_desc']."</font></td>"; print "</tr>"; } echo '<select name="action">' .'<option value="buy">Buy</option>' .'<option value="sell">Sell</option>' .'</select><p>'; echo '<input type="submit" name="submit">'; echo '</form>'; } else { echo('You are a guest'); } ?>
-
Okay, change you query to this mysql_query("UPDATE phpbb_adr_shops_items SET item_owner_id = '1' WHERE item_name = '$itemID'"); That should work, let me know if it does or doesn't.
-
I don't see where you getting the variable $item_name...where is it SUPPOSED to be coming from?
-
Well...that tells us that your variable $item_name is empty or not set. Post your entire updated code. This is very basic MySQL...you should go google for some tutorials. <?php //select the users money $query = mysql_query("SELECT money FROM users WHERE userID='$userID'")or die(mysql_error()); $money = mysql_fetch_assoc($query); //Check if they have enough if ($money['money'] > $price){ //they have enough, now do a query to subtract their money $subtract = mysql_query("UPDATE users SET money=money-$price WHERE userID='$userID'") or die(mysql_error()); } else { //they don't have enough money, give them an error... } ?>
-
You would just make it a link <?php echo "<a href='{$row['url']}' target='_blank'>{$row['link_name']}</a>"; ?>
-
Have you even attempted to code it? This forum is not for people to code things for you, it is for specific help with problems that occur with peoples already existing code. I suggest to go through some online tutorials, or if you not interested in learning PHP/MySQL, then hire a freelancer. www.tizag.com or www.w3schools.com are good places to start.
-
[SOLVED] calculate values from drop downs
pocobueno1388 replied to eideticmnemonic's topic in PHP Coding Help
You need a submit button, here is some working code that I think does what you want. <form method="post"> <select name="startLength"> <option value="25">0-10</option> <option value="50">10-30</option> <option value="100">30-60</option> </select> <select name="EndLength"> <option value="25">0-10</option> <option value="50">10-30</option> <option value="100">30-60</option> </select> <input type="submit" name="submit" value="Add Values!"> </form> <?php if(isset($_POST['submit'])){ $start = $_POST['startLength']; $end = $_POST['EndLength']; $totalcost = ($start + $end)/2; echo '<BR><BR>'; echo 'Your total is: '. number_format($totalcost,2,'.',','). '.'; } ?> When you press submit it will give you the total cost. -
They said the URL was showing up fine, so obviously that is not the problem, plus this probably isn't their entire code. I don't see any problems with what you have. Maybe post more of the script.
-
Are you getting any errors, or is it telling you the record was added?
-
[SOLVED] Getting Data From more than one table with MYSQL
pocobueno1388 replied to $username's topic in PHP Coding Help
Put a die statement after you execute the query to see if there are any errors. $query162 = mysql_query($sql162)or die(mysql_error()); -
What exactly are you trying to accomplish? To pass information through the URL you can simply make a link that looks like this <a href="domain.com/script.php?var=something&anothervar=somethingelse">Link</a> Or you could pass it through a form action and use the same url. You can read more about GET here http://www.tizag.com/phpT/postget.php
-
I believe you would just add AUTO_INCREMENT to the line. $sql = "CREATE TABLE Data ( ID int AUTO_INCREMENT, Firstname text, Lastname text, Age int, )";
-
Here is how your table structure would need to be ------------------------------------------------- TABLE podcasts [this table should already be made] -------------------------------------------------- podcastID other_info ... ... ----------------- TABLE comments ----------------- commentID podcastID [the ID of the podcast from the "podcasts" table] body [the actual comment/feedback] Here are some tutorials for help with the coding http://www.zimmertech.com/tutorials/php/25/comment-form-script-tutorial.php http://www.codewalkers.com/c/a/Database-Articles/PHPMySQL-News-with-Comments/ http://www.dmxzone.com/ShowDetail.asp?NewsId=5990 And there is much more on google if you type in "PHP comment system tutorial".
-
You can just do this <?php if ($password1 == $password2) { ?> <form method="post" action="action.php"> <fieldset> <legend>Login</legend> <label for="username">Username:</label><input type="text" name="username" id="username" value="" /> <label for="password">Password:</label><input type="password" name="password" id="password" value="" /> <div id="security"><img src="security-image.php?width=144" width="144" height="30" alt="Security Image" /></div> <label for="code">Security Image:</label><input type="text" name="code" id="code" value="" /> <input type="submit" name="login" id="login" value="Login" /> </fieldset> </form> <?php } ?>