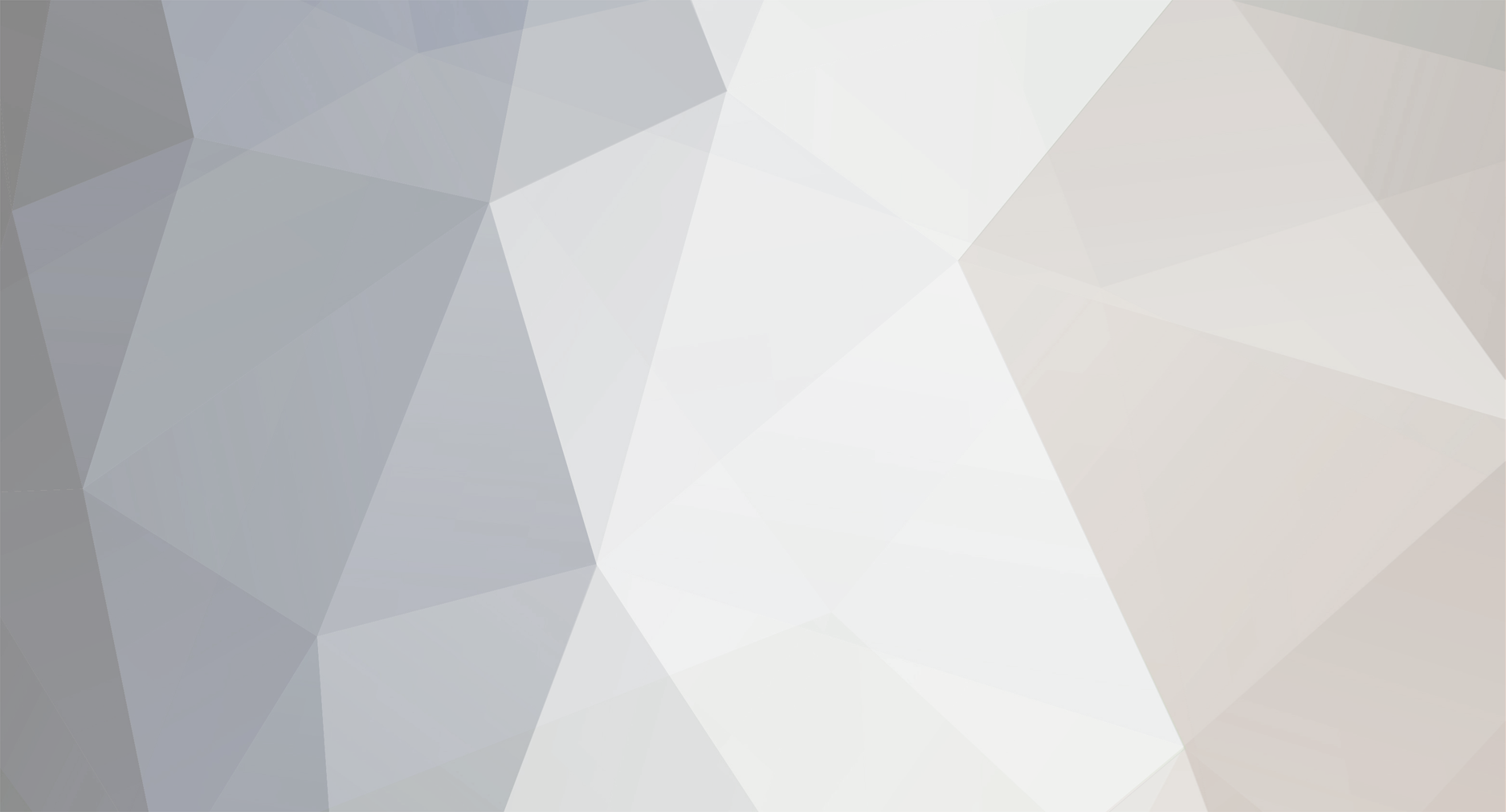
graham23s
-
Posts
890 -
Joined
-
Last visited
Posts posted by graham23s
-
-
Thanks mate stristr did the job
cheers
Graham
-
Hi Guys,
I'm using a curl request to get some html, when i get the html back: $html = curl_exec($ch); i want to check that $html contains a certain string, what would be the best way to do it?
thanks for any help guys
Graham
-
Hi Jax,
Thanks for that mate
it does the jobby hehe
cheers
Graham
-
Hi Guys,
A few people i give scripts to are having problems editing database files etc, so i was just going to write an install.php script for easiness.
What would be the best way to do it? i have 4 fields host,user,pass,database fields i a form once its submitted write a config.php file with the values is that usually how its done?
cheers guys
Graham
-
Hi Mate,
What does your html form look like?
try changing:
if ($_POST["Submit"])
to
if (isset($_POST["Submit"]))
cheers
Graham
-
Hi Guys,
I was wondering if there was any way i can print the contents to screen a domDocument, the path isn't working so it thought i could have a look and go from there.
code:
$dom = new DOMDocument(); $dom->loadHTML($html); $xpath = new DOMXPath($dom); $results = $xpath->query("/html/body/div[3]/center/table/tbody/tr[1]/td/table/tbody/tr/td[2]/div[@id='content']/table[1]/tbody/tr[2]/td[2]/em/font/span");
thanks guys
Graham
-
Hi Guys,
What i'm trying to do is for example if i add 5 products for example i add a watermark on each image with the product name on it, i don't want to upload the generic image everytime i add products i fi can help it.
1) Have a generic image "generic-image.jpg"
2) When i add a product via the form the product name is stamped on the image and the "generic-image.jpg" is renamed "product-name.jpg"
so essentially i have a generic image stored on my server, when i add a product that generic image is them copied into the images folder after being renamed the product name
any help would be appreciated
thanks guys
Graham
-
ah thanks jay perfect
Graham
-
Hi Guys,
I can't seem to get this part bit of code right, i'm doing SEO and i am changing the <title></title> tags according to page.
code:
<?php $q = mysql_query("SELECT `product_name` FROM `fcs_products` WHERE `id`=".$_GET['id'].""); $r = mysql_fetch_array($q); $productName = $r['product_name']; $q1 = mysql_query("SELECT `category_name` FROM `fcs_categories` WHERE `id`=".$_GET['cat-id'].""); $r1 = mysql_fetch_array($q1); $catName = $r1['category_name']; if (isset($_GET['cat-id'])) { $title = (isset($_GET['cat-id'])) ? "$catName" : ""; //$desc = (isset($_GET['cat-id'])) ? "$catName" : ""; } elseif (isset($_GET['id'])) { $title = (isset($_GET['id'])) ? "$productName" : "eBooks Store | Business eBooks | Marketing eBooks | Training Videos"; $desc = (isset($_GET['id'])) ? "$productName" : "the biggest selection of healthcare and software digital downloads"; } else { $title = (isset($_GET['id'])) ? "eBooks Store | Business eBooks | Marketing eBooks | Training Videos" : ""; $desc = (isset($_GET['id'])) ? "" : ""; } ?> <title><?php print $title; ?></title>
What i'm trying to do is if you on the category page $_GET['cat-id'] it displays the category name in the title tags , if your on the product page $_GET['id'] it displays both of those fine, the problem is if your not on the category or product page i just wanted it to display the default title tags by the looks of the code it should work but it doesn't show the default title tags in your on the index page.
