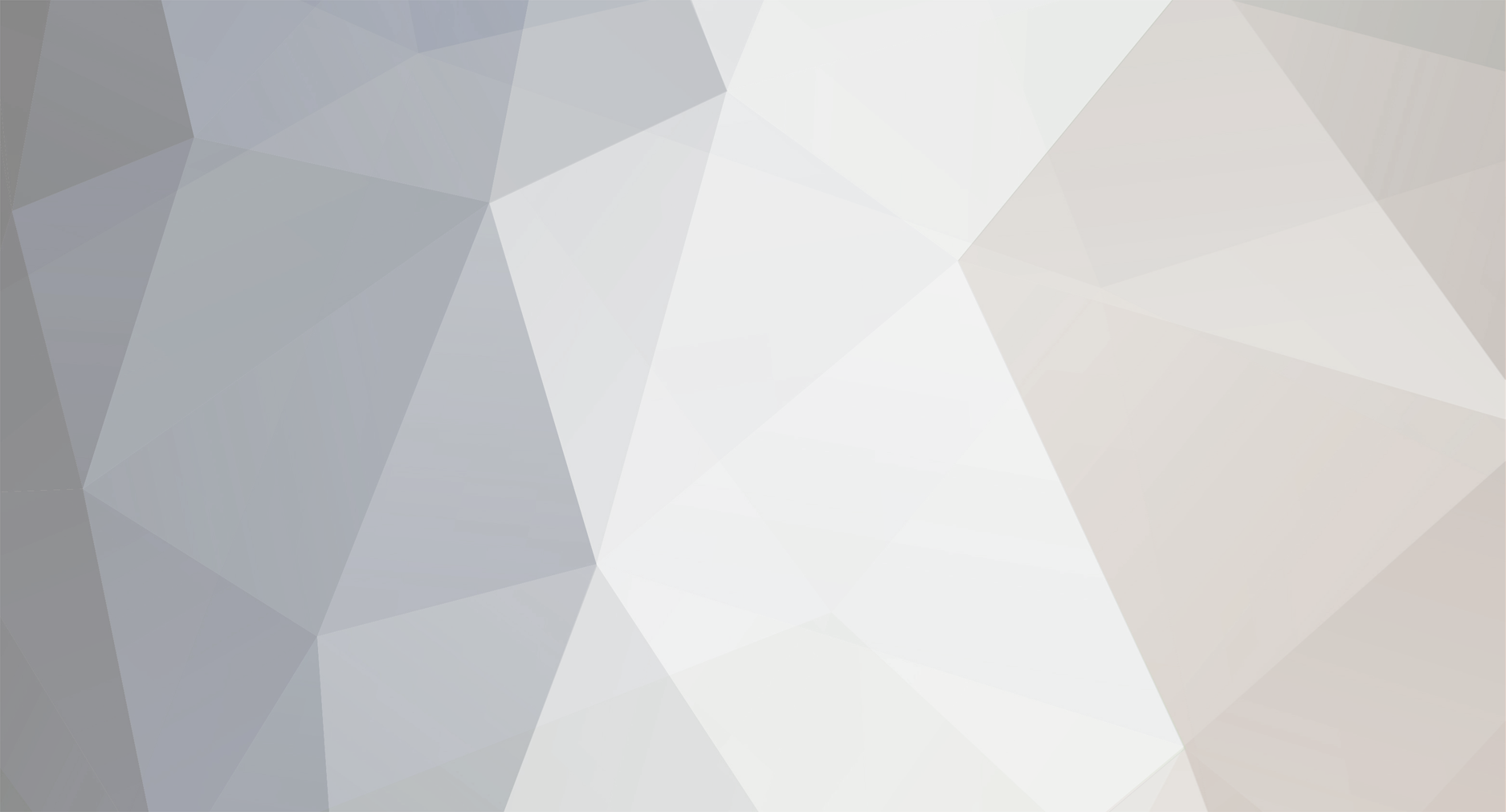
Boo-urns
-
Posts
144 -
Joined
-
Last visited
Never
Posts posted by Boo-urns
-
-
You definitely pointed me in the right direction. I don't recall what I changed that made it work probably a all of the above but its running now!
Thanks!
-
Yea i have echoed the request variable and it wasn't pulling but I checked up on display_errors and it was off so I'll go at it for a bit and hopefully i find the solution! Thanks for the help.
-
Another interesting thing that might help is if I send the variable back via json_encode
$arr = array ('errors'=>$error,'output'=>$output, 'numForms'=>$_REQUEST['numForms']); echo json_encode($arr);
It will display the correct number for numForms.
-
It says Off (on both local and live site)
-
I have a dropdown and when a user selects how many entries they want to add it calls a file to create a form and bring it back. Well it works great locally but not on a live server. Firebug is saying I'm getting a 500 error. (I've tried absolute and relative paths but it's not working.) If I copy the include file on that script page it will run hardcoded but isn't getting the variables. The data posts just fine but PHP isn't pulling the variables.
<?php $numForms = mysql_real_escape_string($_REQUEST['numForms']);
Is something screwed up in the php.ini file or something on the server? Any ideas? I'm stumped
-Corey
-
I wasn't sure if it was a php.ini setting or not.
