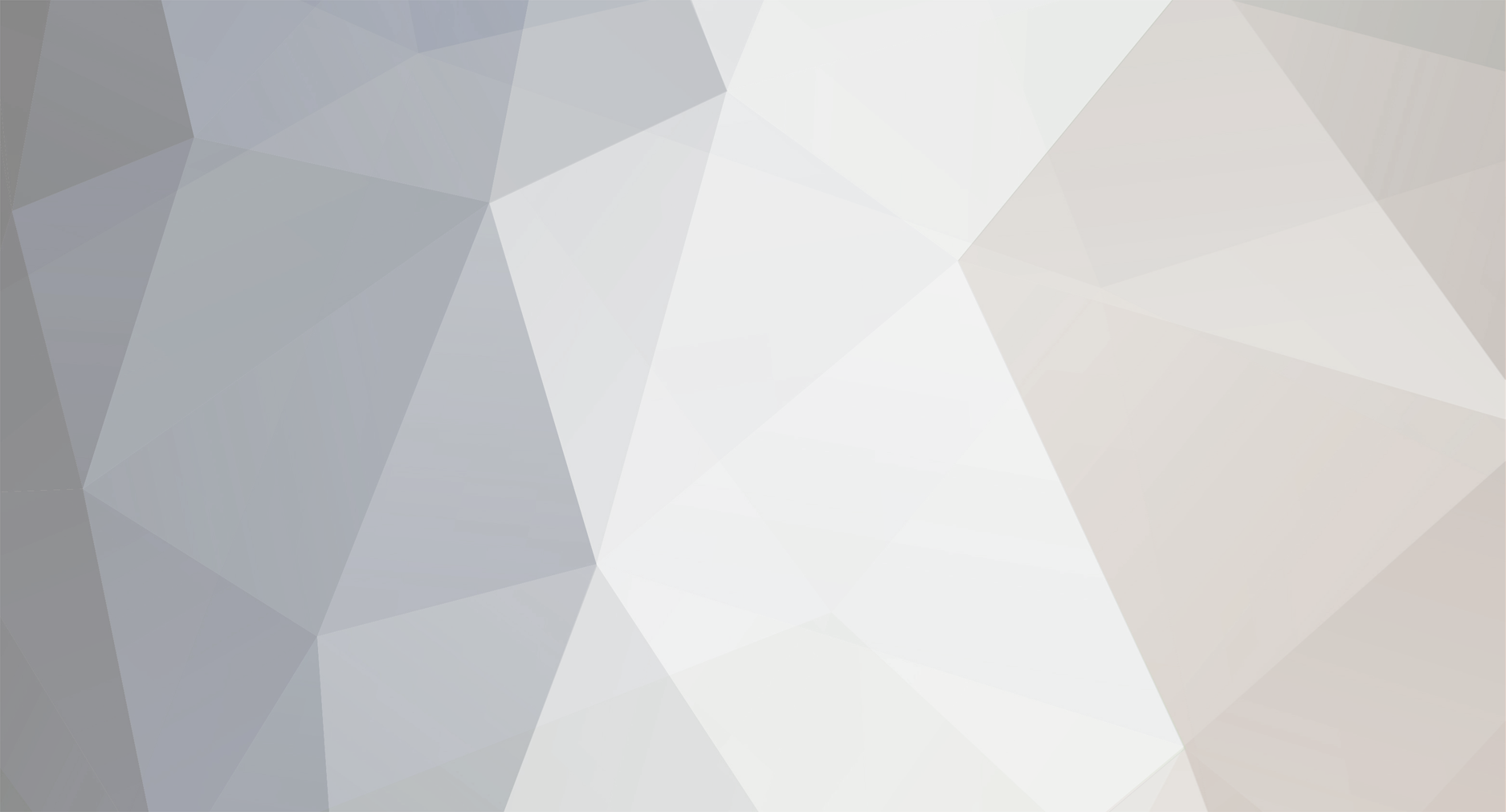
HeyRay2
Members-
Posts
223 -
Joined
-
Last visited
Never
Everything posted by HeyRay2
-
Unknown column 'username' in 'field list'
HeyRay2 replied to CircularStopSign's topic in PHP Coding Help
A carriage return is creating a new line with the "Return" or "Enter" key. If you have a carriage return at the end of a line of code before the line terminator ( [b];[/b] ), it could cause a parse error like the one you are seeing. Make sure those lines of code look like this: [code] $email_check = mysql_num_rows($sql_email_check); if($email_check > 0){ echo "<strong>Your email address has already been used by another member in our database. Please use a different Email address!"; unset($email_address); } include 'join_form.html'; exit(); }[/code] -
Unknown column 'username' in 'field list'
HeyRay2 replied to CircularStopSign's topic in PHP Coding Help
The error you received is telling you that your database query is looking for a field called [b]username[/b], but it does not exist in the database. If you removed the [b]username[/b] field from the database, then you need to remove any references to it in your queries. A snippet of the query code and a description of your database tables would be a good start to figuring out your issue... ;) -
Glad we could help! :) Some basic code to get you started: [b]bar_detail.php[/b] -- Shows details for a bar in the database, and update form. [code] <?php // Get the barID from the URL if( (isset($_GET['barID'])) && (!empty($_GET['barID'])) && (ctype_digit($_GET['barID'])) ){ // Set the barID from the URL $barID = $_GET['barID']; // Check if the update form was submitted if($_GET['Bar_Detail_Update']){ // Update the bar name, description, etc $sql_bar_update = "UPDATE bars SET barName = '".mysql_real_escape_string(trim($_GET['barName']))."', barDescription = '".mysql_real_escape_string(trim($_GET['barDescription']))."' WHERE barID = $barID"; mysql_query($sql_bar_update) or die("Error Updating Bar: ".mysql_error()); // Update the bar attributes, by removing all the attribute relations // then adding back the current selected values $sql_attrib_del = "DELETE FROM attribute_rel WHERE rel_barID = ".$barID.""; mysql_query($sql_attrib_del) or die("Error Removing Attributes: ".mysql_error()); foreach($attrib_checkboxes as $key=>$value){ $sql_attrib_add = "INSERT INTO attribute_rel SET rel_barID = ".$barID.", rel_attributeID = ".$value.""; mysql_query($sql_attrib_add) or die("Error Adding Attribute: ".mysql_error()) } echo "Bar Information Updated!