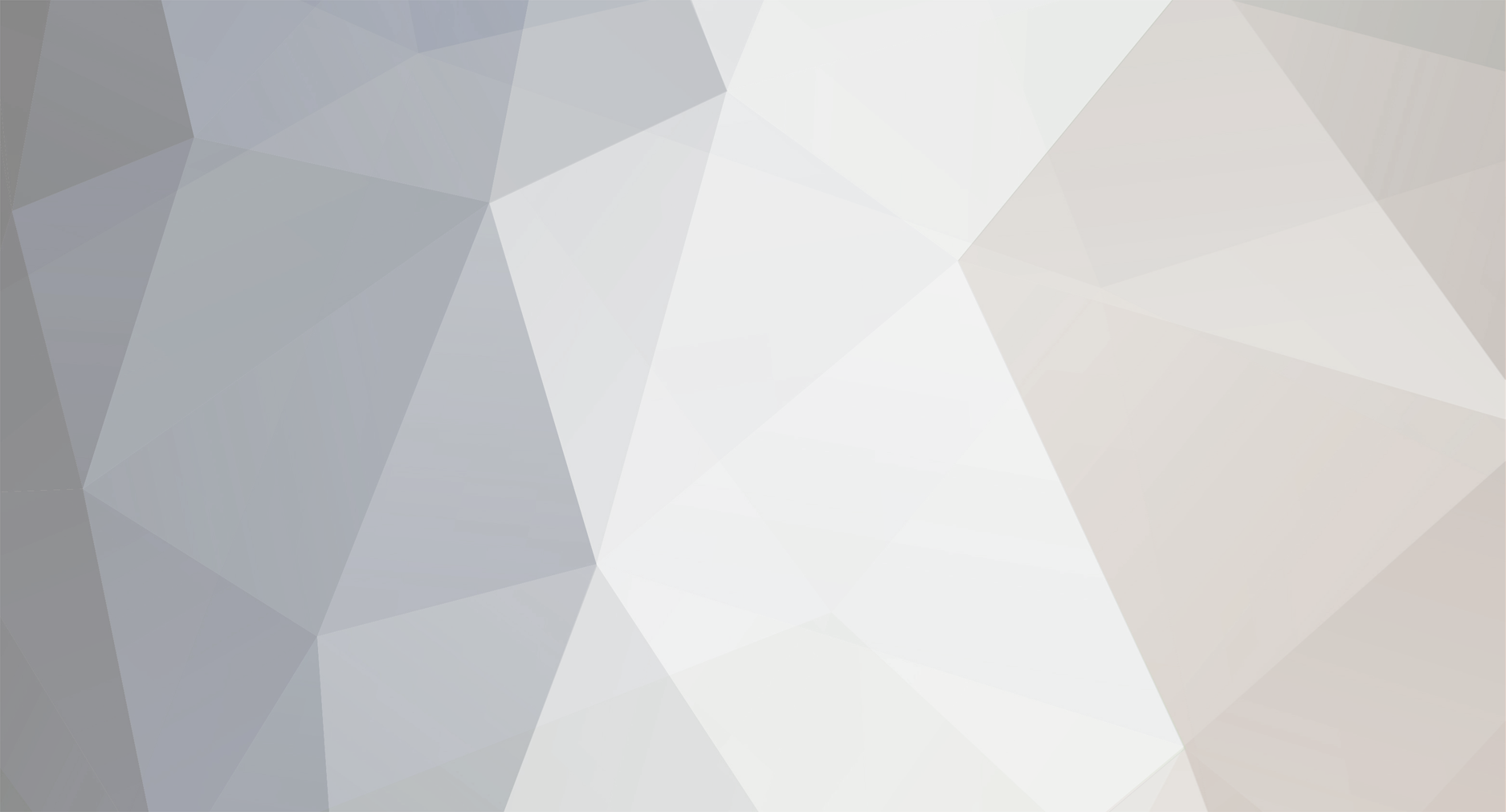
richardw
Members-
Posts
120 -
Joined
-
Last visited
Everything posted by richardw
-
[SOLVED] alternating color depending on results returned
richardw replied to adam291086's topic in PHP Coding Help
Here is one more example, not quite as simple but handles alternating colors in a checkerboard like grid: <?php $columns = 2; // define number of columns - use with a table or css grid // Connection string and query here $num_rows = mysql_num_rows($result); echo "<TABLE BORDER=\"1\" width=\"530\" cellpadding=\"3\" cellspacing=\"2\" bordercolor=\"#000066\">\n"; echo "<tr><td colspan=$columns bgcolor=\"#99CCFF\">"; echo "Put your Title contents here"; echo "</td></tr>"; // changed this to a for loop so we can use the number of rows $x = 0; for($i = 0; $i < $num_rows; $i++) { if ($x == 4) { $x = 1; } else { $x++ ; } if ($x == 1) { $bgcolor = "#F1F5F8"; } elseif ($x == 2) { $bgcolor = "#FFFFFF"; } elseif ($x == 3) { $bgcolor = "#FFFFFF"; } elseif ($x == 4) { $bgcolor = "#F1F5F8"; } else { $bgcolor = ($bgcolor == "#F1F5F8" ? "#FFFFFF" : "#F1F5F8"); } $row = mysql_fetch_array($result); if($i % $columns == 0) { //if there is no remainder, we want to start a new row echo "<TR>\n"; } echo "<TD valign=top bgcolor=$bgcolor width=50%>"; echo "Cell Contents Here"; echo "</TD>\n"; if(($i % $columns) == ($columns - 1) || ($i + 1) == $num_rows) { //if there is a remainder of 1, end the row echo "</TR>\n"; } } echo "</TABLE>\n"; } ?> I find that it helps in displaying results, especially if space is tight and a grid can meet the display requirements. Here is one example where I have used it: http://www.providenceri.com/BuyProvidence/buy_providence.php?category=all I hope this snippet can also be put to use -
As lemmin said, round at the end only. Remove your number_format from all but the variable set to display the results. Best
-
[SOLVED] alternating color depending on results returned
richardw replied to adam291086's topic in PHP Coding Help
Here's one more simple one line statement while ($row = mysql_fetch_array($results)) { $bgcolor = ($bgcolor == "F1F5F8" ? "FFFFFF" : "F1F5F8"); -
Thanks wildteen! It seemed like it would be sraight forward until I saw the code for the functions_weather.php page. Working in Gov't, I too may be able to utilize this solution. richard
-
Do you have server write access? I am thinking of guiding you on a temporary write scenario and then combining the output if can't get your metar code woring through an array.
-
I am now able to get the same error. I have to log out now, but if you are continuing tonight, try this tester as you can edit and process code in real time (simulated) just go to the URL below and enter a name to start the session, it will open up a page with an textarea and a display browser below. It needs the php tags to process the code, hit the "Exceute Code" button. It will return your code in the text area and the results below. It is a quick way to test as you don't have to save each time. http://www.mywebforyou.com/php4me/php_tester/php_start.php Please don't forget to keep the php tags in the textarea <?php ?