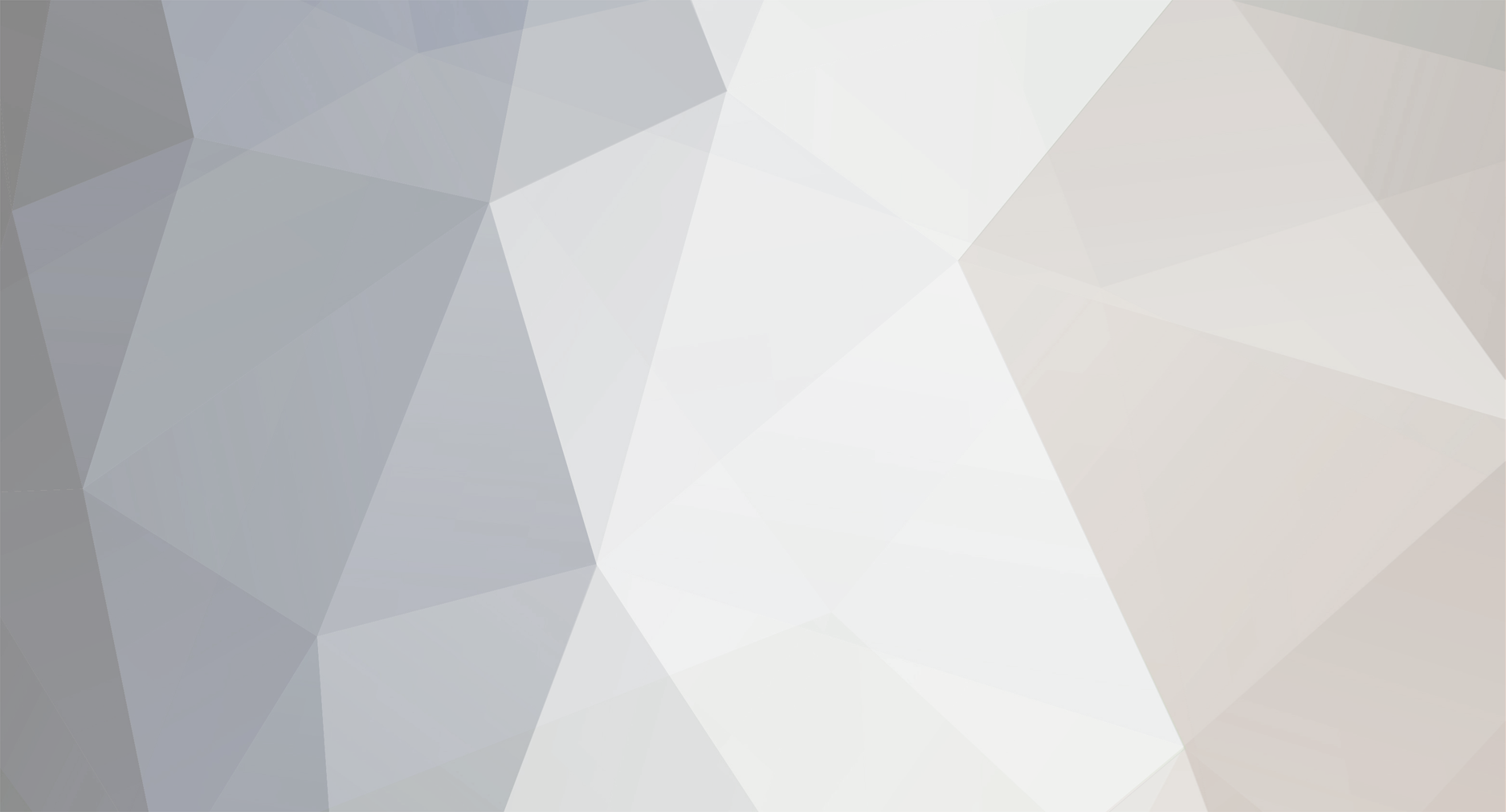
pocobueno1388
Members-
Posts
3,369 -
Joined
-
Last visited
Never
Everything posted by pocobueno1388
-
Use this code and copy and paste anything you see on the screen <?php // if the submit button is clicked, do this. if ($_POST['update']) { $table = $_POST['table']; $returnurl1 = "find_articles.php"; // this is the redirect url after succes $returnurl2 = "edit_articles.php?id=".$_GET['idr']."&mode=modify"; // this is the redirect url after success if ($_GET['mode']=='add') { $insert = "INSERT INTO articles (title, body) VALUES ('".$_POST['title']."', '".$_POST['body']."')"; mysql_query($insert); // redirect after 2 seconds echo "<div><center>Success! Article Added!</center></div>"; echo "<META HTTP-EQUIV=\"Refresh\" CONTENT=\"2; URL=".$returnurl1."\">"; } //if mod eis not add, then the mode is modify else { echo "This line should show up...<p>"; $update = "UPDATE articles SET title = '".$_POST['title']."', body = '".$_POST['body']."' WHERE id ='".$_GET['idr']."'"; $check = mysql_query($update)or die("ERROR - ". mysql_error() ."<br>" . $update); echo $update.'<p>'; echo "<div><center>Success! Job Saved!</center></div>"; //echo "<META HTTP-EQUIV=\"Refresh\" CONTENT=\"2; URL=\"index.php\">"; } } ?>
-
Change your code to this and tell us what it gives you <?php $update = "UPDATE articles SET title = '".$_POST['title']."', body = '".$_POST['body']."' WHERE id ='".$_GET['idr']."'"; $check = mysql_query($update)or die("ERROR - ". mysql_error() ."<br>" . $update); ?>
-
You can create the link like this <a href="your_url.php?action=add">Click</a> Then on "your_url.php" put this code <?php if (isset($_GET['action']) && $_GET['action'] == 'add'){ $query = "UPDATE table SET number=number+1 WHERE condition"; $result = mysql_query($query)or die(mysql_error()); echo "DB updated"; } ?>
