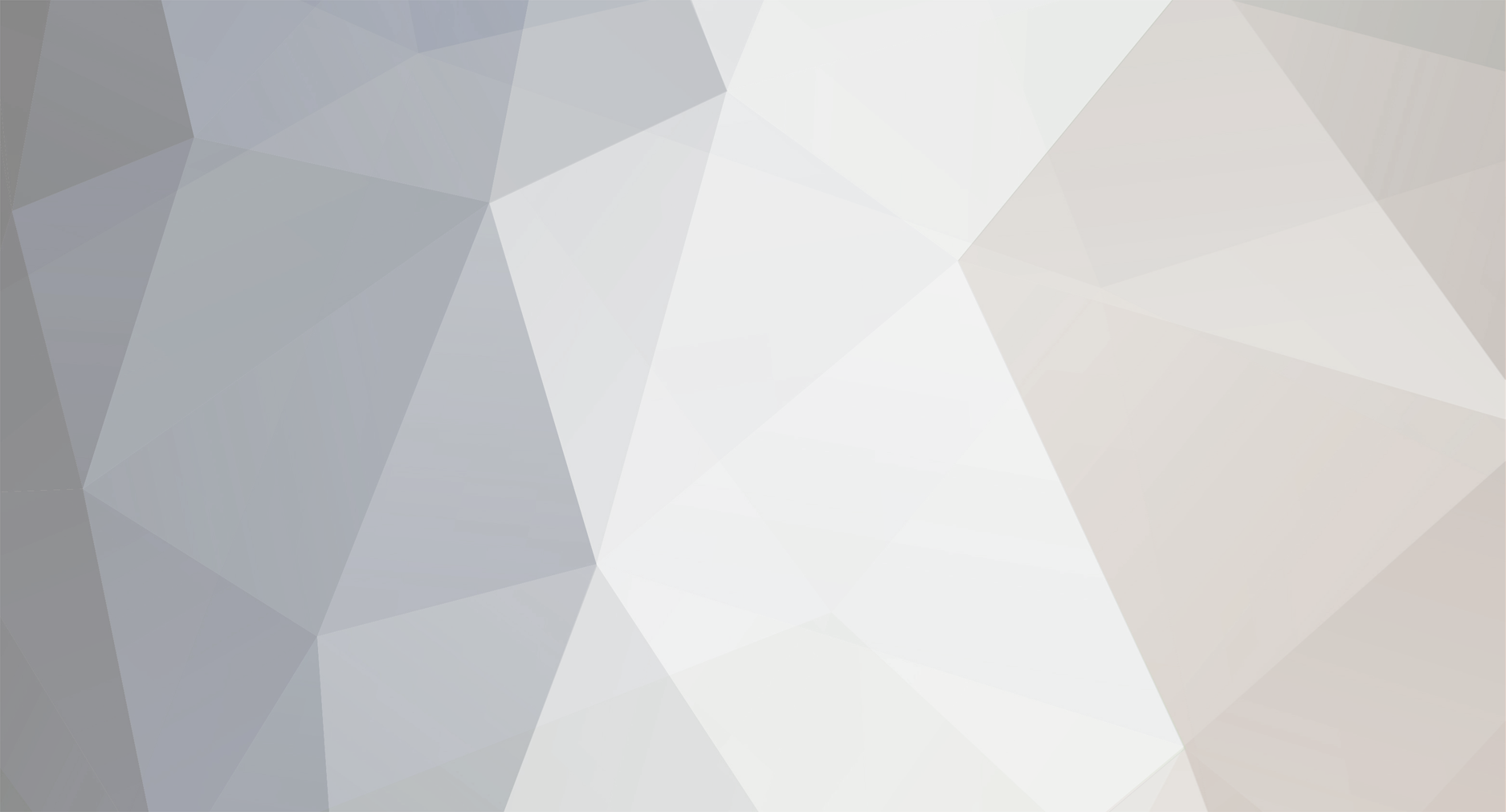
paul2463
-
Posts
955 -
Joined
-
Last visited
Never
Posts posted by paul2463
-
-
Have a look at this site
http://www.phpfreaks.com/MySQL_Reference/Column-Types/2.php
hope it helps
other than that read the manual -
Nothing to do with PHP. No server side language can determine that
without attempting to setting a cookie then having the client do another
request. -
still missing the semi-colon at the end of the implode line, not a question mark
-
http://phpclasses.dotcommers-uk.com/browse/package/1870.html
Active Calendar Class ( Download all files at the bottom of the page when you have finished seeing what it can do for you)
there is a downlaod all files link, save you getting 20 or 30 files yourself -
Hi , Sorry about that maybe I should have checked it before posting it, I have modified it so it works now, here is the code, it takes a date string as the input now in the form of the example at the bottom again
[code]
<?PHP
function formatetimestamp($until){
$months = 0;
$weeks = 0;
$days = 0;
$now = strtotime("now");
$difference = $until - $now;
$test = floor($difference/2592000);
if($test > 0){
$months = $test;
$difference = $difference - ($months*2592000);
}
$test = floor($difference/604800);
if($test > 0){
$weeks = $test;
$difference = $difference - ($weeks*604800);
}
$test = floor($difference/86400);
if ( $test > 0) {
$days = $test + 1;
$difference = $difference - ($days*86400);
}
$output = "You have to wait $months Months, $weeks Weeks, $days Days until this Day";
return $output;
}
echo formatetimestamp(strtotime("31 august 2006"));
?>
[/code]
try it now
again it is not extremely accurate because is predicated on all months having 30 days, to keep it simple, it gives a good idea though -
