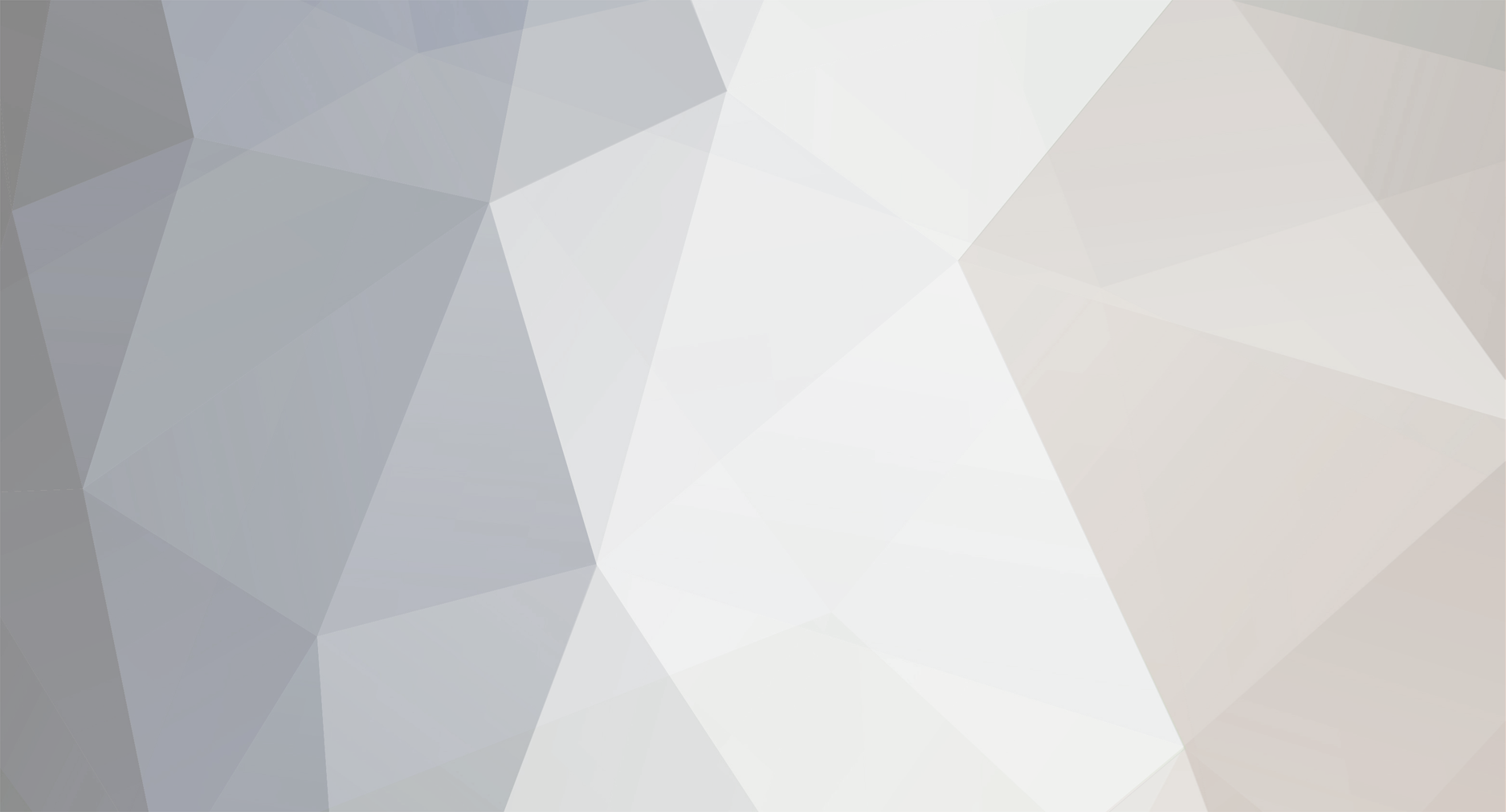
Fehnris
-
Posts
46 -
Joined
-
Last visited
Posts posted by Fehnris
-
-
From looking at your registration form, I can not see a submit button called 'submit_registration_x' which you check further down with another if statement which looks like it enters a new student into the database. Try renaming the submit button of the registration form to 'submit_registration_x'.
