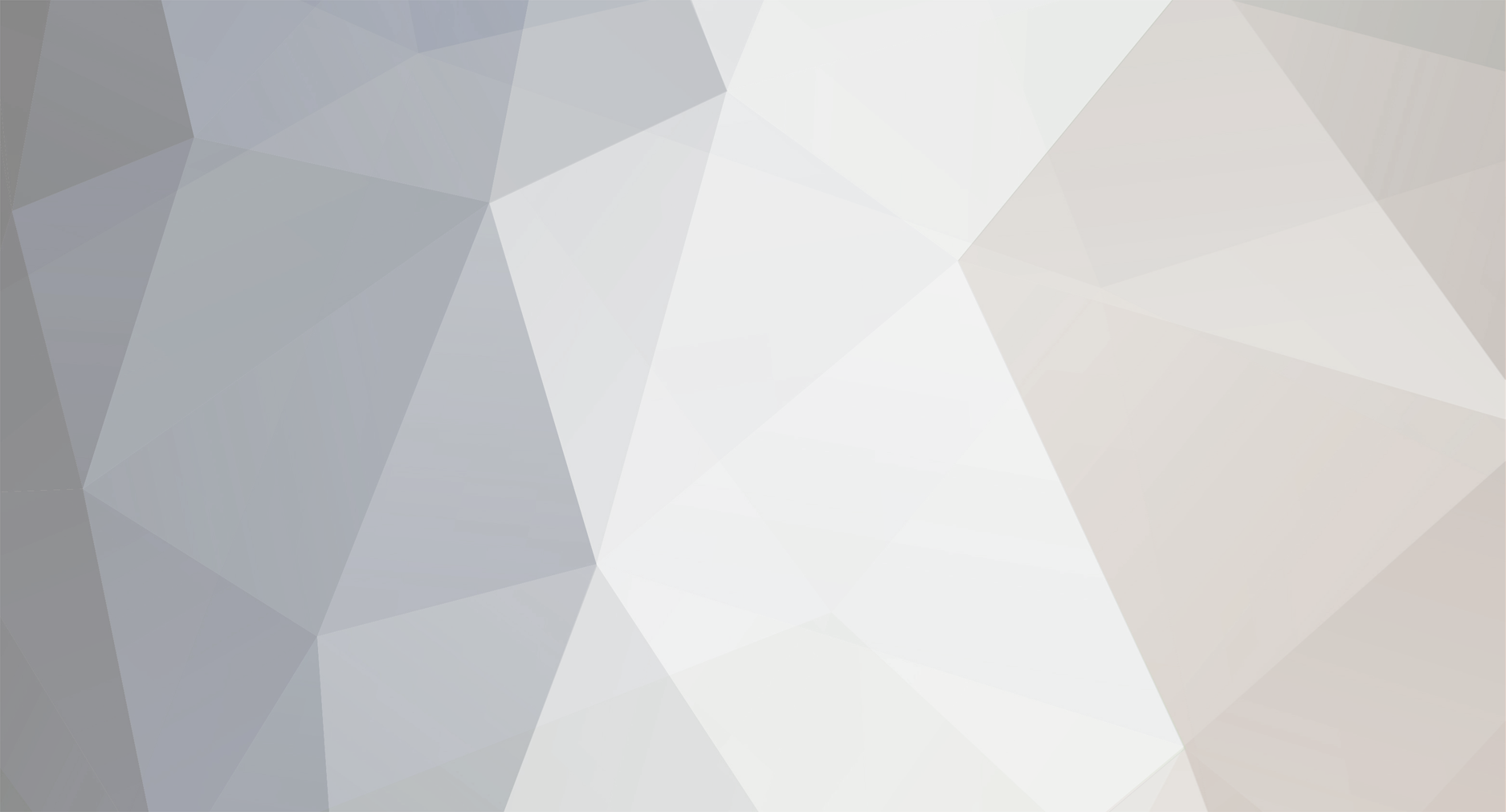
Aeglos
Members-
Posts
87 -
Joined
-
Last visited
Never
Everything posted by Aeglos
-
A common way of caching without checking database for content diferences is to cache the pages only when there is no previous cache of that particular page and you are requesting it. Then, when you update something in the database, you delete all cached pages that will access that data. That way the cache stays current and there are no "check db for new content" calls.
