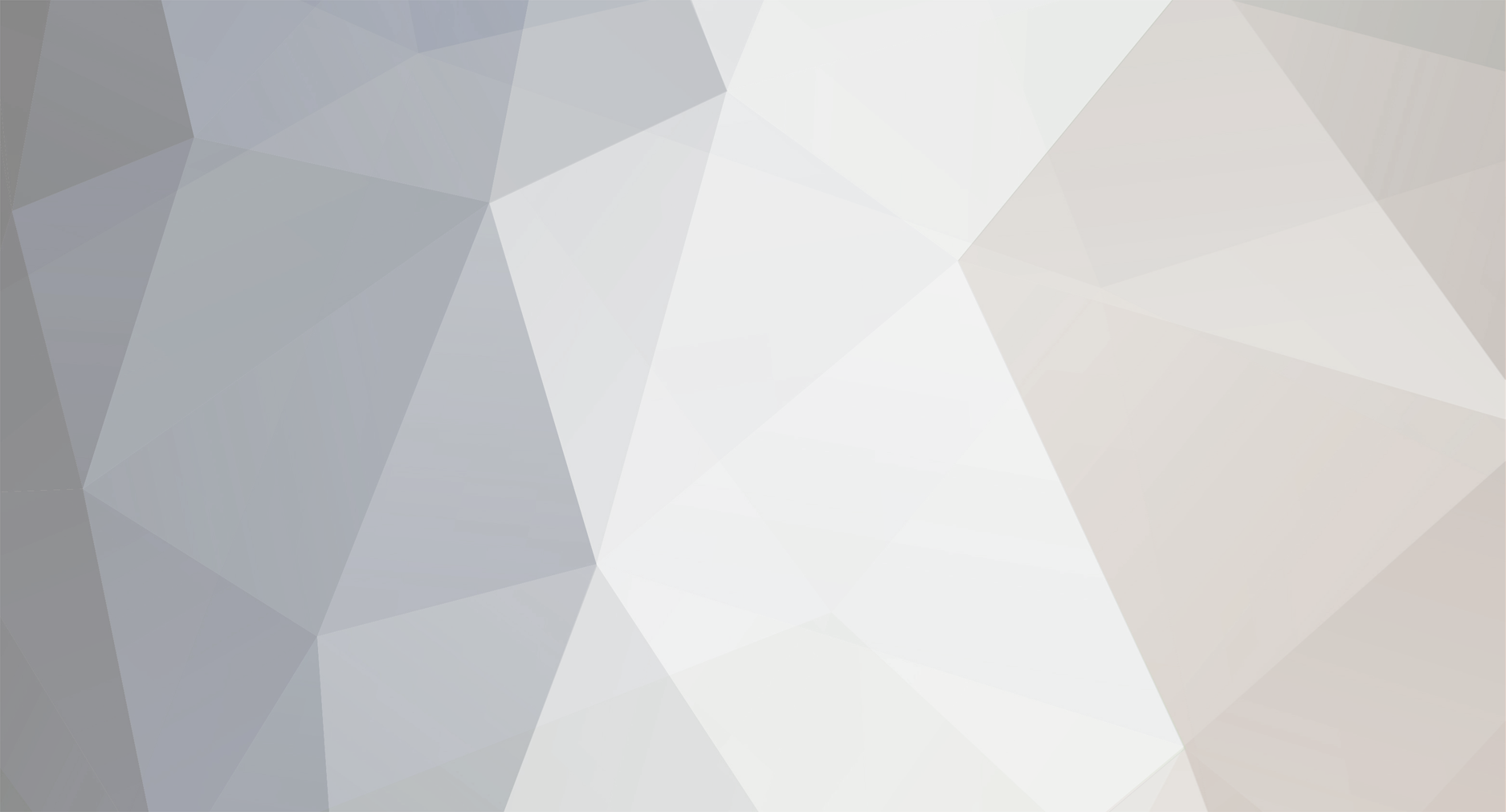
Chappers
Members-
Posts
119 -
Joined
-
Last visited
Never
Everything posted by Chappers
-
Hi, I'm using: <?php $result = mysql_query("SHOW FIELDS FROM $tablename"); to get the field names from a MySQL table, which is working fine. What I would like to do now is to also get the attributes of each field, so for the field ID, it would return "int(10) NOT NULL auto_increment". The reason for this is that I'm creating an admin page that allows me to create and delete tables from my MySQL database without having to log on to my CPanel, then the database, then Admin, then do it all from there. I can do it more quickly and simply from my website like this. At present I have written code to give me full control over the above and it works, but I'd like to be able to not only list each table and its fields, but also the field attributes so I can keep track of it all and see what might need modifying, etc. Which also makes me think: I can create tables with any fields and their respective attributes using my admin page, but is it also possible to modify the field attributes of any given table, and edit the fields themselves (or delete, etc.)? Thanks in advance.
