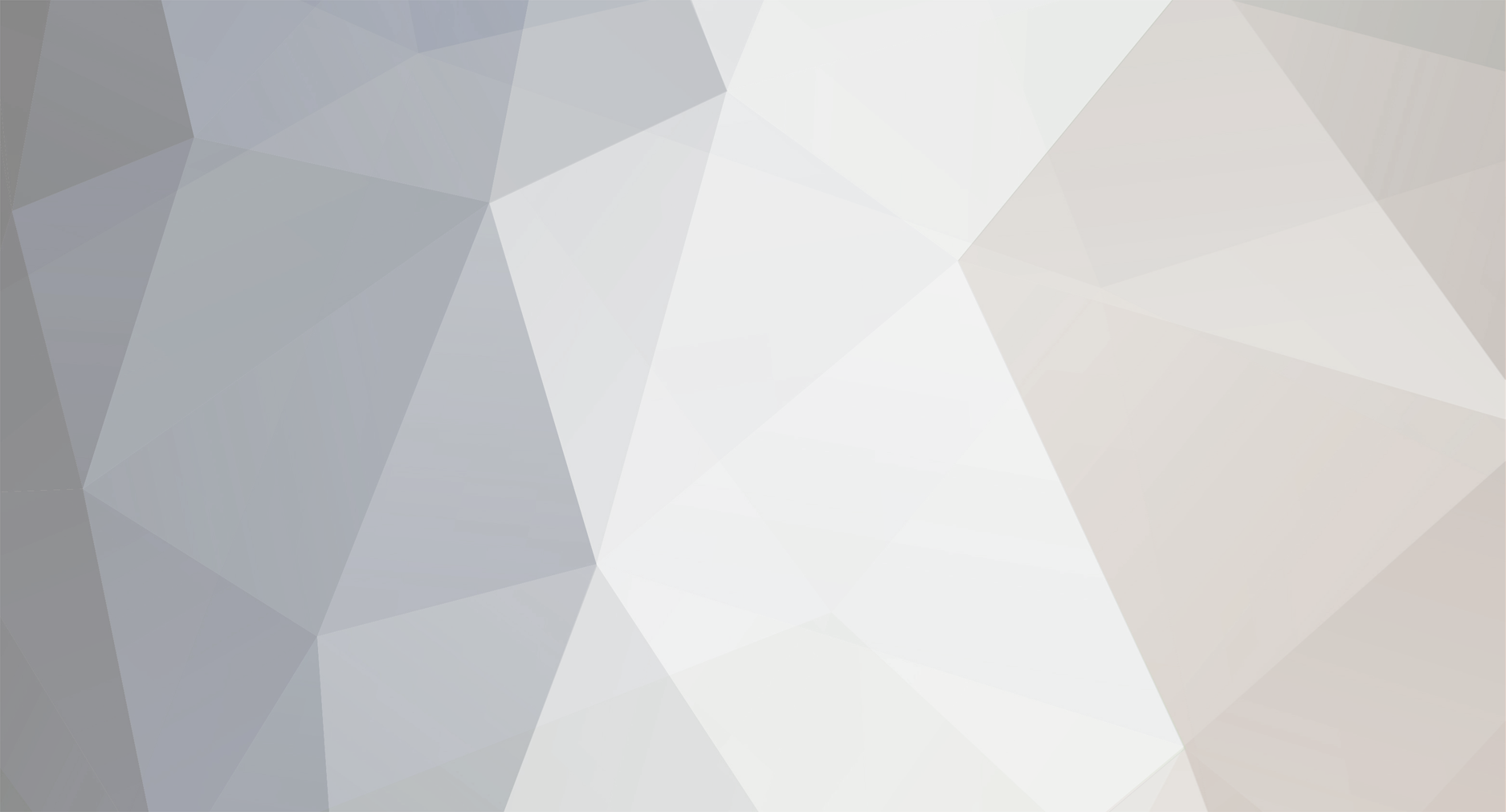
CrazeD
Members-
Posts
136 -
Joined
-
Last visited
Never
Everything posted by CrazeD
-
I'm sorry, I think I mis-read what you wanted to do. Do you just have a few videos that you want to hard-code? I was thinking you were trying to make a youtube-like site, where people would upload videos. If you just want to hard-code a few videos based on the vid_id that's pretty easy. Just use a conditional. For example: <?php switch ($_GET['vid']) { case 001: // Execute your code if test.php?vid=001 break; case 002: // Execute your code if test.php?vid=002 break; } ?> If that's not what you wanted then nevermind...