-
If you don't want to mess with MVC just yet, remember that classes should have a single responsability to them. A class that takes care of Users and Permissions is doing too much, break it up in two classes instead. Classes should (it's not always entierly possible, though) be able to function alone without the need for others, yet also be able to work together with the rest of them in your application. One of the real difficulties in OOP is achieving just that. And yes, everything you can do with common procedural PHP you can do with classes and object oriented approach. The trick is getting it right; It might take a while to grasp the more complex and useful underlying imlpications of OOP. If you are just starting out with classes and OOP, try something small. Decide on ONE thing to port from procedural to OO and do it, then, try implementing it in your site. It will give you a nice real contrast between the object approach and your former procedural. When you are comfortable with that one object, decide on another one and do it. Think of how your new object will interact not only with the procedural code now, but also with your first object... do they have to comunicate? if so, how? do they share common functionality? perhaps they have overlaping responsabilities... refactor them or create a third object that takes care of that common routines. How will this third object "talk" with the other two now? You will probably stumble across very common problems, to which solutions already exist in the form of "Design Patterns". For example, Registry and Dependency Injection are two patterns that attempt to solve the object comunication issue. Front Controller and Page/Action controllers fall in the category that businessman mentioned as "front end", they decide which parts of your site to load an display when visiting different pages. And finally, remember that although MVC is very popular and very useful for web applications (Here is a nice tutorial: click me gently ) it is not the only way to build pages. And it's probably a good idea to get some OOP excercise and grasp of concepts before trying to develop a full scale MVC site. Cheers.
-
A question about design: user and photo classes
Aeglos replied to MelodyMaker's topic in PHP Coding Help
In the case of methods such as deletePhoto() or findAllPhotos() which seem to be common for all pictures but not really that tied to a User object, isn't it easier (better approach/practice?) to create an object that specifically holds common functionality? Such as a Gallery object, or PhotoManager? Then the specific User object is associated with that particular "manager" object which holds the references to all it's pictures (Photo objects) instead and does tasks such as fetching all photos, deleting photos, finding by id or date or size, perhaps sharing photos across users? sorting, etc. -
If I may add my two cents, since I struggled with the same a few weeks ago. After some reading and research I came to the conclusion that there are many "ways" to implement MVC. To name one for example, Codeigniter goes away with the model and integrates it into the controller, which is a departure from the traditional MVC implementation. The traditional way (and what I chose to use) is the "Fat model thin controller" approach. The model contains all your application logic, all your procedures, database interactions, validation, data manipulation, preparing and delivering. The controller is just an elaborate wrapper over your model; deppending on what page you are visiting (controller action) it decides which model methods to call, what data to pass to them, what to do with the data returned, which template to render, where to redirect, etc. It "controls" the application flow. I believe the controller should contain nothing more that very light validation (check if logged in for example) and simple control statements (if -then -else). The model is the true core of your application which spits data ready for your controller to pass to the View as-is with no further modifications. In fact, aside from setting a few variables with model or user input data and pass them around, the controller should touch nor manipulate no data at all. That's the model's job. This way you can create for example a Gallery Model with methods such as fetchById(), addPictureToGallery(), removePictureFromGallery(), deleteGallery(), createSubGallery(), etc. The controller then calls this model methods with GET or POST arguments and the passes the results to the View. If you then create another site which also uses a gallery, you can use the same model (and DB info most likely)... since it does not care where the data comes from it will work fine as long as you supply it in the correct format. But the new controller may get the arguments from POST, from SESSIONS, from another model, wherever. Hope it helps. And if anyone has something to add or correct please do, I'm far from being an expert in MVC. Cheers.
-
maybe array_merge() ? array_merge($result['codelist'], $gamearray);
-
That's because both constructs (foreach() and each() ) use the internal array's pointer (each array has it's own) that indicates which element are they looking at currently, but when they finish iterating through the whole array the pointer is left at the end and not at the beginning again. So after the foreach() the array's pointer is at the end of the array, and when you call the next each() function it tries to traverse the array starting from the pointer, that is, the end, thus it immediatly exits. Use the reset() function to return the pointer to the first element, like this: $prices = array('Tires'=>100,'Oil'=>10,'Spark Plugs'=>4); foreach ($prices as $key => $price) { echo $key .'=>'.$price.'<br />'; } reset($prices); //Resets the pointer. while ($element = each($prices)) { echo $element['key']; echo ' - '; echo $element['value']; echo '<br />'; } That should work.
-
Your honor, I object! It's not really answering your question, but you could take a look at this often cited article: http://www.sitepoint.com/article/beyond-template-engine It might give you some new light and things to consider when thinking about a templating engine.