have i overcomplicated it by any chance
cheers guys
Graham
-
Hi Guys,
Using this code i am able to grab the search results from a website:
<?php if (isset($_GET['searchDeep'])) { print $searchString; // Deep search code $searchString = str_replace( " ","+",$searchString); $search_url = "http://www.site.com/mkplSearchResult.htm?dores=true&includeKeywords=$searchString&firstResult=50"; // make the cURL request to $search_url $ch = curl_init(); curl_setopt($ch, CURLOPT_USERAGENT, 'Firefox (WindowsXP) - Mozilla/5.0 (Windows; U; Windows NT 5.1; en-GB; rv:1.8.1.6) Gecko/20070725 Firefox/2.0.0.6'); curl_setopt($ch, CURLOPT_URL,$search_url); curl_setopt($ch, CURLOPT_FAILONERROR, true); curl_setopt($ch, CURLOPT_AUTOREFERER, true); curl_setopt($ch, CURLOPT_RETURNTRANSFER,true); curl_setopt($ch, CURLOPT_TIMEOUT, 30); $html = curl_exec($ch); if (!$html) { echo "<p class=\"fcs-message-error\">cURL error:" . curl_error($ch) . " (Number " . curl_errno($ch).")</p>"; } curl_close($ch); // parse the html into a DOMDocument $dom = new DOMDocument(); @$dom->loadHTML($html); //$affid = get_option('ma_cb_affkey');if ($affid == '') { $affid = 'lun4tic' ;} $xpath = new DOMXPath($dom); $paras = $xpath->query("//div[@id='results']//tr/td[@class='details']/h4/a"); $para = $paras->item(0); $urlt = $para->textContent; if($urlt == '' | $urlt == null) { echo '<p class="fcs-message-error">No Deep search products found!</p>'; } else { $url = $para->getAttribute('href'); $url = str_replace("zzzzz", "graham25s", $url); $xpath = new DOMXPath($dom); $paras = $xpath->query("//div[@id='results']//td[@class='details']//div[@class='description']"); $para = $paras->item(0); $description = $para->textContent; $xpath = new DOMXPath($dom); $paras = $xpath->query("//div[@id='results']//td[@class='details']//h4/a"); $para = $paras->item(0); $title = $para->textContent; $link = '<a rel="nofollow" href="'.$url.'">'.$title.'</a>'; //print "<br/><strong>".$link."</strong><br/>".$description; print $link; } } ?>
this brings me back 1 search result, i need the document to loop and bring all results back but i'm not sure how to go about it.
any help would be appreaciated.
thanks guys
Graham
-
ah thanks mate, i have ammended all sites with the new code
cheers
Graham
-
Hi Guys,
I'm using tinyMce for my textare fields, when i input video embed code and store it in mysql, when i retrieve the embed code the actual code displays rather than showing the video < for example is &% code, is that soemthing i need to do before the code goes into mysql or when i get it out?
thanks for any help guys
Graham
-
Hi Guys,
My thumbnail function doesn't seem to make a .png thumbnail but it works on .jpg & .gif but not png files can anyone see any errors in my code at all i don't see any:
code:
function resize_image($uploadDirectory, $newFileName) { $original_image = $uploadDirectory; $ext = substr($original_image, strrpos($original_image, '.') + 1); $canvas_width = 65; $canvas_height = 65; $canvas = imagecreatetruecolor($canvas_width, $canvas_height); $white_background = imagecolorallocate($canvas, 255, 255, 255); imagefill($canvas, 0, 0, $white_background); list($image_width, $image_height) = getimagesize($uploadDirectory); $ratio = $image_width / $image_height; if ($ratio > 1) { $new_image_width = 65; $new_image_height = 65 / $ratio; } //$ratio > 1 else { $new_image_width = (float) 65 * $ratio; $new_image_height = 65; } if ($ext == "jpg") { $original_image = imagecreatefromjpeg($original_image); } //$ext == "jpg" if ($ext == "gif") { $original_image = imagecreatefromgif($original_image); } //$ext == "gif" if ($ext == "png") { $original_image = imagecreatefrompng($original_image); } //$ext == "png" imagecopyresampled($canvas, $original_image, 0, 0, 0, 0, $new_image_width, $new_image_height, $image_width, $image_height); $new_thumbnail_name = "thumb-$newFileName"; if ($ext == "jpg") { imagejpeg($canvas, "../imgProducts/img-th/$new_thumbnail_name", 100); return ("$new_thumbnail_name"); } //$ext == "jpg" if ($ext == "gif") { imagegif($canvas, "../imgProducts/img-th/$new_thumbnail_name", 100); return ("$new_thumbnail_name"); } //$ext == "gif" if ($ext == "png") { imagepng($canvas, "../imgProducts/img-th/$new_thumbnail_name", 100); return ("$new_thumbnail_name"); } //$ext == "png" imagedestroy($original_image); imagedestroy($canvas); }
thanks guys
Graham
-
Hi Guys,
Just wondering if it was possible to download an image from a website from a specified url? i'm not sure how i would copy the image from the website to my desktop.
like:
1) specify url
2) use reg ex to find the image source and filename
3) download to a specific location
thanks guys
Graham
-
Hi Guys,
Using this code:
$regex_pattern = "/<a href=\"(.*)\">(.*)<\/a>/"; preg_match_all($regex_pattern,$result,$matches); $i = 0; foreach($matches as $match) { print $match[0]; }
I gather all the "a href" anchor tags on page, but my foreach only outputs the first one and doesn't loop them all? plus the first say 5 anchor tags i don't really need is there a way to start looping from say the 5th one onwards?