<br />\n"; } // Show the detail page // Get the details for the current bar $sql_bar = "SELECT * FROM bars WHERE barID = $barID LIMIT 1"; $bar_result = mysql_query($sql_bar) or die("Error Querying Bar: ".mysql_error()); // Get an array of attributeID's for the current bar $sql_attrib_rel = "SELECT rel_attributeID FROM rel_attributes WHERE rel_barID = $barID"; $attrib_rel_result = mysql_query($sql_attrib_rel) or die("Error Querying Current Attributes: ".mysql_error()); while($row = mysql_fetch_array($attrib_rel_result){ $bar_attrib_array[] = $row['rel_attributeID']; } // Get the full array of attributes $sql_arrtib = "SELECT * FROM attributes ORDER BY attributeName"; $attrib_result = mysql_query($sql_attrib) or die("Error Querying All Attributes: ".mysql_error()); // Start the form echo '<form name="bar_detail" action="'.$_SERVER['PHP_SELF'].'" method="GET">'; // Print the bar details while($bar_row = mysql_fetch_array($bar_result)){ echo '<input type="hidden" name="barID" value="'.$barID.'" />\n Bar: <input type="text" name="barName" value="'.$bar_row['barName'].'" />\n Bar Description: <input type="text" name="barDescription" value="'.$bar_row['barDesc'].'" />\n'; // Print the attribute checkboxes, with current values checked while($attrib_row = mysql_fetch_array($attrib_result){ // Check if the current checkbox should be checked $checked = (in_array($attrib_row['attributeID'], $bar_attrib_array)) ? 'checked="checked"' : NULL; // Print the checkbox echo $attrib_row['attributeName'].' <input type="checkbox" name="attrib_checkboxes[]" value="'.$attrib_row['attributeID'].'" '.$checked.' />\n'; } } // End the form echo '<input type="submit" name="Bar_Detail_Update" value="Update Bar!" /> </form>'; } else { // No barID was passed, error out! echo "Invalid or No Bar ID Specified!"; } ?> [/code]
-
Placing your attributes into their own table is a good idea. You could also place the attributes for each bar into a relation table, which would remove the need to have a serialized list of the attributes in the database. So your MySQL tables would look something like this: [b]bars[/b] barID barName barDescription etc... [b]attributes[/b] attributeID attributeName attributeImage [b]attribute_rel[/b] relID rel_barID rel_attributeID Then for each bar, you can pull an array of attributes currently associated from the relation table([b]attrib_rel[/b]), and compare each to an array of all items from the [b]attributes[/b] table. I'll post some sample code shortly... ;)
-
[code] <? if (!isset($url)) { $url='products/prodall.php';} ?> [/code] Again, with Register Globals off, you [b]must[/b] pull all of your variables from the URL by way of the $_GET array. Change the above line to read: [code] <?php if (!isset($_GET['url'])){ $url='products/prodall.php'; } else { $url = $_GET['url']; } ?> [/code] ... or to save space ... [code] <?php $url = (!isset($_GET['url'])) ? $_GET['url'] : "products/prodall.php"; ?> [/code]
