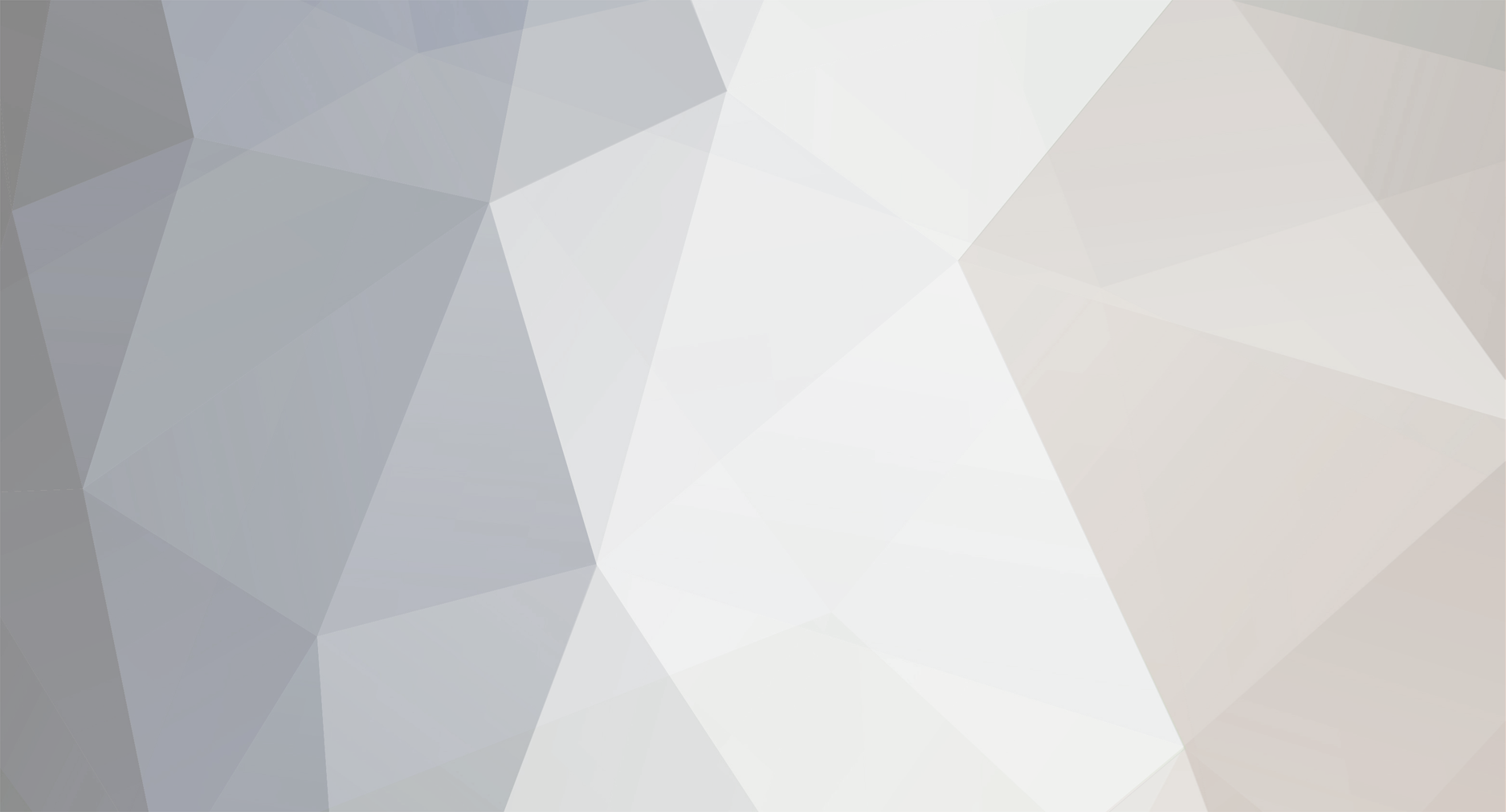
cgm225
Members-
Posts
444 -
Joined
-
Last visited
Never
Everything posted by cgm225
-
I have a two dimensional array with multiple entries, something created like: $this->Data[$id] = array( 'dir' => $dir, 'filename' => $filename ); And then I find a particular key and value in that array like so: return $this->Data[$this->id]; However, how can I reset the $this->Data array to hold just the $this->Data[$this->id] value, and none of the other values that were also in the array, and also still maintaining the two dimensional structure.
