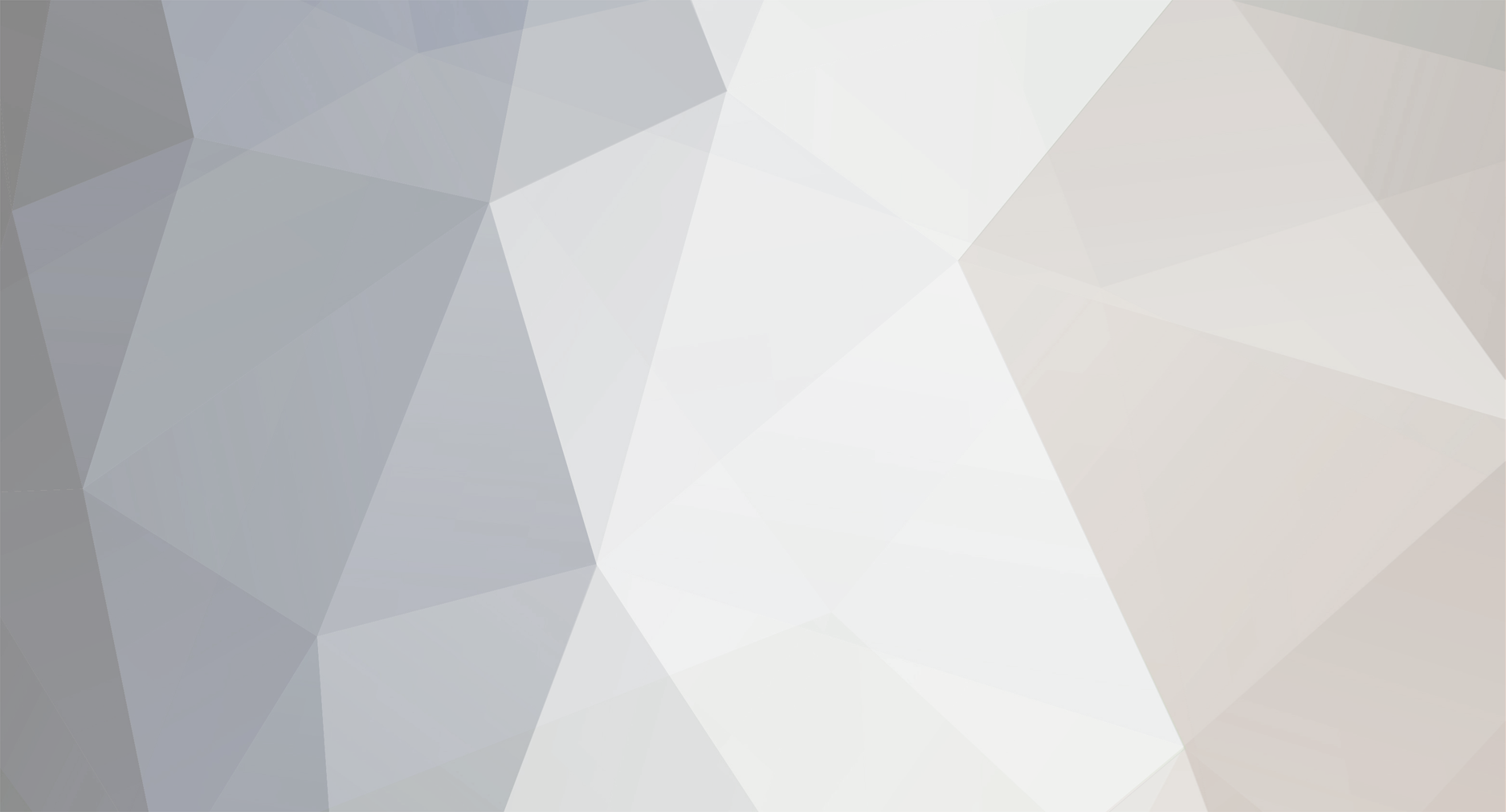
rn14
-
Posts
111 -
Joined
-
Last visited
Never
Posts posted by rn14
-
-
It now prints the values of the array but still get the same error. Thanks for your patience. Anything else it could be?
-
Apologies,
Here it is
$meallist = array();
echo $meallist;
$order->addMultipleItems($meallist[0]);
$items = $order->getItems();
-
When i print the value of $meallist it returns as not containing any values
-
I had copied the code directly with the include ('order.php'); I called the everything the same as what you had. When i remove the include I get a different error. Any suggestions
-
is it because the array is not being filled with ids?
<p><input type="checkbox" name="meal[]" value="<?php echo $row['id']; ?>"
I pass the id to the usage page using the above
-
I hope you are fairly bored I am going to a bit of explaining done!
I get the following error:
Fatal error: Call to a member function addMultipleItems() on a non-object
Thanks again
-
Hi, I use the following to store information in a class and then retrieve it at a latter stage. However what i want to do is when I have stored the first piece of information in the class for the user to be able to return and submit another piece of information(an order in this case) and for that to displayed with the initial piece of information at a later stage. The class object is stored in a session.