> I built this tester for a begining class, but it can handle this script as long as it thinks it's one page. Use the code in the block below as it has the correct array for both stations. It may be that the results need to written to a temporary file, and the script reset for the 2nd station and then combined into one page for output. I had to comment out the include to test it as one page (I hope this helps and I will check back tomorrow) best and fly safely! <?php // **** FUNCTIONS **** function get_metar($station, &$wxInfo) { // This function retrieves METAR information for a given station from the // National Weather Service. It assumes that the station exists. // A slower URL is "ftp://weather.noaa.gov/data/observations/metar/stations/$station.TXT" $fileName = "http://weather.noaa.gov/pub/data/observations/metar/stations/$station.TXT"; $metar = ''; $fileData = @file($fileName); // or die('Data not available'); if ($fileData != false) { list($i, $date) = each($fileData); $utc = strtotime(trim($date)); set_time_data($utc,$wxInfo); while (list($i, $line) = each($fileData)) { $metar .= ' ' . trim($line); } $metar = trim(str_replace(' ', ' ', $metar)); } return $metar; } function set_time_data($utc, &$wxInfo) { // This function formats observation time in the local time zone of server, the // current local time on server, and time difference since observation. $utc is a // UNIX timestamp for Universal Coordinated Time (Greenwich Mean Time or Zulu Time). $timeZoneOffset = date('Z'); $local = $utc + $timeZoneOffset; $wxInfo['OBSERVED'] = date('D M j, H:i T',$local); $now = time(); $wxInfo['NOW'] = date('D M j, H:i T',$now); $timeDiff = floor(($now - $local) / 60); if ($timeDiff < 91) $wxInfo['AGE'] = "$timeDiff min ago"; else { $min = $timeDiff % 60; if ($min < 10) $min = '0' . $min; $wxInfo['AGE'] = floor($timeDiff / 60) . ":$min hr ago"; } } function process_metar($metar, &$wxInfo) { // This function directs the examination of each group of the METAR. The problem // with a METAR is that not all the groups have to be there. Some groups could be // missing. Fortunately, the groups must be in a specific order. (This function // also assumes that a METAR is well-formed, that is, no typographical mistakes.) // This function uses a function variable to organize the sequence in which to // decode each group. Each function checks to see if it can decode the current // METAR part. If not, then the group pointer is advanced for the next function // to try. If yes, the function decodes that part of the METAR and advances the // METAR pointer and group pointer. (If the function can be called again to // decode similar information, then the group pointer does not get advanced.) if ($metar != '') { $metarParts = explode(' ',$metar); $groupName = array('get_station','get_time','get_station_type','get_wind','get_var_wind','get_visibility','get_runway','get_conditions','get_cloud_cover','get_temperature','get_altimeter'); $metarPtr = 1; // get_station identity is ignored $group = 1; while ($group < count($groupName)) { $part = $metarParts[$metarPtr]; $groupName[$group]($part,$metarPtr,$group,$wxInfo); // $groupName