Also it looks like your nesting html forms which is a no no. In your HTML at the bottom you open a form and dont close it before echoing out the $registration_form and $students. -
The fact that when you echo out the query string tells me that for some reason its a problem with the $_POST['username'] variable as your query string echo is blank between the single quotes after username= (high lighted in red). It should contain the username that was sent via the login form.
query = SELECT firstname, lastname, photo FROM `plateau_pros` WHERE username= [color=red]''[/color]
Main reason why I thought it might be a good idea to have an echo to check there is actually something being passed in the $_POST['username'] variable. Something like (shown in red):-
// Enable sessions
session_start();
// Turn on magic quotes to prevent SQL injection attacks
if(!get_magic_quotes_gpc())
set_magic_quotes_runtime(1);
include 'dbconfig.php';
// Connect to database
$conn = mysql_connect($dbhost, $dbuser, $dbpass) or die(mysql_error());
mysql_select_db($dbname) or die(mysql_error());
//new sql query
[color=red]echo "Post username = ".$_POST['username'];[/color]
$sql = "SELECT firstname, lastname, photo FROM `plateau_pros` WHERE username= '".$_POST['username']."'";
$result = mysql_query($sql, $conn) or die(mysql_error());
echo "query = ". $sql. "<br/>";
// Get Record Set
$result = mysql_fetch_array($sql, MYSQL_ASSOC);
If the echo for the username is blank you know its a problem somewhere along the line from the $_POST['username'] being posted to when your php page requests it.
I see your using sessions. I have no experience with using them but am wondering if that might have something to do with getting a blank value.
Maybe someone with more knowledge on sessions could jump in here and say absolutely nothing to do with it or a possibility and why. :) -
Its not possible from the code you have supplied to say whether the $text variables are either an array or part of the database.
There is no association between $text and data in your database. There would be a couple of lines in there after the database connection and database selection to select data. Something like:-
$somequery = "Select......";
$somequeryresult("$somequery");
$text = mysql_fetch_array($somequeryresult);
to give an indication that the $text variables contain your database records.
Nor is there an association between $text and it being an array containing data. There is no array initialisation. Something like:-
$text = array($variable1, $variable2,.....$variableN)).
Quite frankly it doesnt look like the $text values do anything at all.
What does this code do when you run it? You have included a screen grab of what the page is supposed to look like when viewed in a browser but from the code you've posted I just dont see how the code and output match up. -
Looks like the '$_POST['username'] value for some reason isnt coming through. You could also try echoing the $_POST['username'] out before you create your sql string.
That would show you that its not a problem with the username value not making it through to your PHP page.
A quick question, what method does your login form use i.e POST or GET. As your using $_POST to retrieve the username field, you also have to make sure that the login form also uses POST as the method. Havent seen the code for your Login page so appologies if you are using POST. -
You dont need to have the single quotes arround the table name for one thing and also the brackets arround the $sql, as its just a string declaration. Also the $_POST['username'] as its a variable needs to be outside the string.
[code]
$sql = ("SELECT firstname, lastname, photo FROM `plateau_pros` WHERE username= '$_POST['username']' ");
$result = mysql_query($sql, $conn) or die(mysql_error());
[/code]
should be
[code]
$sql = "SELECT firstname, lastname, photo FROM plateau_pros WHERE username= '".$_POST['username']."'";
$result = mysql_query($sql, $conn) or die(mysql_error());
[/code] -
you need to use some sort of 'JOIN' in your mysql 'SELECT' statement. Whats probably happening is that its getting all the records from your 'categories' table AND all the records from your 'tbl_links' table where 'categories.category_id = tbl_links.category_id'. A join would help although my knowledge on mysql joins isnt that wonderful. Possibly something like:-
"SELECT * FROM categories LEFT JOIN tbl_links ON categories.category_id = tbl_links.category_id;
You also need to move the database connection outside and above the if statement because if your 'else' is true there wont be a database connection to get the records. Also you need to move:-
[code]
$result = ("SELECT * FROM categories, tbl_links WHERE categories.category_id = tbl_links.category_id ORDER BY category_name");
$catResult = mysql_query($result) or die("error querying database");
$row = mysql_fetch_array($catResult);
[/code]
out of the 'if' to inside the 'else' otherwise the query wont be executed, resulting in 0 records to loop though and resulting in a blank drop down box.
Also in your html option tags you are setting the viewable text to the 'category' value of each link record so you will only get the categories displayed in the drop down list box. Not sure what you want to display in the list box as you havent really stated whether its ment to be the category or the link or some other information stored in your link table -
you need to have secure shell access to your webserver. After logging in you would type crontab -e. You could then setup a cron job with the command posted my obsidian
-
Rather than having a cron job could you not just check the ban date of the user trying to login and if the ban date is in the future you know not to let the user login.
