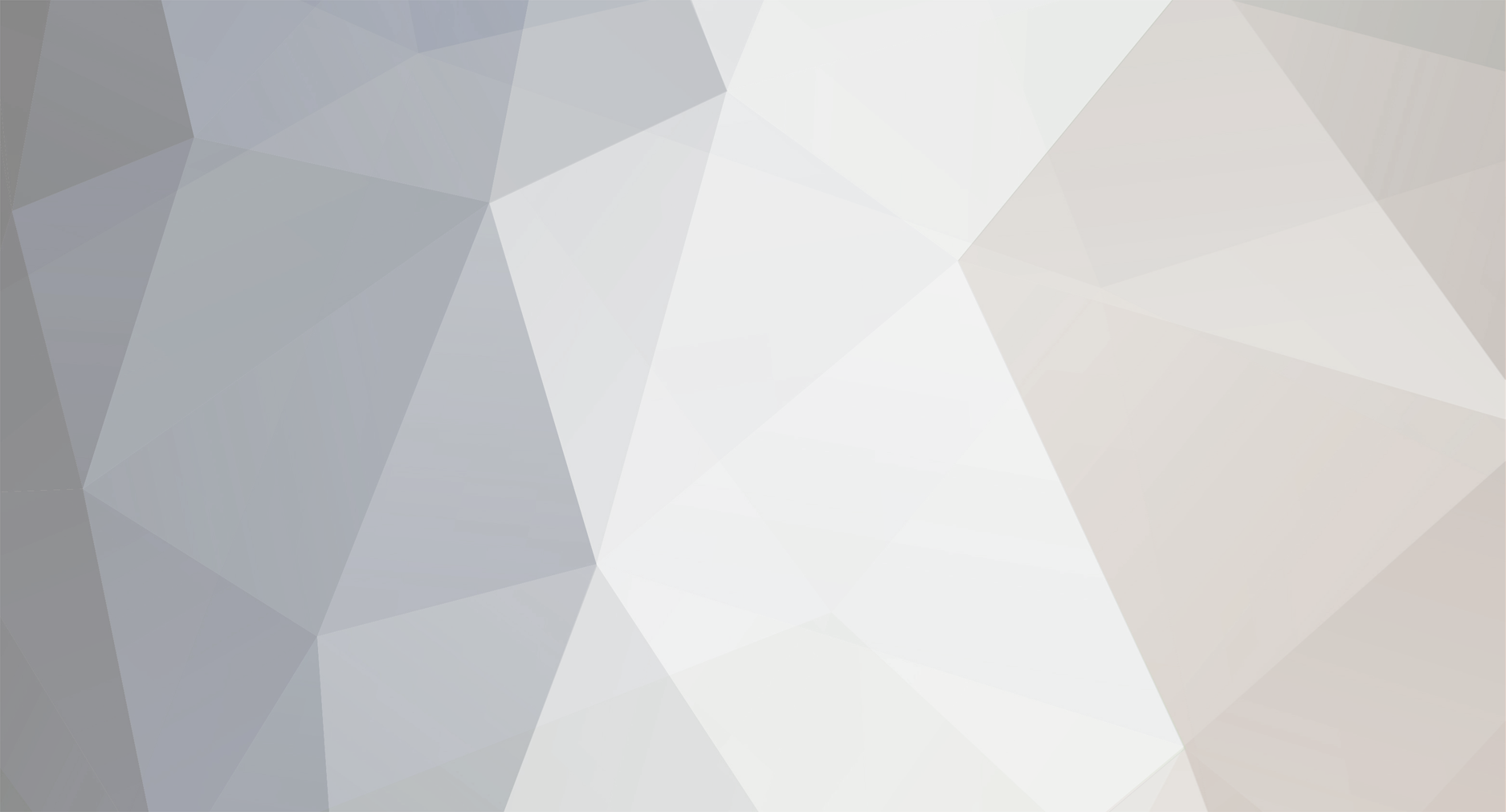
Wuhtzu
Members-
Posts
702 -
Joined
-
Last visited
Never
Everything posted by Wuhtzu
-
[SOLVED] Writing image to folder on server
Wuhtzu replied to bluebutterflyofyourmind's topic in PHP Coding Help
You simply add one of the functions I listed in my last reply after you have used imagecopyresampled: <?php //From your code $thumb = imagecreatetruecolor(100,100); imagecopyresampled($thumb,$Orig,0,0,0,0,100,100,$width,$height); //Save the image as JPEG to a folder imagejpeg($thumb, '/path/to/save/image/to/filename.jpg', 75); //Save the image as PNG to a folder imagepng($thumb, '/path/to/save/image/to/filename.png'); ?> Read up on the functions here: http://php.net/manual/en/function.imagejpeg.php http://php.net/manual/en/function.imagepng.php -
[SOLVED] Writing image to folder on server
Wuhtzu replied to bluebutterflyofyourmind's topic in PHP Coding Help
You might get a more specific / suited answer if you provide some of the code you use to create the thumbnails. The creation of images (resizing ect.) is often very closely combined with saving them as an actual file on the server. But imagejpeg(), imagegif() and imagepng() should do what you want: http://dk2.php.net/manual/en/function.imagejpeg.php -
It is because $_FILES['file']['tmp_name'] is not set. This is only set when the user submits the form and before that happens PHP will throw you "undefined index". You should do something like this: <?php if(isset($_FILES['file']) { Do what you wanna do after submitting } else { Show the form } ?>
-
I haven't come up with the prettiest method of verifying this with PHP but I'm working on it Personally I would like to do this with PHP instead of JavaScript since javascript can be disabled making the application non-functional.
-
I can see 3 solutions: #1: Use javascript as #thebadbad suggested #2: Just treat the image with lowest number as 1st and so forth. If the user selects image2, image4 and image6 translate that into image1, image2, image3 and give a f**k about what the user thought he or she did. #3: Do some validation with PHP and if they did not comply with your standards throw an error.
-
Are the user presented with 6 < input type="file" name="imagex"> at the same time or how is the "flow" of your application?