is a function variable } } else $wxInfo['ERROR'] = 'Data not available'; } function get_station($part, &$metarPtr, &$group, &$wxInfo) { // Ignore station code. Script assumes this matches requesting $station. // This function is never called. It is here for completeness of documentation. if (strlen($part) == 4 and $group == 0) { $group++; $metarPtr++; } } function get_time($part, &$metarPtr, &$group, &$wxInfo) { // Ignore observation time. This information is found in the first line of the NWS file. // Format is ddhhmmZ where dd = day, hh = hours, mm = minutes in UTC time. if (substr($part,-1) == 'Z') $metarPtr++; $group++; } function get_station_type($part, &$metarPtr, &$group, &$wxInfo) { // Ignore station type if present. if ($part == 'AUTO' || $part == 'COR') $metarPtr++; $group++; } function get_wind($part, &$metarPtr, &$group, &$wxInfo) { // Decodes wind direction and speed information. // Format is dddssKT where ddd = degrees from North, ss = speed, KT for knots, // or dddssGggKT where G stands for gust and gg = gust speed. (ss or gg can be a 3-digit number.) // KT can be replaced with MPH for meters per second or KMH for kilometers per hour. function speed($part, $unit) { // Convert wind speed into miles per hour. // Some other common conversion factors (to 6 significant digits): // 1 mi/hr = 1.15080 knots = 0.621371 km/hr = 2.23694 m/s // 1 ft/s = 1.68781 knots = 0.911344 km/hr = 3.28084 m/s // 1 knot = 0.539957 km/hr = 1.94384 m/s // 1 km/hr = 1.852 knots = 3.6 m/s // 1 m/s = 0.514444 knots = 0.277778 km/s if ($unit == 'KT') $speed = round(1.1508 * $part); // from knots elseif ($unit == 'MPS') $speed = round(2.23694 * $part); // from meters per second else $speed = round(0.621371 * $part); // from km per hour $speed = "$speed mph"; return $speed; } if (ereg('^([0-9G]{5,10}|VRB[0-9]{2,3})(KT|MPS|KMH)$',$part,$pieces)) { $part = $pieces[1]; $unit = $pieces[2]; if ($part == '00000') { $wxInfo['WIND'] = 'calm'; // no wind } else { ereg('([0-9]{3}|VRB)([0-9]{2,3})G?([0-9]{2,3})?',$part,$pieces); if ($pieces[1] == 'VRB') $direction = 'varies'; else { $angle = (integer) $pieces[1]; $compass = array('N','NNE','NE','ENE','E','ESE','SE','SSE','S','SSW','SW','WSW','W','WNW','NW','NNW'); $direction = $compass[round($angle / 22.5) % 16]; } if ($pieces[3] == 0) $gust = ''; else $gust = ', gusting to ' . speed($pieces[3], $unit); $wxInfo['WIND'] = $direction . ' at ' . speed($pieces[2], $unit) . $gust; } $metarPtr++; } $group++; } function get_var_wind($part, &$metarPtr, &$group, &$wxInfo) { // Ignore variable wind direction information if present. // Format is fffVttt where V stands for varies from fff degrees to ttt degrees. if (ereg('([0-9]{3})V([0-9]{3})',$part,$pieces)) $metarPtr++; $group++; } function get_visibility($part, &$metarPtr, &$group, &$wxInfo) { // Decodes visibility information. This function will be called a second time // if visibility is limited to an integer mile plus a fraction part. // Format is mmSM for mm = statute miles, or m n/dSM for m = mile and n/d = fraction of a mile, // or just a 4-digit number nnnn (with leading zeros) for