-
Put a die at the end of your query $result = mysql_query($query); Also, try echoing the query out to see if it comes out right.
-
[SOLVED] Form Problem, need debugging tips
pocobueno1388 replied to atticus's topic in PHP Coding Help
Nope, you don't have it set. Change your link to this echo "<div><b>Username:</b> ".$row['cust_id']." | <a href=\"cust_edit.php?cust_id=$row[cust_id]\">Edit User</a><br />"; -
[SOLVED] Form Problem, need debugging tips
pocobueno1388 replied to atticus's topic in PHP Coding Help
Are you sure $_GET['cust_id'] is set? If it isn't, the form isn't going to show. -
[SOLVED] Problem getting Sessions working
pocobueno1388 replied to benn600's topic in PHP Coding Help
Look at your php info <?php phpinfo(); ?> Go down and find where it says "Session Support" and see if it says "enabled". -
Take this for example <?php if (isset($_POST['upload'])){ $name = $_FILES['uploadedfile']['name']; //The name of the file, EX - name.jpg $name = explode('.', $name); $ext = $name[1]; //The type of file/extension, EX - .jpg echo $ext; } ?> <form enctype="multipart/form-data" action="z.php" method="POST"> <input type="hidden" name="MAX_FILE_SIZE" value="100000" /> Choose a file to upload: <input name="uploadedfile" type="file" /><br /> <input type="submit" value="Upload File" /> </form> You need to use the $_FILES variable to retrieve information about the file. First take a look at this line $name = $_FILES['uploadedfile']['name']; Where it says "uploadedfile", that is where you need to put the name of the input, which in the example, comes from this line Choose a file to upload: <input name="uploadedfile" type="file" /><br /> See if you can work out your problem by looking at that, if not just let us know.
