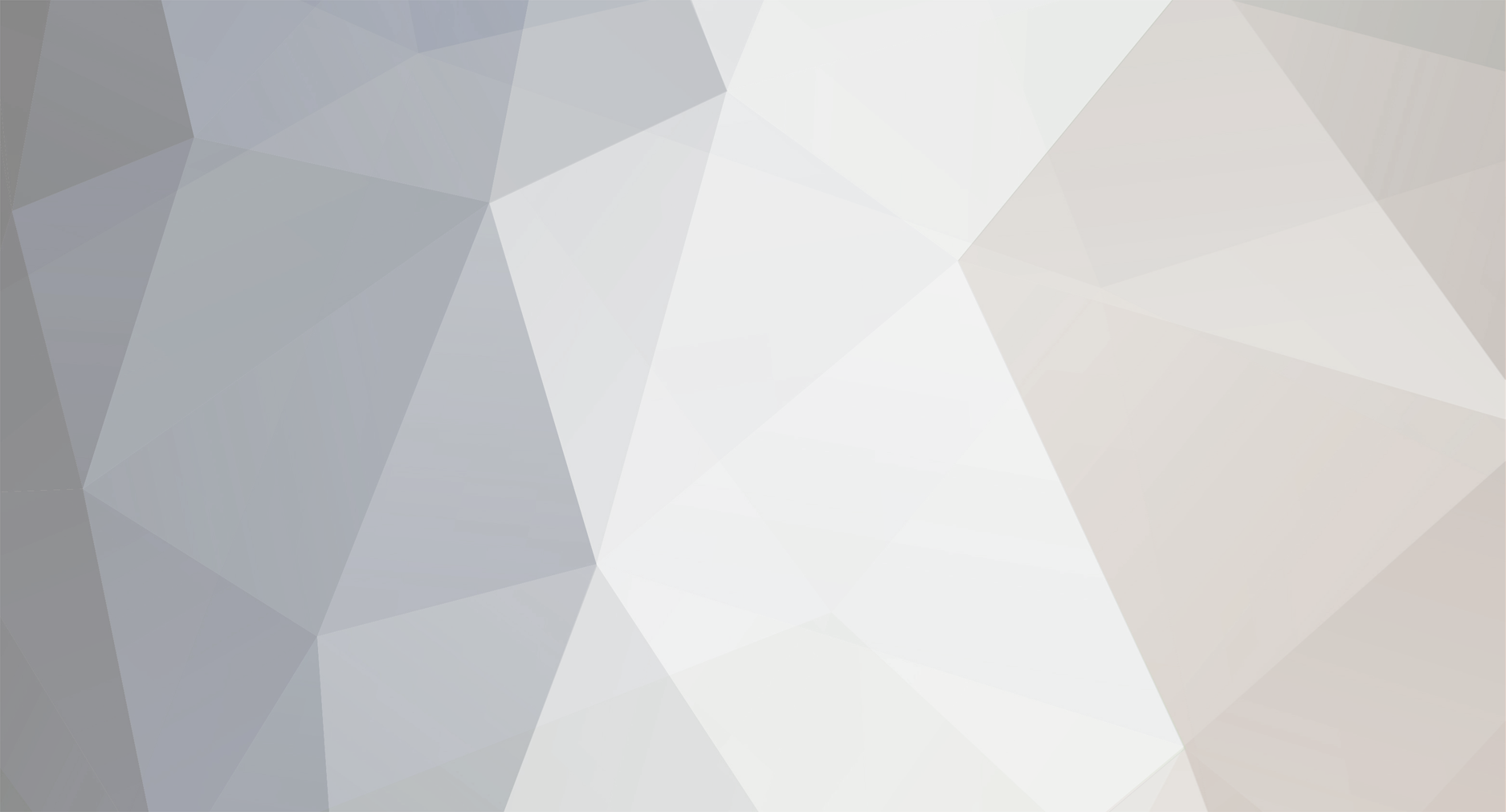
kevinkorb
Members-
Posts
52 -
Joined
-
Last visited
Never
Everything posted by kevinkorb
-
does the session id stay the same every visit?
kevinkorb replied to jwk811's topic in PHP Coding Help
How sessions work: PHP generates a session id and sets that value as a cookie to the user. The characteristics of the cookie are that it only lasts the browser session. (i.e. in internet expolorer once the browser is closed and re-opened, you will have a new session_id.) Firefox 2 treats sessions differently. I believe they call them persistant sessions. It is possible to stay on the same session from time to time of visiting the page even when closing the browser. Populate your eCart in IE and close the browser. Open it back up and the cart should be empty. If not then its not using a standard session, but probably a hand-rolled solution using a cookie. -
Mail Function Inquiry 2nd Time Around PLEASE HELP ME!!!
kevinkorb replied to neo777ph's topic in PHP Coding Help
I would think if you put your email address (or an email you could check) as the sender, any bounces would go back to you. -
does the session id stay the same every visit?
kevinkorb replied to jwk811's topic in PHP Coding Help
You may want to just check the User's IP address instead. [code=php:0] $ip = $_SERVER['REMOTE_ADDR']; [/code] Session id's change. Or you could set a cookie on the client's machine and do it that way. But then they can clear cookies or not accept them etc. -
One thing I've found is the server may respond... however its not healthy and might as well be down... For example.. if you put in http://www.mydomain.com and it comes up as an apache error cause by a bad config file. Or your mysql database is not working so your page comes up but nothing works so it might as well be shot. You could have them create a page called server_check.php which contains a read from the database. Then you call their site.. [code=php:0] $page_to_check = 'http://www.mytesthost.com/server_check.php'; $check_page = file_get_contents($page_to_check); if($check_page != 'The page worked, the db val is 345.') { $result = 'host is down'; } else { $result = 'host is up'; } [/code] This should be a little more valuable.
-
Actually $_SERVER['PHP_SELF'] would return just the filename with no querystring... i.e. http://www.yourdomain.com/index.php It will return '/index.php'; this will help you with your server variables. [code=php:0] <?php echo "<pre>"; print_r($_SERVER); echo "</pre>"; ?> [/code]
-
I understand the process... Can you provide any naming conventions used and how you're downloading the new files?
-
What I would recommend is not sending them the password at all. Do like most sites and send them a link to change their password. When the user wants a new password, it creates 2 random numbers and stores them in the users table. i.e. [code=php:0] $rand1 = rand(111111111,999999999); $rand2 = rand(111111111,999999999); [/code] Then send the user a link to their email that has http://mydomain.com/reset_password.php?rand1=736254629&rand2=837618390 Then verify that and let them set their new password. Upon reset set them new random numbers so that the link can't be used again. Then the password is safe and the unencrypted email means nothing anymore. My 2 cents..
-
Ummm. I'm not sure about the last post... however I've always read not to try your own encryption method. One effective thing is to make your pre-encrypted string is a long enough value that it would make rainbow-tables not work well. Ie. [code=php:0] $pass = $_POST['pass']; $pass = str_pad($pass, 20, '(', 'pad_right'); $enc_pass = md5($pass); [/code] Then the rainbow table would have to go up to 20 characters which would be like storing all values Since there is 95 printable ASCII characters they would have to store 95 to the 20th power of records in the table... which really is obsurd.
-
How would I create random display of multiple fields
kevinkorb replied to simcoweb's topic in PHP Coding Help
Change your query to "SELECT message FROM irclog ORDER BY rand() LIMIT 5" -
In here... while ($file = readdir($dir_handle)) { echo "<a href='$file'>$file</a><br/>"; } change to [code=php:0] while($file = readdir($dir_handle)) { if(substr($file, -4) == '.php') { $length = strlen($file); echo substr($file, 0, ($length-4)); } } [/code] Or that should be close at least... I didn't test.
-
The error actually refers to your data. You are manually entering in data for your 'primary key'. The primary key must be unique. Most people set their primary key as auto_increment and then don't assign it a value at all.. the database takes care of that by the first record inserted is 1 second is 2 and so on.... Check your primary key... then check the record you are inserting. You'll notice there is a record that already exists and the value of the primary key exists.
