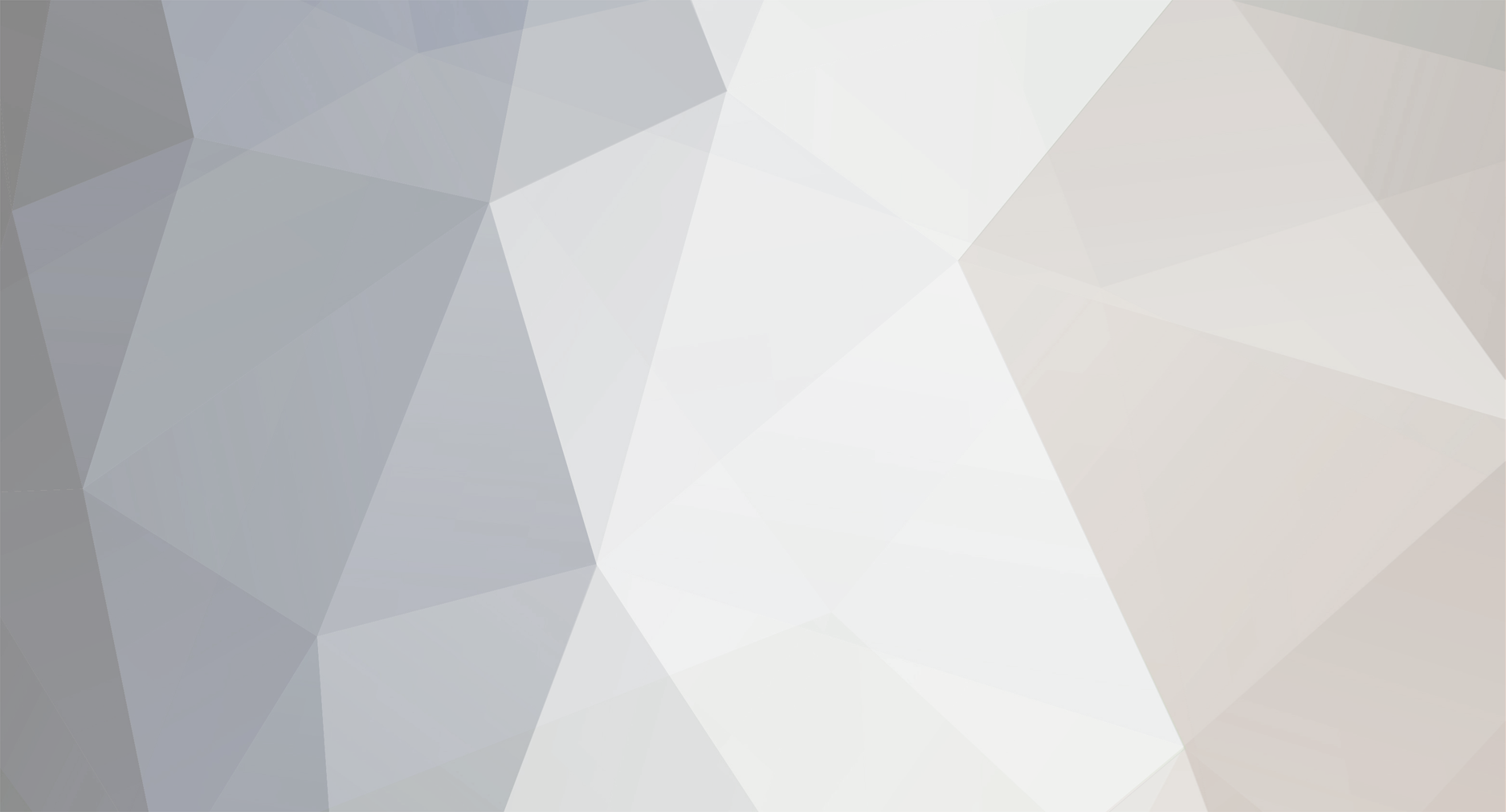
HeyRay2
Members-
Posts
223 -
Joined
-
Last visited
Never
Everything posted by HeyRay2
-
This is a function I've been using for quite some time to ensure all $_GET variables I'm using in SQL queries are safe from injection attacks: // Quote variable to make safe for use in MySQL queries function quoteSmart($value) { // Trim whitespace $value = trim($value); // Stripslashes if (get_magic_quotes_gpc()) { $value = stripslashes($value); } // Quote if not a number or a numeric string if (!is_numeric($value)) { $value = mysql_real_escape_string($value); } return $value; } So to make your $_GET['userid'] variable safe just do: $userid = quoteSmarty($_GET['userid']);
-
Have you tried running this query in a program like PHPMyAdmin to see if you are getting the correct number of result rows? $query = "SELECT * FROM `friends` WHERE `passive_id` = '$puid' AND `accpet` = 'y' LIMIT 3";
-
Are you talking about when you see the "|" (pipe) character in a smarty tag? Something like: {$smarty.now|date_format} If so, that is a tag modifier. It allows you to format a smarty tag or set a default value. More info on modifiers is available here: http://smarty.php.net/manual/en/plugins.modifiers.php
-
[SOLVED] Echoing if there are no results from the DB to display
HeyRay2 replied to HoTDaWg's topic in PHP Coding Help
You can add a variable that checks how many results were returned from the DB query. If there were no results, then echo a "No results" message. Otherwise, run the while() loop. Something like this: <?php //connection stuff here.... echo'<table width="375" height="0" cellpadding="0" cellspacing="0" border="1"><tr><td>Id:</td><td>Action:</td><td>Date:</td><td>Ip:</td></tr><tr>'; $sql = "SELECT id,action,date,read,ip FROM actions WHERE read='nope'"; $result = mysql_query($sql,$connection)or die(mysql_error()); $num_results = mysql_num_rows($result); if($num_results == 0){ echo "No results to display!"; } else{ while($row = mysql_fetch_assoc($result)){ echo'<td>'.$row['id'].'</td><td>'.$row['action'].'</td><td>'.$row['date'].'</td><td>'.$row['ip'].'</td></tr><tr>'; } ] echo'</table>'; ?> -
[SOLVED] Is this possible with a drop down and MySQL?
HeyRay2 replied to suttercain's topic in PHP Coding Help
Glad to hear you rectified the issue. Just to ensure anyone looking at this thread is able to benefit from the solution, I'm assuming you solved the problem by using something along the lines of this: <?php $result = mysql_query("SELECT title, COUNT(*) as numissues FROM comics GROUP BY title") or die(mysql_error()); echo "<select name='title'>"; while($row = mysql_fetch_array($result)){ $rows = $row["numissues"]; // Changed from $rows = mysql_num_rows($result); $title=$row["title"]; echo "<option value='" . $title . "'>" . $title . " (" . $rows . " Issues)</option>'"; } echo "</select>"; ?> -