-
convert all to seperate variables then use mktime to create new timestamp
[code]
//$date = <your date database field>
//$time = <your time database field>
$year = substr(strval($date), 0, 4);
$month = substr(strval($date), 4, 2);
$day = substr(strval($date), 6, 2);
$hour = (int) substr($time, 0, 2);
$minute = substr($time, 3, 2);
$timestamp = mktime($hour, $minute, 0, $month, $day, $year);
[/code]
put that in a loop to check through all your database records and add an update query to update the new timestamp field for each record. -
Look into the function stripslashes()
[url=http://us3.php.net/stripslashes]http://us3.php.net/stripslashes[/url] -
[code]
strtotime("+ " . $_POST['field5'] . " hours " . $_POST['<name of your minutes field>'] . "minutes");
$field5 = date("Y-m-d G:i:s", strtotime("+ " . $_POST['field5'] . " hours " . $_POST['<name of your minutes field>'] . "minutes"));
[/code]
should do the trick. -
You are correct in that you can run it yourself and chances are, the script worked when you ran it in your browser. The reason you get a blank page is because the script doesnt output anything.
You could do a check that its working by querying your database to find entries that the expiry date is older than the current date.
Then run the script from your browser.
Finally check the database to see if the records, found previous to running the script, have been erased. -
Im not sure of other ways to enter a cron job. You could message your web hosting provider to ask if they offer a way to select more options.
That script should be all you would need to delete any records with an expiry date/time older than the current date/time. -
cron jobs are 'automated' meaning that they dont need you to manually execute your php file. You set date and time under the cron job that you want the php file to execute. The email address you specify for the cron job is just for output of the php file. If for instance in your case you wanted to know what email addresses were sent a subscription you could 'echo' out each email address. The echo information or any output would be in the email sent to the address specified for the cron job.
-
If you mean subdomains i.e www.example.com, example.example.com then by not specifying the domain value, you are telling the setcookie function to make the cookie available to all parts of example.com.
If your wanting to set multiple different domains with the same cookie i.e www.example.com, www.example2.com, to my knowledge it can't be done with the setcookie as the cookie is only available to the domain that the setcookie function is executed on. -
That would work although it wouldn't be that accurate in terms of having the password active for exactly 24hrs. You would have to make sure that, to keep the 24hrs time frame, someone would login after the 24hr period has elapsed. Would be fine for a high traffic website but could prove a problem for one with low traffic.
To be honest, crontab is the way to go. It isnt to hard to set up once you know what your dealing with. -
You would need to have access to a webserver hosting a MYSQL database.
Alot of web hosting companies offer MYSQL as part of the hosting package.
You havent stated in your post whether you website is running on a webserver that you own or if its with a hosting company.
If your website is hosted with some hosting company, it might be worth checking with them, if your hosting plan includes a MYSQL database.
If its your own web server, you can download and install MYSQL relatively easily from [url=http://www.mysql.com]http://www.mysql.com[/url] -
Sure.
Not sure what options you have with vDeck but with cPanel you have fields to select 'Minute', 'Hour', 'Day', 'Month', 'Weekday'. How does vDeck let you enter the time? Sorry, I have had no experience with vDeck.
usually with cron jobs you have 5 characters representing the values of the fields about for example:-
minute hour day month weekday
* * * * *
having a cross for each of these would mean the script would run every minute of every hour of every day. To have it run every hour, on the hour you would specify 0 * * * *.
Once you have the cron sorted out all you would need to keep on top of your old records would be a php script to access the MYSQL database and remove any records that have an expiry date older than the current date. Something like:-
[code]
<?
$currentdate = date("Y-m-d H:i:s");
$databaseconnection = mysql_connect ("$HOST", "$USERNAME", "$PASSWORD") or die ('I cannot connect to the database because: ' . mysql_error());
mysql_select_db ("$DATABASENAME");
$querystring = "DELETE from <Database Table> where <expiry date field name> <= '$currentdate'";
$result = mysql_query("$querystring");
?>
[/code]
Upload your new PHP script file to your webspace and enter the path to it under the cron job path that you setup. That should be it. -
Hi Vigiw,
First off, do you know if your web hosting plan offers cron jobs? If you have access to website settings using cPanel then you usually have an icon called 'Cron Jobs'. Not sure if you know what this is so ... basically it is a way to automate script execution on your website.
It would be possible to write a simple PHP script that you could get to execute on a given minute/hour/day by setting up a cron job with details of when you want the script to execute. You could set up a cron job to execute every 30 minutes or less if you wanted.
The other issue with your users entering a number instead of an expiry date could be easy to do aswell. What I would probably do though is still keep the expiration date field the same in the database rather than add a new field for a number of 'hours'. Would mean your existing records wouldnt have to be changed to include a value for the numbers field.
If you were to add something like the following to your form processing page it would give you both a form post date and an expiry date in mysql timestamp format.
