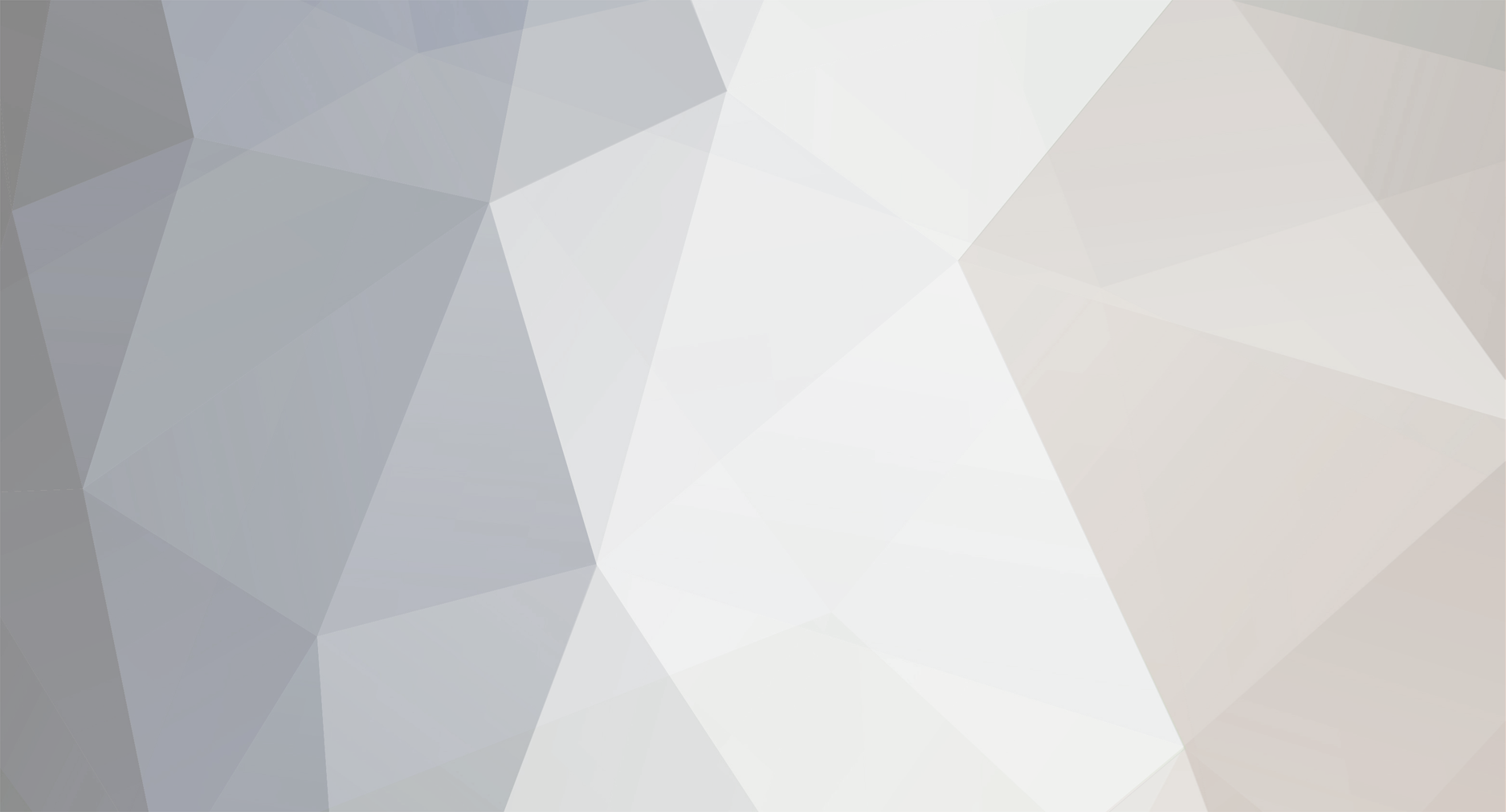
jakebur01
Members-
Posts
885 -
Joined
-
Last visited
Everything posted by jakebur01
-
Disregard that. The array of zip codes is in the correct order. It's my IN() in the mysql query I'm using to pull the users within those zip codes that throwing it out of order. $sql = "SELECT * FROM `users` WHERE `ZIPCODE` IN($List)"; I changed it to: SELECT * FROM `users` WHERE `ZIPCODE` IN($List) ORDER BY FIELD(ZIPCODE, $List) It's working now! Thanks Kicken and to everyone else that helped.
-
Hey Kicken, it’s pulling the zip codes now but not sorting by distance.
