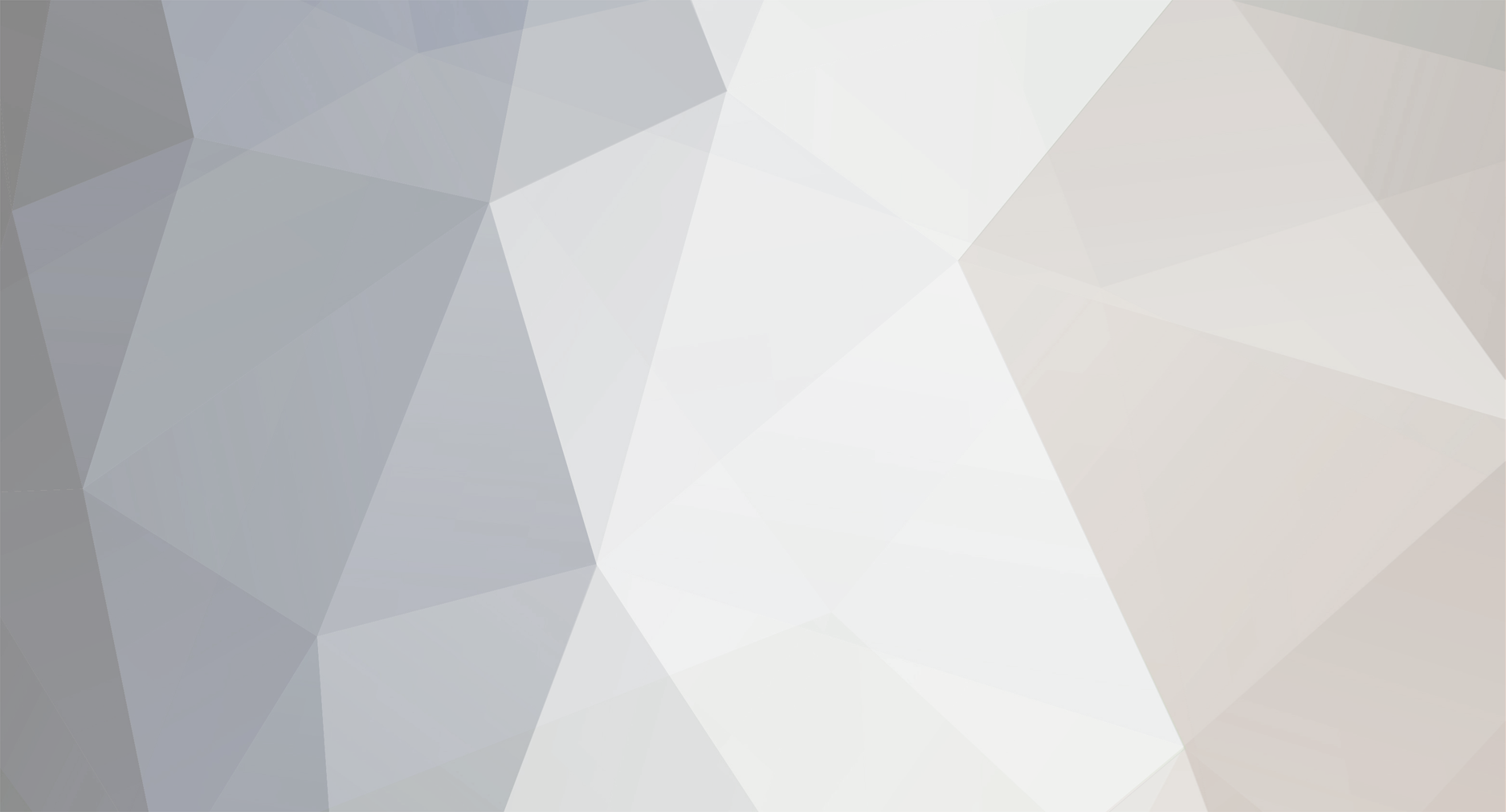
Jenk
-
Posts
778 -
Joined
-
Last visited
Never
Posts posted by Jenk
-
-
the Zend (PHP) engine does it for you.