try this $until needs to be a timestamp and not a date though, see example at the end ( This is based on months only having 30 days though)
[code]
function formatetimestamp($until){
$now = time();
$difference = $until - $now;
$months = floor($difference/2592000);
$difference = $difference - ($months*2592000);
$weeks = floor($difference/604800);
$difference = $difference - ($weeks*604800);
$days = floor($difference/86400);
$difference = $difference - ($days*86400);
$output = "You have to wait $months Months, $weeks Weeks, $days Days until this Day";
return $output;
}
//int mktime ( [int hour [, int minute [, int second [, int month [, int day [, int year [, int is_dst]]]]]]] )
echo formatetimestamp(mktime(0,0,0,12,31,2006)); //output: e.g "You have to wait 5 Months 1 Week 5 Days until this Day"
[/code]
hope that helps
-
You could of course update everything at once by installing <a href="http://www.apachefriends.org/en/xampp.html">XAMPP</a> , it is an apache server with php mysql perl pear and lots of other things all in one package with a windows installer version.
-
I use a fantastic server for my standalone pc to practice writing php / mysql / pear / perl all running on an apache server, its an all in one package called <a href="http://www.apachefriends.org/en/xampp.html">XAMPP</a> ( the linux version used to be called LAMMP)
-
the cronjob that I set up on one of my sites was written purely in PHP as it is a server side task. write the page as what you want to happen at regular intervals, like email your friend etc etc, call it something.php and then either speak to your server provider or set up the cron yourself saying that at 1am every day I want something.php to be run.