-
Try to ve more verbose and specific about your problems, It's hard to guess if so little information is given. Looking at your code It looks like you want to display a list of $events picked according to the $type and $agegroup selected in the drop menus. I see your form is also using the GET attribute, so the variables are sent in the $_GET array to the action page defined in the form (which is empty)... In that action page you must define a query according to the $_GET['agegroup'] and $_GET['type'] variables you passed from the form. There's really not much to say if you don't provide more info, like the database structure, or the page that recieves the submited form, for example.
-
So it's a mixed problem between string composition and array handling. The trick is to loop through the array and with it make a desired string to insert in the query, or ther shorter path; imploding it, or both. Let's say you type hint the argument for the sake of making it mandatory, you can do something like this: function pegevent_get_program_nid_from_showing_by_taxonomy ( $showing_nid, array $terms ) { //Initial empty string. $terms_string = ''; //Loops through the array single-quoting each element; foreach ($terms as $key => $value) { $terms[$key] = " '{$value}' "; } $terms_string = implode(', ', $terms); /* At this point the $terms_string holds a string in the form of: '301', '784', 'foo', 'xyz', '123' If you don't include the quoting loop, you can just implode the array. if so it will have the form: 301, 784, foo, xyz, 123 */ $qs = "select cs.field_program_nid from content_type_content_showing cs LEFT JOIN term_node tn ON (cs.field_program_nid = tn.nid) WHERE tn.tid IN ($terms_string) AND cs.nid = $showing_nid"; $qr = db_query( $qs ); $row = mysql_fetch_array( $qr ); if ($row[0]){ return $row[0]; } } That should do the trick. Regards.
-
I thought it might give an error, and it did... so I guess it's not possible. You can call it statically if it's just a simple generic true/false function, it's the closest to what you wanted. Or simply instatiate it properly beforehand.
-
This is PHP, not Java To access objects properties and methods you use the -> operator. The dot . operator is used to concatenate values. try: if (new SomeClass()->activate(true) == true) echo "It works!"; Can't remember if you can call a method at the same time you instatiate an object though, might give an error. You can also call the function statically without instatiting the object with the :: operator, as in: if (SomeClass::activate(true) == true) echo "It works!";
-
Just remember that everything inside functions or objects happens sequentially, one thing after the other. The PolarBear constructor goes like this (with the calls uncommented): public function PolarBear($bearName,$bearWeight) { parent::Bear($bearName,$bearWeight); $this->color = 'white'; $this->food = 'fish'; $this->name = $bearName; $this->Eat(); $this->Color(); } Notice that BEFORE setting the color to white and food to fish you are calling the parent's (Bear) constructor. So let's do a simple replace to get the equivalent code and see what is going on: public function PolarBear($bearName,$bearWeight) { /* Begin parent's constructor */ $this->name = $bearName; $this->weight = $bearWeight; $this->color = 'brown'; $this->food = 'honey'; $this->NameWeight(); $this->Eat(); //Outputs: Honey $this->Color(); //Outputs: Brown /* End parent's constructor */ $this->color = 'white'; $this->food = 'fish'; $this->name = $bearName; $this->Eat(); //Outputs: Fish $this->Color(); //Outputs: White } You see now? The Bear constructor calls the Eat() and Color() Methods before the PolarBear different properties are defined, thus it can only read the ones that the parent Bear class has set. You can also see that you are setting the same name twice... not really important though. Hope that clears it up some more. Cheers!
-
It's just the same as a normal argument. function video_airtime_by_category($videoID, $catArray) { //Simple loop to echo the arguments. foreach ($catArray as $key=>$value) { echo $key .' -> '. $value .'<br/>'; } echo $videoID; } video_airtime_by_category('69', array('php', 'oop', 'foo')); //valid video_airtime_by_category('69', array('php')); //valid video_airtime_by_category('69', 'php'); // not valid, can't loop through a single variable. That code assumes you pass an array, so you should check if it's a single variable also, like: function video_airtime_by_category($videoID, $categories) { if (is_array($categories) { foreach ($catArray as $key=>$value) { echo $key .' -> '. $value .'<br/>'; } } else { echo $categories; } echo $videoID; } video_airtime_by_category('69', array('php', 'oop', 'foo')); //valid video_airtime_by_category('69', array('php')); //valid video_airtime_by_category('69', 'php'); // also valid Or you can also type hint the second argument to accept ONLY arrays: function video_airtime_by_category($videoID, array $categories) { // some code. } video_airtime_by_category('69', array('php', 'oop', 'foo')); //valid video_airtime_by_category('69', array('php')); //valid video_airtime_by_category('69', 'php'); // not valid, will return an error. What you posted in as function video_airtime_by_category($videoID, $temp = array()) { } Is only giving a default empty array value to the second parameter. If you do that you can basically call the function without the $temp argument or override it, like: //All this ways would be valid video_airtime_by_category('69', array('php', 'oop', 'foo')); video_airtime_by_category('69', array('php')); video_airtime_by_category('69', 'php'); video_airtime_by_category('69'); Whether it will error out or not depends on what the function does. If you don't pass the second argument it will default to an empty array and if you pass it a string it won't be an array inside so you must account for all those cases.