-
Google -> mime types
-
You need some form of URL rewriting. If you are on an Apache web server you will have to look into mod_rewrite....
-
http://dk.php.net/ucwords
-
http://www.w3.org/TR/html4/interact/forms.html#h-17.11.2
-
[SOLVED] easy one! $variable[]= 'hello'; or $variable='hello';
Wuhtzu replied to farkewie's topic in PHP Coding Help
$var[] is an array.... -
http://www.phpfreaks.com/tutorials/141/0.php
-
[SOLVED] Overlaying text ontop of uploaded images
Wuhtzu replied to graham23s's topic in PHP Coding Help
Here is a tutorial about image resizing and watermarking. Found by searching for "gdlib php watermark" http://www.phpit.net/article/image-manipulation-php-gd-part2/ (have a look at page3) -
That's default I would like to see what you ended up doing
-
I can't explain why you code does not work... You have an option specified for the case which does not seem to work. But I do not understand your calculations. Regarding the code I posted I didn't say it was copy'n'paste ready. I only meant the resize mechanism. It has even been represented in a book: http://wuhtzu.dk/random/php_and_mysql_web_development_c26_p560.jpg http://wuhtzu.dk/random/php_and_mysql_web_development_c26_p561.jpg And to demonstrate it works you can have a look at these to images: http://wuhtzu.dk/sandbox/imagemanipulator/image.php?img=100x600.jpg&max_w=600&max_h=600 http://wuhtzu.dk/sandbox/imagemanipulator/image.php?img=600x100.jpg&max_w=3000&max_h=600 (or any of the images at http://wuhtzu.dk/sandbox/imagemanipulator/image.php) If max_w and max_h specifies maximum height and maximum width of the image you want created. If you can make any of these images (the two linked to or any other from the list) break the resize mechanism please let me know.
-
I have tried your code and I know that some combinations of height and with does not work out. For example does an image which is 150x80 px (width x height) resize to 159x84 px. What do you actually wanna do? If you wanted to resize an image to fit within a maximum width and maximum height you would use what I posted since it's the simplest and most logic way of doing such a resize. But since you don't you must want something else... What exactly are you trying to do?
