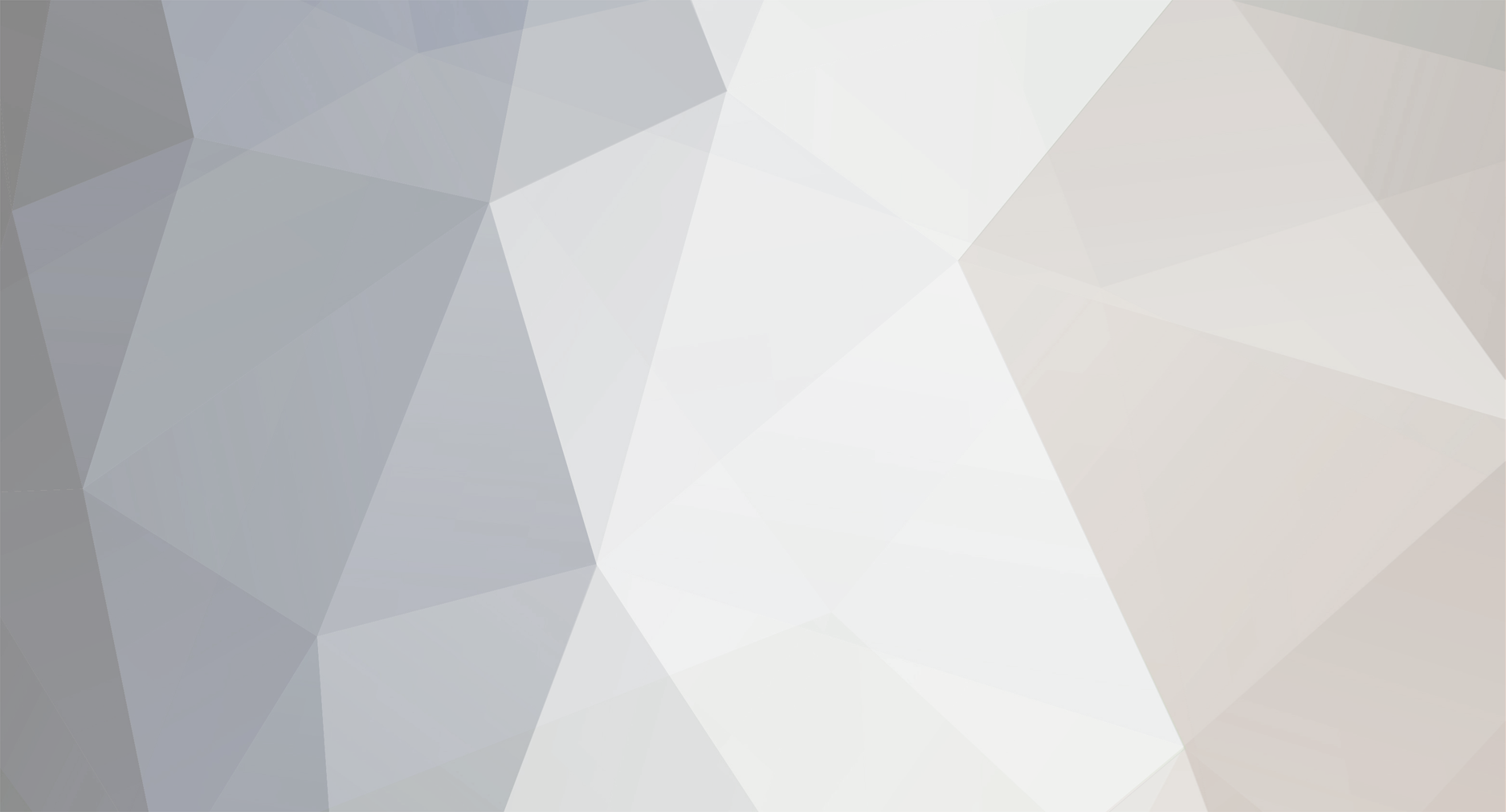
ShoeLace1291
-
Posts
401 -
Joined
-
Last visited
Posts posted by ShoeLace1291
-
-
So I have a query that pulls info from three different tables. The first table is clan matches, second is match players, and third is members. Clan matches is the main table for the query. Match players has a column called matchID, so this table keeps track of each member that played in each match. Then the third join selects from members which gets their username.
