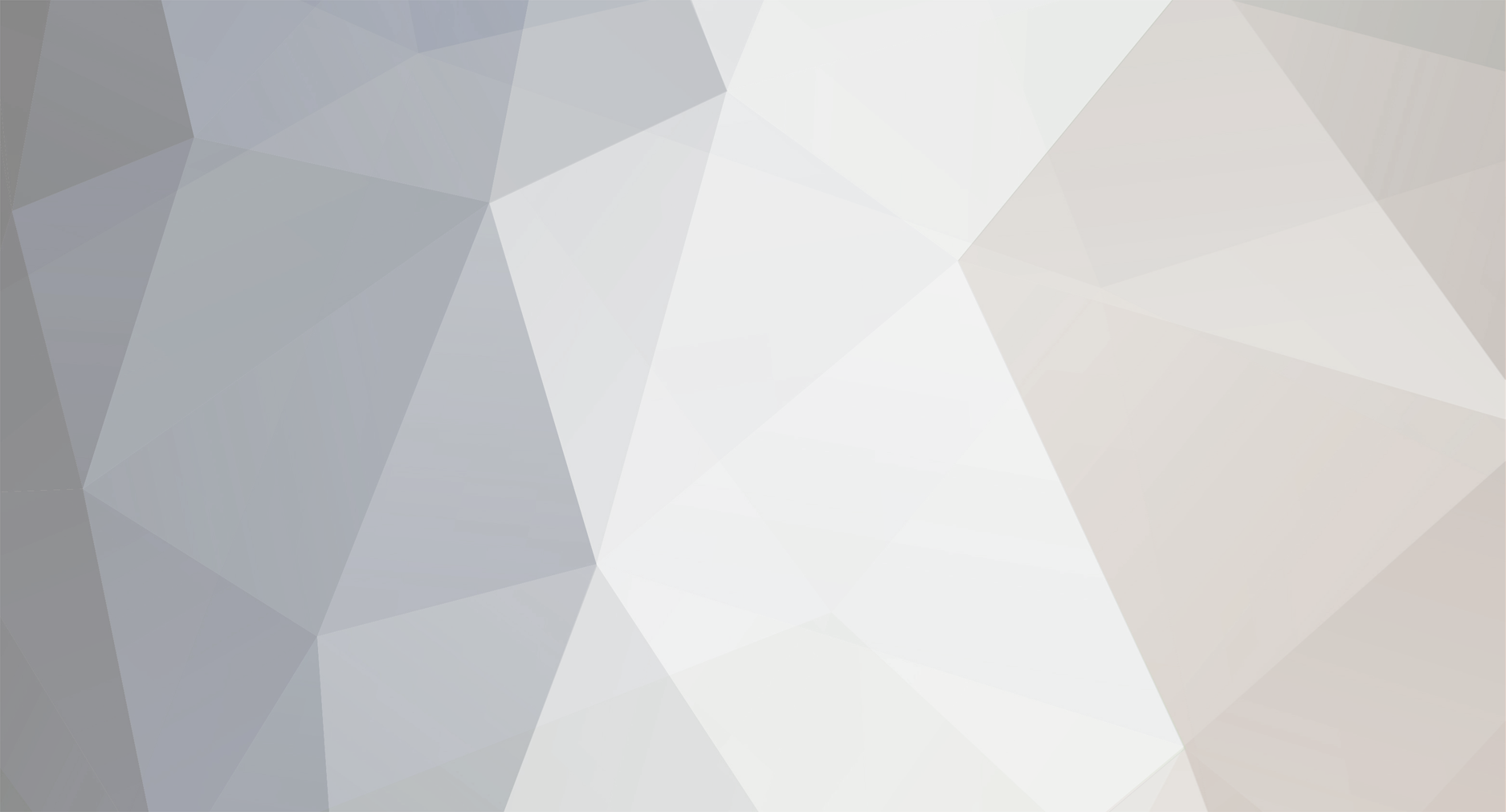
Tyche
Members-
Posts
49 -
Joined
-
Last visited
Never
Everything posted by Tyche
-
[SOLVED] Convert numerics to date for comparison purposes
Tyche replied to Obarnes's topic in PHP Coding Help
Have a look at the mktime function which creates a unix timestamp based on a particular date int mktime ( [int $hour [, int $minute [, int $second [, int $month [, int $day [, int $year [, int $is_dst]]]]]]] ) so you could use something like : $traveldate_timestamp = mktime (12,0,0,substr($traveldate,2,2),substr($traveldate,0,2),substr($traveldate,4,4)); $orderdate_timestamp = mktime (12,0,0,substr($orderdate,2,2),substr($orderdate,0,2),substr($orderdate,4,4)); Once the dates have been converted to a unix timestamp you test the difference between them EDIT : Or use ted_chou12's suggestion posted just before mine -
First of all you don't really need all these headers - especially the "Message ID=" header - A unique message ID will be generated for your email by your SMTP server - Autogenerated headers like these can often get you marked as spam so they really are counter productive in many cases - The "From" header is required and you can use the "Reply_to" The message format headers are probably ok ("MIME Version","Content-type" & "Content-Transfer" I'd check your own IP address against RDNS blacklists - if your IP address is blacklisted then there's next to nothing you can do with headers to clear the problem - www.dnsstuff.com has a good multi blacklist look up (as well as a lot of other very useful tools) look for the Spam Database Lookup test EDIT : Oh and unfortunately there is an mass emailing tool known as VXMailer which many spam filters trap - most only are looking for VXMailer on a "User-Agent" Header but some may be less conservative - unfortunately this is the same as your mailing list name (at least ignoring case) - I wouldn't be suprised if that's triggering the problem
-