Typo my part (sorry I was in a hurry while I wrote the code). Change: $book_list.=$title." by ".$author\n"; ... to ... $book_list.=$title." by ".$author."\n";
-
Allow a User to Custom Sort SQL Results on a PHP Page
HeyRay2 replied to eRott's topic in PHP Coding Help
I'm glad you found a solution. If you're still looking to make your code more efficient, here is another method you can try. The first thing you'll want to do is create a list (array) of the order($s) and sort($d) values that are allowed to be passed to your script. If an invalid value is passed, or a value is not present, you'll use a default value from the list. Add this to the top of your code: // Create an array of allowed order($s_array) and sort($d_array) methods $s_array = array("member","race","class","level","server","faction"); $d_array = array("asc","desc"); // Check if the order($_GET['s']) and sort($_GET['d'] variables were set in the URL. // Otherwise use a default value( first array element ) $s = (isset($_GET's') && in_array($_GET['s'], $s_array)) ? $_GET['s'] : $s_array[0]; $s = (isset($_GET'd') && in_array($_GET['d'], $d_array)) ? $_GET['d'] : $d_array[0]; ... next, we'll create the links for each header from the same arrays using a loop. In each loop, we'll check if the link we are creating is the currently used order and sort value, and change them appropriately. Therefor, if we are ordering by "member" and sorting "ASC", then we'll change the "member" link to sort "DESC". This will allow us to keep all other links set to "ASC" by default when they are first clicked ... .. with that said, replace this ... echo " <table style=\"border: 1px solid #606060;\" width=\"100%\" cellspacing=\"0\" cellpadding=\"0\"><tr class=\"rosterTITLE\"> <td align=\"left\"><a href=\"roster.php?s=member\"><img src=\"lib/roster/images/member.jpg\" border=\"0\"></a></td> <td align=\"right\"><a href=\"roster.php?s=race\"><img src=\"lib/roster/images/race.jpg\" border=\"0\"></a></td> <td align=\"left\"><a href=\"roster.php?s=class\"><img src=\"lib/roster/images/class.jpg\" border=\"0\"></a></td> <td align=\"center\"><a href=\"roster.php?s=level\"><img src=\"lib/roster/images/level.jpg\" border=\"0\"></a></td> <td align=\"center\"><a href=\"roster.php?s=server\"><img src=\"lib/roster/images/server.jpg\" border=\"0\"></a></td> <td align=\"right\"><a