The players table has a column called expEarned, and I would like to get the row containing the highest integer value from that column equal to the appropriate match ID.
I tried out the GREATEST function but I got an SQL error that says this:
Incorrect parameter count in the call to native function 'GREATEST'I've never used the "greatest" function but based on the MYSQL manual, it should be the function I am looking for, right? I guess there's more to it than calling the function with the table column as the parameter. Here's my code:
$recent = db_query(" SELECT m.ID_MATCH AS ID_MATCH, m.opponentName AS opponentName, m.roundWins AS wins, m.roundLosses AS losses, m.kills AS kills, m.losses AS losses, mp.ID_MEMBER, mp.expErned AS mvpEXP, mem.ID_MEMBER AS mvpID, mem.realName AS mvpName FROM {$db_prefix}clan_matches AS M LEFT JOIN {$db_prefix}clan_match_players AS mp ON (GREATEST(mvpEXP)) LEFT JOIN {$db_prefix}members AS mem ON (mvpID = mp.ID_MEMBER) WHERE m.scheduledTime < ".time()." ORDER BY m.ID_MATCH DESC LIMIT 10", __FILE__, __LINE__);
If you haven't figured it out, I'm making a mod for SMF... lol.
-
I was wondering if there was a way to round whole numbers in increments. I need to make a progress bar based on percentages and for every ten percent of progress that is made, I want to add a bar. So for example:
38% would display four bars
21% would display two bars
15% would display one bar.
Are there any PHP functions like this?
-
I'm using a PHP foreach loop to set Javascript array values from PHP array values The problem is that some of the array values contain double quotes(") so it compromises the JS script. Is there a PHP function that automatically escapes each double quote in a given string?
-
So I have a Javascript function that calls an ajax file. This function has two parameters, url, and div. This function calls the statechange function in which the div variable is passed to. In firebug I get an error saying that the div variable is null on one line, but not on other lines that it is used. Here is my source code:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Site Name - Official Website(Beta)</title> <link rel='stylesheet' href='template/style.css' /> <script type='text/javascript'>window.onload = loadAjax('http://localhost/ajaxtest.php', 'test'); function loadAjax(url, divid){ if(window.XMLHttpRequest){ xmlhttp = new XMLHttpRequest(); } else { xmlhttp=new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.onreadystatechange = stateChange(divid); xmlhttp.open("GET",url,true); xmlhttp.send(); } function stateChange(divid){ var area = document.getElementById(divid ); if (xmlhttp.readyState==4 && xmlhttp.status==200){ area.innerHTML=xmlhttp.responseText; } else if (xmlhttp.readyState == 4 && xmlhttp.status == 404){ area.innerHTML = ""; }else if(xmlhttp.readyState != 4){ area.innerHTML = '<img src="http://localhost/ceasarssoldiers/template/images/ajax-loader.gif">'; } } </script> </head> <body> <div id='container'> <div id='header'> <a href='http://example.com/index.php' title='Home'><img src='http://localhost/template/images/banner_logo.png' width='543' height='77' alt="Ceasar's Soldiers.com - Official website beta." border='0' /></a> </div> <div id='navigation'> <ul> <li><a href='#'>Home</a></li> <li><a href='#'>Forums</a></li> <li><a href='#'>Rosters</a></li> <li><a href='#'>Matches</a></li> <li><a href='#'>Challenge</a></li> <li><a href='#'>Enlist</a></li> <li><a href='#'>Login</a></li> <li><a href='#'>Support</a></li> </ul> </div> <div id='test'>asdf</div>
Error Console in Firebug says that Area is null on line 33 which is this:
area.innerHTML = '<img src="http://localhost/ceasarssoldiers/template/images/ajax-loader.gif">';
The purpose of that line is to display the ajax loading image while the page is being loaded. Why is Area null on this line, but not the others?
-
So I was wondering how to get all elements that have an attribute with a certain value. For example, how would I find all elements that have a rel attribute with the value of 'ajax'? I need to find all elements with rel='ajax' in them so I can add an onclick function to all of them.
-
I found a independently created PHP class for the Steam Community API. I tried using it on my installation of Xampp and got the following error:
A non-blocking socket operation could not be completed immediately.I proceeded to try uploading it to a web server and it worked fine. This is the code of the API class:
<?php//====================================================================================
// PHP STEAM API CLASS
//====================================================================================
// Author: |LBTG|Regime (regime@livebythegun.com)
// Version: 0.1
// Date: 07/06/2009
//====================================================================================
// Notes: This is a class that allows you to easily query the Steam community
// API from PHP. It is required that your PHP installation/configuration allows for
// the use of sockets. If it doesn't you could ask your hosting provider to enable
// them for you, or alternatively feel free to re-write this class to use something
// like cURL.
// An example on how to use this class has been provided with this package. There
// should not be any need to edit anything in this file.
//====================================================================================
// Thanks: Many thanks to Valve for providing us with the Steam community API.
// Also thanks to voogru for publishing the algorythm to calculate 64 bit steam ID's.
//====================================================================================
// http://www.steampowered.com/
// http://www.steamcommunity.com/
// http://www.livebythegun.com/
//====================================================================================
Class SteamAPI
{
//- Time out in seconds
public $timeout = 3;
//- API hostname
private $hostname = "steamcommunity.com";
private $socket, $ip_address, $service_port;
/*
-- Function: constructor
-- Purpose: Called when class is created. Used to set some default values.
-- Arguments: none
-- Returns: void
*/
public function __construct()
{
//- Get the IP address for steamcommunity.com
$this->ip_address = gethostbyname($this->hostname);
//- Get the port for the WWW service
$this->service_port = getservbyname('www', 'tcp');
}
/*
-- Function: get_profile_data
-- Purpose: Retrieves profile data from the Steam API for the specified steam ID.
-- Arguments: $steam_id - A steam ID to perform the API call for
-- Returns: An array filled with the profile data, or null if the profile does not exist
*/
public function get_profile_data($steam_id)
{
//- Calculate the 64 bit steam ID
$steam_id64 = $this->get_steamid64($steam_id);
if (!$steam_id64)
{
throw New Exception('Invalid steam ID provided');
}
//- Construct HTTP request
$request = "GET /profiles/" . $steam_id64 . "?xml=1 HTTP/1.1\r\n";
$request .= "Host: " . $this->hostname . "\r\n";
$request .= "Connection: Close\r\n\r\n";
//- Do HTTP request
$this->steam_connect();
$response = $this->steam_request($request);
$this->steam_disconnect();
//- Check if a 302 response is given. This happens if the user has a 'Custom URL' set in their profile.
//- If this is the case, make another request to the custom URL
if (substr($response,0,12) == "HTTP/1.1 302")
{
//- Get the custom URL from the response
if (preg_match( '@Location: http://steamcommunity.com/id/([a-zA-Z0-9_-]+)/@', $response, $location))
{
$custom_url = $location[1];
}
else
{
throw New Exception('Custom URL redirection, but no location found.');
}
//- Construct HTTP request
$request = "GET /id/" . $custom_url . "?xml=1 HTTP/1.1\r\n";
$request .= "Host: " . $this->hostname . "\r\n";
$request .= "Connection: Close\r\n\r\n";
//- Do HTTP request
$this->steam_connect();
$response = $this->steam_request($request);
$this->steam_disconnect();
}
$this->steam_validate($response);
//- If there is no profile XML element, something is not right
if (!preg_match( '@<profile>(.+)</profile>@s', $response, $xmlArray))
{
throw New Exception('Could not parse returned steam community data. XML Root node missing.');
}
//- Store XML without headers
$xml_data = $xmlArray[0];
//- Parse XML. An array of data is returned
$data_array = $this->parse_xml($xml_data);
//- Return the data
return $data_array;
}
/*
-- Function: parse_xml
-- Purpose: Populate an array with the data found in the supplied XML
-- Arguments: $xml_data - The XML data to parse
-- Returns: An array of data from the XML
*/
private function parse_xml($xml_data)
{
//- Create an XMLReader object to parse the XML with
$reader = new XMLReader();
//- Load the XML into it
try {
$reader->XML($xml_data);
}
catch (Exception $e)
{
throw New Exception('Could not parse returned XML: ' . $e->getMessage());
}
//- Initialize an array for the profile data
$data_array = array();
//- Start reading through the XML
while ($reader->read())
{
//- First encounter the root element
if ($reader->nodeType == XMLReader::ELEMENT)
{
$rootnode_name = $reader->name;
//- Read through its subnodes
while ($reader->read())
{
if ($reader->nodeType == XMLReader::ELEMENT)
{
//- Store the name of the subnode
$elementName = $reader->name;
//- Check for sections that will result in a recursive multi dimensional array
if ( $elementName == "friends" || $elementName == "weblinks" ||
$elementName == "groups" || $elementName == "mostPlayedGames" )
{
//- Initialize an array for the data
$sub_array = null;
$sub_array = array();
$sub_counter = 0;
while ($reader->read())
{
//- Check for subnodes
if ($reader->nodeType == XMLReader::ELEMENT &&
($reader->name == "friend" ||
$reader->name == "weblink" ||
$reader->name == "group" ||
$reader->name == "mostPlayedGame"))
{
while ($reader->read())
{
//- Get the elements from the subnodes
if ($reader->nodeType == XMLReader::ELEMENT)
{
$subElementName = $reader->name;
//- The node is not empty so an end node is required
//- Consider the data invalid until it is found
$validDatafield = false;
while ($reader->read())
{
//- Check for the subnode value
if ($reader->nodeType == XMLReader::TEXT
|| $reader->nodeType == XMLReader::CDATA
|| $reader->nodeType == XMLReader::WHITESPACE
|| $reader->nodeType ==
XMLReader::SIGNIFICANT_WHITESPACE)
{
//- Store the value
$elementValue = $reader->value;
}
//- Check if this is the subnode end element
else if ($reader->nodeType == XMLReader::END_ELEMENT
&& $reader->name == $subElementName)
{
//- If so the subnode is complete
$validDatafield = true;
break;
}
}
//- If this is a valid subnode, store it in the data array
if ($validDatafield)
{
$sub_array[$sub_counter][$subElementName] =
$elementValue;
$elementValue = '';
}
}
else if ($reader->nodeType == XMLReader::END_ELEMENT &&
($reader->name == "friend" ||
$reader->name == "weblink" ||
$reader->name == "group" ||
$reader->name == "mostPlayedGame" ))
{
$sub_counter++;
break;
}
}
}
else if ($reader->nodeType == XMLReader::END_ELEMENT
&& $reader->name == $elementName)
{
$validDatafield = false;
$data_array[$elementName] = $sub_array;
break;
}
}
}
//- Check for sections that will result in a recursive single dimensional array
else if ( $elementName == "inGameInfo" || $elementName == "favoriteGame" )
{
//- Initialize an array for the data
$sub_array = null;
$sub_array = array();
while ($reader->read())
{
//- Get the elements from the subnodes
if ($reader->nodeType == XMLReader::ELEMENT)
{
$subElementName = $reader->name;
//- The node is not empty so an end node is required
//- Consider the data invalid until it is found
$validDatafield = false;
while ($reader->read())
{
//- Check for the subnode value
if ($reader->nodeType == XMLReader::TEXT
|| $reader->nodeType == XMLReader::CDATA
|| $reader->nodeType == XMLReader::WHITESPACE
|| $reader->nodeType ==
XMLReader::SIGNIFICANT_WHITESPACE)
{
//- Store the value
$elementValue = $reader->value;
}
//- Check if this is the subnode end element
else if ($reader->nodeType == XMLReader::END_ELEMENT
&& $reader->name == $subElementName)
{
//- If so the subnode is complete
$validDatafield = true;
break;
}
}
//- If this is a valid subnode, store it in the data array
if ($validDatafield)
{
$sub_array[$subElementName] = $elementValue;
$elementValue = '';
}
}
else if ($reader->nodeType == XMLReader::END_ELEMENT &&
( $reader->name == "inGameInfo" ||
$reader->name == "favoriteGame" ))
{
$validDatafield = false;
$data_array[$elementName] = $sub_array;
break;
}
}
}
//- Check for single nodes that do not require a recursive array to be used
else
{
//- An end node is required
//- Consider the data invalid until it is found
$validDatafield = false;
while ($reader->read())
{
//- Check for the subnode value
if ($reader->nodeType == XMLReader::TEXT
|| $reader->nodeType == XMLReader::CDATA
|| $reader->nodeType == XMLReader::WHITESPACE
|| $reader->nodeType == XMLReader::SIGNIFICANT_WHITESPACE)
{
//- Store the value
$elementValue = $reader->value;
}
//- Check if this is the subnode end element
else if ($reader->nodeType == XMLReader::END_ELEMENT
&& $reader->name == $elementName)
{
//- If so the subnode is complete
$validDatafield = true;
break;
}
}
//- If this is a valid subnode, store it in the data array
if ($validDatafield)
{
$data_array[$elementName] = $elementValue;
$elementValue = '';
}
}
}
//- Check for the final rootnode end element
else if ($reader->nodeType == XMLReader::END_ELEMENT && $reader->name == $rootnode_name)
{
break;
}
}
}
}
//- Close the XMLReader
$reader->close();
//- Add the regular steam ID to the array as well
$data_array['steamID32'] = $this->calculate_steamid($data_array['steamID64']);
return $data_array;
}
/*
-- Function: get_steamid64
-- Purpose: Check whether we have a regular steam ID, or a 64 bit steam ID and return a 64 bit steam ID either way
-- Arguments: $steam_arg - Either a regular, or a 64 bit steam ID
-- Returns: The 64 bit steam ID, or false if an invalid argument is provided
*/
private function get_steamid64($steam_arg)
{
if (preg_match('/^STEAM_[0-9]:[0-9]:[0-9]{1,}/i', $steam_arg))
{
return $this->calculate_steamid64($steam_arg);
}
else if (preg_match('/^76561197960[0-9]{6}$/i', $steam_arg))
{
return $steam_arg;
}
else
{
return false;
}
}
/*
-- Function: calculate_steamid64
-- Purpose: Translate a steam ID to a 64 bit steam id as used by Valve
-- Arguments: $steam_id - The steam ID to translate
-- Returns: The 64 bit steam ID, or false if an invalid steam ID is provided
*/
public function calculate_steamid64($steam_id)
{
if (preg_match('/^STEAM_[0-9]:[0-9]:[0-9]{1,}/i', $steam_id))
{
$steam_id = str_replace("_", ":", $steam_id);
list($part_one, $part_two, $part_three, $part_four) = explode(':', $steam_id);
$result = bcadd('76561197960265728', $part_four * 2);
$result = bcadd($result, $part_two);
return bcadd($result, $part_three);
}
else
{
return false;
}
}
/*
-- Function: calculate_steamid
-- Purpose: Translate a 64 bit steam ID to a steam id as used by Valve
-- Arguments: $steam_id64 - The 64 bit steam ID to translate
-- Returns: The steam ID, or false if an invalid 64 bit steam ID is provided
*/
public function calculate_steamid($steam_id64)
{
if (preg_match('/^76561197960[0-9]{6}$/i', $steam_id64))
{
$part_one = substr( $steam_id64, -1) % 2 == 0 ? 0 : 1;
$part_two = bcsub( $steam_id64, '76561197960265728' );
$part_two = bcsub( $part_two, $part_one );
$part_two = bcdiv( $part_two, 2 );
return "STEAM_0:" . $part_one . ':' . $part_two;
}
else
{
return false;
}
}
/*
-- Function: steam_connect
-- Purpose: Initialize a connection to the Steam API
-- Arguments: none
-- Returns: void
*/
private function steam_connect()
{
//- Get the TCP protocol (can also use SOL_TCP instead)
$protocol = getprotobyname('tcp');
//- Create a socket
$this->socket = socket_create(AF_INET, SOCK_STREAM, $protocol);
if ($this->socket === false)
throw New Exception('Could not create socket. Reason: ' . socket_strerror(socket_last_error()));
//- The socket should time out after 3 seconds, so make it non blocking for now
socket_set_nonblock($this->socket);
$error = NULL;
$attempts = 0;
//- The time out value has to be in milliseconds
$timeout_ms = $this->timeout * 1000;
$connected;
//- Connect the socket to the steam server
while (!($connected = @socket_connect($this->socket, $this->ip_address, $this->service_port)) && $attempts++ < $timeout_ms)
{
$error = socket_last_error();
//- If the error is different from the below, there is a problem
if ($error != SOCKET_EINPROGRESS && $error != SOCKET_EALREADY)
{
socket_close($this->socket);
throw New Exception('Could not connect to steam community: ' . socket_strerror($error));
exit;
}
//- Wait 1 second between attempts
usleep(1000);
}
//- If it's still not connected then consider the connection timed out
if (!$connected)
{
socket_close($this->socket);
throw New Exception('Connection to steam community timed out.');
exit;
}
//- Make the socket blocking again
socket_set_block($this->socket);
}
/*
-- Function: steam_request
-- Purpose: Sends a HTTP request to the Steam API
-- Arguments: $request_date - The HTTP request to be sent
-- Returns: The 'raw' response data received from the Steam API
*/
private function steam_request($request_data)
{
//- Write the request to the socket
socket_write($this->socket, $request_data, strlen($request_data));
//- Store the response
$buffer = '';
$response = '';
while ($buffer = socket_read($this->socket, 2048))
{
$response .= $buffer;
}
return $response;
}
/*
-- Function: steam_validate
-- Purpose: Checks the HTTP response code to see if the API request was succesful
-- Arguments: $response_data - The response to be validated
-- Returns: void
*/
private function steam_validate($response_data)
{
//- Check if the HTTP response code is 2xx. If so the request was a success
if (!preg_match('@^HTTP/1.1 2[0-9]{2}@s', $response_data))
{
throw New Exception('Request made to steam community failed (HTTP response code ' .
substr($this->response, 9, 3) . ')');
}
}
/*
-- Function: steam_disconnect
-- Purpose: Disconnect from the Steam API
-- Arguments: none
-- Returns: void
*/
private function steam_disconnect()
{
//- Close the socket
socket_close($this->socket);
}
}
?>
-
You probably want to use AJAX for this. Ajax is asynchronous javascript and XML, but you can output plain text with it as well. Very good explanations can be found at W3Schools.com.
-
I'm trying to make an onclick script that changes the action of a link and form if OK is clicked in the confirm prompt. I'm getting a syntax error in firebug at the return false part.
Basically, if the user clicks OK, I want the script to change the action of the form to the href of the link they clicked and then submit the form. I'm still somewhat new to JS, but here's my code.
<a href="http://localhost/restaurant/admincp/forums/categories/delete/1" onclick="confirm('Are you sure you want to delete the category \'General Discussions\'? This action CANNOT be undone.') ? document.getElementById('actionform').action = this.href; document.getElementById('actionform').submit() : return false;"><img src='http://localhost/restaurant/template/images/delete.png' width='27' height='27' border='0' alt='Delete'></a>
-
I've found a library for using Facebook Connect on CodeIgniter. Found here: http://www.haughin.com/code/facebook/ I'm assuming the same functions from the facebook connect api can be used with the CI library($this->facebook_connect->function), but there is absolutely no documentation on it. What I want to do, is create a custom wall post form. I want to have the users create their message and when submitted, I want to add the message to both their facebook wall, and my database so they appear on my site as well.
The problem with facebook's wall post form is: 1) it's javascript and 2) all it does is post it to facebook. So basically I want to do something like this:
if($this->form_validation->run() == FALSE){ $this->load->view('wallpost_form'); } else { post_to_wall($user, $message); //However the function will work $query = "yadayadayada" }
Any ideas?
-
A couple of buddies and I have started a small company with website projects in mind. We are looking for a web designer that is efficient in web graphic design, HTML, and XHTML/CSS layout design. Since we have literally just launched less than a month ago, we are not expecting a huge amount of income, so for now, this will be an unpaid position. If we grow to the point where our ad revenue exceeds our expenses on server costs, we will pay you a small chunk of those proceeds for your work.
The only requirements we have are that you be efficient in web graphic design, HTML, and XHTML/CSS layout design. We also require you to speak and write in English fluently. It is preferred that you are based in the state of Pennsylvania, but you must atleast be based in the United States.
If you are interested in working with us, please email me at shoelace1291@gmail.com. You must have at least five working examples of your work and at least one reference relative to the position. Any emails mentioning fees will be deleted.
Thanks,
Scott.
-
So I'm making a member group permissions form. I have a selection box to set the group's permission level and if "banned" is selected, I want it to hide the permissions checkbox table(contained in a div called "permissions"). In firebug error console, I get a weird error that says "setting a property that has only a getter", which has completely baffled me. This is the short and sweet code:
<option value='4' onclick="document.getElementById('permissions').style = 'display: none;'">Banned</option>
-
Ok, so I want to make a comment spam filter for my site. I know the basic logic, but have yet to figure out how to write the functions. I have a database table called comments that has a column called "time", which contains a unix timestamp value of when it was posted. Basically what I want to do is this:
When a user tries to post a comment, the script determines with a database query if they have posted a comment in the last 120 seconds.
So basically I have to find the current time, and the time it was 120 seconds before the current time. Then I have to find any comments posted by the user that were made after the 120 second mark.
My database query should then look something like this, right?
$query = mysql_query("SELECT * FROM comments WHERE author = ".$_SESSION['id']." AND time > '".$120secondsago"'");
Please correct me if my logic is wrong, which it very well may be, but how would I find the unix timestamp code from 120 seconds before the current time? Note that 120 seconds is just an example and also that I have not worked with dates in mysql very often.
$query = mysql_query("SELECT * FROM comments WHERE author = ".$_SESSION['id']." AND time
-
I've run into one problem. I have four areas to my site: public, admincp, ownercp, and forum. i want 'public' to be my default site. My htaccess kinda causes the problem because i can only view the other areas with .php and i don't want that.
-
So I've been reading up on the user guide for info on managing applications. I basically want to have three different applications under one domain name. The only problem I encountered(which is a big one), is that you can only view one application at a time. I have three applications, but how would I view each of them, one at a time, by defining the application in my url? for example, if i wanted to view the "admin" application, the url would be http://localhost/admin
-
I've always wanted to be a teacher, but all the traditional school subjects seem to bore me to the point where I don't want anything to do with them. I figured I love web programming and thought it would be cool to become a web programming college professor. You know, teach about PHP, ASP, Databases, etc. Anyone know of any good schools that would have a degree that would prepare you for that type of thing? Preferably in the north east?
-
Ok, so I'm writing a script that deletes a row from a database. It uses a checkbox to determine if that row is to be deleted. What I want to do is make the appropriate area red if the checkbox is selected.
I figured out how to change the color when it's selected, but not how to change it when it's unselected.
This is what I have so far:
<div id='{MEMBER_ID}'> <input type='checkbox' name='delete' id='delete' value='{MEMBER_ID}' onclick="document.getElementById('{MEMBER_ID}').style.background = 'red';"> </div>
So is there an onselect/onunselect event that I could use? I don't want to have to write seperate file for this.