The code runs great locally, I didn't change anything then I put it on a development server. If i do:
$var = $_REQUEST['var'];
That is giving me a 500 error. Maybe that will help?
-
I've been working locally creating some scripts, when I moved it to a development server the main scripts work. (i.e. if i post on that page) However If I am calling a file with AJAX and bringing info back it's not working. I believe it is something with the $_REQUEST['varname'].
The variable passes just fine but isn't getting pulled into a variable on the file I am calling with the request method. If i send the variable straight up as a request back in the json_encode it will show up.
Any ideas on what to change?
Thanks!
-Corey
-
Figured it out. My variables $fName etc...was the array name so it was confusing PHP I guess. It works now!
-
So you're just looking to have 5 not be a static variable? i.e. they can have 7 tickets or 100 tickets etc...?
What I do is I create a table for defaults and have a column for what you're going after. (ticketMax column = whatever maximum number)
Unless, you aren't going to reuse it somewhere else you could always create a variable up top for ticket max and input it in your code
<?php $ticketMax = 10; // or pull the maximum from the db if you do it that way. if($getnumber >= $ticketMax && $opentick['status'] == "Open"){ echo "<b><center>Please wait until one of your tickets is solved before creating another one.</center></b>"; }
I hope that is what you were asking.
-
Can you use bind_param and execute in a for loop? (With the bind outside of it)
<?php // $db is being extended from the mysqli class if($stmt = $db->prepare("INSERT INTO test (fName, lName) VALUES(?, ?)")) { $stmt->bind_param('ss', $fName, $lName); for($i = 1; $i <= $numDeclared; $i++) { // numDeclared is a hidden field to figure out how many forms there are on the page // i am starting on 1 on purpose. $fName = $fName[$i]; $lName = $lName[$i]; // Execute the prepared Statement $stmt->execute(); } // Close the statement $stmt->close(); }
It loops properly but is only inserting the first row (if i have multiple). Also is there a built in real_escape_string when preparing queries? (I'm doing it outside of it right now)
Thanks!
-Corey
-
Or use
unset($arrayName);
That is probably the preferred method to use.
-
Yea Azarian I'm with you on that I'm working on creating something very flexible for common thing programmed etc...
Best of luck man
-
I think you're definitely on the right track. I'm still lazy atm and haven't set it up this way yet.
For the $_GET['page'] I would create an array of possible values and do an if(in_array($_GET['page'], $array)) {
basically if you don't want someone to access a certain page.
-
also if you're not familiar with parsing text blocks use:
nl2br($yourParagraph); // creates the paragraphs instead of mashing everything together
-
Yea that's kind of what i thought make sure it's a standard naming convention. Thanks
-
Yea I could see that being a great way for that.
What if other developers are working on the same project want to use your __autoload func that you wrote up to include them?
Say I write my files class.animal.php and the other guy writes it animal.class.php and another guy writes is just animal.php Or should i just be like this is the way we name files? Any suggestions?
<?php function __autoload($className) { // granted to incorporate different ways to save files I will need to write more code $file = 'classes/class.'.$className.'.php'; include_once($file); } $test = new testAutoload(); // w/ autoload func it will pull file "classes/class.testAutoload.php" ?>
-
Yea I went to school for Multimedia but they didn't stress to much programming and/or require programming minus basic C++ and some Javascript / PHP / etc....but no OOP.
Yea mchl I agree a lot of the examples I have found are class Animal / class Dog extends Animal etc... I do the same as premiso for any programming create an outline of general functionality of what will be needed.
Would autoload be better use in a framework? I have been going over some autoload examples this morning but atm it seems better to just include the file myself.
-
Wow thanks for all the insights about OOP. Along with the links to some tutorials.
I've been through a few tutorials so far and have probably just touched the surface of OOP but want to dive much deeper so I can create some very reusable code in the future and possibly create a framework (in time). I'll most likely have more questions in the future but thanks for the push guys!
-
Some uses of oop are easy to comprehend. Class for Users / Common Validation etc... Would it be a good idea to extend the mysqli class? To create queries and to return the loaded results. Along with that to create it for ease of use in the future should I send it fields / table / WHERE info or would that not be a good idea?
I just need a push
-
Thanks for all the insights guys!! I just started doing OOP programming so its rough and which is why i asked about the abstract and interface. I'm shooting to create classes for everything that will be reused very often in new projects.
-
I've found some good posts here about both, but what I'm not seeing is a reason to use either. Unless there will be multiple developers on the project.
What do you use them for?
Thanks,
-Corey
-
From what you're saying make sure you change the second part of what you're matching to "songName LIKE" as currently it is artistName. That might be your problem.
I did test the code locally on a test table and it worked fine I just threw it variables for easier testing
i.e. LIKE '%$string1%'
Hopefully that helps.
-
So if I'm in a class and i want to use mysqli_query in that class I should use the procedural version and not the oop style since it is already in a class?
i.e. $link = mysqli_connect("localhost", "user", "password", "db");
-
So for my classes is the main change in php5 public/private function and use of extends, constructors...?
http://us.php.net/zend-engine-2.php
I am very new to OOP programming. Thanks for the guidance.
[SOLVED] Extending mysqli class not querying...
in PHP Coding Help
Posted
So my problem is the query. It's not querying the mysqli query function. I have errors being displayed but its not giving me any problems. What am I doing wrong?
-Corey