nnnn = meters. static $integerMile = ''; if (strlen($part) == 1) { // visibility is limited to a whole mile plus a fraction part $integerMile = $part . ' '; $metarPtr++; } elseif (substr($part,-2) == 'SM') { // visibility is in miles $part = substr($part,0,strlen($part)-2); if (substr($part,0,1) == 'M') { $prefix = 'less than '; $part = substr($part, 1); } else $prefix = ''; if (($integerMile == '' && ereg('[/]',$part,$pieces)) || $part == '1') $unit = ' mile'; else $unit = ' miles'; $wxInfo['VISIBILITY'] = $prefix . $integerMile . $part . $unit; $metarPtr++; $group++; } elseif (substr($part,-2) == 'KM') { // unknown (Reported by NFFN in Fiji) $metarPtr++; $group++; } elseif (ereg('^([0-9]{4})$',$part,$pieces)) { // visibility is in meters $distance = round($part/ 621.4, 1); // convert to miles if ($distance > 5) $distance = round($distance); if ($distance <= 1) $unit = ' mile'; else $unit = ' miles'; $wxInfo['VISIBILITY'] = $distance . $unit; $metarPtr++; $group++; } elseif ($part == 'CAVOK') { // good weather $wxInfo['VISIBILITY'] = 'greater than 7 miles'; // or 10 km $wxInfo['CONDITIONS'] = ''; $wxInfo['CLOUDS'] = 'clear skies'; $metarPtr++; $group += 4; // can skip the next 3 groups } else { $group++; } } function get_runway($part, &$metarPtr, &$group, &$wxInfo) { // Ignore runway information if present. Maybe called a second time. // Format is Rrrr/vvvvFT where rrr = runway number and vvvv = visibility in feet. if (substr($part,0,1) == 'R') $metarPtr++; else $group++; } function get_conditions($part, &$metarPtr, &$group, &$wxInfo) { // Decodes current weather conditions. This function maybe called several times // to decode all conditions. To learn more about weather condition codes, visit section // 12.6.8 - Present Weather Group of the Federal Meteorological Handbook No. 1 at // www.nws.noaa.gov/oso/oso1/oso12/fmh1/fmh1ch12.htm static $conditions = ''; static $wxCode = array( 'VC' => 'nearby', 'MI' => 'shallow', 'PR' => 'partial', 'BC' => 'patches of', 'DR' => 'low drifting', 'BL' => 'blowing', 'SH' => 'showers', 'TS' => 'thunderstorm', 'FZ' => 'freezing', 'DZ' => 'drizzle', 'RA' => 'rain', 'SN' => 'snow', 'SG' => 'snow grains', 'IC' => 'ice crystals', 'PE' => 'ice pellets', 'GR' => 'hail', 'GS' => 'small hail', // and/or snow pellets 'UP' => 'unknown', 'BR' => 'mist', 'FG' => 'fog', 'FU' => 'smoke', 'VA' => 'volcanic ash', 'DU' => 'widespread dust', 'SA' => 'sand', 'HZ' => 'haze', 'PY' => 'spray', 'PO' => 'well-developed dust/sand whirls', 'SQ' => 'squalls', 'FC' => 'funnel cloud, tornado, or waterspout', 'SS' => 'sandstorm/duststorm'); if (ereg('^(-|\+|VC)?(TS|SH|FZ|BL|DR|MI|BC|PR|RA|DZ|SN|SG|GR|GS|PE|IC|UP|BR|FG|FU|VA|DU|SA|HZ|PY|PO|SQ|FC|SS|DS)+$',$part,$pieces)) { if (strlen($conditions) == 0) $join = ''; else $join = ' & '; if (substr($part,0,1) == '-') { $prefix = 'light '; $part = substr($part,1); } elseif (substr($part,0,1) == '+') { $prefix = 'heavy '; $part = substr($part,1); } else $prefix = ''; // moderate conditions have no descriptor $conditions .