-
I'm using the haversine formula to calculate the distance between two latitude/longitude pairs. I'm getting a syntax error that says the funcion radians is undefined. I got the code off of the Google Store Locator tutorial which was a mysql query based syntax. I realize that the mysql functions are very different from PHP functions, so what would be the equivalent php function to the mysql "radians" function?
-
I have a table called "events" that I want to gather data from. The events that I want are ones that haven't happend yet. There is a column for date that contains the unix timestamp string. How would I select the rows with dates greater than the current date?
-
Is there a way to find out what the array type is?
Example:
$array1 = array('red', 'pizza', '14'); $array2 = array('color' => 'red', 'food' => 'pizza', 'age' => '14'); if(is_recursive($array1)){ return TRUE; } else if(is_multidimensional($array2){ return TRUE; } else { return FALSE; }
-
I'm using the template parser class in codeigniter to display database results in a view file. Is it possible to parse an array like this:
foreach($query->result() as $news){ $articles[$news->id] = array( 'ID' => $news->id, 'TITLE' => $news->title, 'BODY' => $news->body ); } $data = array( 'NEWS_ARTICLES' => array( $artcicles ); $this->parser->parse('index.tpl', $data);
<div id='news'> <h1>Recent News</h1. {NEWS_ARTICLES} <div class='article'> <h1>{TITLE}</h1> <p>{BODY</p> </div> {/NEWS_ARTICLES} </div>
-
I'm starting on a project of building a simple forum for my website. My question is, what would be the best table structure for the database? I've seen a couple different methods. The simplest is a table for categories, forums, threads, and replies. The only problem with that is that it makes it difficult to gather the thread update info for the forum list on the index. You know, like "Latest thread posted by {USER_NAME} in {THREAD_NAME} at {THREAD_TIME}", that whole deal. The second theory, which is what I'm thinking of using, is having tables for categories, forums, and messages(which would have a column for both messageid and threadid). the only problem with that is that i'm not sure of how to distinguish the difference between a regular reply and a new thread. Any ideas?
-
I'm doing this in CodeIgniter, but I'm getting an SQL syntax error. This is my query:
$query = $this->db->query("SELECT restaurants.id,restaurants.name,restaurants.category,restaurants.ratePoints,restaurants.rateTimes,restaurants.smoking,restaurants.alcahol,avg(menu_items.price) as average_price FROM RESTAURANTS WHERE id = '".$id."' JOIN menu_items.price ON restaurants.id = menu_items.restaurant LIMIT 1");
-
I have a list of news articles that I want to be able to parse with database queries. I read the guide on the template parser class and the variable pairs example that they used is pretty much what I want to do. The only thing is that I really can't figure out how to make it database powered.
$query = $this->db->query("SELECT * FROM news ORDER BY id DESC LIMIT 10"); foreach($query->result() as $news){ $this->news->article($news->id); $data = array( 'ID' => $news->id, 'TITLE' => $this->news->title, 'BODY' => $this->news->body ); } $this->parser->parse('index_body.tpl', $data);
The reason I want to do this is because it makes templating easier and it also makes it easier to execute more complicated scripts in the model without having to do it in the controller.
-
I have to disagree with sunwukung. All of the advantages you listed are also advantages that you have with plain old php. Except for points d and f(f not so much because there are alot of php communities out there, including this one), frameworks and barebone php share all of these advantages.
One huge disadvantage is that if you ever want to go back to plain php, say to help with a project that doesn't involve code igniter, it will take some time to get used to not working with controllers, models, views, libraries, plugins, etc.
However, I do agree with the disadvantages that he listed. As I stated above, it is a learning curve as he had put it. Even though it may get simple database and even file oriented applications finished quickly, there are restrictions to it that may keep you from using your full programming potential.
Summary: If you know CodeIgniter completely, inside and out, yes, it is a good thing to learn because it will help turn multi-month projects into a project that will only last a few weeks, depending on how much free time you have
String to URI Format
in PHP Coding Help
Posted
I've read up on the urlendcode functions in the PHP user manual, but it's all very confusing. How would I convert a simple string that was creeated by a text input to a format such as this:
site.com/articles/Some-URI