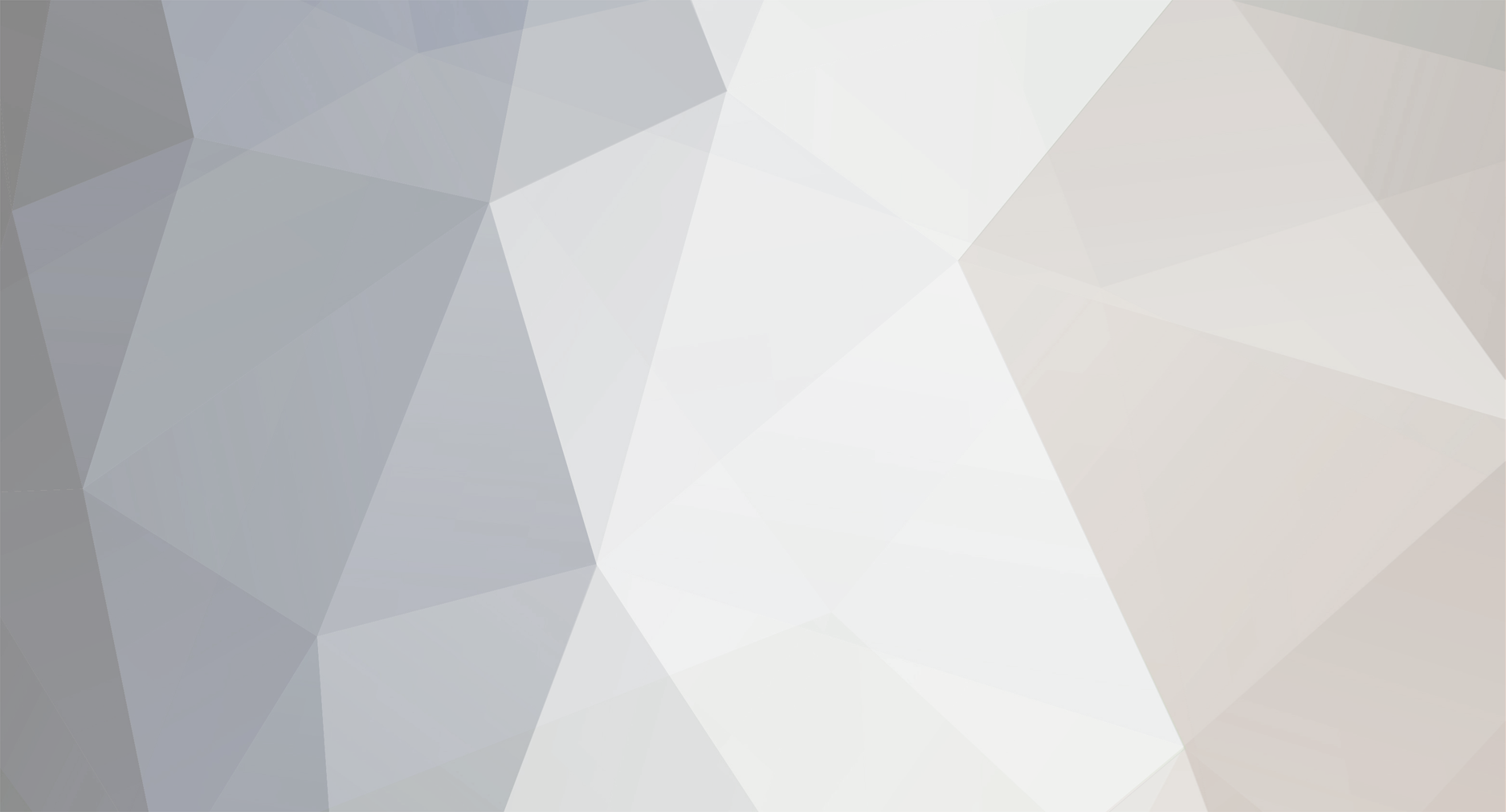
paul2463
-
Posts
955 -
Joined
-
Last visited
Never
Posts posted by paul2463
-
-
or if you are pulling them from the database you could use:-
$query = "SELECT DATE_FORMAT(dateColumn,'%c-%d-%Y') as FDate from tablename";
-
the error does not match the query you dont have a Phone or OwnerID in your query, which query is throwing that error??