This are the classes i use
<?php class Item{ private $id; private $id1; private $id2; private $id3; private $id4; private $id5; private $id6; public function __construct($id, $id1, $id2, $id3, $id4, $id5, $id6) { $this->id = $id; $this->id1 = $id1; $this->id2 = $id2; $this->id3 = $id3; $this->id4 = $id4; $this->id5 = $id5; $this->id6 = $id6; } public function setid($id){$this->id = $id;} public function setid1($id1){$this->id1 = $id1;} public function setid2($id2){$this->id2 = $id2;} public function setid3($id3){$this->id3 = $id3;} public function setid4($id4){$this->id4 = $id4;} public function setid5($id5){$this->id5 = $id5;} public function setid6($id6){$this->id6 = $id6;} public function getid(){return $this->id;} public function getid1(){return $this->id1;} public function getid2(){return $this->id2;} public function getid3(){return $this->id3;} public function getid4(){return $this->id4;} public function getid5(){return $this->id5;} public function getid6(){return $this->id6;} } class Order { private $items = array(); function __construct() { } function addItem($item) { $this->items[] = $item; } function getItems() { return $this->items; } } ?>
This is how I add data to the class:
<? include('test.php'); session_start(); $meallist = array(); foreach ($_REQUEST as $k=>$v) { if ($k=="meal") { $meallist[] = $v; } } print_r($meallist); echo "<br>"; $result = count($meallist, COUNT_RECURSIVE); echo $result; echo "<br>"; $id = $meallist[0][0]; $id1 = $meallist[0][1]; $id2 = $meallist[0][2]; $id3 = $meallist[0][3]; $id4 = $meallist[0][4]; $id5 = $meallist[0][5]; $id6 = $meallist[0][6]; $order = new Order(100); $item = new Item($id,$id1,$id2,$id3,$id4,$id5,$id6); $order->addItem($item); $items = $order->getItems(); foreach ($items as $item) { echo $item->getid() . "<br>"; echo $item->getid1() . "<br>"; echo $item->getid2() . "<br>"; echo $item->getid3() . "<br>"; echo $item->getid4() . "<br>"; echo $item->getid5() . "<br>"; echo $item->getid6() . "<br>"; } $_SESSION['item'] = serialize($item); $_SESSION['order'] = serialize($order); ?>
This is how I retrieve it
<? include('test.php'); session_start(); $item = unserialize($_SESSION['item']); $order = unserialize($_SESSION['order']); $items = $order->getItems(); foreach ($items as $item) { echo $item->getid() . "<br>"; echo $item->getid1(); $id = $item->getid2() . "<br>"; echo $item->getid3() . "<br>"; echo $item->getid4() . "<br>"; echo $item->getid5() . "<br>"; echo $item->getid6() . "<br>"; echo $id; } ?>
Thanks
-
Im really trying to understand this but would really appreciate a little bit more help. I need to return each of those id's for every item would it be neccessary to have an array for id's aswell? Will I still use a session just as I have??
-
I need each id number will i be able to get that by doing like this??
-
This is a stupid question but how will I add the id's to the array??
-
it now works but only returns the first id and not the others???
When I add this
echo $class ->getid1(); echo $class ->getid2(); echo $class ->getid3(); echo $class ->getid4(); echo $class ->getid5(); echo $class ->getid6();
-
I have taken out that piece that overrides and still cant get it to work. I am fairly new to this so apologies for the bad code. I would like to know how to form this better and for it to be able to handle more items. if you could even point me in the right direction here that would be great?
-
still no good i have been looking at this for yours and can not seem to work out what it is. Thanks again. Any other suggestions??
-
Should the variables at the start of the order class by defined with $var instead of var???
-
Thanks for the replies. i have made those changes and i still cant get it to work. any other ideas???
-
I am trying to return the values that are stored in this class at least i think they are:
This is my order class:
<? class order{ var $id; var $id1; var $id2; var $id3; var $id4; var $id5; var $id6; public function _construct ( $or_id, $or_id1, $or_id2, $or_id3, $or_id4, $or_id5, $or_id6 ) { $this->id = $or_id; $this->id1 = $or_id1; $this->id2 = $or_id2; $this->id3 = $or_id3; $this->id4 = $or_id4; $this->id5 = $or_id5; $this->id6 = $or_id6; } public function getid(){return $id;} public function getid1(){return $id1;} public function getid2(){return $id2;} public function getid3(){return $id3;} public function getid4(){return $id4;} public function getid5(){return $id5;} public function getid6(){return $id6;} public function setid($id){$this->id = $id;} public function setid1($id1){$this->id1 = $id1;} public function setid2($id2){$this->id2 = $id2;} public function setid3($id3){$this->id3 = $id3;} public function setid4($id4){$this->id4 = $id4;} public function setid5($id5){$this->id5 = $id5;} public function setid6($id6){$this->id6 = $id6;} } ?>
This is where I fill the class with information
<? include('test.php'); session_start(); $meallist = array(); foreach ($_REQUEST as $k=>$v) { if ($k=="meal") { $meallist[] = $v; } } print_r($meallist); echo "<br>"; $result = count($meallist, COUNT_RECURSIVE); echo $result; echo "<br>"; $id = $meallist[0][0]; $id1 = $meallist[1][0]; $id2 = $meallist[2][0]; $id3 = $meallist[3][0]; $id4 = $meallist[4][0]; $id5 = $meallist[5][0]; $id6 = $meallist[6][0]; echo "<br>"; echo $id; echo "<br>"; $class = new order($id,$id1,$id2,$id3,$id4,$id5,$id6); $_SESSION['class'] = serialize($class); ?>
This is where i try and return these values:
<? session_start(); include('test.php'); $class = unserialize($_SESSION['class']); $class = new order('id','id1','id2','id3','id4','id5','id6'); echo $class ->getid()->id; echo $class ->getid1()->id1; ?>
It just returns nothing and I am certain those values are filled
Thanks in advance for any help
-
Where will I then insert that in my code?
Would I need to make any other changes
Thanks again
-
The only result I have is when I select all the fields from the database and then put them in an array. If I have an edit button next to each field how will that select the id that is displayed in that row of the table automatically??? Im sorry if this sounds stupid
-
There is no reason for storing it in the database would prefer not too. What I want to do is so that when the user is is displayed with a table and a list of results for them to be able to click on the edit button of any result and then be displayed with the info. Basically I dont know how to make ("SELECT * FROM menus where id = $ud_id") id = to the users choice?
-
The button that is stored in the datbase is just a hyperlink to the edit.php page. The hyperlink is stored with each record in the database. The code behind that page is
$ud_id = $_GET['id']; Print "$ud_id"; $link=mysql_connect(DB_HOST,DB_USER,DB_PASSWORD)or die("Could not connect: " . mysql_error()); mysql_select_db("rossnoon14_simp"); $result = mysql_query("SELECT * FROM menus where id = $ud_id"); while($row = mysql_fetch_array($result)) { $data = $row['text']; $title = $row['title']; $image = $row['image']; }
$ud_id = $_GET['id']; - This is the id which is meant to be passed from the code displayed in the first post? Does this explain better? Thanks
-
I have a database table with a number of different food options. I have created a small cms system and I want my admin to be able to edit that table with the food options. The user is presented with a page displaying all the food items and an edit button next to each one. The edit button is also stored in the database and is simply a link to the edit page. However when I select the edit button for each individual item it wont pass the id and details of that item to the edit page?????
I use mysql_fetch_array() to loop through the database and present all the items in the table and then attempt to post the id value to the next page when the edit button of a particular option is selected.
while($row = mysql_fetch_array($result)) { Print "<tr> <td>".$row['id'] . " </td> <td>".$row['title'] . " </td> <td>".$row['edit']." </td>; <td>".$show." </td>; <td>".$delete." </td></tr>"; } $id = $_POST['id'];
From this code I want the id of the individual row which is selected to be$_POST
-
I am curently attempting to put an application together which enables my client to submit a series of pieces of data through a number of forms. All data is sent to the database after it has been submitted. So basically I have a form info submitted and sent to database then another form which confirms success of sending other info and sends more info to the database. All the sending of info to the database works the only problem I have is when the information is submitted its placed in a new window instead of the existing one.
My form works by passing form data to php variables and then posting this to a php script which inserts the data in the database. I just have to get it to work so it remains in the same window instead of opening a new one.Im new to this so it could be something really obvious
<form action="updated_menu1_title.php" method="get" TARGET="_top">
<?php
$oFCKeditor = new FCKeditor('FCKeditor1') ;
$oFCKeditor->BasePath = 'http://www.rossnoonan.com/mffy/fckeditor/' ;
$oFCKeditor->Value = "$title";
$oFCKeditor->Create() ;
?>
<br>
<input type="hidden" name="ud_id" value="<? echo "$id";?>">
<input type="submit" value="Submit">
</form>
-
Hi there,
I am having a problem with FCKeditor which I really need to fix. When I upload an image FCKeditor automatically places <P></p> tags around the image, which messes up my layout. I then pass the image url along with the tags to a database. With the image then been displayed on my webpage from the database. Is there anyway I can stop these tags been sent to the database and just pass the image url. PHP and mysql are the languages been used.
Thanks for your help
-
I had installed PHP5 with apache and mysql version 3.23.52 and I had been using PHP and apache without using mysql successfully. But recently I have wanted to use mysql but only to realise that this version doesn't accept some modern commands. I then attempted to delete all mysql files on my computer this included the mysql files in the php folder. I then dowmloaded a new version of mysql but I still ended up with the php recognising the original version(3.23.52). I have been at this for a number of days with no success. Anybody have any suggestions?
Object Question
in PHP Coding Help
Posted
This is the function
This is usage.php