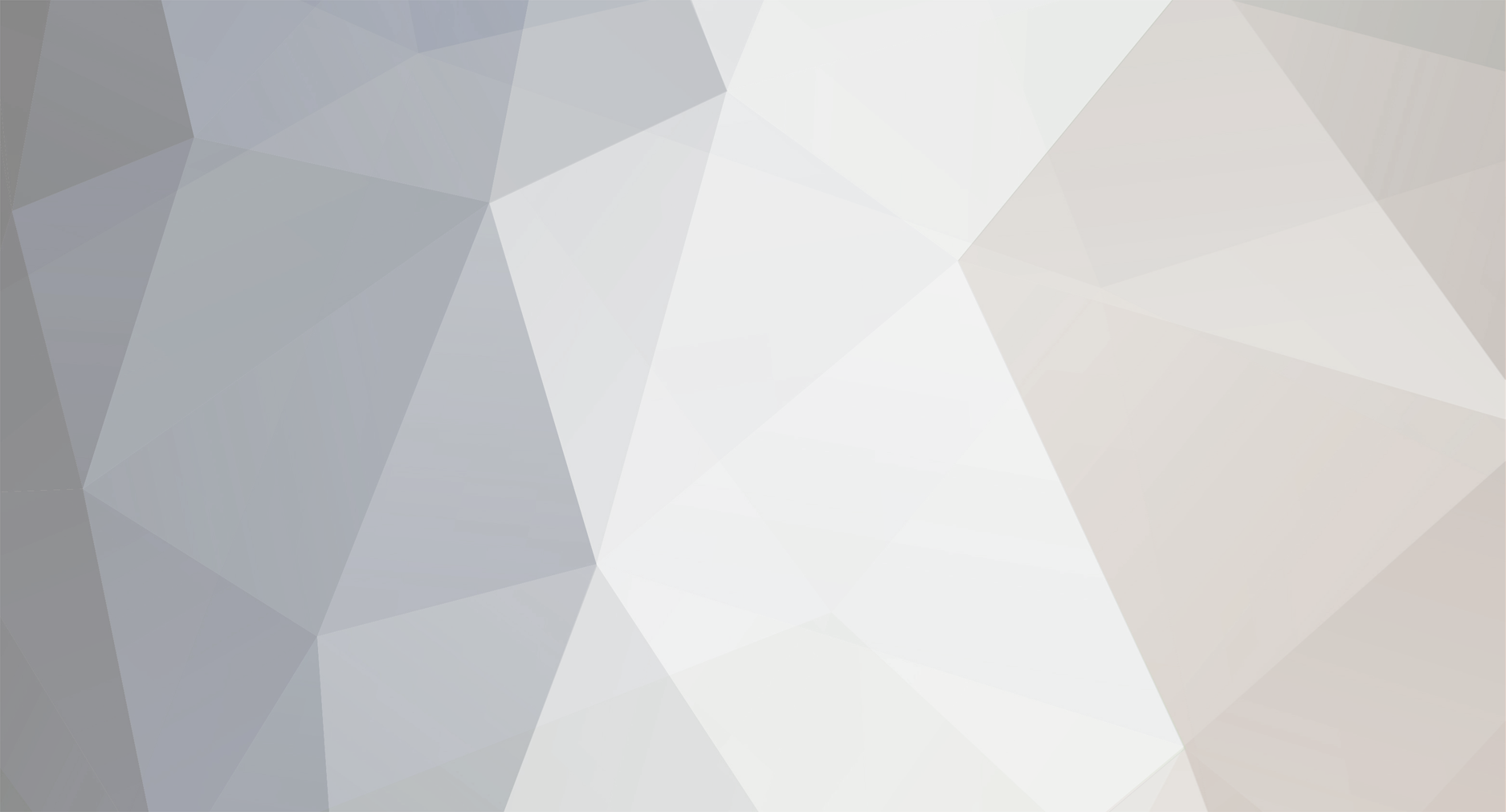
The14thGOD
Members-
Posts
256 -
Joined
-
Last visited
Never
Everything posted by The14thGOD
-
I'm new to the whole OOP thing so please excuse the sloppiness/potential bad code. I've gone through a lot of tutorials and a cookbook I have and have what I thought was a good understanding of it (as much as I can get from reading about it at least). Now I'm trying to put it into practice with a side project. Basically I'm trying to check if a user already exists, here's the db class: (I'm going to assume this is the wrong way to do this so any help (books/links) greatly appreciated) <?php class database{ private $_db_host = DB_HOST; private $_db_name = DB_NAME; private $_db_user = DB_USER; private $_db_pass = DB_PASS; private $_db_link = ''; public function __construct(){ /* *Create a mysqli connection object within the db object *To reference the mysqli functions you have to call: $this->_db_link as if it was $mysqli (outside of a class) */ $this->_db_link = new mysqli($this->_db_host,$this->_db_user,$this->_db_pass,$this->_db_name); if(mysqli_connect_errno()){ die('Could not connect: '.mysqli_connect_error()); } } public function __destruct(){ $this->_db_link->close(); } //this function works, I get the full list public function list_types(){ if($results = $this->_db_link->query('SELECT type FROM types ORDER BY type ASC')){ while($row = $results->fetch_assoc()){ echo $row['type'].'<br />'; } //free result set $results->close(); } } } Here's my incompelte user class: <?php class user{ private $username = ''; public function check_user_exists($user){ if($stmt = $db->_db_link->prepare("SELECT username FROM users WHERE username='?'")){ $stmt->bind_param('s',$username); $username = $user; $stmt->execute(); $stmt->num_rows == 0 ? $chk = true : $chk = false; } return $chk; } } Code that calls user class (db class is called in the configuration, I pretty much always need a db connection, but again, I'm sure this is bad) <?php $db = new database(); //called in a configuration file $user = new