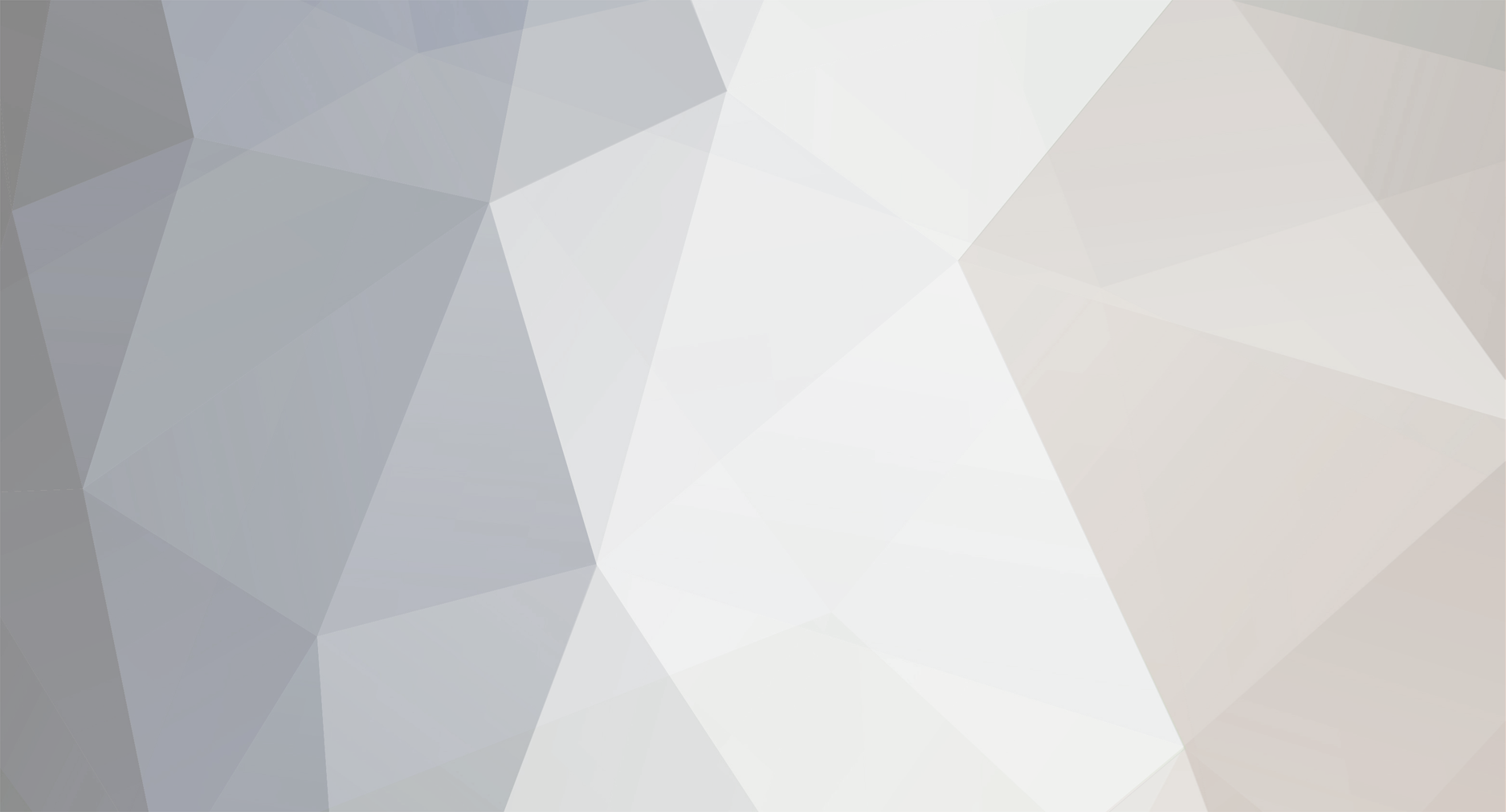
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
For Win7, the design decision was to hide away a bunch of stuff from the illiterate so they couldn't mess things up. It's a "Configure it via a nearly idiot proof wizard, then leave it alone" design. It's not without its flaws (as the internet connection anecdote above demonstrates, and I've actually run into similar problems myself), but, for the most part, that's the kind of computer people want. Power users will find their way regardless of OS. For the layperson, this kind of design is a godsend most of the time. Most people aren't geeks like us. They don't care how it works, only that it does work, works easily, and is compatible with what they use at work with minimal fuss.
