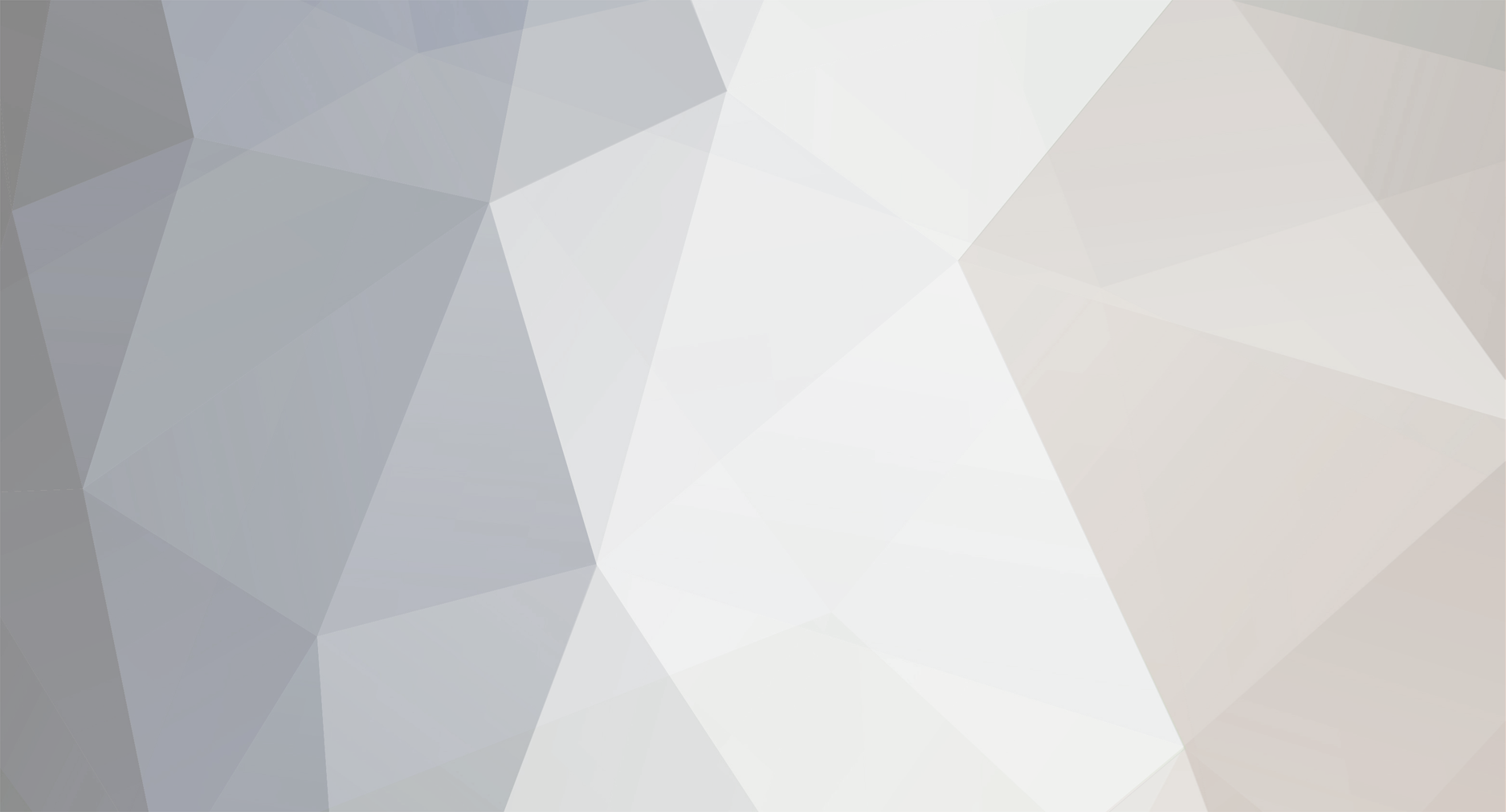
Unholy Prayer
-
Posts
166 -
Joined
-
Last visited
Never
Posts posted by Unholy Prayer
-
-
Well the length is 500 but when it's text my program won't work.