= $join . $prefix; // The 'showers' code 'SH' is moved behind the next 2-letter code to make the English translation read better. if (substr($part,0,2) == 'SH') $part = substr($part,2,2) . substr($part,0,2). substr($part, 4); while ($code = substr($part,0,2)) { $conditions .= $wxCode[$code] . ' '; $part = substr($part,2); } $wxInfo['CONDITIONS'] = $conditions; $metarPtr++; } else { $wxInfo['CONDITIONS'] = $conditions; $group++; } } function get_cloud_cover($part, &$metarPtr, &$group, &$wxInfo) { // Decodes cloud cover information. This function maybe called several times // to decode all cloud layer observations. Only the last layer is saved. // Format is SKC or CLR for clear skies, or cccnnn where ccc = 3-letter code and // nnn = altitude of cloud layer in hundreds of feet. 'VV' seems to be used for // very low cloud layers. (Other conversion factor: 1 m = 3.28084 ft) static $cloudCode = array( 'SKC' => 'clear skies', 'CLR' => 'clear skies', 'FEW' => 'partly cloudy', 'SCT' => 'scattered clouds', 'BKN' => 'mostly cloudy', 'OVC' => 'overcast', 'VV' => 'vertical visibility'); if ($part == 'SKC' || $part == 'CLR') { $wxInfo['CLOUDS'] = $cloudCode[$part]; $metarPtr++; $group++; } else { if (ereg('^([A-Z]{2,3})([0-9]{3})',$part,$pieces)) { // codes for CB and TCU are ignored $wxInfo['CLOUDS'] = $cloudCode[$pieces[1]]; if ($pieces[1] == 'VV') { $altitude = (integer) 100 * $pieces[2]; // units are feet $wxInfo['CLOUDS'] .= " to $altitude ft"; } $metarPtr++; } else { $group++; } } } function get_temperature($part, &$metarPtr, &$group, &$wxInfo) { // Decodes temperature and dew point information. Relative humidity is calculated. Also, // depending on the temperature, Heat Index or Wind Chill Temperature is calculated. // Format is tt/dd where tt = temperature and dd = dew point temperature. All units are // in Celsius. A 'M' preceeding the tt or dd indicates a negative temperature. Some // stations do not report dew point, so the format is tt/ or tt/XX. function get_heat_index($tempF, $rh, &$wxInfo) { // Calculate Heat Index based on temperature in F and relative humidity (65 = 65%) if ($tempF > 79 && $rh > 39) { $hiF = -42.379 + 2.04901523 * $tempF + 10.14333127 * $rh - 0.22475541 * $tempF * $rh; $hiF += -0.00683783 * pow($tempF, 2) - 0.05481717 * pow($rh, 2); $hiF += 0.00122874 * pow($tempF, 2) * $rh + 0.00085282 * $tempF * pow($rh, 2); $hiF += -0.00000199 * pow($tempF, 2) * pow($rh, 2); $hiF = round($hiF); $hiC = round(($hiF - 32) / 1.; $wxInfo['HEAT INDEX'] = "$hiF°F ($hiC°C)"; } } function get_wind_chill($tempF, &$wxInfo) { // Calculate Wind Chill Temperature based on temperature in F and // wind speed in miles per hour if ($tempF < 51 && $wxInfo['WIND'] != 'calm') { $pieces = explode(' ', $wxInfo['WIND']); $windspeed = (integer) $pieces[2]; // wind speed must be in miles per hour if ($windspeed > 3) { $chillF = 35.74 + 0.6215 * $tempF - 35.75 * pow($windspeed, 0.16) + 0.4275 * $tempF * pow($windspeed, 0.16); $chillF = round($chillF); $chillC = round(($chillF - 32) / 1.; $wxInfo['WIND CHILL'] = "$chillF°F ($chillC°C)"; } } } if (ereg('^(M?[0-9]{2})/(M?[0-9]{2}|[X]{2})?