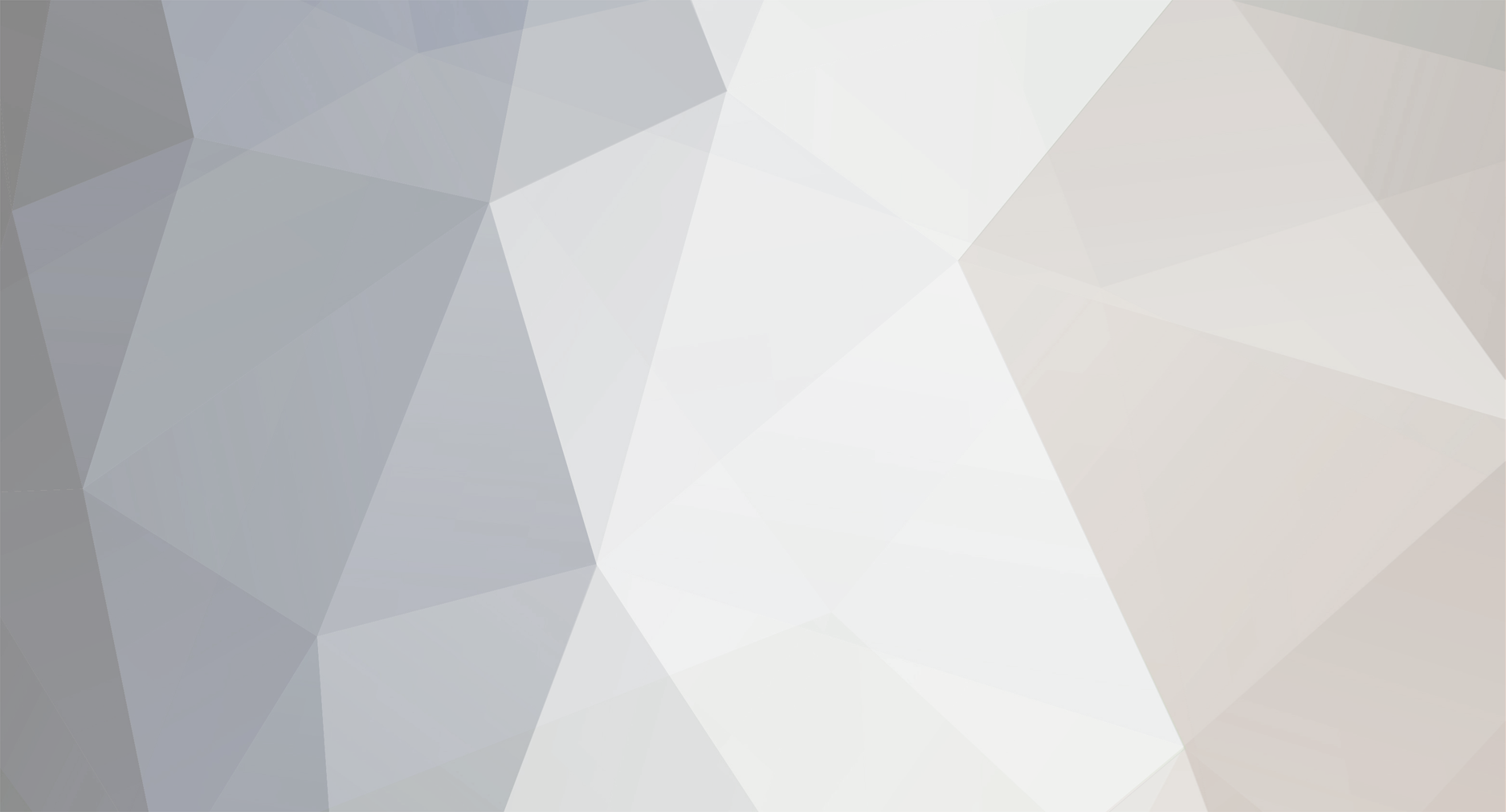
john010117
Members-
Posts
492 -
Joined
-
Last visited
Never
Everything posted by john010117
-
How to make Fields show up as HTML tables? But with more than 1 row.
john010117 replied to !!!!!'s topic in PHP Coding Help
Make a table, and put a variable in each cell. -
If I get what you mean, put the form(s) and the PHP processing script in a same file (like I do for my admin panel). So, in the PHP script, check to make sure that the form was submitted before processing it.
-
Use htmlentities() when filtering the user input.
-
No error messages for the most part. It works fine.
-
Please test my portal syetm for errors
john010117 replied to miniport_owner's topic in Beta Test Your Stuff!
Right after submitting the registration form: No e-mail. You could improve the page layout ALOT. -
Letting users download w/o showing the full path to the file
john010117 replied to john010117's topic in Application Design
Right. Man, I learn something new everyday (in this case, readfile() ). Thanks to both of you. I'll post again if I have any questions. -
You have a nice sense of humor. But don't look at me. I didn't submit that.
-
mkdir() will suit your needs.
-
return You can use variables after that.
-
First, you'll want to know if the username and password they've provided matches against the records in the database. After doing that, you start a session (name based on their user_id or whatever). Ex: <?php session_start(); $username = $_POST['username']; $password = $_POST['password']; // Assuming that you have hashed the password in the database $hashed_pass = md5($password); // Check it against the database $query = mysql_query("SELECT * FROM users WHERE username = '$username' AND password = '$hashed_pass'") OR DIE (mysql_error()); // If they match, start a session if(mysql_num_rows($query)>0) { while($row = mysql_fetch_assoc($query); extract($row); // Assuming that you have a unique id number for each user (named user_id) $_SESSION['uid'] = $user_id; } } else { return false; } ?>
-
... but it's not reliable, as stated in the manual. Try using sessions instead. First page: <?php session_start(); $_SESSION['page_refer'] = $_SERVER['PHP_SELF']; ?> Second page: <?php session_start(); if(!isset($_SESSION['page_refer'])) { return false; } else { //continue with your code } ?>
-
That's the biggest possibility. If you don't want the hassle of turning on php every time you start your PC, just set it to automatically start every time you start your computer.
-
I have a quick question. I don't want my users to know the full path to a file that they're downloading. I instead want to display them as "download.php?file=whatever.zip", and let users download it when they click that link. How would I go about doing that? I know a database is involved (to store the full path to a file), but how would I let them download it when they visit that link?
-
You could get started here. I'm glad that you're switching over to CSS.
-
Do you mean physically print it, or save the data somewhere?
-
You should have posted this in PHP help. If you look at the descriptions on the front page, you'll know where to post.
-
looking for hosting - Paid - CPanel - 1 month only!
john010117 replied to anybody99's topic in HTML Help
Wrong section. -
Right. I understand what you mean. I've seen several forums not have mods at all because they're afraid of the time when it's time to upgrade.
-
Use CSS instead of HTML tables... it's what I just said.
-
One thing that I say to everyone nowadays is: Do not use HTML tables for a layout!!! Use CSS. With CSS, you could make your design way better. But ultimately, it's your choice. I just hope you make a good choice.
-
Fine. One link (pictures) doesn't work. PS: I'm glad that you've accepted my quote submission (by Albert Einstein).
-
Your feedback form doesn't work.
-
For one, you can get started on mysql_real_escape_string(). But make sure to implement other features.
-
All you need is mail(). But be make sure to add tons of security features, so there won't be any spammers.