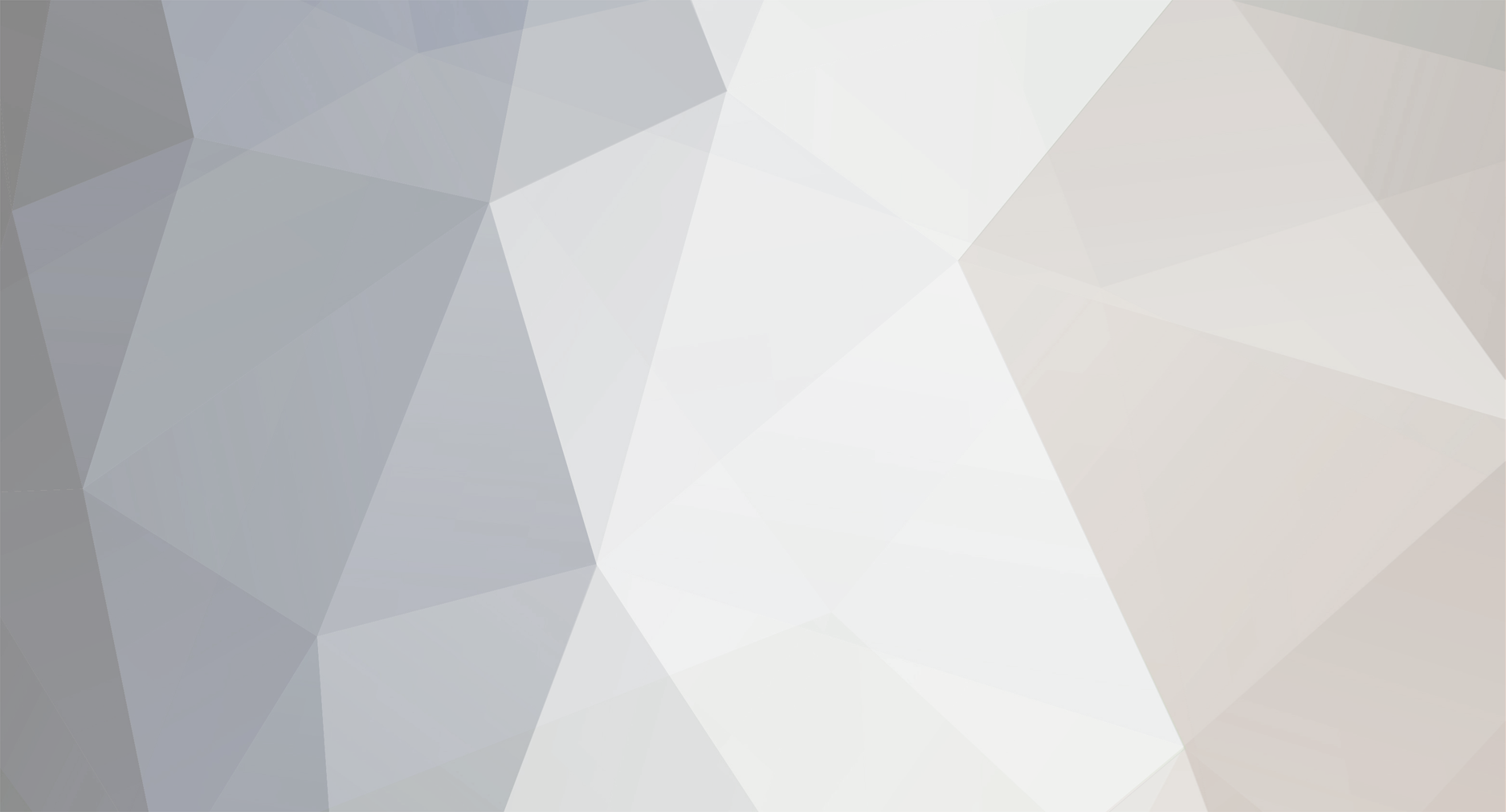
AncientSage
Members-
Posts
56 -
Joined
-
Last visited
Never
Everything posted by AncientSage
-
Hello, I'm in need of knowing how to get data from one field, and from another, and so that they are associated. For example... [code] CREATE TABLE config ( config_name varchar(255) NOT NULL, config_value varchar(255) NOT NULL, PRIMARY KEY (config_name) ); INSERT INTO config (config_name, config_value) VALUES ('max_file_size', '10000'); [/code] I'd want to get config_name's row max_file_size, and then have it draw the value 10000 out associated with it. This is what I have so far... [code] SELECT config_name, config_value FROM config WHERE config_name = "max_file_size" ORDER BY config_name [/code] Will it work because it is primary key? Or do I have to do something else?
