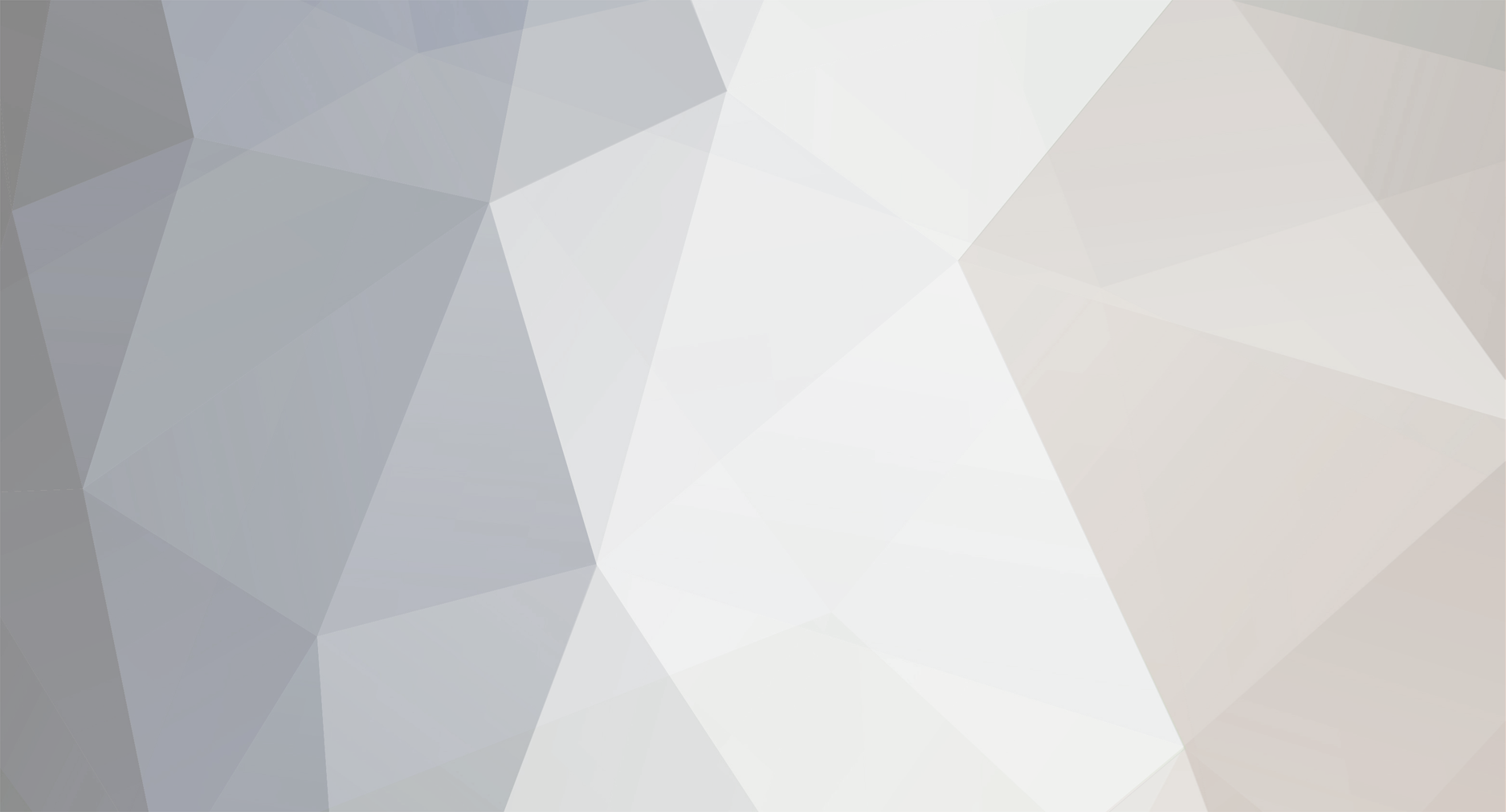
blue-genie
Members-
Posts
69 -
Joined
-
Last visited
Everything posted by blue-genie
-
<?php session_start(); function getRandom() { $maxVal= $_REQUEST['maxValue']; $random_number = rand(0, $maxVal); echo "$random_number"; } getRandom(); ?> if i test this with testRandom.php?maxVal=100 for example i keep getting 0 i'm not getting any syntax errors but if i hardcode the max value it works as expected. What am i doing wrong? blu.
