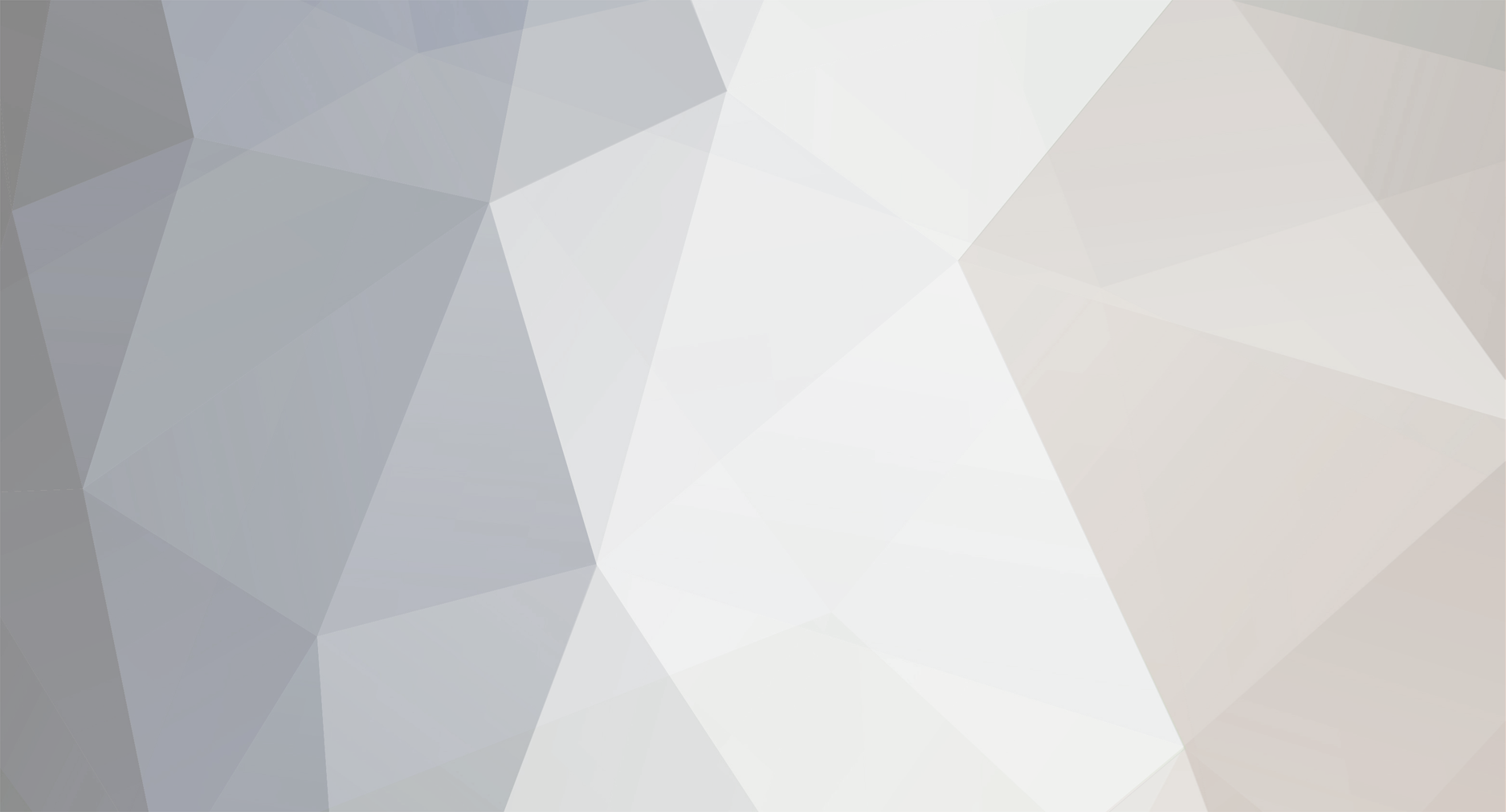
kid_drew
Members-
Posts
65 -
Joined
-
Last visited
Never
Everything posted by kid_drew
-
Also, and not to be picky, but your insert command should reference the array members with quotes. Otherwise, it treats the string as a constant and has to figure out that it isn't, which is an extra step and will slow down the server if it has a lot of requests. To illustrate: Use this: VALUES ('{$_POST['fname']}' , '{$_POST['age']}' , '{$_POST['color']}');"; Instead of: VALUES ('$_POST[fname]' , '$_POST[age]' , '$_POST[color]');"; [quote author=a2bardeals link=topic=116755.msg475889#msg475889 date=1164835509] ok so i am playing around with a simple script. a form with a post action sending to a page with the sql insert code: my form is simple: [code]<form action="do_register.php" method="POST"> Name: <input type="text" name="fname" size="20"> Age: <select name="age"> <option value="1">1</option> <option value="2">2</option> <option value="3">3</option> <option value="4">4</option> <option value="5">5</option> <option value="6">6</option> </select> Favorite Color: <select name="color"> <option value="red">Red</option> <option value="green">Green</option> <option value="blue">Blue</option> <option value="orange">Orange</option> <option value="purple">Purple</option> <option value="pink">Pink</option> </select> <input type="SUBMIT" value="GO"> </form>[/code] this sends the data just fine. it goes to "do_register.php" then i insert the data: [code] <? $con = mysql_connect("localhost", "user", "pass"); mysql_select_db("database", $con); $sql = "INSERT INTO 'table' (`fname`, `age` , `color`) VALUES ('$_POST[fname]' , '$_POST[age]' , '$_POST[color]');"; if (!mysql_query($sql,$con)) { die('Error: ' . mysql_error()); } echo 'Thanks, '; echo $fname; echo '<br>Your info has been submitted!'; ?> [/code] it all seems fine after you post but when i call the results there is one row with the data and another blank row inserted after it. The ID row which is the index is set to auto_increment and it assignes two rows (eg. one submission = one row id:23 with the data and one row id:24 with no data.) Any ideas? [/quote]
-
You can do this in a couple of ways. I would probably issue a select command "select * from blog_entries where..." and then loop through the entries in php: $query = "select * from blog_entries where..."; $result = mysql_query($query) or die(mysql_error()); while($row = mysql_fetch_assoc($result)){ //html format code here } Anyone have a more eloquent solution?
-
use the "ON DUPLICATE UPDATE" command. INSERT INTO unc_members (userid, unc_group) VALUES ($id, $group) ON DUPLICATE UPDATE set unc_group = '$group'
-
Hey guys, I want to write a script to automatically send an e-mail to all my community members and perform a site/db cleanup once a day, and I can't think of an elegant way to do it in php. Anyone have any ideas? Maybe in another scripting language? -Drew
-
This probably isn't a php question, but I thought I'd throw it out there. I have an ad that I want to post in multiple cities on Craigslist. Instead of going to each city's page and typing in the exact same ad, what I want to do is create a script that will load up many windows or tabs with the form for a different city in each window, auto fill the form, and have it waiting at the verification page for me to type in the word to submit the ad. Anyone have any idea how to do that? perl maybe?
-
Sorry, I guess that wasn't clear. I want a class that I can plug into an existing website that will parse text and pick out the tags between colons and replace with smilies. I.e., if it finds :grin: or something similar, it will replace with ;D just like this board does. I'd like to just have a list of tags and replacement images stored in my db. Or if anyone has a good tutorial for how to create a smilie engine quickly, I'd take that too. [quote author=tomfmason link=topic=110917.msg449076#msg449076 date=1160366826] I am not realy sure what you mean by smiley engine? Please explain what it is and what it does [/quote]
-
Anyone know where I can find a free smiley engine to use on a website? I could write one, but I bet there's already one out there.
-
Ok, so let's skip the obvious question of "why the hell would you ever want to do this" and just understand that this is the situation that I'm in. I am attempting to write a php script (because that's what I know) for a friend's project which is created with DotNetNuke, an ASP.NET environment. I have created a PHP session and everything seems to work correctly unless the user logs into DotNetNuke, at which point the PHP session gets blown away. Anyone know why an ASP.NET login would kill a PHP session? Again, I know the setup is ridiculous and I should never be writing PHP scripts for an ASP environment. Don't flame me... ;D
-
Getting lat and long is not hard. There are plenty of free geocoders out there. Google Map's API will do it.
-
Ok, so I'm a veteran programmer beginning to venture into the world of PHP. I'm looking for an idea of how people go about structuring their files in large projects. I've heard of people keeping their PHP and HTML code separate, and I really don't understand how that is done seeing as how so much is done inline. Does everyone just use smarty templates? What about file structure? Does everyone have a typical header/body/footer structure where they include/require header and footer files in every page and fill in the content in between?
-
I'm not sure how long the longer queries are going to take. I don't have any traffic yet. =) I think they have the potential to take 10-20 seconds.
-
What about the fork() function? Could you fork to run the query process in the background, run a do/while loop on your wait screen and redirect only when the forked process updates a session variable, or something similar? I've never used fork, so I'm totally shooting in the dark.
-
Does anyone know of a good way to implement a wait screen while some large query is being computed? I can create a wait screen with a nice animated gif and all that, but I'm not sure how to do the timing. I.e., how do you immediately go to another page after a form submit and wait at that screen until the data is ready then load the results page, or is that even possible in php?
-
Ok, so this isn't really a PHP question, but I figured someone on this site would have some ideas for me. I'm trying to calculate the distance between two user-input physical addresses, and I can't find an API that will do it. I want to actually be able to return the distance values to hold in an array so I can manipulate them. Google Maps will do the geocoding, but no routing, and I can't see that Yahoo does either. Mapquest has a routing function in their API, but the function doesn't actually return any data, it simply adds the points and lines to an existing map image and dumps the data to the screen. Besides, Mapquest's API sucks. Any ideas?
-
Hey guys, I'm having a problem with a left join statement. When I query the database from the phpmyadmin console, it works fine, but I'm having problems with the mysql_query call in php. The query is: "select * from users left join images on users.username = images.username AND images.list_order = 0" This is supposed to grab all users whether they have images or not, but if they do, the images fields should be populated instead of NULL. if the user has an image listed in the "images" table, everything works fine. The problem is that if the user does not have an image listed in table "images", then the username field returns NULL. Again, the query looks like it works perfectly if I do it from the phpmyadmin console, but not in PHP. Any ideas?