-
For some reason PHPMyAdmin isn't letting me change one of my fields from TEXT to VARCHAR. I have all the necessary fields filled out but it doesn't change when it goes back to the table structure. This is causing my PHP program to not work correctly. Anyone know what could be causing this?
-
Hmm... I'll give it a try.
-
I'm making my own forum for my website and I wanted to make subforums. I was wondering if I should make a whole separate database table or if I should just add an extra field in the forums table? Anyone know the best way to do this?
-
So how would I make {SITE_NAME} display the site's name if I were using this code:
$variables = assign_block_vars('header', array( 'SITE_NAME' => $sitename, 'SLOGAN' => $slogan) );
-
Did you try looking at hotscripts.com? They have alot of good PHP programs and I think they have some PHP calendars.
-
I'm not writing a mod for PHPbb, but so far I have 'SITE_NAME' => $sitename, as one of my things and I have {SITE_NAME} in one of my tpl files but it still displays {SITE_NAME} on the browser page.
-
Ok, So how do I get something like {SITE_NAME} to display a string that gets the site name from a database? If my code is
$settings = mysql_query("select * from ubs_settings where id=1");
while($s=mysql_fetch_array($settings))
{
$sitename=$s["sitename"];
$slogan=$s["slogan"];
}
how would I make {SITE_NAME} to display $sitename?
-
Yeah, I didn't understand that at all. You're going to have to explain that a bit for me. Thanks.
-
Ok, I've seen in PHP programs such as PHPbb2 where they use I think arrays to use things like {SITE_NAME} instead of $sitename. I keep seeing things like 'RANK_IMAGE' => $rank_image, and what not. How do I do this?
-
Ah, now it's working. Thank you very much for your help.
-
It's still not doing what I want it to. It isn't adding the semicolon at the end of the lines which is causing the configuration file to not work correctly.
-
Will those strings work in a database configuration file even though they don't have the $ in front of it? Or is there a way to write to certain lines of the page you're writing the content to?
-
It kinda worked but I didn't save the form as an HTML file. I just changed the GET function to POST function but it didn't include the strings, it just wrote the data from the inputs.
-
Hmm... forgot the permissions but then after i changed them, all it wrote to the file was this:
= '';
= '';
= '';
= '';
-
I'm trying to create an install file for my program. I have a form where the user inputs the database information and strings to represent the GET function for each of the inputs. How would I be able to use the fwrite(); function to write different strings to a file? Here's my coding to show what I mean. Any help would be greatly appreciated.
<?php echo "<table align='center' cellspacing='1' cellpadding='1' border='0'> <tr> <td align='center' colspan='2'>MySQL Info</td> </tr><tr> <form action='install.php' method='POST'> <td align='right'>Database Name:</td> <td align='left'><input type='text' name='db_name' size='30'></td> </tr><tr> <td align='right'>Username:</td> <td align='left'><input type='text' name='username' size='30'></td> </tr><tr> <td align='right'>Database Password:</td> <td align='left'><input type='text' name='password' size='30'></td> </tr><tr> <td align='right'>Host Name:<br><small>Usually 'local host'</small></td> <td align='left'><input type='text' name='host' size='30'></td> </tr><tr> <td align='center' colspan='2'><input type='submit' name='Submit' value='Submit'></td> </tr> </form> </table>"; if($_POST['Submit']) { $dbname = $_GET['db_name']; $username = $_GET['username']; $password = $_GET['password']; $host = $_GET['host']; $file = fopen("config.php", "w"); fwrite($file,"$database = '$dbname'; $user = '$username'; $pass = '$password'; $dbhost = '$host';"); fclose($file); echo "The configuration file was successfully updated. Click below to continue with the installation."; } ?>
-
I changed both of my navigation menus to lists but it's still not doing what I want it to. Can someone please help me with this?
