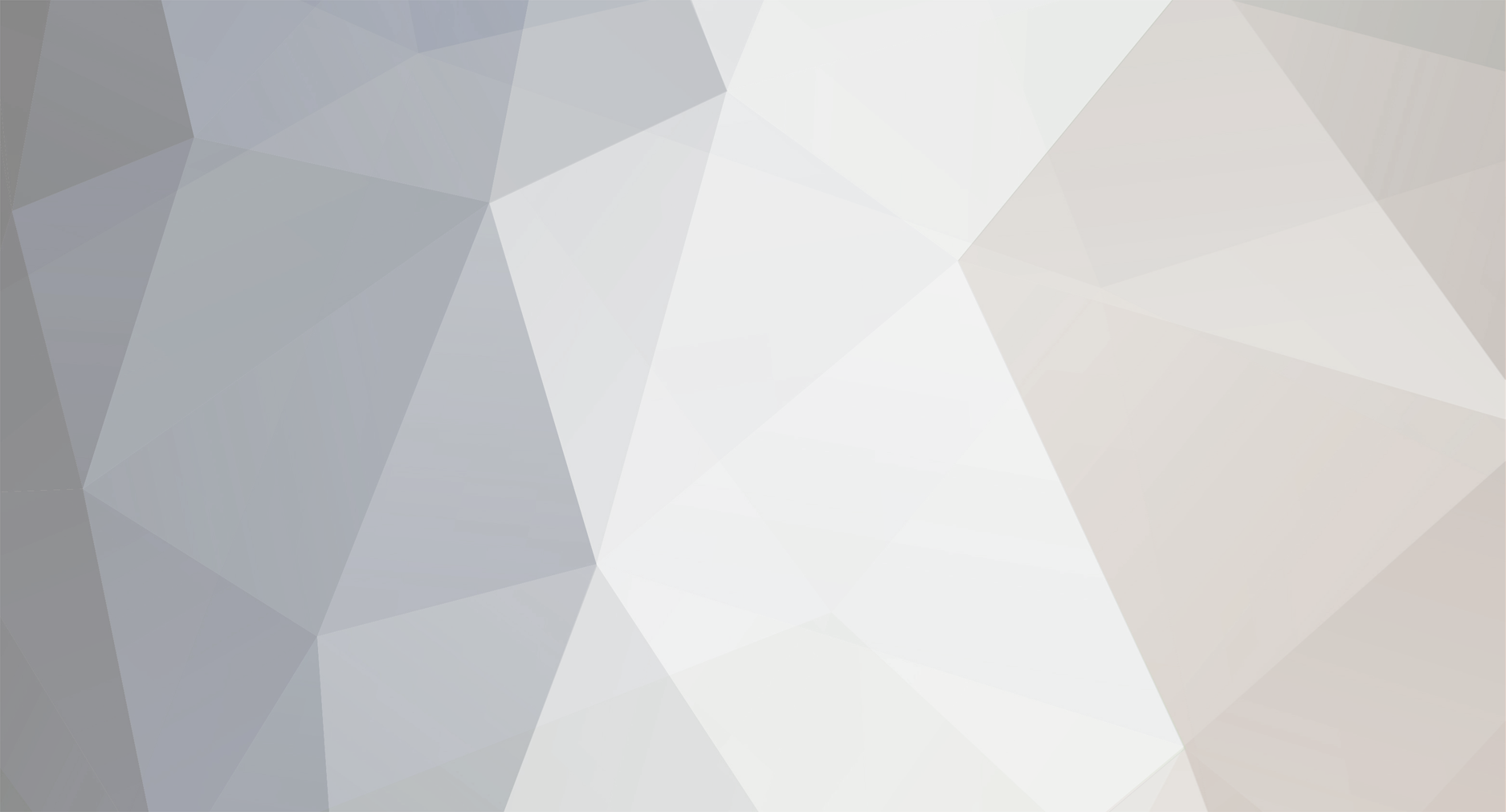
Asheeown
-
Posts
471 -
Joined
-
Last visited
Posts posted by Asheeown
-
-
Like jesi said you can post your code and either her or myself can help you fix your problem
-
Obviously it's not successfully getting through
try registering a session by first putting this at the very top of your page or in a page that your going to include:
[code]
<?php
session_start();
header("Cache-control: private");
?>
[/code]
Then register any old session like so
[code]
$_SESSION['Test'] = "TestVariables";
[/code] -
Your probably not an organizer but in my code this is how i would set something up to keep track of it:
[code]
<?php
if(blahblahblah) {
DO STUFF
} else {
DO OTHER STUFF
} // End of if(blahblahblah)
?>
[/code]
label your closings so you know if you closed something or not...use your tab key spread functions out....organize it a bit put things you open on one line the closing on the same and anything you open in between tab once before you do it
-
do you use sessions or cookies and if so whats your username session or cookie called
-
sure give me a few minutes to work something up here for ya
-
Something to get you going...pretty difficult when you aren't running the actual project but enough code so you can take over and put in your own values such as database info, etc.