-
I just did some reading up on unset() and the way the array behaves has nothing to do with the internal pointer of the array. Unset() simply destroys the value of an variable but the variable it self still exists. So if you unset($array[2]) then $array[2] will still exist but contain no value. So that is why the index is still used even though you have unset() it. Try this for the learning experience: <?php $var = 'I have a value'; //Will output "I have a value"; echo $var; unset($var); //Will output '$var is still set' if(isset($var)) { echo '$var is still set'; echo '<br>'; } else { echo '$var is not set'; echo '<br>'; } //Will output nothing echo $var; ?> Sorry for giving the wrong explanation at first
-
Ahh okay The explanation "without deleting the array it self" is a pretty good picture but of course not technically correct. I don't know how to explain it technically correct, but someone might. It has to do with the internal pointer of the array. It apparently does not jump back to index which has been made available by unset(). To get an idea of what the internal pointer is try look at current(), next(), prev() ect.: http://dk.php.net/manual/en/function.next.php (the example)
-
What might have happened before the code you posted is that the previously array indexes (0, 1, 2, 3, 4) have already been used: <?php //Create an array containing 5 elements $array = array("1st Element", "2nd Element", "3rd Element", "4th Element", "5th Element"); //Another way of doing that $array[0] = "1st Element"; . . . $array[4] = "5th Element"; //Now if you add another element it will get the key 5: $array[] = "6th Element"; //This will become $array[5] since 0-4 has already been used ?>
-
The code posted will make $array[0] contain the value '6'. If it actually has $array[5] contain '6' you are holding back some code Edit: Unfortunately you can't color text inside the codebox It would be a very nice feature though!
-
Unless you wanna stretch and cut in your image (which I don't think you want to) just stick with this very simple code: <?php //Max height and width $max_height = 600; $max_width = 600; //Getting image size $size=getimagesize($image); $width=$size[0]; $height=$size[1]; //Calculating the new height and width $x_ratio=$max_width/$width; $y_ratio=$max_height/$height; if(($width <= $max_width) && ( $height <= $max_height)){ $tn_width=$width; $tn_height=$height; } else if(($x_ratio*$height) < $max_height){ $tn_height=ceil($x_ratio*$height); $tn_width=$max_width; } else{ $tn_width=ceil($y_ratio*$width); $tn_height=$max_height; } ?> I have been playing around with your code a little and the variable names are very confusing and your "cases" (different situations with the hight and width) do not seem logic. Unless you want stretching and cutting the above code works.
-
How about trying a larger file? Even your local apache server will take some time to process a 100MB file....
-
Check out this thread / post: http://www.phpfreaks.com/forums/index.php/topic,165733.msg728987.html#msg728987 The code holds a working method of calculating new height / width for pictures and it works not matter what dimensions you want and what dimensions you have. Of course this is build on the concept of maximum height and maximum width. If you want a thumbnail of maximum 80x80 px and have a picture which is 20x160 px you will end up with a picture of 10x80 px.
-
I don't know why I haven't noticed, but your if() statements is still wrong syntax.... <?php //Wrong if($price = 4); { } //Correct if($price == 4) { } ?> So the reason why your code do what it does is because even when for example if($price == 2) is true it does nothing because you have ended the statement with ; before you got to the part where you specify what to do. And apparently { $mode = "something"; } works even with the brackets around it. So you just keep on reassigning new values to $mode until it finally is assigned $mode = "price BETWEEN 300000 AND 400000"; By the way use a switch instead of all those if statements: <?php switch($price) { case 1: $mode = "price <= 100000"; break; case 2: $mode = "price BETWEEN 100000 AND 200000"; break; case 3: $mode = "price BETWEEN 200000 AND 300000"; break; case 4: $mode = "price BETWEEN 300000 AND 400000"; break; } ?>
-
Nothing seems out of the ordinary. $_POST['price'] should contain the value '3' if the user chooses < option value='3'>€200,000 - €300,000< /option> and if you usee == to compair the values in your if() statement the $mode variable should be set too... What happens if you set $price = 3; in our code (hard code it)?