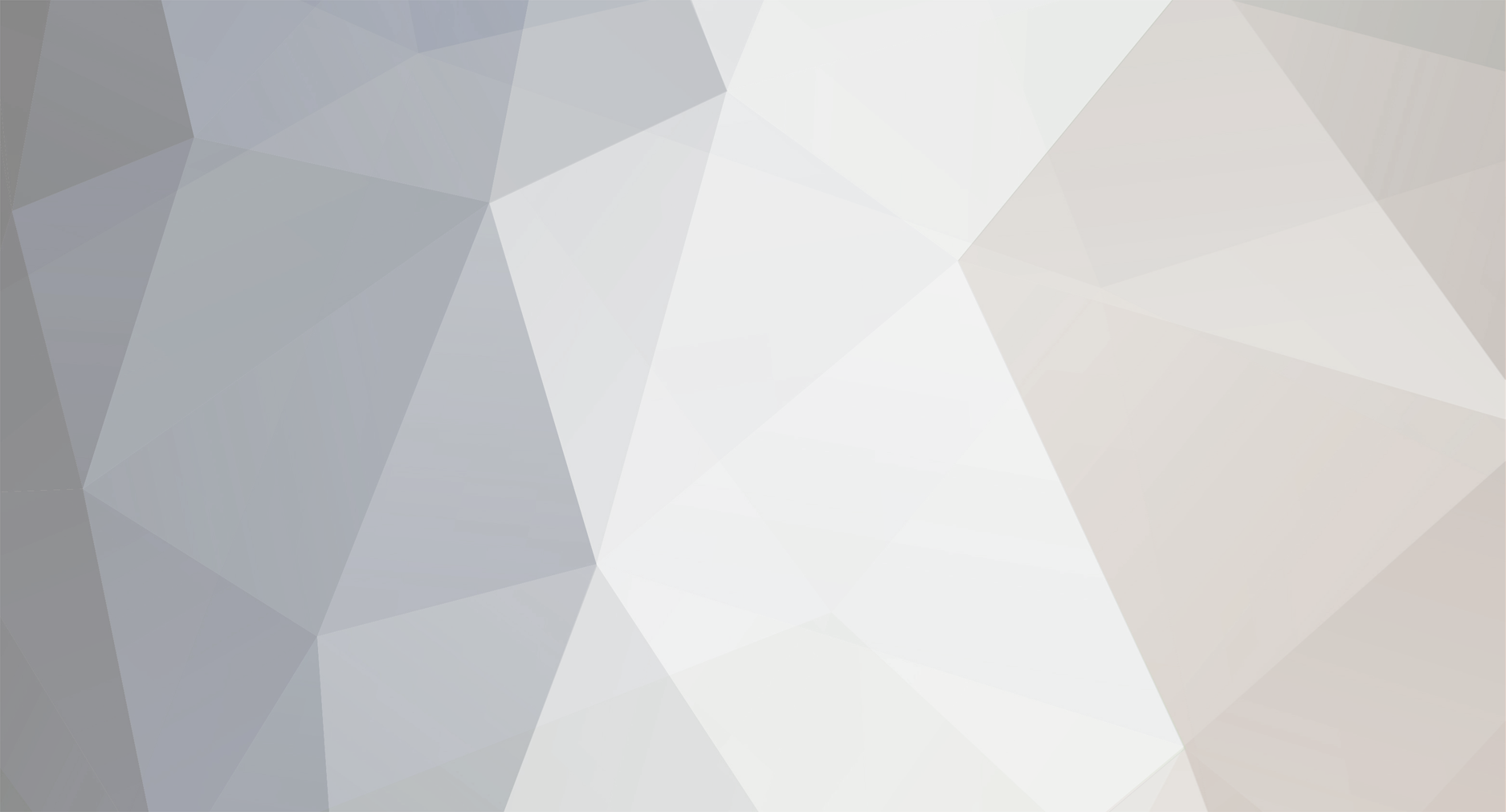
c4onastick
Members-
Posts
216 -
Joined
-
Last visited
Never
Profile Information
-
Gender
Not Telling
c4onastick's Achievements

Member (2/5)
0
Reputation
-
Try to stay away from the these: .+? or .*? Instead, use something more specific (and greedy) like this: $string = '[this] is a [example] string [end]'; echo preg_replace('/\[[^\]]+\]/', '', $string); Cheers!
