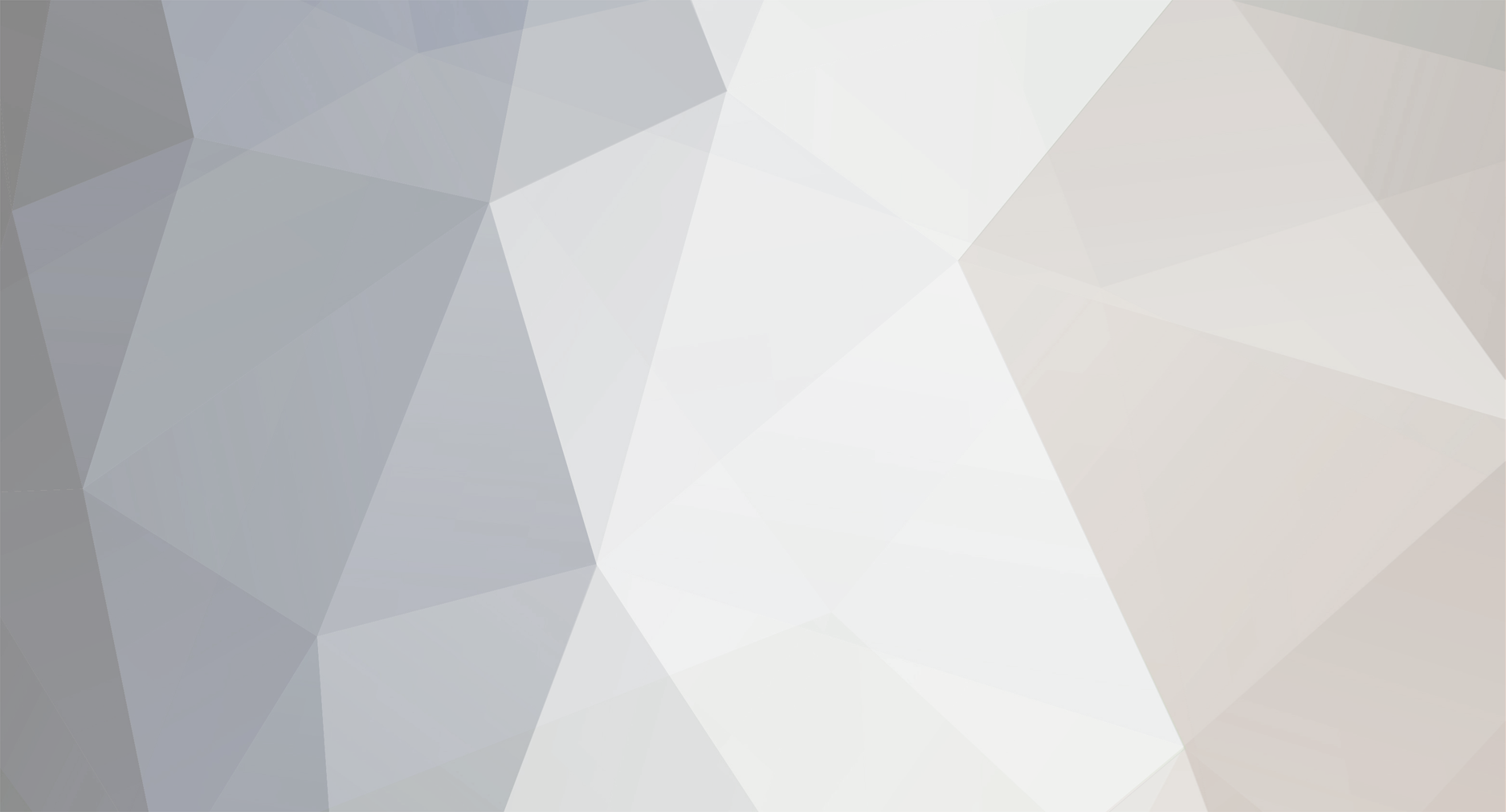
Aeglos
Members-
Posts
87 -
Joined
-
Last visited
Never
Everything posted by Aeglos
-
Form handling in MVC (validating / error reporting)
Aeglos replied to Wuhtzu's topic in Application Design
You don't need separate views for the same form. In my home made framework I solved that issue with the following aproach: My validator class stores all error messages in an internal array. When a form is posted, I run validation methods on all the posted input and then retrieve the error list. If the error list is empty, I display an error view and the form again. If not, I process the form. Code wise it's something like this (very simplified theoric example) some_controller.php <?php public function insert_comments() { if ($_POST['submit']) { //Validate $this->validator->isUsername($_POST['username']); $this->validator->isText($_POST['text']); $this->e = $this->validator->getErrors(); if (empty($this->e)) { $this->form_process(); //MySQL insert, update or whatever. } else { //Error display $this->template->loadTemplate('errors'); $this->template->populateTemplate($this->e); $this->template->showTemplate(); //Normal form display $this->template->loadTemplate('comments'); $this->template->showTemplate(); } else { //Normal form display $this->template->loadTemplate('comments'); $this->template->showTemplate(); } } ?> So, the form normaly looks like this: -------------------- Comments: Username: [_______] Text: __________________ | | | | |_________________| [submit] --------------------- But, if there are errors, they are presented with: The following errors were encountered: * Error 1 * Error 2 * Error 3 -------------------- Comments: Username: [_______] Text: __________________ | | | | |_________________| [submit] --------------------- The error mini template is just a div with an ordered list inside. Easily skinable through CSS to stand out from the rest, and is universal for the whole site. The technique is also extensible so that the form with errors contains the submited (albeit faulty) input for accesibility's sake with a simple $this->populateTemplate($_POST); after the error template. Also, having control structures in your views is not bad. My views are full of foreachs and ifs, as do codeigniter views. Otherwise it's difficult to implement dynamic size tables or lists. In the end, use the method that suits you best. Try a couple of alternatives and see what works for you. I'm sure there will be more ways to solve this posted by the more knowledgeable members, and will be far more efficient and elegant than mine. Regards. -
If I may add my 2 cents. I found out two errors in your code that might also be the problem. First: The marked variables are not declared anywhere in your class or method. They should be $db_username and $db_password Second: That's a logical comparison symbol (double ='s) and not an assignment. Your are basicaly comparing your class member (variable) with the mysql_error() function. It should read: Hope it helps. Cheers.
-
$sql = "UPDATE e107_user_extended SET user_DKP = user_DKP + '$dkp' WHERE user_extended_id = '".$select."' "; Try that, dunno... might work.
-
In here: $sql = 'UPDATE user_extended SET user_DKP = user_DKP + $dkp WHERE user_extended_id = $select'; Use double quotes when evaluating variables inside a string, like this: $sql = "UPDATE user_extended SET user_DKP = user_DKP + $dkp WHERE user_extended_id = $select"; or else escape the string like in the rest of your code: $sql = 'UPDATE user_extended SET user_DKP = user_DKP + '.$dkp.' WHERE user_extended_id = '.$select; And you are not "executing" that sql statement anywhere, as in your missing this: mysql_query($sql);
-
List multipal random output with DB updates if certian outputs...hmmm
