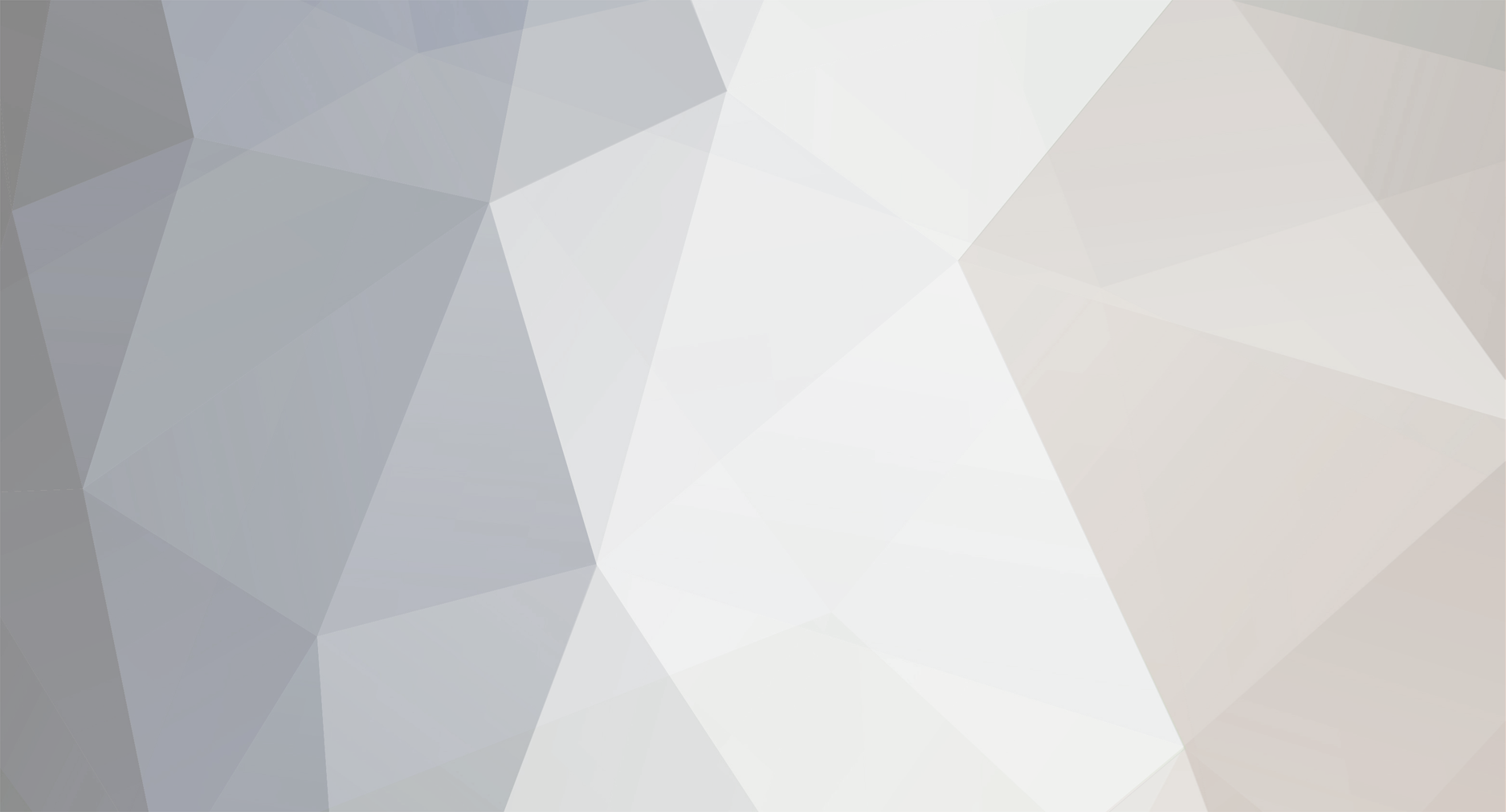
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
Hmm, didn't know you could do that, but there is no reason to do that. The point of the switch, from what I see it, is that you don't have to repeat the variable name you are checking all the time.
-
Just noticed you use a GET value and a variable in Danish, so here is a word of advice: It's better to have your variable and function names in English. They must be named like this (regex): ^[_a-zA-Z][_a-zA-Z0-9]*$ (i.e. it must only contain the letters a through z (upper case and lower case), an underscore and digits and it must start with an underscore or one of the before mentioned letters) and at some point you might need to use a name where the allowed characters are not sufficient.
-
Switches are only used to check if something equals something else. That's just the way switches are. You'll have to use an if structure to use any other operators.
-
Any errors? Does it say sent and you don't get the mail or does it say failed when you run the script?
-
If you need the source of the current file you can do file_get_contents(__FILE__);
-
You can't.