href=\"roster.php?s=faction\"><img src=\"lib/roster/images/faction.jpg\" border=\"0\"></a></td></tr> "; ... with this ... echo ' <table style="border: 1px solid #606060;" width="100%" cellspacing="0" cellpadding="0"><tr class="rosterTITLE">'; foreach($s_array as $s_item){ // If the order($s) equals the link we are printing out, and we're sorting ASC, then switch to DESC. Otherwise, use ASC $d_item = ($s_item == $s && $d == $d_array[0]) ? "desc" : "asc"; echo '<td align="left"><a href="roster.php?s='.$s_item.'&d='.$d_item.'"><img src="lib/roster/images/'.$s_item.'.jpg" border="0"></a></td>'; } echo '</tr>'; ... Last, we'll update your SQL query to take advantage of the order and sort variable we set earlier. That way you only need one query, and it will change dynamically when these variables change ... ... replace this ... $sql = mysql_query("SELECT * FROM members ORDER BY name ASC LIMIT $from, $max_results"); ... with this ... $sql = mysql_query("SELECT * FROM members ORDER BY $s $d LIMIT $from, $max_results"); ... and you should be all set ... =-D -
To count the number of users in the database you could run a query like this: $result= mysql_query("SELECT COUNT(*) FROM bl_admin") ... and then show it like this ... echo 'Total Users: '. mysql_result($result, 0); To show the number of users online, you could first set up a field in your bl_admin table that would track the last time a user performed an action (perhaps this is what your lasttime field is for?). The easiest way would be to have code included on each page that would update that information on each page reload. I'll assume you are using sessions to track users that are logged in. Something like: if($_SESSION['logged_in']){ $sql = "UPDATE bl_admin SET lasttime = NOW() WHERE username = '$username'; mysql_query($sql) or die(mysql_error()); } ... then to show the users that are online, you'll need to set a "timeout" value. This will set a period of inactivity that will determine if a user is no longer online. $timeout = 300; // in seconds // Get a timestamp for the timeout time $timestamp_limit = time() - $timeout; // Create and run the query $result = mysql_query("SELECT username FROM bl_admin WHERE UNIX_TIMESTAMP(lasttime) > $timestamp_limit; $result_numrows = mysql_num_rows($result); $result_array = mysql_fetch_array($result); for($i=0;$i < $result_numrows;$i++){ if($i == 0){ echo '# of Users Online: '.$result_numrows.'<br>'; echo 'Users Online: '; } echo $result_array['username'].','; }
-
avatar.alex, I'm working on some sample code for you to help you figure this out. I'll reply back in a little while with it.