-
Yes. Here is the script... [code] <html> <head> <title>PHP Quiz</title> </head> <body> <?php //Check to see if null values were submitted... if($_POST['question1'] == "" || $_POST['question2'] == "") { ?> <form action="quiz.php" method="POST"> <b>Is php a programming language?</b><br> <select name="question1"> <option value="" selected>Select An Answer</option> <option value="yes">Yes</option> <option value="no">No</option> </select> <br> <b>Ok, now, is C?</b><br> <select name="question2"> <option value="" selected>Select An Answer</option> <option value="yes">Yes</option> <option value="no">No</option> </select> <br> <input type="submit" value="Submit Questions!"> </form> <?php } else { /*Variables, $x records questions correct. $y is the number of questions. $z is ammount of questions wrong.*/ $x = 0; $y = 2; $z = 0; //Check the values of the select questions. if($_POST['question1'] == "yes") { $x++; $q1is = "<br />Question 1: RIGHT"; } else { $q1is = "<br />Question 1: WRONG"; } if($_POST['question2'] == "yes") { $x++; $q2is = "<br />Question 2: RIGHT"; } else { $q2is = "<br />Question 2: WRONG"; } ?> <!--Some random HTML can go here...such as styling the page, leave the php inbetween these two comments--> <?php if($x<1){$msg="You need to study more.";} if($x>0 && $x<2){$msg="Nice, but you could do better.";} if($x==2){$msg="Perfect!";} echo 'You got '. $x .' out of '. $y .' questions correct. '.$msg; echo $q1is; echo $q2is; ?> <!--Some random HTML can go here...such as styling the page--> <?php } ?> </body> </html> [/code] That should output what you are asking.
-
I'm assuming you know basic PHP, if that be the case, then you should be able to understand and modify this. I'm using 2 questions for an example. [code] <html> <head> <title>PHP Quiz</title> </head> <body> <?php //Check to see if null values were submitted... if($_POST['question1'] == "" || $_POST['question2'] == "") { ?> <form action="quiz.php" method="POST"> <b>Is php a programming language?</b><br> <select name="question1"> <option value="" selected>Select An Answer</option> <option value="yes">Yes</option> <option value="no">No</option> </select> <br> <b>Ok, now, is C?</b><br> <select name="question2"> <option value="" selected>Select An Answer</option> <option value="yes">Yes</option> <option value="no">No</option> </select> <br> <input type="submit" value="Submit Questions!"> </form> <?php } else { //Variables, $x records questions correct. $y is the number of questions. $x = 0; $y = 2; //Check the values of the select questions. if($_POST['question1'] == "yes") { $x++; } if($_POST['question2'] == "yes") { $x++; } ?> <!--Some random HTML can go here...such as styling the page, leave the php inbetween these two comments--> <?php echo 'You got '. $x .' out of '. $y .' questions correct.'; ?> <!--Some random HTML can go here...such as styling the page--> <?php } ?> </body> </html> [/code] Note: This should be stored in a file named quiz.php, unless the form action is changed.
-
Well, the data hasn't been unserialized when it was stored into the $rowsarr variable, would it need to be re-serialized when stored back in? As well, I don't have a need to implement the implode future yet, as I've not added any form of template to it. The data is displayed as... something|something|something At the moment, when I do integrate some form of template, or more features to the script I am writing, I'll explode and implode on the | as well.
-
I like the idea of the site, an editable wiki for programmers. Now, for the HTML section, I would recommend changing it a bit... No need to give each small element their own page, just create an HTML elements page, and put them in that. Or something to that effect. Anyway, I'm bookmarking the site, I'd recommend what everyone else has so far as well.