-
I'd use the preg suite. In general, it's much better than ereg. I would modify the whole section of code work something like this: $filename = 'word1-word75-elephant.html'; $filename = preg_replace('/\..+$/', '', $filename); //chop off the extension $filenames = preg_split('/-/', $filename); //split the words into an array $filename = join(' ', $filenames); //rejoin the array with spaces print_r($filename); Or I suppose you could cut out the middle-man and use: $filename = 'word1-word75-elephant.html'; $filename = preg_replace('/\..+$/', '', $filename); $filename = preg_replace('/-/', ' ', $filename); print_r($filename);
-
I suppose to take a stab at answering your original question about a phone number validating regex, I'd do something like this: /^1?([-_. ])?\(?\d{3}\)?(?(1)\1|([-_. ])?)\d{3}(?(2)\2|([-_. ]))\d{4}$/ Validation gets tricky and ugly quick in regex. This might not be any easier to wrap your head around but here's the break down (I've bolded the parts in question): Regex: ^1?([-_. ])?\(?\d{3}\)?(?(1)\1|([-_. ])?)\d{3}(?(2)\2|([-_. ]))\d{4}$ Matches an optional '1' at the beginning, as in 1-888-555-1234. Regex: ^1?([-_. ])?\(?\d{3}\)?(?(1)\1|([-_. ])?)\d{3}(?(2)\2|([-_. ]))\d{4}$ Matches the optional punctuation between the '1' and the area code, as in 1-888-555-1234. Regex: ^1?([-_. ])?\(?\d{3}\)?(?(1)\1|([-_. ])?)\d{3}(?(2)\2|([-_. ]))\d{4}$ These match optional parenthesis around the area code, as in (888)555-1234. Regex: ^1?([-_. ])?\(?\d{3}\)?(?(1)\1|([-_. ])?)\d{3}(?(2)\2|([-_. ]))\d{4}$ Matches the three digit area code. Regex: ^1?([-_. ])?\(?\d{3}\)?(?(1)\1|([-_. ])?)\d{3}(?(2)\2|([-_. ]))\d{4}$ This is where it really gets complicated, this is a regex conditional (if, then, else) construct. It says if the first parentesis (blue) matched, match whatever was matched before. Otherwise, match one of the optional separators. Regex: ^1?([-_. ])?\(?\d{3}\)?(?(1)\1|([-_. ])?)\d{3}(?(2)\2|([-_. ]))\d{4}$ Again match three digits, as in 888-123-4567. Regex: ^1?([-_. ])?\(?\d{3}\)?(?(1)\1|([-_. ])?)\d{3}(?(2)\2|([-_. ]))\d{4}$ Here's the next tricky part, it's possible the first capture will fail so in that case the (green) parenthesis will have our delimiter in it. In the case that that also fails, match a required delimiter out of the character class. (You could also make this optional to allow numbers like 8881234567 or (888)1234567 in) Regex: ^1?([-_. ])?\(?\d{3}\)?(?(1)\1|([-_. ])?)\d{3}(?(2)\2|([-_. ]))\d{4}$ Finally, match four digits at the end of the string, as in 888-123-4567. Now it is true that you don't have to use that conditional regex here, but if you leave it out, it'll let things like 1-888_123.4567 in which I'm not sure you want. The regex for that would look like this: /^1?([-_. ])?\(?\d{3}\)?([-_. ])?\d{3}([-_. ])\d{4}$/ I fear I may have muddied the waters a bit, but hopefully that helps. Cheers!
-
Ah! The picture is becoming clearer! '^' (like so many things in programming) means two different things depending on it's context. Outside of a character class (things inside [square brackets]) it's an anchor. Meaning it matches a position in a string not a character. '^' matches the beginning of the string. However, as the first character inside a character class (like I used above) it negates the character class. [^\d] Means match anything that's not a digit.
-
The slashes at the beginning and end of the regex are the delimiters, they essentially mark where the pattern starts and ends. Regex is handy in this respect in that you can define your own delimiter. I use '%' a lot for using regex on HTML or XML since there's lots of slashes in the text (this saves you from having to escape them all). Parenthesis in regex are used for two things, capture and grouping. What are you going to do with the telephone number in the above example? People have a billion different ways of entering phone numbers (ex. 555-123-4567, 555.123.4567, 555123456, etc. like your example above) so it might be easier to just strip everything that's not a number and then make sure there's 9 to 10 digits there. In PHP that'd look something like this: $phone_no = '1-555-123-4567'; $phone_no = preg_replace('/[^\d]/', '', $phone_no); if(strlen($phone_no) == 10){ echo '10 digits'; //Do something with 10 digits }elseif(strlen($phone_no) == 11){ echo '11 digits'; //Do something with 11 digits }else{ echo 'Busted phone number'; //Throw an error } Hope that helps!
-
[quote author=spookztar link=topic=119794.msg716635#msg716635 date=1192368723] 1) Using "^" and "$" isn't really required when using preg_match(), is it? [/quote] Wrapping your regex in "^" and "$" ensures that the match is applied to the entire file name. It anchors the regex to beginning of the string ("^") and the end of the string ("$") and pretty much says, "the beginning to the end must match this". [quote author=spookztar link=topic=119794.msg716635#msg716635 date=1192368723] 2) To better accomodate any future formats, wouldn't it be more advisable to just specifically exclude certain dangerous extensions? [/quote] You can do that, but most of the time it's better to create an alternation of what you explicitly want to allow. Sometimes new dangerous extensions can creep in unexpectedly if you just exclude a few.
-
Ok, this is a much higher level design than what I'm doing (or have ever done, for that matter). Let me see if I can't walk you through it to make sure I've got the logic correct. (And to clarify for anyone that reads this later.) First, create a factory class: class PageFactory { ... } Define a constructor function that is invoked when the PageFactory class is instanced: public static function construct($classname) { ... } If the string (class) passed via $classname has not been defined or implemented: if !((class_exists($classname) || interface_exists($classname))) { ... } Deconstruct the classname based on the PEAR naming scheme, to set the path to the class: $root = dirname(__FILE__); // current directory - assuming it's the root $path = str_replace("_", DIRECTORY_SEPARATOR, $classname); Require the class: require $root . DIRECTORY_SEPARATOR . strtolower(dirname($path)) . DIRECTORY_SEPARATOR . basename($path) . ".php"; And return an new instance of the class: return new $classname; So, if we wanted to implement the factory to display our pages it would be invoked like this: <?php require_once('PageFactory.php'); $page = new PageFactory('Pages_DatabaseContent'); // Where, using the PEAR naming convention, the DatabaseContent class would be located // in /usr/local/www/pages/DatabaseContent.php assuming that the document root is // /usr/local/www/ // Call the display or execute function in the DatabaseContent class $page->execute(); ?> Is that right? Very interesting, so essentially, the factory interprets the argument, finds and requires the correct file containing the class and hands off to the class specified in the argument. I would be very interested to hear how you manage include files. An efficient system would allow for much modularity and encapsulation. Thank you again for your insights!
-
How do you handle page conent both modularly and dynamically?
c4onastick replied to c4onastick's topic in Application Design
I posted in the OOP Child Board under PHP help. The link in my previous post should work. -
Continuation of this thread. How do you implement dynamic content using factories and reflection? Let's say I have a class for displaying a page that looks something like this: <?php class page { var $content; function display() { $this->display_header(); $this->display_body(); $this->display_footer(); } private function display_header() { echo "<html>\n<head><title>Practice with OO PHP</title></head>\n"; } private function display_body() { echo "<body>$this->content</body>\n"; } private function display_footer() { echo "</html>"; } }?> And a sub classes that displays specific content: <?php class page_with_content extends page { function display() { $this->display_header(); $this->get_content($arg); $this->display_body(); $this->display_footer(); } function get_content($arg) { //Gets special content here, possibly database calls $this->content = $content_from_above_db_calls; } }?> How do I implement a factory or reflection to dynamically get instances of different pages?
-
How do you handle page conent both modularly and dynamically?
c4onastick replied to c4onastick's topic in Application Design
Thanks for your insight. 1) Multiple files/pages actually would be easier to maintain, and class inheritance would minimize most of my upkeep time. I do like the concept too. 2) I currently have a setup sort of like that. Currently I have an invocation roughly following this: <?php $page = new page_class(); $content = new page_content(); ... $page->content = $content->get_content(); $page->display(); ?> I just have a attribute of page_class that holds the place where the content is to go. I then use the page_content class to fill page_class' content variable. Two completely separate objects linked by one assignment. 3) This is the place where I would like to get to. I understand sub-classing the pages to reflect their individual content, but I'm not sure how to do it dynamically through a "factory" or via "reflection". I would love it you could point me in the right direction for getting a factory or some reflection techniques working. I've started a thread here where we can continue this discussion, thanks again for your help! -
How do you handle page conent both modularly and dynamically?
c4onastick replied to c4onastick's topic in Application Design
Thanks for the quick reply! Actually I'm not really hell bent on that. I would, however, like to minimize the upkeep effort (since I almost always end up maintaining them all anyway), make it as modular as possible so as facilitate easy expansion (since the I never quite know what I want the first time around), and expand my programming knowledge by applying some good design principles (always looking to learn something new to do it better!). I had thought of using a hash in that manner and at this point its beyond me why I decided against it. Reflection? Please do continue, if you wouldn't mind, I'm just getting off the ground with OO concepts any chance I get to apply them is great! Thanks again! -
Hello everyone, It has been a little while but I'm back to programming PHP. I've been working on a few "fun" projects and my "hammer" solution for displaying page content is what I'd like to improve upon. Usually I find myself writing a class to display the page, it simply prints all the required bits and bobs in the correct order in the desired format. What changes is the content in the central div. Since all these sites are database driven there are numerous db calls to create the content that's displayed in the content div. Previously, I'd always written a monstrous case block: if($_GET['page'] == 'home'){ //home content here }elseif($_GET['page'] == 'form'){ //db calls and other content here }elseif(... ... } return $content; That way I could use one "page" (index.php) to display all the content from. How do people usually handle getting content like this to the page that displays it? Any links or suggestions would be great! Thanks in advance!
-
"\\" should work. Windows (you wouldn't have this problem on a *NIX machine) has some issues with that though, I've had to use four "\\\\" to get what I wanted before. Moral of the story: try more!
-
I'm working on a eBay listing template system (more on that later) and I would like some advice and input from you all before I get neck deep in unusable code. My client runs his own business which utilizes eBay frequently (albeit not exclusively) for some of his sales. He hired another programmer and myself to design a standalone UI (using eBay's API) for him to streamline some of his more repetitive listings. The lead programmer (and myself) would later like to release what we come up with under the GPL so we're aiming to keep this as modular as possible. We've already discussed writing it in strict OO, (interestingly enough, I was the one pulling for OO -- its usually the opposite) and settled on using more of a 'globals.php' (file with a bunch of reused functions and variables) include approach instead. Here's a quick run down of the current features we're planning: FTP 'redirect' of uploaded pictures -- Upload pictures to the webserver and redirect them to another server via FTP Image resizing Template engine -- Allowing certain parts of the listing to be saved to a template to cut down on the 'copy-paste' portion of a similar listing. We'd like to keep it modular so that anyone in the future could come in and add another module and use it. We ran into a few differences in opinion during our first couple of design meetings (aside from the OO issue) that I'd like to hear everyone's input on. Basically, we'd like to make the UI as fluid as possible, reacting both to the loaded template (or the default) and to the modules that the template employs. So far we've decided on generating a 'configuration' file that we can just write config variables (FTP server address, username, etc.) to and just require in the main UI page. Usually I've seen this done using variables ($var1, $var2, etc.), however the issue came up that we could define them as constants and in doing so protect them from being overwritten. Is this a good way to do this? Some of the configuration settings need to be aware of what template is being used (to get the required information for global variables from the user). I had initially thought of writing a module parser to identify both the modules and it's required globals (and their type), but the more I write of it, the more it seems like there's a better way (I don't have access to a DB, one of the constraints of this project). How can I more easily make the configuration file aware of the current template's module and variable requirements? (We did discuss caching this in a session variable.) It really boils down to the fact that I need to incorporate two different sets of data: the 'behind the scenes' information and the listing template data. The problem we're running into is that it's definately turning into a cyclic reference. The template defines something that needs to be in the configuration file but the configuration file has to know what the template needs. So far we've proposed two solutions. Parse the modules, and define everything in the configuration file (even if its not needed). Separate (read: add another step for the user) the basic configuration and the module configuration. Any thoughts (or links with helpful articles) would be much appreciated. Thanks in advance!