-
Nice one, [b]Barand[/b]. I concede. I recommend his method in this case. ;)
-
Any variables that you pass through the URL (example: http://www.yoursite.com/yourpage.php?var1=foo&var2=bar), you will access from the $_GET array: [code] <?php $var1 = $_GET['var1']; // $var1 equals "foo" $var2 = $_GET['var2']; // $var2 equals "bar" ?> [/code]
-
Sounds like a job for a subquery: [code] <?php case "never": $who="&&(member.userid NOT IN (SELECT user_id FROM orders GROUP BY user_id))"; $title_who='Members who\'ve never bought anything'; break; ?> [/code]
-
[code] <?php switch (TRUE){ case ($i <= $k): echo "<br>image1"; case ($i <= $l): echo "<br>image2"; break; default: echo "<br>image3"; break; } ?> [/code] Depending on your goal, [b]switch()[/b] can be more efficient. It basically allows you to check a variable against multiple values or conditions. The [b]switch[/b] block I provided you will match all cases that evaluate to "TRUE", and perform an action under those matching cases. The [b]break[/b] lines tell the [b]switch()[/b] to stop executing, and no other cases will be checked. I left out the [b]break[/b] in the first case so that the first and second case would always be checked. If neither are matched, then the [b]default[/b] case executes. There is more than one way to accomplish what you are trying to do, I just prefer the [b]switch()[/b] method when I know I might need to match more than one conditional.
-
What is not working?
-
Allright, I'm seeing the use of variables that are only assigned if RegisterGlobals is turned on in your php.ini file. Your new web host may not have this option enabled by default. Create and run the following script: [b]info.php[/b] [code] <?php // Show all PHP information phpinfo(); ?> [/code] Look for entries regarding Register Globals, if they are set to "1", your problem lies elsewhere. If they are set to "0", then you need to make some changes to your variables. Let us know what you find out... ;)
-
[code] <?php switch (TRUE){ case ($i <= $k): echo "<br>image1"; case ($i <= $l): echo "<br>image2"; break; default: echo "<br>image3"; break; } ?> [/code]
-
[url=http://www.sinagtala.net/eric/]http://www.sinagtala.net/eric/[/url] gives out a pre-parsed copy of [b]products.php[/b]. Make a copy of your [b]products.php[/b] in the same folder mentioned above, but with a [b].phps[/b] extension, or just paste the code from your [b]products.php[/b] file here and we'll take a look at it.