-
Yes, that was a problem, thanks for finding it. However, it's still not unsetting the variable, or, at least, not writing it to the text file correct. As it re-writes the entire file (how I have it set), but it's all blank, no text is actualy written. Here is the function I'm calling... [code] function delete_arr_element() { global $root; $textfile = $root . "filelist.txt"; if(isset($_POST['checksubmit'])) { if(isset($_POST['rowsarr'])) { if(isset($_POST['row'])) $rowsarr = $_POST['rowsarr']; foreach($_POST['row'] as $i) unset($rowsarr[$i]); $fp = fopen($textfile, "w"); flock($fp, LOCK_EX); fwrite($fp, $rowsarr . "\n"); flock($fp, LOCK_UN); } } } [/code]
-
[code] foreach($rows as $x => $row) { $arr = unserialize($row); if($arr == ""){ continue; } echo '<input type="checkbox" name="row[]" value="'. $x .'">' . $arr . "<br /> "; echo '<input type="hidden" name="rowarr" value="' . $rows . '">'; echo "<input type=\"hidden\" name=\"checksubmit\">"; echo "<br /><input type=\"submit\" value=\"Delete Selected\">"; echo "</form>"; } [/code] $rows contains a few arrays, it's gathering the from a text file. It's supposed to gather the array, and put it into a text file, that's all good, except this little array problem. So the hidden input would be a string? Do I need to change rowarr to an array, or is it an array by default? Edit: I attempted to put this as... rowsarr[] for the name attribute, will that work?
-
Hello, I believe I am doing this correctly...anyway... [code] $postrows = $_POST['rowsarr']; if(isset($_POST['row'])) foreach($_POST['row'] as $i) unset($postrows[$i]); [/code] Returns me this error: Fatal error: Cannot unset string offsets in /root/includes/functions.php on line 46 I get the same error if I don't assign $_POST to a variable. Before I assigned post to a variable, I had this for the unset... unset($_POST['rowsarr'][$i]) Note: In the form, a variable containing an array is submitted as the value, would this effect anything?
-
That's what I attempted earlier, I must've done something wrong though, as it didn't work last time I attempted. Anyway, thanks for the help, and it's working now after applying it to the code the way you posted.
-
It's not displaying any now, here is the code... [code] $fp = fopen($textfile, "r"); $file = file_get_contents($textfile, False, NULL); $u_rows = unserialize($file); $rows = explode("\n", $u_rows); delete_row($rows); //Pay no attention to this, it doesn't effect the overall code. } echo "<form action=\"" . append_sid("admin.php") . "\" method=\"POST\">"; foreach($rows as $x => $row) { if($row == ""){ continue; } echo '<input type="checkbox" name="row[]" value="'. $x .'">' . $row . "<br /> "; } echo "<input type=\"hidden\" name=\"checksubmit\">"; echo "<br /><input type=\"submit\" value=\"Delete Selected\">"; echo "</form>"; [/code]
-
I have multiple arrays stored in a text file, they're serialized, so if I don't unserialize them, they come back in the serialized form. Anyway, I've unserialized them, and attempted to make it so they work, but now it's only returning one of the arrays in my foreach loop. As if it's only unserializing one array, and getting rid of the rest... Example... s:40:"text "; s:40:"text "; s:40:"text "; Now, it's only returning the first line, I need it to unserialize all 3 arrays, and display them all. Any ideas how that would be done? Do I need to use the explode function before or after I unserialize?
-
Array not writing to file as expected...
AncientSage replied to AncientSage's topic in PHP Coding Help
+1 rule of PHP I did not know (or more so, of the serialize() function). I guess that would be my problem then, as it is storing it as it should now. Thanks. -
I'm serializing the array, and all, but it's writing it back to the text file as "Array". [code] $textfile = "filelist.txt"; if (file_exists($textfile) && is_file($textfile)) { $fp = fopen($textfile, "r"); $file = file_get_contents($textfile, False, NULL); $rows = explode("\n", $file); if(isset($_POST['checksubmit'])) { if(isset($_POST['row'])) foreach($_POST['row'] as $i) unset($rows[$i]); serialize($rows); $fpw = fopen($textfile, "w"); flock($fpw, LOCK_EX); fwrite($fpw, $rows); flock($fpw, LOCK_UN); } } echo "<form action=\"" . append_sid("admin.php") . "\" method=\"POST\">"; foreach($rows as $x => $row) { if($row == ""){ continue; } echo '<input type="checkbox" name="row[]" value="'. $x .'">' . $row . "<br /> "; } echo "<input type=\"hidden\" name=\"checksubmit\">"; echo "<br /><input type=\"submit\" value=\"Delete Selected\">"; echo "</form>"; } [/code] Now, as you can see, I'm using explode() on part of the array, not sure it that could cause part of the problem. Anyway, what is wrong in this code that would be causing the array to keep writing back as Array?