-
Well, remove those calls from the constructor then It's cleaner to do something like: $nikolai = new Bear('Nikolai',235); $jon = new Bear('Jon',300); $maria = new Bear('Maria',100); $banavage = new PolarBear('Banavage',500); $nikolai->info(); $jon->info(); $maria->info(); $banavage->info(); where the info() method is like: public function info() { $this->NameWeight(); $this->Eat(); $this->Color(); } And as a side note, this belongs in the OOP subforum
-
It seems you are somewhat confused The loop you have there will only repeat itself as many times as results you get from the database, and the number of iterations you want seems to be unrelated to it (due to the $photo = $photo+1 part). Try this: while($row = mysql_fetch_array($result)) { //This will loop through 0 to 9999. Note that an "infinite" loop WILL timeout your script, so better make it finite. for ($photo=0; $photo<10000; $photo++) { echo "<a href='big/" . $row['Ref'] . " ($photo).jpg' target='_blank'><img style='border: solid 3px #000000' title='' src='sml/" . $row['Ref'] . ".jpg' /> </a>"; } } mysql_close($dbh);
-
You are calling the eat() and color() methods in the Bear constuctor, while those in PolarBear are commented out. Therefore the PolarBear is always Brown and eats Honey since it hasn't set it's color to white or it's food to fish before it's parent's constructor is called. Uncomment the calls in PolarBear and you should see something like: Hi, my name is Banavage and I weigh 500 kgs Banavage eats honey Banavage is brown Banavage eats fish Banavage is white
-
Is the said file included in community.php? as in something in the likes of: include 'lostNFound.inc.php'; On the top of the page?
-
As for overloading, PHP doesn't support traditional method overloading like in C# or Java as far as I know. What is called as "Overloading" in PHP5 are ways of creating objects methods and members on the fly, rather than different functions named the same. I believe you can emulate overloading somewhat in a number of ways. The first thing that comes to mind without any further searching is the func_num_args() and func_get_arg() functions, which returns the number of arguments passed to it, and the said arguments . So you might do: <?php public function bark() { $numArgs = func_num_args; if ($numArgs == 1) { //bark()'s a number of times specified by a single argument if it's provided. for ($i=0; $i<func_get_arg(0); $i++) { $this->woof(); } } else { //If no arguments, or more than one are provided, bark()'s a single time. $this->woof(); } } //Somwhere else in the code you can call: $object->bark(); //func_num_args() will return 0 $object->bark(3); //func_num_args() willl return 1, func_get_arg(0) will return 3. ?> Another solution would be to specify all arguments with default values, for example: <?php public function bark($numTimes = 1) { if ($numTimes > 1) { //bark()'s a number of times specified by $numTimes. for ($i=0; $i<$numTimes; $i++) { $this->woof(); } } else { //bark()'s a single time. $this->woof(); } } //Somwhere else in the code... $object->bark(); //$numTimes defaults to 1 $object->bark(3); //$numTimes is now 3 ?> As for the second question, passing arguments to the base class constructor (If I get your question correctly). Be aware that the child class automatically inherits from the base class any properties or methods defined in it, including it's constructor. Suppose this two classes: <?php class Dog { protected $name, $age; public function __construct($name, $age) { $this->name = $name: $this->age = $age; } } ?> And a child: (Notice it has no defined constructor nor properties) <?php class Pitbull extends Dog { public function barkAge() { echo $this->name . 'barks that he is' . $this->age . 'years old'; } } ?> This is completely valid code: <?php $dog = new Pitbull('Spike', 4); $dog->barkAge(); //Will output: "Spike barks that he is 4 years old" ?> The pitbull object has access to all it's parent's methods (including the constructor) and its methods. You can, of course, override them. Like: <?php class Pitbull extends Dog { protected $furColor; public function __construct($furColor) { $this->furColor = $furColor; } } ?> A pitbull object still has its $name and $age properties, but now the constructor overrides his parent's and sets a $furColor instead. If you wanted to keep the parent's functionality, you can do: <?php class Pitbull extends Dog { protected $furColor; public function __construct($name, $age, $furColor) { parent::__construct($name, $age); $this->furColor = $furColor; } } ?> And voila! Hope that helps clear up things on PHP's OOP. Cheers.
