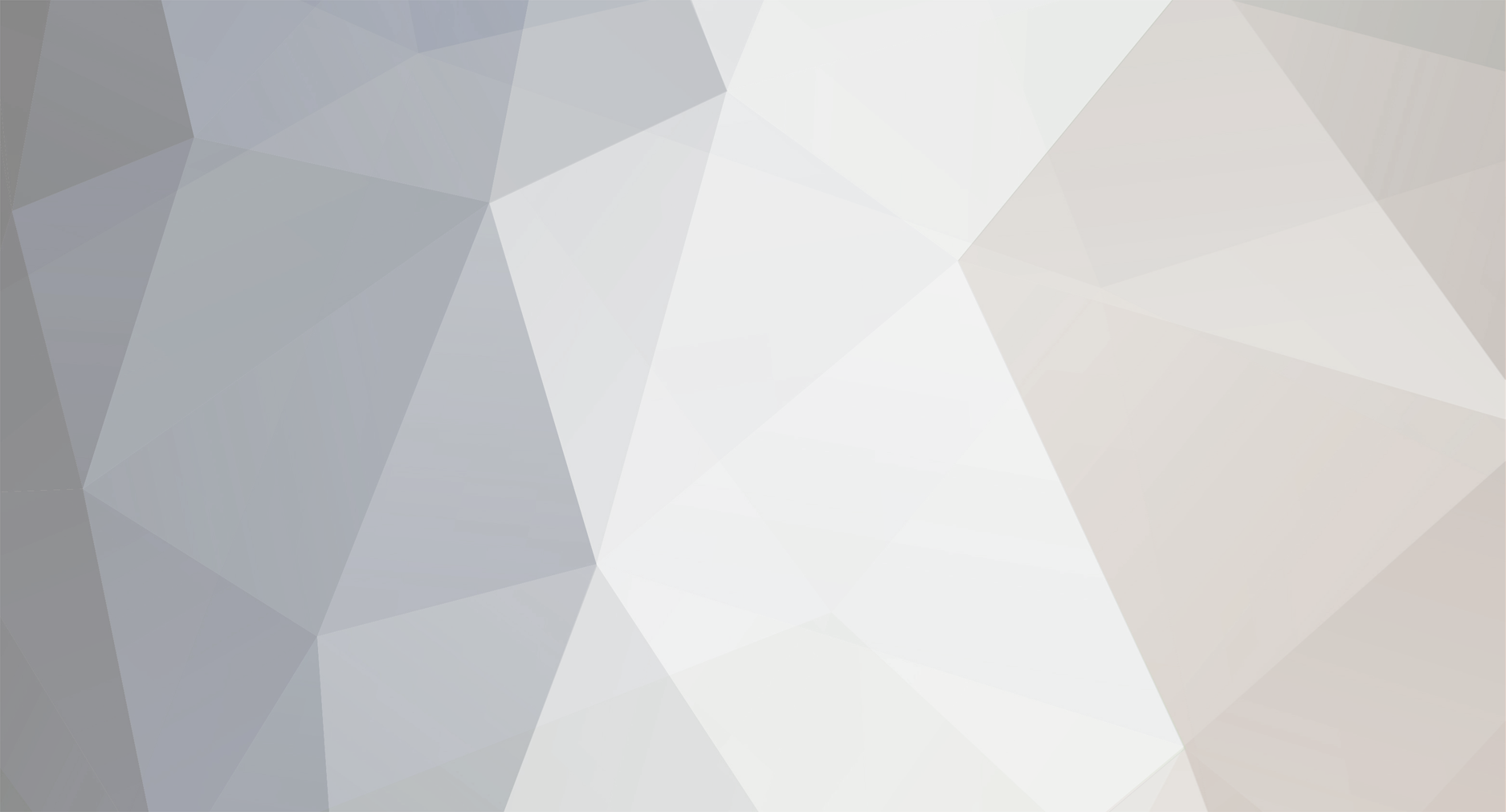
mbtaylor
-
Posts
96 -
Joined
-
Last visited
Never
Posts posted by mbtaylor
-
-
-
mbtaylor - You keep beating me to it by just a hair, hah.
-
We put
$this->Login = $value;
You put
$this->$Login = $value;
NOTE THE $!
-
You are accessing your properties wrong. It should be like this:
<?php class HAPI{ var $Login; var $Pass; var $Hapikey; var $Auth; function SetLogin($value){ $this->Login = $value; } function SetPass($value){ $this->Pass = $value; } function SetAuthKey($value){ $this->Authkey = $value; } } $hyp = new HAPI(); echo "1<br>"; $hyp->SetLogin("Username"); echo "2<br>Login: "; echo $hyp->Login; echo " <-"; ?>
-
Amen to Chris.