-
You could use a MySQL table for this. You could have a row called vid_id, and then a row called description and a row called location, for example. Say your vid_id is 001, description is blah blah blah, and the location is www.somewhere.com/media/vid_001.swf You could do something like this: <?php $sql = "SELECT * FROM your_table_name WHERE vid_id={$_GET['vid']} LIMIT 1"; if ($result = mysql_query ($sql)) { $row = mysql_fetch_array ($result); print '<object width="550" height="400"> <param name="movie" value="'.$row['location'].'"> <embed src="'.$row['location'].'" width="550" height="400"> </embed> </object>'; } else { // Query failed print '<p>Error: <b>'.mysql_error().'</b></p>'; } ?>
-
Okay I tried that and that didn't work either. Just says invalid file. I had it as image/jpeg at first, according to this it should work: http://www.phpfreaks.com/mimetypes.php
-
Pay attention to what your errors say. It tells you it's a syntax error. That means it has something to do with quotations, semi colons, commas, etc.
-
Anyone? I'd like to get rollin with this.
-
I'm working on an image gallery. I've never done this sort of thing before with uploads and whatnot, so I'm working the bugs out of my upload script. I can't seem to make it upload a .jpeg extension. What gives? <?php if (isset ($_POST['submit'])) { if ($_FILES['file']['type'] == "image/gif") { $type = ".gif"; } elseif ($_FILES['file']['type'] == "image/jpeg") { $type = ".jpg"; } $rand = rand(1,10000000); if (($_FILES["file"]["type"] == "image/gif") || ($_FILES["file"]["type"] == "image/djpeg") && ($_FILES["file"]["size"] < 2000000)) { if ($_FILES["file"]["error"] > 0) { echo "Return Code: " . $_FILES["file"]["error"] . "<br />"; } else { echo "Upload: " . $_FILES["file"]["name"] . "<br />"; echo "Type: " . $_FILES["file"]["type"] . "<br />"; echo "Size: " . ($_FILES["file"]["size"] / 1024) . " Kb<br />"; echo "Temp file: " . $_FILES["file"]["tmp_name"] . "<br />"; if (file_exists("uploads/".$rand."" . $_FILES["name"])) { echo $_FILES['file']["name"] . " already exists. "; } else { move_uploaded_file($_FILES["file"]["tmp_name"], 'uploads/'.$rand.''.$type.''); echo "Stored in: " . "upload/" . $_FILES["file"]["name"]; print '<p>'.$type.'</p>'; } } } else { echo "Invalid file"; } } else { ?> <form action="gallery.php" enctype="multipart/form-data" method="post"> <input type="hidden" name="MAX_FILE_SIZE" value="30000" /> <input type="file" name="file" /><br /> <input type="submit" name="submit" value="Upload" /> </form> <?php } ?>
-
Instead just put your regular HTML into the PHP file.
-
Yes... I have figured that much out on my own.... How is it he can see images from one part of the FTP and not the other? That doesn't make any sense at all.
-
Everyone else can see the site just fine.
-
Hi, a clan member can't seem to view our clan site?! He can view the site, but none of the images show up. It's like he can't see any image from that part of the FTP, but in a different directory he can. For example, "root/forum/pic.jpg" he can see, but "root/ad/pic.jpg" he can not see. He's tried both Firefox and Internet Explorer, tried clearing cache/cookies/temp internet files, etc, but nothing seems to work. http://bioxion.com/ad is my site. Any ideas?
-
No no no, you're not listening. It goes into the table as a one-line string, it doesn't have breaks in it.
-
No, I'm not talking spacing between each row. Like say you have a textarea, and you enter: "blah blah blah blah blah blah blah blah blah blah" It goes into the MySQL table like this: "blah blah blah blah blah blah blah blah blah blah" Is there any way to change that?
-
Is there a way to make the contents of my MySQL table the same as the way it went in? For example, if I insert: 1 2 3 4 5 6 into my MySQL table, it comes back out as: 1 2 3 4 5 6 Is there a way to fix that? My field type is TEXT, if that matters. Thanks.