-
The double colon is a static operator, you cannot use it to call an instantiated object, it will call the method of the class as if it were just a function.
Learn to pass the objects around, in true to OOP.
<?php $mysql = new SQL; $B = new B($mysql); // pass the $mysql object to $B so it can store it within it's properties and utilise it. ?>
-
That's a code smell. You should not rely on this.You can output headers where ever you like if you use ob_start and ob_end_flush.
I used to reserve these for building my cms's as not so many would be in the cms for long periods so I thought it would not matter about server load and runtime etc. I read an article recently that suggest that if you have lots of output statements all over (echo, print_r etc.) using the output buffer can actually reduce the processing time of the script significantly. I did a little benchmarking and my results agreed - even on a fairly small script that didn't do too much...
-
PHP5 will give warnings with different things like static class functions etc. and it is hassle to switch over so that suggestion isn't so good.
However, you could probably download the source, find the file with the version number in it, edit it and compile it.
error_reporting(E_ALL ^ E_STRICT);
problem solved.
-
Such as what? Like I said, PHP5 is backwards compatible with PHP4.
-
er.. no.
I work with auditing in my current role, and you'd get a MAJOR grilling if I were to discover one of my teams was fradulently identifying tools.
You're client/customer wouldn't be pleased either.
PHP4 code will work in PHP5 anyway.
-
<?php while (($file = readdir($dirhandle)) !== false) { if (!in_array($file, array('.', '..')) { // do stuff. } } ?>
-
Zend is very good, but can be overwhelming to anyone not familiar with MVC, Design Patterns and OO in general.
My own framework is merely an adaptation from Zend so that I can be sure to keep consistency, as Zend is not final yet. -
I wonder what the answer to that is, then?with the if method exists code it would be stupid
That is a flaw in your design. Observers never - ever - re-run constructors. I can't think of any objects that ever do re-run constructors. The only instances where a constructor is called explicity is when an extended class calls it's parent/superior classes constructor.5. __construct() you dont seem to understand making the new object runs the constructor, then you add the observers and then you once again would have to run the constructor.
Bias? No. A Practice learnt through experience? Yes. Documented (via books/lecture papers) good practice by people such as Martin Fowler, "Gang of Four", etc.? Yes. The references I make to "interface" is not that of "you must use the keyword 'implements'" it is referencing that you should use a common name for your observer method(s) throughout all objects that share that functionality. Let's take a different look at it. Java's "toString()" method, existing on all objects. As a developer, I can be assured that which ever object I need to get the string value from, it will always be the toString method I need to call. Your example, I will need to look up some documentation (which may not exist anymore) or open the class and have a look. I can't just call the toString() function, because I cannot be assured it will exist. The same applies to the method for observers. If I want to notify my observers of a new state, I can be assured that they will all have the "notify()" method. In your example, I would need to first check they do/don't have "index()" and then if they don't, I'll see if they have the method with the same name as the state change method. If they don't have that, they'll never know of the state change.They are a different approach, your idea that no interface is wrong is bias.You are also still ignoring the issue of including multiple behaviours into one object - which is bad practice, period - and also that you have abstracted the observable - not the observer. RE: GTK I couldn't give a monkeys if you are using this for the online bible; it is bad practice to include multiple behaviours into objects. Extensions are used to remove duplication of code; by storing said code into one class that can be utilised by many others that will require this code, but the code on it's own will not be a complete object. What you originally posted was complete code, it will function fully as an observable (observer manager) object. It does not need an "init" function within the same object, it does not need any other functionality, so it does not need to be extended to make use of it. "class Foo extends Observable { public function init() {} }" would be a valid, fully functional object with what you have posted - this is why it is unecessary to make it abstract, and also unecessary to specifiy it must have an init() method, when init() is never called by any of your abstract class methods, and so none of them are dependant on it.
