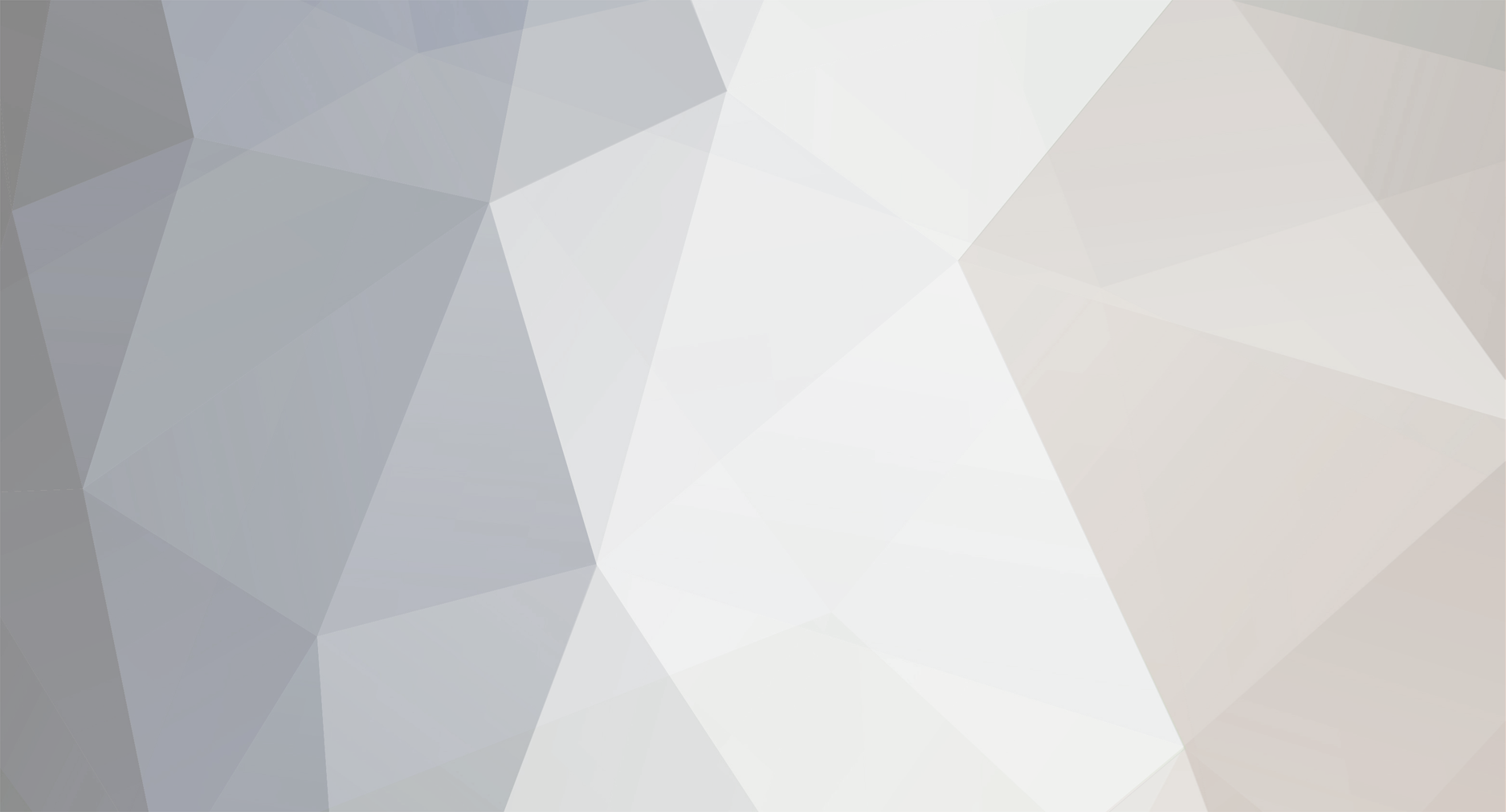
HeyRay2
Members-
Posts
223 -
Joined
-
Last visited
Never
Everything posted by HeyRay2
-
A lot of the information you get from the MySQL pagination tutorials around the web will still apply. Your source of data only differs. You'll still calculate how many results you have in total, how many you want to print out on each page, and you want to provide links to the other results. I would highly recommend reading some of those tutorials to get started, negating anything that is database related and replacing it with file functions. Here is a good one to start with: [url=http://www.phpfreaks.com/tutorial_cat/25/Page-Number--Pagination.php]http://www.phpfreaks.com/tutorial_cat/25/Page-Number--Pagination.php[/url]
-
What is the best way to link an image with a record in mysql
HeyRay2 replied to AdRock's topic in PHP Coding Help
You can use the auto-incremented ID of your last submitted news item for your image filename by doing something like the following: [code=php:0] <?php // The news insert query $sql = "INSERT INTO news SET title = '".$code['title']."'" //your insert statement, etc... // Run the query mysql_query($sql) or die("Error adding news: ".mysql_error()); // Image saving path $image_path = "path/to/images"; // Get the ID Of the last inserted news item $last_news_id = mysql_insert_id(); // Get the ID of the last inserted news item for the upload file name $image_filename = $last_news_id.".jpg"; // Put the image and path together $image_fullpath = $image_path . $image_filename; // Save the image move_uploaded_file($_FILES['image']['tmp_name'], $image_fullpath); // Update the DB entry $sql2 = "INSERT INTO news SET image = '$image_filename' WHERE news_id = $last_news_id"; mysql_query($sql2) or die("Error updating image name: ".mysql_error()); ?> [/code] Also, before we will be able to assist you more with why your line of code isn't working correctly, we need to see the code that you are using to display / add / update your news entries. ;) -
What code are you using on your other php pages to ensure that users cannot bypass your login screen? If you aren't already I would recommend using sessions. In your login code, add something like this: [code=php:0] if(mysql_num_rows($query) == 0){ echo "No such user found or login details are incorrect."; exit; }else{ session_start(); $_SESSION['username'] = $username; echo "Welcome back ".$username."!"; exit; } [/code] and on each page that you want to prevent unauthenticated users from accessing, add this around your page code [code=php:0] <?php // initialize the session session_start(); // Check if the user session was created if(isset($_SESSION['username'])){ // YOUR CODE HERE // YOUR CODE HERE // YOUR CODE HERE } else{ // direct the user to the login page Header("Location: /login.php"); } ?> [/code]
-
How to eliminate words (a-z) in a phone field?
HeyRay2 replied to steviechan83's topic in PHP Coding Help
I see the easiest method as requiring users to enter their phone number in XXX-XXX-XXXX format, and on your HTML form next to your phone input field, note this. If a user isn't smart enough to input their phone number in the method almost every other website in existence would require, then let them know the right way to do it. -