user(); if($user->check_user_exists($username)){ echo 'user does not exist'; exit(0); } The error: Fatal error: Call to a member function prepare() on a non-object which is $db->_db_link->prepare() but $db->_db_link->query() will work... It's weird to me that the db connection is inside another object, is this right? Any and all help would be greatly appreciated (google is not helping me find a mysqli prepared oop in class example). No one I work with/know has a good understanding of what OOP is so I'm stuck trying to learn it on my own. Thanks, Justin edit #1: at first i thought it was the fact $_db_link = private, but i changed it to public and it had no effect
-
solved with: http://php.net/manual/en/function.usort.php
-
Is there a way to sort an array of objects by the objects variable name or a property within the object? I'm new to OOP so sorry if this is dumb. <?php //example code $members = array(); $member_name = 'John Doe'; $member_last_name = 'Doe'; $_GET['filter'] == 'alpha' ? $array_safe_name = str_replace(' ','_',$member_last_name) : $array_safe_name = str_replace(' ','_',$member_name); $array_safe_name = str_replace('.','',$array_safe_name); $array_safe_name = str_replace("'",'',$array_safe_name); ${$array_safe_name} = new njl_member($member_name,$member_title,$member_image,$member_phone,get_permalink()); array_push($members,${$array_safe_name}); foreach($members as $member){ //do stuff } ?> ksort/asort obviously wont work (as far as I know them....) So if I have 'John Doe', 'Joe Adams', 'Jane Smith', I'd like the foreach to display them Joe Adams John Doe Jane Smith Thanks for any and all help, Justin
-
thanks for those links!
-
Would you recommend just a txt file xml or does it not really matter in that case? The txt file would probably be smallest, but harder to present/stylize. txt file I would have to come up with some system for new lines etc. I'm not sure if there is a standard out there. Is there a better option out there than fopen() etc? Just seems like that may get ugly if lots of people are accessing different files at once on the server.
