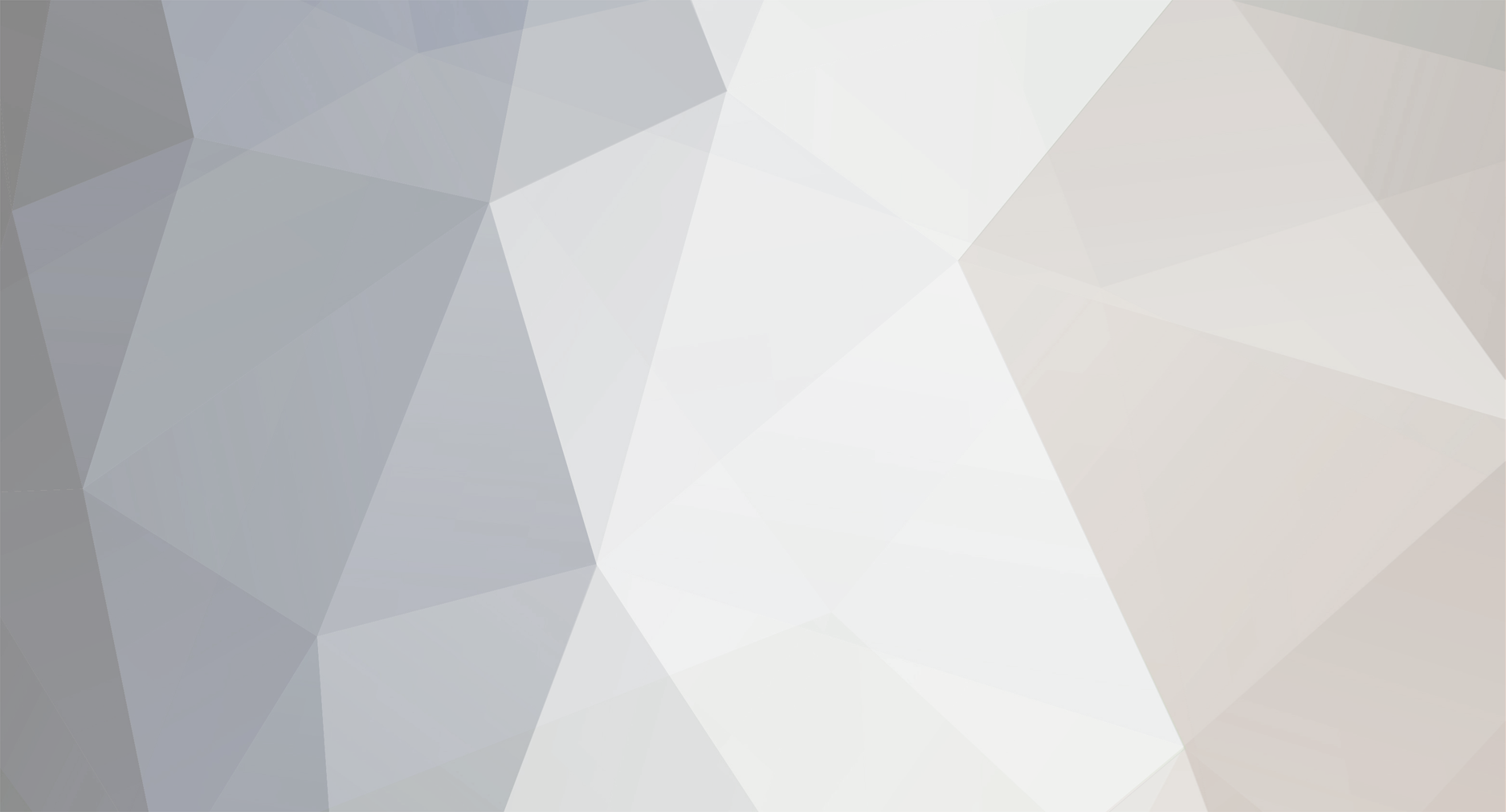
HeyRay2
Members-
Posts
223 -
Joined
-
Last visited
Never
Everything posted by HeyRay2
-
You're on the right track, but you will need to increment your iterator variable each time you go through the loop, so try it one of two ways: $i = 1; while ($i <= $phpNum) { $gnum = $_POST["gnum{$i}"]; $teama = $_POST["team{$i}a"]; $teamb = $_POST["team{$i}b"]; $sql = mysql_query("INSERT INTO $week (gnum, team1, team2) VALUES ('$gnum' , '$teama' , '$teamb')"); $i++; } ... or ... for ($i = 1; $i <= $phpNum; $i++) { $gnum = $_POST["gnum{$i}"]; $teama = $_POST["team{$i}a"]; $teamb = $_POST["team{$i}b"]; $sql = mysql_query("INSERT INTO $week (gnum, team1, team2) VALUES ('$gnum' , '$teama' , '$teamb')"); }
-
You'll need to store this value on the server, either in a text file or database of some sort. Search the forums for keywords related to saving to text files or databases, and you should get on the right track.