If you used substr with strpos you could find the position of the X and use that, but you would still have to call it twice one to get the beginning and one for the end whereas explode does it in one line and I believe is a simpler way to do it.
-
Yup, I'd use explode too
-
Try this:
${"addqty$cnt"}
If thats what I think you are meaning. You are wanting to create dynamic variable name, right?
-
or
if (strlen ($_POST['username']) > 0) { #username has a value }else{ # it doesnt }
Theres always a dozen ways to do the same thing
Bear in mind that you should ALWAYS check POST data for potential invalid input before doing anything with it. Otherwise you will get SQL injections, XSS attacks etc.
-
You need to watch your variable types. You are looking for a boolean e.g true/false not a string I presume so it should be:
if (!$usertrace){ echo ("\nInvalid User"); }
writing if (!$usertrace) is the same as saying if $usertrace isn't true do this.
Also try empty for the variable:
if (empty($_REQUEST['username'])) { echo ("\nInvalid User"); }
Though it could be because the is_null needs to be in the if brackets (ive not actually used the is_null function I either use empty or test for true/false).
-
I have recently written a multi lingual CMS system. The approach I used was to COMPLETELY SEPARATE the content and other structure. You would have one table containing say your productID, price, etc and a linking languageID which would link to a product_content table containing all the translations and text content for your products.
The separate languages would be separated by languageID and products linked to the product_content table by productID. They would be linked using a JOIN SQL statement.
So with this approach, you are not needing a separate table for each language, and are not duplicating anything in nice relational design.
It works in a similar way for pages and page content. Pages are definated in 1 table, and link to the language in a page_content table via pageID and languageID.
Does that make sense? Its basically using a 1:Many relationship for the product:text.
You then also need to think about labels, things like "Submit" etc on buttons. For that I had a labels table, which defined labels and the language text for each. You can then lookup the label text based on a keyword using an sql query.
-
Just my sense of humour.
-
-
-
if (mysql_num_rows ($query) > 0) { # do something }
-
* sigh *
-
Firstly use the code tags
if (!empty($image_out)) { header("Content-type: $image_type"); echo $image_out; } else if($current_category.categoryid eq "6325") header("Content-type: image/gif"); func_readfile($default_image6325, true); } else { header("Content-type: image/gif"); func_readfile($default_image, true); }
The problems looks like $current_category.categoryid is not a valid variable name.
It would have to be either $current_category or $categoryid, or maybe even $current_category_id
IF you are wanting to create a dynamic variable it would be ${"$ccurrent_category$categoryid"}.
-
Create a php page e.g image.php and insert this code:
<? $pic = $_GET['pic']; $width = $_GET['width']; if (strstr ($pic, "jpg")){ $im = imagecreatefromjpeg($pic); }else{ $im = imagecreatefromgif($pic); } $px = (imagesx($im) - 7.5 * strlen($string)) / 2; $old_x=imageSX($im); $old_y=imageSY($im); $new_w=(int)($width); if (($new_w<=0) or ($new_w>$old_x)) { $new_w=$old_x; } $new_h=($old_x*($new_w/$old_x)); $thumb_w=$new_w; $thumb_h=$old_y*($new_h/$old_x); $thumb=ImageCreateTrueColor($thumb_w,$thumb_h); imagecopyresampled($thumb,$im,0,0,0,0,$thumb_w,$thumb_h,$old_x,$old_y); imagejpeg($thumb,"",90); imagedestroy($thumb); header("Content-type: image/jpeg"); ?>
You can then resize images like:
http://mywebsites/image.php?pic=images/somepic.jpg&width=130
Added: This requires the GD library compiled into PHP
-
-
98% Compatible
-
CSS definately doesnt support Gifs as backgrounds.
I suggest you look at SWFObject:
-
This is basic stuff mate, I suggest you read the Zend 101 beginners tutorial and skim through appropriate sections of the PHP Manual.
devzone.zend.com/node/view/id/627
-
just test first if the checkbox var is empty or not before including it. You are getting the blank line from the \n.
if (!empty($checkbox1))$message .= "$checkbox1\n"; \\ and so on with all your vars mail ($message......)
-
You need to have your host change the php.ini file (if they will) there may be limits for a reason - e.g so users dont use all their resources uploading large files all the time.
You will need to get your host to change their IPTables rulesets to implement the iptables bits...
Get yourself a dedicated server and learn a bit of linux mate! You have total control then.
-
If you are just looking to round it use the round function. E.g:
$float = 1.999999999999999999999 $float = round ($float, 2); echo $float;
2 = the number of places to round to.
iterating through mysql_fetch_array
in PHP Coding Help
Posted
My preferred method is:
That will print out all elements from your query. You can also use the same to make your associative array elements into variables like: