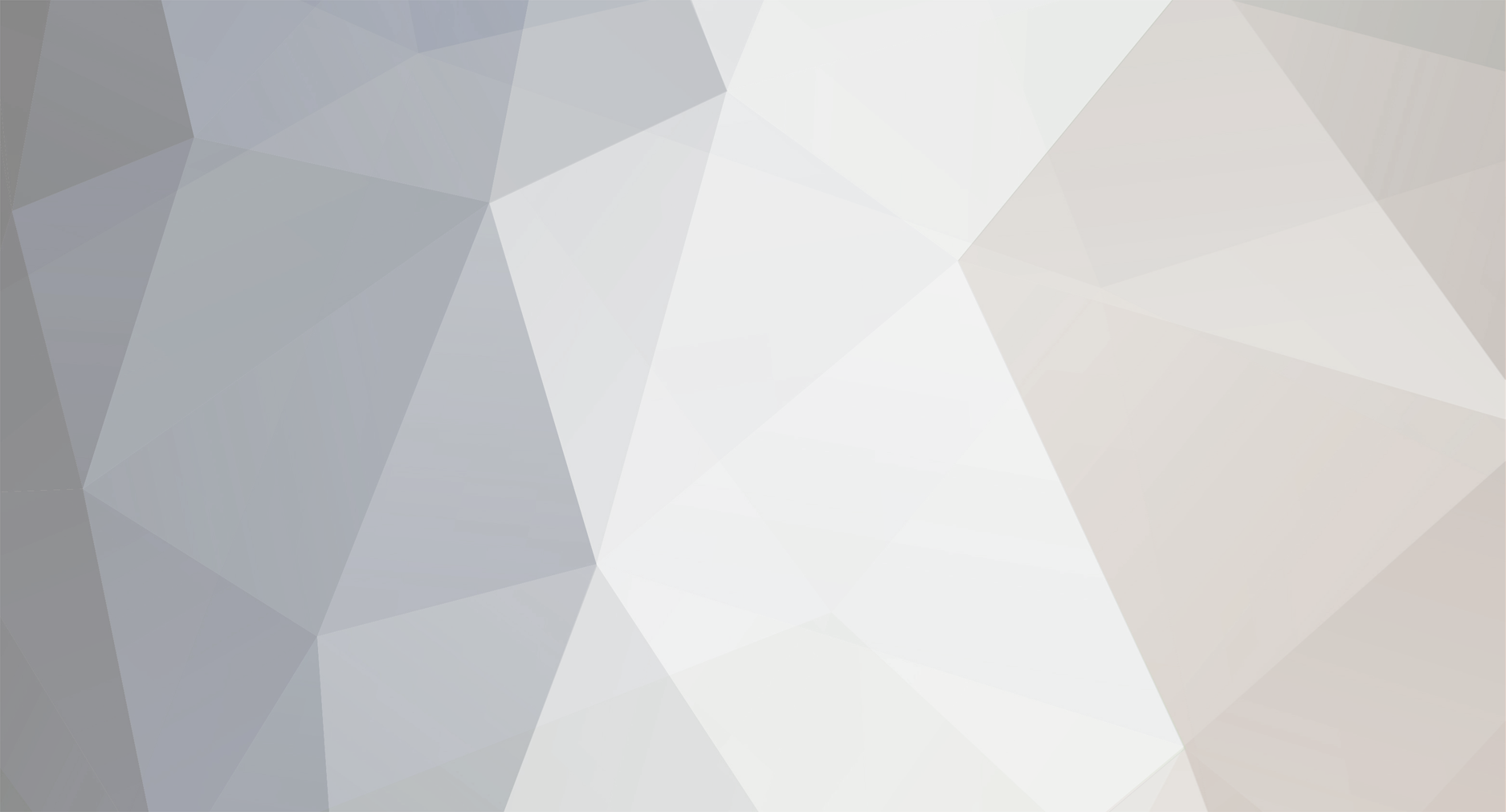
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
No offense, but you might want to check up on the Language Reference chapter in the manual. The query still needs to be a string.
-
What version of MySQL are you using? Maybe it doesn't like the USING notation... Try this instead: SELECT SELECT p.*, u.*, c.* FROM poems p INNER JOIN users u ON u.user_id = p.user_id INNER JOIN categories c ON c.category_id = p.category_id WHERE category_id = {$categoryId} ORDER BY p.poem_name DESC
-
Hmm... not as far as I'm concerned. Consider the following example: class Parent { public function method() { /* ... */ } } class ChildA extends Parent { /* ... */ } class ChildB extends Parent { /* ... */ } Now if I say $obj->method() you cannot know if I'm talking about calling it on Parent, ChildA or ChildB. However, if I say ChildA::method() then you immediately know which class I refer to. You cannot see the name of the class when looking at a variable holding an instance of a class. That's why one would use the double colon notation even though the member you are referring to is not static.
-
Ah... you added an ORDER BY clause... Remove the semi-colon in WHERE category_id = {$categoryId}; ORDER BY p.poem_name DESC
-
Yes, it does. You can, however, also use the foo::bar notation to simply refer to the member called bar in the class foo. I didn't instantiate any object in that snippet so the name of the object you would invoke bark() on would be unknown. I could have said $dog->bark(), but strictly speaking I could have called the object $cat.
-
Did you change your db schema? Try to run the query manually and check it's output (using e.g. phpMyAdmin). Also, <a href="testgedicht.php?poem_id=<?php echo $row['p.poem_id']; ?>"> <?php echo $row['p.poem_name']." | ".$row['u.user_name']; ?> should be <a href="testgedicht.php?poem_id=<?php echo $row['poem_id']; ?>"> <?php echo $row['poem_name']." | ".$row['user_name']; ?>
-
No, it's not really possible. If you look at my first code example then you'll see that if I changed elem1 to be size:50px; then elem1 elem2 would be: 50px * .9 = 45px instead of 9px.
-
No. E.g. 1% is just 1%. It doesn't mean anything by itself. 1% = 1/100 = .01 You need to know what you are taking x% of. This will be the parent element.
-
You cannot say that x% = x px. It doesn't work that way. Percentages is a way of specifying the size relatively, but pixels is a way of specifying the size in absolute measure. Take a look at this for instance (pseudo-code): elem1 { size: 10px; } elem1 elem2 { size: 90%; } The size (whatever that might mean) of an elem2 element inside an elem1 element will be: 10px * .9 = 9px Now check this out: elem1 { size: 10px; } elem1 elem2 { size: 90px; } Now the size of an elem2 element inside an elem1 element will be 90px and 90px is always 90px.
-
Don't just jump into the patterns immediately though. You need to grasp fundamental OOP concepts such as polymorphism, encapsulation, abstraction, inheritance, composition, decoupling, dependency injection etc. to understand why a particular pattern might be useful. Also remember that no pattern is set in stone; its implementation might differ depending on the context in which it is used.
-
Partially. What you are describing is about the separation of concerns, which I suppose you can say OOP to some extent is as well. For instance, the MVC pattern separates things into models, controllers, and views like you've separated your frontend into HTML, CSS, and Javascript. What you are talking about is also about progressive enhancement though, and that's not what OOP is about. Try to take a look at The Object-Oriented Thought Process. It's a good beginner's book. I haven't read all of it though. It didn't give me very much new information so I got bored with it.
-
Just because it's for a school doesn't mean it shouldn't be properly designed. Using Dj Kat's db schema: SELECT p.*, u.*, c.* FROM poems p INNER JOIN users u USING (user_id) INNER JOIN categories c USING (category_id) WHERE poem_id = ?;
-
Dunno what you mean exactly with teach how to use it. Learn the theory first and learn some design patterns. People often say that class Dog extends Mammal { //... public function bark() { // ... } // ... } is not a real world example, but that's because they haven't got the point of OOP yet. It IS a real world example. OOP is about how objects in the real world interact with each other, and both dogs and mammals exist in the real world and Dogs inherit things that are generic to all mammals, but dogs also have behavior and traits that are specific to dogs and that not all mammals have. Dogs can bark and as such the Dog object has a bark() method. So if you want your dog to bark you call the Dog::bark() method. You don't care specifically how the bark() method is implemented, you just want it to bark. This is one of the cornerstone design principles in OOD (object oriented design): "Code to an interface, not an implementation." The bark() is part of the Dog interface, the body of that method is the implementation. You basically need to figure out what objects your application is composed of. Then you need to figure out how these objects interact and design their interface or API. You can do this using a UML diagram, but if it's a small application then you might not need to make a diagram. When you have figured that out you code it. Another way to figure out what would be the most sensible interface is to use the TDD (test driven development) methodology and then write the unit tests before you write the implementation. In that way you get to figure out what your requirements are and if the interface you had in mind is usable. You can then write the implementation and if you did it correctly then all your unit tests should pass.
-
From the manual:
-
Checking against a predefined array is only practical as long as it is small and relatively static. If you wish to become a programmer then SQL is a highly useful tool so you would want to learn it anyway.
-
I partly agree on that. I also write everything in English but it is a matter of cosistency. If you write one thing in Dutch the other functions tables ect should also be Dutch. It's too confusing using multiple language so stick with one instead. Right, but English is a de facto standard in programming. It's fair to assume that programmers know English, but it isn't fair to assume that people know another language. Furthermore, variables must for instance match the pattern ^[a-zA-Z_][a-zA-Z0-9_]*$ and many non-English languages contain other characters. Spanish as ñ, German has ß, Danish has æ and in many languages you use various forms of (other) diacritical marks. Then there are the languages which doesn't even use ASCII characters such as Arabic or Japanese. The code should always be written in English and then you can do stuff like i18n in your template files. Besides, all the library functions/classes and the keywords are in English as well.
-
It's bad database and application design.
-
You can use the in_array function for this, but if contains as many IDs as you say then it would be better to store all the IDs in a database and check if it's there using SQL.
-
And as a side-note, it's generally considered good practice to make name all variables, functions, classes, methods, properties, database tables, table fields, etc. in English regardless of what the output language is going to be like.
-
I don't see why it would be a problem to use the PK instead.
-
You're testing like e.g. WHERE URL = 'test=4'. I don't see the point in that. Why not just WHERE id = '4'?
-
Yeah, as I said, it sounds like you're talking about magic quotes.
-
Is the URL field in your table the primary key (the id)? If that's the case then try to delete $str = "test="; $test = "{$str}" . "{$test}"; Dj Kat, good catch.
-
Try this: SELECT a.products_name, a.products_id, b.products_id, b.products_price FROM products_description a INNER JOIN products b USING (products_id) WHERE b.products_price = '$price' AND a.products_name = '$name'; You may want to take a look at this tutorial as well: http://www.phpfreaks.com/tutorial/data-joins-unions You might want to explain how it doesn't work though.
-
Sounds like you're looking for magic quotes. Don't use it.