-
Regarding the query, you have: $checkInfo = mysql_query("SELECT * FROM `user` WHERE `Email` = '$Email'") or die(mysql_error()); // ... $checkUserInfo = mysql_fetch_array($checkInfo); Then, later on, you have: $query = mysql_query("SELECT * FROM `user` WHERE `Email` = '$Email'") or die(mysql_error()); $user = mysql_fetch_array($query); Same query, same data. It's redundant, and unnecessary. For the password, your code leads me to believe that you don't store passwords as a hashed value within your db, which is a security flaw. It's far easier for someone with bad intent to brute force a non-hashed password than a hashed one. What you should do is something like: $password = validate($_POST['password']); // where validate() runs the password through regex so it's legit if (sha1($password) == $user['password'])) // or md5($password) if you saved it in the db with md5 { // do whatever }
-
I don't see why this is so difficult. You know how to retrieve data from the db, correct? If so, just do something like: $count = 0; while($row = mysql_fetch_assoc($result)) { if ($count % 4 == 0) { echo '<br /'>; } echo '<div class="item"><img src="path/to/image" alt="' . $row['alt'] . '" /></div>'; ++$count; } Where the div's class sets its size and floats it to the left.
-
1. Don't repeat queries. You already obtain the user info at the beginning. There's no reason to run the same query to get the same data you already have. 2. Don't use 'or die' to handle errors. It's a sloppy, inelegant way of doing it. Not only that, but dying with mysql_error() gives anyone with bad intent an idea of how your db is structured. It's a security risk. 3. Why aren't you checking the user's password in your db query? From the looks of it, you're not saving it as a hashed value. Bad idea. 4. Why aren't you validating or escaping user input at all? 5. Why are you passing the user's password to a session?
-
Here's what I'd do, if I were you: 1. Decide what you WANT to do. What do you like most in terms of computer-related activities? What do you like the least? 2. Research your local educational options. What schools are nearby? Are they legit? Do any of them have Associate's programs? 3. Combine the two. What kind of courses are offered by the local schools? Do they match your interests? Ultimately, the choice is yours alone to make. That means its up to you to do your due dilligance to make an informed decision. That means actually figuring out what you want to do and doing the legwork to make it happen. We can't give you any real advice if you don't even know what you'd ideally like to do.
-
As an aside, you shouldn't use addslashes to escape your data. Use mysql_real_escape_string instead.
-
Quick, dirty, and untested: <!DOCTYPE html> <html> <head></head> <body> <form name="form1" action="<?php echo $SERVER['PHP_SELF']; ?>" method="post"> <select name="country" id="country"> <option value="brazil">Brazil</option> </select> <div id="city"></div> <input type="submit" name="submit" value="Submit" /> </form> </body> <script type="text/javascript"> var oSelect = document.getElementById('country'); oSelect.onchange = function() { if (this.options[this.selectedIndex].value == 'brazil') { // do stuff } } </script> </html>
-
Why not just: foreach ($options as $key => $value) { $this->$key = $value; }
-
1. You need to tie your onchange event to the select element, not one of it's options. 2. You need to check it's selected index, not its value (google 'javascript select onchange' to see examples of what I mean). 3. You do have a submit button for your form, correct? You need a way to send the value to PHP.
-
Why would you want to cast $_POST as an object?
-
Try: foreach ($this as $value) { $value = mysql_real_escape_string($value); } Keep in mind, this will apply mysql_real_escape_string to every data member of your object. This also assumes you're using this code from within the object itself, like so: class test { private $firstName = "Forrest"; private $lastName = "Gump"; public function escapeData() { foreach ($this as $value) { $value = mysql_real_escape_string($value); } } } $example = new test(); $example->escapeData();
-
What, exactly, is inside of jQuery's $(document).ready() function? I ask because you don't want to put your function definition there. Only it's invocation.
-
Check box (no code this time well not yet any way)
KevinM1 replied to JasonBruce88's topic in PHP Coding Help
Just store them in a session: session_start(); $_SESSION['checkbox'] = $_POST['checkbox']; And retrieve them in another page from that session session_start(); $checkbox = $_SESSION['checkbox']; -
Like the error says, the eregi function is deprecated. Use one of the preg functions instead.
-
I don't think you're looking at extend in the right way. It doesn't extend your current class. It specifies that one class (template) is the child of a parent class (globalconf). Your parent class doesn't have access to members defined in the child. So globalconf doesn't magically get the echome method you define in template.
-
Yes.
-
Remember, every page that you want to use sessions on requires that you have session_start at the beginning of your code. So, something like: session_start(); // code code code $_SESSION['rowId'] = $row['id']; In the other file: session_start(); $rowId = $_SESSION['rowId']; That said, I think your problem stems from some confusion over how PHP and JavaScript interact with each other. Quite simply, unless you're using AJAX, they don't. PHP can't automatically tell which checkbox is selected. Your PHP script is finished running once your page is displayed on the screen. JavaScript runs in the browser, which is why it can react to things like click events. You have two ways of sending the checkbox data to the other page - AJAX, which is probably more complicated than you want to get, or making your table a form and posting the values to the other page when the form is submitted.
-
You can't concatenate echo statements. In other words: echo 'something' . echo 'something else'; Doesn't work. You should concatenate the strings instead: echo '<div>' . 'test' . '</div><br/><br/><br/>'; Of course, this can (and should) be treated as just one string, as you're not gaining anything by concatenating here. echo '<div>test</div><br /><br /><br />'; Also, when using double-quoted strings, you don't need to concatenate to display a variable's contents. So: echo "<div>$test</div><br /><br /><br />"; Will print out the value of $test within that div. echo '<div>$test</div><br /><br /><br />'; Will simply print out $test itself.
-
PHP already has a couple of built-in OOP database interfaces - mysqli and pdo. Unless you're writing this for your own educational needs, I suggest not reinventing the wheel.
-
Try: for ($i = 0; $i < 21; ++i) { if ($i % 3 == 0) { echo '<div style="margin-bottom:3px;text-align:right;">{$products1[$i]}: <input type="text" name="{$products1[$i]}" value="" size="6"></div>'; } else { echo '{$products1[$i]}: <input type="text" name="{$products1[$i]}" value="" size="6">'; } } The {}'s in the echos force the arrays to print their values. I like it better than entering-leaving-reentering the string with the . operator.
-
The errors tell me that your functions.php file is borked. The error resides there. Show code?
-
Your secret key exists in the session, correct? Well, you need to either use that value directly, or assign it to a variable which you then pass to the function. In other words: $user = check_user($_SESSION['secret_key']); or $secret_key = $_SESSION['secret_key']; $user = check_user($secret_key); Remember: if you need to use variables across pages, put them in a session. EDIT: or, if you're worried about the secret key being compromised, save it somewhere (file, db), then read it from that data store when you need to access it.
-
Use the modulus operator: if ($i % 3 == 0) { // add break } else { // process as normal }
-
Don't use 'global.' Pass it as an argument to the function instead.
-
You have two options: 1. Define your own escape_data() function, since nothing named escape_data() exists in PHP (hence your 'undefined' error). 2. Use the escape function that relates to the kind of database you're using, such as mysql_real_escape_string.