I'm not sure why your code isn't working The following code however will extract the "name" values from within each "place" node <?php error_reporting(E_STRICT); $doc = new DOMDocument; $doc->load("http://www.43places.com/service/search_places?api_key=1234&q=Ann+Arbor"); $nodeList = $doc->getElementsByTagName('name'); foreach($nodeList as $node){ echo "$node->nodeValue\n"; } ?>
-
You are using assignment instead of comparsion in your if statments change if ($building = 'SteakHouse') { to if ($building == 'SteakHouse') { You also do the same on the DrugFactory if statement
-
Inserting a character at specified position in string
Tyche replied to play_'s topic in PHP Coding Help
Try $mystring = 'abc'; $findme = 'a'; $pos = strpos($mystring, $findme); $strtoinsert = 'xxx'; if ($pos === false) { echo "The string '$findme' was not found in the string '$mystring'"; } else { echo "The string '$findme' was found in the string '$mystring'"; echo " and exists at position $pos"; $newstring = substr($mystring,0,$pos).$strtoinsert.substr($mystring,$pos); echo "\nThe new string is '$newstring'"; } -
Almost right - the setlocale only impacts strftime not date try : $mydate=strftime('%c'); - time will still be local server time also date() requires a date formatting string - so you could use $mydate = date('r'); which will use the RFC 2822 date format e.g. Tue, 27 Feb 2007 12:53:07 +0500 for a US EST based server or use gmdate('r') to return the GMT time EDIT: Sorry not really thinking you can convert the date to UK format using $mydate=strftime('%d/%m/%Y'); To read 27/02/2007
-
It sounds like your are generating a mysql_error but the die statement is not closing off your html so no error is visible in your browser Try replacing the 3 occurances of die(mysql_error()) with die(mysql_error()."</body></html>")
-
You need to put your Regex Pattern in delimiters, try if (preg_match("/^tn%/",$myword)) { do something... Or use strpos - it's a bit more efficient in this case e.g. if (strpos($myword,"tn")===0) { do something...
-
Add the field name before the <= $curr_page_SQL = "SELECT User_Name, Points, Rank, Resource_1 FROM General_Stats WHERE Rank >= ".$lower_page_num." AND RANK <= ".$higher_page_num." ORDER BY Rank, User_Name";
-
It is your html and I've posted a solution on your original post.