-
I have a rather large project I'm investigating and starting to put together the database. I'm looking for the best way to store chat logs. I have chat systems I'm looking to store. Possibly a third but that one will probably need a more unique solution and is in phase two. The first is much like a chat room, 3-8 people at a time. The other will be only two people like Google Chat. I started looking at SQLite but that looks like an alternative thing to MySQL? I have enough to learn so I'd rather not add another thing to this project. I want to use MySQL. I'm not sure how to do the chat thing yet either (once I know how I'm storing the chats I will have more of a direction on this). This project has the potential for a very large userbase so I need something fast. At first I was thinking about doing flat files but that seems like its going to take a toll on the drive. But I'm not sure of a way to do it via database that would be fast. I'm not exactly an expert on database/server performance so any help would be greatly appreciated. If you need any clarification on some things let me know and if I can answer I will. Thanks, Justin
-
help clear my understand my understanding of...
The14thGOD replied to The14thGOD's topic in MySQL Help
Ah, ok. Thanks, I got it now. -
NULL vs NOT NULL I know it's been asked a billion+1 times but I'm more of trying to clear up my understanding of it.... Through countless searches/reading/books, what I seem to understand is: NULL is on a field that can be left blank, 0 (if int), or NULL; NOT NULL is on a field that can not be blank, 0 (if int) or NULL. Yet in my databases all my fields are set to NOT NULL and I have blank fields... (this is where I'm confused) Shouldn't I get an error saying that the field can not be NULL? I have a big project I'm doing so I'm trying to make it so my db is running smoothly and one of the suggestions was make sure all fields that can be are NOT NULL. (I'm going to bed shortly so I may not respond till the morning...) Thanks for any and all help. Justin
-
Rewrite rules not working on goDaddy server
The14thGOD replied to The14thGOD's topic in Apache HTTP Server
godaddys apache version is 1.3.33 where all the other servers i've tested on are 2.x, looking into possible mod_rewrite issues, if anyone has any info please let me know! -
Trying to get this to work on godaddy but it's not, any ideas? I've done a lot of googleing but all the options (turn multi-view off, rewrite base, follow syslinks) have failed. Anyone have any experience with this? This is just a CMS I made. It works on several other servers, so I'm a little lost as to why it's not working. I did do the mod_rewrite is enabled test and it worked so no idea whats the issue. I get the 500 internal server error. Here's the code: RewriteEngine On RewriteRule ^feed.xml feed.php [L] RewriteRule ^([a-z0-9-_]+)$ page.php?theurl=$1 [L] RewriteRule ^([a-z0-9-_]+)/([a-z0-9-_]+)$ page.php?theurl=$1/$2 [L] RewriteRule ^([a-z0-9-_]+)/([a-z0-9-_]+)/([a-z0-9-_]+)$ page.php?theurl=$1/$2/$3 [L] Thanks for any and all help. Justin p.s. if anyone who knows mod_rewrite better than me could help me condense that into one line, that would be amazing. I tried once before but failed and haven't tried since. Once this project gets launched it's one of my next goals.
-
For some reason our host can not point our subdomain to a folder (even though every other host I've had does it just fine...) but I don't know server set up well enough to tell them what they are missing. Basically I need http://m.domain.com to point (not re-direct) to http://domain.com/m/ I also need to get whatever page they are accessing. I've tried finding htaccess rules with google but none of them seem to be working. Probably user error. One of the most promising ones i found: RewriteEngine On RewriteCond %{HTTP_HOST} ^m\.domain\.com/(.*)$ RewriteRule ^(.*)$ /m/$1 [L] ##or RewriteCond %{HTTP_HOST} m\.domain\.com/^(.*) RewriteRule ^(.*)$ http://domain.com/%1/$1 [R=301,L] I don't understand these all that well so I apologize for anything stupid. Thanks for any and all help, Justin
-
I'm not sure how it logs in to phpmyadmin, this server is on visi, but this user has all permissions. Not sure what an update trigger is on the table...I'd assume no, I don't typically mess with sql other than data inserting etc. It's all the code that is triggered in the event it is 'published.' It's in an if statement, but it runs as testing has proved. Double checked code and there's nothing else that could set it back to true without conditions are met (also tested to see if conditions were being set off on accident, and they were not) Ya..I'm with everyone at the moment. No idea what could be going wrong...