$',$part,$pieces)) { $tempC = (integer) strtr($pieces[1], 'M', '-'); $tempF = round(1.8 * $tempC + 32); $wxInfo['TEMP'] = "$tempF°F ($tempC°C)"; get_wind_chill($tempF, $wxInfo); if (strlen($pieces[2]) != 0 && $pieces[2] != 'XX') { $dewC = (integer) strtr($pieces[2], 'M', '-'); $dewF = round(1.8 * $dewC + 32); $wxInfo['DEWPT'] = "$dewF°F ($dewC°C)"; $rh = round(100 * pow((112 - (0.1 * $tempC) + $dewC) / (112 + (0.9 * $tempC)), ); $wxInfo['HUMIDITY'] = $rh . '%'; get_heat_index($tempF, $rh, $wxInfo); } $metarPtr++; $group++; } else { $group++; } } function get_altimeter($part, &$metarPtr, &$group, &$wxInfo) { // Decodes altimeter or barometer information. // Format is Annnn where nnnn represents a real number as nn.nn in inches of Hg, // or Qpppp where pppp = hectoPascals. // Some other common conversion factors: // 1 millibar = 1 hPa // 1 in Hg = 0.02953 hPa // 1 mm Hg = 25.4 in Hg = 0.750062 hPa // 1 lb/sq in = 0.491154 in Hg = 0.014504 hPa // 1 atm = 0.33421 in Hg = 0.0009869 hPa if (ereg('^(A|Q)([0-9]{4})',$part,$pieces)) { if ($pieces[1] == 'A') { $pressureIN = substr($pieces[2],0,2) . '.' . substr($pieces[2],2); // units are inches Hg $pressureHPA = round($pressureIN / 0.02953); // convert to hectoPascals } else { $pressureHPA = (integer) $pieces[2]; // units are hectoPascals $pressureIN = round(0.02953 * $pressureHPA,2); // convert to inches Hg } $wxInfo['BAROMETER'] = "$pressureHPA hPa ($pressureIN in Hg)"; $metarPtr++; $group++; } else { $group++; } } function print_wxInfo($wxInfo) { // Prints each piece of information stored in the array. // If an error occurs in retrieving a METAR file, check for index called 'ERROR'. // (Modify this function to fit your needs.) $dots = '...............'; foreach($wxInfo As $wxIndex => $wx) { if (strlen($wx) != 0) echo $wxIndex . substr($dots,0,strlen($dots)-strlen($wxIndex)) . " $wx<BR>\n"; } } ?> <?php // require_once ("functions_weather.php"); $location = array("KMIA","KJFK"); foreach ($location as $station) { $wxInfo['STATION'] = $station; $metar = get_metar($station,$wxInfo); process_metar($metar,$wxInfo); echo "METAR = $metar<BR><BR>\n"; print_wxInfo($wxInfo); } ?>
-
can you provide another station. By accident I ran the code through a tester with "COLD" still in there, and most of the data was cached so If I can test it with another station that is real. METAR = KJFK 272351Z 26012KT 10SM FEW050 SCT250 18/11 A3004 RMK AO2 SLP172 60010 T01780111 10200 20178 53028 STATION........ KJFK OBSERVED....... Sat Oct 27, 16:51 PDT NOW............ Sat Oct 27, 17:08 PDT AGE............ 17 min ago WIND........... W at 14 mph VISIBILITY..... 10 miles CLOUDS......... scattered clouds TEMP........... 64°F (18°C) DEWPT.......... 52°F (11°C) HUMIDITY....... 64% BAROMETER...... 1017 hPa (30.04 in Hg) METAR = STATION........ COLD OBSERVED....... Sat Oct 27, 16:51 PDT NOW............ Sat Oct 27, 17:08 PDT AGE............ 17 min ago WIND........... W at 14 mph VISIBILITY..... 10 miles CLOUDS......... scattered clouds TEMP........... 64°F (18°C) DEWPT.......... 52°F (11°C) HUMIDITY....... 64% BAROMETER...... 1017 hPa (30.04 in Hg) ERROR.......... Data not available
-
What is the block of code in fubctions_weather.php? Also, try include_once() as they handle errors differently.