-
PHP code: <?php // ... $result = mysql_query("SELECT * FROM uploads where uploader = 'steviez'"); $uploads = array(); while($row = mysql_fetch_array($result)) { $uploads[] = $row; } // we'll assume that the smarty object is called $smarty $smarty->assign('uploads', $uploads); // ... ?> Smarty template code: {section name=upload loop=$uploads} <tr class="sub_table highlight"> <td></td> <td>{$uploads[upload].name}</td> <td>1 (MB)</td> <td><a href="#" target="_blank">Click here</a></td> <td><a href="#">Click Here</a></td> <td><a href="#">Remove</a> <a href="#">Add</a></td> </tr> {/section} Look, I have never touched Smarty before, yet I was able to create this in no time. Reading the Smarty manual might be a good idea.
-
I don't know what your agreement says, but I suppose that when you've handed the files over to them it will be their own responsibility to keep their files safe from leakage. As long as you don't give the files to anyone else I suppose you'll be fine.
-
You could pass it to your class constructor or the method where it is needed. You could also look into the singleton or registry pattern. Singleton <?php class SingletonTest { static private $instance; // private constructor to enforce the usage of SingletonTest::get_instance() private function __construct() {} public function get_instance() { if(!(self::$instance instanceof self)) { self::$instance = self; } return self::$instance; } public function test() { echo "Hello World"; } } $obj = SingletonTest::get_instance(); $obj->test(); // output: Hello World ?> Registry Note that the registry here uses a singleton. <?php class TestClass { public function test() { echo "Hello World"; } } class Registry { private static $registry = array(); private static $instance; private function __construct() {} public static function get_instance() { if(!(self::$instance instanceof self)) { self::$instance = new self; } return self::$instance; } public function set(&$item, $name = null) { if(is_object($item) && is_null($name)) { $name = get_class($item); } else if(is_null($name)) { throw new Exception('Non-objects must have a name'); } $name = strtolower($name); self::$registry[$name] = $item; } public function &get($name) { if(self::exists($name)) { $name = strtolower($name); return self::$registry[$name]; } else { throw new Exception("The item '{$name}' does not exist in the registry"); } } public static function exists($name) { return array_key_exists(strtolower($name), self::$registry); } } $obj = new TestClass(); $registry = Registry::get_instance(); $registry->set($obj, 'test'); //... $registry = Registry::get_instance(); $obj = $registry->get('test'); $obj->test(); // output: Hello World ?> You could then use an autoloader so you don't have to use include/require every time.
-
Not even large companies like Microsoft and Adobe are able to protect their software from privacy, what are you trying to accomplish? If people really want to pirate your software then they'll find a way.
-
You don't put it anywhere. You modify it and take out the things you need to fit your needs The important part for you is this: header( "Content-Disposition: attachment; filename=".basename($file)); It's a header which tells the browser that the file is to be downloaded and what the name of the file is.
-
See: http://www.phpfreaks.com/forums/index.php/topic,95433.0.html
-
Don't encode it in the variable you create. PHP decodes all the GET variables and therefore you do not have e.g. %20 instead of a space in GET queries. I.e. make $searchString = 'King Kong (2005)'; instead. Just a regular associative array, not an object (nor is your string an object).
-
Just don't use a marquee. :-\ I like my text staying where it is. It's much easier to read it that way. I'm sure most other people agree.
-
border-radius: 10px; There is just one problem though. Few browsers support this. Check this out: http://www.alistapart.com/articles/customcorners/
-
S/he could do a comma separation in one field instead of two fields like Google Trends does. That way you can also have as many contestants as you'd like.
-
You will 99.99999% not have your files in the root directory.
-
Have a topics table and a posts table. Use a has-many relationship to link the posts to a topic by having a column called topic_id in posts. Then you could do this to get all the posts in topic id 5: SELECT * FROM posts WHERE topic_id = 5; I'd also put the first post in the posts table as well instead of a column called body in topics or something like that.
-
You need to do one of the following things: Provide something other people don't. Provide something in a better and/or cheaper way than other people do.
-
No, if the => operator is omitted the variable after as will contain the value.
-
It signifies the same. The thing to the right of the => operator is the value and to the left is the key. In other words: You are looping through the array $array and at each iteration you can access the value as $value and the key as $i inside the loop. You can read more about foreach here: http://php.net/foreach
-
It's a way of specifying the key in order to create an associative array instead of the default enumerated array. <?php $array = array('key' => 'value'); echo $array['key']; // output: value $array = array('value'); echo $array[0]; //output: value ?> See: http://www.php.net/manual/en/language.types.array.php
-
Well, the Security::checkAuth() method is declared as static and therefore $this is not defined within that method. Just get the error object inside that method.
-
PHP did have limited OOP support in version 4. See: http://php.net/oop (OOP in PHP4) and http://php.net/oop5 (OOP in PHP5)
-
Have you tried taking a look at the MVC, model-view-controller, pattern? The controller processes user actions, the model handles the data and the view handles the view/template. You can check it out on Wikipedia and there are a couple of links in the resources topic regarding the MVC pattern. Personally I found that the easiest way to grasp this pattern is by taking a look at frameworks which utilize it. Examples of such could be CakePHP or CodeIgniter.