-
Most of your form fields are named "username", then you have the "id" attribute as the name, you want to switch that. So, you form should look like this: <center> <font face='verdana, arial, helvetica' size='2' align='center'>Enter the requested Username and Password </font> <form method="post"> <table width="400" border="0" cellspacing="1" cellpadding="2" > <tr> <td width="100" ><font face='verdana, arial, helvetica' size='2' align='center'>Username</font></td> <td><input name="username" type="text" id="username"></td> </tr> <tr> <td width="100"><font face='verdana, arial, helvetica' size='2' align='center'>Password</td> <td><input name="password" type="text" id="password"></font></td> </tr> <tr> <td width="100" ><font face='verdana, arial, helvetica' size='2' align='center'>First Name</font></td> <td><input name="fname" type="text" id="fname"></td> </tr> <tr> <td width="100" ><font face='verdana, arial, helvetica' size='2' align='center'>Last Name</font></td> <td><input name="lname" type="text" id="lname"></td> </tr> <tr> <td width="100" ><font face='verdana, arial, helvetica' size='2' align='center'>E-mail</font></td> <td><input name="email" type="text" id="email"></td> </tr> <tr> <td width="100"> </td> <td> </td> </tr> <tr> <td width="100"> </td> <td><font face='verdana, arial, helvetica' size='2' align='center'><input name="add" type="submit" id="add" value="Add New User"></font></td> </tr> </table> </form></center>
-
It will include 1 and 9 as well.
-
Yes, now $n will hold a random number between 1 and 9
-
You need the rand() function http://us3.php.net/rand
-
If that one doesn't work, try this. SELECT * FROM table WHERE `date` BETWEEN NOW() AND NOW() + INTERVAL 14 DAY I agree, what's up with storing it in a TEXT field?
-
Please click "Topic Solved" so everyone doesn't come into the topic.
-
[SOLVED] insert character specified number of times
pocobueno1388 replied to brendan6's topic in PHP Coding Help
Here is a working version of your function <?php function repeat($string,$number){ $output= ''; for($i=0;$i<$number; $i++){ $output .= $string; } return $output; } echo repeat('*', 1); ?> -
You need to wrap it in brackets. $sql="INSERT INTO pyramid(PyramidID,Serialnum,Upline) VALUES ('$lastID','$cardID','{$_POST['upline']}','11111')";
-
Your going to have to explain exactly what you want cleaned up. I'm not sure if what your asking has anything to do with PHP, but from the sound of things it seems like it should be in the HTML or CSS section.
-
EDIT: Beat to it Indent your code and it will be much easier to see <?php //Check if any IP address's are banned include 'ban.php'; include 'php/config.php'; include 'php/opendb.php'; //Flood Protection if (isset($_POST['upload'])) { $ip = $_SERVER['REMOTE_ADDR']; $period = 10; $delete = mysql_query("DELETE FROM `posters` WHERE `date` < '".(($now = time()) - $period)."'"); $query = mysql_fetch_array(mysql_query("SELECT * FROM posters")); if ($query['ip']==$ip) { echo '<span style="color:#B94A4A; text-decoration:blink; font-weight: bold; font-size: 24px;">Error. Flood Protection Has Not Expired.</span><br /><br />'; echo '<div align="center" class="smalltext">Copyright <a href="http://antistuff.net/">antistuff</a> 2007© All rights reserved.</div>'; exit; } else { // Variables $width = 0; $heigth = 0; $filePath = "files/"; $filename = $_FILES['userfile']['name']; $filesize = $_FILES['userfile']['size']; $fileType = $_FILES['userfile']['type']; $tmpName = $_FILES['userfile']['temp']; $ip = $_SERVER['REMOTE_ADDR']; $Type = Array('Bytes', 'KB', 'MB', 'GB', 'TB', 'ZB' ); $times=0; while ($fileSize > 1024 ) { $fileSize = $fileSize / 1024; $fileSize = round($fileSize); $times++; } //Allows uppercase filetypes. $fileType = strtolower(strtoupper(strstr($fileName, '.'))); if (($fileType != '.gif')||($fileType != '.jpeg')||($fileType != '.jpg')||($fileType != '.png')||($fileType != '.zip')||($fileType != '.rar')||($fileType != '.doc')|| ($fileType != '.txt')||($fileType != '.mp3')||($fileType != '.wav')||($fileType != '.avi')||($fileType!