you can link it to other pages by using includes statements but dont link the other way or people can find your cronjob script and keep calling it slowing your server running scripts that you dont want to happen
hope that helps. -
Hi you could try array_filter() without a callback function, if i got this right from the manual, taking your example above and call the array $yourArray we can get
[code]
$myArray = array_filter($yourArray);
print_r($myArray); [/code]
would give
[code]
array
(
[0] = blue
[1] = red
)[/code]
so then you would just have to look for the last item in $myArray as it will not be NULL, because array_filter() will remove all the entries of input that are equal to FALSE i.e NULL
hope that helps
-
Not being that experienced in mySql, I have had a quick look for you and I think I may have spotted your problem
lines 14 - 17 connect to your database server
your line 18 is trying to insert into the database, but you are not yet connected to the database untill line 19
try this
[code]<?php
$username = $_POST['username'];
$password = $_POST['password'];
$email = $_POST['email'];
$picture = $_POST["picture"];
$ip = $REMOTE_ADDR;
$username = strip_tags($username);
$email = strip_tags($email);
$picture = strip_tags($picture);
$password = md5($password);
if ( !$username || !$password || !$email || !$picture) {
die( "All fields are required!" );
}
$connect = mysql_connect( "x", "x", "x" );
if ( ! $connect ) {
die( "Could not connect to SQL server" );
}
mysql_select_db( "cydrive_test", $connect ) or die ("Could not open database:" .mysql_error() );
$insertstatement = "INSERT INTO Users VALUES ('$username', '$password', '$email')";
$selectresult = mysql_query( "SELECT * FROM Users WHERE username=’$username’", $connect );
$numrows = mysql_num_rows( $result );
if ( $numrows > 0 ) {
print "The username you have chosen is in use. Please choose another one.";
} else {
mysql_query($insertstatment, $connect )or die( "Couldn't add data to table" );
print "Registration successful! Login <a href="login.php">
here</a>";
}
?>[/code]
-
[code]
<html><body>
<script language="javascript">
var $i=5;
<?php $k = $i;?>
</script>
</body></html>[/code]
when you create the javascript variable, make it readable as a variable by PHP by using the PHP standard method of giving it a $ at the beginning. -
Have you defined the DateAdd() function anywhere in your program
as far as I am aware there is no pre-defined function of that name in PHP.
-
[quote]Code:
<?php
$nowdate = strtotime("now"); // creates a date string of todays date
$newdate = strtotime("+2 day", $nowdate); // adds 2 days onto that date string
$mydate = date("d-m-Y", $newdate) //creates a new date object from that string (03-08-2006)
?>
is one way to do it
This can be done much more simply:
Code:
<?php
$mydate = date('Y-m-d',strtotime('today +2 days'));
?>
Ken [/quote]
thanks for that Ken but I spilt it into three tasks to identify what each step actually did, once each step is understood then the shortened version could be used.
Paul
-
[code]
$nowdate = strtotime("now"); // creates a date string of todays date
$newdate = strtotime("+2 day", $nowdate); // adds 2 days onto that date string
$mydate = date("d-m-Y", $newdate) //creates a new date object from that string (03-08-2006)
[/code]
is one way to do it -
hello Again
just in case anyone else was having this problem and also could not figure out what was causing the error. I have managed to sort it out and keep at least some of my hair in the process, the problem with the code I posted, WAS NOTHING, it was doing exactly what I asked it to, it checked the first two values, returnval = true (they are not the same) it then compared the next two, returnval = false( they are the same) then it checks the final two, returnval = true because they are different, do therefore the program checks everything and if the final two values are different it always returned true. what I needed wasa method for it to break out of the loop if a match was found returning false here is the way to do that:
[code]
function compare(){
var BPDates = PHPDateArray(); //function works fine
var JSDates = JSDateArray(); //function works fine
var joinArray = BPDates.concat(JSDates); //contains: 2006-07-12,2006-07-13,2006-07-13,2006-07-14 (middle 2 the same)
var returnval;
for (i=0; i<joinArray.length; i++) {
for (j=i+1; j<joinArray.length; j++) {
if (joinArray[i]==joinArray[j]) {
returnval = false;
break;
}
else{
returnval = true;
}
}
}
return returnval;
}[/code]
notice the BREAK Statement after any values are the same and returnval was set to false, this kicks it out of the loops stopping any more comparisons that may return TRUE.
Well there we go, an answer to a problem now solved.