-
Perhaps posting the code from your [b]products.php[/b] will get us on the right track to helping you figure out your issue... ;)
-
Change your [b]foreach()[/b] loop to a [b]for()[/b] loop so you can track what loop iteration you are on, and only print the top table on the first iteration. You'll notice that with this method you'll access each item in the [b]$spaces[/b] array using [b]$spaces[$i][/b] instead of [b]$space[/b]: [code] <?php for($i=0;$i < count($spaces);$i++){ if( $i == 0 ){ // Print the top table, because we are on the first iteration ?> <!-- **************************TOP TABLE************************* --> <table border="0" width="500" cellpadding="2" cellspacing="1"> <tr> <td colspan="2"><span class="leaseTitle"><?= $spaces[$i]['address'] ?></span></td> </tr> <tr> <td valign="top" align="right" class="leaseInfo">District:</td> <td class="leaseText" valign="top"><?php= $spaces[$i]['name'] ?></td> </tr> <tr> <td valign="top" class="leaseInfo" align="right">Contacts:</td> <td class="leaseText" valign="top"><a class="hplink" href="mailto:howard.tenenbaum@slgreen.com">Howard J. Tenenbaum</a>, 212-216-1685 (SL Green Leasing, LLC)<br><a class="hplink" href="mailto:gary.rosen@slgreen.com">Gary M. Rosen</a>, 212-216-1687 (SL Green Leasing, LLC)<br></td> </tr> </table> <br /> <a href="property.php?id=<?php= $spaces[$i]['id'] ?>"><input class="button" type="button" value="Detailed Property Information" onClick="document.location.href='property.php?id=<?php= $spaces[$i]['id'] ?>';"></a> <br /><br /><br /> <!-- ************************** END TOP TABLE ************************* --> <?php } // Print the bottom table <!-- **************************BOTTOM TABLE************************* --> <table width="500" cellpadding="1" cellspacing="1" border="0"> <tr align="center" bgcolor="#666666"> <td class="smtypeWt">Floor<br><img src="images/gresources/x.gif" alt="SL Green" width="65" height="1" border="0"><br></td> <td class="smtypeWt">Suite<br><img src="images/gresources/x.gif" alt="SL Green" width="60" height="1" border="0"><br></td> <td class="smtypeWt">Square<br>Feet<br><img src="images/gresources/x.gif" alt="SL Green" width="50" height="1" border="0"><br></td> <td class="smtypeWt">Available<br><img src="images/gresources/x.gif" alt="SL Green" width="60" height="1" border="0"><br></td> <td class="smtypeWt">Rent/SF<br><img src="images/gresources/x.gif" alt="SL Green" width="60" height="1" border="0"><br></td> <td class="smtypeWt">Term<br><img src="images/gresources/x.gif" alt="SL Green" width="45" height="1" border="0"><br></td> <td class="smtypeWt">Direct/<br>Sublet<br><img src="images/gresources/x.gif" alt="SL Green" width="60" height="1" border="0"><br></td> <td class="smtypeWt">Retail/<br>Office<br><img src="images/gresources/x.gif" alt="SL Green" width="50" height="1" border="0"><br></td> <td class="smtypeWt">Prebuilt<br><img src="images/gresources/x.gif" alt="SL Green" width="50" height="1" border="0"><br></td> </tr> <?php $bgcolor = ($bgcolor == "#99CCFF") ? "#ccccFF" : "#99CCFF"; ?> <tr bgcolor="<?php= $bgcolor ?>"> <td align="right" class="smtype" valign="top"><?php= $spaces[$i]['floor'] ?></td> <td class="smtype" valign="top"><?php= $spaces[$i]['suite'] ?></td> <td align="right" class="smtype" valign="top"><?php= $spaces[$i]['sqfeet'] ?></td> <td align="center" class="smtype" valign="top"><?php= $spaces[$i]['availability'] ?> <img src="/images/null.gif" width="1" height="1"></td> <td align="right" class="smtype" valign="top"><?php= $spaces[$i]['rentsqfeet'] ?></td> <td align="right" class="smtype" valign="top"><?php= $spaces[$i]['term'] ?><img src="/images/null.gif" width="1" height="1"></td> <td align="center" class="smtype" valign="top"><?php= $spaces[$i]['issublet'] ?></td> <td align="center" class="smtype" valign="top"><?php= $spaces[$i]['isretail'] ?