-
Thanks for the help everyone, it's working now.
-
Yes, as seen in my code... echo "<input type=\"checkbox\" name=\"" . $x . "\">" $x is auto-incremented, and there are multiple input fields. I need to somehow find the name attribute out, and then allow it to be accessed by the $_POST global variable.
-
Hello, I'm attempting to contain a variable number within an array, for example... $array[$x] $x is a number, the number is sent through a form. However, since I do not know that number, I am unsure of how to put it into the array slot. $array[$_POST[$x]] Won't work, perhaps if I pass it via a GET method or something it will work? Or is there another way? Perhaps it's because $x is no longer in the script once it is executed, $x is in the name element of a form. My code... [code] $textfile = "filelist.txt"; if (file_exists($textfile) && is_file($textfile)) { $fp = fopen($textfile, "r"); $file = file_get_contents($textfile, False, NULL); $rows = explode("\n", $file); if(isset($_POST['checksubmit'])) { unset($rows[$x]); } $x = 0; echo "<form action=\"" . append_sid("admin.php") . "\" method=\"POST\">"; foreach($rows as $row) { if($row == ""){ continue; } echo "<input type=\"checkbox\" name=\"" . $x . "\">" . $row . "<br /> "; $x++; } echo "<input type=\"hidden\" name=\"checksubmit\">"; echo "<br /><input type=\"submit\" value=\"Delete Selected\">"; echo "</form>"; } [/code]
-
'k, thanks. Now, I have one more question... In a foreach loop, is it possible to exclude the last row? (Which is, blank.)
-
Hello, Currently, I need to create a script that deletes a select row from a text file, at each \n character, that would mark a row... Now, let's say I have this for information... (in a text file) text|somemoretext|finaltext ytext|ysomemoretext|yfinaltext I'd want my script to display that, which it does, but I'd also want the row number returned to the script, so it would know which row to delete. Any ideas how I'd do this? I could just use write, and re-write all the rows updated to the file, but if there is a way to just delete a row, then I'd like to take that path. Thanks.
-
[quote author=SemiApocalyptic link=topic=100595.msg397228#msg397228 date=1152907873] I think you can with PHP 5 [/quote] 'k, looks like I'll have to use PHP 5 then. Thanks.
-
Hello, Is it possible to use the file_exist() function to check if a file exist via FTP, as I need to make sure a file exist on an external server? Thanks.
-
Hello, I want to store php code inside a php variable, but not have it executed until called by the eval() function, if that is, how you would do it. However, I'd guess you put quotes around the code you want to execute later, but my code has some quotes in it, therefore, I must use a back-slash to escape them, that's where I'd guess I run into the problem. Does eval() execute the back-slashes, or do I have to use the explode (or implode, whichever is needed) function to make it work correctly? For example... [code] $upload = 'ftp_put($conn, "/".$_FILES[\'ufile\'][\'name\'], $workDir."/".$tmpName, FTP_BINARY);'; eval($upload); [/code] Is there even a need to put quotes around the code to make it so it does not execute when the variable is read? I've never used the eval() function before on another note...
-
Thanks, and I should be able to take it on my own from here, the preg_match function seems to be able to do what I want it to do.
-
Well, actualy... I want php to find links if there are any, then find what is assigned to the href attribute, that being, the URL. For example, let's say someone enters the following HTML into a text-area... {a href=http://www.phpfreaks.com/}Click Here{/a} (apparently, this forum allows HTML, so...replace { & } with the greater/lesser than symbols when reading) I'd want php to find what is assigned to href, then for example, if it contains the characters php, then I'd want script to end and return an error.
-
Hello, Currently, I'd like to check a text area to see if there are any links, if so, check the href attribute for certain characters, and quit the script if they have those certain characters... I'm guessing it would involve searching for "href" and all text assigned to href would then be checked by some php function, however, I don't know which functions would be used for any of this, if someone could list the functions needed, I'll do the research on them. Thanks.
-
Whoops, that might've been my problem, 'cept I've upped the value and removed it, yet it still doesn't work.