-
Well, you're screwed if your object class needs to extend another class, yet you need to include observer functionality. You're breaking the entire purpose of an observer by tightly coupling the object/class, and you don't seem to understand interfaces at all (i.e. if it's a particular object, it should have the same interface as all other objects with the same behaviour.)
And to address your points:
1. ok.
2. Yes they are. They will be blob/god objects because they cater for more behavioural functionality than is needed.
3. You're sounding like businessman, the same person you instructed to take advice from others.. Having non-uniform states and interfaces for multiple objects will be a big mess. Documentation is supposed to accompany and hint, not completely dictate and explain.
4. __construct() ..
-
It's an abstract method, it *has* to be implemented else you cannot extend the abstract class.Also another contradiction you have is the abstract method init(). You say you don't want to force an "index" method on your object that extend Observable but at the same time, you are forcing them to implement an init() method..
I just wanted to clarify that implementing a method in an abstract class does not force a child class to implement the afore mentioned method. If the child class does not override the parent's method the child class will just inherit the abstract classes method. It is sometimes the case that you don't want children to override certain behaviors of the parent hence the use of the `final` keyword.
Interfaces on the other hand will force any classes which implement them to actually provide their own implementations of the methods set forth by the interface.
I think this was just misspoken by jenk "them to implement an init() method" => "them to inherit an init() method".
Best,
Patrick
-
change it to:
<?php echo shell_exec('tar cvf images.tar /path/to/images/ && gzip images.tar'); ?>
to see errors.
-
<?php shell_exec('tar cvf images.tar /path/to/images/dir/ && gzip images.tar'); ?>
-
Also another contradiction you have is the abstract method init(). You say you don't want to force an "index" method on your object that extend Observable but at the same time, you are forcing them to implement an init() method..
You also seem to be confusing the Observer with the Observable. The Observable (which I prefer to call the Observer Manager) is the one that other objects tell it "Hey, I've changed something. This is my message: something!" which then goes and tells all the registered Observers "something."
The observer is then responsible for reacting to the change.
Here is an example of an observer; my previous example was the Observable (Observer Manager)
<?php class Foo implements Observer { private $_message; public function __construct ($message) { $this->_message = $message; } public function notify ($state) { if ($state == 'BAR') { $this->printMessage(); } } public function printMessage () { echo $this->_message; } } ?>
Here is a class that Foo is Observing:
<?php class Bar { private $_foobar; public function __construct () { $this->_foobar = 'FOOBAR!'; } } ?>
And here we have the context:
<?php $observerManager = new ObserverManager(); $observerManager->registerObserver(new Foo('FooBar!')); $bar = new Bar(); $observerManager->changeState('BAR'); ?>
The output will of course be:
FooBar!
From that context, do you now see why they observer manager does not need to be (and should not be) abstract?
-
First bit:about your first point, my book shelf says otherwise. And if there is an instance where I cant or dont want to extend it, it will be very easy to make an exception where needed. All the books and documentation I have seen show an extended version. The classes I intend to use it on will be designed in a way they need the extra weight. because my adding the extra weight it allows me to just move code to the observers instead of the main class, I could use abstraction but there are a few things I am thinking about that would be quite better with the observable class.
About the second point you are wrong because I don't want the default index method to be forced, I want it to be optional an interface would make this required. if I load an object with a method called something if I set the state to the name of the method to the name of the state the method will be executed, this behavior is dynamic and cannot be interfaced.
That's contradicting your other posts - you "could" abstract it? You are abstracting it. Infact you are forcing it to be abstract. Extensions are not optional. A class either extends another class, or it does not. There is no calculated decision. (This is of course refering to run-time, not you typing out the definitions.) I also fail to see why you have forced abstraction, because what else would this behaviour need? Everything is there!