-
What would that class look like?
-
Currently for my applications I generate pagination with maybe 20 lines of procedural code. However, it is generally not that reusable and requires a fair amount of reworking if I use it in a new script. My question is, are there any OOP approaches to pagination? If so, could you provide some example code or tutorial? Thanks in advance!
-
[SOLVED] Only print/echo third value in array...
cgm225 replied to cgm225's topic in PHP Coding Help
perfect thank you -
[SOLVED] Only print/echo third value in array...
cgm225 replied to cgm225's topic in PHP Coding Help
What if the key values are not 1, 2, 3, 4 but rather 1000, 1001, 1002, or something else, how can I echo/print the third position regardless of the key name? -
If I have an array with several entries, how can I echo/print only the third one?
-
[SOLVED] Prepared statement only returning first row/entry...
cgm225 replied to cgm225's topic in PHP Coding Help
Got it.. I needed.. $this->photoData[$id] = array( ); -
I have the following method that returns photo entries from a MySQL database. However, even when there are multiple database entries/rows, the array only returns with one entry. I have tried to debug it, and it does not seem to be a problems with the limits or binding. Any ideas? public function findPhotos() { $query = 'SELECT id, filename, caption, date, location, album, timestamp FROM images WHERE album = ? ORDER BY filename ASC LIMIT ?, ?'; $statement = $this->connection->prepare($query); $statement->bind_param('sii', $this->albumData['id'], $this->skip, $this->numberRows); $statement->bind_result($id, $filename, $caption, $date, $location, $album, $timestamp); $statement->execute(); while($statement->fetch()) { $this->photoData = array( 'id' => $id, 'filename' => $filename, 'caption' => $caption, 'date' => $date, 'location' => $location, 'album' => $album, 'timestamp' => $timestamp ); } }
-
What is the regular expression for just numbers? I have this for numbers and letters: $regex = '/[^-_A-z0-9]++/';
-
Is it possible to "exit" the execution of an included file?
cgm225 posted a topic in PHP Coding Help
Is it possible to "exit" the action/execution of an included file, but NOT the execution of the file that included it? Restated, if I have: SomeFile.php '--------------included AnotherFile.php Is is possible to exit AnotherFile.php but continue on with executing SomeFile.php Thanks in advance! -
What does your directory structure look like for your applications? Where do you put your config files, your media files (like photos, etc), etc? I am curious what the best way to structure my web server directory is...
-
Well, what do you do?
-
Do I need to close my mysqli object? I am hearing different things, and this article says I need it... What are the communities thoughts?
-
Like this? public function setRegistryArray($registryItems) { foreach ($registryItems as $key => $value) { $this->set($key, $value); } }
-
I tried that and was having trouble with the logic.. could you give me some sample code?
-
I am creating a new method in which I pass a $registryItems variable that is an array, all as follow public function setRegistryArray($registryItems) { //$registryItems is an array() } Within that method, I want to use the following existing method so I can add the $registryItems array values to the registry: //Sets registry variable public function set($label, $object) { $this->store[$label] = $object; } However, I am uncertain how to do this. Thank you in advance!
-
I have a notes/blog class that returns entries from a MySQL database. Everything works fine, but I wanted to know if there was a better way to implement the class. Currently, I can grab either (1) many entries or (2) one entry, and, depending on which, I output the returned data (1) within a foreach loop, or (2) within a simple if statement. My question is, is there a way to make my procedural code more efficient where I am only needing one block of code to echo these variables, not two? Any other feedback is also appreciated Thanks in advance! Example use of notes/blog class: //Setting entry variable $regex = '/[^-_A-z0-9]++/'; $entry = isset($_GET['entry']) ? preg_replace($regex, '', $_GET['entry']) : null; //Setting MySQLi connection object and selecting database $mysqli = $this->registry->get('mysqli'); $mysqli->select_db('exampl_db'); //Instatiating new Notes class $notes = new Notes($mysqli); if (!$entry) { $notes->setLimits(); $notes->findEntries(); //First block of procedural code outputting many entries foreach($notes->getData() as $note) { echo $note['id'] . "<br>"; echo $note['title'] . "<br>"; echo $note['date'] . "<br>"; echo nl2br($note['note']) . "<br>"; echo $note['timestamp'] . "<br>"; } } else { $notes->findEntry($entry); $note = $notes->getData(); //Second block of procedural code outputting one entry if ($note) { echo $note['id'] . "<br>"; echo $note['title'] . "<br>"; echo $note['date'] . "<br>"; echo nl2br($note['note']) . "<br>"; echo $note['timestamp'] . "<br>"; } } Notes class: <?php class Notes { //Declaring variables private $connection; private $id; private $data = array(); //Sets MySQLi connection public function __construct(mysqli $connection) { $this->connection = $connection; } /* Sets limits for prepared statement used in the getEntries() method. If * limits are not manually set, then default values are used. */ public function setLimits($skip = 0, $numberRows = 10000) { $this->skip = $skip; $this->numberRows = $numberRows; } /* Creates a two dimensional array in which entry id numbers are stored in * the first dimension, and then for each id number, a second array (i.e. * the second dimension) is assigned, which contains all the field values * for that particular entry. */ public function findEntries() { $query = 'SELECT id, title, note, date, timestamp FROM notes ORDER BY id DESC LIMIT ?, ?'; $statement = $this->connection->prepare($query); $statement->bind_param('is', $this->skip, $this->numberRows); $statement->bind_result($id, $title, $date, $note, $timestamp); $statement->execute(); while($statement->fetch()) { $this->data[$id] = array( 'id' => $id, 'title' => $title, 'date' => $date, 'note' => $note, 'timestamp' => $timestamp ); } } /* Creates a one dimensional array in which an entry's id number and all * other field values are stored. */ public function findEntry($id) { $query = 'SELECT id, title, date, note, timestamp FROM notes WHERE id = ?'; $statement = $this->connection->prepare($query); $statement->bind_param('s', $id); $statement->bind_result($id, $title, $date, $note, $timestamp); $statement->execute(); if($statement->fetch()) { $this->data = array( 'id' => $id, 'title' => $title, 'date' => $date, 'note' => $note, 'timestamp' => $timestamp ); } } //Returns the one or two dimensional array of data public function getData() { if (!empty($this->data)){ return $this->data; } else { return false; } } } ?>
-
Making MySQLi connection avaliable to all MVC modules...
cgm225 replied to cgm225's topic in PHP Coding Help
Thanks again for all your help. Could you explain a little more about the class's accessors, and using a foreach loop? I am not sure what you mean, and what I am supposed to be doing. Also, I have the $mysqli->close in the index.php5 so I do not have to redundantly close it in each module. No good? What should I be doing? Finally, what is wrong with using those constants? Should I just be directly inserting those values into the mysqli statement? Thanks! -
Making MySQLi connection avaliable to all MVC modules...
cgm225 replied to cgm225's topic in PHP Coding Help
How does this look? I used a registry. Do you have any feedback for things I can improve? Index.php5 <?php //Initializing session session_start(); //General includes require_once 'configurations.php5'; //General configurations require_once MVC_ROOT . '/MysqliSetConnect.php5'; //MySQLi settings|connection require_once MVC_ROOT . '/Authentication.php5'; //User authentication require_once MVC_ROOT . '/RegistryItems.inc.php5'; //Default registry items require_once MVC_ROOT . '/ModelViewController.php5';//MVC with registry class //Instantiating registry $registry = new Registry($registryItems); //Instatiating front controller $controller = FrontController::getInstance(); $controller->setRegistry($registry); $controller->dispatch(false); //Closing MySQLi object created in MysqliSetConnect.php5 (included above) $mysqli->close(); ?> My MVC WITH a registry class <?php class FrontController extends ActionController { //Declaring variable(s) private static $instance; protected $controller; //Class construct method public function __construct() {} //Starts new instance of this class with a singleton pattern public static function getInstance() { if(!self::$instance) { self::$instance = new self(); } return self::$instance; } public function dispatch($throwExceptions = false) { /* Checks for the GET variables $module and $action, and, if present, * strips them down with a regular expression function with a white * list of allowed characters, removing anything that is not a letter, * number, underscore or hyphen. */ $regex = '/[^-_A-z0-9]++/'; $module = isset($_GET['module']) ? preg_replace($regex, '', $_GET['module']) : 'home'; $action = isset($_GET['action']) ? preg_replace($regex, '', $_GET['action']) : 'frontpage'; /* Generates Actions class filename (example: HomeActions) and path to * that class (example: home/HomeActions.php5), checks if $file is a * valid file, and then, if so, requires that file. */ $class = ucfirst($module) . 'Actions'; $file = $this->pageDir . '/' . $module . '/' . $class . '.php5'; if (!is_file($file)) { throw new FrontControllerException('Page not found!'); } require_once $file; /* Creates a new instance of the Actions class (example: $controller * = new HomeActions(), and passes the registry variable to the * ActionController class. */ $controller = new $class(); $controller->setRegistry($this->registry); try { //Trys the setModule method in the ActionController class $controller->setModule($module); /* The ActionController dispatchAction method checks if the method * exists, then runs the displayView function in the * ActionController class. */ $controller->dispatchAction($action); } catch(Exception $error) { /* An exception has occurred, and will be displayed if * $throwExceptions is set to true. */ if($throwExceptions) { echo $error->errorMessage($error); //Full exception echoed } else { echo $error->errorMessage(null); //Simple error messaged echoed } } } } abstract class ActionController { //Declaring variable(s) protected $registry; protected $module; protected $registryItems = array(); //Class construct method public function __construct(){} public function setRegistry($registry) { //Sets the registry object $this->registry = $registry; /* Once the registry is loaded, the MVC root directory path is set from * the registry. This path is needed for the MVC classes to work * properly. */ $this->setPageDir(); } //Sets the MVC root directory from the value stored in the registry public function setPageDir() { $this->pageDir = $this->registry->get('pageDir'); } //Sets the module public function setModule($module) { $this->module = $module; } //Gets the module public function getModule() { return $this->module; } /* Checks for actionMethod in the Actions class (example: doFrontpage() * within home/HomeActions.php5) with the method_exists function and, if * present, the actionMethod and displayView functions are executed. */ public function dispatchAction($action) { $actionMethod = 'do' . ucfirst($action); if (!method_exists($this, $actionMethod)) { throw new FrontControllerException('Page not found!'); } $this->$actionMethod(); $this->displayView($action); } public function displayView($action) { if (!is_file($this->pageDir . '/' . $this->getModule() . '/' . $action . 'View.php5')) { throw new FrontControllerException('Page not found!'); } //Sets $this->actionView to the path of the action View file $this->actionView = $this->pageDir . '/' . $this->getModule() . '/' . $action . 'View.php5'; //Sets path of the action View file into the registry $this->registry->set('actionView', $this->actionView); //Includes template file within which the action View file is included require_once $this->pageDir . '/default.tpl'; } } class Registry { //Declaring variables private $store; //Class constructor public function __construct($store = array()) { $this->store = $store; } //Sets registry variable public function set($label, $object) { $this->store[$label] = $object; } //Gets registry variable public function get($label) { if(isset($this->store[$label])) { return $this->store[$label]; } return false; } public function getRegistryArray() { return $this->store; } } class FrontControllerException extends Exception { public function errorMessage($error) { //If and throwExceptions is true, then the full exception is returned. $errorMessage = isset($error) ? $error : $this->getMessage(); return $errorMessage; } } ?> RegistryItems.inc.php5 <?php $registryItems = array(); $registryItems['pageDir'] = MVC_ROOT; //MVC root path **required by MVC classes $registryItems['mysqli'] = $mysqli; //MySQLi connection object $registryItems['auth'] = $auth; //MysqliAuthentication class object ?> Thanks again! -
I have seen include files with the following extensions: somefile.php somefile.inc.php somefile.inc What is the most appropriate file extension for include?