></td> <td align="center" class="smtype" valign="top"><?php= $spaces[$i]['isprebuilt'] ?></td> </tr> <tr bgcolor="<?= $bgcolor ?>"><td align="left" class="smtype" valign="Top" colspan="9"> <?php= $spaces[$i]['notes'] ?></td> </tr> <tr bgcolor="<?php= $bgcolor ?>"><td colspan="9" class="smtype"><a class="hplink" href="#" onClick="window.open('floorplan.php?spaceid=$spaces[$i]['id']','spacewin','toolbar=no,status=no,directories=no,scrollbars=yes');">Floorplans</a></td> </tr> <tr align="center"> <td class="smtype" colspan="9"><img src="images/gresources/x.gif" width="1" height="4" border="0"></td> </tr> <?php } // Done printing bottom table ?> </table> <!-- **************************END BOTTOM TABLE************************* --> [/code] Hope this helps! ;)
-
[code]<?php echo $_SERVER['PHP_SELF']; ?>[/code] That variable is replaced by the name of the php file that printed out your html form, so only use it if that same php file also does your form processing. Otherwise, enter the name of the file that you want to process the form... ;)
-
Never rely only on client-side scripting to verify data. It's good to give the user a quick heads-up if they miss a field, but don't allow that to be your only line of defense. Here's a good thread on server-side data validation and error checking, which includes checking for blank values. http://www.phpfreaks.com/forums/index.php/topic,36973.0.html For your client-side error checking, post some code and we'll do what we can to help out... ;)
-
[code] <form name="form1" method="post" action=""> [/code] You have not defined [b]action[/b] in your form, so the data you submit doesn't get sent anywhere. Try this: [code] <form name="form1" method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>"> [/code]
-
How to create a dropdown menu with items from a DB
HeyRay2 replied to techiefreak05's topic in PHP Coding Help
[b]mysql_numrows()[/b] should be [b]mysql_num_rows()[/b]... -
http://www.ajaxfreaks.com/tutorials.php
-
[b]redarrow[/b]'s example, from [url=http://www.mysql.org/doc/refman/4.1/en/date-and-time-functions.html]MySQL.org[/url], is missing a comparison. Otherwise, it should work fine. Try this: [code] <?php $query = "SELECT something FROM tbl_name WHERE DATE_SUB(CURDATE(),INTERVAL 30 DAY) <= date_col"; ?> [/code]
-
You make a database call to record that a link was clicked. How much harder is it to do one more query right below the first and grab that same record, grab the URL and make a [b]header()[/b] call to it. ??? Doesn't sound like that much more work.
-
Something like this will get you started (you'll need add / update confirmation logic and such): [code] <?php $SSN = "123-45-6789"; if (preg_match("/^\d{3}-\d{2}-\d{4}$/", $SSN)) { // SSN is valid // Check if SSN already in database $sql = "SELECT COUNT(*) as ssn_match FROM patient_table WHERE ssn = '$SSN'"; $result = mysql_query($sql) or die("Error querying SSN: ".mysql_error()); $row = mysql_fetch_row($result); if($row['ssn_match'] == 0){ // No matches, add patient as new $sql_new = "INSERT INTO patient_table SET ssn = '$SSN', other_field = 'other_value'"; mysql_query($sql_new) or die("Error adding patient: ".mysql_error()); } else { // Match found, update current patient $sql_update = "UPDATE patient_table SET other_field = 'other_value' WHERE ssn = '$SSN' LIMIT 1"; mysql_query($sql_update ) or die("Error updating patient: ".mysql_error()); } } else { // SSN is invalid, show error echo "SSN must be in the format XXX-XX-XXXX (where X is a number 0 - 9)"; } ?> [/code]
-
Actually, it has everything to do with your current queries, because I can't help you determine what row data to parse if you can't provide me with what row data you are querying. Post your query code here so I can what data you are pulling, so then I can tell help you access that data.