Aeglos replied to ViciousC's topic in PHP Coding Help
Use code tags in the forum, and try to organize your code a bit. There are a couple of errors in your code ($page variable inside first array element? some escaped HTML in the middle of nowhere down at the bottom?). Here I fixed it a bit. (There are still some grammar errors in your array). <?php function search() { global $userrow, $numqueries; if (isset($_POST['explor'])) { $title = "Search"; $my_array = array("<font color=#C0C0C0>Burnt buildings and rubble everywhere.</font>", "<font color=#C0C0C0>You found Nothing...</font>", "<font color=#CB5634>You come across a old man looking for food.", "<font color=#C0C0C0>You found Nothing...</font>", "<font color=#C0C0C0>You found Nothing...</font>", "<font color=#CB5634>The sound of bombs going off in the distance.</font>", "<font color=#C0C0C0>You found Nothing...</font>", "<font color=#CB5634>You can smell a faint gas oder from a main line under the street.</font>", "<font color=#C0C0C0>You found Nothing...</font>", "<font color=#C0C0C0>You found Nothing...</font>", "<font color=#CB5634>You feel eyes upon you.</font>", "<font color=#C0C0C0>You found Nothing...</font>", "<font color=#CB5634>Your being followed.</font>", "<font color=#00CE00>You found Money...</font>", "<font color=#C0C0C0>You found Nothing...</font>", "<font color=#CB5634>You have no idea where you are right now.</font>", "<font color=#C0C0C0>You found Nothing...</font>", "<font color=#0000FF>What a long day.</font>", "<font color=#C0C0C0>You found Nothing...</font>", "<font color=#CB5634>As you walk you see lifeless bodies you use to call friends.</font>", "<font color=#C0C0C0>You found Nothing...</font>", "<font color=#CB5634>There is a noise ... just a rat.</font>", "<font color=#C0C0C0>You found Nothing...</font>", "<font color=yellow> You Found a Stone</font>", "<font color=#C0C0C0>You found Nothing...</font>"); for ($i=0; $i<=10; $i++) { // Here you should change the '10' for the number of times the player searched. $random = array_rand($my_array); $parola .= $my_array[$random]; } } if (isset($_POST['submit'])) { $title = "Search"; } else { $title = "Search"; $page .= "<form action=index.php?do=search method=post> <table align=center width=100%><tr><td align=center bgcolor=red valign=top>SEARCH</td></tr> <tr><td align=left>".$parola." <center><input align=center name=explor type=submit value=SEARCH /> </center></td></tr></table> <center><a href=index.php?do=towninf>GO BACK[/url]</center> </form>"; } display($page, $title); } ?> The array element that contains "You found some money" is key number 13 I believe. So you need to check inside your for loop: <?php if ($random == '13') { updateDatabaseFunctionOrOtherThing($arguments); } ?> Since I assume this is a game, and updating the database a bunch of times inside a for loop would be somewhat unwise, do something like: <?php if ($random == 13) { // if you found money $moneyGained += rand(1,69); //add some coins. } //Then OUTSIDE the for loop updateMoneyInDatabaseFunction($moneyGained); ?> Regards. -
I had written a huge post about this but discarded it. Better get straight to the point. I'm working on a personal MVC framework, using the a singleton registry to pass objects around (database and templates to my controllers for example). But i'm finding that every single object I load to the registry could, instead, be a separate singleton and be called on demand, thus eliminating the need for a registry entirely. So far I could part with the registry and make a singleton for the template, database, validator, log, etc classes, and moving the URI router to front controller, with the same effect. Is there something wrong with this approach? They all need just one