-
Set them to "0" 1 = featured 0 = not
-
See where you want to start is take a page throw a form on there with say the name of your game then with your sessions or however you keep track of who is logged on call him from the database and select what league he is in...then call every user from that league and string a drop down box. basically its going to go to the page you specify with the form values which you can insert as a pending request in the database and when a user logs on he/she can simply confirm it by clicking on a button...if you want a starter code i could probably get you something
-
You want a user to submit scores and then anyone to just come along and confirm those scores?
-
One thing about your first index page is you don't have
[code]
<?php
session_start();
header("Cache-control: private");
?>
[/code]
insert that above your code on index.php -
http://www.phpfreaks.com/phpmanual/page/function.mail.html
A whole tutorial on mail
when your form sends to another page just use
$Email = $_POST['Email']
Or whatever your form object is called...the variable will be set to the data entered into the form object and you can use it from there -
$password = $_COOKIE['pass'];
$unencrypted = MD5($password); -
[code]<?php
session_start();
header("Cache-control: private");
?>
<?php
$ip = $_SERVER["REMOTE_ADDR"];
$songname = $_GET['songname'];
$songartist = $_GET['songartist'];
//first, check if the users ip needs to be added to the database.
function insertuser($id,$ip,$voted){
include "config.php";
if (isset($_SESSION['firsttime'])){
$insertsong = "INSERT INTO users (`id`, `ip`, `voted`) VALUES ('','".$ip."','".$voted."')";
$insertquery=mysql_query($insertsong, $conn)or die(mysql_error());
if($insertquery=mysql_query($insertsong)){
echo "You can request or vote for two more songs";
}else{
echo "An error has occurred. An administrator has been notified. thanks";
}
}
}
//if the user has voted before, once he requestes, increase his voted by 1
function previoususer($ip){
include "config.php";
if (isset($_SESSION['previoususer'])){
$findhim="SELECT voted FROM users WHERE ip = $ip UPDATE voted SET voted=voted+1";
mysql_query($findhim,$conn)or die(mysql_error());
}
}
function request($songname,$songartist){
//get the variables and secure them blah blah blah
$songname = strtolower($songname);
$songartist = strtolower($songartist);
$songname=str_replace(" ","",$songname);
$songartist=str_replace(" ","",$songartist);
$songname=str_replace("%20","",$songname);
$songartist=str_replace("%20","",$songartist);
$songname=addslashes($songname);
$songartist=addslashes($songartist);
//secure it some more! ALRIGHT (h)
if (!get_magic_quotes_gpc()) {
foreach ($_REQUEST as $el) {
$el = mysql_real_escape_string($el);
}
}
//Check if the song already exists
include "config.php";
$sql = "SELECT songname AND songartist FROM songs WHERE songname='".$songname."' AND songartist='".$songartist."'";
$result=mysql_query($sql,$conn)or die(mysql_error());
if(mysql_num_rows($sql) > 0){
$query="UPDATE votes FROM songs SET votes=votes+1";
$gettowork= mysql_query($query,$conn)or die(mysql_error());
if($gettowork){
echo "Your request was submitted succesfully";
}else{
echo "An error occured, your request was not successful";
}
}elseif(mysql_num_rows < 1){
$songvotes= 0;
$insertsong = "INSERT INTO songs (`songid`, `songname`, `songartist`, `songvotes`) VALUES ('','".$songname."','".$songartist."','".$songvotes."')";
$insertquery=mysql_query($insertsong, $conn)or die(mysql_error());
if($insertquery){
echo "The song was requested successfully";
}else{
echo "There was an error. The song was not requested successfully";
}
}
//begin the actual script
//determine whether this is hacking attempt/the user is lazy
if(!empty ($songname) && ($songartist)){
error_reporting(E_ALL);
include "config.php";
request();
insertuser();
previoususer();
mysql_close();
exit();
}else{
echo "A field was left blank";
exit();
}
}
insertuser('',$ip,'1');
previoususer($ip);
?>[/code]
Okay try this...this is without the request function something is screwed up in the bottom half of that function try this and if it gives you a white screen no info check your database to see if your vote number or anything was changed...first delete your ip from your database table just see if these two functions work before we move onto request -
[code]
<?