Not in PHP... But yes in JavaScript: Copied from [url=http://javascript.internet.com/forms/image-upload-preview.html]The JavaScript Source[/url] [code] <!-- TWO STEPS TO INSTALL IMAGE UPLOAD PREVIEW: 1. Copy the coding into the HEAD of your HTML document 2. Add the last code into the BODY of your HTML document --> <!-- STEP ONE: Paste this code into the HEAD of your HTML document --> <HEAD> <script type="text/javascript"> <!-- Begin /* This script and many more are available free online at The JavaScript Source!! http://javascript.internet.com Created by: Abraham Joffe :: http://www.abrahamjoffe.com.au/ */ /***** CUSTOMIZE THESE VARIABLES *****/ // width to resize large images to var maxWidth=100; // height to resize large images to var maxHeight=100; // valid file types var fileTypes=["bmp","gif","png","jpg","jpeg"]; // the id of the preview image tag var outImage="previewField"; // what to display when the image is not valid var defaultPic="spacer.gif"; /***** DO NOT EDIT BELOW *****/ function preview(what){ var source=what.value; var ext=source.substring(source.lastIndexOf(".")+1,source.length).toLowerCase(); for (var i=0; i<fileTypes.length; i++) if (fileTypes[i]==ext) break; globalPic=new Image(); if (i<fileTypes.length) globalPic.src=source; else { globalPic.src=defaultPic; alert("THAT IS NOT A VALID IMAGE\nPlease load an image with an extention of one of the following:\n\n"+fileTypes.join(", ")); } setTimeout("applyChanges()",200); } var globalPic; function applyChanges(){ var field=document.getElementById(outImage); var x=parseInt(globalPic.width); var y=parseInt(globalPic.height); if (x>maxWidth) { y*=maxWidth/x; x=maxWidth; } if (y>maxHeight) { x*=maxHeight/y; y=maxHeight; } field.style.display=(x<1 || y<1)?"none":""; field.src=globalPic.src; field.width=x; field.height=y; } // End --> </script> </HEAD> <!-- STEP TWO: Copy this code into the BODY of your HTML document --> <BODY> <div align="center" style="line-height: 1.9em;"> Test it by locating a valid file on your hard drive: <br> <input type="file" id="picField" onchange="preview(this)"> <br> <img alt="Graphic will preview here" id="previewField" src="spacer.gif"> <br> <div style="font-size: 7pt;"> Script submitted by: <a href="http://www.abrahamjoffe.com.au/">Sydney Wedding Video / DVD</a></div> </div> <p><center> <font face="arial, helvetica" size"-2">Free JavaScripts provided<br> by <a href="http://javascriptsource.com">The JavaScript Source</a></font> </center><p> <!-- Script Size: 2.43 KB -->[/code]
-
I'm glad you figured out your issue. I was mentioning lowering your number of DB calls, as you seem to be updating user information with an updated value, then pulling that [B]exact same[/B] value from the DB 1 line of code later, when you had the updated version of the value already set in a variable. I just don't see the need to check the status when you have a variable that tells you the status 1 line of code earlier. Not much could have changed in that fraction of a second. Anyway, if you want to try it, this should get rid of some of your db calls: [code=php:0] <?php // start if($_SESSION['controller'] === true) { // updates paid time period $select = "SELECT * FROM userinfo WHERE id = '$_SESSION[id]';"; $query = mysql_query($select);// query for info $row = mysql_fetch_array($query); // fetch