-
[SOLVED] Trying to make a forum, need help with listing MySQL info
CrazeD replied to CrazeD's topic in PHP Coding Help
I was going to try that, but I heard it's not good to run a query inside of a query. -
I know, the dreaded 500 error... a tidbit to read
CrazeD replied to siwelis's topic in PHP Coding Help
Maybe time for a different host? -
I'm trying to code a simple little forum. It seems easy enough, but I'm having trouble displaying the index. Basically, I have to: - Select from forums_cat (to list the category) - Select from forums_topic (to list the topics for that category) - Repeat How do I make it repeat? It lists the categories as a whole block and then stuffs the topics under that as a whole block. I suspect it's because I'm using the while() loop on my query, and if I LIMIT 1 on the category query everything is fine. Code: <?php if (empty ($_GET['action'])) { if (empty ($_GET['cat'])) { print '<table border="1" cellpadding="3" cellspacing="3" class="tutorials_table" width="90%"> <tr> <td width="10%" align="center" bgcolor="#000000"><b>Status</b></td> <td width="66%" align="center" bgcolor="#000000"><b>Forum</b></td> <td width="12%" align="center" bgcolor="#000000"><b>Threads</b></td> <td width="12%" align="center" bgcolor="#000000"><b>Posts</b></td> </tr> </table>'; $sql = "SELECT * FROM forums_cat"; if ($r = mysql_query ($sql)) { while ($row = mysql_fetch_array ($r)) { print ' <table border="1" class="tutorials_table" width="90%"> <tr> <td align="left" bgcolor="#000000"><b><font size="4"<a href="index.php?site=forums&cat='. $row['cat_id'] .'">'. $row['name'] .'</a></font></b></td> </tr> </table> '; $cat_id = $row['cat_id']; } } else { print mysql_error(); } print '<table border="1" class="tutorials_table" width="90%">'; $topicsql = "SELECT * FROM forums_topic WHERE cat_id=$cat_id"; if ($r = mysql_query ($topicsql)) { while ($row = mysql_fetch_array ($r)) { print ' <tr> <td width="10%" align="center" bgcolor="#202020">'. $row['status'] .'</td> <td width="66%" align="left" bgcolor="#202020"><font size="3"><b><a href="index.php?site=forums&cat='. $row['cat_id'] .'&tid='. $row['topic_id'] .'">'. $row['name'] .'</a></b></font><br /> <font size="2">'. $row['description'] .'</font></td> <td width="12%" align="center" bgcolor="#202020">5304</td> <td width="12%" align="center" bgcolor="#202020">30405</td> </tr> '; } } else { print mysql_error(); } print '</table>'; } } ?> Thanks.
-
I get this error: Here is my code: <?php include('_mysql.php'); function gettemplate($template,$endung="html") { $templatefolder = "templates"; return str_replace("\"","\\\"",implode("",file($templatefolder."/".$template.".".$endung))); } if (isset ($_POST['submit'])) { $username = $_POST['username']; $pass = $_POST['password']; $enc_pass = md5($pass); if($username != "" && $password != ""){ $query = "SELECT * FROM users WHERE username='$username' AND user_password='$enc_pass'"; $gay=mysql_query($query); $num=mysql_num_rows($gay); if($num > 0) { $_SESSION['username'] = $username; $_SESSION['loggedin'] = 1; $_SESSION['user_level'] = @mysql_result($result, 0, user_level); eval ("\$login = \"".gettemplate("loginsuccessful")."\";"); } else { // Did not much eval ("\$loginerror = \"".gettemplate("login_enterunamepwd")."\";"); } } else { // Enter both a username and password eval ("\$loginerror = \"".gettemplate("login_enterunamepwd")."\";"); } } else { // Submit not entered header ('Location: index.php'); } mysql_close(); ?> This is a login script. This worked for me before, but now I get this error. What is the problem? Thanks. EDIT: Nevermind I got it. I spent all night trying to fix it and then I post for help and right as I do I figure it out. :/
-
What is this called? $var->ACTION(); I see this a lot, and I'm not sure what it means or if there is a name for what it's doing. I'd like to learn about this. Thanks.
-
Anyone? ......
-
Anyone? I'd really like to get this fixed.
-
MM, that almost works. Now when I make the page smaller, it makes the blocks drop down. Also, it's not centered. I appreciate the help dude.
-
Um... www.pr0n.bioxion.com/index.php Don't think that worked either? html { height: 100%; } body { text-align: center; background: #4d4d4d; } .banner { position: relative; top:20px; left:50px; right:50px; float: left } .container { text-align: left; margin-left: auto; margin-right: auto; } .left { position: relative; left:113px; top:190px; text-align: center; float: left } .right { position: relative; right:113px; top:190px; float: left } .center { position: relative; left:260px; right:260px; top:190px; float: left } .footer { position: relative; bottom: 20px; } a {text-decoration: none} a:link { color:#d0a700 } a:visited { color:#d0a700 } a:active { color:#d0a700 } a:hover { color:#767676 } The rest is like this: <div class="container"> <div class="header"> header stuff </div> <div class="left"> left stuff </div> <div class="right"> right stuff </div> <div class="center"> center stuff </div> </div> What's wrong?
-
Javascript would probably be better for that than CSS.
-
So then... something like this? <div class="container"> <div class="left"> blah blah </div> <div class="center"> blah blah </div> <div class="right"> blah blah </div> </div> Like that?
-
Wow..... Actually that's called in my header. This page didn't have my header because I was testing something. lol... I'm an idiot, thank you.