-
this is what I opted for, not sure if it's the best way. <?php session_start(); include 'config.php'; include 'opendb.php'; $gameID= $_REQUEST['gameID']; $gameRNG= $_REQUEST['gameRNG']; $insert = mysql_query("INSERT INTO gameinstance (gameID, gameRNGResult, gameDateTime) VALUES ('$gameID', '$gameRNG', now())"); if(!$insert){ echo '<?xml version="1.0"?>'; echo '<dataxml>'; die("There's little problem: ".mysql_error()); $MyError = "An error occured while saving data. Please try again later." + mysql_error(); echo "<sqlError>".$MyError."</sqlError>"; echo '</dataxml>'; } else { $gameInstanceNo = mysql_insert_id(); printf("Last inserted record has id %d\n", mysql_insert_id()); mysql_query("UPDATE gameInstance SET gameInstance_No='$gameInstanceNo' WHERE USN_Gameinstance='$gameInstanceNo'"); echo '<?xml version="1.0"?>'; echo '<dataxml>'; $success = mysql_insert_id(); echo "<success>".$success."</success>"; echo '</dataxml>'; } ?>
-
oh sorry i didn't refresh my page, didn't see your response. what do you mean by let sql handle this? auto increment?
-
what i'm doing is before I insert a new row, i want to get the last value in the gameInstance_No field, increment that by 1 and do the insert. while I run the select statement it returns the value correctly however the insert is putting a zero. $sql = "SELECT gameInstance_No FROM gameinstance ORDER BY gameInstance_No DESC LIMIT 0,1"; $result = mysql_query($sql); if (mysql_num_rows($result)){ $gameInstanceNo = $row['gameInstance_No']+1; }else{ $gameInstanceNo = 0; } thanks.
-
I managed to get assistance from another forum. If you're encountering the same problem PM me for the result.
-
this is the closest i've got so far and it's inserting each time in the correct column but in different rows. //let's exclude the gameID for now as that works. so instead of inserting into (name, prize, odds) values(shark, sharkprize, 2); its creating 3 rows first: shark , 0, 0 second: 0, sharkprize, 0 third:0, 0, 2 <?php //session_start(); $debugMe=FALSE; include 'config.php'; include 'opendb.php'; //$gameID = $_REQUEST['gameID']; $gameID = 3; //this will set $arr to something like "Symbol=Shark,Odds=1,Prize=sharkprize" //$arr = $_REQUEST['arr']; $arr = "Symbol=Shark,Odds=1,Prize=sharkprize"; if( $debugMe ) { echo "<br><br>'arr' before explode"; var_dump($arr); } //break the string at the commas, giving you an array of three elements //after explode //$data[0]="Symbol=Shark" //$data[1]="Odds=1" //$data[2]="Prize=sharkprize" $data = explode(",", $arr); if( $debugMe ) { echo "<br><br>'data' after 'arr' explode"; var_dump($data); } //now iterate over each of the elements foreach($data as $k=>$v) { if( $debugMe ) { echo "<br>value of 'v' in foreach "; var_dump($v); } //break the value of each array at the "=" symbol. //If you have never used the "list()" construct, refer to: //http://www.php.net/manual/en/function.list.php list($key,$value)=explode("=",$v); //delete the "old" $data[$k] unset($data[$k]); //now append the $key and $value to $data[$k] $data[$k][$key]=mysql_real_escape_string($value); } if( $debugMe ) { echo "<br><br>'data' after foreach"; var_dump($data); exit; } echo '<?xml version="1.0"?>'; echo '<dataxml>'; if ( is_array( $data ) ) { foreach($data as $key=>$v) { echo '<forLoop>insidefor loop'.$data[$key]['Symbol'].'</forLoop>'; echo '<forLoop>insidefor loop'.$data[$key]['Prize'].'</forLoop>'; echo '<forLoop>insidefor loop'.$data[$key]['Odds'].'</forLoop>'; $insert = mysql_query("INSERT INTO gameprizes(name, prize, odds, gameID) VALUES('{$data[$key]['Symbol']}','{$data[$key]['Prize']}','{$data[$key]['Odds']}','{$gameID}')"); } if (!$insert) { echo "<sqlError>An error occured while saving data. Please try again later.". mysql_error() ."</sqlError>"; } else { echo "<success>prizes added</success>"; } } echo '</dataxml>'; exit; ?>
-
no the gameID is another variable that is parsed from the flash which i'm currently just hardcoding to test it. there is a prizeID that is not null auto increment.