-
you would not call the function direct, you would post the values back to the page and have the function at the top of the page, first of all checking to see if the posted value match what is needed
if (isset($_POST['purchase'])) { $amended = reduceItem($_POST['id'], $_POST['qty']); if ($amended == 1) { echo "Table updated"; } else { echo "For some reason table not updated"; } unset $_POST['id']; // to prevent refresh unset $_POST['qty']; }
-
yes you would pass the function the ID number of the item you want to change, I chose 14 as it is a nice number and I like it and it has no bearing on your problem
-
fora start off you can remove the semi colon from the "While($row" line it will stop it working
-
something like this??
function reduceItem($id, $qty) { $query = "UPDATE tablename SET quantity = quantity - '$qty' WHERE tableid = '$id'"; if ($result = mysql_query($query)) { $rows = mysql_affected_rows($result);//should be equal to 1 or 0 as an id is being used } else { $rows = 0; // unable to carry out query set affected rows to 0 } return $rows; } $amended = reduceItem(14, 1); //reduce item 14 by 1 echo $amended; //1 if the reduction has happened, 0 if no rows affected or error with the query
-
change this line
$account = mysql_query("SELECT * FROM members WHERE MemberRef='$MemberRef' AND Password='$Password'");
to read
$account = mysql_query("SELECT Password FROM members WHERE MemberRef='$MemberRef' ");
so it pulls the password for that selected MemberRef, before you were checking to see if both the memberRef and Password existed, this would never happen if you input a wrong password
hope that helps
-
if you are checking it against the string "HOME" then that is the way it should look
-
change this bit
if (isset($_POST['submit']) && $error_stat == 0) { $account = mysql_query("SELECT * FROM members WHERE MemberRef='$MemberRef' AND Password='$Password'"); if ($MemberRef != $Password) { $error_stat = 1; //Set the message to tell the user to enter a username $Password2_message = '*Incorrect Password*'; } }
to this, i have put some comments in so you should be able to follow it through
if (isset($_POST['submit']) && $error_stat == 0) { $account = mysql_query("SELECT * FROM members WHERE MemberRef='$MemberRef' AND Password='$Password'"); $numrows = mysql_num_rows($account); //get rows returned if ($numrows > 0)// if more than 0 its in database, if not throw new error message { $row = mysql_fetch_assoc($result); $dbpassword = $row['Password']; //get the password from the database if ($dbpassword != $Password) { // check it agains the inputted password, if not the same $error_stat = 1; //Set the message to tell the user to enter a username $Password2_message = '*Incorrect Password*'; } } //new error message $error_stat = 1; $new_message = "MemberRef and Password do not exist"; }
-
thats maths syntax to make that happen and I dont know what it is it may be
$query = "UPDATE tablename SET pricecolumn = pricecolumn - ((pricecolumn/100)*15)";
-
i am confused as to what you are checking....
so $MemberRef and $password come from the post variable
then you run a query to select everything from the database that match the two variables
then you check to see if $MemberRef does not equal $password
are you trying to get the password from the database and check to see if the entered password matches the one in the database?