-
I see two things wrong with the following line based on what I'm reading: If this is what you're seeing in the URL after you submit your form, then you are passing your form variables using the $_GET array, not the $_POST array. Change your form submit method to "POST" if you are not already. Additionally, if you're trying to pass a multi select list from an HTML form, you have to append brackets to the end of the form element name. So instead of using "project_list" for the name, you would need to use "project_list[]". Otherwide, each time a"project_list" value was defined, it would overwrite the existing value because the value is being treated as a string. If you use "project_list[]", then each new definition will add an element to the array, as the values would be treated as array elements. Good luck!
-
Possible to populate a "checkbox from a mysql database?
HeyRay2 replied to suttercain's topic in PHP Coding Help
boo_lolly's suggestion should work well. Another option is that you could use your "vin" field and set it as UNIQUE in your database, as I'm assuming that you'll not have two rows in your db with the same vin number. This could be used instead of a "record_id" field and would provide a quick way to distinguish rows. Also, when updating your db to reflect which "letter_sent" checkboxes are checked, you can save quite a few db calls by consildating boo_lolly's code into a single query without the need for a loop. Try changing: <?php foreach($_POST['checkboxes'] as $key => $val){ $sql = "UPDATE canada SET letter_sent = '1' WHERE id = '$val'"; } ?> to read: <?php $sql = "UPDATE canada SET letter_sent = '1' WHERE id IN ('" . implode(",", $_POST['checkboxes']). "')"; ?> -
Regular Expressions are your best method of implementing a language filter. I recommend taking the time to read up on it, because you'll find many uses for it once you understand how powerful it is. There are hundreds of tutorials out there that will help you get a hang of it, including video tutorials: http://www.phpvideotutorials.com/regex/ Good luck!
-
Here's another example that uses a slightly different method, but produces the same result: http://www.mredkj.com/tutorials/tableaddrow.html Good luck!
-
You could do this with JavaScript and save the time and hassle of reloading the page. There is some good example code at the below address: http://www.dustindiaz.com/add-and-remove-html-elements-dynamically-with-javascript/ Change that code too input textboxes and you should be good to go.
-
I've been helped here many times, so it's the least I can do to help out a fellow PHP Freak!
-
Doh! My bad! Add this to the end of each of your links: &Submit=Submit That will cause your code to think that the form was submitted each time you click a link to another results page. Sorry I missed that! :-\ Full code to change for clarification: if ($pageno == 1) { echo " FIRST PREV "; } else { echo " <a href='{$_SERVER['PHP_SELF']}?pageno=1§ion=$section&category=$category&Submit=Submit'>FIRST</a> "; $prevpage = $pageno-1; echo " <a href='{$_SERVER['PHP_SELF']}?pageno=$prevpage§ion=$section&category=$category&Submit=Submit'>PREV</a> "; } // if echo " ( Page $pageno of $lastpage ) "; if ($pageno == $lastpage) { echo " NEXT LAST "; } else { $nextpage = $pageno+1; echo " <a href='{$_SERVER['PHP_SELF']}?pageno=$nextpage§ion=$section&category=$category&Submit=Submit'>NEXT</a> "; echo " <a href='{$_SERVER['PHP_SELF']}?pageno=$lastpage§ion=$section&category=$category&Submit=Submit'>LAST</a> "; } // if P.S. - You can test that this last change works simply by adding '&Submit=Submit' to the URL in your browsers address bar after you've done a search on your script.
-
I'm not sure why my change caused another problem with your LIMIT statement, I think I'm starting to see several other items that are causing your issue, so let's go through them. You are defining your category and section variables in your form using the POST method, so when you initially load the page, your $_POST variables are not yet set because you haven't submitted the form, which causes your query to return 0 results. We should add code to check if the form has been submitted. After you have submitted the form, the $_POST variables finally are set and your query successfully returns results. However, once you click on a link, the page refreshes and your $_POST variables are once again not set which causes your query to return 0 results again. Also, when your numrows variable gets set to 0 (because of your query not returning any results) this causes your next query to fail as well, so we should write a conditional for when no results are returned to avoid this. I would suggest submitting your form using the GET method, so that way your variables are passed in the URL (along with your pageno variable), and adding a conditional to check if the form was submitted. Let start by changing how your form was submitted to use the GET method. Change the line: <form name="form1" method="post" action="<?php echo $PHP_SELF?>"> to: <form name="form1" method="GET" action="<?php echo $PHP_SELF?>"> Next, let's add code to check if the form was submitted. Add the following lines of code around your existing code: // ... your HTML form ... <? // this is where your PHP code starts // Check if the form was submitted if ($_GET['Submit']) { // THE REST OF YOUR CODE } else { // The form was not submitted yet echo "Please search to see results..." } ?> // this is where your PHP code ends Next, let's change how your category and section vaiables get defined. Change the following lines: //GET FORM DATA $section = $_POST['section']; $category = $_POST['category']; To: //GET FORM DATA $section = $_GET['section']; $category = $_GET['category']; Next up, let's check if any results were returned when we set the numrows variable. After this line: $numrows = $query_data[0]; Add the following code: // Check if any results were returned if ($numrows < 1){ // No results returned, show a message echo "No Results Returned! Please try searching again!"; } else { And after these lines: //POPULATE TEXTFIELD WITH CSS CODE FOR TEMPLATE print "<tr><td><textarea name='textfield' wrap='OFF' cols='50' rows='7'>".$codes."</textarea></td></tr>"; print '</table><br /><br />'; } Add another closing bracket: } Lastly, in order to be sure that your section and category variables get passed between pages, you need to add them to each of your pagination links. Add the following line to the end of each link in your code: §ion=$section&category=$category So for the sake of clarity, here is the full code with my changes added: <!-- FORM TO DISPLAY DATABASE TEMPLATES --> <form name="form1" method="GET" action="<?php echo $PHP_SELF?>"> <select name="section" size="1" multiple> <option selected>layouts</option> </select> <select name="category" size="1"> <option selected>animals</option> <option>Anime</option> </select> <br> <input type="submit" name="Submit" value="Submit"> </form> <!-- END DISPLAY FORM --> <?php // Check if the form was submitted if ($_GET['Submit']) { //GET FORM DATA $section = $_GET['section']; $category = $_GET['category']; if (isset($_GET['pageno'])) { $pageno = $_GET['pageno']; } else { $pageno = 1; } // if require("connectDB.php"); //DATABASE CONNECTION SEQUENCE $query = "SELECT count(*) FROM upload WHERE category = '" . mysql_real_escape_string($category) . "'"; $result = mysql_query($query) or trigger_error("FATAL ERROR: " . mysql_error(), E_USER_ERROR); $query_data = mysql_fetch_row($result); $numrows = $query_data[0]; // Check if any results were returned if ($numrows < 1){ // No results returned, show a message echo "No Results Returned! Please try searching again!"; } else { $rows_per_page = 3; $lastpage = ceil($numrows/$rows_per_page); $pageno = (int)$pageno; if ($pageno < 1) { $pageno = 1; } elseif ($pageno > $lastpage) { $pageno = $lastpage; } // if $limit = 'LIMIT ' .($pageno - 1) * $rows_per_page .',' .$rows_per_page; $query = "SELECT * FROM upload WHERE category = '" . mysql_real_escape_string($category) . "'$limit"; print $query; $result = mysql_query($query) or trigger_error("FATAL ERROR", E_USER_ERROR); if ($pageno == 1) { echo " FIRST PREV "; } else { echo " <a href='{$_SERVER['PHP_SELF']}?pageno=1§ion=$section&category=$category'>FIRST</a> "; $prevpage = $pageno-1; echo " <a href='{$_SERVER['PHP_SELF']}?pageno=$prevpage§ion=$section&category=$category'>PREV</a> "; } // if echo " ( Page $pageno of $lastpage ) "; if ($pageno == $lastpage) { echo " NEXT LAST "; } else { $nextpage = $pageno+1; echo " <a href='{$_SERVER['PHP_SELF']}?pageno=$nextpage§ion=$section&category=$category'>NEXT</a> "; echo " <a href='{$_SERVER['PHP_SELF']}?pageno=$lastpage§ion=$section&category=$category'>LAST</a> "; } // if //FETCH SQL DATA AND PRINT IT TO THE SCREEN while($row = mysql_fetch_array($result)){ $id = $row["id"]; $codes = $row["codes"]; // $codes = ereg_replace("INSERTURLHERE", , $codes); NOT CURRENTLY USING THIS FEATURE print '<table width="400" border="2" cellspacing="0" cellpadding="0">'; //DISPLAY THUMBNAIL IMAGE FROM DATABASE print ("<tr><td><img src=\"download.php?id=$id\"></td></tr>"); //POPULATE TEXTFIELD WITH CSS CODE FOR TEMPLATE print "<tr><td><textarea name='textfield' wrap='OFF' cols='50' rows='7'>".$codes."</textarea></td></tr>"; print '</table><br /><br />'; } } require("disconnectDB.php"); } else { // The form was not submitted yet echo "Please search to see results..." } ?> Let me know how this works for you.
-
The issue appears to be with how you are calculating your $limit variable. Try changing this line: $limit = 'LIMIT ' .($pageno - 1) * $rows_per_page .',' .$rows_per_page; to this: $limit = ' LIMIT '.($pageno * $rows_per_page) - $rows_per_page.','.$rows_per_page;
-
This thread may get you on the right track: http://www.phpfreaks.com/forums/index.php/topic,131036.0.html Good luck!
-
You can check if a variable contains only numbers in several ways: [b]is_numeric()[/b] method [code] <?php $foo = 20 // <== your variable if( is_numeric($foo) ){ echo 'Variable is a number.'; } else { echo 'Variable is NOT a number'; } ?> [/code] [b]Regular Expression[/b] [code] <?php $foo = 20; if( preg_match('/^[0-9]$/', $foo)){ echo 'Variable is a number.'; } else { echo 'Variable is NOT a number'; } ?> [/code] I'm assuming that you'll eventually want to check other fields that may not be numbers, so I would recommend creating an validation / error handling class like the one mentioned at the link below: http://www.phpfreaks.com/tutorials/117/0.php
-
Passing The Table To Query From The Address Bar
HeyRay2 replied to JustinK101's topic in PHP Coding Help
I would say the most secure way to do this is to change to using $_POST variables for your table names? Is there a specific reason you are passing this information by way of $_GET? -
The code you last pasted is still malformed. The opening bracket ( [b]{[/b] ) before this line: [code]header('Location: http://'.$_SERVER['HTTP_HOST'].$_SERVER['PHP_SELF'].'?success');[/code] should be moved up to be right after this line: [code]if(mail($recipient, $subject, $message, $headers))[/code] So your code block should change from this: [code] <?php if(mail($recipient, $subject, $message, $headers)) # send confirmation email if($sendnotification == true) { $notification_message = "Thank you for contacting $websitetitle, $sender_name. We have received your email and will be in touch shortly"; $notification_subject = "Thanks for your message to $websitetitle."; mail($sender_email, $notification_subject, $notification_message, "From: $recipient"); { header('Location: http://'.