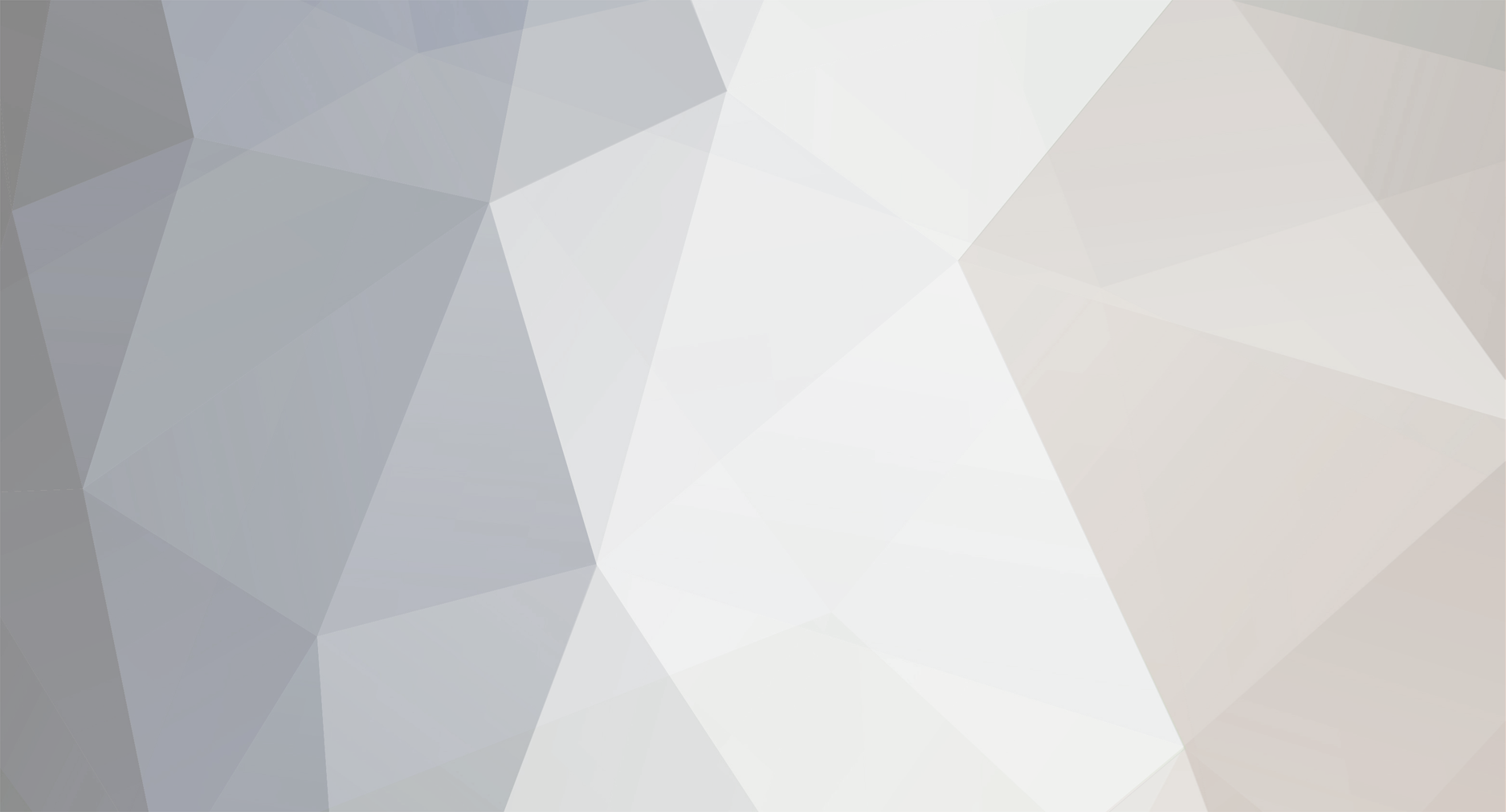
bltesar
Members-
Posts
109 -
Joined
-
Last visited
Never
Everything posted by bltesar
-
When you receive the values via GET or POST, store them in a session variable, say an array. [code] //get all your POSTed information and store it in a session variable(an array) foreach($_POST as $key=>$value) { $sent_values[$key]=$value; } $_SESSION['sent_values']=$sent_values; [/code] then if invalid data is received, you can send the client back automatically using [code] header('Location:http://mydomain.com/form_page.php?error=name'); //redirects back to form page exit(); //this must-have prevents subsequent code from running [/code] on your form page, get the sent_values session variable and load your form inputs with the values [code] //check for existence of session variable holding sent values if(isset($_SESSION['sent_values'])) { $sent_values=$_SESSION['sent_values']; //create variables from the array populated with the sent values foreach($sent_values as $key=>$value) { $$key=$value; } } //you can use the querystring appended at the end of the URL to print the error message if($_GET['error']=='name'){echo 'name is missing'); [/code] Then your form input elements look like this: <input type="text" name="input_1" value="<?PHP echo $input_1;?>"> you might want to destroy the SESSION variable so that the form clears on reload: [code] $_SESSION['sent_values']=array(); unset($_SESSION['sent_values']); [/code]
-
This really looks OK. Can you be more specific about the output of the page? I didn't understand the id 1.. in favs? yes-no; id 2.. in favs? no-yes.. id 3 in favs? no-no-yes.. ( id 4: being, not in favs.. ) no-no-no-no. where as it should be id1.. in favs? yes; id2.. in favs? yes; id3.. in favs? yes; id 4.. in favs? no.