-
varchar, i know its better to use boolean in this case, but i just like reading true/false, I'm not worried about db performance for this site =D
-
lol I have no idea dude, this is so simple it's stupid, at first I was thinking it had something to do with me not looking at this for a while, but everyone is scratching their heads... The new code didn't produce anything new, just went through like normal.
-
All the errors I get are normal from what I can tell: Notice: import_request_variables() [function.import-request-variables]: No prefix specified - possible security hazard in /path/to/press.php on line 5 Notice: Undefined variable: date in /path/to/press.php on line 9 Notice: Undefined offset: 1 in /path/to/press.php on line 10 Notice: Undefined offset: 2 in /path/to/press.php on line 10 Notice: Undefined variable: send in /path/to/press.php on line 11 Notice: Undefined variable: edit in /vpath/to/press.php on line 44
-
Nothing In the database, draft is still set to 'true' I'm unfamiliar with the 'or die()' but I even put exit(); under it but I just got a blank page. Does die() stop the whole script?
-
Argh sorry, I was troubleshooting it with someone while making this post, apparently I left that out too. I tested in phpMyAdmin and it worked in there just fine.
-
Didn't work, plus it works in all the other queries as is :/ that's the only reason I didn't try that method. It makes no sense, not sure what else I can do.. oh and just so there's no question about it, I have verified that the 1st query works.
-
sorry, the 2nd one doesn't run.
-
I have a page that updates a press release and it runs one query but not the other. No errors as far as the query goes (some 'undefined variables' in other parts of the script). Here's the code: <?php $updateQuery = "UPDATE website AS w INNER JOIN preview AS p ON (w.uid = p.uid) SET w.navtitle=p.navtitle, w.title=p.title, w.keywords=p.keywords, w.description=p.description, w.url=p.url, w.body=p.body, w.the_date=p.the_date, w.status=p.status WHERE w.uid = '$_GET[uid]'"; mysql_query($updateQuery); $updateQuery2 = "UPDATE preview SET draft='false' WHERE uid='$_GET[uid]'"; mysql_query($updateQuery2); echo $updateQuery2; echo mysql_error(); exit(); header("Location: $_SERVER[php_SELF]?edit=true&uid=$_GET[uid]&edit_suc=true"); exit(0); ?> Query: UPDATE preview SET draft='false' WHERE uid='5pjm94ap98' I have no idea what it could be. Any help is appreciated, Justin
-
I'm trying to update an RSS feed/xml file with fopen etc but it's not working. The file doesn't even get touched, I sent myself an email with the string and that works. I've changed the path multiple times to try and target it but nothing seems to work. Here's the code: <?php function write_xml_feed() { $xml = '<?xml version="1.0" encoding="utf-8"?> <rss xmlns:atom="http://www.w3.org/2005/Atom" version="2.0"> <channel> <title>hidden</title> <link>http://www.me.com/</link> <description> </description> <generator>Feeder 1.4.10(222) http://reinventedsoftware.com/feeder/</generator> <docs>hidden</docs> <language>en</language> <pubDate>'.date('l, d F Y h:i:s').' -0600</pubDate> <lastBuildDate>'.date('l, d F Y h:i:s').' -0600</lastBuildDate>'; //Get items from database that are press releases (ie date != 0000-00-00 $xmlquery = "SELECT title,url,body,DATE_FORMAT(the_date, '%W, %d %M %Y %l:%i:%s') AS display_date FROM website WHERE the_date != 0000-00-00 ORDER BY the_date DESC"; $xmlresults = mysql_query($xmlquery); while($xmlrow = mysql_fetch_assoc($xmlresults)){ $xml.= '<item>'; $xml.= '<title>'.$xmlrow['title'].'</title>'; $xml.