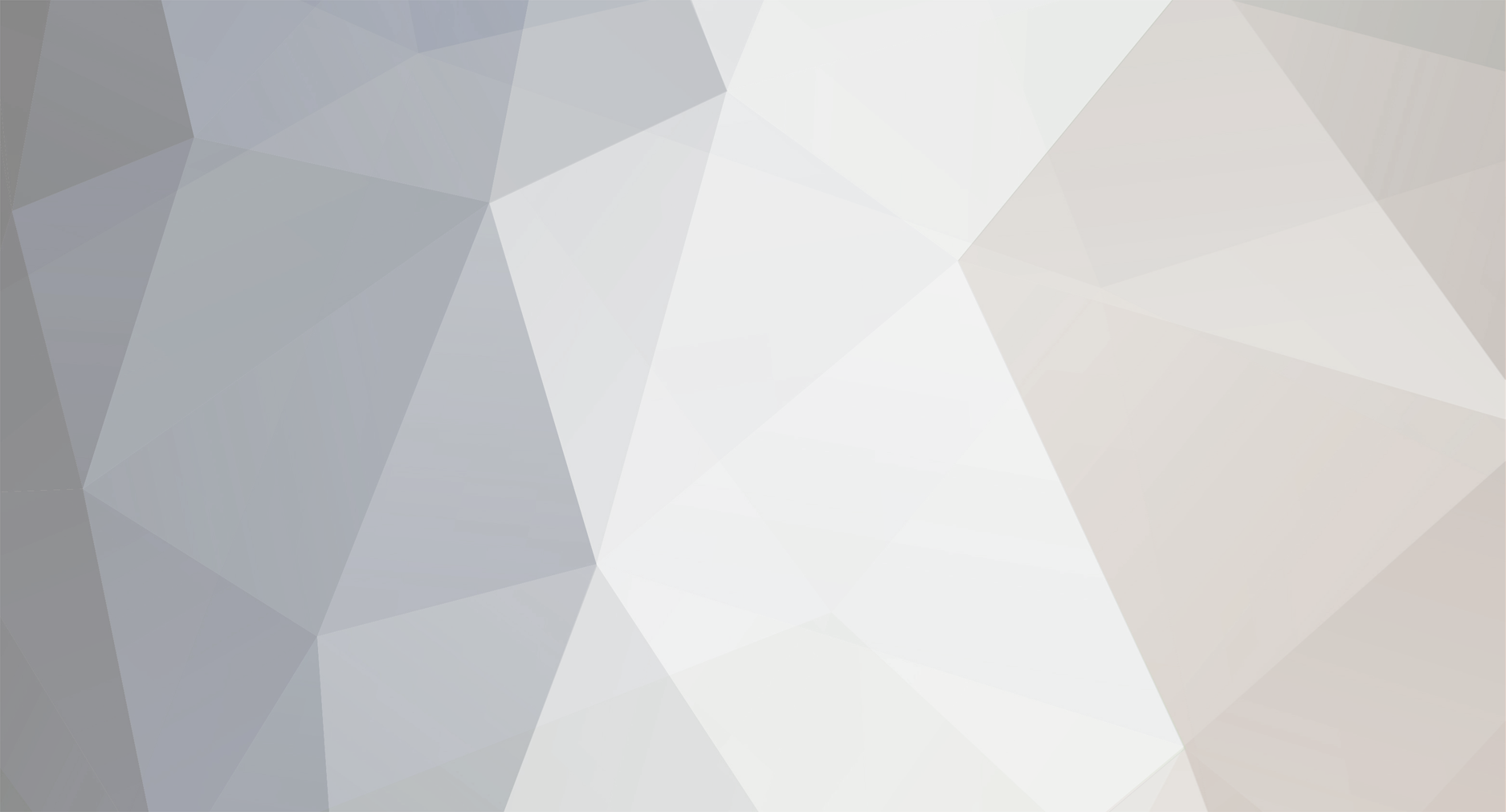
pkSML
-
Posts
191 -
Joined
-
Last visited
Posts posted by pkSML
-
-
Many results here: http://pksml.net/search/PHP+file+upload+tutorial
-
Yah, your quandry is confusing...
You can access form variables submitted by the GET method as
$_GET['name'] and $_GET['last']
-OR-
$_REQUEST['name'] and $_REQUEST['last'] (this method gets variables submitted with either GET or POST)
-
Any more information about these dates? Are they in purely random order regarding past/future dates?