-
This is what I use to do this.
Say the user clicks on a link that's index.php?id=2
<?php echo "<a href='test.php'>Main</a> <a href='test.php?id=2'>Other Page</a>"; $id = $_GET['id']; //If the id is blank or it's just index.php... if($id == "") { echo "<p align='center'>Welcome to the index page.</p>"; } //If the id is equal to "2"... if($id == "2") { echo "<p align='center'>Welcome to the other page</p>"; } ?>
Hope this helps.
-
Bump.
-
You should try position: absolute; bottom: 0; that should attach your div to the bottom of the page at all times.
Hint: to clean up your code a little bit you don't need all those divs on the nav links. you could:
1.
<a href="index.php" class="navlink">Index</a><br />
2. Have:
#nav a { background-color: #ffecec; text-align: right; color: #ea9898; font-family: arial; font-size: 10px; padding: 3px; vertical-align: middle; width: 150px; border-bottom: 1px solid #ea9898; border-right: 1px solid #ea989a; border-left: 1px solid #ea989a; margin-bottom: 0px; margin-top: 0px; }
with
<a href="index.php">Index</a>
hope that helps!
-Chris
Uh, that kind of made it worse with the position attribute. http://www.dev.mtechdev.com/portal/index.php
-
Ok, I am trying to make my edit content page so you click on a looped link and the link takes you to a form where you can edit the table row for it. I'm thinking it's my navigation page that isn't working. I would greatly appreciate it if someone could help me. Hint: The code stops functioning the way I want it to towards the bottom at the if statement to start the edit page. Here's my code:
<?php require_once('config.php'); echo "<link rel=stylesheet href='style.css'>"; echo "<table align='center' cellspacing='1' cellpadding='1' border='0'> <tr> <td align='center' colspan='4'>Ultimate Affiliates Manager v1.0 Administration Center</td> </tr><tr> <td class='button'><a href='admin.php?act=add'>Add</a></td> <td class='button'><a href='admin.php?act=edit'>Edit</a></td> <td class='button'><a href='admin.php?act=delete'>Delete</a></td> <td class='button'><a href='admin.php?act=view'>View</a></td> </tr><tr> <td align='center' colspan='4'><a href='admin.php'>Admin Index</a></td> </tr><tr>"; //What page should we be viewing? $act = $_GET["act"]; //If the action is blank... if($act == "") { echo "<td colspan='4'><p align='center'><b>Welcome</b></p></td> </tr><tr> <td colspan='4' class='content' width='50%'>Welcome to the administration center of the Ultimate Affiliates Manager v1.0. From this area you can manage all of your affiliates. You can add new sites, delete sites, or modify existing sites. You can even view your existing affiliates. Just use the navigation area at the top of your screen to work your way around the admin panel.</td>"; } //If the action is "add"... if($act == "add") { //If the form was submitted... if($_POST['Submit']) { //Shorten up the variables to insert the information. $site_name = $_POST['site_name']; $description = $_POST['description']; $site_url = $_POST['site_url']; $banner_url = $_POST['banner_url']; //Our handy database insertion code mysql_query("INSERT INTO affiliates (site_name,description, site_url,banner_url) values('$site_name', '$description', '$site_url', '$banner_url')"); echo "<p align='center'>The site has been added to your affiliates.<br>Click <a href='add.php'>here</a> to return to the Add Affiliates page.