array if ($row['timeperiod'] != "none") { // check status of timeperiod $currentdate = "08/23/06"; //date("m/d/y");// get's todays date $paydate = $row['paydate']; // get's db pay date $currentdate = strtotime($currentdate); // test later with later date $paydate = strtotime($paydate); $datetoupdate = "08/23/06"; //date("m/d/y"); $number_days = floor(($currentdate - $paydate)/86400); // 86400 = seconds per day $updatedversion = $row['timeperiod'] - $number_days; // new time period if($row['timeperiod'] != $updatedversion){ if($updatedversion <= 0){ $paid = "no"; $paypalid = "not applicable"; $paydate = "not applicable"; $timeperiod = "none"; $updatepayinfo = "UPDATE userinfo SET paid = '$paid', paypalid = '$paypalid', paydate = '$paydate', timeperiod = '$timeperiod' WHERE id = '$_SESSION[id]';"; $query4 = mysql_query($updatepayinfo); } else { $update = "UPDATE userinfo SET timeperiod = '$updatedversion', paydate = '$datetoupdate' WHERE username = '$_SESSION[username]';"; $query2 = mysql_query($update); } } } // Closes check for timeperiod equaling none. }// closes test to update paid ?> [/code]
-
[B]strtotime()[/B] has issues with certian date formats, so you're probably getting invalid timestamps when you pass the function a date in ("m/d/y") format. According to the [url=http://www.gnu.org/software/tar/manual/html_node/tar_109.html]GNU Dates Input Format[/url], one of the best recommeded formats is ("Y-m-d"). Ensure that the [B]paydate[/B] value in your database is in this same format, so you will be comparing timestamps correctly. Also, there should be no need for you to update the [B]paydate[/B] field in your database when you are simply checking is there are days left in [B]timeperiod[/B]. Also, this will cause you to have incorrect information on when a user last paid, so I would only update this value when a user pays for an extended time period, or their timeperiod expires. Lastly, you are making up to 5 database calls (3 "SELECT", 2 "UPDATE") where you only need 2 (One "SELECT", One "UPDATE"). Here is some revised code including my two recommendations: [code=php:0] if($_SESSION['controller'] === true) { // updates paid time period $select = "SELECT * FROM userinfo WHERE id = '$_SESSION[id]';"; $query = mysql_query($select);// query for info $row = mysql_fetch_array($query); // fetch array if ($row['timeperiod'] != "none") { // check status of timeperiod $currentdate = strtotime(date("Y-m-d"); // get todays date, changed to timestamp $paydate = strtotime($row['paydate']); // change paydate to timestamp $number_days = floor(($currentdate - $paydate)/86400); // 86400 = seconds per day $updatedversion = $row['timeperiod'] - $number_days; // new time period if ($row['timeperiod'] != $updatedversion) { if ($updatedversion <= 0) { $paid = "no"; $paypalid = "not applicable"; $paydate = "not applicable"; $timeperiod = "none"; $updatepayinfo = "UPDATE userinfo SET paid = '$paid', paypalid = '$paypalid', paydate = '$paydate', timeperiod = '$timeperiod' WHERE id = '$_SESSION[id]';"; $query4 = mysql_query($updatepayinfo); } else { $update = "UPDATE userinfo SET timeperiod = '$updatedversion' WHERE username = '$_SESSION[username]';"; $query2 = mysql_query($update); } } } } [/code] Let me know how this works out for you.
