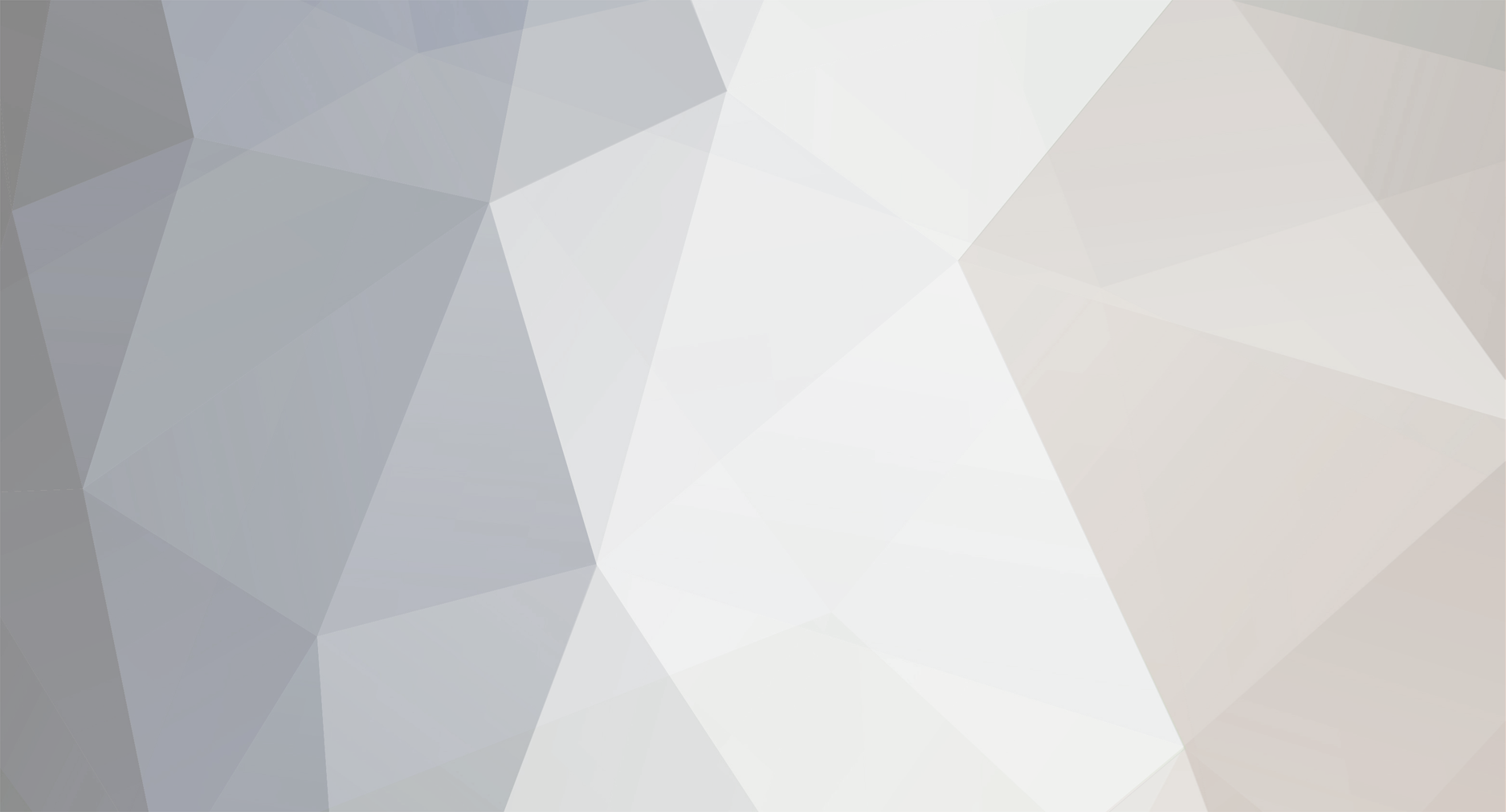
StormTheGates
Members-
Posts
170 -
Joined
-
Last visited
Everything posted by StormTheGates
-
I have a login script, and recently someone made a script in VB that tries to login 1000s of times. This has the problem of opening 1000s of connections to my MYSQL db. Any ideas how I can block this without a script check?
-
Hey all. I am running Fedora Core 5 and I was wondering how I can turn on MYSQL so that it logs all UPDATEs made to my DB? Thank you.
-
Maybe just add the right amount of seconds to the time of your servers timestamp?
-
[SOLVED] Problem with checking require fields.