$ip = $_SERVER["REMOTE_ADDR"];
$songname = $_GET['songname'];
$songartist = $_GET['songartist'];
//first, check if the users ip needs to be added to the database.
function insertuser($id,$ip,$voted){
include "config.php";
if (isset($_SESSION['firsttime'])){
$insertsong = "INSERT INTO users (`id`, `ip`, `voted`) VALUES ('','".$ip."','".$voted."')";
$insertquery=mysql_query($insertsong, $conn)or die(mysql_error());
if($insertquery=mysql_query($insertsong)){
echo "You can request or vote for two more songs";
}else{
echo "An error has occurred. An administrator has been notified. thanks";
}
}
}
//if the user has voted before, once he requestes, increase his voted by 1
function previoususer($ip){
include "config.php";
if (isset($_SESSION['previoususer'])){
$findhim="SELECT voted FROM users WHERE ip = $ip UPDATE voted SET voted=voted+1";
mysql_query($findhim,$conn)or die(mysql_error());
}
}
function request($songname,$songartist){
//get the variables and secure them blah blah blah
$songname = strtolower($songname);
$songartist = strtolower($songartist);
$songname=str_replace(" ","",$songname);
$songartist=str_replace(" ","",$songartist);
$songname=str_replace("%20","",$songname);
$songartist=str_replace("%20","",$songartist);
$songname=addslashes($songname);
$songartist=addslashes($songartist);
//secure it some more! ALRIGHT (h)
if (!get_magic_quotes_gpc()) {
foreach ($_REQUEST as $el) {
$el = mysql_real_escape_string($el);
}
}
//Check if the song already exists
include "config.php";
$sql = "SELECT songname AND songartist FROM songs WHERE songname='".$songname."' AND songartist='".$songartist."'";
$result=mysql_query($sql,$conn)or die(mysql_error());
if(mysql_num_rows($sql) > 0){
$query="UPDATE votes FROM songs SET votes=votes+1";
$gettowork= mysql_query($query,$conn)or die(mysql_error());
if($gettowork){
echo "Your request was submitted succesfully";
}else{
echo "An error occured, your request was not successful";
}
}elseif(mysql_num_rows < 1){
$songvotes= 0;
$insertsong = "INSERT INTO songs (`songid`, `songname`, `songartist`, `songvotes`) VALUES ('','".$songname."','".$songartist."','".$songvotes."')";
$insertquery=mysql_query($insertsong, $conn)or die(mysql_error());
if($insertquery){
echo "The song was requested successfully";
}else{
echo "There was an error. The song was not requested successfully";
}
}
//begin the actual script
//determine whether this is hacking attempt/the user is lazy
if(!empty ($songname) && ($songartist)){
session_start();
error_reporting(E_ALL);
$ip = $_SERVER["REMOTE_ADDR"];
include "config.php";
request();
insertuser();
previoususer();
mysql_close();
exit();
}else{
echo "A field was left blank";
exit();
}
}
insertuser('',$ip,'1');
previoususer($ip);
request($songname,$songartist);
?>
[/code]
I changed numerous things try that -
Np...one thing i suggest for your database connecting is make a file called DBconnector or something have it connect to your database and include it in your files this way your functions can query your database without all those extra variables just PM me or post here again if you have anymore questions
-
Well if your sending your form to index2.php which I understand to be the page we were working on, that page isn't using the functions it's creating them. See when you send that person to the page it compiles all those functions and puts them in standby waiting for you to use them
For example say you wanted to insert a user using that function
[code]
<?php
function insertuser($id,$ip,$voted) {
if (isset($_SESSION['firsttime'])){
$insertsong = "INSERT INTO users (`id`, `ip`, `voted`) VALUES ('','".$ip."','".$voted."')";
$insertquery=mysql_query($insertsong, $conn)or die(mysql_error());
if($insertquery=mysql_query($insertsong)){
echo "You can request or vote for two more songs";
}else{
echo "An error has occurred. An administrator has been notified. thanks";
}
}
}
$ip = $_SERVER["REMOTE_ADDR"];
$voted=1;
insertuser('',$ip,$voted);
?>[/code]
Any variables you want inside your function have to be added to the list just like database stuff -
One thing you dont have to worry about is an error in your coding causing the white screen such as a closing or opening not being there or being there without needed the page loads.