instance to work, no need for multiple validator objects, or multiple database objects, and they all are often called multiple times through the controllers, making it seems that Foo::bar() is far more eficient for coding and readability than instatiating the registry, loading the object in it, instatiating the registry elsewhere when needed, calling the registered object, and the calling the desired method. Perhaps I'm not using the registry at it's fullest?... Using only singletons may also lead to tight coupling issues I think, and seems like a dirty replacement for the global namespace. Is there any advice on this? Or some reading/articles out there that tackle this? Thanks in advance, cheers.
-
Can someone tell me how to fix these fwrite lines?
Aeglos replied to DSGamer3002's topic in PHP Coding Help
Your double quotes are badly escaped. Change /" to \". Slashes anb Backslashes are NOT the same. Cheers. -
There is no difference (and besides the method name there isn't much to compare...). The error simply states that the object "gallerysizer" does not have a "loadImage()" method declared inside it. Perhaps there's a typo somewhere inside the gallerysizer class?
-
You are not saving the file anywhere. You need to use move_uploaded_file() before your fopen() block, like: <?php move_uploaded_file($_FILES['new_file']['tmp_name'], $destination_file); ?> Where $destination_file contains the destination folder and filename where you want to save your file. for example: "../images/chuck_norris.jpg". EDIT: Blargh, I just noticed that it might not be what you need since you seem to want to only extract the contents of a posted file instead of saving the actual file. I just got discombobulated... *dies*
-
There is a far easier way since the random generator it's not really random. It's just some fancy math applied on a seeded number. Random generators simulate "randomness" using very fast changing seeds, like the microseconds on the clock, thus the value outputed changes every time the seed changes. If you supply a constant seed, the random generator gets stuck. So if you seed the random generator with the current date, voilá, problem solved. <?php $seed = date("d"); //get the day. srand($seed); //seeds the random generator with it. echo $rand(); //get the not so random number ?> That way the rand() function will output the same number until the day changes. You can also specify ranges in the rand() function, it won't affect the behavior through the day. Be aware though, that the number WILL repeat itself over the months (ie. 25th September will return the same number as the 25th of December). You can overcome that problem by appliying some extra math and dates to the seed. Like $seed = $day+$month+$year; Hope it helps, cheers.
-
Blah, silly me, forgot an important part. You're basically saving the three photos to the same file, Try this at the beggining of your code: <?php $target = "../images/"; $target1 = $target . basename( $_FILES['photo']['name']); $target2 = $target . basename( $_FILES['photo1']['name']); $target3 = $target . basename( $_FILES['photo2']['name']); ?> Then at the if(): <?php if ( move_uploaded_file($_FILES['photo']['tmp_name'], $target1) && move_uploaded_file($_FILES['photo2']['tmp_name'], $target2) && move_uploaded_file($_FILES['photo3']['tmp_name'], $target3) ) { ...etc... ?>
-
Evidently it's there, since you only call move_uploaded_file() with 'photo' and never with 'photo2' and 'photo3'. Try: <?php if ( move_uploaded_file($_FILES['photo']['tmp_name'], $target) && move_uploaded_file($_FILES['photo2']['tmp_name'], $target) && move_uploaded_file($_FILES['photo3']['tmp_name'], $target) ) { ...rest of your code... ?> That way you check for the three uploads, if one fails, all fails.