-
[SOLVED] Using Data transfer objects between script & HTML
Aeglos replied to matrixbegins's topic in PHP Coding Help
The point is that the line you indicated right there does not matter at all. The key is having the class file included BEFORE the session. If it does not work for you then something else must be wrong. I just tested this with 3 files I made from scratch and worked perfectly. Try this ultra simple example, SHOULD work... class.php <?php class Something{ private $foobar; public function getFoobar(){ return $this->foobar; } public function setFoobar($foobar){ $this->foobar = $foobar; } } ?> first.php <?php session_start(); include_once('class.php'); $text = 'It Verks!'; $fb = new Something(); $fb->setFoobar($text); $_SESSION['fb'] = $fb; echo '<a href="second.php">Click!</a>'; ?> second.php <?php include_once('class.php'); session_start(); $fb = $_SESSION['fb']; echo $fb->getFoobar(); ?> I just ran this and worked. As for passing the variables directly as an array, it's no problem. The $_SESSION array is simply a place intended to store user-specific persistent data (although we end up throwing all kinds of data in there). There is no problem in doing something like $_SESSION['userDataArray'] = $user->getData(); or similar. In fact, it might even be a good practice to do so. -
[SOLVED] Using Data transfer objects between script & HTML
Aeglos replied to matrixbegins's topic in PHP Coding Help
As rhodesa stated, your class has to be included before session_start(), the session handler has to know the class definition before it can recreate it. second.php <?php include_once("ClassDemo.php"); // Include before session_start session_start(); $user=new ClassDemo(); // This line is not needed. $user=$_SESSION["userObj"]; echo $user->getUser(); echo"<br>"; echo $user->getType(); ?> -
[SOLVED] Using Data transfer objects between script & HTML
Aeglos replied to matrixbegins's topic in PHP Coding Help
Be aware that you are overwritting variables all over the place. For example, in getInfo.php you are doing this: (I assume that the [ b] tags are not part of your code). <?php $info=new userInfo(); // $info is now an instance of the userInfo class $info=$info->getInfo($query); // info now contains the return value from the getInfo(); method, it is no longer an instance of the userInfo class. if($info->getEmail()==$email) // info->getEmail DOES NOT EXIST since $info is not an object. ?> Again in myHome.php <?php $user=new userInfo(); // $user is a new instance of the userInfo clas $user=$_SESSION["thisuser"]; // $user is now overwritten with the session variable. ?> That's probably why you get an error, you replaced the object with some random data. -
[SOLVED] Using Data transfer objects between script & HTML
Aeglos replied to matrixbegins's topic in PHP Coding Help
session_start() is the function that allows you to KEEP the $_SESSION superglobal across your scripts. HTTP is a stateless protocol, that's why variables don't preserve themselves between pages. So, for the server to maintain the $_SESSION array across all the pages you have to engage session functionality with session_start(). Simply include it on top of ALL your pages that will use the session functionality, but remember that you MUST call the function BEFORE anything is sent to the web browser, i.e. before any print() or echo. I don't think that including the files before session_start() makes a difference, but I can be wrong and can't hurt to try. Example: On page1.php <?php //Incude necessary stuff session_start(); $clown = new FunnyObject(); $clown->makeMeLaugh(); //Store it $_SESSION['clown'] = $clown; //Link to page2.php here ?> On page2.php <?php //Incude necessary stuff session_start(); //Retrieve it $clown = $_SESSION['clown'] //Use it! $clown->makeMeLaughAgain(); ?> This will NOT work: <?php echo 'This is my webpage!'; session_start() // <- You cant initialize it here since you already echo'ed something on screen. ?> And finally, if you don't want to use sessions, take a look at object serialization, which is the default procedure that session functionality uses to pass objects between pages. Hope this helps,. Cheers. -
retrive the php file name that is currently executed on web server.
Aeglos replied to yingzhao's topic in PHP Coding Help
Take a look at the $_SERVER superglobal variable, mainly $_SERVER['PHP_SELF']: http://php.net/manual/en/reserved.variables.server.php It'll probably contain some more things depending on your actual URLs so you might need to prep it through some explode() to remove unwanted data such as the local directory if the PHP_SELF returns something like: "new/site/file.php" -
if (mysql_num_rows($result) = 1) {... should be if (mysql_num_rows($result) == 1) {...