</p>"; } //If the form was not submitted... elseif(empty ($_POST['Submit'])) { //Display our form. echo "<table align='center' cellspacing='1' cellpadding='1' border='0'> <tr> <td align='center' colspan='2'><p align='center'><b>Add an Affiliate<b></p></td> </tr><tr> <form action='add.php' method='POST'> <td align='right'>Site Name:</td> <td align='left'><input type='text' name='site_name' size='30'></td> </tr><tr> <td align='right'>Description:</td> <td align='left'><input type='text' name='description' size='30'></td> </tr><tr> <td align='right'>Site URL:<br><small>Must include http:// at the beginning.</small></td> <td align='left'><input type='text' name='site_url' size='30'></td> </tr><tr> <td align='right'>Banner URL:<br><small>Must include http:// at the beginning.</small></td> <td align='left'><input type='text' name='banner_url' size='30'></td> </tr><tr> <td align='center' colspan='2'><input type='submit' value='Submit' name='Submit'></td> </form> </tr> </table>"; } } $aid = $_GET["id"]; if($act == "edit") { $affiliates = mysql_query("SELECT * FROM affiliates"); while($a=mysql_fetch_array($affiliates)) { $id=$a["id"]; $affiliate_name=$a["site_name"]; $description=$a["description"]; $site_url=$a["site_url"]; $banner_url=$a["banner_url"]; echo "<td align='center' colspan='4'><a href='$site_url' target='new'>$affiliate_name<br><img src='$banner_url'></a><a href='admin.php?act=edit&id=$id'>[X]</a></td> </tr><tr>"; } if($aid == "$id") { $display = mysql_query("SELECT * FROM affiliates WHERE id = $aid"); while($d=mysql_fetch_array($display)) { $affiliate_name2=$d["site_name"]; $description2=$d["description"]; $site_url2=$d["site_url"]; $banner_url2=$d["banner_url"]; } if($_POST['Edit Affiliate']) { $affiliate_name3 = $_POST["site_name"]; $description3 = $_POST["descriptoin"]; $site_url3 = $_POST["site_url"]; $banner_url3 = $_POST["banner_url"]; mysql_query("UPDATE affiliates WHERE id = $aid(site_name,description,site_url,banner_url) values('$affiliate_name3', '$description3', '$site_url3', '$banner_url3')"); echo "<td align='center' class='content'>The affiliate has been updated.</td>"; } elseif(empty ($_POST['Edit Affiliate'])) { echo "<table align='center' cellspacing='1' cellpadding='1' border='0' width='50%'> <tr> <form action='admin.php?act=edit' method='POST'> <td align='center' colspan='2'>Edit $affiliate_name2</td> </tr><tr> <td align='right'>Site Name:</td> <td align='left'><input type='text' name='site_name' value='$affiliate_name2' size='30'></td> </tr><tr> <td align='right'>Description:</td> <td align='left'><input type='text' name='description' value='$description2' size='30'></td> </tr><tr> <td align='right'>Site URL:<br><small>Must begin with http://</small></td> <td align='left'><input type='text' name='site_url' value='$site_url2' size='30'></td> </tr><tr> <td align='right'>Banner URL:<br><small>Must begin with http://</small></td> <td align='left'><input type='text' name='banner_url' value='$banner_url2' size='30'></td> </tr><tr> <td align='center' colspan='2'><input type='submit' name='Edit Affiliate'></td> </tr> </table></form>"; } } } ?>
-
Ok, I'm making an affiliates management program and I want to give the user the option to display their banners either vertically or horizontally. Would it be possible to have an if statement inside of a string? Would something like this work?
$display = if($banner_display == horizontal)... { echo " "; }
The ... means that there's more but don't feel like writing it. Would that work?
-
Hmm... not sure what you mean by faux column method. Please explain.