-
I have a simple registry class working.. just need to tweak it...
cgm225 replied to cgm225's topic in PHP Coding Help
Thank you for all your help. I will look into all those things. Also, a follow-up question.. I want to make sure I am keeping my module logic separate from my MVC logic. However, my user authentication class/module is used by almost all my other modules/apps, and so I have been including it in my MVC class as shown below (maybe use control-F with "user authentication" to find the line faster). Is this ok to do, or is there somewhere better I should be setting this variable? <?php class FrontController extends ActionController { //Declaring variable(s) private static $instance; protected $controller; //Class construct method public function __construct() {} //Starting new instance of this class with a singleton pattern public static function getInstance() { if(!self::$instance) { self::$instance = new self(); } return self::$instance; } public function dispatch($throwExceptions = false) { /* Checking for the GET variables $module and $action, and, if present, will strip them down with a regular expression function with a white list of allowed characters, removing anything that is not a letter, number, underscore or hyphen. */ $regex = '/[^-_A-z0-9]++/'; $module = isset($_GET['module']) ? preg_replace($regex, '', $_GET['module']) : 'home'; $action = isset($_GET['action']) ? preg_replace($regex, '', $_GET['action']) : 'frontpage'; /* Generating Actions class filename (example: HomeActions) and path to that class (example: home/HomeActions.php5), checking if $file is a valid file, and then, if so, requiring that file. */ $class = ucfirst($module) . 'Actions'; $file = $this->pageDir . '/' . $module . '/' . $class . '.php5'; if (!is_file($file)) { throw new FrontControllerException('Page not found!'); } require_once $file; /* Creating a new instance of the Actions class (example: $controller = new HomeActions(), and passing page directory variable to the ActionController class. */ $controller = new $class(); $controller->setRegistry($this->registry); try { //Using the setModule method in the ActionController class $controller->setModule($module); /* The ActionController dispatchAction method checks if the method exists, then runs the displayView function in the ActionController class. */ $controller->dispatchAction($action); } catch(Exception $error) { /* An exception has occurred, and will be displayed if $throwExceptions is set to true. */ if($throwExceptions) { echo $error->errorMessage($error); //Full exception echoed } else { echo $error->errorMessage(null); //Simple error messaged echoed } } } } abstract class ActionController { //Declaring variable(s) protected $registry; protected $module; protected $viewData = array(); //Class construct method public function __construct(){} public function setRegistry($registry) { //Sets the registry object $this->registry = $registry; /* Once the registry is loaded, the MVC root directory path is set from * the registry. This path is needed for the MVC classes to work * properly. */ $this->setPageDir(); /* Setting variables for objects external to the MVC that are used in * multiple modules. These objects add NO functionality to the actual * MVC classes, but are used by multiple modules. */ $this->auth = $this->registry->get('auth'); //User authentication object } //Sets the MVC root directory from the value stored in the registry public function setPageDir() { $this->pageDir = $this->registry->get('pageDir'); } //Sets the module public function setModule($module) { $this->module = $module; } //Gets the module public function getModule() { return $this->module; } //Placing a value in the $viewData array at the key position public function setVar($key, $value) { $this->viewData[$key] = $value; } //Returns a value from the $viewData array located at the key position public function getVar($key) { if (array_key_exists($key, $this->viewData)) { return $this->viewData[$key]; } } //Gets the viewData array public function getViewData($viewData) { $this->viewData = $viewData; } /* Checking for actionMethod in the Actions class (example: doFrontpage() within home/HomeActions.php5) with the method_exists function and, if present, the actionMethod and displayView functions are executed. */ public function dispatchAction($action) { $actionMethod = 'do' . ucfirst($action); if (!method_exists($this, $actionMethod)) { throw new FrontControllerException('Page not found!'); } $this->$actionMethod(); $this->displayView($action); } public function displayView($action) { if (!is_file($this->pageDir . '/' . $this->getModule() . '/' . $action . 'View.php5')) { throw new FrontControllerException('Page not found!'); } //Setting $this->actionView to the path of the action View file $this->actionView = $this->pageDir . '/' . $this->getModule() . '/' . $action . 'View.php5'; /* Creating a new instance of the View class and passing the $pageDir, $viewData, and actionView variables */ $view = new View(); $view->setRegistry($this->registry); $view->getViewData($this->viewData); $view->setVar('actionView',$this->actionView); $view->render(); } } class View extends ActionController { public function render() { //Including default template settings require_once $this->pageDir . '/defaultTplSettings.php5'; /* Merging the default template variables with variables provided in the $this->viewData array, taken from the Actions class, giving priority to those provided by the action class, then extracting the array with the extract() function, which creates a variable for every array value, and the name of the value (the variable name) is the key's value. */ $templateSettings = array_merge($defaultTemplateSettings, $this->viewData); extract($templateSettings, EXTR_OVERWRITE); //Including template file within which the action View file is included require_once $this->pageDir . '/default.tpl'; } } class FrontControllerException extends Exception { public function errorMessage($error) { /* If and throwExceptions is true, then the full exception will be returned. */ $errorMessage = isset($error) ? $error : $this->getMessage(); return $errorMessage; } } ?> -
I have a simple registry class working.. just need to tweak it...
cgm225 replied to cgm225's topic in PHP Coding Help
How does this look? Does my file naming look ok? And am I including everything in good locations? Do you have any other feedback? Thanks again! My index.php5 (where I instantiate the registry): <?php //Initializing session session_start(); //General includes require_once 'configurations.php5'; //General configurations require_once MVC_ROOT . '/MysqliSetConnect.php5'; //MySQLi settings & connection require_once MVC_ROOT . '/Registry.php5'; //Registry class and items require_once MVC_ROOT . '/Authentication.php5'; //User authentication //Instantiating registry $registry = new Registry($registryItems); //Including MVC classes and instatiating front controller require_once MVC_ROOT . '/ModelViewController.php5'; $controller = FrontController::getInstance(); $controller->setRegistry($registry); $controller->dispatch(false); //Closing MySQLi object created in MysqliSetConnect.php5 (included above) $mysqli->close(); ?> My Registry.php5 <?php //Registry class class Registry { //Declaring variables private $store; //Class constructor public function __construct($store = array()) { $this->store = $store; } //Sets registry variable public function set($label, $object) { if(!isset($this->store[$label])) { $this->store[$label] = $object; } } //Gets registry variable public function get($label) { if(isset($this->store[$label])) { return $this->store[$label]; } return false; } } /* Requiring file with a $registryItems array for use in the Registry class * constructor. This is NOT required for the registry class to work properly. */ require_once MVC_ROOT . '/RegistryItems.php5'; ?> My RegistryItems.php5 <?php $registryItems = array(); $registryItems['pageDir'] = MVC_ROOT; //**Required by MVC classes $registryItems['mysqli'] = $mysqli; ?> My ModelViewController.php5 <?php class FrontController extends ActionController { //Declaring variable(s) private static $instance; protected $controller; //Class construct method public function __construct() {} //Starting new instance of this class with a singleton pattern public static function getInstance() { if(!self::$instance) { self::$instance = new self(); } return self::$instance; } public function dispatch($throwExceptions = false) { /* Checking for the GET variables $module and $action, and, if present, will strip them down with a regular expression function with a white list of allowed characters, removing anything that is not a letter, number, underscore or hyphen. */ $regex = '/[^-_A-z0-9]++/'; $module = isset($_GET['module']) ? preg_replace($regex, '', $_GET['module']) : 'home'; $action = isset($_GET['action']) ? preg_replace($regex, '', $_GET['action']) : 'frontpage'; /* Generating Actions class filename (example: HomeActions) and path to that class (example: home/HomeActions.php5), checking if $file is a valid file, and then, if so, requiring that file. */ $class = ucfirst($module) . 'Actions'; $file = $this->pageDir . '/' . $module . '/' . $class . '.php5'; if (!is_file($file)) { throw new FrontControllerException('Page not found!'); } require_once $file; /* Creating a new instance of the Actions class (example: $controller = new HomeActions(), and passing page directory variable to the ActionController class. */ $controller = new $class(); $controller->setRegistry($this->registry); try { //Using the setModule method in the ActionController class $controller->setModule($module); /* The ActionController dispatchAction method checks if the method exists, then runs the displayView function in the ActionController class. */ $controller->dispatchAction($action); } catch(Exception $error) { /* An exception has occurred, and will be displayed if $throwExceptions is set to true. */ if($throwExceptions) { echo $error->errorMessage($error); //Full exception echoed } else { echo $error->errorMessage(null); //Simple error messaged echoed } } } } abstract class ActionController { //Declaring variable(s) protected $registry; protected $module; protected $viewData = array(); //Class construct method public function __construct(){ /* Setting variables for objects external to the MVC that are used in * multiple modules. These objects add NO functionality to the actual * MVC classes, but are used by multiple modules. THIS WILL BE GOING * THE REGISTRY.. NOT FINISHED!! */ $this->auth = MysqliAuthentication::getInstance(); //User authentication } public function setRegistry($registry) { //Sets the registry object $this->registry = $registry; /* Once the registry is loaded, the MVC root directory path is set from * the registry. */ $this->setPageDir(); } //Sets the MVC root directory from the value stored in the registry public function setPageDir() { $this->pageDir = $this->registry->get('pageDir'); } //Sets the module public function setModule($module) { $this->module = $module; } //Gets the module public function getModule() { return $this->module; } //Placing a value in the $viewData array at the key position public function setVar($key, $value) { $this->viewData[$key] = $value; } //Returns a value from the $viewData array located at the key position public function getVar($key) { if (array_key_exists($key, $this->viewData)) { return $this->viewData[$key]; } } //Gets the viewData array public function getViewData($viewData) { $this->viewData = $viewData; } /* Checking for actionMethod in the Actions class (example: doFrontpage() within home/HomeActions.php5) with the method_exists function and, if present, the actionMethod and displayView functions are executed. */ public function dispatchAction($action) { $actionMethod = 'do' . ucfirst($action); if (!method_exists($this, $actionMethod)) { throw new FrontControllerException('Page not found!'); } $this->$actionMethod(); $this->displayView($action); } public function displayView($action) { if (!is_file($this->pageDir . '/' . $this->getModule() . '/' . $action . 'View.php5')) { throw new FrontControllerException('Page not found!'); } //Setting $this->actionView to the path of the action View file $this->actionView = $this->pageDir . '/' . $this->getModule() . '/' . $action . 'View.php5'; /* Creating a new instance of the View class and passing the $pageDir, $viewData, and actionView variables */ $view = new View(); $view->setRegistry($this->registry); $view->getViewData($this->viewData); $view->setVar('actionView',$this->actionView); $view->render(); } } class View extends ActionController { public function render() { //Including default template settings require_once $this->pageDir . '/defaultTplSettings.php5'; /* Merging the default template variables with variables provided in the $this->viewData array, taken from the Actions class, giving priority to those provided by the action class, then extracting the array with the extract() function, which creates a variable for every array value, and the name of the value (the variable name) is the key's value. */ $templateSettings = array_merge($defaultTemplateSettings, $this->viewData); extract($templateSettings, EXTR_OVERWRITE); //Including template file within which the action View file is included require_once $this->pageDir . '/default.tpl'; } } class FrontControllerException extends Exception { public function errorMessage($error) { /* If and throwExceptions is true, then the full exception will be returned. */ $errorMessage = isset($error) ? $error : $this->getMessage(); return $errorMessage; } } ?> -
I have the following simple registry class working, but I wanted to know where I should be including all my registry->set() methods that are used to put variables/objects in the registry. Should I have a settings file? If so, is there a way to include those variables in a simple way in a .ini file without a redundant $registry->set statement each line? Could you provide me with some example code? Thank you all in advance! My registry class class Registry { //Declaring variables private $store = array(); static private $thisInstance = null; //Class constructor public function __construct() {} static public function getInstance() { if(self::$thisInstance == null) { self::$thisInstance = new Registry(); } return self::$thisInstance; } //Setting registry variable public function set($label, $object) { if(!isset($this->store[$label])) { $this->store[$label] = $object; } } //Getting registry variable public function get($label) { if(isset($this->store[$label])) { return $this->store[$label]; } return false; } } Example of current instantiation (which I do in my index.php5 file) //Instantiating registry & setting variables/objects $registry = new Registry(); $registry->set('pageDir', MVC_ROOT); //Registering page directory $registry->set('mysqli', $mysqli); //Registering MySQLi object
-
Making MySQLi connection avaliable to all MVC modules...
cgm225 replied to cgm225's topic in PHP Coding Help
bump -
I use gmail, but does hotmail have any filter features? Maybe you could filter that mail to your inbox?