StormTheGates replied to ubuntu's topic in PHP Coding Help
You sure? I just tested this code on my server: <?php if($_POST['submit']){ $test = $_POST['from']; die("+$test+"); } ?> <form action="v.php" method="post"> <table cellpadding="0" cellspacing="0" border="0" width="94%" align="center"> <tr> <td valign="top"><b>From</b></td> <td valign="top"><b> : </b></td> <td valign="top"><select name="from" class="field"> <option value="-">---------- Location ----------</option> <option value="66">Kuala Lumpur</option> <option value="67">Batu Pahat</option> <option value="68">Muar</option> </select></td> </tr> <tr> <td valign="top"><b>To</b></td> <td valign="top"><b> : </b></td> <td valign="top"><select name="to" class="field"> <option value="-">---------- Location ----------</option> <option value="66">Kuala Lumpur</option> <option value="67">Batu Pahat</option> <option value="68">Muar</option> </select></td> </tr> <tr> <td valign="top"><b>Month</b></td> <td valign="top"><b> : </b></td> <td valign="top"><select name="month" class="field"> <option value="-">-----------------</option> <option value="jan">January</option> <option value="feb">February</option> <option value="mar">March</option> <option value="apr">April</option> <option value="may">May</option> <option value="jun">June</option> <option value="jul">July</option> <option value="aug">August</option> <option value="sep">September</option> <option value="oct">October</option> <option value="nov">November</option> <option value="dec">December</option> </select></td> </tr> <tr> <td valign="top"><b>Year</b></td> <td valign="top"><b> : </b></td> <td valign="top"><select name="year" class="field"> <option value="-">-----------------</option> <option value="2007">2007</option> <option value="2008">2008</option> </select></td> </tr> <tr> <td valign="top"><b>Day</b></td> <td valign="top"><b> : </b></td> <td valign="top"><select name="day" class="field"> <option value="-">-----------------</option> <option value="mon">Monday</option> <option value="tue">Tuesday</option> <option value="wed">Wednesday</option> <option value="thu">Thursday</option> <option value="fri">Friday</option> <option value="sat">Saturday</option> <option value="sun">Sunday</option> </select></td> </tr> <tr> <td valign="top"><b>Date</b></td> <td valign="top"><b> : </b></td> <td valign="top"><select name="day_of_week" class="field"> <option value="-">-----------------</option> <option value="1">1</option> <option value="2">2</option> <option value="3">3</option> <option value="4">4</option> <option value="5">5</option> <option value="6">6</option> <option value="7">7</option> <option value="8">8</option> <option value="9">9</option> <option value="10">10</option> <option value="11">11</option> <option value="12">12</option> <option value="13">13</option> <option value="14">14</option> <option value="15">15</option> <option value="16">16</option> <option value="17">17</option> <option value="18">18</option> <option value="19">19</option> <option value="20">20</option> <option value="21">21</option> <option value="22">22</option> <option value="23">23</option> <option value="24">24</option> <option value="25">25</option> <option value="26">26</option> <option value="27">27</option> <option value="28">28</option> <option value="29">29</option> <option value="30">30</option> <option value="31">31</option> </select></td> </tr> <tr> <td valign="top"><b>Time</b></td> <td valign="top"><b> : </b></td> <td valign="top"><select name="time" class="field"> <option value="-">-----------------</option> <option value="twelve">12:00pm</option> <option value="third">03:00pm</option> <option value="fifth">05:00pm</option> </select></td> </tr> <tr> <td valign="top"><b>Quantity</b></td> <td valign="top"><b> : </b></td> <td valign="top"><select name="quantity" class="field"> <option value="-">-----------------</option> <option value="one">1</option> <option value="two">2</option> </select></td> </tr> <tr><td> <input type="submit" name="submit" value="Submit"> </td></tr> </table> </form> And it displayed fine. -
I figured it out, array_count_values is my friend this go around.
-
[SOLVED] Problem with checking require fields.
StormTheGates replied to ubuntu's topic in PHP Coding Help
Aright so its not even picking up the post variables. Two more things: 1. I see in your html you lack a <form action="page.php" method="post"> </form> element? Could that be it? 2. If you have one do this at the very top do this: $var = $_POST['from']; die("+$var+"); That will tell you what is being picked up if anything. -
[SOLVED] Problem with checking require fields.
StormTheGates replied to ubuntu's topic in PHP Coding Help
Change your code with stuff like this: <?php $error = NULL; if($_POST['from'] == "-"){ die("This is working so far!"); $error .= "Error, you haven't select from field.<br>"; } if($_POST['to'] == "-"){ die("This is working so far!"); $error .= "Error, you haven't select to field.<br>"; } if($_POST['month'] == "-"){ die("This is working so far!"); $error .= "Error, you didn't select month.<br>"; } if($_POST['year'] == "-"){ die("This is working so far!"); $error .= "Error, you didn't select year.<br>"; } if($_POST['day'] == "-"){ die("This is working so far!"); $error .= "Error, you didn't select day.<br>"; } if($_POST['day_of_week'] == "-"){ die("This is working so far!"); $error .= "Error, you didn't select date.<br>"; } if($_POST['time'] == "-"){ die("This is working so far!"); $error .= "Error, you didn't select departure time.<br>"; } if($_POST['quantity'] == "-"){ die("This is working so far!"); $error .= "Error, you didn't select quantity.<br>"; } if (!is_null($error)) { die($error); } ?> Tell me what happens. -
[SOLVED] Display entry from database to browser
StormTheGates replied to ubuntu's topic in PHP Coding Help
Yea the above code should work then. -
You could always route through GMails SMTP server?