-
Because your combo box has to query a database for it values as the user selection changes, this is all done server-side. This means you have to send that change to the server for a new query, which will mean a page reload. However, you can make your script [B]appear[/B] to not reload using AJAX. You can read up on it here: [url=http://www.ajaxfreaks.com]AJAX Freaks[/url]
-
The working code example you mention calls a method on an object ([B]$Profile[/B]) regardless of whether or not a form has been submitted. Your code will fail with the "method on a non-object" if the following conditional is false: [code=php:0] if(move_uploaded_file($_FILES['uploaded']['tmp_name'], $target)) { [/code] This is because the [B]$upload[/B] object will not be defined in this case. You need to ensure that you don't make calls on [B]$upload[/B] if you have not defined it. That's what my code change does for you. The only part of your code I changed is the last 5 lines from: [code=php:0] } } $upload->parse("upload"); $page_content = $upload->text("upload"); [/code] to: [code=php:0] $upload->parse("upload"); $page_content = $upload->text("upload"); } else { // Error message. Upload failed die("Error Uploading!"); } } [/code] Did you try my change? Did it make any difference?
-
Perhaps... [code=php:0] $query = "SELECT * FROM product WHERE dept LIKE '%".$trimmed."%' OR type LIKE '%".$trimmed."%' OR prodName LIKE '%".$trimmed."%' OR shopName LIKE '%".$trimmed."%' ORDER BY dept, type, prodName, shopName, brand" [/code] Or you could look into setting up your database for full-text searching with [url=http://www.phpfreaks.com/tutorials/129/0.php]this PHP Freaks Tutorial[/url]. ;D
-
I don't believe you'll need to change any PHP code to modify buttons in Coppermine. Modifying your templates should suffice. There is a thread on this at the Coppermine forums: http://coppermine-gallery.net/forum/index.php?topic=24107.0
-
If you want PHP to get new values based on what you select in the first box, you'll have to submit the form with the value of that box and run a new query for the new values. If you want JavaScript to populate the new values, you will need to run a query in advance for each set of values the combo box can hold.
-
Perhaps... [code] <?PHP if (ereg(".inc.php",$HTTP_SERVER_VARS['PHP_SELF'])) { echo "<html>\r\n<head>\r\n<title>Forbidden 403</title>\r\n</head>\r\n<body><h3>Forbidden 403</h3>\r\nThe document you are requesting is forbidden.\r\n</body>\r\n</html>"; exit; } $target = "rosco/"; $target = $target . basename( $_FILES['uploaded']['name']); $ok=1; //This is the size condition if ($uploaded_size > 100) { $errorMsg = $lang['front']['upload']['error_filesize']; $ok=0; //This is the type condition } elseif (!($uploaded_type=="image/gif")) { $errorMsg = $lang['front']['upload']['error_filetype']; $ok=0; //Here we check that $ok was not set to 0 by an error } elseif ($ok==0) { $errorMsg = $lang['front']['upload']['upload_failed']; } else { if(move_uploaded_file($_FILES['uploaded']['tmp_name'], $target)) { $upload = new XTemplate ("skins/".$config['skinDir']."/styleTemplates/content/upload.tpl"); $upload->assign("LANG_UPLOAD_TITLE",$lang['front']['upload']['upload_title']); if(isset($ok) == 1) { $upload->assign("LANG_UPLOAD_DESCRIPTION",$lang['front']['upload']['upload_success']); $upload->parse("upload.session_true.no_error"); } elseif(isset($errorMsg)) { $upload->assign("VAL_ERROR",$errorMsg); $upload->parse("upload.session_true.error"); } else { $upload->assign("LANG_UPLOAD_DESCRIPTION",$lang['front']['upload']['upload_description']); $upload->parse("upload.session_true.no_error"); } if($ccUserData[0]['customer_id']>0) { $upload->parse("upload.session_true"); } else { $upload->assign("LANG_LOGIN_REQUIRED",$lang['front']['upload']['login_required']); $upload->parse("upload.session_false"); } $upload->parse("upload"); $page_content = $upload->text("upload"); } else { // Error message. Upload failed die("Error Uploading!"); } ?> [/code]
-
Multi-Column HTML Table Results from two Database Columns
HeyRay2 replied to kevdoug's topic in PHP Coding Help