-
Ok, I want my navigation bar on the left side to be touching the bottom of my page at all times, no matter what content is on the page next to it. My site consists of dynamic php database content and I have design tutorials that are very long and when I view them, the navbar doesn't touch the bottom of the page. How would I do this? This is my source:
<HTML> <head> <title>//M-Tech Development</title> <link rel=stylesheet href="styles/default/mtech.css"> </head> <body> <div align="center" class="logostrip" height="120"><img src="styles/default/images/logo.gif"></div> <div align="center" class="midbar" width="800" id="headlinks"><a href="index.php">INDEX</a> | <a href="support.php">SUPPORT</a> | <a href="forums/index.php">FORUMS</a> | <a href="staff.php">STAFF</a></div><div id="middle"> <div align="left" id="nav"> <div class="navtop">Main Navigation</div> <div class="navlink"><a href="index.php">Index</a></div> <div class="navlink"><a href="news/news.php">News Archives</a></div> <div class="navlink"><a href="support.php">Tech Support</a></div> <div class="navlink"><a href="feedback.php">Site Feedback</a></div> <div class="navtop">Tutorials</div> <div class='navlink'><a href='viewprogram.php?id=1'>Adobe PhotoShop</a></div><div class='navlink'><a href='viewprogram.php?id=2'>The GIMP</a></div><div class='navlink'><a href='viewprogram.php?id=3'>PHP Programming</a></div><div class='navlink'><a href='viewprogram.php?id=4'>Cascading Style Shee</a></div><div class='navlink'><a href='viewprogram.php?id=5'>HTML Coding</a></div> <div class="navtop">Services</div> <div class="navlink"><a href="hosting.php">Hosting</a></div> <div class="navlink"><a href="services/sitedesign.php">Site Design</a></div> <div class="navlink"><a href="services/installation.php">Script Installation</a></div> <div class="navtop">Downloads</div> <div class="navlink"><a href="downloads.php">Free Scripts</a></div> <div class="navlink"><a href="downloads/templates.php">Site Templates</a></div> <div class="navlink"><a href="downloads/resources.php">Program Resources</a></div> <div class="navtop">Community</div> <div class="navlink"><a href="irc.php">IRC Chat</a></div> <div class="navlink"><a href="forums/index.php">Discussion Forums</a></div> <div class="navlink"><a href="services/installation.php">Script Installation</a></div> <div class="navlink"><a href="affiliates.php">Affiliates</a></div> <div class='navlink' id='navbottom'><a href='http://i3.photobucket.com/albums/y82/Daredeviles/Buttons/sglogo.jpg'><img src='http://i3.photobucket.com/albums/y82/Daredeviles/Buttons/sglogo.jpg' width='88' height='31' alt='Storm Gaming'></a><a href='http://www.dev.mtechdev.com/portal/styles/default/images/bbu.gif'><img src='http://www.dev.mtechdev.com/portal/styles/default/images/bbu.gif' width='88' height='31' alt='bbUltimate.com'></a></div></div><div align='center' id='news' class='content'><p align='left'><b>Site Under Construction</b></p><br> <p align='left'>The site is still under construction. If you are navigating through the site, don't expect all areas to be complete.</p><br> <p align='right'><b>Posted by Unholy Prayer on 2007-01-21.</b></div><div align='right' id='members'> <div class='navtop'>Members Area</div> <div class='navlink'><a href='login.php'>Login</a></div> <div class='navlink'><a href='logout.php'>Logout</a></div> <div class='navlink'><a href='login.php?act=password'>Lost Password</a></div> <div class='navlink'><a href='faqs.php'>Why Register?</a></div> <div id='poll'> <div class='navtop'>Weekly Poll</div> <div class='navlink'><p align='center'>Do you like our site template?</p><br> <input type='radio'>Yes<br><br><input type='radio'>No<br><br><input type='submit' value='Vote Now'></div> <div class='navtop'>Advertisement</div> <div class='navlink'><script type="text/javascript"><!-- google_ad_client = "pub-1347884490353570"; google_ad_width = 120; google_ad_height = 240; google_ad_format = "120x240_as"; google_ad_type = "text_image"; //2007-02-12: mtech_website google_ad_channel = "7842738859"; //--></script> <script type="text/javascript" src="http://pagead2.googlesyndication.com/pagead/show_ads.js"> </script> </div></div> </div></div></div><div align="center" id="copyright" class="midbar"> ©2006-2007 //M-Tech Development, All Rights Reserved. </div>