-
That's very helpful, thanks a lot. I didn't know those things, so it'll help me understand why my code sometimes does unexpected things.
-
You are, of course, absolutely right - the problem was elsewhere. The syntax checker I use online was correctly finding an issue, but wrongly stating what the issue was, as it sometimes does (but it's good overall to find errors). I would have realised if perhaps I hadn't been doing this at 3am... For others who might search and find this I'll explain: I was using this snippet of code from a much larger page: <?php $result = mysql_query("DROP TABLE $table"); if (mysql_error()) { $errmsg = "<span class='error'>Query failed.<br>MySQL Error Number: ".mysql_errno()."<br>MySQL Error Description: ".mysql_error()."</span>"; echo $errmsg; } else { echo "Table $table deleted"; } ?> That worked fine, until I changed it to use an array so that I can print all error/success messages afterwards on a specific area of the page. I removed the <span class...> from the error message as I could add a class to the whole contents of the $msgs[] array myself before printing it. My test code was: <?php $result = mysql_query("DROP TABLE $table"); if (mysql_error()) { $msgs[] = "Query failed.<br>MySQL Error Number: ".mysql_errno()."<br>MySQL Error Description: ".mysql_error()."; } else { $msgs[] = "Table $table deleted"; } foreach ($msgs as $message) { echo $message; }?> It was the code above that wasn't working, creating an error wrongly attributed to the $msgs[] square bracket. It was my lack of understanding about the correct format to use when mixing normal words and functions, if that's how to describe it. It was this: <?php // Below is the incorrect line, only one double-quotes at the end of it. $msgs[] = "Error inserting data.<br>MySQL Error Number: ".mysql_errno()."<br>MySQL Error Description: ".mysql_error()."; // This one works $msgs[] = "Error inserting data.<br>MySQL Error Number: ".mysql_errno()."<br>MySQL Error Description: ".mysql_error().""; ?> So, trouble started when I removed the span class attribute because I didn't realise that when I removed the </span> at the end, I needed to leave the extra set of double quotes. I still don't properly get the business of functions and words mixed together and using "." to switch between the two, but it's working now anyway.
-
Oh, I do want the variable name to be interpreted. I want $another from my example to be entered into $msgs[] as the string it originally was, but either way, it produces an error. I've tried: <?php $msgs[] = "This $example failed"; $msgs[] = "This ".$example." failed"; $msgs[] = "This '$example' failed"; $msgs[] = "This {$example} failed"; $msgs[] = $example; ?> And all create a parse error: Parse error: parse error, unexpected ']', expecting T_STRING or T_VARIABLE or T_NUM_STRING
-
Hi, just a quickie. This works, so I can get as many error/success messages as I want during a script, then have them all print out wherever I want them to: <?php if (empty($example)) { $msgs[] = "Example did not work";} else { $msgs[] = "Example worked";} if (empty($another)) { $msgs[] = "Another did not work";} else { $msgs[] = "Another worked";} foreach ($msgs as $message) { echo "$message<br>";} So in a certain section I get all messages printed one after another. No problem. But if I need to add the name of a variable which was involved to $msgs[], so I know exactly what it's referring to, like this: <?php if (empty($example)) { $msgs[] = "Example did not work";} else { $msgs[] = "Example worked";} if (empty($another)) { $msgs[] = "$another did not work";} else { $msgs[] = "$another worked";} foreach ($msgs as $message) { echo "$message<br>";} The code fails with an error regarding the square brackets. Is there a way I can do it this way but have $msgs[] = "Blah blah $variable blah blah"; and have it work? Thanks!
-
Thanks everyone, plenty to think about there and try. The main reason I liked it grabbing words like 'ass' wherever they were in a word was because I didn't then have to add every possible combination to the filter list, like 'dumbass', 'asshole' and so on. Of course, it then grabs things like class, assign, assistant... so that'd mean making a permitted word list containing every possible word containing ass that's alright to let through. Either way, it's a task. Of course, if someone is set upon swearing, they'll get around it with things like 'a sshole' and so on, but I'm hoping just to limit the amount of opportunistic swearers who can't be bothered trying to outsmart the filter. It's either that or create a word list hundreds or thousand of words long, trying to consider every permutation of a word like 'b itch', 'bi tch', 'b i t c h' and ever on. So I think just trying to limit it is the only viable solution. I may try implementing the permitted words filter and just add words as they come up that are getting blocked but shouldn't be. Thanks again for so much help and some great suggestions!
