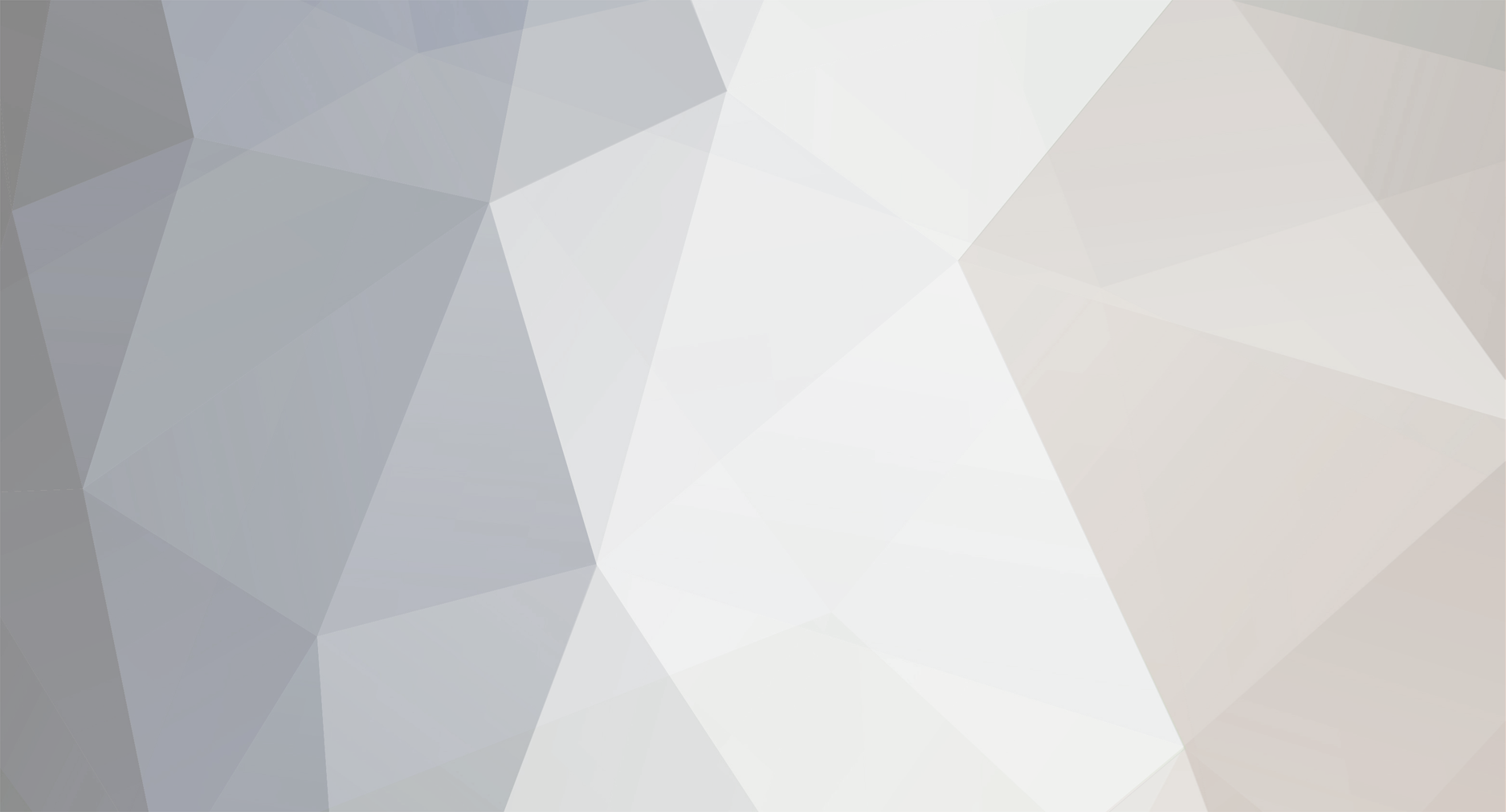
Matthew!!!
-
Posts
2 -
Joined
-
Last visited
Never
Matthew!!!'s Achievements

Newbie (1/5)
0
Reputation
Matthew!!! has no recent activity to show
0
We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.