-
Thanks, but I’d rather solve this and pull from my own database.
-
$sql = ' SELECT DISTINCT zip FROM ( SELECT zip, (3958*3.1415926*sqrt((latitude-?)*(latitude-?) + cos(latitude/57.29578)*cos(?/57.29578)*(longitude-?)*(longitude-?))/180) as distance FROM zip_codes ) r WHERE r.distance < ? ORDER BY r.distance '; $stmt = $this->db->prepare($sql); $stmt->bind_param('dddddd', $zip->latitude, $zip->latitude, $zip->latitude, $zip->longitude, $zip->longitude, $radius); $stmt->bind_result($rowZip); $stmt->execute(); $zip_codes = []; while ($stmt->fetch()){ $zip_codes[] = $rowZip; } return $zip_codes;
-
Hey, that listed them. Although, they are still not listed by nearest. They are all random.
-
What is it looking for? Is it thinking that is a function, or looking for a database table?
-
Fatal error: Uncaught mysqli_sql_exception: FUNCTION imsafe.zip does not exist in /home/zipclass.php:99 Stack trace: #0 /home/zipclass.php(99): mysqli->prepare('\n SELECT...') #1 /home/zipclass.php(34): ZipCodeUtility->get_zipcodes_by_radius(Object(stdClass), 100) #2 /home/TOOL_zipcodetest.php(45): ZipCodeUtility->search_radius(71075, 100) #3 {main} thrown in /home/zipclass.php on line 99 imsafe is the name of the database the table is zip_codes
-
Fatal error: Uncaught Error: Call to a member function bind_param() on boolean
-
How can I order this query of zip codes within the radius by nearest? /** * Get Zipcode By Radius * @param string $zip US zip code * @param ing $radius Radius in miles * @return array List of nearby zipcodes */ protected function get_zipcodes_by_radius ( $zip, $radius ) { $sql = 'SELECT distinct(zip) FROM zip_codes WHERE (3958*3.1415926*sqrt((latitude-'.$zip->latitude.')*(latitude-'.$zip->latitude.') + cos(latitude/57.29578)*cos('.$zip->latitude.'/57.29578)*(longitude-'.$zip->longitude.')*(longitude-'.$zip->longitude.'))/180) <= '.$radius.';'; $zip_codes = array(); if ( $result = $this->db->query( $sql ) ) { while( $row = $result->fetch_object() ) { array_push( $zip_codes, $row->zip ); } $result->close(); } return $zip_codes; }
-
Nevermind. I figured it out. You make your links to your page as the friendly links and htaccess reads and converts the seo friendly link or directs it to the appropriate page.
-
Ok. My input is https://www.test.com/listings.php?ident=TEST&facility=library. I'd like the output to be: https://www.test.com/TEST/LIBRARY. I'd like to make my urls seo friendly.
-
I'm attempting to rewrite my urls but don't seem to be getting it to work. I've tried: RewriteEngine On RewriteRule /(.*)/(.*)/$ listings.php?ident=$1&facility=$2 And I've also tried: RewriteEngine On RewriteRule ^([^/\.]+)/([^/\.]+)?$ listings.php?ident=$1&facility=$2 Any suggestions?
-
Of course, these same users will need to be able to edit and or add some directory listings. I can try to build it from scratch, but it sure would save me a lot of time if there is something already out there I could implement.
-
I have an idea for a site that is sort of like a directory which I already have built. I'd like to incorporate some social media into it allowing each directory listing to get comments and star ratings. I don't like envato/code canyon packaged sites. I'd rather be flexible and mostly use all of my own code. However, I have seen a star rating class on phpclasses, but I have not come across a user/comment class or script yet that I can customize into my directory listings. If I can find a class I can add on to my site that already has a user profile, and all of the ajax and css for commenting/rating, I would use if it I could customize it into the site I already have. I'd just rather not use an entire social media site someone has built then try to add my code and pages into it and have to depend on the developer for support. Does anyone know of any rating/commenting classes or have any suggestions?
-
Yea. I guess I could add a button to the landing page. Click here to activate.
-
There are two pages. auth_registration and auth_activate. I myself submit the form on auth_registration using one of my emails and immediately the auth_activate page is accessed. I'm suspect of these facebook and google ad trackers that track registration and purchase: <script async src="https://www.googletagmanager.com/gtag/js?id=AW-36573573"></script> <script> window.dataLayer = window.dataLayer || []; function gtag(){dataLayer.push(arguments);} gtag('js', new Date()); gtag('config', 'AW-3763567'); </script> <!-- Facebook Pixel Code --> <script> !function(f,b,e,v,n,t,s) {if(f.fbq)return;n=f.fbq=function(){n.callMethod? n.callMethod.apply(n,arguments):n.queue.push(arguments)}; if(!f._fbq)f._fbq=n;n.push=n;n.loaded=!0;n.version='2.0'; n.queue=[];t=b.createElement(e);t.async=!0; t.src=v;s=b.getElementsByTagName(e)[0]; s.parentNode.insertBefore(t,s)}(window, document,'script', 'https://connect.facebook.net/en_US/fbevents.js'); fbq('init', '36573737376'); fbq('track', 'PageView'); </script> <noscript><img height="1" width="1" style="display:none" src="https://www.facebook.com/tr?id=65474567&ev=PageView&noscript=1" /></noscript> <!-- End Facebook Pixel Code -->
-
Yes. It is the full link with the variables in the url that is being run.
-
The bot would have to be accessing the page at the moment the user is clicking the submit button on the register page. How does the bot know the page exists?
-
The link just contains their id url encoded and the activation page checks to see if it’s already activated. This IP address is accessing the page the moment the user is registered and the email is sent out to them from the registration page. Another user signed up and a different ip address accessed the activation page the moment their check your email for verification email with the link was sent to them. I looked that address up and it was also from amazon data.
-
I have a registration page that mails a link to the users email. The link in the email takes the user to the activation page which sends out a welcome email and updates the database as activated if the user is not already activated. For some reason, this page is being accessed by an Amazon ip address with multiple browsers in the user_agent. Whatever this is is automatically activating my users and sending them a welcome email without them even accessing the page. The only suspect I can think of is I have a javascript snippit for google ads and also facebook on some of my pages. I don't know if they could somehow be accessing the page or not. It must be following the activation link in the mail() script I have on the registration page. I'm mailing myself the following when the activation page is accessed. I get an amazon ip, multiple browsers, and no referer. $browser = $_SERVER['HTTP_USER_AGENT']; $ip = $_SERVER['REMOTE_ADDR']; $referer = $_SERVER['HTTP_REFERER'];
-
No, I don't. But I'm learning. It has the x-www-form-urlencoded header. It then is json encoded and has the json header. So, I guess it used both.
-
This did it: https://www.google.com/amp/s/www.geeksforgeeks.org/how-to-receive-json-post-with-php/amp/
-
I have some data I'm posting from Swift to my php file via x-www-form-urlencoded. How can I decode this POST data?
-
I’m getting ready to play around with swift and php. I want to do a basic login and send some data back and forth. It doesn’t look that complicated. Swift sends a request, php processes it and returns it in a json format. What about authentication. Has anyone had experience with this? When you POST via urlsession or alamofire a password for php to handle, is that post text not something someone could capture in between. What’s the best and simplest way to approach all of this?
-
I believe I may have it. $originalstart = date("Y-m-d"); $originalstart = date("Y-m-d",strtotime('-24 months', strtotime($originalstart))); for( $i= 0 ; $i <= 25 ; $i++ ) { $start = date('Y-m-01',strtotime('+'."$i".' month', strtotime($originalstart))); $firstday = date('Y-m-d', strtotime('next Sunday', strtotime('last Friday', strtotime('first day of last month', strtotime($start))))); $lastday = date('Y-m-d', strtotime('next Saturday', strtotime('last Friday', strtotime('first day of this month', strtotime($start))))); echo "$firstday - $lastday<br />"; } 2017-10-01 - 2017-10-28 2017-10-29 - 2017-11-25 2017-11-26 - 2017-12-30 2017-12-31 - 2018-01-27 2018-01-28 - 2018-02-24 2018-02-25 - 2018-03-31 2018-04-01 - 2018-04-28 2018-04-29 - 2018-05-26 2018-05-27 - 2018-06-30 2018-07-01 - 2018-07-28 2018-07-29 - 2018-09-01 2018-09-02 - 2018-09-29 2018-09-30 - 2018-10-27 2018-10-28 - 2018-12-01 2018-12-02 - 2018-12-29 2018-12-30 - 2019-01-26 2019-01-27 - 2019-02-23 2019-02-24 - 2019-03-30 2019-03-31 - 2019-04-27 2019-04-28 - 2019-06-01 2019-06-02 - 2019-06-29 2019-06-30 - 2019-07-27 2019-07-28 - 2019-08-31 2019-09-01 - 2019-09-28 2019-09-29 - 2019-10-26 2019-10-27 - 2019-11-30