-
hey ignace, thanks for getting back to me. I'm going to need more help then that, I'm clueless. where does that code snippet go in? my problem at the moment is that i'm putting bits and pieces together and stuffing it up. I'm a flash developer, and this is beyond my comfort zone, big time! if i stick that code in where I'm assuming it goes, i end up with the number 2 and my gameID being inserted into the db for each column. I'd really appreciate if you could dig me out of this massive hole i've created.
-
i've got bits and pieces of code from various places, but I'm not able to achieve the final outcome I require. As a non php person, I'm trying to fiddle with the bits of code I get but nothing seems to work. here's an example I got from another forum <?php include 'config.php'; include 'opendb.php'; $gameID = '1'; $arr = "Symbol=Shark,Odds=1,Prize=sharkprize&Symbol=Crab,Odds=2,Prize=crabprize"; $data = explode(",", $arr); foreach($data as $k=>$v) { list($key,$value)=explode("=",$v); //now append the $key and $value to $data[$k] $data[$k][$key]=mysql_real_escape_string($value); } echo '<?xml version="1.0"?>'; echo '<dataxml>'; if ( is_array( $data ) ) { foreach($data as $key=>$v) { $insert = mysql_query("INSERT INTO gameprizes(name, prize, odds, gameID) VALUES('{$data[$key]['Symbol']}','{$data[$key]['Odds']}','{$data[$key]['Prize']}','{$gameID}')"); } if (!$insert) { //die("There's little problem: ".mysql_error()); $MyError = "An error occured while saving data. Please try again later." + mysql_error(); echo "<sqlError>".$MyError."</sqlError>"; } else { echo "<success>prizes added</success>"; } } echo '</dataxml>'; exit; ?> I nedd Shark insertedinto name, 0 into odds, and sharkprize into prize, this code inserts S, S, 0, 1, 1, 1 and s, s, 1
-
okay am i even remotely close (This gives me an <b>Warning</b>: Invalid argument supplied for foreach() in <b>C:\xampp\htdocs\... error) when testing in browser <?php session_start(); include 'config.php'; include 'opendb.php'; $gameID = $_REQUEST['gameID']; $arr = $_REQUEST['arr']; $data = explode(",", $arr); foreach($data as $k => $v) { $data[$k] = explode("=", $v); foreach($raw[$key] as $value){ $info = explode('=', $value); $data[$key][$info['0']] = $info['1']; } } echo '<?xml version="1.0"?>'; echo '<dataxml>'; if ( is_array( $data ) ){ $n = count($data); for ( $i = 1; $i < $n; $i++ ){ $key = 'i'.$i; // Read the various keys, you should know what to expect! // if( !empty($data[$key]['Symbol'])){ $insert = mysql_query('INSERT INTO gameprizes(name, prize, odds, gameID) VALUES("'.$data[$key]['Symbol'].'","'.$data[$key]['Odds'].'", "'.$data[$key]['Prize'].'", "'.$gameID.'")'); } } } if (!$insert) { die("There's little problem: ".mysql_error()); $MyError = "An error occured while saving data. Please try again later." + mysql_error(); echo "<sqlError>".$MyError."</sqlError>"; } else { echo '</dataxml>'; } ?>
-
Matthew can you help me out here please. I'm going around in circles i need to loop through the array and do an insert. if there's an error i do an echo '<?xml version="1.0"?>'; echo '<dataxml>'; die("There's little problem: ".mysql_error()); $MyError = "An error occured while saving data. Please try again later." + mysql_error(); echo "<sqlError>".$MyError."</sqlError>"; echo '</dataxml>'; once all the inserts are done i need to echo another bit of xml to say it's done. currently the insert never happens and i'm not sure where to put the success message since it's in a loop and i end up with mailformed xml.