-
Use "<=" not "=<" as below $curr_page_SQL = "SELECT User_Name, Points, Rank, Resource_1 FROM General_Stats WHERE Rank >= ".$lower_page_num." AND <= ".$higher_page_num." ORDER BY Rank, User_Name";
-
% signs come after the value not before on a width attribute use <td width = '15%'> etc
-
use a comma instead of && ORDER BY event_date, event_time
-
If I understand you correctly then your code is wrong If you are trying to divide the time into 4 chunks of 6 hour each begininng at 00:00, 06:00, 12:00 & 18:00 you need to use $time = date('G'); if ($time >= 0 && $time < 6) { $nunatt = 1; } elseif ($time >= 6 && $time < 12) { $nunatt = 2; } elseif ($time >= 12 && $time < 18) { $nunatt = 3; } elseif ($time >= 18 && $time < 24) { $nunatt = 4; } or probably simpler $time=date('G') $nunatt=1; if ($time >= 6)$nunatt++; if ($time >= 12)$nunatt++; if ($time >= 18)$nunatt++; date('G') returns the value of the current hour as an integer (not as a decimal) - Your original code wouldn't set $nunatt when the hour component of time was 1,2,3,4,5,6 12 or 18 (8 hours every day)
-
if ( ! isset($_SERVER['DOCUMENT_ROOT'] ) ) $_SERVER['DOCUMENT_ROOT'] = str_replace( '\\', '/', substr( $_SERVER['SCRIPT_FILENAME'], 0, 0-strlen($_SERVER['PHP_SELF']) ) ); is supposed to work under IIS but I've never tested myself
-
In the line $fields = fgetcsv($fp, 1000, ","); You specify the maximum line length in your source file as 1000 - The example line as posted by you is actually 994 characters long but I suspect it may be longer (either leading blanks or missing delimiters) - I'd suggest you increase this number - once fgetcsv reaches the limit it treats further data as a new line. EDIT: If you are running php 5 you don't need this parameter and can drop it
-
Use the MySQL REPLACE(str,from_str,to_str) string function For example UPDATE table SET url=REPLACE(url,"http://myorldurl.net/","http://mynewurl.org/") LIMIT 1; Try that and if it works on the first record remove the LIMIT 1 and re-run the query
-
The error is caused by the () you used on line 12 : echo $sweets(category_id); As it's an arry you need to use [] to reference elements Try this instead echo $sweets['category_id'];
-
Assuming one of your fields used in the MATCH AGAINST is a number (such as price) then it looks like a known MySQL bug (or feature according to the MySQL developers) - see http://bugs.mysql.com/bug.php?id=9907 but basically when a numeric field is converted to a string using implicit conversions then the collation set becomes binary (and therefore case sensitive) It does state that it will be fixed in v 5.1 You could try adding explicit conversions of the numeric fields - but I can't confim if that would work If this isn't the issue then I'm not sure what is.
-
Are you sure the Collation on the external webserver is latin1_swedish_ci (which is case-insensitive - thats what the _ci stands for) and not say latin1_bin (which effectively forces case sensitivity) ? The only way I know of turning off the case-sensitivity is to change the Collation - you can do that at the column,table or database level - to change the column Collation (and char set) use ALTER TABLE tbl_tabel MODIFY COLUMN field1 CHARACTER SET latin1 COLLATE latin1_swedish_ci ;
-
You haven't posted exactly what the problem is, but the first statement in your code won't work as you are not escaping the double quotes within the string - try putting \ before the internal " as below [code] echo "<html><script type='javascript'>function check(){ card_no = document.form.card_no.value; if(card_no.value.length < 16){ alert('card value blank'); return false; } if(name==\"\"){ alert('enter your name'); return false; } if(exp_date\"\"){ alert('card value blank'); return false; } }</script><body>"; [/code]
-
[SOLVED] Join a table more than once from a single table?
Tyche replied to iamgregg's topic in MySQL Help
You can achive this by adding the table `users` in the FROM section twice but using a different alias each time e.g. SELECT pairs.user_1, users_1.name, pairs.user_2, users_2.name FROM pairs, users AS users_1,users AS users_2 WHERE pairs.user_1 = users_1.id AND pairs.user_2 = users_2.id -
If you only have one record then you've already assigned it to the $data variable The next mysql_fetch_array will return false because you have no more data so the echo is never processed. .. try [code] $result = mysql_query("SELECT * FROM tblNews LIMIT 0 , 10"); if (!$result) { echo("The database could not be accessed at the moment"); } else { while($row = mysql_fetch_array($result)) { echo $row['heading']; } } [/code]
-
I'd check your variables you refer to $user[color=red][b]s[/b][/color]['posX'][/b] and then $user['posY']) I'm guessing both should be $user as thats the same array for holding the username