-
Try this: <?php mysql_connect("localhost", "root", "") or die(mysql_error()); mysql_select_db("registration") or die(mysql_error()); if (isset($_POST['submitpost'])) { $test = $_POST['grp']; $test2 = $_POST['userpost']; echo "Right here... $test ...there should be the grp field data<br>"; echo "Here should be the textarea data... $test2 ...if submit's working"; if(is_numeric($_POST['grp'])) { $id = $_POST['grp']; $result = mysql_query("SELECT * FROM groups WHERE id = '$id'") or die(mysql_error()); $group = mysql_fetch_array($result, MYSQL_ASSOC); $groupname = $group['name']; $groupid = $group['id']; $result2 = mysql_query("SELECT id FROM users WHERE username = '$username'") or die(mysql_error()); $usera = mysql_fetch_array($result2, MYSQL_ASSOC); $userid = $usera['id']; $userpostg = mysql_real_escape_string($_POST['userpost']); // This gets today's date $date = time(); //This puts the day, month, and year in separate variables $day = date('d', $date); $month = date('F', $date); $year = date('Y', $date); } // This makes sure they did not leave any fields blank if (empty($_POST['userpost'])) { die('You did not enter a post'); } // Now we insert it into the database $add_post2 = mysql_query("INSERT INTO posts_groups (gid, gname, uid, uname, post, month, day, year) VALUES ('$groupid', '$groupname', $userid', '$username', '$userpostg', '$month', '$day', '$year')") or die(mysql_error()); echo <<<chappers <form action="{$_SERVER['PHP_SELF']}" method="post"> <table border="0" width="800" align="center" height="100"> <tr> <td align="center"><textarea name="userpost" type="description" cols="50" rows="5" STYLE="color: #FFFFFF; font-family: Verdana; font-weight: bold; font-size: 12px; background-color: #72A4D2;"></textarea></td></tr> <tr><input type='hidden' name='grp' value='{$_GET['grp']}'></td></tr> <tr><td align="center"><input type="submit" name="submitpost" value="Post"></td></tr> </table> </form> chappers; } else { echo <<<chappers <form action="{$_SERVER['PHP_SELF']}" method="post"> <table border="0" width="800" align="center" height="100"> <tr> <td align="center"><textarea name="userpost" type="description" cols="50" rows="5" STYLE="color: #FFFFFF; font-family: Verdana; font-weight: bold; font-size: 12px; background-color: #72A4D2;"></textarea></td></tr> <tr><input type='hidden' name='grp' value='{$_GET['grp']}'></td></tr> <tr><td align="center"><input type="submit" name="submitpost" value="Post"></td></tr> </table> </form> chappers; } ?> And if it doesn't work, say what's happening or not happening. You'll notice I've added echo "Right here... $_POST['grp'] ...there should be the grp field data"; and same for textarea field. That's to make sure that when you submit the form, everything's being sent across.
-
Thanks for that. Before I use it, is there no way to use a kind of 'permitted' list of words instead, so any words in the permitted list, whether they match parameters of the swearword list or not, get ignored? Another issue I've just come across is that although the text in the comment field seems to be stored in the MySQL database with line breaks where they should be, upon retrieving the text from the database and echoing it onto the page, all the line breaks have gone and the whole text is just one long, long sentence. How might I get around that? Thanks again.
-
Hi everyone, Wonder if someone could help with my swear filter please, I've written it myself but now reached extent of my limited abilities... This is shortened version of code: <form method='post' action='<?php echo $_SERVER['PHP_SELF'] ?>'> <table cellpadding='0' cellspacing='0' border='0'> <tr><td><textarea name='comment' rows='4' cols='80'></textarea></td></tr> <tr><td colspan='2' align='center'><input class='submitbutton' type='submit' name='submit' value='Post'></td></tr> </table> </form> <?php function SwearFilter($str) { $swearwords = array("arse", "ass", "bitch"); $replacement = "<span class='badwords'>[censored]</span>"; foreach ($swearwords as $swearword) { $str = eregi_replace($swearword, $replacement, $str); } return $str; } if (isset($_POST['submit'])) { $comment = $_POST['comment']; $comment = SwearFilter($comment); echo $comment } ?> Trouble with this is that a word from the swearword list can be contained within another word and still be found, i.e. class becomes "cl[censored]". I wasn't overly bothered about that until I found one result of this is that should the first word in the swearlist checked against the comment data find a match (so it finds "arse"), the string then has <span class='badwords'>[censored]</span> added to it, so when the function moves to the next word in the swearlist, which is "ass", it finds a match in "class" from the previous match and changes "<span class='badwords'>[censored]</span>" into "[censored]='badwords'>[censored]</span>". I realise I could simply put "ass" as the first word in the swearlist and then only people submitting the form with ass in it at any point gets censored rather than my "span class..." but what would be even better is if I could add a list of permitted words. The other option is to ensure only whole words matching those in my list get grabbed, but I'd rather have a permitted words list if that's possible? Thanks for any help or advice!
-
But within <td>s where I am using multiple <p>s one after another, wouldn't I then have a gap made up of standard <p> gap and the extra 5px set to bottom margin?
-
Hi everyone, After years of using tables, I'm having a mid-table crisis. It was after coming across this problem again: <table width="100%" cellpadding="0" cellspacing="0" border="0"> <tr> <td colspan="2"> <p class="text">Hello world.</p> <p class="text">And hello again in a new paragraph block.</p> </td> </tr> <tr> <td><p class="text">I want to show you a picture.</p></td> <td width="110" align="center"><img src=gimp.jpg"></td> </tr> <tr> <td colspan="2"> <p class="text">More text.</p> </td> </tr> </table> Now the trouble with the above: With just one <td> and all the text contained within different paragraphs <p></p> there is an empty line between each paragraph, separating them neatly. Since I want images at the side of some paragraphs, it means I have to do what the code above shows - end the initial <td> and create others so the image can go in its own. Trouble with that is that the neat gaps between paragraphs is lost and the text just displays in one large block. To add a space between paragraphs, I have to add <br> at the end of a paragraph before closing the <td> and beginning the next. That leaves a much bigger gap than the <p> normally gives and so looks bad. I can't see a way around it, so I got looking into divs again, even though I'd been warned before not to use them. I can't yet remember how to use them to display the code above as I'd want it, but regardless, is it the best way to go? Or is there an alternative to tables and divs? What's best? Someone please help! Thank ye, James
-
Hi, I know you can make an array containing multiple values, and print them, like this: $folder = array("hello", "world"); foreach ($folder as $contents) { echo "$contents<br>"; } and I know you can make an array in which you can keep adding single values, like this: $folder[] = "hello"; $folder[] = "world"; foreach ($folder as $contents) { echo "$contents<br>"; } but how do you make an array that you can keep adding multiple values to? This doesn't work, as it outputs the array() as "Array" followed by "and goodbye": $folder[] = array("hello", "world"); $folder[] = "and goodbye"; foreach ($folder as $contents) { echo "$contents<br>"; } and you can't add more than one value using the format $folder[] = "whatever", "whatever"); as it brings up a server error. Is there a simple answer? Thanks a lot!
-
[SOLVED] Image resize not returning jpeg image
Chappers replied to Chappers's topic in PHP Coding Help
Finally fixed this annoying fault! Here's what you need to do: Within Internet Explorer: Tools > Internet Options Go to Advanced tab Go to Reset Internet Explorer settings section Click on Reset... button Tick Delete Personal Settings box Click the Reset button Follow instructions that appear and restart IE. It is ESSENTIAL that you tick the box to delete personal settings, or the problem will remain! The above steps finally cured this problem. Original images displayed simply using img src="" were saving correctly, but any image, in any format, outputting from PHP imagecreatefromjpeg () and imagejpeg() or other formats (imagegif, etc), were saving as untitled.bmp when right-clicked and 'saved as...'. Hope this helps others! And thanks to all those who tried to help me with this. -
[SOLVED] Image resize not returning jpeg image
Chappers replied to Chappers's topic in PHP Coding Help
Tried your code but it's still happening in IE7. Must be a local problem then... -
[SOLVED] Image resize not returning jpeg image
Chappers replied to Chappers's topic in PHP Coding Help
I read somewhere that IE requires an image to be cached before it can correctly recognise the filetype. So I've downloaded a toolbar to show headers and stuff sent when on a webpage. Here's what came up for my test-upload page: HTTP/1.1 200 OK Date: Tue, 14 Oct 2008 20:33:35 GMT Server: Apache Cache-Control: no-store, must-revalidate, max-age=0, proxy-revalidate, no-transform Expires: Tue, 14 Oct 2008 20:33:35 GMT Pragma: no-cache Keep-Alive: timeout=1000, max=99971 Connection: Keep-Alive Transfer-Encoding: chunked Content-Type: image/jpeg No caching is turned on. Could this be the problem, and how do I change that behaviour to test if it is anyway? Thanks a lot to everyone trying to help. -
[SOLVED] Image resize not returning jpeg image
Chappers replied to Chappers's topic in PHP Coding Help
Hi, thanks for the replies. I have tried adding what madtechie suggested: header("Content-Disposition: filename=\"" . $filename . "\";" ); exactly as written above, since I'm using the variable $filename. Still no difference. Out of interest, I had already tried adding this: header('Content-Disposition: attachment; filename="newimage.jpg"'); to the file before posting on here, after Googling the problem. That also made no difference. I just always get this: http://i442.photobucket.com/albums/qq147/chappers27/sample.jpg -
[SOLVED] Image resize not returning jpeg image
Chappers replied to Chappers's topic in PHP Coding Help
I have made a test page so you can see it in action. The index page contains all information required, such as what browser I'm using, etc. There's a link on the page to go to the outputted resized image. As I say on the page, there is someone else on a forum suffering this problem who believes header information is lost or damaged during the resize and hence why this trouble occurs. Why doesn't everyone get the same trouble with these resize scripts then? http://www.demo.freephphostonline.com/ -
[SOLVED] Image resize not returning jpeg image
Chappers replied to Chappers's topic in PHP Coding Help
I've tried it and tried it. I don't know much about it all, but could it be how PHP is set up with the host I'm with? Incidentally, if I save it and try to open it with Paint Shop Pro in its image.bmp format, it opens properly. If I rename the image to end with .jpg, PSP won't open it, saying it isn't a jpeg image. -
Hi, I'm using this code to resize images: <?php // The file $filename = 'image2.jpg'; // Set a maximum height and width $width = 200; $height = 200; // Content type header('Content-type: image/jpeg'); // Get new dimensions list($width_orig, $height_orig) = getimagesize($filename); $ratio_orig = $width_orig/$height_orig; if ($width/$height > $ratio_orig) { $width = $height*$ratio_orig; } else { $height = $width/$ratio_orig; } // Resample $image_p = imagecreatetruecolor($width, $height); $image = imagecreatefromjpeg($filename); imagecopyresampled($image_p, $image, 0, 0, 0, 0, $width, $height, $width_orig, $height_orig); // Output imagejpeg($image_p, null, 100); ?> Thing is, when I right-click on the displayed image to save it, it's coming up as a bmp image. I want to tailor the code to save the file after resizing, but it needs to be in jpeg format. This code is from the PHP manual, so what's going wrong? Thanks!
-
Read about variable scope, and it's all clear now. Thanks for your help and time.
-
I've just spent this time reading about functions and practising with them, and I'm finally getting to grips with them to some extent, especially after you pointed out the obvious that things I use like isset() and so on are functions, but not defined by me. Never thought of it like that. Just out of interest, I discovered that when making a function, I had to define a variable to be used with it, like: function makebold($text) { $text = "<b>$text</b>"; return($text); } echo makebold($text); So $text is used, yet I can write: $phrase="hello world"; function makebold($phrase); And it will still output correctly. How come it replaces the preset variable $text with any variable I choose? And is there a way of writing functions without having to state a variable (just curious)? The other thing I found was that if I remove return($text); the function no longer works. I don't understand why, since the code for making the text bold is still present.
-
Thanks for the explanations, although I'm afraid they went over my head. But, thanks to you putting names to these different things (classes, objects) I've been able to find the relevant parts in the PHP manual - and boy, is there some learning for me to do. I think I got way over my head with this, and now the scary spectre of classes and objects awaits my poor, unprepared brain. Still trying to grasp how to use functions...
-
Hi, Just a quick question. Have found some code using things like: $this->current_step = (integer) $step; and wondered what -> means, and what (integer) does as I haven't seen a plain word just written in brackets like that. I've also seen this: ^ used and don't know what it does, and words preceded by an ampersand, like this: $installer = &new Installer(realpath('.')); which I also don't understand. Searched the PHP manual but can't find reference to any of these? Thanks!
-
Viability of realtime chat room coded in PHP only?
Chappers replied to Chappers's topic in PHP Coding Help
Thanks for the info, I had a feeling it wouldn't be possible with PHP alone. Sorry for being dim, but I really do know nothing about javascript and the like...what is the framework for? Is it for offline testing, etc? I think there's a big learning curve ahead of me. -
Hi everyone, Just wondering if coding a realtime chat room solely in PHP is viable? I know most use Java, but I can't learn that and the free ones have too many limitations. By realtime I mean that what you type in the box appears instantly on the screen along with other users input. I'm not asking for anybody to do it for me, etc., just as to whether it's possible but incredibly hard, or just impossible altogether. Thanks.