-
I'm not familiar with PHP_AUTH_USER and how that gets set, but I have some suggestions that might help. First of all, to find out where the problem is, you could add to echo 'Authorization Required' .'user='.$PHP_AUTH_USER.', pw='.$PHP_AUTH_PW the problem might be in the setting of these variables. if that's not it, perhaps the usernames in your password.txt file are preceded by spaces or other characters
-
have you looked at the php.net info on this subject? http://us3.php.net/manual/en/ref.openssl.php
-
Perhaps it's too obvious, but your question doesn't really make sense. Please provide more info.
-
redirect user after email is found in SQL DB
bltesar replied to hey_suburbia's topic in PHP Coding Help
perhaps you have some blank spaces/lines following the final '?>' any such spaces/lines are sent as HTML and will cause the error you see -
Your question is somewhat ambiguous. you have - [quote]$result = mysql_query("SELECT * FROM replies WHERE id='$zid' ORDER BY id ASC"); [/quote] indicating that for each id, there are multiple results. Yet for each form generated for each record, you UPDATE the database for the same id. It seems to me if for one id you have ten different username/post pairs, and then you submit one of the forms and run your UPDATE query, that you will overwrite the ten existing records to the same value. lets say you have for each id multiple username/post pairs in your database. To maintain the multiple unique records for each id, you will need to create another id that identifies each unique pair. So your db will look like such- user_id post_id username post you then use post_id, the unique id for each username/post pair to name your form input elements: [code] elseif(!isset($submit)){ $result = mysql_query("SELECT * FROM replies WHERE user_id='$zid' ORDER BY id ASC"); while($row = mysql_fetch_array($result)){ $post_id=$row['post_id']; $name = $row['username']; $post = $row['post']; ?> <h2>::Edit Posts::</h2> <form method="post" action="<?php echo $PHP_SELF ?>"> Name: <input type="text" name="ename_<?PHP echo $post_id;?>" maxlength="20" value="<? echo $name; ?>"><br> Post:<textarea name="epost_<?PHP echo $post_id;?>"><? echo $post; ?></textarea> </form> <? } <input type="submit" name="submit" value="Update Posts"> [/code] then when you get your post variables, you can use the string functions on the keys to find the username/post pairs and create your database UPDATE queries.
-
Help - Wondering how to input a variable, analyze it, then output
bltesar replied to smc's topic in PHP Coding Help
You'll have to provide more specific and detailed information. What do you mean by 'level' and 'exp'? -
store paypal confirmation information in a database when received via GET, check the database to make sure the confirmation has not already been used for first time requests, set a SESSION variablle to indicate that downloading is authorized and require the buyer to get their download on that session only if you want customers to be able to return and re-download whatever it is they're buying, or their purchase allows access to multiple downloads over a period of time, you will need to set up user accounts with password access. If they are required to submit sensitive information that can be accessed by logging in, only then will they not give out their login information and allow others to get the downloads. of course, what's to stop people from emailing whatever they download? Now if my suggestions are not helpful, please do not be offended! More information might be needed.
-
It looks like you have omitted a lot of the actual code here. There is not enough information for me to make sense of it. A lot of the variables are not explained and the logical structure is incomplete. Post the entire code or at least more and maybe I can help.
-
Yup, the code was fine all along! Browser compatibility issues are really a pain.
-
I really couldn't sort through all your code, but I can tell you a few things that might help. First of all, I'm not sure, but I think 'go' is a reserved keyword in JavaScript. I recall naming something 'go' and it didn't work. I've created similar setups and I discovered that different browsers use different object models and the correct path to your frame can vary. Try this - if(top.site) { var rightframe=top.site; } else { var rightframe=parent.frames[1]; } use rightframe in place of your parent.frames.site
-
yes and no also work as values for these boolean attributes. 'auto' is not, however, a valid value.
-
I'm not sure, but there are ways to test for that. You could set a delay that is followed by an update to a database field, request the page and close your browser before the delay is up, and then check to see if the database was updated. It is a very interesting question, please let me know if you triy this test. I often figure things out by devising these sorts of tests because I often find it very difficult to locate the information I need in books. As for the second question, I'm not exactly sure what you're looking to do. One option might be to record the time of request in a database. When the user logs onto another computer, the time is gotten from the database, compared to the current time, and then the necessary delay time can be figured.