= '.mov')||($fileType != '.swf')||($fileType != '.fla')) { echo '<span style="color:#B94A4A; text-decoration:blink; font-weight: bold; font-size: 24px;">Error. Wrong FileType.</span><br /><br />'; echo '<div align="center" class="smalltext">Copyright <a href="http://antistuff.net/">antistuff</a> 2007© All rights reserved.</div>'; exit; } else if ($filesize > 5242880) { echo '<span style="color:#B94A4A; text-decoration:blink; font-weight: bold; font-size: 24px;">Error. File Too Big.</span><br /><br />'; echo '<div align="center" class="smalltext">Copyright <a href="http://antistuff.net/">antistuff</a> 2007© All rights reserved.</div>'; exit; } else if (file_exists("files/".$filename)) { $r_1 = rand(1,1000); $r_2 = rand(1000,2000); $r_3 = rand(3000,7000); $filename = $r1 . $r2 . $r3 . $filename ; } else { $result = move_uploaded_file($tmpName, $filePath); if (!$result) { echo '<span style="color:#B94A4A; text-decoration:blink; font-weight: bold; font-size: 24px;">Error. Try Again.</span><br /><br />'; echo '<div align="center" class="smalltext">Copyright <a href="http://antistuff.net/">antistuff</a> 2007© All rights reserved.</div>'; exit; } else if (!get_magic_quotes_gpc()) { $filename = addslashes($filename); $filepath = addslashes($filepath); } if (($fileType == '.gif')||($fileType == '.jpeg')||($fileType == '.jpg')||($fileType == '.png')||($fileType == '.swf')) { list($width, $height, $type, $attr) = getimagesize("files/".$filename); } $date = time(); mysql_query("INSERT INTO posters (ip,date) VALUES ('$ip','$date')") or die(mysql_error()); mysql_query("INSERT INTO upload (name, size, type, ip, path, width, height) VALUES ('$fileName', '$fileSize', '$fileType', '$ip', '$filePath', '$width', '$height')") or die('Error, query failed : ' . mysql_error()); $newfilePath = str_replace(' ','%20',$filePath); $finddetails = mysql_query("SELECT * FROM upload ORDER BY id DESC LIMIT 1"); $filedetails = mysql_fetch_array($finddetails); $id2 = $filedetails['id']; echo '<span style="color:#8FC73D; text-decoration:blink; font-weight: bold; font-size: 24px;">Success</span><br />'; echo "Direct Link: <a href=". $newfilePath .">$fileName</a><br />"; if (($fileType == '.gif')|| ($fileType == '.jpeg') || ($fileType == '.jpg') || ($fileType == '.png') || ($fileType == '.mp3') || ($fileType == '.wav') || ($fileType == '.swf')) { echo "Share Link: <a href=". $websiteview . $id2 .">$fileName</a><br />"; //Size $Type = Array('Bytes', 'KB', 'MB', 'GB', 'TB', 'ZB' ); $times=0; while ($fileSize > 1024 ) { $fileSize = $fileSize / 1024; $fileSize = round($fileSize); $times++; } echo 'Size: '; echo $fileSize.' '.$Type[$times]; echo '<br /><br />'; } //Images display an image below information. elseif (($fileType == '.gif')||($fileType == '.jpeg')||($fileType == '.jpg')||($fileType == '.png')) { echo "<img src=". $newfilePath ."><br /><br />"; } //Swfs display an embedded Swf below information. elseif ($fileType == '.swf') { echo "<embed src=". $newfilePath ." width=". $width ." height=". $height ."><br /><br />"; } } } } ?>
-
A question mark in an if statement would be the Ternary Operator, which is a way to shorten an If/Else statement. http://www.totallyphp.co.uk/tutorials/using_if_else_ternary_operators.htm
-
It might be because one of your field names is "Time". Do this $query = mysql_query("INSERT INTO `hospital` (HospitalID,CountryID,UserID,`Time`,When,IllnessType) Values ('1','1','$ID','7200','$Date','Autumn Flu')") or die(mysql_error());
-
[SOLVED] how is this not working???!!! simple
pocobueno1388 replied to mybluehair's topic in PHP Coding Help
Just to elaborate on the last post You need to change this echo 'welcome $one to here'; To echo "welcome $one to here"; -
Try this SELECT c.file_info FROM cust c LEFT JOIN upload u ON u.cust_id = c.cust_id
-
Ah, okay. http://www.tizag.com/phpT/fileupload.php
-
Are you wanting a "progress bar"? Like it tells you the percentage complete? http://blog.joshuaeichorn.com/archives/2005/05/01/ajax-file-upload-progress/ Not sure if I misunderstood or not, correct me if I'm wrong.