thanks guys
Graham
-
Hi Guys,
I'm trying to generate 9 random digits, i have done rand(0, 999999999) but i was wanting the script to generate 9 random digits exactly not 8 or 7 but consistantly 9 i cant think of a way to dfo ity lol
any help would be great
thanks guys
Graham
-
Hi Guys
The output would be like:
site1.com/page1 added on 2010-01-31 23:17:53
site1.com/page2 added on 2010-01-31 23:17:53
site2.com/page1 added on 2010-01-31 23:17:56
site2.com/page2 added on 2010-01-31 23:17:56
site2.com/page3 added on 2010-01-31 23:17:56
they output one after each other, to make them a little better i was trying to seperate them like:
site1.com/page1 added on 2010-01-31 23:17:53
site1.com/page2 added on 2010-01-31 23:17:53
-------------------------------------------------------------- <- seperator
site2.com/page1 added on 2010-01-31 23:17:56
site2.com/page2 added on 2010-01-31 23:17:56
site2.com/page3 added on 2010-01-31 23:17:56
thanks guys
Graham
-
Hi Guys,
In a search query on my site i have the basic code setup like this:
<?php $s = mysql_query("SELECT * FROM `cpa` WHERE `raw_search` LIKE '%$search%' ORDER BY `raw_search` DESC"); while ($r = mysql_fetch_array($s)) { print $r['link'] . "<br />"; } ?>
This prints all the search data in a row, the only thing that groups the data together is the time stamp it was inserted in to the database with.
search1 (timestamp1)
search1 (timestamp1)
search2 (timestamp2)
search2 (timestamp2)
search2 (timestamp2)
what i am trying to do is put a <hr> under the last result to seperate them up a bit on page like:
search1 (timestamp1)
search1 (timestamp1)
_________________
search2 (timestamp2)
search2 (timestamp2)
search2 (timestamp2)
i can't think of way to do it, any help would be appreciated.
thanks guys
Graham
-
Hi Mate,
ahh that worked great! can't believe i miseed that one
cheers
Graham
-
Hi Guys,
What i'm trying to do is pull from and xml file and loop from the database, if 2 codes match then perform an action.
code:
<?php include("inc/inc-dbconnection.php"); $call_url = 'http://www.site.com/load_xml.php?id=15320&subid=&geoip='.$user_ip; $xml = simplexml_load_file($call_url); //START Simple Output foreach($xml->offer as $offers) { $c1 = $offers->country; $q = mysql_query("SELECT * FROM `cpa_country_codes`"); while ($r = mysql_fetch_array($q)) { $c2 = $r['code_country']; if ($c1 == $c2) { //Perform action } } } ?>
This is what i have concocted so far.
any help would be appreciated
thanks guys
Graham
-
Hi Guys,
I know this sounds a bit crazy, but i'm passing over a URL as aparmeter on my $_GET query like:
httpwww.site.com/page.php?param1=1¶m2=2¶m3=http://www.url.com
on localhost the GET values display properly but on my live site i get an error:
Forbidden
You don't have permission to access /cpa-xml.php on this server.
Additionally, a 404 Not Found error was encountered while trying to use an ErrorDocument to handle the request.
is there something i should do to the http string before sending?
cheers guys
Graham
-
Hi Guys,
when i upload a product to my site i upload the price in USD and GBP, im trying to think of a way users can display the price in their countries currency.
any help would be appreciated im stumped
Graham
-
Hi Guys,
This is astrange one lol, when i upload a product to my site i do:
product-name (then i make the image the same name) product-name.jpg
if i have:
£-or-$-product-name.jpg any of those characters in the image name. it displays and works fine on localhost but not when i do it on my server.
thumb-$1000-something.jpg <-- displays fine on localhost but the "$" makes it not show on my live site, i'm not sure what the heck could be up
any help would be great
thanks guys
Graham
-
ah thanks mate.
Graham
Scraping images
in PHP Coding Help
Posted
Hi Guys,
I'm using bing to scrape some images a user types in a search box:
code:
$im = $element->innertext; this returns a different image each time when i print it out, but when i do the update it stores the same image in the database rather than a seperate one, is theree a way i can update the database to use a different image each time rather than the same one?
thanks for any help guys
Graham