-
flash is sending some variables to php. $gameID = $_REQUEST['gameID'];//gets this as 1, 2 etc $arr = $_GET['arr'];//gets this as i1=Symbol=Octopus222,Odds=2,Prize=puss&i2=Symbol=Lobster222,Odds=2,Prize=puss i need to convert this to an array. i tried $data = array($arr); didn't work. if i echo $data i get Array
-
mikesta707, thanks for the pointer but I can get the variables from flash into php. I'm struggling with spliting the string I Post to PHP for PHP to insert it. I've been fiddling a bit, and testing in my browser with the following URL. http://localhost/fishing/fishing/savePrizes.php?i1=Symbol=Oyster,Odds=1,Prize=test1&i2=Symbol=Sardine,Odds=2,Prize=test2&i3=Symbol=Lobster,Odds=4,Prize=test4&i4=Symbol=skeleton,Odds=5,Prize=test5 my resulting echos: Array ( [i1] => Array ( [symbol] => Oyster [Odds] => 1 [Prize] => test1 ) [i2] => Array ( [symbol] => Sardine [Odds] => 2 [Prize] => test2 ) [i3] => Array ( [symbol] => Lobster [Odds] => 4 [Prize] => test4 ) [i4] => Array ( [symbol] => skeleton [Odds] => 5 [Prize] => test5 ) ) Array size with count: 4inside for loopinside not empty should insert Oysterinside for loopinside not empty should insert Sardineinside for loopinside not empty should insert Lobster so it's getting the values but the insert is not happening, and it's not giving me any errors either. here's my PHP thus far: <?php session_start(); include 'config.php'; include 'opendb.php'; $gameID = $_REQUEST['gameID']; $data = array(); foreach($_GET as $key => $value){ $raw[$key] = explode(',' ,$_GET[$key]); foreach($raw[$key] as $value){ $info = explode('=', $value); $data[$key][$info['0']] = $info['1']; } } print_r($data); if ( is_array( $data ) ){ // Find the number of keys in the array // echo "Array size with count: ".count($data); $n = count($data); for ( $i = 1; $i < $n; $i++ ){ echo "inside for loop"; $key = 'i'.$i; // Read the various keys, you should know what to expect! // if( !empty($data[$key]['Symbol'])){ echo "inside not empty should insert ".$data[$key]['Symbol']; $sql = 'INSERT INTO gamePrizes(name, prize, odds, gameID) VALUES("'.$data[$key]['Symbol'].'","'.$data[$key]['Odds'].'", "'.$data[$key]['Prize'].'",, "'.$gameID.'",)'; }else{ $errorMsg = "Error inserting into db ".sql.error(); echo $errorMsg; } } } ?>
-
Hi Will. Yes the flash sends the data through via a post. i can get the variable back to php, i'm thinking in this format i1=Symbol=Oyster,Odds=1,Prize=test1&i2=Symbol=Sardine,Odds=2,Prize=test2&i3=Symbol=Lobster,Odds=4,Prize=test4&i4=Symbol=skeleton,Odds=5,Prize=test5 but what I need help with is that php then takes that and does an INSERT into db with that data. So i'm assuming it needs to be converted to an array or somethign that PHP can loop through. I don' t know how to do that. PHP will get it as a string.
-
Here's my predicament. I have a flash form that pull data via php and displays some data. The user then selects various items and it populates a datagrid component (in flash), now I need to be able to save that selection back to the database. I was told PHP can't accept an array from flash. So here's my question. I can format the results any which way , i.e. string/array etc to send to the PHP.I need to know on the php side. 1. what format is best for large amount of data from a datagrid. 2. how to take that data and do an insert into in using a loop or whatever. total noob so keep it to 1st grader terminology if you can assist please. thanks :-)