and this is my css:
body { background-color: #fff6f6; font-family: arial; font-size: 11px; color: #b30e0e; margin-top: 0px; margin-bottom: 0px; margin-left: 0px; margin-right: 0px; } #nav { float:left; width: 170px; margin-right :10px; padding: 0 10 0 0; margin-bottom: 0px; } #members { float:right; float: top; width: 170px; margin-left :10px; padding: 0 0 0 0; align: top; } #headlinks{ float:left; width: 100%; margin: 0px; padding: 0 0 0 0; height: 18px; vertical-align: center; } #tutorial{ float: right; width: 50%; margin: 0px; vertical-align: center; } #copyright{ float:left; width: 100%; margin: 0px; padding: 0 0 0 0; height: 18px; vertical-align: center; } #news{ float:center; width: 75%; padding: 10 0 10 10; align: center; } .midbar { background-image: url(images/c_top.gif); text-align: center; font-family: arial; font-size: 10px; color: #FFFFFF; vertical-align: middle; } .midbar a:link, .midbar a:active, .midbar a:visited { color: #FFFFFF; text-decoration: none; } .midbar a:hover { color: #FFFFFF; text-decoration: underline; } .navtop { background-color: #fdd9d9; font-family: arial; font-size: 10px; text-align: center; padding: 3px; vertical-align: middle; width: 150px; border-right: 1px solid #ea989a; border-left: 1px solid #ea989a; margin: 0px; } .navlink { background-color: #ffecec; text-align: right; color: #ea9898; font-family: arial; font-size: 10px; padding: 3px; vertical-align: middle; width: 150px; border-bottom: 1px solid #ea9898; border-right: 1px solid #ea989a; border-left: 1px solid #ea989a; margin-bottom: 0px; margin-top: 0px; } .navlink a:link, .navlink a:active, .navlink a:visited { color: #ea9898; text-decoration: none; } .navlink a:hover { color: #ea9898; text-decoration: none; } .table { background-color: #b30e0e; border: 1px solid #b30e0e; } .content { background-color: #ffecec; text-align: left; color: #ea9898; font-family: arial; font-size: 10px; padding: 3px; vertical-align: middle; border: 1px solid #ea9898; } .content a:link, .content a:hover, .content a:visited, .content a:active { color: #b30e0e; text-decoration: none; } .sidebar { background-image: url(images/s_bar.gif); height: 100%; width: 15px; margin: 0px 0px 0px 0px; } .logostrip { background-image: url(images/logobg.gif); text-align: center; } .inforow { background-color: #fff6f6; text-align: left; padding: 4px; color: #ea9898; } .inforow a:link, .inforow a:hover, .inforow a:visited, .inforow a:active { color: #ea9898; text-decoration: none; } .newshead { text-align: left; color: #ea9898; font-weight: bold; font-family: arial; font-size: 10px; } .newsmessage { text-align: left; color: #ea9898; font-weight: normal; font-family: arial; font-size: 10px; } .newsinfo { text-align: right; color: #ea9898; font-weight: bold; font-family: arial; font-size: 10px; .navbottom{ background-color: #ffecec; text-align: center; color: #ea9898; font-family: arial; font-size: 10px; vertical-align: middle; width: 150px; border-bottom: 1px solid #ea9898; border-right: 1px solid #ea989a; margin-bottom: 0px; margin-top 0px; height: 100%; } .navbottom a:link, .navbottom a:active, .navbottom a:visited { color: #ea9898; text-decoration: none; }
Thanks in advance.
Count Row From Two Tables...
in PHP Coding Help
Posted
Ok, I'm making a forum software for my website and I want to put the number of replies for the listed forum. How would I count the number of rows of the replies table that are in the certain forum?