-
More rather, you want to retrieve every line that does not begin with the pound sign.
so...
Read the file into an array - the file() function
Make a foreach loop that reads the array
(Put a variable in the foreach loop that will keep track of the track number)
Put a conditional if statement that asks if the line begins with #
If not, display the track number variable, increment it, and use pathinfo() to extract the filename for display
Make sense?
-
Glad you got it working. When working with more complex data (ie binary data or non-standard characters), serialize the array and make it a hidden form element. Then unserialize it and you'll have the original array in its perfect form.
PS You may want to base64 the serialized data to make sure nothing is lost in the POST operation.
-
Can you use an absolute path to the images? If you're using relative URLs, that might make things get hairy.
You could also use absolute paths relative to your docroot. Call the image like so: <img src="/images/whatever.png">
This will look in docroot/images/ for the file. Make sense?
-
You would need to know the exact context of the data you want. You could use preg_match to grab data between <table> and </table>.
But what if there is more than one table in the page? Then you'd need preg_match_all() and know which table to grab. PHP is only as smart as you tell it to be.
Also, is there a specific format that this data is you're trying to retrieve? That might make things easier.
Is this data dynamic? Does it have a specific scheme? ...
Any more information will help us help you.
-
Just have a file named settings.php.
Make the file like so:
<?php $title = "The Coolest Website Ever"; // The name of your site $other_variable = "true"; ... ?>
And then include settings.php before any HTML is produced in the main script.
-
To do this the easy way, have your background available on the web. Then just create a simple HTML email.
For example:
<?php $from = "me@myhost.com"; $to = "you@yourhost.com"; $subject = "Hello!"; $headers = "From: {$from}\r\n"; $headers .= "MIME-Version: 1.0\r\n"; $headers .= "Content-Type: text/html; charset=ISO-8859-1\r\n\r\n"; $message = '<HTML> <HEAD> <TITLE></TITLE> </HEAD> <BODY background="http://us.i1.yimg.com/us.yimg.com/i/us/sch/gr/bggreendiag.gif"> message goes here <img height=1 width=1 src="http://pksml.net/blank.gif"> </BODY> </HTML>'; //send message if (mail("$to", "$subject", "$message", "$headers")) {echo "Mail sent!";} else {echo "Mail NOT sent!";} ?>
Notice the background in the body tag of the HTML message.
To show pictures, I found it necessary to place one in the body of the HTML, so I just put a blank transparent picture there.
-
You could use PHP Mailer. It allows you to include attachments.
URL: http://sourceforge.net/project/showfiles.php?group_id=26031
-
if(ereg("^447[0-9]{9}$",$value)){ $value1 = substr($value, 3); $value1 = "07" . $value1; echo $value1; }
This will trim off the first three characters, which are guaranteed to be 447 because of your conditional statement.
May not be the most efficient way to do it, but, hey, it works!
-
Your PHP.ini file:
[Date] ; Defines the default timezone used by the date functions date.timezone =
In this directive, you need to list a timezone identifier, of which the list can be found here: http://us.php.net/manual/en/timezones.php
Also go into Windows and check your timezone. Double click the time at the bottom right of your screen in the system tray, click the timezone tab and set it properly.
-
Thank you,
I'm happy that you took time to anser my question.
You must be very dedicted to this forum if you are ansering replies on Thanksgiving
Staying at home this year. Between cleaning house and helping with dinner, there's plenty of time for PHP fun!
-
Script is much improved here --> http://code-bin.homedns.org/64
Same usage. Ex: image.php?I=1234567890.jpg
-
Yes I think that teh session will work for me.
Let me make sure my thought behind it is correct though.
At the top of my form to submit I will start a session and set some session variable to 'a value'
on the script processing page before processing script i check if $_SESSION[somevar] == 'a value'
Is that right? I am at work right now and i cannot tranfer files up to my server in order to test it out.
Also Sessions are new to me and I have only just read a couple of tutorials on them. Could anyone tell me how the server knows that the user has closed the browser. Or maybe a place were i can read up more on how sessions work I like to have a good understanding of things before i put them in my code.
At the top of the form page (before any output), you must have this PHP code:
<?php session_start(); $_SESSION['var'] = "your-form"; ?>
This type of session does not save data as a cookie, and is not a "persistent session". The session will automatically end when the user's browser is closed. By 'end', I mean the browser will not remember the session id any more.
In the form processing script, put this at the very beginning:
<?php if (!isset($_SESSION['var'])) {die("You didn't use my form!");} ?>
-
Secure it? Yes. This is easy, actually!