But one thing i'm wondering is are you including this page somewhere and using these functions? -
Give this a try tell me how it goes
[code]
<?
//first, check if the users ip needs to be added to the database.
function insertuser(){
include "config.php";
$ip = $_SERVER["REMOTE_ADDR"];
if (isset($_SESSION['firsttime'])){
$voted=1;
$insertsong = "INSERT INTO users (`id`, `ip`, `voted`) VALUES ('','".$ip."','".$voted."')";
$insertquery=mysql_query($insertsong, $conn)or die(mysql_error());
if($insertquery=mysql_query($insertsong)){
echo "You can request or vote for two more songs";
}else{
echo "An error has occurred. An administrator has been notified. thanks";
}
}
}
//if the user has voted before, once he requestes, increase his voted by 1
function previoususer(){
include "config.php";
$ip= $_SERVER["REMOTE_ADDR"];
if (isset($_SESSION['previoususer'])){
$findhim="SELECT voted FROM users UPDATE voted SET voted=voted+1";
mysql_query($findhim,$conn)or die(mysql_error());
}
}
function request(){
//get the variables and secure them blah blah blah
$songname = $_REQUEST['songname'];
$songartist = $_REQUEST['songartist'];
$songname = strtolower($songname);
$songartist = strtolower($songartist);
$songname=str_replace(" ","",$songname);
$songartist=str_replace(" ","",$songartist);
$songname=str_replace("%20","",$songname);
$songartist=str_replace("%20","",$songartist);
$songname=addslashes($songname);
$songartist=addslashes($songartist);
//secure it some more! ALRIGHT (h)
if (!get_magic_quotes_gpc()) {
foreach ($_REQUEST as $el) {
$el = mysql_real_escape_string($el);
}
}
//Check if the song already exists
include "config.php";
$sql = "SELECT songname AND songartist FROM songs WHERE songname='".$songname."' AND songartist='".$songartist."'";
$result=mysql_query($sql,$conn)or die(mysql_error());
if(mysql_num_rows($sql) > 0){
$query="UPDATE votes FROM songs SET votes=votes+1";
$gettowork= mysql_query($query,$conn)or die(mysql_error());
if($gettowork){
echo "Your request was submitted succesfully";
}else{
echo "An error occured, your request was not successful";
}
}elseif(mysql_num_rows < 1){
$songvotes= 0;
$insertsong = "INSERT INTO songs (`songid`, `songname`, `songartist`, `songvotes`) VALUES ('','".$songname."','".$songartist."','".$songvotes."')";
$insertquery=mysql_query($insertsong, $conn)or die(mysql_error());
if($insertquery){
echo "The song was requested successfully";
}else{
echo "There was an error. The song was not requested successfully";
}
}
//begin the actual script
//determine whether this is hacking attempt/the user is lazy
if(!empty ($songname) && ($songartist)){
session_start();
error_reporting(E_ALL);
$ip = $_SERVER["REMOTE_ADDR"];
include "config.php";
request();
insertuser();
previoususer();
mysql_close();
exit();
}else{
echo "A field was left blank";
exit();
}
?>
[/code]
I organized it a bit to show more of where things start and end you also had an unnecessary closing to a statement which is your white page problem -
Hold on I caught something that may be your problem im looking into it now and testing it myself
a few session questions.
in PHP Coding Help
Posted
theres your problem:
replace with
if(defined('inSite')){