Having the user only enter a number instead of an expiry date though does mean you would need to add a bit more PHP coding to your file that processes the form and enters the data into the database. Wouldnt be to hard to convert the date they posted the form + number of hours into an expiration date before entering the expiration date into the database.
[code]
$hours = $_REQUEST['hours']; //the input hours field from the form of the calling page.
if ($hours == "")
{
$hours = 0; //checking for an empty string input - the user just submitted the form without specifying a value for hours.
}
$postdatetimestamp = time(); //creates UNIX timestamp for post date (current time).
$expiretimestamp = $postdatetimestamp + ($hours * 3600); //creates UNIX timestamp for post date plus so many hours (expiry time).
$postdatemysqltimestamp = date("Y-m-d H:i:s", $postdatetimestamp); //formats UNIX timestamp to MYSQL timestamp for post date.
$expiremysqltimestamp = date("Y-m-d H:i:s", $expiretimestamp); //formats UNIX timestamp to MYSQL timestamp for expiry date.
[/code]
..... connection to MYSQL database to add record including '$postdatemysqltimestamp' as the Post Date and '$expiremysqltimestamp' as the Expiry Date.
Hope all that helps you :)
-
Hi there,
I have a question regarding php/mysql and ssh.
I hope im in the right place to ask this. Well, here goes.
My question is does anyone know the best way to create a ssh tunnel using only PHP and then being able to use that tunnel to connect securely to a remote MYSQL database?
This procedure has been driving me loopy trying to figure out a way to do it. Ive found various library functions as part the PHP language but the information ive found on them, tends to be abit vague with a lack of working examples.
I hope there is someone out there that has already had experience with this and can offer some help.
Thanks in advance.
2 questions about list code
in PHP Coding Help
Posted
Your code with the mysql statements moved would look something like this.
[code]
<div id="main">
<h1>Add Link</h1>
<p>Please fill out the details below to add a link to the database. <strong>All of the fields must be completed before the form can be submitted.</strong></p>
<div style="width:100%">
<?php
include ('connections/config.php');
include ('connections/dbconn.php');
if(isset($_POST['add']))
{
$linkTitle = $_POST['inpTitle'];
$linkDesc = $_POST['inpDescription'];
$linkUrl = $_POST['inpLinkUrl'];
$linkCategory = $_POST['inpCat'];
$linkDisplay = $_POST['inpDisplay'];
$query = "INSERT INTO tbl_links (link_url, link_title, link_desc, category_id, link_display) VALUES ( '$linkUrl', '$linkTitle', '$linkDesc', '$linkCategory', '$linkDisplay')";
mysql_query($query) or die('Error, insert query failed');
echo "<p class='message'>Your Links have been successfully added, click here to <a href='add_link.php'>add another link</a></p>";
}
else
{
?>
<form method="post">
<table cellpadding="5">
<tr>
<th width="120">Field Name</th>
<th width="378">Field Inputs</th>
</tr>
<tr>
<td width="120"><div align="right"><strong>Category :</strong></div></td>
<td><select>
<?php
$result = "SELECT * FROM categories ORDER BY category_name";
$catResult = mysql_query($result) or die("error querying database");
$row = mysql_fetch_array($catResult);
echo "<option value=''>Select one</option>";
while($row = mysql_fetch_assoc($catResult)){
echo "<option value='$row[link_id]'>$row[category_name]</option>";
}
//Put at the bottom of the PHP statement
include ('connections/dbclose.php');
?>
</select> </td>
</tr>
<tr>
<td width="120"><div align="right"><strong>Title :</strong></div></td>
<td><input type="text" name="inpTitle" class="inputbox" size="72" /></td>
</tr>
<tr>
<td width="120"><div align="right"><strong>Description :</strong></div></td>
<td><input type="text" name="inpDescription" class="inputbox" size="72" /></td>
</tr>
<tr>
<td colspan="2"> </td>
</tr>
<tr>
<td width="120"><div align="right"><strong>Link URL <em>('http;//' not required)</em> :</strong></div></td>
<td><input type="text" name="inpLinkUrl" value="www.sitename.com" class="inputbox" size="72" /></td>
</tr>
<tr>
<td colspan="2"> </td>
</tr>
<tr>
<td width="120"><div align="right"><strong>Display Link :</strong></div></td>
<td><input name="inpDisplay" type="checkbox" class="inputbox" value="Yes" checked="CHECKED"/></td>
</tr>
<tr>
<td colspan="2"> </td>
</tr>
<tr>
<td width="120"><div align="right"><strong>Click to submit :</strong></div></td>
<td><input type="submit" value="Add This Link" name="add" id="add"/></td>
</tr>
</table>
</form>
<?
}
?>
</div><!--tablediv-->
</div><!--main--->
[/code]