<?php $folder = "./uploads/"; // Must have a trailing slash $file = $_GET['I']; // Image number/name $path_parts = pathinfo($file); // This will strip these: ../ ./ $filename = "{$folder}{$path_parts[basename]}"; if (!file_exists($filename)) {die("File doesn't exist.");} else{ if ($path_parts[extension] == "jpg") {header('Content-type: image/jpeg'); // Output header} elseif ($path_parts[extension] == "png") {header('Content-type: image/png'); // Output header} elseif ($path_parts[extension] == "gif") {header('Content-type: image/gif'); // Output header} elseif ($path_parts[extension] == "jpeg") {header('Content-type: image/jpeg'); // Output header} header('Content-length: ' . filesize($filename)); readfile($filename); } ?>
You'll need to put the extension in the query string for this to work.
If you saved this script as image.php in your root WWW directory, here's how your HTML should look:
<img src="/image.php?I=000000004.jpg">
-
stephen (pksml) nice code.....
must also point out to all regular exsperesion users that preg_replace regex is the way foward becouse
from php 6 the eregi function is turned off and preg function is defualt be warned.......
why i tell you this becouse if u get a job in php your be confused why you can not use eregi in the future and
trust me getting a free host or hoster to change there php.ini is very hard....
this is only advise from me so learn preg regex oposed to eregi regex
if you need to learn regex use a program called http://www.regexbuddy.com/ very good....
Thanks for that bit of news (and the compliment:).
I use http://www.snapfiles.com/get/regexcoach.html for Regex help. You might give it a try. Best of all, it's freeware!
-
You can't reliably block the source from being viewed. The best option is to set a $_SESSION variable on your form page, then check for its existence on the process page. $_SERVER['HTTP_REFERER'] is unreliable at best as it can easily be spooked or not sent at all.
I never thought of that before. Sessions are a great idea! And a session-based captcha would be a further security.
Sessions *almost* guarantee your server originated the form that the user is submitting.
-
Glad to help. It helps you, me, and any other people who see it!
-
Found this at the PHP manual: (modified for you)
<?php function hyperlink ($string) { $string = preg_replace('#(^|\s)([a-z]+://([^\s\w/]?[\w/])*)#is', '\\1<a href="\\2">\\2</a>', $string); $string = preg_replace('#(^|\s)((www|ftp)\.([^\s\w/]?[\w/])*)#is', '\\1<a href="http://\\2">\\2</a>', $string); $string = preg_replace('#(^|\s)(([a-z0-9._%+-]+)@(([.-]?[a-z0-9])*))#is', '\\1<a href="mailto:\\2">\\2</a>', $string); return $string; } ?>
It seems to work for all examples I've tried.
PS I posted the code here --> http://code-bin.homedns.org/63 for permanent reference.
-
In Windows --> http://drupal.org/node/31506
More links: http://pksml.net/search/windows+cron+job
In Linux --> http://www.adminschoice.com/docs/crontab.htm
More links: http://pksml.net/search/setting+up+cron+job
-
You might see this script --> http://elouai.com/force-download.php
-
I'm speaking outside of my Windows-only world, so I don't know if this is a possibility for you.
Could you fork a PHP script that will start encoding immediately when a user uploads a file to you?
-
I should add the table structure...
- Table structure for table `tblhours` -- CREATE TABLE IF NOT EXISTS `tblhours` ( `ID` int(10) NOT NULL auto_increment, `technician` char(30) NOT NULL, `client` char(50) NOT NULL, `hours` float NOT NULL, `mileage` tinyint(10) NOT NULL, `billable` varchar(10) NOT NULL, `nobillable` varchar(10) NOT NULL, `remote_bench` varchar(10) NOT NULL, `servicedate` date default NULL, PRIMARY KEY (`ID`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=1 ;
We'll start with the basics and then this will be a lot easier.
For each yes/no entry in your database, use the boolean data type.
Change billable and remote_bench to BOOL NULL rather than varchar.
You shouldn't need the column "nobillable", right?
And when you're inputting all this data with your form, make billable and remote bench a checkbox. This will put the data in the db properly.
Edit: Is remote_bench supposed to be either 'remote' or 'bench'?
[SOLVED] Time zones?
in PHP Coding Help
Posted
You could store everything using unix time, which can be accessed by time();
We'll say the user is GMT +500 and the original time is held in $orig_time, so format your date like this: