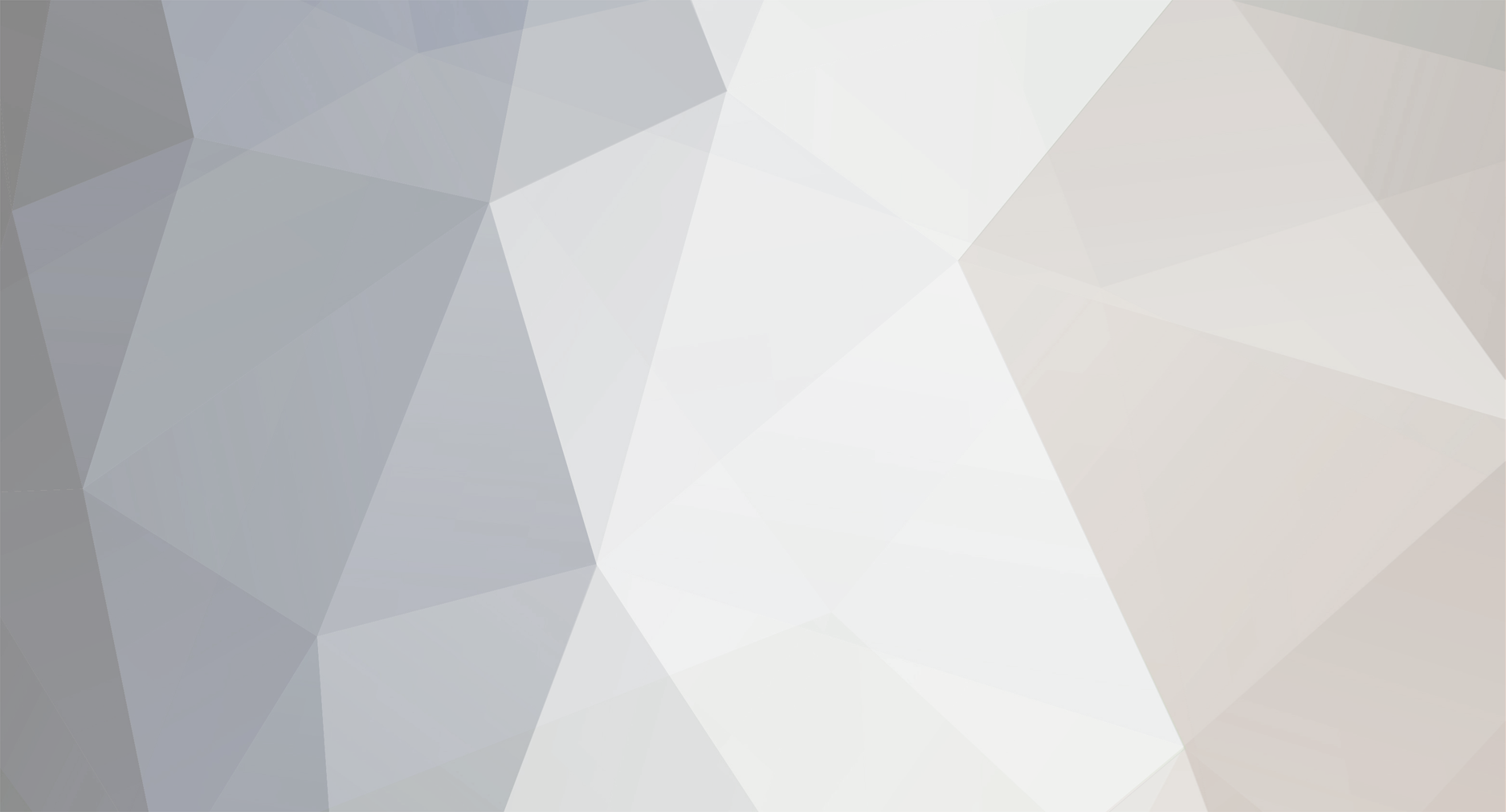
seran128
Members-
Posts
38 -
Joined
-
Last visited
Never
Everything posted by seran128
-
Works great!!!!!!!! thanks
-
I have two pages the first page has index.php [code]<? include('functions.php'); $content = getcontent(); ?>[/code] then later in that page I want to <? echo $content ?> so i can place the results of the function anywhere on my page. Functions.php [code]function getcontent(){ global $connection; $sql="select * from content"; $result=mysql_query($sql,$connection) or die(mysql_error()); while($row=mysql_fetch_array($result)) { ?> <table width="200" border="1"> <tr> <td><? echo stripslashes($row['content']); ?></td> </tr> <tr> <td>...................................................</td> </tr> </table> <? } return $news; }[/code] the problem is that it print the table out at the top of the page. I need to get the results of this function into a var named $news then I can echo that on the first page and controll its placement
-
I did that and now it is posting to the db but now I have another problem. When I past html code into the WYSIWYG editor it saves fine but when i call the function that displays the code <? getpagecontent(1); ?> It shows the html code on the page and not the product of the code.
-
I have a form that posts html code to a mysql db blob field, from a WYSIWYG editor. HOw can I get this to save if there are escape characters in the html code being posted and is there a special way of getting the code back out? the code that posts [code]$table = "webcontent_regions"; $content=$_POST["test1"]; $id=$_POST["id"]; $sql="update $table set content='$content' where regionId='$id'"; //echo $sql; $result=mysql_query($sql,$connection) or die(mysql_error()); header("location: ../list/wc_list.php");[/code] The code that retrieves the data [code]function getpagecontent($inId){ global $connection; $sql="select content from webcontent_regions where regionId='$inId'"; $result=mysql_query($sql,$connection) or die(mysql_error()); while($row=mysql_fetch_array($result)) { $content=$row['content']; } echo $content; }[/code]
-
I have a table that looks like this tbl_product ID Cost 1 5.23 2 3.76 7 25.22 now I want to run a query that returns the value of the difference between two product id's like psudeo code finalcost = (select cost from tbl_products where id = '1') - (select cost from tbl_products where id = '2') in this case finalcost should be 1.47 Is there a way to do this in one SQL statment
-
Multiple values from a database function [Solveded]
seran128 replied to seran128's topic in PHP Coding Help
thats it thanks!!!!!!!!! -
Multiple values from a database function [Solveded]
seran128 replied to seran128's topic in PHP Coding Help
i did a echo $sql; in the function and I get select * from webcontent_regions where regionId='31' When I cut and paste that query in navicat I do get a result -
Multiple values from a database function [Solveded]
seran128 replied to seran128's topic in PHP Coding Help
my new code but still not working adminfunctions.php [code]<? require("connection.php"); function geteditcontent($inTable,$inId){ $sql="select * from $inTable where regionId='$inId'"; $result=mysql_query($sql,$connection) or die(mysql_error()); while($row=mysql_fetch_array($result)) { $info[] = $row; } return $info; } ?>[/code] listcontent.php [code]<? include("../include/adminfunctions.php"); $fa =$_POST["fa"]; $id=$_POST["ItemId"]; $info = geteditcontent("webcontent_regions","$id"); ?> <form name="example" id="example" method="post" action="../include/formsubmit.php?mode=content"> <input type="hidden" name="id" value="<? echo $id; ?>" /> <div align="left"> <table width="100%" border="0"> <tr> <td colspan="2" class="ListTableHeader" ><div align="center"><strong>Update Content</strong></div></td> </tr> <tr> <td class="Results" >Region Id: <? echo $id ?> <div align="left"></div> Region Description: <? echo $info[0]['description'] ?> <div align="left"></div></td> </tr> <tr> <td> <textarea id="textarea1" name="test1" style="height: 100%; width: 100%;"><? echo $info[0]['content'] ?> </textarea></td> </tr> </table> <input type="submit" name="Submit" value="Save Content"> <input type="button" name="btnCancel" value="Cancel" onclick="JavaScript:history.back()" > </div> </form>[/code] -
I am new to php. I have come over from the darkside ASP/.net. My question how would i return the values from function to another page. My function is; adminfunctions.php [code]<? require("connection.php"); function geteditcontent($inTable,$inId){ $sql="select * from $inTable where regionId='$inId'"; $result=mysql_query($sql,$connection) or die(mysql_error()); while($row=mysql_fetch_array($result)) { $regionId=$row['regionID']; $description=$row['description']; $content=$row['content']; return; } ?>[/code]on my page I have listcontent.php [code]<? include("../include/adminfunctions.php"); $fa =$_POST["fa"]; $id=$_POST["ItemId"]; geteditcontent("webcontent_regions","$id"); ?> <form name="example" id="example" method="post" action="../include/formsubmit.php?mode=content"> <input type="hidden" name="id" value="<? echo $id; ?>" /> <div align="left"> <table width="100%" border="0"> <tr> <td colspan="2" class="ListTableHeader" ><div align="center"><strong>Update Content</strong></div></td> </tr> <tr> <td class="Results" >Region Id:<? echo $id ?> <div align="left"></div> Region Description:<? echo $description ?> <div align="left"></div></td> </tr> <tr> <td> <textarea id="textarea1" name="test1" style="height: 100%; width: 100%;"><? echo $content ?> </textarea></td> </tr> </table> <input type="submit" name="Submit" value="Save Content"> <input type="button" name="btnCancel" value="Cancel" onclick="JavaScript:history.back()" > </div> </form>[/code]
-
My question is how do I get this to work using classes? I would like to learn how to use classes in PHP but I have no clue on how to get data back from a function in a class.