$_SERVER['HTTP_HOST'].$_SERVER['PHP_SELF'].'?success'); } else { header('Location: http://'.$_SERVER['HTTP_HOST'].$_SERVER['PHP_SELF'].'?failure'); } } ?> [/code] ... to this ... [code] <?php if(mail($recipient, $subject, $message, $headers)) { # send confirmation email if($sendnotification == true) { $notification_message = "Thank you for contacting $websitetitle, $sender_name. We have received your email and will be in touch shortly"; $notification_subject = "Thanks for your message to $websitetitle."; mail($sender_email, $notification_subject, $notification_message, "From: $recipient"); header('Location: http://'.$_SERVER['HTTP_HOST'].$_SERVER['PHP_SELF'].'?success'); } else { header('Location: http://'.$_SERVER['HTTP_HOST'].$_SERVER['PHP_SELF'].'?failure'); } } ?> [/code]
-
Looks like it's printing the correct results for each page! :) Some formatting changes to your pagination link layout and you'll be all set! Glad to help! ;)
-
Geez! I totally missed this! :o Your code is not printing results from the right query (we're printing from the [b]$query_mem_1[/b] query, which was used to get the total amount of results, so we're always starting at result 0)! We should be using results from the the [b]$query_mem_1_limit[/b] query! Change this code: [code=php:0] // Determine the number of pages $num_pages = ceil($numOfRows / $max_results); // Determine the starting result to print $start_result = ($page * $max_results) - $max_results; // Append to our query with our result limit $query_mem_1_limit = $query_mem_1 . " LIMIT ".$start_result.",".$max_results.""; // Display the query echo $query_mem_1_limit.'<br>'; // Query for our current page of results $mem_1_limit = mysql_query($query_mem_1_limit, $connection) or die(mysql_error()); $row_mem_1_limit = mysql_fetch_assoc($mem_1_limit); // Print The results $numOfPageRows = mysql_num_rows($mem_1_limit); for ($i = 0; $i < $numOfPageRows; $i++){ $id = mysql_result ($mem_1, $i, "id"); $model = mysql_result ($mem_1, $i, "model"); $img = mysql_result ($mem_1, $i, "img"); $type = mysql_result ($mem_1, $i, "type"); $psi1 = mysql_result ($mem_1, $i, "psi1"); $maxflow = mysql_result ($mem_1, $i, "maxflow"); $use = mysql_result ($mem_1, $i, "use"); $cylinder = mysql_result ($mem_1, $i, "cylinder"); [/code] ... to this ... [code=php:0] // Determine the number of pages $num_pages = ceil($numOfRows / $max_results); // Determine the starting result to print $start_result = ($page * $max_results) - $max_results; // Append to our query with our result limit $query_mem_1_limit = $query_mem_1 . " LIMIT ".$start_result.",".$max_results.""; // Query for our current page of results $mem_1_limit = mysql_query($query_mem_1_limit, $connection) or die(mysql_error()); while( $row_mem_1_limit = mysql_fetch_assoc($mem_1_limit) ){ $id = $row_mem_1_limit['id']; $model = $row_mem_1_limit['model']; $img = $row_mem_1_limit['img']; $type = $row_mem_1_limit['type']; $psi1 = $row_mem_1_limit['psi1']; $maxflow = $row_mem_1_limit['maxflow']; $use = $row_mem_1_limit['use']; $cylinder = $row_mem_1_limit['cylinder']; [/code] That should fix things up for you! ;)
-
Why even have a hidden [b]action[/b] form element when you can just check if the form was submitted (if the user submitted the form, it can be assumed that they want to "register"). Take out this line in your form: [code] <input type="hidden" name="action"> [/code] And change these lines of code: [code=php:0] if (empty($_POST)){ $_POST['action'] = ""; } switch($_POST['action']) { case "register": create_account(); break; default: register_form(); break; } [/code] to this: [code=php:0] if ($_POST){ create_account(); } else { register_form(); } [/code]
-
I know that there were syntax errors in the code I posted ( Posted for example, not for direct consumption... ;) ). Please post [b]YOUR[/b] code, exactly as it is in [b]YOUR[/b] file, with [b]YOUR[/b] changes included. Also, add this... [code=php:0] // Echo the query echo $query_mem_1_limit.'<br>'; [/code] ...after this code: [code=php:0] // Append to our query with our result limit $query_mem_1_limit = $query_mem_1 . " LIMIT ".$start_result.",".$max_results.""; [/code] ;)
-
Comment out this line in your [b]admin_transact.php[/b] file, and post back if you still get the header error: [code=php:0] header('Location: admin.php'); [/code] [b]NOTE:[/b] You may be stuck at a blank page when you comment this out, but this will get us on the right track on what is causing your header error. ;)
-
You've modified the code that I posted. Please post the code [b]exactly[/b] as you are using it now, so I can see exactly where the issue lies. Right off the bat though, I can see that we need to globalize several variables for use in your [b]result()[/b] function. Add these lines to the top of the function: [code] <?php // Show results function result($psi01, $psi02, $gpm1, $gpm2, $use01, $mod, $page, $max_results) { global $psi, $gpm; // ... REST OF THE FUNCTION // ... // ... } ?> [/code] Thanks... ;)
-
The first problem I can see is that the value from these radio buttons... [code] <input type="radio" name="posttype" value="0"> General Update<br /> <input type="radio" name="posttype" value="1"> Picture Update<br /> <input type="radio" name="posttype" value="2"> Art Update<br /> [/code] ...are not being assigned to a variable in your [b]makeentry.php[/b] file. You have: [code=php:0] $posttype = $_POST['post_type']; [/code] but with the name of the input field being [b]posttype[/b], that should read: [code=php:0] $posttype = $_POST['posttype']; [/code] Next, this conditional will never be executed... [code=php:0] if((!$posttype) || (!$posttitle) || (!$post_body)) { if(!$posttype){ $post_type = 0; } if(!$posttitle){ $posttitle = "None"; } if(!$post_body) { echo "You need to make an update, duh!"; include "update.php"; exit(); } [/code] ... because right before it you defined all three of the variables you check the existance of. The variables may not contain any information, but they do exist. Try changing your conditional to (some syntax fixes included as well): [code=php:0] if( empty($posttype) || empty($posttitle) || empty($post_body) ){ if( empty($posttype) ){ $posttype = 0; } if( empty($posttitle) ){ $posttitle = "None"; } if( empty($post_body) ){ echo "You need to make an update, duh!"; include "update.php"; exit(); } } [/code] Your query looks correctly formatted, but if you could post the contents of your [b]db.php[/b] file, we can ensure that you're calling the DB connection properly. If you are getting any errors when loading the page, please post them here as well. Good luck... ;)
-
I think you're looking for: [code=php:0] ldap_modify($ds, $dn, $new); [/code] ;)
-
Here's a thread on OpenLDAP.org that deals with this issue. http://www.openldap.org/lists/openldap-software/200212/msg00200.html Happy reading ... ;)
-
A couple things with your code... [code=php:0] if ($num = '0'){ [/code] This line is using the assignment operator ([b]=[/b]), instead of the comparison operator ([b]==[/b]), which mean that when this line of code is executed, it is [b]ALWAYS[/b] reassigning the variable [b]$num[/b] to the string [b]'0'[/b]. This will cause the [b]if()[/b] part of your conditional to always be [b]TRUE[/b], even if there were result rows returned by your query. Change your code to look like this, and you should be good to go: [code] <?php $sql = "SELECT cart_id, stock_id, qty, price FROM t01_cart WHERE cart_id = '".GetCartId()."' "; $result = mysql_query($sql, $dbLink)or die ('sql error'.mysql_error()); $num = mysql_num_rows($result); if ($num == 0){ echo 'Your basket is empty'; } else { echo $num; } ?> [/code]