-
You need to have code that will check if the user has previously uploaded an image before. Something like: <?php // For register_global on PHP settings////////////////////////////////////////////// $member = $_COOKIE['member']; session_start(); // you must put this to read session variables///////////////////// if (empty($member) || !isset($member)) // fail to read the browser cookie/////////// { // Try to read session if (empty($_SESSION['member']) || !isset($_SESSION['member'])) { header("Location: login.php"); // redirect user to login////////////////////// exit; } else { $member = $_SESSION['member']; } } //Includes... ////////////////////////////////////////////////////////////////////// include("includes/db_connection.php"); include("includes/constants.php"); include("includes/header.php"); include("includes/loginnav.php"); ?> <p align="left">Welcome, <b><font color="red"><? echo $_COOKIE['member'] ?></font></b>! (<a href="logout.php">Logout</a>) - [ <a href="change_password.php">Change Password Or E-mail Address</a> ]-[ <a href="edit_profile.php">Edit Profile</a> ]-[ <a href="upload_photo.php">Upload Photo</a> ]</p> <?php // Set the photo name from the form submission. If no image was submitted, this will be empty $photo = $_FILES["imgfile"]["name"]; $random_digit = rand(000000,999999); $renamed_photo = $random_digit.$_FILES["imgfile"]["name"]; $username = mysql_escape_string($_POST['username']); // Create a query to check if the current user has uploaded a photo previously, so we can overwrite it $sql = "SELECT photo FROM membership WHERE username='$username' LIMIT 1"; // Set a varialbe for the DB connection $result = mysql_query($sql, $conn) or die(mysql_error()); // Run the query in a while loop while($row = mysql_fetch_array($result){ // Set the photo name to the name already saved in the DB so we can overwrite the existing photo variables and file $photo = $row['photo']; $renamed_photo = $photo } $max_image_size = '300000'; // Bytes only... if (empty($photo)) { echo "<p>Sorry <i>$username</i> You Never Selected A Photo To Upload <a href=\"javascript: history.go(-1)\">Go Back</a> And Upload One!</p>"; exit; } // Validation for Image...///////////////////////////////////////////////////////////// if ($_FILES['imgfile']['size'] > $max_image_size) { die ("<p>Sorry <i>$username</i> That Image Is Bigger Than The Allowed Size Of 3mb Please <a href=\"javascript: history.go(-1)\">Go Back</a> And Upload Another!</p>"); } $uploadpath = "uploads/"; // <- Upload folder... $uploadpath = $uploadpath.$renamed_photo; if (!move_uploaded_file($_FILES["imgfile"]["tmp_name"], $uploadpath)) die("<p>Sorry <i>$username</i> There Was An Error Uploading Your Image!"); echo("<p><br />The Image (<b><font color=\"red\">" .$_FILES["imgfile"]["name"]. "</b></font>) Has Been Uploaded Successfully!<br />"); // Create our query...///////////////////////////////////////////////////////////////// $sql = "UPDATE membership SET photo='$renamed_photo' WHERE username='$username'"; // Run our query...//////////////////////////////////////////////////////////////////// $result = mysql_query($sql, $conn) or die(mysql_error()); // Show success message...///////////////////////////////////////////////////////////// if($result) { echo "<p><a href=\"javascript: history.go(-1)\">Back To My Account</a></p>"; } ?>
-
Change this line of code: $nameuse = filename; ... to ... $nameuse = $_FILES['userfile']['name']; ... and that will stop your previous file from being overwritten. With the code the way it was, it was always naming the file "filename".
-
Here's another method: <?php if ( isset( $_POST['submit'] ) ) { if(count($_POST['checkBox']) > 0){ foreach($_POST['checkBox'] as $checkbox_file) include $checkbox_file; } } // Create an array of files that can be included $include_files = array("file1.html", "file2.html", "file3.html"); ?> <form action="<?=$_SERVER['PHP_SELF']?>" method="post"> <?php foreach($include_files as $file) echo $file.': <input type="checkbox" name="checkBox[]" value="'.$file.'" /><br>'; ?> <input type="submit" name="submit" value="Submit" /> </form> Just add all the names of the files that can be included into the $include_files variable and you'll be all set. Also, Glyde is correct. PHP is best learned by messing around with it. Try things, be willing to make mistakes, and ask for help when you get stuck. Good luck!
-
kenrbnsn, you beat me to the punch... Nice work!
-
How do I figure out the "full path" to my directory
HeyRay2 replied to jodiellewellyn's topic in PHP Coding Help
Your web host must have error reporting turned off: Try this: <?php phpinfo(); ?> ... and scroll down the "PHP Variables" section ... -
How do I figure out the "full path" to my directory
HeyRay2 replied to jodiellewellyn's topic in PHP Coding Help
All the following $_SERVER variables should contain your "full path": $_SERVER["DOCUMENT_ROOT"] $_SERVER["PHPRC"] Also, you could always just make a file with a syntax error that will spit out an error with the full path to the file. Something like... <?php foreach() ?> ... should generate an error. PHP errors are so useful sometimes... -
Sorry, I had to go and spend some time with Flash to get myself used to passing information from it to PHP again. Barand, you are a genius. You always justify those stars next to your name . optikalefx, change the "var" names in your Flash form fields to be something like this: gnum fields: gnum[1] gnum[2] gnum[3] ... and so on ... teama fields: teama[1] teama[2] teama[3] ... and so on ... teamb fields: teamb[1] teamb[2] teamb[3] ... and so on ... When you submit the flash form, you'll get an array for gnum, teama and teamb values each of which you can loop through easily, something like: foreach($_POST['gnum'] as $key => $value){ $sql = mysql_query("INSERT INTO $week (gnum, team1, team2) VALUES ('".$value."' , '".$_POST['teama'][$key]."' , '".$_POST['teamb'][$key]."')"); } This will allow you to use the $gnum array keys as pointers for the corresponding $teama and $teamb arrays Obviously you'll want to put in some error checking either in your Flash actionscript or in your PHP code to check for empty fields, but that's not really a concern at the moment. Good luck!