-
using PHP to authenticate password protected files/folders
bltesar replied to bltesar's topic in PHP Coding Help
Perhaps it would help if I gave a particular example. I have a folder that holds images, and access to that folder requires authentication. When I create a page that contains images from this folder and I access the page, a pop-up validataion box opens up. Rather than assign a username/password via server admin for each user, I want to have only one username/password and I'm wondering if there is a way to submit this username/password behind the scenes with PHP. The reason for this is that I want users to gain access by registering and creating their own username password and all the validation to be done using PHP/MySQL, but I also want server level authentication to block the images from the general public. -
Fix the first error and the rest will go away. What does your mysql_connect() statement look like?
-
Sorry, there were some mistakes in the last block of code, the function to be used for POST submits: [code] var openedWindow; get_data(user_selection){ //user_selection is sent via POST to process dynamic PHP page window.forms[0].user_selection.value=user_selection; if(openedWindow==null) { openedWindow=window.open("", "", "height=400, width=500, resizable=false, etc."); } window.forms[0].submit(); } [/code] set show_data.php as the form's action
-
There are a couple of ways that I know to do this. I assume that the liks from your calendar will all open the same window that is dynamically generated based on the user selection. Your links could look like this: <a href="javascript:get_data('thischoice');>text identifying this choice</a> and the function looks like this: [code] var openedWindow; get_data(user_selection){ //user_selection is sent via GET to process dynamic PHP page newURL='show_data.php?user_selection='+user_selection; if(openedWindow!=null) { openedWindow.location.href=newURL; } else { openedWindow=window.open(newURL, "", "height=400, width=500, resizable=false, etc."); } } [/code] the same can be done using the POST method. set newURL as the form's action and openedWindow as the target. The function then looks like this: [code] var openedWindow; get_data(user_selection){ //user_selection is sent via POST to process dynamic PHP page window.forms[0].user_selection.value='+user_selection; if(openedWindow=null) { openedWindow=window.open("", "", "height=400, width=500, resizable=false, etc."); } window.forms[0].submit(); } [/code]
-
your form tag should look like this: <form action = "search.php" onsubmit="verifForm(this)" method = "POST"> and your veriForm function should look like this: [code] function verifForm(formulaire){ if( formulaire.name.value == "") { alert('Le champ est vide !!!'); return false; } else { return true; } } [/code] and your submit button should look like this: <input type="submit" value="Envoye"> incidentally, you do not need to pass an argument to the veriForm function if the function will only operate on one form. Instead of the formulaire argument, you can use document.forms[0].name.value;
-
The PHP function sleep($secstodelay); will delay execution of your PHP code; however, if the client has, in the intervening time, browsed to another URL, I do not think the completed page will load. If I am wrong about that, then your problem is solved. If I am correct, I have another option. Use frames, one hidden and one visible. When the user clicks for your delayed window, set a JavaScript delay in the hidden frame, which, when time is up, changes the window.location.href property of the visible frame. That way, users can go whereever they want in the visible frame and your delayed page will load when ready. Keep in mind that once the href is changed, there can be another delay for the page to actually load in the window. During that time, if the user clicks a link, the href change request will be aborted. This behavior can be seen when you click one link on a page and before it changes you can click a different link and load that instead. To avoid this problem, you could use document.write() to erase the contents of the visible frame pending loading of your page. Finally, behavior of the back and forward browser buttons can vary with different browsers. I believe that most of the browsers out there will go back and forward within frames, which would work fine with the solutions presented.
-
What do you mean by automatically resized, and what exactly does it do in Mozilla but not IE? I tried it in IE and it opens exactly as I expected. My Mozilla browser insists on opening links in tabs, so I couldn't see what that does.
-
The window.open method takes the following arguments ("URL", "windowName" [,"windowFeatures"][,replaceFlag]) The scrollbar option is a boolean field, so you can put (in the 'windowFeatures' part) scrollbars=true, scrollbars=1, or just scrollbars omitting the 'scrollbars' is equivalent to scrollbars=false
-
christo, I checked into this and discovered that you can use the onSubmit as I previously described. put the onSubmit="valid_func()" in the <form> tag. have the function 'return true' for good data and 'return false' for bad data. The 'return false' will cancel the submission.
-
using PHP to authenticate password protected files/folders
bltesar posted a topic in PHP Coding Help
My server administration allows me to password protect files and folders. When attempts are made to access the protected files, a dialogue window opens requesting username and password. Can anyone tell me if it is possible, using PHP, to gain access to these protected files without the dialogue window opening? -
OK, where and how are the different rows to be displayed? Do you want the choice of one dropdown box to control the choices of another dropdown box?