-
OK what I want to do is create a class that I can include on my other pages, call function from that class and return those resluts to the calling page. Any references to online materials on php classes would be great! My code should be something like; class_db.php class db(){ //Top level db connection var $db_name="db_name"; var $db_host="localhost"; var $db_user="db_user"; var $db_password="db_password"; $connection=mysql_connect($db_host,$db_user,$db_password) or die("I Couldn't connect"); $db=mysql_select_db($db_name,$connection) or die("I Couldn't select your database"); function getlist($inTable){ $sql="select * from $inTable order by ID"; $result=mysql_query($sql,$connection) or die(mysql_error()); //I need to return my $result here but don't know how } function updatelist($inTable,$description,$id){ $sql="update $inTable set description='$description' where regionId='$id'"; $result=mysql_query($sql,$connection) or die(mysql_error()); //I need to return my $result here but don't know how } function deletelist($inTable,$id){ $sql="delete from $inTable where regionId='$id'"; $result=mysql_query($sql,$connection) or die(mysql_error()); //I need to return my $result here but don't know how } //also need to close any dbconnection but don't know how //end the class } page.php include("class_db.php"); ....... <? getlist(my_table) $i = 0; //Need to return the output from the function so the following line can use it. while($row=mysql_fetch_array($result)) { $i++; echo "<tr class=\"d".($i & 1)."\">"; ?> ....other HTML code Is Here........ <? } ?>
-
I was thinking would it not be better to do something like <?php function dblistvalues($inTable){ $db_name="db_name";// Database name $db_host="localhost"; $db_user="db_user"; $db_password="db_password"; $table=$inTable; $connection=mysql_connect($db_host,$db_user,$db_password) or die("I Couldn't connect"); $db=mysql_select_db($db_name,$connection) or die("I Couldn't select your database"); $sql="select * from $table order by ID"; $result=mysql_query($sql,$connection) or die(mysql_error()); } ?> Then on the other page call the function dblistvalues("my_table"); while($row=mysql_fetch_array($result)) {.......... but how would I return the value back to this page using this example?
-
I have a function named dblistvalues values in my connection.php file [code]<? function dblistvalues($inTable){ $db_name="db_name";// Database name $db_host="localhost"; $db_user="db_user"; $db_password="db_password"; $table="$inTable"; // Table name $connection=mysql_connect($db_host,$db_user,$db_password) or die("I Couldn't connect"); $db=mysql_select_db($db_name,$connection) or die("I Couldn't select your database"); } ?>[/code] I then call this function in another file called get_list.php my code snippet is [code]<? dblistvalues(my_table); $sql="select * from $table order by ID"; $result=mysql_query($sql,$connection) or die(mysql_error()); while($row=mysql_fetch_array($result)) { ?> [/code] The error I get is Warning: mysql_query(): supplied argument is not a valid MySQL-Link resource if I do the same code in the function but remove the function part it works fine. But I want to reuse the code for seperate tables that is why I put it into a function so I can pass table names to it.