The code for accessing your Oracle database won't change. You're simply changing the logic that prints out the values you pulled from the database, so you will only need the basic layout of the code example you are trying to use. Like so: [code] <?php $usrname = $_COOKIE[user_cookie]; $conn = OCILogon("kevin", "kevin", "") or die ("connection failed"); $stmt = OCIParse($conn, "select * from product where user_name = '$usrname' "); OCIExecute($stmt); $i = 0; // Your counter for which item in the column you are on $max_columns = 6; // The maximum columns per row // Start your table for your results echo "<table>"; // Run your while loop to start printing out your results while (OCIFetch($stmt)) { // Set your values to be printed in each "while" loop $img = OCIResult($stmt,'IMAGE'); $titl = OCIResult($stmt,'TITLE'); // open row if counter is zero if($i == 0){ echo "<tr>"; } echo "<td>"; // This is going to be a nested table to keep your results formatted correctly echo " <table> <tr> <td><a href=". $img ." target='new'><img src=' " . $img . "' height='75' width='100'></a></td> </tr> <tr> <td>$titl</td> </tr> </table>"; // close out the cell for the nested table echo "</td>"; // increment counter - if counter = max columns, reset counter and close row if(++$i == $max_columns) { echo "</tr>"; $i=0; } // end if } // end while // clean up table if we didn't fill a while row up to $max_columns - makes your code valid! if($i < $max_columns) { for($j=$i; $j<$max_columns;$j++){ // Print some blank cells to fill up empty space echo "<td> </td>"; } // Close the row if we are on the last column if($j == ($max_columns - 1)){ echo "</tr>"; } } // Release your database results and close the connection OCIFreeStatement($stmt); OCILogoff($conn); // End the table echo "</table>"; ?> [/code] -
Multi-Column HTML Table Results from two Database Columns
HeyRay2 replied to kevdoug's topic in PHP Coding Help
What problem are you having with the code? Are you getting error messages? Is your table not printing correctly? A little more information is needed before we can truly help... :) -
[url=http://www.phpfreaks.com/forums/index.php?action=search]http://www.phpfreaks.com/forums/index.php?action=search[/url] I'm sure it's been covered somewhere here before. Good luck... ;)
-
Anytime... :) This a great place for PHP help, so stop by any time you have a question! ;)
-
Because both queries rely on the same variable, [B]$Manufacturer[/B], you can run both queries one directly after the other. Also, please contain any pasted code into {code}{/code} (replace { and } with [ and ]) for easier reading for other forum readers.
-
We need a little more information before we can suggest the best coding method. What is your goal with searching the IP addresses? What is the purpose of the script? How are the tables in your database structured?
-
Anything within the quotes of... [code]echo " ";[/code] Will be printed to your web browser, so simply layout your table to how wildteen88 mentioned and you should be good to go... :)
-
Can you link the page you are creating so we can see exactly what you are seeing?
-
Forms can be submitted in either POST or GET methods, which you define as below: [code] <form name="myform" method="POST"> [/code] or [code] <form name="myform" method="GET"> [/code] The POST method is more secure, in that the form values are passed "behind-the-scenes", which is good for ensuring that the information is not broadcast in the URL and cannot be manipulated outsite of the script. I recommend this method for items like user login scripts and other items that have sensitive data to be passed. The GET method is less secure, but can a good choice where you want the form values passed through the URL. This is good for search scripts and the like where you would want a user to "bookmark" the page. Whichever method you choose, you can access the variables by the corresponding $_POST or $_GET variable array. Alternatively, you can use the $_REQUEST variable array for either form type, but be prepared to validate the contents of the variable because you won't know whether the information was passed by POST or GET.
-
Don't rely only on the file extension. Unscrupulous people will change them on you to upload malicious code and "unwanted" files on your server. A better method is to use an array of MIME filetypes and their associated extensions. This gives you two points of security that every file uploaded must adhere to in order to be considered valid. Like so: [code] <?php $valid_files = array(); $valid_files[0] = array("image/png", "png"); $valid_files[1] = array("image/jpeg", "jpg"); $filename = $_POST['file']; $ftype = $_POST['file']['type']; $fext = explode(".", $filename); // Since some people use "." in their filenames, we'll take the last item in the $fext array $fext = $fext[count($fext)-1]; // Set a variable to flag if we find a valid file. Set to 0 by default (not valid until we verify) $file_is_valid = 0; foreach($valid_files as $key => $value){ // $value[0] is the MIME type // $value[1] is the file extension if( ($value[0] == $ftype) && ($value[1] == $fext) ){ $file_is_valid = 1; } } if( $file_is_valid = 1 ){ // Upload file } else { // Error out. File is not allowed } ?> [/code]