-
Have you tried putting the station data into an array? <?php require_once ("functions_weather.php"); $location = array("KJFK","COLD"); foreach ($location as $station) { $wxInfo['STATION'] = $station; $metar = get_metar($station,$wxInfo); process_metar($metar,$wxInfo); echo "METAR = $metar<BR><BR>\n"; print_wxInfo($wxInfo); } ?> best, richard
-
You're right the ) does need to be there to balance your code. I use a code based designer phpDesigner 2007 and it has a great debugger. I can't see what's in the includes, but if you try this software, it may be able to reveal any potential problems. Also, have you reveresd the session_start and ob_start
-
Try this SELECT * FROM members WHERE membershipno LIKE '%". $search ."%'
-
$result=mysql_query($sql) or die(mysql_error()); // extra ), possibly causing this to be an undefined function // Should be $result=mysql_query($sql) or die(mysql_error(); Also it appears that the session start headers were already sent in ob_start. As long as PHP is buffering the ouytput, the client can not get in. Call session_start before ob_start
-
SELECT max(photo_id) as lastid FROM photos WHERE photo_filename <>''; Or did you want to grab the filename and check to see if it was uploaded and then either display or show error?
-
I use the . in my select query. WHERE id = ". $_GET["id"]; or try for test purposes redefine the id variable by adding it on the line before the $sql="UPDATE ..." $id = ". $_GET["id"]; best
-
This is the code that I use in my .htaccess file AddType application/x-httpd-php .php .html
-
htaccess can solve the problem. Take a look at this link: http://www.spiderpro.com/bu/buphph001.html
-
One item that I use as part of a select that checks a specific field value is "metaphone".
-
How or Where are you defining the $id used to update the record? WHERE id='$id'";
-
Here is a sample file to upload a tutorial in say "PDF" format. This example assumes there is a Maximum file size. It then inserts the filename and title into a Database and uploads the file to a directory of your choice (make sure permissions are set for writing). The database will allow you to easily list your files. It is a good idea to follow the advice on the previous post not to make the file active by adding another field for approval (i.e. approved with values of 0 or 1 ) with the default being inactive. There is probably more here than you need, but it is a way of uploading an existing file. <?php if ($submit) { foreach($HTTP_POST_FILES as $value) { foreach($value as $k => $v) { echo $k.' => '.$v.'<br>'; } } ?> <?php // In PHP earlier then 4.1.0, $HTTP_POST_FILES should be used instead of $_FILES if($HTTP_POST_FILES['userfile']['name'] == '') { echo 'You did not select a file to upload'; } elseif($HTTP_POST_FILES['userfile']['size'] == 0) { echo 'There appears to be a problem with the file your are uploading'; } elseif($HTTP_POST_FILES['userfile']['size'] > $MAX_FILE_SIZE) { echo 'The file you selected is too large'; } elseif(!filesize($HTTP_POST_FILES['userfile']['tmp_name'])) { echo 'The file you selected is not a valid file type'; } else { $uploaddir = '/usr/local/www/vhosts/Your_PATH_HERE/docs/'; // remember the trailing slash! $uploadfile = $uploaddir. $HTTP_POST_FILES['userfile']['name']; if(move_uploaded_file($HTTP_POST_FILES['userfile']['tmp_name'], $uploadfile)) { echo 'Upload file success!'; } else { echo 'There was a problem uploading your file.