Paul -
Hello,
I have written a function to compare information in an array that doesnt seem to work properly. here is the code:
[code]
function compare(){
var BPDates = PHPDateArray(); //function works fine
var JSDates = JSDateArray(); //function works fine
var joinArray = BPDates.concat(JSDates); //contains: 2006-07-12,2006-07-13,2006-07-13,2006-07-14 (middle 2 the same)
var returnval;
for (i=0; i<joinArray.length; i++) {
for (j=i+1; j<joinArray.length; j++) {
if (joinArray[i]==joinArray[j]) {
returnval = false;
}
else{
returnval = true;
}
}
}
return returnval;
}[/code]
It keeps returning TRUE even though there are two slots with the same data in
I have tried adding some other code to it such as:
[code]
if ((joinArray[i].toString())==(joinArray[j].toString()))
[/code]
but that doesnt seem to make it pick up the fact that the middle two bits of data are the same, as a date and a string in this case, can anyone spot my problem please?
-
Hello People
I am trying to write a function that produces a string variable in a form, that if it is written to html, it will be accepted by javascript for an array object, the format that is accepted is as follows
[code]"Saab","Volvo","BMW"[/code]
my function code is as follows
[code]
function arraytojs($array){
$str = "";
foreach ($array as $val):
$str .= "'".$val."',";
endforeach;
$str = rtrim($str, ",");
return $str;
}[/code]
my problem is that this produces a string in the form of
[code]
'Saab','Volvo','BMW'[/code]
I would like the single quotes to be double quotes that will make it compatible to a javascript array call, but when it try and rewrite the function with double quotes in the place of the single quotes I get errors thrown up, I am struggling to figure it out. any help please? -
Hello,
I am not after specific answers for this question as I like trying to work things out for myself, all I need is a bit of direction. Here is my question. I have a PHP/MySQL query result set, if I place these results in an array object, can this object be passed to a javascript function which also has an array object so they can be compared? if the answer is yes can someone point me to the relevant pages of a relevant manual for the answers. many thanks
Paul -
Hi to place the code box around the code you have to use the code tags which are difficult to show as they poroduce a code box if typed so I will place them inside some quotes to try and show them, here we go
[code]"code" and to end "/code"[/code]
replace the quote marks with square brackets such as [****]
[quote]and to make it a quote and not a code box place the word "quote" inside the square brackets without the quote marks[/quote] -
Hi it is quite difficult to answer without seeing the folder make ups, if they are both in the same folder i.e htdocs/supplier then the include statement should be
[code]
include ("supplierfunctions.php");
[/code]
note the inclusion of the .php which your origonal include did not have
if they are in different folders i.e the calling page is not in the same folder as supplierfunctions.php you could try
[code]
include ("../Supplier/supplierfunctions.php");
[/code]
see how you get on -
I have even now changed it for a FOR loop, I have claculated the number of days difference between the start date and end date and use that in the loop, here is the code
[code]
var sDate = new Date(sy,sm,sd);
var eDate = new Date(ey,em,ed);
var dates = new Array();
var num = (eDate - sDate)/86400000;
for (i=0 ; i<=num ; i++) {
dates[i] = sDate;
sDate.setDate(sDate.getDate()+1);
}[/code]
I still get an array object that is the correct length but it is filled with the same date again, the day after the end date, crazy eh? why isnt it doing what I ask, which is to place sDate into slot i then add a day onto it then place the new sDate into the next slot? -
Thanks will give that a go
OK tried it but I get exactly the same result, I must admit that I dont understand how the origonal solution didnt work, but it didnt, anyone else got any ideas please -
Hi here is the code that will create the table for you:
[code]
CREATE TABLE `comments_visitors` (
`id_comm` int(11) unsigned NOT NULL auto_increment,
`idart_comm` int(11) unsigned NOT NULL default '0',
`text_comm` varchar(255) NOT NULL default '',
`idusr_comm` int(11) unsigned NOT NULL default '0',
`date_comm` date NOT NULL default '0000-00-00',
`valid_comm` tinyint(1) unsigned NOT NULL default '0',
PRIMARY KEY (`id_comm`)
)[/code]
hope that helps as well
newbie here
in MySQL Help
Posted