= '<link>http://www.me.com/'.$xmlrow['url'].'</link>'; $xml.= '<description><![CDATA['; $xml.= $xmlrow['body']; $xml.= ']]></description>'; $xml.= '<pubDate>'.$xmlrow['display_date'].' -0600</pubDate>'; $xml.= '<guid isPermaLink="false">hidden</guid>'; $xml.= '</item>'; } //closing xml $xml.= '</channel></rss>'; mail('justinh@me.com','stupid xml',$xml,"From: justinh@me.com"); //open feed file and overwrite it $handle = fopen('http://me.com/feed.xml','w+'); fwrite($handle,$xml); fclose($handle); return true; } ?> Anyone got any ideas? Thanks for any and all help. Justin P.S. The only other idea i had is that $xml is HUGE, is there an issue/limit with fwrite? (Trying to find the answer via php.net/google)
-
Sorry, not sure where to post this question, this seemed like the best choice. I have made a CMS for a client and they need to be able to publish/edit press releases. The issue comes when whatever they publish in the press releases category, it needs to also update an XML file which is a RSS feed. I was not involved in the making of the site (it's a few years old) and anyone who knows anything about it is gone. So I'm looking to find out what is the best way to update this file. The 2 best options I have come up with are: 1. Anytime a new press release is made or a press release is edited, create a new XML file from the information in the database. 2. Add the new press release to the file when it is added. If a press release is edited, find out it's row number (stored by date) and use something like SimpleXML to replace the node/delete the node or something of that nature. There seems to be only 1 or 2 press releases in a given month. If anyone has any good links for the latter method can you please post them? I haven't found much on SimpleXML and manipulating of nodes. I addChild etc... Sorry I am not fluent with XML..yet.. Thanks for any and all help, Justin
-
Solved it, for future reference, if anyone cares. You need to specify the path for Safari, to at least the folder (havn't tried doing just '/'). ie: <?php setcookie('name','value',time()+3600*24*90,'/path/to/folder/'); ?>
-
Safari isnt reading the cookie through php. But if I go to prefs>security>show cookies, it's there. Anyone got any ideas? Code is below, works in FF. <?php if(isset($_SESSION['username'])){ $user_query = "SELECT * FROM challenge_users WHERE username=LOWER('".mysql_real_escape_string($_SESSION['username'])."') AND password='".mysql_real_escape_string($_SESSION['password'])."' LIMIT 1"; $user_result = mysql_query($user_query); if(mysql_num_rows($user_result) != 0){ $user_row = mysql_fetch_assoc($user_result); if(!isset($_COOKIE['cotw'])){ //set cookie to avoid doing double cookies //also set session incase user doesnt accept cookies setcookie("cotw","$row[username];$row[password];$row[name];$row[email]",time()+3600*24*90); } $loggedin = true; } }elseif(isset($_COOKIE['cotw'])){ $xplod = explode(';',$_COOKIE['cotw']); $user_query = "SELECT * FROM challenge_users WHERE username=LOWER('".mysql_real_escape_string($xplod[0])."') AND password='".mysql_real_escape_string($xplod[1])."' LIMIT 1"; echo $user_query; $user_result = mysql_query($user_query); if(mysql_num_rows($user_result) != 0){ $user_row = mysql_fetch_assoc($user_result); $_SESSION['username'] = $user_row['username']; $_SESSION['password'] = $user_row['password']; $_SESSION['name'] = $user_row['name']; $_SESSION['email'] = $user_row['email']; $loggedin = true; $test = true; } } ?> Thanks for any and all help. Justin
-
correction. I think sessions are working, just not the cookies