-
Your problem is here <td style="background:'.$style.'"> change to <td style="background:<?php echo $style;?>">
-
from root.. /usr/sbin/./updatedb then locate php.ini It will show you where your php.ini files are.. you may be fixing a wrong one. If you installed Zend Optimizer it re-locates your php.ini file.
-
From a text area when I use $myData = mysqli_real_escape_string($myData); It converts all my line breaks to actual text '\r\n' Any way to prevent this or recover from this. do I really have to run my data through [code=php:0] $string = str_replace('\r\n', ' ', $string); [/code]
-
SQL Code Stripping... preventing SQL injections?
kevinkorb replied to mschrank99's topic in PHP Coding Help
So then to use it.. (obviously) [code=php:0] $_POST = clean_array($_POST); //Or to leave the $_POST intact. $_clean = clean_array($_POST); [/code] -
SQL Code Stripping... preventing SQL injections?
kevinkorb replied to mschrank99's topic in PHP Coding Help
A way I like to clean my $_POST array... [code=php:0] <?php function clean_array($array) { $new_array = array(); foreach($array AS $key => $val) { $new_array[$key] = mysql_real_escape_string($val); } return $new_array; } ?> [/code] -
I'm trying to output it back into a textarea to look exactly like it did originally... With the nl2br it will be test1<br>test2<br>test3
-
When I post a string from a textbox, I then clean it for mysql... When I output the string to an update textarea, it actually comes out \r\n instead of the line breaks. i.e. $_POST['myTextArea']; $clean = mysqli_real_escape_string($_POST['myTextArea']); mysqli_query("INSERT INTO myTable (myVal) VALUES ('$clean');"); When getting the data back out Say I entered in.. test1 test2 test3 my textbox populates test1\r\ntest2\r\ntest3\r\n when I do a stripslashes on it it outputs test1rntest2rntest3rn How do I get the database to recognize the breaks and not literal text? Or how do I get the data out as planned? Thanks.
-
Ya, but the good thing about the other one is you can litter that all throughout your project. If you find a better escaping method you can just change the 'clean_array()' method once to use whatever logic you'd use to clean all your arrays. Abstraction But I you made a good point with the array_map function. So change the class to be [code] <?php Class Filter { public static function clean_array($array) { return array_map('mysql_real_escape_string', $array); } } ?> [/code]
-
Is this effective in preventing SQL injections.. it seems like it would be to me. What would make this not generally ideal to do before entering user submitted data into the database? [code] <?php Class Filter { public static function clean_array($array) { foreach($array AS $key => $val) { $array[$key] = mysqli_real_escape_string($val); } } } // Then cleaning the post array. $clean = Filter::clean_array($_POST); $query = "INSERT INTO table (f1,f2,f3) VALUES ('{$clean['f1']'}, '{$clean['f2']'}, '{$clean['f3']'});"; mysqli_query($db, $query); ?> [/code]
-
Here is my query:: SELECT sum( bcf.score ) AS score, pu.username, bc.timestamp, bc.timestamp, bc.comment, bc.id FROM blog_comments AS bc LEFT JOIN blog_comments_feedback AS bcf ON bc.id = bcf.comment_id JOIN peatot_users AS pu ON pu.id = bc.commenter_id GROUP BY bc.id ORDER BY score DESC LIMIT 0 , 30" The score can be negative or positive. However when there are no associated records in bcf, score is null which throws that after all the negatives. Any ideas. Thanks.
-
I compiled php 5.1.6, apache 1.3x, mysql 5.xx all from source and everything works well except mysqli doesn't seem to be installed. Here is my configure .. './configure' '--with-apxs=/www/bin/apxs' '--with-mysql=/usr/local/mysql' '--with-zlib-dir=/usr/include' '--with-jpeg-dir=/usr/local/lib' '--with-gd' '--with-curl' '--with-mysqli=shared,/usr/local/mysql/bin/mysql_config' '--enable-ftp' '--enable-soap' '--enable-trans-sid' '--enable-mcrypt' '--enable-mbstring' I am running CentOS 4 and I can't seem to find anything in the php.ini to enable (is that just windows?) Any help would be great. Thanks.
-
$string = 'the rapist 4'; I want to remove all non-alpha characters to produce $new_string = 'therapist'; Thanks a bunch... I'm sure this is cake for most of you.