-
The error array element being set to "1" leads me to believe there was an error reading the file. Have you tried other mp3 files? Perhaps try a very small mp3 (sound byte under 500k would be a good test) to see if your PHP configuration limit for max_post_size is too low (the setting for all parts of a form submission).'
-
You mentioned changing hosts recently. Did you update the information in your connect.php file to reflect the database connection information for your new host? If so, perhaps posting the code in your connect.php file here might lead us in the right direction for your issue...
-
The implode() function requries an array as it's input, and pulling an array directly out of a SQL query won't work for that. Try replacing this code: if ($_POST['to'] == "all"){ $query = mysql_query("SELECT email FROM applicant WHERE subscribe = 'yes'") or die(mysql_error()); while ($sendemail = mysql_fetch_array($query)){ $dbemail = $sendemail['email']; echo '$dbemail'; } } if ($_POST['to'] == "notall"){ $specialization = $_POST['specialization']; $query = mysql_query("SELECT email FROM applicant WHERE specialization LIKE '%".$specialization."%' AND subscribe = 'yes'") or die(mysql_error()); while ($sendemail = mysql_fetch_array($query)){ $dbemail = $sendemail['email']; $dbemail = implode(',',$dbemail); print_r($dbemail); } } if ($_POST['to'] == "peremail"){ $dbemail = $_POST['email']; } ... with something like this ... $dbemail = array(); if ($_POST['to'] == "all"){ $query = mysql_query("SELECT email FROM applicant WHERE subscribe = 'yes'") or die(mysql_error()); while($row = mysql_fetch_array( $query )){ $dbemail[] = $row['email']; } } if ($_POST['to'] == "notall"){ $specialization = $_POST['specialization']; $query = mysql_query("SELECT email FROM applicant WHERE specialization = '$specialization' AND subscribe = 'yes'") or die(mysql_error()); while($row = mysql_fetch_array( $query )){ $dbemail[] = $row['email']; }; } if ($_POST['to'] == "peremail"){ $dbemail[] = $_POST['email']; } ... and then change this line ... $mail->SendHTML($dbemail,$subject,$original_msg); ... to use the implode() function on the $dbemail array ... $mail->SendHTML(implode(",", $dbemail),$subject,$original_msg);
-
In order to get multiple book details in your email, you would need to make your $book variable an array and add each book to it as you gathered it's details. Something like: $book = array(); foreach ($cart as $product_id => $qty) { $book[] = get_book_details($product_id); } Then you would loop through that array while creating the text for your email. Something like: $book_list = ""; foreach($book as $single_book){ extract($single_book) $book_list .= $title." by ".$author\n"; } .. and then add that to your $mess variable: $mess = "<html>\n" ."<head>\n" ."<title>Test Mail</title>\n" ."</head>\n" ."<body>\n" ."This is an html email test<br />\n" ."<p><b>Your order has been processed. Please print this email as your reference</b></p>\n" ."<p>Your purchases were:\n" ."$book_list"</p>" ."</body>\n</html>\n";
-
Try this: for ($i=0; $i<= 3; $i++) { $gnum = "gnum".$i; $teama = "team".$i."a"; $teamb = "team".$i."b"; $sql = mysql_query("INSERT INTO $week (gnum, team1, team2) VALUES ('".$_POST[$gnum]."' , '".$_POST[$teama]."' , '".$_POST[$teamb]."')"); }
-
PHP cannot create popups. That needs to be done with JavaScript. This link makes popup creation pretty simple: http://javascript.internet.com/generators/popup-window.html
-
You mentioned that when you were making an update to a single student that the information was being entered into the database successfully. Can you paste a copy of that code so we can compare it to the code you are using for multiple updates?