<br>'; print_r($HTTP_POST_FILES); } } ?> <?php $db = mysql_connect("localhost", "YourUsername", "PASSWORD") or die("Could not connect"); $provridb = mysql_select_db("YOUR_DB_NAME",$db); $name = $HTTP_POST_FILES['userfile']['name']; $sql = "INSERT INTO tutorial ( title,filename,category, ) VALUES ('$title','$name','$category')"; $result = mysql_query($sql); echo "Thank you! Your file was posted \n"; ?><br><br> <a href="add-doc.php"><span class="caption"><font color="#0033FF">Add Another Document</font></span></a> | <a href="logout2.php"><span class="caption"><font color="#0033FF">LOGOUT</font></span></a> | <a href="#">Go to DOC List page</a> (not active yet) <br> <?php } else{ // display form ?> <form enctype="multipart/form-data" name="docs" method="post" action="add-doc.php"> <input type="hidden" name="category" value="env_doc"> <span class="caption">Please provide the following information</span>:<br> <hr size="=0" color="#CCCCCC" width="200" align="left"> <br> <br> File Title: <input name="title" type="Text" size="62"> <br> <br> <input type="hidden" name="MAX_FILE_SIZE" value="900000"> Upload this file: <input name="userfile" size="50" type="file"> <BR> <br> <br> <input type="Submit" name="submit" value="Submit File"> <input type="reset" name="Clear" value="Clear"> <br> </p> </form> </p> <?php } // end if ?>
-
convert mysql date format "2007-04-01" to "April 2007" output
richardw replied to scotchegg78's topic in PHP Coding Help
I see you have two good options. Are you all set with the the output? Only asking because of the "compare and output the correct month string" comment. Best -
[SOLVED] Creating Dynamic PHP Pages from a MySQL Database
richardw replied to Gates_of_Janus's topic in PHP Coding Help
Here's some my sql / php code to use as an example. You will have to modify to fit your server and database structure. <?php $conn= mysql_connect("localhost" ,"username" ,"pwwd" ) ; if(!$conn) { echo "Unable to connect to DB: " . mysql_error(); exit; } if (!mysql_select_db("YourDatabaseName")) { echo " Unable to select Your DatabaseName Database: " . mysql_error() ; exit; } // SELECT Query to display a specific record: directory $sql ="SELECT * FROM Your DatabaseName WHERE id = ". $HTTP_GET_VARS["id"]; // There are other options than this GET $result = mysql_query($sql); $row = mysql_fetch_array($result); // sample output format <?php echo $row["id"] ?> <?php echo $row["date"] ?> <?php echo $row["f_name"] ?> <?php echo $row["m_name"] ?> <?php echo $row["l_name"] ?> <?php echo $row["title"] ?> <?php echo $row["email"] ?> <?php echo $row["phone"] ?> <?php echo $row["fax"] ?> <?php echo $row["expertise"] ?> Best -
convert mysql date format "2007-04-01" to "April 2007" output
richardw replied to scotchegg78's topic in PHP Coding Help
Just to confirm, the exidting date is a string "2007-04-01" and not a timestamp or other date field format? -
[SOLVED] Most important PHP functions to learn in 3 hours
richardw replied to richardw's topic in Miscellaneous
Thanks Everyone! Richard -
[SOLVED] Most important PHP functions to learn in 3 hours
richardw replied to richardw's topic in Miscellaneous
Thanks for the siteppoint link, I will add it to me resources handout. I was just hoping to get feedback suggestive of actual usage. Writing the class with examples will still be the fun part. I just want to make the most of limited time. Should I pull this thread or is there a better forum for this? Richard