If you're books are suggesting to include multiple behaviours within a single object, I suggest you burn those books. That is bad OO practice, period. It's known as "Blob classes" or "God classes."
Second part:
That's the fundamentals of interface design. Objects that are observable should implement the same method for notification. Period. You will end up with a huge mess of "ok, which method is used for notification now?"
-
So that you can enforce type hinting of your objects, and share that type hinting across many objects.
If objectA implements interfaceA, you can be sure that objectA will have those methods and properties specified in interfaceA.
-
that line ($this->etc.) is completely out of place.
-
htmlspecialchars() or htmlentities()
-
Possibly. Without the code on our screen we couldn't say the exact cause.
-
The aim of the observer is to loosely couple objects, not directly tie them. Extending breaks the first rule of this - it is class dependant, not object/interface dependant. It is also a code smell, because you will have multiple objects with two (or more..) behaviours. Objects should be concise. Let's say you extend foo with Observable. You want foo, but don't need the observer everytime - yet because of the extension, you are stuck with it. That's extra weight which you don't need.I'm also not quite sure why you are using it as abstract.. the observerable (observer manager) should be a standalone object in it's own right.
I always thought it was meant to be abstract?? but that said I will continue to use it as abstract because I plan on using this class in multiple objects an it is just good to do for a code reuse and consistency point of view.
No, it doesn't. If you register an object for observing, but it doesn't have a $state or $default method, you'll never know.if my application is trying to force an observer upon an non-observable object, I would like to know about it.
yeah but the way I'm using method exists rules that out.
-
Similar, but I opt for interfacing over method_exists() - if my application is trying to force an observer upon an non-observable object, I would like to know about it.
I'm also not quite sure why you are using it as abstract.. the observerable (observer manager) should be a standalone object in it's own right.
A chopped down verison:
<?php interface Observer { public function notify($state); // what the object does with the state is up to the object. } final class ObserverManager { private $_observers = array(); private $_state; public function registerObserver (Observer $ob) { $this->_observers[] = $ob; } public function changeState ($state = null) { $this->_state = $state; $this->observe(); } public function observe(); { foreach ($this->_observers as $ob) { $ob->notify($this->_state); } } } ?>
-
Being pedantic, but it means outside of an object.well that means your using $this outside of a class. make sure that your inside of a class.
<?php class Foo { public static fooBar () { $this->foobaroo = 1; } } Foo::fooBar(); // fatal error: $this outside of object context. ?>
-
Gentlemen, please refrain from foul language. Firstly because it is against the rules, but secondly because it can be difficult to grasp the context it is used within. Text does not carry body language, nor tone of voice, so please be careful.
-
I'd go easy on the extenstions.
I personally dislike the way terminology has adapted the whole parent/child thing for classes and their extensions as it can confuse things. It's like giving someone a prosthetic limb and calling it their child limb.. just not right.
Anyway, back on topic:
Yes, a car would (could) be broken down to several object instead of one big one, that is also what I would do.
What I would do instead of extending car with engine, I'd use the engine object as a member object of car, something like (ignoring class definitions for now..):
<?php $car = new Car; $engine = new Engine; $car->installEngine($engine); // assign $engine to car. ?>
This way, you can allow for modifications of your Car, providing the components (engine, wipers, seats, etc.) all have the same interface (the publically accessible methods and properties of the object)
So, let's have a look at the Car class:
<?php class Car { private $_engine; public function installEngine ($eng) { $this->_engine = $eng; } public function driveTo ($destination) { if (!$this->_engine->isRunning) $this->_engine->start(); // gentlemen, start your engines! /** * calculations for how to move the car to $destination goes here.. * this could also use a $driver, if the car is a limosuine or bus or whatever.. * the possibilites with OO are collosall... */ } } ?>
So we can see from there, that providing the the parameter passed to the method installEngine has a method start() and a property isRunning, the Car object does not need (nor care) what type of engine it has installed.
This is what OO is about, modularity.
\n does not work?
in PHP Coding Help
Posted