-
you would do the same maths for adding 15% to do the subtraction
$query = "UPDATE tablename SET pricecolumn = pricecolumn - 15%"; //however you do that math
-
where do you set the variables $MemberRef and $Password
-
$query = "UPDATE tablename SET pricecolumn = pricecolumn * 1.15";
-
try this then, you will notice I have added an "or die" statement to the queries to make sure they are doing what they should and are not failing inside the function and also changed the name of each query and corrsponding calls to that query to save any possible mix up of data
function fcsteaches() { include 'config.php'; //Connect to apache server(MySQL) and select database. mysql_connect("$host", "$username", "$password")or die("cannot connect"); mysql_select_db("$db_name")or die("cannot select DB"); //get the avg eaches per bay $query1 = "SELECT quantity FROM frcstqunt"; $result1 = mysql_query($query1) or die ("Error in query 1" . mysql_error()); while ($row1 = mysql_fetch_assoc($result1)) { $arrayqunt[] = $row1['quantity']; } //sort the array values rsort($arrayqunt); //get number of locations from currentlocations $query2 = "SELECT * FROM currentlocations"; $result2 = mysql_query($query2) or die ("Error in query 2 " . mysql_error()); $numrows2 = mysql_num_rows($result2); //slice array so only top movers are left $arrayslice = array_slice($arrayqunt, 0, $numrows2); //sum array $arrayfinal = array_sum($arrayslice); //get number of bays from locsperbay $query3 = "SELECT * FROM locsperbay"; $result3 = mysql_query($query3) or die ("error in query 3 . mysql_error()); $numrows3 = mysql_num_rows($result3); $avgfcsteaches = $arrayfinal/$numrows3; return $avgfcsteaches; }//end function ?>
-
your problem is that you are not assigning the return value to a variable
try something like this
<?php $answer = fcsteaches(); echo $answer; ?>
-
write a small javascript function to create a popup, there are many examples on google etc, then get the function to run onHover()
<a href="http://www.yourhtmlsource.com/javascript/popupwindows.html"> Have a look here </a>
-
I think the problem is that you cannot have a function send header information from within the function, unless you can put the "exit;" after the header call. it seems that the answer to the function is "false" and therefore it is sending that header data, then it comes back from the function and tries to send the header info again. it would be better if your function just returned either true or false and then based on that answer send the user to where he/she needs to go
if ($returnvalue == FALSE) { header('Location: http://www.runningprofiles.com/members/error.php'); } else { header('Location: http://somewhereelse.php'); }
-
what is function.php line 57?? that is what is outputting something , even if its an error signal it is an output sent to the browser and the header function will not work
-
move the style below the includes
<?php ini_set('error_reporting', E_ALL); session_start(); include ("../header.php"); require_once '../settings.php'; $id = $_SESSION['user_id']; ?> <style type="text/css"> <!-- body { margin-left: 1px; margin-top: 1px; margin-right: 1px; margin-bottom: 1px; } --> </style> <table colspan='0' width="100%" cellpadding="0" bgcolor="#FFFFFF"> <tr> <td width="13%" height="505" align="center" valign="top"><table width="100%" height="505" align="center" bgcolor="#D6E0E0"> <tr> <td height="58" colspan="2" align="center" valign="top" bgcolor="#D6E0E0"><p>Menu</p> <p><? if($id == 1){ echo "<a href=\"admin/index.php\">Admin Index</a>\n";}?></p> </td> </tr> <tr> <td height="361" colspan="2" align="center" valign="top" bgcolor="#D6E0E0"><a href="http://www.runningprofiles.com/logout.php">Logout</a> </td> </tr> </table> </td> <td width="87%" align="left" valign="top"> <?php if (isset($_GET['section'])) { $section = $_GET['section']; } else { $section = 'main'; } $file = "include/".$section.".php"; if (file_exists($file)) { require($file); } ?> </td> </tr> </table> </td> </tr> </table>
-
you are missing a closing single speech mark on
$change = $_POST['change];
-
if you are doing a second check i believe you have to use elseif because as far as I was aware it went
if (bananas are yellow) { eat them } else { there are any colour than yellow and are not fit to eat }
if you wish to add another check it should be
if (bananas are yellow) { eat them } elseif (bananas are green) { leave them a while then eat them } else { there are any colour than yellow or green and are not fit to eat }
hope that helps
-
if ( in_array ( get_level_access ( $_SESSION['user_id'] ), $kt ) ) { $access = TRUE; } else //what was this other check for?? { echo "error"; }
-
too many braces
if ( in_array ( get_level_access ( $_SESSION['user_id'] ), $kt ) ) { $access = TRUE; } else( $access ==TRUE ) { echo "error"; }
[SOLVED] echo timestamp as dd/mm/yyyy
in PHP Coding Help
Posted
read my first post and also read the mysql manual on the DATE_FORMAT() function