-
[SOLVED] Display entry from database to browser
StormTheGates replied to ubuntu's topic in PHP Coding Help
Well I thought thats what you wanted? Or do you want to just display something out of the SQL statement? If you want to display the thing from the SQL query do something like this: <?php $connection = mysql_connect("$host", "$user", "$password") or die(mysql_error()); $db = mysql_select_db($database, $connection) or die(mysql_error()); $result = mysql_query("SELECT select fullname from pendaftaran where username = '$_COOKIE[username]'") or die(mysql_error()); $row = mysql_fetch_array($result); $fullname = $row['fullname']; ?> <html> <title><head>Display entry from database</head></title> <body> <p><?php echo $fullname; ?> </body> </html> -
[SOLVED] Display entry from database to browser
StormTheGates replied to ubuntu's topic in PHP Coding Help
Its because you ended the SQL string to early: $sql = "select fullname from pendaftaran where username = '$_COOKIE[username]'" or die(mysql_error()); Needs to be $sql = "select fullname from pendaftaran where username = \'$_COOKIE[username]\' or die(mysql_error());"; -
Hey all. Normally I would find something out like this myself but I am mentally exhausted after coding 3000 lines of code today. So I was wondering if anyone knows a way to count unique array elements? For example I have an array of 144 elements, some of them will be family1, some will be family2, family3, family4, or none. How to I count how many are family1? I am trying to determine who has the most amount of territories in an array.
-
[SOLVED] Problem with checking require fields.
StormTheGates replied to ubuntu's topic in PHP Coding Help
I dont see any form around your table telling it to submit anywhere as POST style. -
Ok I think the best way to learn is to code stuff and try it out Basically Ive been programming PHP for 3 years and I find Session variables much better than globals. When someone says "Validate form info", that normally means check to see if the user is doing what they are supposed to do. For instance: if(!$_POST['username']){ echo "You need to enter a username!"; } elseif(!$_POST['password']){ echo "You need to enter a password!"; } else { //Login Stuff here }
-
Nope, dosnt work.
-
Use something like this to generate an array of admin names: $admins = array(); $result = mysql_query("SELECT name FROM users WHERE status='Admin'); while($row = mysql_fetch_array($result)) { array_push($admins, $row['name']); }
-
[SOLVED] Upload image to mysql with php
StormTheGates replied to twistisking's topic in PHP Coding Help
Have you checked your PHP.ini and mysql settings to see the maxium upload settings? And correct me if I am wrong but can images even be uploaded to a MYSQL db? Maybe a link to it but not the actual code of the image itself :S -
To be honest with you PHP is a very secure language if configured properly. As for other languages, ASP is a no-go. Any language that has a period of time where you can add a % at the end of a variable URL and see all the source code is deffinitly a no go. Perl is a very good and powerful language, although diminishing rapidly. Python is also good, however its prevalence is very limited, so getting assistance is hard. I recomend PHP because there are alot of help sites, good documentations, and many many features and configurable options. Coupled with basic security software on your server I dont think youll ever encounter a problem.
-
Its called youtube or google video buddy Check this out though to: http://www.planet-source-code.com/vb/scripts/ShowCode.asp?lngWId=8&txtCodeId=1786
-
Ah I see. Well here is what I would do $names = array("Greg", "Jim", "Bob"); $i = 0; while($i < 4){ mysql_query("INSERT INTO `table` (`name`, `subject`, `msg`) VALUES ( '$names[$i]', 'This is a subject', 'This is the message'"); $i++; } Something like that?
-
Any help is appreciated. for($num=0; $num<=9; $num+=1){ $exsettings='$settings['.$num.']'; eval("\$exsettings = \"$exsettings\";"); if($exsettings=='1'){ $check$num='checked'; }else{ $check$num=''; } } Basically I need the variables to stack, for instance $check$num would wind up to be increasing such as $check1 $check2 $check3. How do I make it do this?
-
I am not quite getting what you are trying to do. Like you have a bunch of users with messages and you want to insert them all 1 by 1 into the db? In that case why just not use a loop?
-
One way would be to have a frame on something like sliced.php, and then have everything load within that frame. Make it 100% width and height and invisible or something.
-
PHP is server side, it will only do things when stuff goes to your server. When you hit f1 f2 f3 you are sending stuff to your browser and your own computer, not the server. So no, there isnt. Thats JS only.
-
[SOLVED] Displaying multiple items from database
StormTheGates replied to wrathican's topic in PHP Coding Help
Hes not nasty hes adorable! *cuddles his query* I think the problem with yours is that it only gets stuff from one table, when apparently there are 4 tables.