-
Then you can use getimagesize(). It's right in the examples, something like: <?php list($width, $height) = getimagesize($uploaded_image); if ( ($width > 81) || ($height > 33) ){ echo 'upload invalid'; }else{ do_upload_thingy(); } ?>
-
Take a look at the image functions in php, particullary: http://www.php.net/manual/en/function.imagecopyresampled.php and the related ones needed (imagejpg(), imagecreatetruecolor(), etc) There's a couple of examples that can help you work your way up from them. Alternatively, you can just use CSS to force the submited images to 81x33, although they will look ugly sometimes and will not save you server space. Hope that helps, Cheers.
-
http://www.php.net/manual/en/language.types.array.php
-
That's because the table is echoed partway through the script, while the rest is only assembled inside $display_block through the script and then echoed at the end of it. To fix it, instead of echoing the table data, just store it inside another $display_table (or whatever name suits you) variable and echo it after the $display_block.
-
[SOLVED] welcome message for front of site help
Aeglos replied to thewooleymammoth's topic in PHP Coding Help
I figured it out, this is working (although a bit ugly, can be optimized I guess). <?php $lastart = 1; while ($lastart != 0){ $filename = $lastart.'.txt'; if (is_readable($filename)){ $openfile = fopen($filename, 'r') or die('error1'); $content = fread($openfile, filesize($filename)) or die ('error2'); $date = date('F d Y H:i:s.', filemtime($filename)); } else{ $content =null; } if (!$content){ $lastart = 0; } else{ echo '<table> <tr> <td>'.$date.'</td> </tr> <tr> <td>'.$content.'</td> </tr> <tr> <td>written by eric wooley</td> </tr> </table>'; $lastart++; } } ?> Note that I'm not a double quote fan Cheers. -
[SOLVED] welcome message for front of site help
Aeglos replied to thewooleymammoth's topic in PHP Coding Help
First, your loop never gets to the table echo <?php ... fclose($openfile); if(!fread($openfile, 'r')){ $lastart=0; }else{ ... ?> Since you terminate de file resource, fread will always return false, wich validates the first condition resseting $lastart and rewinding the while loop, ad nausea. Second, I don't believe you can do this (do enlighten me if I'm wrong ). <?php "... <td>"."date(F d Y H:i:s., filemtime($filename)"."</td> ..." ?> Try this for the echo: <?php echo "<table> <tr> <td>".date(F d Y H:i:s., filemtime($filename)."</td> </tr> <tr> <td>".$content."</td> </tr> <tr> <td> </td> </tr> </table>"; ?> As for looping through all the numbered files, I belive you only need to increment $lastart right after the echo (inside the if) by one ( $lastart++ Remember to move $lastart=1 out of the while loop though, else you'd return to file 1.txt eternally. Hope it helps, cheers. -
$var1 = $var2 . $var3; That will give you $var1 = 123456. The point operator ( . ) joins two variables, the plus operator simply arithmeticaly adds them, as in: $var1 = 123+456 = 579
-
I get "Division by Zero" with your code. Replace the “s and ’s with proper "s and 's, as in your first line. Notice they are different in the rest of your code.
-
[SOLVED] i no your all gettin anooyed at me but...
Aeglos replied to richarro1234's topic in PHP Coding Help
The closing double quote is missing at the mysql_query() statement in mjdamato's code. -
I you want to convert an amount of seconds into hours:minutes:seconds try this: <?php function foo($seconds) { $hours = floor($seconds/3600); $timeleft = $seconds - ($hours*3600); $minutes = floor($timeleft/60); $seconds = $timeleft - ($minutes*60); echo $hours.':'.$minutes.':'.$seconds; } ?> There's bound to be some better way to do this, and you'll probably have to modify it to use it in... whatever is it that you need it... but I really didn't catch entierely what you were trying to do anyway Cheers.
-
Try modifying the first block of code. Basically, if your $query variable is empty show a simple message, else continue with the rest of your code. <?php $query = mysql_query("SELECT * from somewhere;") or die(mysql_error()); $bg = '#eeeeee'; if (empty($query)) { echo 'No results found'; } else { ?> Do remember to include the closing bracket for that else at your last code block, after $n++ That would be a quick, dirty (and untested) solution, I hope. PS. Mixing shorthand tags (ie. <? ?>) with normal tags (ie. <?php ?>) is not the best of practices IMHO. In fact, it's best to avoid shorthands altogether unless you have a very good reason to use them and you can be 100% sure they will be always enabled server-side where you host your site, to avoid your code suddenly breaking with no apparent reason. Cheers.
-
<?php $row['body'] .= '... <a href="'.$news['href'].'">[more]</a>'; ?> Unless the commas were intentional (which makes no sense ).
-
In case you haven't solved it yet; You are missing a closing double quote in this line: $msteste="SELECT Admin FROM Website_acc WHERE strUserID = $login; Should read: $msteste="SELECT Admin FROM Website_acc WHERE strUserID = '$login'"; or $msteste="SELECT Admin FROM Website_acc WHERE strUserID = '".$login."'";